Hal Drivers for L4
Dependents: BSP OneHopeOnePrayer FINAL_AUDIO_RECORD AudioDemo
Fork of STM32L4xx_HAL_Driver by
stm32l4xx_ll_fmc.c
00001 /** 00002 ****************************************************************************** 00003 * @file stm32l4xx_ll_fmc.c 00004 * @author MCD Application Team 00005 * @version V1.1.0 00006 * @date 16-September-2015 00007 * @brief FMC Low Layer HAL module driver. 00008 * This file provides firmware functions to manage the following 00009 * functionalities of the Flexible Memory Controller (FMC) peripheral memories: 00010 * + Initialization/de-initialization functions 00011 * + Peripheral Control functions 00012 * + Peripheral State functions 00013 * 00014 @verbatim 00015 ============================================================================== 00016 ##### FMC peripheral features ##### 00017 ============================================================================== 00018 [..] The Flexible memory controller (FMC) includes following memory controllers: 00019 (+) The NOR/PSRAM memory controller 00020 (+) The NAND memory controller 00021 00022 [..] The FMC functional block makes the interface with synchronous and asynchronous static 00023 memories and 16-bit PC memory cards. Its main purposes are: 00024 (+) to translate AHB transactions into the appropriate external device protocol. 00025 (+) to meet the access time requirements of the external memory devices. 00026 00027 [..] All external memories share the addresses, data and control signals with the controller. 00028 Each external device is accessed by means of a unique Chip Select. The FMC performs 00029 only one access at a time to an external device. 00030 The main features of the FMC controller are the following: 00031 (+) Interface with static-memory mapped devices including: 00032 (++) Static random access memory (SRAM). 00033 (++) NOR Flash memory. 00034 (++) PSRAM (4 memory banks). 00035 (++) Two banks of NAND Flash memory with ECC hardware to check up to 8 Kbytes of 00036 data 00037 (+) Independent Chip Select control for each memory bank 00038 (+) Independent configuration for each memory bank 00039 00040 @endverbatim 00041 ****************************************************************************** 00042 * @attention 00043 * 00044 * <h2><center>© COPYRIGHT(c) 2015 STMicroelectronics</center></h2> 00045 * 00046 * Redistribution and use in source and binary forms, with or without modification, 00047 * are permitted provided that the following conditions are met: 00048 * 1. Redistributions of source code must retain the above copyright notice, 00049 * this list of conditions and the following disclaimer. 00050 * 2. Redistributions in binary form must reproduce the above copyright notice, 00051 * this list of conditions and the following disclaimer in the documentation 00052 * and/or other materials provided with the distribution. 00053 * 3. Neither the name of STMicroelectronics nor the names of its contributors 00054 * may be used to endorse or promote products derived from this software 00055 * without specific prior written permission. 00056 * 00057 * THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" 00058 * AND ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE 00059 * IMPLIED WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE 00060 * DISCLAIMED. IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE 00061 * FOR ANY DIRECT, INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL 00062 * DAMAGES (INCLUDING, BUT NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR 00063 * SERVICES; LOSS OF USE, DATA, OR PROFITS; OR BUSINESS INTERRUPTION) HOWEVER 00064 * CAUSED AND ON ANY THEORY OF LIABILITY, WHETHER IN CONTRACT, STRICT LIABILITY, 00065 * OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) ARISING IN ANY WAY OUT OF THE USE 00066 * OF THIS SOFTWARE, EVEN IF ADVISED OF THE POSSIBILITY OF SUCH DAMAGE. 00067 * 00068 ****************************************************************************** 00069 */ 00070 00071 /* Includes ------------------------------------------------------------------*/ 00072 #include "stm32l4xx_hal.h" 00073 00074 /** @addtogroup STM32L4xx_HAL_Driver 00075 * @{ 00076 */ 00077 00078 #if defined (HAL_SRAM_MODULE_ENABLED) || defined(HAL_NOR_MODULE_ENABLED) || defined(HAL_NAND_MODULE_ENABLED) 00079 00080 /** @defgroup FMC_LL FMC Low Layer 00081 * @brief FMC driver modules 00082 * @{ 00083 */ 00084 00085 /* Private typedef -----------------------------------------------------------*/ 00086 /* Private define ------------------------------------------------------------*/ 00087 /** @defgroup FMC_LL_Private_Constants FMC Low Layer Private Constants 00088 * @{ 00089 */ 00090 00091 /* ----------------------- FMC registers bit mask --------------------------- */ 00092 /* --- BCRx Register ---*/ 00093 /* BCRx register clear mask */ 00094 #define BCRx_CLEAR_MASK ((uint32_t)(FMC_BCRx_MBKEN | FMC_BCRx_MUXEN |\ 00095 FMC_BCRx_MTYP | FMC_BCRx_MWID |\ 00096 FMC_BCRx_FACCEN | FMC_BCRx_BURSTEN |\ 00097 FMC_BCRx_WAITPOL | FMC_BCRx_WAITCFG |\ 00098 FMC_BCRx_WREN | FMC_BCRx_WAITEN |\ 00099 FMC_BCRx_EXTMOD | FMC_BCRx_ASYNCWAIT |\ 00100 FMC_BCRx_CPSIZE | FMC_BCRx_CBURSTRW)) 00101 /* --- BTRx Register ---*/ 00102 /* BTRx register clear mask */ 00103 #define BTRx_CLEAR_MASK ((uint32_t)(FMC_BTRx_ADDSET | FMC_BTRx_ADDHLD |\ 00104 FMC_BTRx_DATAST | FMC_BTRx_BUSTURN |\ 00105 FMC_BTRx_CLKDIV | FMC_BTRx_DATLAT |\ 00106 FMC_BTRx_ACCMOD)) 00107 00108 /* --- BWTRx Register ---*/ 00109 /* BWTRx register clear mask */ 00110 #define BWTRx_CLEAR_MASK ((uint32_t)(FMC_BWTRx_ADDSET | FMC_BWTRx_ADDHLD |\ 00111 FMC_BWTRx_DATAST | FMC_BWTRx_ACCMOD)) 00112 00113 /* --- PCR Register ---*/ 00114 /* PCR register clear mask */ 00115 #define PCR_CLEAR_MASK ((uint32_t)(FMC_PCR_PWAITEN | FMC_PCR_PBKEN |\ 00116 FMC_PCR_PTYP | FMC_PCR_PWID |\ 00117 FMC_PCR_ECCEN | FMC_PCR_TCLR |\ 00118 FMC_PCR_TAR | FMC_PCR_ECCPS)) 00119 00120 /* --- SR Register ---*/ 00121 /* SR register clear mask */ 00122 #define SR_CLEAR_MASK ((uint32_t)(FMC_SR_FEMPT)) 00123 00124 /* --- PMEM Register ---*/ 00125 /* PMEM register clear mask */ 00126 #define PMEM_CLEAR_MASK ((uint32_t)(FMC_PMEM_MEMSET | FMC_PMEM_MEMWAIT |\ 00127 FMC_PMEM_MEMHOLD | FMC_PMEM_MEMHIZ)) 00128 00129 /* --- PATT Register ---*/ 00130 /* PATT register clear mask */ 00131 #define PATT_CLEAR_MASK ((uint32_t)(FMC_PATT_ATTSET | FMC_PATT_ATTWAIT |\ 00132 FMC_PATT_ATTHOLD | FMC_PATT_ATTHIZ)) 00133 /** 00134 * @} 00135 */ 00136 00137 /* Private macro -------------------------------------------------------------*/ 00138 /** @defgroup FMC_LL_Private_Macros FMC Low Layer Private Macros 00139 * @{ 00140 */ 00141 00142 /** 00143 * @} 00144 */ 00145 00146 /* Private variables ---------------------------------------------------------*/ 00147 /* Private function prototypes -----------------------------------------------*/ 00148 /* Exported functions --------------------------------------------------------*/ 00149 00150 /** @defgroup FMC_LL_Exported_Functions FMC Low Layer Exported Functions 00151 * @{ 00152 */ 00153 00154 /** @defgroup FMC_LL_Exported_Functions_NORSRAM FMC Low Layer NOR SRAM Exported Functions 00155 * @brief NORSRAM Controller functions 00156 * 00157 @verbatim 00158 ============================================================================== 00159 ##### How to use NORSRAM device driver ##### 00160 ============================================================================== 00161 00162 [..] 00163 This driver contains a set of APIs to interface with the FMC NORSRAM banks in order 00164 to run the NORSRAM external devices. 00165 00166 (+) FMC NORSRAM bank reset using the function FMC_NORSRAM_DeInit() 00167 (+) FMC NORSRAM bank control configuration using the function FMC_NORSRAM_Init() 00168 (+) FMC NORSRAM bank timing configuration using the function FMC_NORSRAM_Timing_Init() 00169 (+) FMC NORSRAM bank extended timing configuration using the function 00170 FMC_NORSRAM_Extended_Timing_Init() 00171 (+) FMC NORSRAM bank enable/disable write operation using the functions 00172 FMC_NORSRAM_WriteOperation_Enable()/FMC_NORSRAM_WriteOperation_Disable() 00173 00174 00175 @endverbatim 00176 * @{ 00177 */ 00178 00179 /** @defgroup FMC_LL_NORSRAM_Exported_Functions_Group1 Initialization and de-initialization functions 00180 * @brief Initialization and Configuration functions 00181 * 00182 @verbatim 00183 ============================================================================== 00184 ##### Initialization and de-initialization functions ##### 00185 ============================================================================== 00186 [..] 00187 This section provides functions allowing to: 00188 (+) Initialize and configure the FMC NORSRAM interface 00189 (+) De-initialize the FMC NORSRAM interface 00190 (+) Configure the FMC clock and associated GPIOs 00191 00192 @endverbatim 00193 * @{ 00194 */ 00195 00196 /** 00197 * @brief Initialize the FMC_NORSRAM device according to the specified 00198 * control parameters in the FMC_NORSRAM_InitTypeDef 00199 * @param Device: Pointer to NORSRAM device instance 00200 * @param Init: Pointer to NORSRAM Initialization structure 00201 * @retval HAL status 00202 */ 00203 HAL_StatusTypeDef FMC_NORSRAM_Init(FMC_NORSRAM_TypeDef *Device, FMC_NORSRAM_InitTypeDef* Init) 00204 { 00205 /* Check the parameters */ 00206 assert_param(IS_FMC_NORSRAM_DEVICE(Device)); 00207 assert_param(IS_FMC_NORSRAM_BANK(Init->NSBank )); 00208 assert_param(IS_FMC_MUX(Init->DataAddressMux )); 00209 assert_param(IS_FMC_MEMORY(Init->MemoryType )); 00210 assert_param(IS_FMC_NORSRAM_MEMORY_WIDTH(Init->MemoryDataWidth )); 00211 assert_param(IS_FMC_BURSTMODE(Init->BurstAccessMode )); 00212 assert_param(IS_FMC_WAIT_POLARITY(Init->WaitSignalPolarity )); 00213 assert_param(IS_FMC_WAIT_SIGNAL_ACTIVE(Init->WaitSignalActive )); 00214 assert_param(IS_FMC_WRITE_OPERATION(Init->WriteOperation )); 00215 assert_param(IS_FMC_WAITE_SIGNAL(Init->WaitSignal )); 00216 assert_param(IS_FMC_EXTENDED_MODE(Init->ExtendedMode )); 00217 assert_param(IS_FMC_ASYNWAIT(Init->AsynchronousWait )); 00218 assert_param(IS_FMC_WRITE_BURST(Init->WriteBurst )); 00219 assert_param(IS_FMC_CONTINOUS_CLOCK(Init->ContinuousClock )); 00220 assert_param(IS_FMC_WRITE_FIFO(Init->WriteFifo )); 00221 assert_param(IS_FMC_PAGESIZE(Init->PageSize )); 00222 00223 /* Set NORSRAM device control parameters */ 00224 if(Init->MemoryType == FMC_MEMORY_TYPE_NOR) 00225 { 00226 MODIFY_REG(Device->BTCR[Init->NSBank ], BCRx_CLEAR_MASK, (uint32_t)(FMC_NORSRAM_FLASH_ACCESS_ENABLE |\ 00227 Init->DataAddressMux |\ 00228 Init->MemoryType |\ 00229 Init->MemoryDataWidth |\ 00230 Init->BurstAccessMode |\ 00231 Init->WaitSignalPolarity |\ 00232 Init->WaitSignalActive |\ 00233 Init->WriteOperation |\ 00234 Init->WaitSignal |\ 00235 Init->ExtendedMode |\ 00236 Init->AsynchronousWait |\ 00237 Init->WriteBurst |\ 00238 Init->ContinuousClock |\ 00239 Init->WriteFifo |\ 00240 Init->PageSize ) 00241 ); 00242 } 00243 else 00244 { 00245 MODIFY_REG(Device->BTCR[Init->NSBank ], BCRx_CLEAR_MASK, (uint32_t)(FMC_NORSRAM_FLASH_ACCESS_DISABLE |\ 00246 Init->DataAddressMux |\ 00247 Init->MemoryType |\ 00248 Init->MemoryDataWidth |\ 00249 Init->BurstAccessMode |\ 00250 Init->WaitSignalPolarity |\ 00251 Init->WaitSignalActive |\ 00252 Init->WriteOperation |\ 00253 Init->WaitSignal |\ 00254 Init->ExtendedMode |\ 00255 Init->AsynchronousWait |\ 00256 Init->WriteBurst |\ 00257 Init->ContinuousClock |\ 00258 Init->WriteFifo |\ 00259 Init->PageSize ) 00260 ); 00261 } 00262 00263 /* Specific bits on bank1 register for bank2..4 */ 00264 if(Init->NSBank != FMC_NORSRAM_BANK1) 00265 { 00266 /* Configure Write FIFO mode when Write Fifo is enabled for bank2..4 */ 00267 SET_BIT(Device->BTCR[FMC_NORSRAM_BANK1], (uint32_t)(Init->WriteFifo )); 00268 00269 /* Configure synchronous mode when Continuous clock is enabled for bank2..4 */ 00270 if(Init->ContinuousClock == FMC_CONTINUOUS_CLOCK_SYNC_ASYNC) 00271 { 00272 Init->BurstAccessMode = FMC_BURST_ACCESS_MODE_ENABLE; 00273 MODIFY_REG(Device->BTCR[FMC_NORSRAM_BANK1], FMC_BCRx_BURSTEN | FMC_BCR1_CCLKEN, (uint32_t)(Init->BurstAccessMode |\ 00274 Init->ContinuousClock )); 00275 } 00276 } 00277 00278 return HAL_OK; 00279 } 00280 00281 00282 /** 00283 * @brief DeInitialize the FMC_NORSRAM peripheral 00284 * @param Device: Pointer to NORSRAM device instance 00285 * @param ExDevice: Pointer to NORSRAM extended mode device instance 00286 * @param Bank: NORSRAM bank number 00287 * @retval HAL status 00288 */ 00289 HAL_StatusTypeDef FMC_NORSRAM_DeInit(FMC_NORSRAM_TypeDef *Device, FMC_NORSRAM_EXTENDED_TypeDef *ExDevice, uint32_t Bank) 00290 { 00291 /* Check the parameters */ 00292 assert_param(IS_FMC_NORSRAM_DEVICE(Device)); 00293 assert_param(IS_FMC_NORSRAM_EXTENDED_DEVICE(ExDevice)); 00294 assert_param(IS_FMC_NORSRAM_BANK(Bank)); 00295 00296 /* Disable the FMC_NORSRAM device */ 00297 __FMC_NORSRAM_DISABLE(Device, Bank); 00298 00299 /* De-initialize the FMC_NORSRAM device */ 00300 /* FMC_NORSRAM_BANK1 */ 00301 if(Bank == FMC_NORSRAM_BANK1) 00302 { 00303 Device->BTCR[Bank] = 0x000030DB; 00304 } 00305 /* FMC_NORSRAM_BANK2, FMC_NORSRAM_BANK3 or FMC_NORSRAM_BANK4 */ 00306 else 00307 { 00308 Device->BTCR[Bank] = 0x000030D2; 00309 } 00310 00311 Device->BTCR[Bank + 1] = 0x0FFFFFFF; 00312 ExDevice->BWTR[Bank] = 0x0FFFFFFF; 00313 00314 return HAL_OK; 00315 } 00316 00317 00318 /** 00319 * @brief Initialize the FMC_NORSRAM Timing according to the specified 00320 * parameters in the FMC_NORSRAM_TimingTypeDef 00321 * @param Device: Pointer to NORSRAM device instance 00322 * @param Timing: Pointer to NORSRAM Timing structure 00323 * @param Bank: NORSRAM bank number 00324 * @retval HAL status 00325 */ 00326 HAL_StatusTypeDef FMC_NORSRAM_Timing_Init(FMC_NORSRAM_TypeDef *Device, FMC_NORSRAM_TimingTypeDef *Timing, uint32_t Bank) 00327 { 00328 uint32_t tmpr = 0; 00329 00330 /* Check the parameters */ 00331 assert_param(IS_FMC_NORSRAM_DEVICE(Device)); 00332 assert_param(IS_FMC_ADDRESS_SETUP_TIME(Timing->AddressSetupTime )); 00333 assert_param(IS_FMC_ADDRESS_HOLD_TIME(Timing->AddressHoldTime )); 00334 assert_param(IS_FMC_DATASETUP_TIME(Timing->DataSetupTime )); 00335 assert_param(IS_FMC_TURNAROUND_TIME(Timing->BusTurnAroundDuration )); 00336 assert_param(IS_FMC_CLK_DIV(Timing->CLKDivision )); 00337 assert_param(IS_FMC_DATA_LATENCY(Timing->DataLatency )); 00338 assert_param(IS_FMC_ACCESS_MODE(Timing->AccessMode )); 00339 assert_param(IS_FMC_NORSRAM_BANK(Bank)); 00340 00341 /* Set FMC_NORSRAM device timing parameters */ 00342 MODIFY_REG(Device->BTCR[Bank + 1], \ 00343 BTRx_CLEAR_MASK, \ 00344 (uint32_t)(Timing->AddressSetupTime |\ 00345 ((Timing->AddressHoldTime ) << POSITION_VAL(FMC_BTRx_ADDHLD)) |\ 00346 ((Timing->DataSetupTime ) << POSITION_VAL(FMC_BTRx_DATAST)) |\ 00347 ((Timing->BusTurnAroundDuration ) << POSITION_VAL(FMC_BTRx_BUSTURN)) |\ 00348 (((Timing->CLKDivision )-1) << POSITION_VAL(FMC_BTRx_CLKDIV)) |\ 00349 (((Timing->DataLatency )-2) << POSITION_VAL(FMC_BTRx_DATLAT)) |\ 00350 (Timing->AccessMode ))); 00351 00352 /* Configure Clock division value (in NORSRAM bank 1) when continuous clock is enabled */ 00353 if(HAL_IS_BIT_SET(Device->BTCR[FMC_NORSRAM_BANK1], FMC_BCR1_CCLKEN)) 00354 { 00355 tmpr = (uint32_t)(Device->BTCR[FMC_NORSRAM_BANK1 + 1] & ~(((uint32_t)0x0F) << POSITION_VAL(FMC_BTRx_CLKDIV))); 00356 tmpr |= (uint32_t)(((Timing->CLKDivision )-1) << POSITION_VAL(FMC_BTRx_CLKDIV)); 00357 MODIFY_REG(Device->BTCR[FMC_NORSRAM_BANK1 + 1], FMC_BTRx_CLKDIV, tmpr); 00358 } 00359 00360 return HAL_OK; 00361 } 00362 00363 /** 00364 * @brief Initialize the FMC_NORSRAM Extended mode Timing according to the specified 00365 * parameters in the FMC_NORSRAM_TimingTypeDef 00366 * @param Device: Pointer to NORSRAM device instance 00367 * @param Timing: Pointer to NORSRAM Timing structure 00368 * @param Bank: NORSRAM bank number 00369 * @param ExtendedMode: FMC Extended Mode 00370 * This parameter can be one of the following values: 00371 * @arg FMC_EXTENDED_MODE_DISABLE 00372 * @arg FMC_EXTENDED_MODE_ENABLE 00373 * @retval HAL status 00374 */ 00375 HAL_StatusTypeDef FMC_NORSRAM_Extended_Timing_Init(FMC_NORSRAM_EXTENDED_TypeDef *Device, FMC_NORSRAM_TimingTypeDef *Timing, uint32_t Bank, uint32_t ExtendedMode) 00376 { 00377 /* Check the parameters */ 00378 assert_param(IS_FMC_EXTENDED_MODE(ExtendedMode)); 00379 00380 /* Set NORSRAM device timing register for write configuration, if extended mode is used */ 00381 if(ExtendedMode == FMC_EXTENDED_MODE_ENABLE) 00382 { 00383 /* Check the parameters */ 00384 assert_param(IS_FMC_NORSRAM_EXTENDED_DEVICE(Device)); 00385 assert_param(IS_FMC_ADDRESS_SETUP_TIME(Timing->AddressSetupTime )); 00386 assert_param(IS_FMC_ADDRESS_HOLD_TIME(Timing->AddressHoldTime )); 00387 assert_param(IS_FMC_DATASETUP_TIME(Timing->DataSetupTime )); 00388 assert_param(IS_FMC_ACCESS_MODE(Timing->AccessMode )); 00389 assert_param(IS_FMC_NORSRAM_BANK(Bank)); 00390 00391 /* Set NORSRAM device timing register for write configuration, if extended mode is used */ 00392 MODIFY_REG(Device->BWTR[Bank], \ 00393 BWTRx_CLEAR_MASK, \ 00394 (uint32_t)(Timing->AddressSetupTime |\ 00395 ((Timing->AddressHoldTime ) << POSITION_VAL(FMC_BTRx_ADDHLD)) |\ 00396 ((Timing->DataSetupTime ) << POSITION_VAL(FMC_BTRx_DATAST)) |\ 00397 (Timing->AccessMode ))); 00398 } 00399 else 00400 { 00401 Device->BWTR[Bank] = 0x0FFFFFFF; 00402 } 00403 00404 return HAL_OK; 00405 } 00406 00407 00408 /** 00409 * @} 00410 */ 00411 00412 00413 /** @defgroup FMC_NORSRAM_Exported_Functions_Group2 Peripheral Control functions 00414 * @brief management functions 00415 * 00416 @verbatim 00417 ============================================================================== 00418 ##### FMC_NORSRAM Control functions ##### 00419 ============================================================================== 00420 [..] 00421 This subsection provides a set of functions allowing to control dynamically 00422 the FMC NORSRAM interface. 00423 00424 @endverbatim 00425 * @{ 00426 */ 00427 00428 /** 00429 * @brief Enables dynamically FMC_NORSRAM write operation. 00430 * @param Device: Pointer to NORSRAM device instance 00431 * @param Bank: NORSRAM bank number 00432 * @retval HAL status 00433 */ 00434 HAL_StatusTypeDef FMC_NORSRAM_WriteOperation_Enable(FMC_NORSRAM_TypeDef *Device, uint32_t Bank) 00435 { 00436 /* Check the parameters */ 00437 assert_param(IS_FMC_NORSRAM_DEVICE(Device)); 00438 assert_param(IS_FMC_NORSRAM_BANK(Bank)); 00439 00440 /* Enable write operation */ 00441 SET_BIT(Device->BTCR[Bank], FMC_WRITE_OPERATION_ENABLE); 00442 00443 return HAL_OK; 00444 } 00445 00446 /** 00447 * @brief Disables dynamically FMC_NORSRAM write operation. 00448 * @param Device: Pointer to NORSRAM device instance 00449 * @param Bank: NORSRAM bank number 00450 * @retval HAL status 00451 */ 00452 HAL_StatusTypeDef FMC_NORSRAM_WriteOperation_Disable(FMC_NORSRAM_TypeDef *Device, uint32_t Bank) 00453 { 00454 /* Check the parameters */ 00455 assert_param(IS_FMC_NORSRAM_DEVICE(Device)); 00456 assert_param(IS_FMC_NORSRAM_BANK(Bank)); 00457 00458 /* Disable write operation */ 00459 CLEAR_BIT(Device->BTCR[Bank], FMC_WRITE_OPERATION_ENABLE); 00460 00461 return HAL_OK; 00462 } 00463 00464 /** 00465 * @} 00466 */ 00467 00468 /** 00469 * @} 00470 */ 00471 00472 /** @defgroup FMC_LL_Exported_Functions_NAND FMC Low Layer NAND Exported Functions 00473 * @brief NAND Controller functions 00474 * 00475 @verbatim 00476 ============================================================================== 00477 ##### How to use NAND device driver ##### 00478 ============================================================================== 00479 [..] 00480 This driver contains a set of APIs to interface with the FMC NAND banks in order 00481 to run the NAND external devices. 00482 00483 (+) FMC NAND bank reset using the function FMC_NAND_DeInit() 00484 (+) FMC NAND bank control configuration using the function FMC_NAND_Init() 00485 (+) FMC NAND bank common space timing configuration using the function 00486 FMC_NAND_CommonSpace_Timing_Init() 00487 (+) FMC NAND bank attribute space timing configuration using the function 00488 FMC_NAND_AttributeSpace_Timing_Init() 00489 (+) FMC NAND bank enable/disable ECC correction feature using the functions 00490 FMC_NAND_ECC_Enable()/FMC_NAND_ECC_Disable() 00491 (+) FMC NAND bank get ECC correction code using the function FMC_NAND_GetECC() 00492 00493 @endverbatim 00494 * @{ 00495 */ 00496 00497 /** @defgroup FMC_LL_NAND_Exported_Functions_Group1 Initialization and de-initialization functions 00498 * @brief Initialization and Configuration functions 00499 * 00500 @verbatim 00501 ============================================================================== 00502 ##### Initialization and de-initialization functions ##### 00503 ============================================================================== 00504 [..] 00505 This section provides functions allowing to: 00506 (+) Initialize and configure the FMC NAND interface 00507 (+) De-initialize the FMC NAND interface 00508 (+) Configure the FMC clock and associated GPIOs 00509 00510 @endverbatim 00511 * @{ 00512 */ 00513 00514 /** 00515 * @brief Initializes the FMC_NAND device according to the specified 00516 * control parameters in the FMC_NAND_HandleTypeDef 00517 * @param Device: Pointer to NAND device instance 00518 * @param Init: Pointer to NAND Initialization structure 00519 * @retval HAL status 00520 */ 00521 HAL_StatusTypeDef FMC_NAND_Init(FMC_NAND_TypeDef *Device, FMC_NAND_InitTypeDef *Init) 00522 { 00523 /* Check the parameters */ 00524 assert_param(IS_FMC_NAND_DEVICE(Device)); 00525 assert_param(IS_FMC_NAND_BANK(Init->NandBank )); 00526 assert_param(IS_FMC_WAIT_FEATURE(Init->Waitfeature )); 00527 assert_param(IS_FMC_NAND_MEMORY_WIDTH(Init->MemoryDataWidth )); 00528 assert_param(IS_FMC_ECC_STATE(Init->EccComputation )); 00529 assert_param(IS_FMC_ECCPAGE_SIZE(Init->ECCPageSize )); 00530 assert_param(IS_FMC_TCLR_TIME(Init->TCLRSetupTime )); 00531 assert_param(IS_FMC_TAR_TIME(Init->TARSetupTime )); 00532 00533 /* Set NAND device control parameters */ 00534 /* NAND bank 3 registers configuration */ 00535 MODIFY_REG(Device->PCR, PCR_CLEAR_MASK, (Init->Waitfeature |\ 00536 FMC_PCR_MEMORY_TYPE_NAND |\ 00537 Init->MemoryDataWidth |\ 00538 Init->EccComputation |\ 00539 Init->ECCPageSize |\ 00540 ((Init->TCLRSetupTime ) << POSITION_VAL(FMC_PCR_TCLR)) |\ 00541 ((Init->TARSetupTime ) << POSITION_VAL(FMC_PCR_TAR)))); 00542 00543 return HAL_OK; 00544 00545 } 00546 00547 /** 00548 * @brief Initializes the FMC_NAND Common space Timing according to the specified 00549 * parameters in the FMC_NAND_PCC_TimingTypeDef 00550 * @param Device: Pointer to NAND device instance 00551 * @param Timing: Pointer to NAND timing structure 00552 * @param Bank: NAND bank number 00553 * @retval HAL status 00554 */ 00555 HAL_StatusTypeDef FMC_NAND_CommonSpace_Timing_Init(FMC_NAND_TypeDef *Device, FMC_NAND_PCC_TimingTypeDef *Timing, uint32_t Bank) 00556 { 00557 /* Check the parameters */ 00558 assert_param(IS_FMC_NAND_DEVICE(Device)); 00559 assert_param(IS_FMC_SETUP_TIME(Timing->SetupTime )); 00560 assert_param(IS_FMC_WAIT_TIME(Timing->WaitSetupTime )); 00561 assert_param(IS_FMC_HOLD_TIME(Timing->HoldSetupTime )); 00562 assert_param(IS_FMC_HIZ_TIME(Timing->HiZSetupTime )); 00563 assert_param(IS_FMC_NAND_BANK(Bank)); 00564 00565 /* Set FMC_NAND device timing parameters */ 00566 /* NAND bank 3 registers configuration */ 00567 MODIFY_REG(Device->PMEM, PMEM_CLEAR_MASK, (Timing->SetupTime |\ 00568 ((Timing->WaitSetupTime ) << POSITION_VAL(FMC_PMEM_MEMWAIT)) |\ 00569 ((Timing->HoldSetupTime ) << POSITION_VAL(FMC_PMEM_MEMHOLD)) |\ 00570 ((Timing->HiZSetupTime ) << POSITION_VAL(FMC_PMEM_MEMHIZ)))); 00571 00572 return HAL_OK; 00573 } 00574 00575 /** 00576 * @brief Initializes the FMC_NAND Attribute space Timing according to the specified 00577 * parameters in the FMC_NAND_PCC_TimingTypeDef 00578 * @param Device: Pointer to NAND device instance 00579 * @param Timing: Pointer to NAND timing structure 00580 * @param Bank: NAND bank number 00581 * @retval HAL status 00582 */ 00583 HAL_StatusTypeDef FMC_NAND_AttributeSpace_Timing_Init(FMC_NAND_TypeDef *Device, FMC_NAND_PCC_TimingTypeDef *Timing, uint32_t Bank) 00584 { 00585 /* Check the parameters */ 00586 assert_param(IS_FMC_NAND_DEVICE(Device)); 00587 assert_param(IS_FMC_SETUP_TIME(Timing->SetupTime )); 00588 assert_param(IS_FMC_WAIT_TIME(Timing->WaitSetupTime )); 00589 assert_param(IS_FMC_HOLD_TIME(Timing->HoldSetupTime )); 00590 assert_param(IS_FMC_HIZ_TIME(Timing->HiZSetupTime )); 00591 assert_param(IS_FMC_NAND_BANK(Bank)); 00592 00593 /* Set FMC_NAND device timing parameters */ 00594 /* NAND bank 3 registers configuration */ 00595 MODIFY_REG(Device->PATT, PATT_CLEAR_MASK, (Timing->SetupTime |\ 00596 ((Timing->WaitSetupTime ) << POSITION_VAL(FMC_PMEM_MEMWAIT)) |\ 00597 ((Timing->HoldSetupTime ) << POSITION_VAL(FMC_PMEM_MEMHOLD)) |\ 00598 ((Timing->HiZSetupTime ) << POSITION_VAL(FMC_PMEM_MEMHIZ)))); 00599 00600 return HAL_OK; 00601 } 00602 00603 00604 /** 00605 * @brief DeInitialize the FMC_NAND device 00606 * @param Device: Pointer to NAND device instance 00607 * @param Bank: NAND bank number 00608 * @retval HAL status 00609 */ 00610 HAL_StatusTypeDef FMC_NAND_DeInit(FMC_NAND_TypeDef *Device, uint32_t Bank) 00611 { 00612 /* Check the parameters */ 00613 assert_param(IS_FMC_NAND_DEVICE(Device)); 00614 assert_param(IS_FMC_NAND_BANK(Bank)); 00615 00616 /* Disable the NAND Bank */ 00617 __FMC_NAND_DISABLE(Device, Bank); 00618 00619 /* Set the FMC_NAND_BANK registers to their reset values */ 00620 WRITE_REG(Device->PCR, 0x00000018); 00621 WRITE_REG(Device->SR, 0x00000040); 00622 WRITE_REG(Device->PMEM, 0xFCFCFCFC); 00623 WRITE_REG(Device->PATT, 0xFCFCFCFC); 00624 00625 return HAL_OK; 00626 } 00627 00628 /** 00629 * @} 00630 */ 00631 00632 00633 /** @defgroup FMC_LL_NAND_Exported_Functions_Group2 FMC Low Layer Peripheral Control functions 00634 * @brief management functions 00635 * 00636 @verbatim 00637 ============================================================================== 00638 ##### FMC_NAND Control functions ##### 00639 ============================================================================== 00640 [..] 00641 This subsection provides a set of functions allowing to control dynamically 00642 the FMC NAND interface. 00643 00644 @endverbatim 00645 * @{ 00646 */ 00647 00648 00649 /** 00650 * @brief Enables dynamically FMC_NAND ECC feature. 00651 * @param Device: Pointer to NAND device instance 00652 * @param Bank: NAND bank number 00653 * @retval HAL status 00654 */ 00655 HAL_StatusTypeDef FMC_NAND_ECC_Enable(FMC_NAND_TypeDef *Device, uint32_t Bank) 00656 { 00657 /* Check the parameters */ 00658 assert_param(IS_FMC_NAND_DEVICE(Device)); 00659 assert_param(IS_FMC_NAND_BANK(Bank)); 00660 00661 /* Enable ECC feature */ 00662 SET_BIT(Device->PCR, FMC_PCR_ECCEN); 00663 00664 return HAL_OK; 00665 } 00666 00667 00668 /** 00669 * @brief Disables dynamically FMC_NAND ECC feature. 00670 * @param Device: Pointer to NAND device instance 00671 * @param Bank: NAND bank number 00672 * @retval HAL status 00673 */ 00674 HAL_StatusTypeDef FMC_NAND_ECC_Disable(FMC_NAND_TypeDef *Device, uint32_t Bank) 00675 { 00676 /* Check the parameters */ 00677 assert_param(IS_FMC_NAND_DEVICE(Device)); 00678 assert_param(IS_FMC_NAND_BANK(Bank)); 00679 00680 /* Disable ECC feature */ 00681 CLEAR_BIT(Device->PCR, FMC_PCR_ECCEN); 00682 00683 return HAL_OK; 00684 } 00685 00686 /** 00687 * @brief Disables dynamically FMC_NAND ECC feature. 00688 * @param Device: Pointer to NAND device instance 00689 * @param ECCval: Pointer to ECC value 00690 * @param Bank: NAND bank number 00691 * @param Timeout: Timeout wait value 00692 * @retval HAL status 00693 */ 00694 HAL_StatusTypeDef FMC_NAND_GetECC(FMC_NAND_TypeDef *Device, uint32_t *ECCval, uint32_t Bank, uint32_t Timeout) 00695 { 00696 uint32_t tickstart = 0; 00697 00698 /* Check the parameters */ 00699 assert_param(IS_FMC_NAND_DEVICE(Device)); 00700 assert_param(IS_FMC_NAND_BANK(Bank)); 00701 00702 /* Get tick */ 00703 tickstart = HAL_GetTick(); 00704 00705 /* Wait until FIFO is empty */ 00706 while(__FMC_NAND_GET_FLAG(Device, Bank, FMC_FLAG_FEMPT) == RESET) 00707 { 00708 /* Check for the Timeout */ 00709 if(Timeout != HAL_MAX_DELAY) 00710 { 00711 if((Timeout == 0)||((HAL_GetTick() - tickstart ) > Timeout)) 00712 { 00713 return HAL_TIMEOUT; 00714 } 00715 } 00716 } 00717 00718 /* Get the ECCR register value */ 00719 *ECCval = (uint32_t)Device->ECCR; 00720 00721 return HAL_OK; 00722 } 00723 00724 /** 00725 * @} 00726 */ 00727 00728 /** 00729 * @} 00730 */ 00731 00732 /** 00733 * @} 00734 */ 00735 00736 /** 00737 * @} 00738 */ 00739 00740 /** 00741 * @} 00742 */ 00743 00744 #endif /* HAL_SRAM_MODULE_ENABLED || HAL_NOR_MODULE_ENABLED || HAL_NAND_MODULE_ENABLED */ 00745 00746 /** 00747 * @} 00748 */ 00749 00750 /************************ (C) COPYRIGHT STMicroelectronics *****END OF FILE****/ 00751
Generated on Tue Jul 12 2022 11:35:16 by
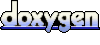