Hal Drivers for L4
Dependents: BSP OneHopeOnePrayer FINAL_AUDIO_RECORD AudioDemo
Fork of STM32L4xx_HAL_Driver by
stm32l4xx_ll_i2c.h
00001 /** 00002 ****************************************************************************** 00003 * @file stm32l4xx_ll_i2c.h 00004 * @author MCD Application Team 00005 * @version V1.1.0 00006 * @date 16-September-2015 00007 * @brief Header file of I2C LL module. 00008 ****************************************************************************** 00009 * @attention 00010 * 00011 * <h2><center>© COPYRIGHT(c) 2015 STMicroelectronics</center></h2> 00012 * 00013 * Redistribution and use in source and binary forms, with or without modification, 00014 * are permitted provided that the following conditions are met: 00015 * 1. Redistributions of source code must retain the above copyright notice, 00016 * this list of conditions and the following disclaimer. 00017 * 2. Redistributions in binary form must reproduce the above copyright notice, 00018 * this list of conditions and the following disclaimer in the documentation 00019 * and/or other materials provided with the distribution. 00020 * 3. Neither the name of STMicroelectronics nor the names of its contributors 00021 * may be used to endorse or promote products derived from this software 00022 * without specific prior written permission. 00023 * 00024 * THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" 00025 * AND ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE 00026 * IMPLIED WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE 00027 * DISCLAIMED. IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE 00028 * FOR ANY DIRECT, INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL 00029 * DAMAGES (INCLUDING, BUT NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR 00030 * SERVICES; LOSS OF USE, DATA, OR PROFITS; OR BUSINESS INTERRUPTION) HOWEVER 00031 * CAUSED AND ON ANY THEORY OF LIABILITY, WHETHER IN CONTRACT, STRICT LIABILITY, 00032 * OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) ARISING IN ANY WAY OUT OF THE USE 00033 * OF THIS SOFTWARE, EVEN IF ADVISED OF THE POSSIBILITY OF SUCH DAMAGE. 00034 * 00035 ****************************************************************************** 00036 */ 00037 00038 /* Define to prevent recursive inclusion -------------------------------------*/ 00039 #ifndef __STM32L4xx_LL_I2C_H 00040 #define __STM32L4xx_LL_I2C_H 00041 00042 #ifdef __cplusplus 00043 extern "C" { 00044 #endif 00045 00046 /* Includes ------------------------------------------------------------------*/ 00047 #include "stm32l4xx.h" 00048 00049 /** @addtogroup STM32L4xx_LL_Driver 00050 * @{ 00051 */ 00052 00053 #if defined (I2C1) || defined (I2C2) || defined (I2C3) 00054 00055 /** @defgroup I2C_LL I2C 00056 * @{ 00057 */ 00058 00059 /* Private types -------------------------------------------------------------*/ 00060 /* Private variables ---------------------------------------------------------*/ 00061 00062 /* Private constants ---------------------------------------------------------*/ 00063 00064 /* Private macros ------------------------------------------------------------*/ 00065 00066 /* Exported types ------------------------------------------------------------*/ 00067 /* Exported constants --------------------------------------------------------*/ 00068 /** @defgroup I2C_LL_Exported_Constants I2C Exported Constants 00069 * @{ 00070 */ 00071 00072 /** @defgroup I2C_LL_EC_CLEAR_FLAG Clear Flags Defines 00073 * @brief Flags defines which can be used with LL_I2C_WriteReg function 00074 * @{ 00075 */ 00076 #define LL_I2C_ICR_ADDRCF I2C_ICR_ADDRCF 00077 #define LL_I2C_ICR_NACKCF I2C_ICR_NACKCF 00078 #define LL_I2C_ICR_STOPCF I2C_ICR_STOPCF 00079 #define LL_I2C_ICR_BERRCF I2C_ICR_BERRCF 00080 #define LL_I2C_ICR_ARLOCF I2C_ICR_ARLOCF 00081 #define LL_I2C_ICR_OVRCF I2C_ICR_OVRCF 00082 /** 00083 * @} 00084 */ 00085 00086 /** @defgroup I2C_LL_EC_GET_FLAG Get Flags Defines 00087 * @brief Flags defines which can be used with LL_I2C_ReadReg function 00088 * @{ 00089 */ 00090 #define LL_I2C_ISR_TXE I2C_ISR_TXE 00091 #define LL_I2C_ISR_TXIS I2C_ISR_TXIS 00092 #define LL_I2C_ISR_RXNE I2C_ISR_RXNE 00093 #define LL_I2C_ISR_ADDR I2C_ISR_ADDR 00094 #define LL_I2C_ISR_NACKF I2C_ISR_NACKF 00095 #define LL_I2C_ISR_STOPF I2C_ISR_STOPF 00096 #define LL_I2C_ISR_TC I2C_ISR_TC 00097 #define LL_I2C_ISR_TCR I2C_ISR_TCR 00098 #define LL_I2C_ISR_BERR I2C_ISR_BERR 00099 #define LL_I2C_ISR_ARLO I2C_ISR_ARLO 00100 #define LL_I2C_ISR_OVR I2C_ISR_OVR 00101 #define LL_I2C_ISR_BUSY I2C_ISR_BUSY 00102 /** 00103 * @} 00104 */ 00105 00106 /** @defgroup I2C_LL_EC_IT IT Defines 00107 * @brief IT defines which can be used with LL_I2C_ReadReg and LL_I2C_WriteReg functions 00108 * @{ 00109 */ 00110 #define LL_I2C_CR1_TXIE I2C_CR1_TXIE 00111 #define LL_I2C_CR1_RXIE I2C_CR1_RXIE 00112 #define LL_I2C_CR1_ADDRIE I2C_CR1_ADDRIE 00113 #define LL_I2C_CR1_NACKIE I2C_CR1_NACKIE 00114 #define LL_I2C_CR1_STOPIE I2C_CR1_STOPIE 00115 #define LL_I2C_CR1_TCIE I2C_CR1_TCIE 00116 #define LL_I2C_CR1_ERRIE I2C_CR1_ERRIE 00117 /** 00118 * @} 00119 */ 00120 00121 /** @defgroup I2C_LL_EC_ADDRESSING_MODE ADDRESSING MODE 00122 * @{ 00123 */ 00124 #define LL_I2C_ADDRESSING_MODE_7BIT ((uint32_t) 0x00000000) /*!<Master operates in 7-bit addressing mode. */ 00125 #define LL_I2C_ADDRESSING_MODE_10BIT I2C_CR2_ADD10 /*!<Master operates in 10-bit addressing mode.*/ 00126 /** 00127 * @} 00128 */ 00129 00130 /** @defgroup I2C_LL_EC_OWNADDRESS1 OWNADDRESS1 00131 * @{ 00132 */ 00133 #define LL_I2C_OWNADDRESS1_7BIT ((uint32_t)0x00000000) /*!<Own address 1 is a 7-bit address. */ 00134 #define LL_I2C_OWNADDRESS1_10BIT I2C_OAR1_OA1MODE /*!<Own address 1 is a 10-bit address.*/ 00135 /** 00136 * @} 00137 */ 00138 00139 /** @defgroup I2C_LL_EC_OWNADDRESS2 OWNADDRESS2 00140 * @{ 00141 */ 00142 #define LL_I2C_OWNADDRESS2_NOMASK I2C_OAR2_OA2NOMASK /*!<Own Address2 No mask. */ 00143 #define LL_I2C_OWNADDRESS2_MASK01 I2C_OAR2_OA2MASK01 /*!<Only Address2 bits[7:2] are compared. */ 00144 #define LL_I2C_OWNADDRESS2_MASK02 I2C_OAR2_OA2MASK02 /*!<Only Address2 bits[7:3] are compared. */ 00145 #define LL_I2C_OWNADDRESS2_MASK03 I2C_OAR2_OA2MASK03 /*!<Only Address2 bits[7:4] are compared. */ 00146 #define LL_I2C_OWNADDRESS2_MASK04 I2C_OAR2_OA2MASK04 /*!<Only Address2 bits[7:5] are compared. */ 00147 #define LL_I2C_OWNADDRESS2_MASK05 I2C_OAR2_OA2MASK05 /*!<Only Address2 bits[7:6] are compared. */ 00148 #define LL_I2C_OWNADDRESS2_MASK06 I2C_OAR2_OA2MASK06 /*!<Only Address2 bits[7] are compared. */ 00149 #define LL_I2C_OWNADDRESS2_MASK07 I2C_OAR2_OA2MASK07 /*!<No comparison is done. All Address2 are acknowledged.*/ 00150 /** 00151 * @} 00152 */ 00153 00154 /** @defgroup I2C_LL_EC_I2C_ACKNOWLEDGE ACKNOWLEDGE 00155 * @{ 00156 */ 00157 #define LL_I2C_ACK ((uint32_t) 0x00000000) /*!<ACK is sent after current received byte. */ 00158 #define LL_I2C_NACK I2C_CR2_NACK /*!<NACK is sent after current received byte.*/ 00159 /** 00160 * @} 00161 */ 00162 00163 /** @defgroup I2C_LL_EC_ADDRSLAVE ADDRSLAVE 00164 * @{ 00165 */ 00166 #define LL_I2C_ADDRSLAVE_7BIT ((uint32_t)0x00000000) /*!<Slave Address in 7-bit. */ 00167 #define LL_I2C_ADDRSLAVE_10BIT I2C_CR2_ADD10 /*!<Slave Address in 10-bit.*/ 00168 /** 00169 * @} 00170 */ 00171 00172 /** @defgroup I2C_LL_EC_MODE MODE 00173 * @{ 00174 */ 00175 #define LL_I2C_MODE_RELOAD I2C_CR2_RELOAD /*!<Enable Reload mode. */ 00176 #define LL_I2C_MODE_AUTOEND I2C_CR2_AUTOEND /*!<Enable Automatic end mode.*/ 00177 #define LL_I2C_MODE_SOFTEND ((uint32_t)0x00000000) /*!<Enable Software end mode. */ 00178 /** 00179 * @} 00180 */ 00181 00182 /** @defgroup I2C_LL_EC_GENERATE GENERATE 00183 * @{ 00184 */ 00185 #define LL_I2C_GENERATE_NOSTARTSTOP ((uint32_t)0x00000000) /*!<Don't Generate Stop and Start condition. */ 00186 #define LL_I2C_GENERATE_STOP I2C_CR2_STOP /*!<Generate Stop condition (Size should be set to 0). */ 00187 #define LL_I2C_GENERATE_START_READ (uint32_t)(I2C_CR2_START | I2C_CR2_RD_WRN) /*!<Generate Start for read request. */ 00188 #define LL_I2C_GENERATE_START_WRITE I2C_CR2_START /*!<Generate Start for write request. */ 00189 #define LL_I2C_GENERATE_RESTART_7BIT_READ (uint32_t)(I2C_CR2_START | I2C_CR2_RD_WRN) /*!<Generate Restart for read request, slave 7Bit address. */ 00190 #define LL_I2C_GENERATE_RESTART_7BIT_WRITE I2C_CR2_START /*!<Generate Restart for write request, slave 7Bit address. */ 00191 #define LL_I2C_GENERATE_RESTART_10BIT_READ (uint32_t)(I2C_CR2_START | I2C_CR2_RD_WRN | I2C_CR2_HEAD10R) /*!<Generate Restart for read request, slave 10Bit address. */ 00192 #define LL_I2C_GENERATE_RESTART_10BIT_WRITE I2C_CR2_START /*!<Generate Restart for write request, slave 10Bit address.*/ 00193 /** 00194 * @} 00195 */ 00196 00197 /** @defgroup I2C_LL_EC_DIRECTION DIRECTION 00198 * @{ 00199 */ 00200 #define LL_I2C_DIRECTION_WRITE ((uint32_t)0x00000000) /*!<Write transfer, slave enters receiver mode. */ 00201 #define LL_I2C_DIRECTION_READ I2C_ISR_DIR /*!<Read transfer, slave enters transmitter mode.*/ 00202 /** 00203 * @} 00204 */ 00205 00206 /** @defgroup I2C_LL_EC_DMA_REG_DATA DMA register data 00207 * @{ 00208 */ 00209 #define LL_I2C_DMA_REG_DATA_TRANSMIT (uint32_t)0 /*!<Get address of data register used for transmission */ 00210 #define LL_I2C_DMA_REG_DATA_RECEIVE (uint32_t)1 /*!<Get address of data register used for reception */ 00211 /** 00212 * @} 00213 */ 00214 00215 /** 00216 * @} 00217 */ 00218 00219 /* Exported macro ------------------------------------------------------------*/ 00220 /** @defgroup I2C_LL_Exported_Macros I2C Exported Macros 00221 * @{ 00222 */ 00223 00224 /** @defgroup I2C_LL_EM_WRITE_READ Common Write and read registers Macros 00225 * @{ 00226 */ 00227 00228 /** 00229 * @brief Write a value in I2C register 00230 * @param __INSTANCE__ I2C Instance 00231 * @param __REG__ Register to be written 00232 * @param __VALUE__ Value to be written in the register 00233 * @retval None 00234 */ 00235 #define LL_I2C_WriteReg(__INSTANCE__, __REG__, __VALUE__) WRITE_REG(__INSTANCE__->__REG__, (__VALUE__)) 00236 00237 /** 00238 * @brief Read a value in I2C register 00239 * @param __INSTANCE__ I2C Instance 00240 * @param __REG__ Register to be read 00241 * @retval Register value 00242 */ 00243 #define LL_I2C_ReadReg(__INSTANCE__, __REG__) READ_REG(__INSTANCE__->__REG__) 00244 /** 00245 * @} 00246 */ 00247 00248 /** @defgroup I2C_LL_EM_CONVERT_TIMINGS Convert SDA SCL timings 00249 * @{ 00250 */ 00251 /** 00252 * @brief Configure the SDA setup, hold time and the SCL high, low period. 00253 * @param __PRESCALER__ This parameter must be a value between 0 and 0xF. 00254 * @param __DATA_SETUP_TIME__ This parameter must be a value between 0 and 0xF. (tscldel = (SCLDEL+1)xtpresc) 00255 * @param __DATA_HOLD_TIME__ This parameter must be a value between 0 and 0xF. (tsdadel = SDADELxtpresc) 00256 * @param __CLOCK_HIGH_PERIOD__ This parameter must be a value between 0 and 0xFF. (tsclh = (SCLH+1)xtpresc) 00257 * @param __CLOCK_LOW_PERIOD__ This parameter must be a value between 0 and 0xFF. (tscll = (SCLL+1)xtpresc) 00258 * @retval Value between 0 and 0xFFFFFFFF 00259 */ 00260 #define __LL_I2C_CONVERT_TIMINGS(__PRESCALER__, __DATA_SETUP_TIME__, __DATA_HOLD_TIME__, __CLOCK_HIGH_PERIOD__, __CLOCK_LOW_PERIOD__) \ 00261 ((((uint32_t)(__PRESCALER__) << (uint32_t)POSITION_VAL(I2C_TIMINGR_PRESC)) & I2C_TIMINGR_PRESC) | \ 00262 (((uint32_t)(__DATA_SETUP_TIME__) << (uint32_t)POSITION_VAL(I2C_TIMINGR_SCLDEL)) & I2C_TIMINGR_SCLDEL) | \ 00263 (((uint32_t)(__DATA_HOLD_TIME__) << (uint32_t)POSITION_VAL(I2C_TIMINGR_SDADEL)) & I2C_TIMINGR_SDADEL) | \ 00264 (((uint32_t)(__CLOCK_HIGH_PERIOD__) << (uint32_t)POSITION_VAL(I2C_TIMINGR_SCLH)) & I2C_TIMINGR_SCLH) | \ 00265 (((uint32_t)(__CLOCK_LOW_PERIOD__) << (uint32_t)POSITION_VAL(I2C_TIMINGR_SCLL)) & I2C_TIMINGR_SCLL)) 00266 /** 00267 * @} 00268 */ 00269 00270 /** 00271 * @} 00272 */ 00273 00274 /* Exported functions --------------------------------------------------------*/ 00275 /** @defgroup I2C_LL_Exported_Functions I2C Exported Functions 00276 * @{ 00277 */ 00278 00279 /** @defgroup I2C_LL_EF_Configuration Configuration 00280 * @{ 00281 */ 00282 00283 /** 00284 * @brief Enable I2C peripheral (PE = 1). 00285 * @rmtoll CR1 PE LL_I2C_Enable 00286 * @param I2Cx I2C Instance. 00287 * @retval None 00288 */ 00289 __STATIC_INLINE void LL_I2C_Enable(I2C_TypeDef* I2Cx) 00290 { 00291 SET_BIT(I2Cx->CR1, I2C_CR1_PE); 00292 } 00293 00294 /** 00295 * @brief Disable I2C peripheral (PE = 0). 00296 * @note When PE = 0, the I2C SCL and SDA lines are released. 00297 * Internal state machines and status bits are put back to their reset value. 00298 * When cleared, PE must be kept low for at least 3 APB clock cycles. 00299 * @rmtoll CR1 PE LL_I2C_Disable 00300 * @param I2Cx I2C Instance. 00301 * @retval None 00302 */ 00303 __STATIC_INLINE void LL_I2C_Disable(I2C_TypeDef* I2Cx) 00304 { 00305 CLEAR_BIT(I2Cx->CR1, I2C_CR1_PE); 00306 } 00307 00308 /** 00309 * @brief Check if the I2C peripheral is enabled or disabled. 00310 * @rmtoll CR1 PE LL_I2C_IsEnabled 00311 * @param I2Cx I2C Instance. 00312 * @retval State of bit (1 or 0). 00313 */ 00314 __STATIC_INLINE uint32_t LL_I2C_IsEnabled(I2C_TypeDef* I2Cx) 00315 { 00316 return (READ_BIT(I2Cx->CR1, I2C_CR1_PE) == (I2C_CR1_PE)); 00317 } 00318 00319 /** 00320 * @brief Configure Digital Noise Filter. 00321 * @note If the analog filter is also enabled, the digital filter is added to analog filter. 00322 * This filter can only be programmed when the I2C is disabled (PE = 0). 00323 * @rmtoll CR1 DNF LL_I2C_SetDigitalFilter 00324 * @param I2Cx I2C Instance. 00325 * @param DigitalFilter This parameter must be a value between 0x00 (Digital filter disabled) and 0x0F (Digital filter enabled and filtering capability up to 15*ti2cclk). This parameter is used to configure the digital noise filter on SDA and SCL input. The digital filter will filter spikes with a length of up to DNF[3:0]*ti2cclk. 00326 * @retval None 00327 */ 00328 __STATIC_INLINE void LL_I2C_SetDigitalFilter(I2C_TypeDef* I2Cx, uint32_t DigitalFilter) 00329 { 00330 MODIFY_REG(I2Cx->CR1, I2C_CR1_DNF, DigitalFilter << POSITION_VAL(I2C_CR1_DNF)); 00331 } 00332 00333 /** 00334 * @brief Get the current Digital Noise Filter configuration. 00335 * @rmtoll CR1 DNF LL_I2C_GetDigitalFilter 00336 * @param I2Cx I2C Instance. 00337 * @retval 0..0xF 00338 */ 00339 __STATIC_INLINE uint32_t LL_I2C_GetDigitalFilter(I2C_TypeDef* I2Cx) 00340 { 00341 return (uint32_t)(READ_BIT(I2Cx->CR1, I2C_CR1_DNF) >> POSITION_VAL(I2C_CR1_DNF)); 00342 } 00343 00344 /** 00345 * @brief Enable Analog Noise Filter. 00346 * @note This filter can only be programmed when the I2C is disabled (PE = 0). 00347 * @rmtoll CR1 ANFOFF LL_I2C_EnableAnalogFilter 00348 * @param I2Cx I2C Instance. 00349 * @retval None 00350 */ 00351 __STATIC_INLINE void LL_I2C_EnableAnalogFilter(I2C_TypeDef* I2Cx) 00352 { 00353 CLEAR_BIT(I2Cx->CR1, I2C_CR1_ANFOFF); 00354 } 00355 00356 /** 00357 * @brief Disable Analog Noise Filter. 00358 * @note This filter can only be programmed when the I2C is disabled (PE = 0). 00359 * @rmtoll CR1 ANFOFF LL_I2C_DisableAnalogFilter 00360 * @param I2Cx I2C Instance. 00361 * @retval None 00362 */ 00363 __STATIC_INLINE void LL_I2C_DisableAnalogFilter(I2C_TypeDef* I2Cx) 00364 { 00365 SET_BIT(I2Cx->CR1, I2C_CR1_ANFOFF); 00366 } 00367 00368 /** 00369 * @brief Check if Analog Noise Filter is enabled or disabled. 00370 * @rmtoll CR1 ANFOFF LL_I2C_IsEnabledAnalogFilter 00371 * @param I2Cx I2C Instance. 00372 * @retval State of bit (1 or 0). 00373 */ 00374 __STATIC_INLINE uint32_t LL_I2C_IsEnabledAnalogFilter(I2C_TypeDef* I2Cx) 00375 { 00376 return (READ_BIT(I2Cx->CR1, I2C_CR1_ANFOFF) != (I2C_CR1_ANFOFF)); 00377 } 00378 00379 /** 00380 * @brief Enable DMA transmission requests. 00381 * @rmtoll CR1 TXDMAEN LL_I2C_EnableDMAReq_TX 00382 * @param I2Cx I2C Instance. 00383 * @retval None 00384 */ 00385 __STATIC_INLINE void LL_I2C_EnableDMAReq_TX(I2C_TypeDef* I2Cx) 00386 { 00387 SET_BIT(I2Cx->CR1, I2C_CR1_TXDMAEN); 00388 } 00389 00390 /** 00391 * @brief Disable DMA transmission requests. 00392 * @rmtoll CR1 TXDMAEN LL_I2C_DisableDMAReq_TX 00393 * @param I2Cx I2C Instance. 00394 * @retval None 00395 */ 00396 __STATIC_INLINE void LL_I2C_DisableDMAReq_TX(I2C_TypeDef* I2Cx) 00397 { 00398 CLEAR_BIT(I2Cx->CR1, I2C_CR1_TXDMAEN); 00399 } 00400 00401 /** 00402 * @brief Check if DMA transmission requests are enabled or disabled. 00403 * @rmtoll CR1 TXDMAEN LL_I2C_IsEnabledDMAReq_TX 00404 * @param I2Cx I2C Instance. 00405 * @retval State of bit (1 or 0). 00406 */ 00407 __STATIC_INLINE uint32_t LL_I2C_IsEnabledDMAReq_TX(I2C_TypeDef* I2Cx) 00408 { 00409 return (READ_BIT(I2Cx->CR1, I2C_CR1_TXDMAEN) == (I2C_CR1_TXDMAEN)); 00410 } 00411 00412 /** 00413 * @brief Enable DMA reception requests. 00414 * @rmtoll CR1 RXDMAEN LL_I2C_EnableDMAReq_RX 00415 * @param I2Cx I2C Instance. 00416 * @retval None 00417 */ 00418 __STATIC_INLINE void LL_I2C_EnableDMAReq_RX(I2C_TypeDef* I2Cx) 00419 { 00420 SET_BIT(I2Cx->CR1, I2C_CR1_RXDMAEN); 00421 } 00422 00423 /** 00424 * @brief Disable DMA reception requests. 00425 * @rmtoll CR1 RXDMAEN LL_I2C_DisableDMAReq_RX 00426 * @param I2Cx I2C Instance. 00427 * @retval None 00428 */ 00429 __STATIC_INLINE void LL_I2C_DisableDMAReq_RX(I2C_TypeDef* I2Cx) 00430 { 00431 CLEAR_BIT(I2Cx->CR1, I2C_CR1_RXDMAEN); 00432 } 00433 00434 /** 00435 * @brief Check if DMA reception requests are enabled or disabled. 00436 * @rmtoll CR1 RXDMAEN LL_I2C_IsEnabledDMAReq_RX 00437 * @param I2Cx I2C Instance. 00438 * @retval State of bit (1 or 0). 00439 */ 00440 __STATIC_INLINE uint32_t LL_I2C_IsEnabledDMAReq_RX(I2C_TypeDef* I2Cx) 00441 { 00442 return (READ_BIT(I2Cx->CR1, I2C_CR1_RXDMAEN) == (I2C_CR1_RXDMAEN)); 00443 } 00444 00445 /** 00446 * @brief Get the data register address used for DMA transfer 00447 * @rmtoll TXDR TXDATA LL_I2C_DMA_GetRegAddr\n 00448 * RXDR RXDATA LL_I2C_DMA_GetRegAddr 00449 * @param I2Cx I2C Instance 00450 * @param Direction This parameter can be one of the following values: 00451 * @arg @ref LL_I2C_DMA_REG_DATA_TRANSMIT 00452 * @arg @ref LL_I2C_DMA_REG_DATA_RECEIVE 00453 * @retval Address of data register 00454 */ 00455 __STATIC_INLINE uint32_t LL_I2C_DMA_GetRegAddr(I2C_TypeDef * I2Cx, uint32_t Direction) 00456 { 00457 register uint32_t data_reg_addr = 0; 00458 00459 if (Direction == LL_I2C_DMA_REG_DATA_TRANSMIT) 00460 { 00461 /* return address of TXDR register */ 00462 data_reg_addr = (uint32_t)&(I2Cx->TXDR); 00463 } 00464 else 00465 { 00466 /* return address of RXDR register */ 00467 data_reg_addr = (uint32_t)&(I2Cx->RXDR); 00468 } 00469 00470 return data_reg_addr; 00471 } 00472 00473 /** 00474 * @brief Enable Clock stretching. 00475 * @note This bit can only be programmed when the I2C is disabled (PE = 0). 00476 * @rmtoll CR1 NOSTRETCH LL_I2C_EnableClockStretching 00477 * @param I2Cx I2C Instance. 00478 * @retval None 00479 */ 00480 __STATIC_INLINE void LL_I2C_EnableClockStretching(I2C_TypeDef* I2Cx) 00481 { 00482 CLEAR_BIT(I2Cx->CR1, I2C_CR1_NOSTRETCH); 00483 } 00484 00485 /** 00486 * @brief Disable Clock stretching. 00487 * @note This bit can only be programmed when the I2C is disabled (PE = 0). 00488 * @rmtoll CR1 NOSTRETCH LL_I2C_DisableClockStretching 00489 * @param I2Cx I2C Instance. 00490 * @retval None 00491 */ 00492 __STATIC_INLINE void LL_I2C_DisableClockStretching(I2C_TypeDef* I2Cx) 00493 { 00494 SET_BIT(I2Cx->CR1, I2C_CR1_NOSTRETCH); 00495 } 00496 00497 /** 00498 * @brief Check if Clock stretching is enabled or disabled. 00499 * @rmtoll CR1 NOSTRETCH LL_I2C_IsEnabledClockStretching 00500 * @param I2Cx I2C Instance. 00501 * @retval State of bit (1 or 0). 00502 */ 00503 __STATIC_INLINE uint32_t LL_I2C_IsEnabledClockStretching(I2C_TypeDef* I2Cx) 00504 { 00505 return (READ_BIT(I2Cx->CR1, I2C_CR1_NOSTRETCH) != (I2C_CR1_NOSTRETCH)); 00506 } 00507 00508 /** 00509 * @brief Enable Wakeup from STOP. 00510 * @note This bit can only be programmed when Digital Filter is disabled. 00511 * @rmtoll CR1 WUPEN LL_I2C_EnableWakeUpFromStop 00512 * @param I2Cx I2C Instance. 00513 * @retval None 00514 */ 00515 __STATIC_INLINE void LL_I2C_EnableWakeUpFromStop(I2C_TypeDef* I2Cx) 00516 { 00517 SET_BIT(I2Cx->CR1, I2C_CR1_WUPEN); 00518 } 00519 00520 /** 00521 * @brief Disable Wakeup from STOP. 00522 * @rmtoll CR1 WUPEN LL_I2C_DisableWakeUpFromStop 00523 * @param I2Cx I2C Instance. 00524 * @retval None 00525 */ 00526 __STATIC_INLINE void LL_I2C_DisableWakeUpFromStop(I2C_TypeDef* I2Cx) 00527 { 00528 CLEAR_BIT(I2Cx->CR1, I2C_CR1_WUPEN); 00529 } 00530 00531 /** 00532 * @brief Check if Wakeup from STOP is enabled or disabled. 00533 * @rmtoll CR1 WUPEN LL_I2C_IsEnabledWakeUpFromStop 00534 * @param I2Cx I2C Instance. 00535 * @retval State of bit (1 or 0). 00536 */ 00537 __STATIC_INLINE uint32_t LL_I2C_IsEnabledWakeUpFromStop(I2C_TypeDef* I2Cx) 00538 { 00539 return (READ_BIT(I2Cx->CR1, I2C_CR1_WUPEN) == (I2C_CR1_WUPEN)); 00540 } 00541 00542 /** 00543 * @brief Enable General Call. 00544 * @note When enabled the Address 0x00 is ACKed. 00545 * @rmtoll CR1 GCEN LL_I2C_EnableGeneralCall 00546 * @param I2Cx I2C Instance. 00547 * @retval None 00548 */ 00549 __STATIC_INLINE void LL_I2C_EnableGeneralCall(I2C_TypeDef* I2Cx) 00550 { 00551 SET_BIT(I2Cx->CR1, I2C_CR1_GCEN); 00552 } 00553 00554 /** 00555 * @brief Disable General Call. 00556 * @note When disabled the Address 0x00 is NACKed. 00557 * @rmtoll CR1 GCEN LL_I2C_DisableGeneralCall 00558 * @param I2Cx I2C Instance. 00559 * @retval None 00560 */ 00561 __STATIC_INLINE void LL_I2C_DisableGeneralCall(I2C_TypeDef* I2Cx) 00562 { 00563 CLEAR_BIT(I2Cx->CR1, I2C_CR1_GCEN); 00564 } 00565 00566 /** 00567 * @brief Check if General Call is enabled or disabled. 00568 * @rmtoll CR1 GCEN LL_I2C_IsEnabledGeneralCall 00569 * @param I2Cx I2C Instance. 00570 * @retval State of bit (1 or 0). 00571 */ 00572 __STATIC_INLINE uint32_t LL_I2C_IsEnabledGeneralCall(I2C_TypeDef* I2Cx) 00573 { 00574 return (READ_BIT(I2Cx->CR1, I2C_CR1_GCEN) == (I2C_CR1_GCEN)); 00575 } 00576 00577 /** 00578 * @brief Configure the Master to operate in 7-bit or 10-bit addressing mode. 00579 * @note Changing this bit is not allowed, when the START bit is set. 00580 * @rmtoll CR2 ADD10 LL_I2C_SetMasterAddressingMode 00581 * @param I2Cx I2C Instance. 00582 * @param AddressingMode This parameter can be one of the following values: 00583 * @arg @ref LL_I2C_ADDRESSING_MODE_7BIT 00584 * @arg @ref LL_I2C_ADDRESSING_MODE_10BIT 00585 * @retval None 00586 */ 00587 __STATIC_INLINE void LL_I2C_SetMasterAddressingMode(I2C_TypeDef* I2Cx, uint32_t AddressingMode) 00588 { 00589 MODIFY_REG(I2Cx->CR2, I2C_CR2_ADD10, AddressingMode); 00590 } 00591 00592 /** 00593 * @brief Get the Master addressing mode. 00594 * @rmtoll CR2 ADD10 LL_I2C_GetMasterAddressingMode 00595 * @param I2Cx I2C Instance. 00596 * @retval Returned value can be one of the following values: 00597 * @arg @ref LL_I2C_ADDRESSING_MODE_7BIT 00598 * @arg @ref LL_I2C_ADDRESSING_MODE_10BIT 00599 */ 00600 __STATIC_INLINE uint32_t LL_I2C_GetMasterAddressingMode(I2C_TypeDef* I2Cx) 00601 { 00602 return (uint32_t)(READ_BIT(I2Cx->CR2, I2C_CR2_ADD10)); 00603 } 00604 00605 /** 00606 * @brief Set the Own Address1. 00607 * @rmtoll OAR1 OA1 LL_I2C_SetOwnAddress1\n 00608 * OAR1 OA1MODE LL_I2C_SetOwnAddress1 00609 * @param I2Cx I2C Instance. 00610 * @param OwnAddr This parameter must be a value between 0 and 0x7F. 00611 * @param OwnAddrSize This parameter can be one of the following values: 00612 * @arg @ref LL_I2C_OWNADDRESS1_7BIT 00613 * @arg @ref LL_I2C_OWNADDRESS1_10BIT 00614 * @retval None 00615 */ 00616 __STATIC_INLINE void LL_I2C_SetOwnAddress1(I2C_TypeDef* I2Cx, uint32_t OwnAddr, uint32_t OwnAddrSize) 00617 { 00618 MODIFY_REG(I2Cx->OAR1, I2C_OAR1_OA1 | I2C_OAR1_OA1MODE, OwnAddr | OwnAddrSize); 00619 } 00620 00621 /** 00622 * @brief Enable acknowledge on Own Address1 match address. 00623 * @rmtoll OAR1 OA1EN LL_I2C_EnableOwnAddress1 00624 * @param I2Cx I2C Instance. 00625 * @retval None 00626 */ 00627 __STATIC_INLINE void LL_I2C_EnableOwnAddress1(I2C_TypeDef* I2Cx) 00628 { 00629 SET_BIT(I2Cx->OAR1, I2C_OAR1_OA1EN); 00630 } 00631 00632 /** 00633 * @brief Disable acknowledge on Own Address1 match address. 00634 * @rmtoll OAR1 OA1EN LL_I2C_DisableOwnAddress1 00635 * @param I2Cx I2C Instance. 00636 * @retval None 00637 */ 00638 __STATIC_INLINE void LL_I2C_DisableOwnAddress1(I2C_TypeDef* I2Cx) 00639 { 00640 CLEAR_BIT(I2Cx->OAR1, I2C_OAR1_OA1EN); 00641 } 00642 00643 /** 00644 * @brief Check if Own Address1 acknowledge is enabled or disabled. 00645 * @rmtoll OAR1 OA1EN LL_I2C_IsEnabledOwnAddress1 00646 * @param I2Cx I2C Instance. 00647 * @retval State of bit (1 or 0). 00648 */ 00649 __STATIC_INLINE uint32_t LL_I2C_IsEnabledOwnAddress1(I2C_TypeDef* I2Cx) 00650 { 00651 return (READ_BIT(I2Cx->OAR1, I2C_OAR1_OA1EN) == (I2C_OAR1_OA1EN)); 00652 } 00653 00654 /** 00655 * @brief Set the 7bits Own Address2. 00656 * @note This action has no effect if own address2 is enabled. 00657 * @rmtoll OAR2 OA2 LL_I2C_SetOwnAddress2\n 00658 * OAR2 OA2MSK LL_I2C_SetOwnAddress2 00659 * @param I2Cx I2C Instance. 00660 * @param OwnAddr Value between 0 and 0x7F. 00661 * @param OwnAddrMask This parameter can be one of the following values: 00662 * @arg @ref LL_I2C_OWNADDRESS2_NOMASK 00663 * @arg @ref LL_I2C_OWNADDRESS2_MASK01 00664 * @arg @ref LL_I2C_OWNADDRESS2_MASK02 00665 * @arg @ref LL_I2C_OWNADDRESS2_MASK03 00666 * @arg @ref LL_I2C_OWNADDRESS2_MASK04 00667 * @arg @ref LL_I2C_OWNADDRESS2_MASK05 00668 * @arg @ref LL_I2C_OWNADDRESS2_MASK06 00669 * @arg @ref LL_I2C_OWNADDRESS2_MASK07 00670 * @retval None 00671 */ 00672 __STATIC_INLINE void LL_I2C_SetOwnAddress2(I2C_TypeDef* I2Cx, uint32_t OwnAddr, uint32_t OwnAddrMask) 00673 { 00674 MODIFY_REG(I2Cx->OAR2, I2C_OAR2_OA2 | I2C_OAR2_OA2MSK, OwnAddr | OwnAddrMask); 00675 } 00676 00677 /** 00678 * @brief Enable acknowledge on Own Address2 match address. 00679 * @rmtoll OAR2 OA2EN LL_I2C_EnableOwnAddress2 00680 * @param I2Cx I2C Instance. 00681 * @retval None 00682 */ 00683 __STATIC_INLINE void LL_I2C_EnableOwnAddress2(I2C_TypeDef* I2Cx) 00684 { 00685 SET_BIT(I2Cx->OAR2, I2C_OAR2_OA2EN); 00686 } 00687 00688 /** 00689 * @brief Disable acknowledge on Own Address2 match address. 00690 * @rmtoll OAR2 OA2EN LL_I2C_DisableOwnAddress2 00691 * @param I2Cx I2C Instance. 00692 * @retval None 00693 */ 00694 __STATIC_INLINE void LL_I2C_DisableOwnAddress2(I2C_TypeDef* I2Cx) 00695 { 00696 CLEAR_BIT(I2Cx->OAR2, I2C_OAR2_OA2EN); 00697 } 00698 00699 /** 00700 * @brief Check if Own Address1 acknowledge is enabled or disabled. 00701 * @rmtoll OAR2 OA2EN LL_I2C_IsEnabledOwnAddress2 00702 * @param I2Cx I2C Instance. 00703 * @retval State of bit (1 or 0). 00704 */ 00705 __STATIC_INLINE uint32_t LL_I2C_IsEnabledOwnAddress2(I2C_TypeDef* I2Cx) 00706 { 00707 return (READ_BIT(I2Cx->OAR2, I2C_OAR2_OA2EN) == (I2C_OAR2_OA2EN)); 00708 } 00709 00710 /** 00711 * @brief Configure the SDA setup, hold time and the SCL high, low period. 00712 * @note This bit can only be programmed when the I2C is disabled (PE = 0). 00713 * @rmtoll TIMINGR TIMINGR LL_I2C_SetTiming 00714 * @param I2Cx I2C Instance. 00715 * @param TimingValue This parameter must be a value between 0 and 0xFFFFFFFF. 00716 * @note This parameter is computed with the STM32CubeMX Tool. 00717 * @retval None 00718 */ 00719 __STATIC_INLINE void LL_I2C_SetTiming(I2C_TypeDef* I2Cx, uint32_t TimingValue) 00720 { 00721 WRITE_REG(I2Cx->TIMINGR, TimingValue); 00722 } 00723 00724 /** 00725 * @brief Get the Timing Prescaler setting. 00726 * @rmtoll TIMINGR PRESC LL_I2C_GetTimingPrescaler 00727 * @param I2Cx I2C Instance. 00728 * @retval 0..0xF 00729 */ 00730 __STATIC_INLINE uint32_t LL_I2C_GetTimingPrescaler(I2C_TypeDef* I2Cx) 00731 { 00732 return (uint32_t)(READ_BIT(I2Cx->TIMINGR, I2C_TIMINGR_PRESC) >> POSITION_VAL(I2C_TIMINGR_PRESC)); 00733 } 00734 00735 /** 00736 * @brief Get the SCL low period setting. 00737 * @rmtoll TIMINGR SCLL LL_I2C_GetClockLowPeriod 00738 * @param I2Cx I2C Instance. 00739 * @retval 0..0xFF 00740 */ 00741 __STATIC_INLINE uint32_t LL_I2C_GetClockLowPeriod(I2C_TypeDef* I2Cx) 00742 { 00743 return (uint32_t)(READ_BIT(I2Cx->TIMINGR, I2C_TIMINGR_SCLL) >> POSITION_VAL(I2C_TIMINGR_SCLL)); 00744 } 00745 00746 /** 00747 * @brief Get the SCL high period setting. 00748 * @rmtoll TIMINGR SCLH LL_I2C_GetClockHighPeriod 00749 * @param I2Cx I2C Instance. 00750 * @retval 0..0xFF 00751 */ 00752 __STATIC_INLINE uint32_t LL_I2C_GetClockHighPeriod(I2C_TypeDef* I2Cx) 00753 { 00754 return (uint32_t)(READ_BIT(I2Cx->TIMINGR, I2C_TIMINGR_SCLH) >> POSITION_VAL(I2C_TIMINGR_SCLH)); 00755 } 00756 00757 /** 00758 * @brief Get the SDA hold time. 00759 * @rmtoll TIMINGR SDADEL LL_I2C_GetDataHoldTime 00760 * @param I2Cx I2C Instance. 00761 * @retval 0..0xF 00762 */ 00763 __STATIC_INLINE uint32_t LL_I2C_GetDataHoldTime(I2C_TypeDef* I2Cx) 00764 { 00765 return (uint32_t)(READ_BIT(I2Cx->TIMINGR, I2C_TIMINGR_SDADEL) >> POSITION_VAL(I2C_TIMINGR_SDADEL)); 00766 } 00767 00768 /** 00769 * @brief Get the SDA setup time. 00770 * @rmtoll TIMINGR SCLDEL LL_I2C_GetDataSetupTime 00771 * @param I2Cx I2C Instance. 00772 * @retval 0..0xF 00773 */ 00774 __STATIC_INLINE uint32_t LL_I2C_GetDataSetupTime(I2C_TypeDef* I2Cx) 00775 { 00776 return (uint32_t)(READ_BIT(I2Cx->TIMINGR, I2C_TIMINGR_SCLDEL) >> POSITION_VAL(I2C_TIMINGR_SCLDEL)); 00777 } 00778 00779 /** 00780 * @} 00781 */ 00782 00783 /** @defgroup I2C_LL_EF_IT_Management IT_Management 00784 * @{ 00785 */ 00786 00787 /** 00788 * @brief Enable TXIS interrupt. 00789 * @rmtoll CR1 TXIE LL_I2C_EnableIT_TX 00790 * @param I2Cx I2C Instance. 00791 * @retval None 00792 */ 00793 __STATIC_INLINE void LL_I2C_EnableIT_TX(I2C_TypeDef* I2Cx) 00794 { 00795 SET_BIT(I2Cx->CR1, I2C_CR1_TXIE); 00796 } 00797 00798 /** 00799 * @brief Disable TXIS interrupt. 00800 * @rmtoll CR1 TXIE LL_I2C_DisableIT_TX 00801 * @param I2Cx I2C Instance. 00802 * @retval None 00803 */ 00804 __STATIC_INLINE void LL_I2C_DisableIT_TX(I2C_TypeDef* I2Cx) 00805 { 00806 CLEAR_BIT(I2Cx->CR1, I2C_CR1_TXIE); 00807 } 00808 00809 /** 00810 * @brief Check if the TXIS Interrupt is enabled or disabled. 00811 * @rmtoll CR1 TXIE LL_I2C_IsEnabledIT_TX 00812 * @param I2Cx I2C Instance. 00813 * @retval State of bit (1 or 0). 00814 */ 00815 __STATIC_INLINE uint32_t LL_I2C_IsEnabledIT_TX(I2C_TypeDef* I2Cx) 00816 { 00817 return (READ_BIT(I2Cx->CR1, I2C_CR1_TXIE) == (I2C_CR1_TXIE)); 00818 } 00819 00820 /** 00821 * @brief Enable RXNE interrupt. 00822 * @rmtoll CR1 RXIE LL_I2C_EnableIT_RX 00823 * @param I2Cx I2C Instance. 00824 * @retval None 00825 */ 00826 __STATIC_INLINE void LL_I2C_EnableIT_RX(I2C_TypeDef* I2Cx) 00827 { 00828 SET_BIT(I2Cx->CR1, I2C_CR1_RXIE); 00829 } 00830 00831 /** 00832 * @brief Disable RXNE interrupt. 00833 * @rmtoll CR1 RXIE LL_I2C_DisableIT_RX 00834 * @param I2Cx I2C Instance. 00835 * @retval None 00836 */ 00837 __STATIC_INLINE void LL_I2C_DisableIT_RX(I2C_TypeDef* I2Cx) 00838 { 00839 CLEAR_BIT(I2Cx->CR1, I2C_CR1_RXIE); 00840 } 00841 00842 /** 00843 * @brief Check if the RXNE Interrupt is enabled or disabled. 00844 * @rmtoll CR1 RXIE LL_I2C_IsEnabledIT_RX 00845 * @param I2Cx I2C Instance. 00846 * @retval State of bit (1 or 0). 00847 */ 00848 __STATIC_INLINE uint32_t LL_I2C_IsEnabledIT_RX(I2C_TypeDef* I2Cx) 00849 { 00850 return (READ_BIT(I2Cx->CR1, I2C_CR1_RXIE) == (I2C_CR1_RXIE)); 00851 } 00852 00853 /** 00854 * @brief Enable Address match interrupt (slave mode only). 00855 * @rmtoll CR1 ADDRIE LL_I2C_EnableIT_ADDR 00856 * @param I2Cx I2C Instance. 00857 * @retval None 00858 */ 00859 __STATIC_INLINE void LL_I2C_EnableIT_ADDR(I2C_TypeDef* I2Cx) 00860 { 00861 SET_BIT(I2Cx->CR1, I2C_CR1_ADDRIE); 00862 } 00863 00864 /** 00865 * @brief Disable Address match interrupt (slave mode only). 00866 * @rmtoll CR1 ADDRIE LL_I2C_DisableIT_ADDR 00867 * @param I2Cx I2C Instance. 00868 * @retval None 00869 */ 00870 __STATIC_INLINE void LL_I2C_DisableIT_ADDR(I2C_TypeDef* I2Cx) 00871 { 00872 CLEAR_BIT(I2Cx->CR1, I2C_CR1_ADDRIE); 00873 } 00874 00875 /** 00876 * @brief Check if Address match interrupt is enabled or disabled. 00877 * @rmtoll CR1 ADDRIE LL_I2C_IsEnabledIT_ADDR 00878 * @param I2Cx I2C Instance. 00879 * @retval State of bit (1 or 0). 00880 */ 00881 __STATIC_INLINE uint32_t LL_I2C_IsEnabledIT_ADDR(I2C_TypeDef* I2Cx) 00882 { 00883 return (READ_BIT(I2Cx->CR1, I2C_CR1_ADDRIE) == (I2C_CR1_ADDRIE)); 00884 } 00885 00886 /** 00887 * @brief Enable Not acknowledge received interrupt. 00888 * @rmtoll CR1 NACKIE LL_I2C_EnableIT_NACK 00889 * @param I2Cx I2C Instance. 00890 * @retval None 00891 */ 00892 __STATIC_INLINE void LL_I2C_EnableIT_NACK(I2C_TypeDef* I2Cx) 00893 { 00894 SET_BIT(I2Cx->CR1, I2C_CR1_NACKIE); 00895 } 00896 00897 /** 00898 * @brief Disable Not acknowledge received interrupt. 00899 * @rmtoll CR1 NACKIE LL_I2C_DisableIT_NACK 00900 * @param I2Cx I2C Instance. 00901 * @retval None 00902 */ 00903 __STATIC_INLINE void LL_I2C_DisableIT_NACK(I2C_TypeDef* I2Cx) 00904 { 00905 CLEAR_BIT(I2Cx->CR1, I2C_CR1_NACKIE); 00906 } 00907 00908 /** 00909 * @brief Check if Not acknowledge received interrupt is enabled or disabled. 00910 * @rmtoll CR1 NACKIE LL_I2C_IsEnabledIT_NACK 00911 * @param I2Cx I2C Instance. 00912 * @retval State of bit (1 or 0). 00913 */ 00914 __STATIC_INLINE uint32_t LL_I2C_IsEnabledIT_NACK(I2C_TypeDef* I2Cx) 00915 { 00916 return (READ_BIT(I2Cx->CR1, I2C_CR1_NACKIE) == (I2C_CR1_NACKIE)); 00917 } 00918 00919 /** 00920 * @brief Enable STOP detection interrupt. 00921 * @rmtoll CR1 STOPIE LL_I2C_EnableIT_STOP 00922 * @param I2Cx I2C Instance. 00923 * @retval None 00924 */ 00925 __STATIC_INLINE void LL_I2C_EnableIT_STOP(I2C_TypeDef* I2Cx) 00926 { 00927 SET_BIT(I2Cx->CR1, I2C_CR1_STOPIE); 00928 } 00929 00930 /** 00931 * @brief Disable STOP detection interrupt. 00932 * @rmtoll CR1 STOPIE LL_I2C_DisableIT_STOP 00933 * @param I2Cx I2C Instance. 00934 * @retval None 00935 */ 00936 __STATIC_INLINE void LL_I2C_DisableIT_STOP(I2C_TypeDef* I2Cx) 00937 { 00938 CLEAR_BIT(I2Cx->CR1, I2C_CR1_STOPIE); 00939 } 00940 00941 /** 00942 * @brief Check if STOP detection interrupt is enabled or disabled. 00943 * @rmtoll CR1 STOPIE LL_I2C_IsEnabledIT_STOP 00944 * @param I2Cx I2C Instance. 00945 * @retval State of bit (1 or 0). 00946 */ 00947 __STATIC_INLINE uint32_t LL_I2C_IsEnabledIT_STOP(I2C_TypeDef* I2Cx) 00948 { 00949 return (READ_BIT(I2Cx->CR1, I2C_CR1_STOPIE) == (I2C_CR1_STOPIE)); 00950 } 00951 00952 /** 00953 * @brief Enable Transfer Complete interrupt. 00954 * @note Any of these events will generate interrupt : 00955 * Transfer Complete (TC) 00956 * Transfer Complete Reload (TCR) 00957 * @rmtoll CR1 TCIE LL_I2C_EnableIT_TC 00958 * @param I2Cx I2C Instance. 00959 * @retval None 00960 */ 00961 __STATIC_INLINE void LL_I2C_EnableIT_TC(I2C_TypeDef* I2Cx) 00962 { 00963 SET_BIT(I2Cx->CR1, I2C_CR1_TCIE); 00964 } 00965 00966 /** 00967 * @brief Disable Transfer Complete interrupt. 00968 * @note Any of these events will generate interrupt : 00969 * Transfer Complete (TC) 00970 * Transfer Complete Reload (TCR) 00971 * @rmtoll CR1 TCIE LL_I2C_DisableIT_TC 00972 * @param I2Cx I2C Instance. 00973 * @retval None 00974 */ 00975 __STATIC_INLINE void LL_I2C_DisableIT_TC(I2C_TypeDef* I2Cx) 00976 { 00977 CLEAR_BIT(I2Cx->CR1, I2C_CR1_TCIE); 00978 } 00979 00980 /** 00981 * @brief Check if Transfer Complete interrupt is enabled or disabled. 00982 * @rmtoll CR1 TCIE LL_I2C_IsEnabledIT_TC 00983 * @param I2Cx I2C Instance. 00984 * @retval State of bit (1 or 0). 00985 */ 00986 __STATIC_INLINE uint32_t LL_I2C_IsEnabledIT_TC(I2C_TypeDef* I2Cx) 00987 { 00988 return (READ_BIT(I2Cx->CR1, I2C_CR1_TCIE) == (I2C_CR1_TCIE)); 00989 } 00990 00991 /** 00992 * @brief Enable Error interrupts. 00993 * @note Any of these errors will generate interrupt : 00994 * Arbitration Loss (ARLO) 00995 * Bus Error detection (BERR) 00996 * Overrun/Underrun (OVR) 00997 * @rmtoll CR1 ERRIE LL_I2C_EnableIT_ERR 00998 * @param I2Cx I2C Instance. 00999 * @retval None 01000 */ 01001 __STATIC_INLINE void LL_I2C_EnableIT_ERR(I2C_TypeDef* I2Cx) 01002 { 01003 SET_BIT(I2Cx->CR1, I2C_CR1_ERRIE); 01004 } 01005 01006 /** 01007 * @brief Disable Error interrupts. 01008 * @note Any of these errors will generate interrupt : 01009 * Arbitration Loss (ARLO) 01010 * Bus Error detection (BERR) 01011 * Overrun/Underrun (OVR) 01012 * @rmtoll CR1 ERRIE LL_I2C_DisableIT_ERR 01013 * @param I2Cx I2C Instance. 01014 * @retval None 01015 */ 01016 __STATIC_INLINE void LL_I2C_DisableIT_ERR(I2C_TypeDef* I2Cx) 01017 { 01018 CLEAR_BIT(I2Cx->CR1, I2C_CR1_ERRIE); 01019 } 01020 01021 /** 01022 * @brief Check if Error interrupts is enabled of disabled. 01023 * @rmtoll CR1 ERRIE LL_I2C_IsEnabledIT_ERR 01024 * @param I2Cx I2C Instance. 01025 * @retval State of bit (1 or 0). 01026 */ 01027 __STATIC_INLINE uint32_t LL_I2C_IsEnabledIT_ERR(I2C_TypeDef* I2Cx) 01028 { 01029 return (READ_BIT(I2Cx->CR1, I2C_CR1_ERRIE) == (I2C_CR1_ERRIE)); 01030 } 01031 01032 /** 01033 * @} 01034 */ 01035 01036 /** @defgroup I2C_LL_EF_FLAG_management FLAG_management 01037 * @{ 01038 */ 01039 01040 /** 01041 * @brief Indicate the status of Transmit data register empty flag. 01042 * RESET: When next data is written in Transmit data register. 01043 * SET: When Transmit data register is empty. 01044 * @rmtoll ISR TXE LL_I2C_IsActiveFlag_TXE 01045 * @param I2Cx I2C Instance. 01046 * @retval State of bit (1 or 0). 01047 */ 01048 __STATIC_INLINE uint32_t LL_I2C_IsActiveFlag_TXE(I2C_TypeDef* I2Cx) 01049 { 01050 return (READ_BIT(I2Cx->ISR, I2C_ISR_TXE) == (I2C_ISR_TXE)); 01051 } 01052 01053 /** 01054 * @brief Indicate the status of Transmit interrupt flag. 01055 * RESET: When next data is written in Transmit data register. 01056 * SET: When Transmit data register is empty. 01057 * @rmtoll ISR TXIS LL_I2C_IsActiveFlag_TXIS 01058 * @param I2Cx I2C Instance. 01059 * @retval State of bit (1 or 0). 01060 */ 01061 __STATIC_INLINE uint32_t LL_I2C_IsActiveFlag_TXIS(I2C_TypeDef* I2Cx) 01062 { 01063 return (READ_BIT(I2Cx->ISR, I2C_ISR_TXIS) == (I2C_ISR_TXIS)); 01064 } 01065 01066 /** 01067 * @brief Indicate the status of Receive data register not empty flag. 01068 * RESET: When Receive data register is read. 01069 * SET: When the received data is copied in Receive data register. 01070 * @rmtoll ISR RXNE LL_I2C_IsActiveFlag_RXNE 01071 * @param I2Cx I2C Instance. 01072 * @retval State of bit (1 or 0). 01073 */ 01074 __STATIC_INLINE uint32_t LL_I2C_IsActiveFlag_RXNE(I2C_TypeDef* I2Cx) 01075 { 01076 return (READ_BIT(I2Cx->ISR, I2C_ISR_RXNE) == (I2C_ISR_RXNE)); 01077 } 01078 01079 /** 01080 * @brief Indicate the status of Address matched flag (slave mode). 01081 * RESET: Clear default value. 01082 * SET: When the received slave address matched with one of the enabled slave address. 01083 * @rmtoll ISR ADDR LL_I2C_IsActiveFlag_ADDR 01084 * @param I2Cx I2C Instance. 01085 * @retval State of bit (1 or 0). 01086 */ 01087 __STATIC_INLINE uint32_t LL_I2C_IsActiveFlag_ADDR(I2C_TypeDef* I2Cx) 01088 { 01089 return (READ_BIT(I2Cx->ISR, I2C_ISR_ADDR) == (I2C_ISR_ADDR)); 01090 } 01091 01092 /** 01093 * @brief Indicate the status of Not Acknowledge received flag. 01094 * RESET: Clear default value. 01095 * SET: When a NACK is received after a byte transmission. 01096 * @rmtoll ISR NACKF LL_I2C_IsActiveFlag_NACK 01097 * @param I2Cx I2C Instance. 01098 * @retval State of bit (1 or 0). 01099 */ 01100 __STATIC_INLINE uint32_t LL_I2C_IsActiveFlag_NACK(I2C_TypeDef* I2Cx) 01101 { 01102 return (READ_BIT(I2Cx->ISR, I2C_ISR_NACKF) == (I2C_ISR_NACKF)); 01103 } 01104 01105 /** 01106 * @brief Indicate the status of Stop detection flag. 01107 * RESET: Clear default value. 01108 * SET: When a Stop condition is detected. 01109 * @rmtoll ISR STOPF LL_I2C_IsActiveFlag_STOP 01110 * @param I2Cx I2C Instance. 01111 * @retval State of bit (1 or 0). 01112 */ 01113 __STATIC_INLINE uint32_t LL_I2C_IsActiveFlag_STOP(I2C_TypeDef* I2Cx) 01114 { 01115 return (READ_BIT(I2Cx->ISR, I2C_ISR_STOPF) == (I2C_ISR_STOPF)); 01116 } 01117 01118 /** 01119 * @brief Indicate the status of Transfer complete flag (master mode). 01120 * RESET: Clear default value. 01121 * SET: When RELOAD=0, AUTOEND=0 and NBYTES date have been transferred. 01122 * @rmtoll ISR TC LL_I2C_IsActiveFlag_TC 01123 * @param I2Cx I2C Instance. 01124 * @retval State of bit (1 or 0). 01125 */ 01126 __STATIC_INLINE uint32_t LL_I2C_IsActiveFlag_TC(I2C_TypeDef* I2Cx) 01127 { 01128 return (READ_BIT(I2Cx->ISR, I2C_ISR_TC) == (I2C_ISR_TC)); 01129 } 01130 01131 /** 01132 * @brief Indicate the status of Transfer complete flag (master mode). 01133 * RESET: Clear default value. 01134 * SET: When RELOAD=1 and NBYTES date have been transferred. 01135 * @rmtoll ISR TCR LL_I2C_IsActiveFlag_TCR 01136 * @param I2Cx I2C Instance. 01137 * @retval State of bit (1 or 0). 01138 */ 01139 __STATIC_INLINE uint32_t LL_I2C_IsActiveFlag_TCR(I2C_TypeDef* I2Cx) 01140 { 01141 return (READ_BIT(I2Cx->ISR, I2C_ISR_TCR) == (I2C_ISR_TCR)); 01142 } 01143 01144 /** 01145 * @brief Indicate the status of Bus error flag. 01146 * RESET: Clear default value. 01147 * SET: When a misplaced Start or Stop condition is detected. 01148 * @rmtoll ISR BERR LL_I2C_IsActiveFlag_BERR 01149 * @param I2Cx I2C Instance. 01150 * @retval State of bit (1 or 0). 01151 */ 01152 __STATIC_INLINE uint32_t LL_I2C_IsActiveFlag_BERR(I2C_TypeDef* I2Cx) 01153 { 01154 return (READ_BIT(I2Cx->ISR, I2C_ISR_BERR) == (I2C_ISR_BERR)); 01155 } 01156 01157 /** 01158 * @brief Indicate the status of Arbitration lost flag. 01159 * RESET: Clear default value. 01160 * SET: When arbitration lost. 01161 * @rmtoll ISR ARLO LL_I2C_IsActiveFlag_ARLO 01162 * @param I2Cx I2C Instance. 01163 * @retval State of bit (1 or 0). 01164 */ 01165 __STATIC_INLINE uint32_t LL_I2C_IsActiveFlag_ARLO(I2C_TypeDef* I2Cx) 01166 { 01167 return (READ_BIT(I2Cx->ISR, I2C_ISR_ARLO) == (I2C_ISR_ARLO)); 01168 } 01169 01170 /** 01171 * @brief Indicate the status of Overrun/Underrun flag (slave mode). 01172 * RESET: Clear default value. 01173 * SET: When an overrun/underrun error occures (Clock Stretching Disabled). 01174 * @rmtoll ISR OVR LL_I2C_IsActiveFlag_OVR 01175 * @param I2Cx I2C Instance. 01176 * @retval State of bit (1 or 0). 01177 */ 01178 __STATIC_INLINE uint32_t LL_I2C_IsActiveFlag_OVR(I2C_TypeDef* I2Cx) 01179 { 01180 return (READ_BIT(I2Cx->ISR, I2C_ISR_OVR) == (I2C_ISR_OVR)); 01181 } 01182 01183 /** 01184 * @brief Indicate the status of Bus Busy flag. 01185 * RESET: Clear default value. 01186 * SET: When a Start condition is detected. 01187 * @rmtoll ISR BUSY LL_I2C_IsActiveFlag_BUSY 01188 * @param I2Cx I2C Instance. 01189 * @retval State of bit (1 or 0). 01190 */ 01191 __STATIC_INLINE uint32_t LL_I2C_IsActiveFlag_BUSY(I2C_TypeDef* I2Cx) 01192 { 01193 return (READ_BIT(I2Cx->ISR, I2C_ISR_BUSY) == (I2C_ISR_BUSY)); 01194 } 01195 01196 /** 01197 * @brief Clear Address Matched flag. 01198 * @rmtoll ICR ADDRCF LL_I2C_ClearFlag_ADDR 01199 * @param I2Cx I2C Instance. 01200 * @retval None 01201 */ 01202 __STATIC_INLINE void LL_I2C_ClearFlag_ADDR(I2C_TypeDef* I2Cx) 01203 { 01204 SET_BIT(I2Cx->ICR, I2C_ICR_ADDRCF); 01205 } 01206 01207 /** 01208 * @brief Clear Not Acknowledge flag. 01209 * @rmtoll ICR NACKCF LL_I2C_ClearFlag_NACK 01210 * @param I2Cx I2C Instance. 01211 * @retval None 01212 */ 01213 __STATIC_INLINE void LL_I2C_ClearFlag_NACK(I2C_TypeDef* I2Cx) 01214 { 01215 SET_BIT(I2Cx->ICR, I2C_ICR_NACKCF); 01216 } 01217 01218 /** 01219 * @brief Clear Stop detection flag. 01220 * @rmtoll ICR STOPCF LL_I2C_ClearFlag_STOP 01221 * @param I2Cx I2C Instance. 01222 * @retval None 01223 */ 01224 __STATIC_INLINE void LL_I2C_ClearFlag_STOP(I2C_TypeDef* I2Cx) 01225 { 01226 SET_BIT(I2Cx->ICR, I2C_ICR_STOPCF); 01227 } 01228 01229 /** 01230 * @brief Clear Transmit data register empty flag (TXE). 01231 * @note This bit can be clear by software in order to flush the transmit data register (TXDR). 01232 * @rmtoll ISR TXE LL_I2C_ClearFlag_TXE 01233 * @param I2Cx I2C Instance. 01234 * @retval None 01235 */ 01236 __STATIC_INLINE void LL_I2C_ClearFlag_TXE(I2C_TypeDef* I2Cx) 01237 { 01238 I2Cx->ISR = I2C_ISR_TXE; 01239 } 01240 01241 /** 01242 * @brief Clear Bus error flag. 01243 * @rmtoll ICR BERRCF LL_I2C_ClearFlag_BERR 01244 * @param I2Cx I2C Instance. 01245 * @retval None 01246 */ 01247 __STATIC_INLINE void LL_I2C_ClearFlag_BERR(I2C_TypeDef* I2Cx) 01248 { 01249 SET_BIT(I2Cx->ICR, I2C_ICR_BERRCF); 01250 } 01251 01252 /** 01253 * @brief Clear Arbitration lost flag. 01254 * @rmtoll ICR ARLOCF LL_I2C_ClearFlag_ARLO 01255 * @param I2Cx I2C Instance. 01256 * @retval None 01257 */ 01258 __STATIC_INLINE void LL_I2C_ClearFlag_ARLO(I2C_TypeDef* I2Cx) 01259 { 01260 SET_BIT(I2Cx->ICR, I2C_ICR_ARLOCF); 01261 } 01262 01263 /** 01264 * @brief Clear Overrun/Underrun flag. 01265 * @rmtoll ICR OVRCF LL_I2C_ClearFlag_OVR 01266 * @param I2Cx I2C Instance. 01267 * @retval None 01268 */ 01269 __STATIC_INLINE void LL_I2C_ClearFlag_OVR(I2C_TypeDef* I2Cx) 01270 { 01271 SET_BIT(I2Cx->ICR, I2C_ICR_OVRCF); 01272 } 01273 01274 /** 01275 * @} 01276 */ 01277 01278 /** @defgroup I2C_LL_EF_Data_Management Data_Management 01279 * @{ 01280 */ 01281 01282 /** 01283 * @brief Prepare the generation of a ACKnowledge or Non ACKnowledge condition after the address receive match code or next received byte. 01284 * @note Usage in Slave mode only. 01285 * @rmtoll CR2 NACK LL_I2C_AcknowledgeNextData 01286 * @param I2Cx I2C Instance. 01287 * @param TypeAcknowledge This parameter can be one of the following values: 01288 * @arg @ref LL_I2C_ACK 01289 * @arg @ref LL_I2C_NACK 01290 * @retval None 01291 */ 01292 __STATIC_INLINE void LL_I2C_AcknowledgeNextData(I2C_TypeDef* I2Cx, uint32_t TypeAcknowledge) 01293 { 01294 MODIFY_REG(I2Cx->CR2, I2C_CR2_NACK, TypeAcknowledge); 01295 } 01296 01297 /** 01298 * @brief Handles I2Cx communication when starting transfer or during transfer (TC or TCR flag are set). 01299 * @rmtoll CR2 SADD LL_I2C_HandleTransfer\n 01300 * CR2 ADD10 LL_I2C_HandleTransfer\n 01301 * CR2 RD_WRN LL_I2C_HandleTransfer\n 01302 * CR2 START LL_I2C_HandleTransfer\n 01303 * CR2 STOP LL_I2C_HandleTransfer\n 01304 * CR2 RELOAD LL_I2C_HandleTransfer\n 01305 * CR2 NBYTES LL_I2C_HandleTransfer\n 01306 * CR2 AUTOEND LL_I2C_HandleTransfer\n 01307 * CR2 HEAD10R LL_I2C_HandleTransfer 01308 * @param I2Cx I2C Instance. 01309 * @param SlaveAddr Specifies the slave address to be programmed. 01310 * @param SlaveAddrSize This parameter can be one of the following values: 01311 * @arg @ref LL_I2C_ADDRSLAVE_7BIT 01312 * @arg @ref LL_I2C_ADDRSLAVE_10BIT 01313 * @param TransferSize Specifies the number of bytes to be programmed. This parameter must be a value between 0 and 255. 01314 * @param EndMode This parameter can be one of the following values: 01315 * @arg @ref LL_I2C_MODE_RELOAD 01316 * @arg @ref LL_I2C_MODE_AUTOEND 01317 * @arg @ref LL_I2C_MODE_SOFTEND 01318 * @param Request This parameter can be one of the following values: 01319 * @arg @ref LL_I2C_GENERATE_NOSTARTSTOP 01320 * @arg @ref LL_I2C_GENERATE_STOP 01321 * @arg @ref LL_I2C_GENERATE_START_READ 01322 * @arg @ref LL_I2C_GENERATE_START_WRITE 01323 * @arg @ref LL_I2C_GENERATE_RESTART_7BIT_READ 01324 * @arg @ref LL_I2C_GENERATE_RESTART_7BIT_WRITE 01325 * @arg @ref LL_I2C_GENERATE_RESTART_10BIT_READ 01326 * @arg @ref LL_I2C_GENERATE_RESTART_10BIT_WRITE 01327 * @retval None 01328 */ 01329 __STATIC_INLINE void LL_I2C_HandleTransfer(I2C_TypeDef* I2Cx, uint32_t SlaveAddr, uint32_t SlaveAddrSize, uint32_t TransferSize, uint32_t EndMode, uint32_t Request) 01330 { 01331 MODIFY_REG(I2Cx->CR2, I2C_CR2_SADD | I2C_CR2_ADD10 | I2C_CR2_RD_WRN | I2C_CR2_START | I2C_CR2_STOP | I2C_CR2_RELOAD | I2C_CR2_NBYTES | I2C_CR2_AUTOEND | I2C_CR2_HEAD10R, 01332 SlaveAddr | SlaveAddrSize | TransferSize << POSITION_VAL(I2C_CR2_NBYTES) | EndMode | Request); 01333 } 01334 01335 /** 01336 * @brief Indicate the value of transfer direction (slave mode). 01337 * RESET: Write transfer, Slave enters in receiver mode. 01338 * SET: Read transfer, Slave enters in transmitter mode. 01339 * @rmtoll ISR DIR LL_I2C_GetTransferDirection 01340 * @param I2Cx I2C Instance. 01341 * @retval Returned value can be one of the following values: 01342 * @arg @ref LL_I2C_DIRECTION_WRITE 01343 * @arg @ref LL_I2C_DIRECTION_READ 01344 */ 01345 __STATIC_INLINE uint32_t LL_I2C_GetTransferDirection(I2C_TypeDef* I2Cx) 01346 { 01347 return (uint32_t)(READ_BIT(I2Cx->ISR, I2C_ISR_DIR)); 01348 } 01349 01350 /** 01351 * @brief Return the slave matched address. 01352 * @rmtoll ISR ADDCODE LL_I2C_GetAddressMatchCode 01353 * @param I2Cx I2C Instance. 01354 * @retval 0..0x3F 01355 */ 01356 __STATIC_INLINE uint32_t LL_I2C_GetAddressMatchCode(I2C_TypeDef* I2Cx) 01357 { 01358 return (uint32_t)(READ_BIT(I2Cx->ISR, I2C_ISR_ADDCODE) >> POSITION_VAL(I2C_ISR_ADDCODE) << 1); 01359 } 01360 01361 /** 01362 * @brief Read Receive Data register. 01363 * @rmtoll RXDR RXDATA LL_I2C_ReceiveData8 01364 * @param I2Cx I2C Instance. 01365 * @retval 0..0xFF 01366 */ 01367 __STATIC_INLINE uint8_t LL_I2C_ReceiveData8(I2C_TypeDef* I2Cx) 01368 { 01369 return (uint8_t)(READ_BIT(I2Cx->RXDR, I2C_RXDR_RXDATA)); 01370 } 01371 01372 /** 01373 * @brief Write in Transmit Data Register . 01374 * @rmtoll TXDR TXDATA LL_I2C_TransmitData8 01375 * @param I2Cx I2C Instance. 01376 * @param Data 0..0xFF 01377 * @retval None 01378 */ 01379 __STATIC_INLINE void LL_I2C_TransmitData8(I2C_TypeDef* I2Cx, uint8_t Data) 01380 { 01381 WRITE_REG(I2Cx->TXDR, Data); 01382 } 01383 01384 /** 01385 * @} 01386 */ 01387 01388 01389 /** 01390 * @} 01391 */ 01392 01393 /** 01394 * @} 01395 */ 01396 01397 #endif /* I2C1 || I2C2 || I2C3 */ 01398 01399 /** 01400 * @} 01401 */ 01402 01403 #ifdef __cplusplus 01404 } 01405 #endif 01406 01407 #endif /* __STM32L4xx_LL_I2C_H */ 01408 01409 /************************ (C) COPYRIGHT STMicroelectronics *****END OF FILE****/ 01410
Generated on Tue Jul 12 2022 11:35:16 by
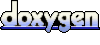