Hal Drivers for L4
Dependents: BSP OneHopeOnePrayer FINAL_AUDIO_RECORD AudioDemo
Fork of STM32L4xx_HAL_Driver by
stm32l4xx_ll_dac.h
00001 /** 00002 ****************************************************************************** 00003 * @file stm32l4xx_ll_dac.h 00004 * @author MCD Application Team 00005 * @version V1.1.0 00006 * @date 16-September-2015 00007 * @brief Header file of DAC LL module. 00008 ****************************************************************************** 00009 * @attention 00010 * 00011 * <h2><center>© COPYRIGHT(c) 2015 STMicroelectronics</center></h2> 00012 * 00013 * Redistribution and use in source and binary forms, with or without modification, 00014 * are permitted provided that the following conditions are met: 00015 * 1. Redistributions of source code must retain the above copyright notice, 00016 * this list of conditions and the following disclaimer. 00017 * 2. Redistributions in binary form must reproduce the above copyright notice, 00018 * this list of conditions and the following disclaimer in the documentation 00019 * and/or other materials provided with the distribution. 00020 * 3. Neither the name of STMicroelectronics nor the names of its contributors 00021 * may be used to endorse or promote products derived from this software 00022 * without specific prior written permission. 00023 * 00024 * THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" 00025 * AND ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE 00026 * IMPLIED WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE 00027 * DISCLAIMED. IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE 00028 * FOR ANY DIRECT, INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL 00029 * DAMAGES (INCLUDING, BUT NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR 00030 * SERVICES; LOSS OF USE, DATA, OR PROFITS; OR BUSINESS INTERRUPTION) HOWEVER 00031 * CAUSED AND ON ANY THEORY OF LIABILITY, WHETHER IN CONTRACT, STRICT LIABILITY, 00032 * OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) ARISING IN ANY WAY OUT OF THE USE 00033 * OF THIS SOFTWARE, EVEN IF ADVISED OF THE POSSIBILITY OF SUCH DAMAGE. 00034 * 00035 ****************************************************************************** 00036 */ 00037 00038 /* Define to prevent recursive inclusion -------------------------------------*/ 00039 #ifndef __STM32L4xx_LL_DAC_H 00040 #define __STM32L4xx_LL_DAC_H 00041 00042 #ifdef __cplusplus 00043 extern "C" { 00044 #endif 00045 00046 /* Includes ------------------------------------------------------------------*/ 00047 #include "stm32l4xx.h" 00048 00049 /** @addtogroup STM32L4xx_LL_Driver 00050 * @{ 00051 */ 00052 00053 #if defined (DAC1) 00054 00055 /** @defgroup DAC_LL DAC 00056 * @{ 00057 */ 00058 00059 /* Private types -------------------------------------------------------------*/ 00060 /* Private variables ---------------------------------------------------------*/ 00061 00062 /* Private constants ---------------------------------------------------------*/ 00063 /** @defgroup DAC_LL_Private_Constants DAC Private Constants 00064 * @{ 00065 */ 00066 00067 /* Internal masks for DAC channels definition */ 00068 /* To select into literal LL_DAC_CHANNEL_x the relevant bits for: */ 00069 /* - channel bits position into registers CR, MCR, CCR, SHHR, SHRR */ 00070 /* - channel bits position into register SWTRIG */ 00071 /* - channel register offset of data holding register */ 00072 /* - channel register offset of data output register */ 00073 /* - channel register offset of sample-and-hold time register */ 00074 00075 #define DAC_CR_CH1_BITOFFSET ((uint32_t) 0) /* Position of channel bits into registers CR, MCR, CCR, SHHR, SHRR of channel 1 */ 00076 #define DAC_CR_CH2_BITOFFSET ((uint32_t)16) /* Position of channel bits into registers CR, MCR, CCR, SHHR, SHRR of channel 2 */ 00077 #define DAC_CR_CHx_BITOFFSET_MASK (DAC_CR_CH1_BITOFFSET | DAC_CR_CH2_BITOFFSET) 00078 00079 #define DAC_SWTR_CH1 (DAC_SWTRIGR_SWTRIG1) /* Channel bit into register SWTRIGR of channel 1. This bit is into area of LL_DAC_CR_CHx_BITOFFSET but excluded by mask DAC_CR_CHx_BITOFFSET_MASK (done to be enable to trig SW start of both DAC channels simultaneously). */ 00080 #define DAC_SWTR_CH2 (DAC_SWTRIGR_SWTRIG2) /* Channel bit into register SWTRIGR of channel 2. This bit is into area of LL_DAC_CR_CHx_BITOFFSET but excluded by mask DAC_CR_CHx_BITOFFSET_MASK (done to be enable to trig SW start of both DAC channels simultaneously). */ 00081 #define DAC_SWTR_CHX_MASK (DAC_SWTR_CH1 | DAC_SWTR_CH2) 00082 00083 #define DAC_REG_DHR12R1_REGOFFSET ((uint32_t)0x00000000) /* Register DHR12Rx channel 1 taken as reference */ 00084 #define DAC_REG_DHR12R2_REGOFFSET ((uint32_t)0x00030000) /* Register offset of DHR12Lx channel 2 versus DHR12Rx channel 1 (shifted left of 16 bits) */ 00085 #define DAC_REG_DHR12L1_REGOFFSET ((uint32_t)0x00100000) /* Register offset of DHR12Lx channel 1 versus DHR12Rx channel 1 (shifted left of 20 bits) */ 00086 #define DAC_REG_DHR12L2_REGOFFSET ((uint32_t)0x00400000) /* Register offset of DHR12Lx channel 2 versus DHR12Rx channel 1 (shifted left of 20 bits) */ 00087 #define DAC_REG_DHR8R1_REGOFFSET ((uint32_t)0x02000000) /* Register offset of DHR8Rx channel 1 versus DHR12Rx channel 1 (shifted left of 24 bits) */ 00088 #define DAC_REG_DHR8R2_REGOFFSET ((uint32_t)0x05000000) /* Register offset of DHR8Rx channel 2 versus DHR12Rx channel 1 (shifted left of 24 bits) */ 00089 #define DAC_REG_DHR12Rx_REGOFFSET_MASK (DAC_REG_DHR12R1_REGOFFSET | DAC_REG_DHR12R2_REGOFFSET) 00090 #define DAC_REG_DHR12Lx_REGOFFSET_MASK (DAC_REG_DHR12L1_REGOFFSET | DAC_REG_DHR12L2_REGOFFSET) 00091 #define DAC_REG_DHR8Rx_REGOFFSET_MASK (DAC_REG_DHR8R1_REGOFFSET | DAC_REG_DHR8R2_REGOFFSET) 00092 #define DAC_REG_DHRx_REGOFFSET_MASK (DAC_REG_DHR12Rx_REGOFFSET_MASK | DAC_REG_DHR12Lx_REGOFFSET_MASK | DAC_REG_DHR8Rx_REGOFFSET_MASK) 00093 00094 #define DAC_REG_DOR1_REGOFFSET ((uint32_t)0x00000000) /* Register DORx channel 1 taken as reference */ 00095 #define DAC_REG_DOR2_REGOFFSET ((uint32_t)0x10000000) /* Register offset of DORx channel 1 versus DORx channel 2 (shifted left of 28 bits) */ 00096 #define DAC_REG_DORx_REGOFFSET_MASK (DAC_REG_DOR1_REGOFFSET | DAC_REG_DOR2_REGOFFSET) 00097 00098 #define DAC_REG_SHSR1_REGOFFSET ((uint32_t)0x00000000) /* Register SHSRx channel 1 taken as reference */ 00099 #define DAC_REG_SHSR2_REGOFFSET ((uint32_t)0x00001000) /* Register offset of SHSRx channel 1 versus SHSRx channel 2 (shifted left of 12 bits) */ 00100 #define DAC_REG_SHSRx_REGOFFSET_MASK (DAC_REG_SHSR1_REGOFFSET | DAC_REG_SHSR2_REGOFFSET) 00101 00102 /* Miscellaneous data */ 00103 #define DAC_DIGITAL_SCALE_12BITS ((uint32_t)4095) /* Full-scale digital value with a resolution of 12 bits (voltage range determined by analog voltage references Vref+ and Vref-, refer to reference manual) */ 00104 00105 /** 00106 * @} 00107 */ 00108 00109 00110 /* Private macros ------------------------------------------------------------*/ 00111 /** @defgroup DAC_LL_Private_Macros DAC Private Macros 00112 * @{ 00113 */ 00114 00115 /** 00116 * @brief Driver macro reserved for internal use: isolate bits with the 00117 * selected mask and shift them to the register LSB 00118 * (shift mask on register position bit 0). 00119 * @param __BITS__ Bits in register 32 bits 00120 * @param __MASK__ Mask in register 32 bits 00121 * @retval Bits in register 32 bits 00122 */ 00123 #define __DAC_MASK_SHIFT(__BITS__, __MASK__) \ 00124 (((__BITS__) & (__MASK__)) >> POSITION_VAL((__MASK__))) 00125 00126 /** 00127 * @brief Driver macro reserved for internal use: set a pointer to 00128 * a register from a register basis from which an offset 00129 * is applied. 00130 * @param __REG__ Register basis from which the offset is applied. 00131 * @param __REG_OFFFSET__ Offset to be applied (unit: number of registers). 00132 * @retval Pointer to register address 00133 */ 00134 #define __DAC_PTR_REG_OFFSET(__REG__, __REG_OFFFSET__) \ 00135 ((uint32_t *)((uint32_t) ((uint32_t)(&(__REG__)) + ((__REG_OFFFSET__) << 2)))) 00136 00137 /** 00138 * @} 00139 */ 00140 00141 00142 /* Exported types ------------------------------------------------------------*/ 00143 /* Exported constants --------------------------------------------------------*/ 00144 /** @defgroup DAC_LL_Exported_Constants DAC Exported Constants 00145 * @{ 00146 */ 00147 00148 /** @defgroup DAC_LL_EC_GET_FLAG DAC flags 00149 * @brief Flags defines which can be used with LL_DAC_ReadReg function 00150 * @{ 00151 */ 00152 #define LL_DAC_SR_CAL_FLAG1 (DAC_SR_CAL_FLAG1) 00153 #define LL_DAC_SR_CAL_FLAG2 (DAC_SR_CAL_FLAG2) 00154 #define LL_DAC_SR_BWST1 (DAC_SR_BWST1) 00155 #define LL_DAC_SR_BWST2 (DAC_SR_BWST2) 00156 #define LL_DAC_SR_DMAUDR1 (DAC_SR_DMAUDR1) 00157 #define LL_DAC_SR_DMAUDR2 (DAC_SR_DMAUDR2) 00158 /** 00159 * @} 00160 */ 00161 00162 /** @defgroup DAC_LL_EC_IT DAC interruptions 00163 * @brief IT defines which can be used with LL_DAC_ReadReg and LL_DAC_WriteReg functions 00164 * @{ 00165 */ 00166 #define LL_DAC_CR_DMAUDRIE1 (DAC_CR_DMAUDRIE1) /*!< DAC channel 1 interruption DMA underrun */ 00167 #define LL_DAC_CR_DMAUDRIE2 (DAC_CR_DMAUDRIE2) /*!< DAC channel 2 interruption DMA underrun */ 00168 /** 00169 * @} 00170 */ 00171 00172 /** @defgroup DAC_LL_EC_CHANNEL DAC channels 00173 * @{ 00174 */ 00175 #define LL_DAC_CHANNEL_1 (DAC_REG_SHSR1_REGOFFSET | DAC_REG_DOR1_REGOFFSET | DAC_REG_DHR12R1_REGOFFSET | DAC_REG_DHR12L1_REGOFFSET | DAC_REG_DHR8R1_REGOFFSET | DAC_CR_CH1_BITOFFSET | DAC_SWTR_CH1) /*!< DAC channel 1 */ 00176 #define LL_DAC_CHANNEL_2 (DAC_REG_SHSR2_REGOFFSET | DAC_REG_DOR2_REGOFFSET | DAC_REG_DHR12R2_REGOFFSET | DAC_REG_DHR12L2_REGOFFSET | DAC_REG_DHR8R2_REGOFFSET | DAC_CR_CH2_BITOFFSET | DAC_SWTR_CH2) /*!< DAC channel 2 */ 00177 /** 00178 * @} 00179 */ 00180 00181 /** @defgroup DAC_LL_EC_MODE DAC operating mode 00182 * @{ 00183 */ 00184 #define LL_DAC_MODE_NORMAL_OPERATION ((uint32_t)0x00000000) /*!< DAC channel in mode normal operation */ 00185 #define LL_DAC_MODE_CALIBRATION (DAC_CR_CEN1) /*!< DAC channel in mode calibration */ 00186 /** 00187 * @} 00188 */ 00189 00190 /** @defgroup DAC_LL_EC_TRIGGER_SOURCE DAC trigger source 00191 * @{ 00192 */ 00193 #define LL_DAC_TRIGGER_TIM2_TRGO (DAC_CR_TSEL1_2 ) /*!< Conversion trigger selected as external trigger from TIM2 TRGO for the selected DAC channel */ 00194 #define LL_DAC_TRIGGER_TIM4_TRGO (DAC_CR_TSEL1_2 | DAC_CR_TSEL1_0) /*!< Conversion trigger selected as external trigger from TIM4 TRGO for the selected DAC channel */ 00195 #define LL_DAC_TRIGGER_TIM5_TRGO ( DAC_CR_TSEL1_1 | DAC_CR_TSEL1_0) /*!< Conversion trigger selected as external trigger from TIM5 TRGO for the selected DAC channel */ 00196 #define LL_DAC_TRIGGER_TIM6_TRGO ((uint32_t)0x00000000) /*!< Conversion trigger selected as external trigger from TIM6 TRGO for the selected DAC channel */ 00197 #define LL_DAC_TRIGGER_TIM7_TRGO ( DAC_CR_TSEL1_1 ) /*!< Conversion trigger selected as external trigger from TIM7 TRGO for the selected DAC channel */ 00198 #define LL_DAC_TRIGGER_TIM8_TRGO ( DAC_CR_TSEL1_0) /*!< Conversion trigger selected as external trigger from TIM8 TRGO for the selected DAC channel */ 00199 #define LL_DAC_TRIGGER_EXT_IT9 (DAC_CR_TSEL1_2 | DAC_CR_TSEL1_1 ) /*!< Conversion trigger selected as external trigger from EXTI Line9 event for the selected DAC channel */ 00200 #define LL_DAC_TRIGGER_SOFTWARE (DAC_CR_TSEL1_2 | DAC_CR_TSEL1_1 | DAC_CR_TSEL1_0) /*!< Conversion trigger selected as internal SW start for the selected DAC channel */ 00201 /** 00202 * @} 00203 */ 00204 00205 /** @defgroup DAC_LL_EC_WAVE_GENERATION_MODE DAC wave generation mode 00206 * @{ 00207 */ 00208 #define LL_DAC_WAVEGENERATION_NONE ((uint32_t)0x00000000) /*!< DAC channel wave generation mode disabled. */ 00209 #define LL_DAC_WAVEGENERATION_NOISE (DAC_CR_WAVE1_0) /*!< DAC channel wave generation mode enabled, set generated noise wave. */ 00210 #define LL_DAC_WAVEGENERATION_TRIANGLE (DAC_CR_WAVE1_1) /*!< DAC channel wave generation mode enabled, set generated triangle wave. */ 00211 /** 00212 * @} 00213 */ 00214 00215 /** @defgroup DAC_LL_EC_NOISE_LFSR_UNMASK_BITS DAC wave generation - Noise LFSR unmask bits 00216 * @{ 00217 */ 00218 #define LL_DAC_NOISE_LFSR_UNMASK_BIT0 ((uint32_t)0x00000000) /*!< Noise wave generation, unmask LFSR bit0, for the selected DAC channel */ 00219 #define LL_DAC_NOISE_LFSR_UNMASK_BITS1_0 ( DAC_CR_MAMP1_0) /*!< Noise wave generation, unmask LFSR bits[1:0], for the selected DAC channel */ 00220 #define LL_DAC_NOISE_LFSR_UNMASK_BITS2_0 ( DAC_CR_MAMP1_1 ) /*!< Noise wave generation, unmask LFSR bits[2:0], for the selected DAC channel */ 00221 #define LL_DAC_NOISE_LFSR_UNMASK_BITS3_0 ( DAC_CR_MAMP1_1 | DAC_CR_MAMP1_0) /*!< Noise wave generation, unmask LFSR bits[3:0], for the selected DAC channel */ 00222 #define LL_DAC_NOISE_LFSR_UNMASK_BITS4_0 ( DAC_CR_MAMP1_2 ) /*!< Noise wave generation, unmask LFSR bits[4:0], for the selected DAC channel */ 00223 #define LL_DAC_NOISE_LFSR_UNMASK_BITS5_0 ( DAC_CR_MAMP1_2 | DAC_CR_MAMP1_0) /*!< Noise wave generation, unmask LFSR bits[5:0], for the selected DAC channel */ 00224 #define LL_DAC_NOISE_LFSR_UNMASK_BITS6_0 ( DAC_CR_MAMP1_2 | DAC_CR_MAMP1_1 ) /*!< Noise wave generation, unmask LFSR bits[6:0], for the selected DAC channel */ 00225 #define LL_DAC_NOISE_LFSR_UNMASK_BITS7_0 ( DAC_CR_MAMP1_2 | DAC_CR_MAMP1_1 | DAC_CR_MAMP1_0) /*!< Noise wave generation, unmask LFSR bits[7:0], for the selected DAC channel */ 00226 #define LL_DAC_NOISE_LFSR_UNMASK_BITS8_0 (DAC_CR_MAMP1_3 ) /*!< Noise wave generation, unmask LFSR bits[8:0], for the selected DAC channel */ 00227 #define LL_DAC_NOISE_LFSR_UNMASK_BITS9_0 (DAC_CR_MAMP1_3 | DAC_CR_MAMP1_0) /*!< Noise wave generation, unmask LFSR bits[9:0], for the selected DAC channel */ 00228 #define LL_DAC_NOISE_LFSR_UNMASK_BITS10_0 (DAC_CR_MAMP1_3 | DAC_CR_MAMP1_1 ) /*!< Noise wave generation, unmask LFSR bits[10:0], for the selected DAC channel */ 00229 #define LL_DAC_NOISE_LFSR_UNMASK_BITS11_0 (DAC_CR_MAMP1_3 | DAC_CR_MAMP1_1 | DAC_CR_MAMP1_0) /*!< Noise wave generation, unmask LFSR bits[11:0], for the selected DAC channel */ 00230 /** 00231 * @} 00232 */ 00233 00234 /** @defgroup DAC_LL_EC_TRIANGLE_AMPLITUDE DAC wave generation - Triangle amplitude 00235 * @{ 00236 */ 00237 #define LL_DAC_TRIANGLE_AMPLITUDE_1 ((uint32_t)0x00000000) /*!< Triangle wave generation, amplitude of 1 LSB of DAC output range, for the selected DAC channel */ 00238 #define LL_DAC_TRIANGLE_AMPLITUDE_3 ( DAC_CR_MAMP1_0) /*!< Triangle wave generation, amplitude of 3 LSB of DAC output range, for the selected DAC channel */ 00239 #define LL_DAC_TRIANGLE_AMPLITUDE_7 ( DAC_CR_MAMP1_1 ) /*!< Triangle wave generation, amplitude of 7 LSB of DAC output range, for the selected DAC channel */ 00240 #define LL_DAC_TRIANGLE_AMPLITUDE_15 ( DAC_CR_MAMP1_1 | DAC_CR_MAMP1_0) /*!< Triangle wave generation, amplitude of 15 LSB of DAC output range, for the selected DAC channel */ 00241 #define LL_DAC_TRIANGLE_AMPLITUDE_31 ( DAC_CR_MAMP1_2 ) /*!< Triangle wave generation, amplitude of 31 LSB of DAC output range, for the selected DAC channel */ 00242 #define LL_DAC_TRIANGLE_AMPLITUDE_63 ( DAC_CR_MAMP1_2 | DAC_CR_MAMP1_0) /*!< Triangle wave generation, amplitude of 63 LSB of DAC output range, for the selected DAC channel */ 00243 #define LL_DAC_TRIANGLE_AMPLITUDE_127 ( DAC_CR_MAMP1_2 | DAC_CR_MAMP1_1 ) /*!< Triangle wave generation, amplitude of 127 LSB of DAC output range, for the selected DAC channel */ 00244 #define LL_DAC_TRIANGLE_AMPLITUDE_255 ( DAC_CR_MAMP1_2 | DAC_CR_MAMP1_1 | DAC_CR_MAMP1_0) /*!< Triangle wave generation, amplitude of 255 LSB of DAC output range, for the selected DAC channel */ 00245 #define LL_DAC_TRIANGLE_AMPLITUDE_511 (DAC_CR_MAMP1_3 ) /*!< Triangle wave generation, amplitude of 512 LSB of DAC output range, for the selected DAC channel */ 00246 #define LL_DAC_TRIANGLE_AMPLITUDE_1023 (DAC_CR_MAMP1_3 | DAC_CR_MAMP1_0) /*!< Triangle wave generation, amplitude of 1023 LSB of DAC output range, for the selected DAC channel */ 00247 #define LL_DAC_TRIANGLE_AMPLITUDE_2047 (DAC_CR_MAMP1_3 | DAC_CR_MAMP1_1 ) /*!< Triangle wave generation, amplitude of 2047 LSB of DAC output range, for the selected DAC channel */ 00248 #define LL_DAC_TRIANGLE_AMPLITUDE_4095 (DAC_CR_MAMP1_3 | DAC_CR_MAMP1_1 | DAC_CR_MAMP1_0) /*!< Triangle wave generation, amplitude of 4095 LSB of DAC output range, for the selected DAC channel */ 00249 /** 00250 * @} 00251 */ 00252 00253 /** @defgroup DAC_LL_EC_OUTPUT_MODE DAC channel output mode 00254 * @{ 00255 */ 00256 #define LL_DAC_OUTPUT_MODE_NORMAL ((uint32_t)0x00000000) /*!< The selected DAC channel output is on normal mode */ 00257 #define LL_DAC_OUTPUT_MODE_SAMPLE_AND_HOLD (DAC_MCR_MODE1_2) /*!< The selected DAC channel output is on sample-and-hold mode */ 00258 /** 00259 * @} 00260 */ 00261 00262 /** @defgroup DAC_LL_EC_OUTPUT_BUFFER DAC channel output buffer 00263 * @{ 00264 */ 00265 #define LL_DAC_OUTPUT_BUFFER_ENABLE ((uint32_t)0x00000000) /*!< The selected DAC channel output is buffered: higher drive current capability, but also higher current consumption */ 00266 #define LL_DAC_OUTPUT_BUFFER_DISABLE (DAC_MCR_MODE1_1) /*!< The selected DAC channel output is not buffered: lower drive current capability, but also lower current consumption */ 00267 /** 00268 * @} 00269 */ 00270 00271 /** @defgroup DAC_LL_EC_OUTPUT_CONNECTION DAC channel output connection 00272 * @{ 00273 */ 00274 #define LL_DAC_CONNECT_GPIO ((uint32_t)0x00000000) /*!< The selected DAC channel output is connected to external pin */ 00275 #define LL_DAC_CONNECT_INTERNAL (DAC_MCR_MODE1_0) /*!< The selected DAC channel output is connected to on-chip peripherals via internal paths. On STM32L4, output connection depends on output mode (normal or sample and hold) and output buffer state. Refer to comments of function @ref LL_DAC_SetOutputConnection(). */ 00276 /** 00277 * @} 00278 */ 00279 00280 /** @defgroup DAC_LL_EC_REGISTERS Registers compliant with specific purpose 00281 * @{ 00282 */ 00283 /* List of DAC registers intended to be used (most commonly) with */ 00284 /* DMA transfer. */ 00285 /* Refer to function @ref LL_DAC_DMA_GetRegAddr(). */ 00286 #define LL_DAC_DMA_REG_DATA_12BITS_RIGHT_ALIGNED DAC_REG_DHR12Rx_REGOFFSET_MASK /*!< DAC channel data holding register 12 bits right aligned */ 00287 #define LL_DAC_DMA_REG_DATA_12BITS_LEFT_ALIGNED DAC_REG_DHR12Lx_REGOFFSET_MASK /*!< DAC channel data holding register 12 bits left aligned */ 00288 #define LL_DAC_DMA_REG_DATA_8BITS_RIGHT_ALIGNED DAC_REG_DHR8Rx_REGOFFSET_MASK /*!< DAC channel data holding register 8 bits right aligned */ 00289 /** 00290 * @} 00291 */ 00292 00293 /** 00294 * @} 00295 */ 00296 00297 /* Exported macro ------------------------------------------------------------*/ 00298 /** @defgroup DAC_LL_Exported_Macros DAC Exported Macros 00299 * @{ 00300 */ 00301 00302 /** @defgroup DAC_LL_EM_WRITE_READ Common write and read registers macros 00303 * @{ 00304 */ 00305 00306 /** 00307 * @brief Write a value in DAC register 00308 * @param __INSTANCE__ DAC Instance 00309 * @param __REG__ Register to be written 00310 * @param __VALUE__ Value to be written in the register 00311 * @retval None 00312 */ 00313 #define LL_DAC_WriteReg(__INSTANCE__, __REG__, __VALUE__) WRITE_REG(__INSTANCE__->__REG__, (__VALUE__)) 00314 00315 /** 00316 * @brief Read a value in DAC register 00317 * @param __INSTANCE__ DAC Instance 00318 * @param __REG__ Register to be read 00319 * @retval Register value 00320 */ 00321 #define LL_DAC_ReadReg(__INSTANCE__, __REG__) READ_REG(__INSTANCE__->__REG__) 00322 00323 /** 00324 * @} 00325 */ 00326 00327 /** @defgroup DAC_LL_EM_HELPER_MACRO DAC helper macro 00328 * @{ 00329 */ 00330 00331 /** 00332 * @brief Helper macro to get DAC channel number in decimal format 00333 * from literals LL_DAC_CHANNEL_x. 00334 * Example: 00335 * __LL_DAC_CHANNEL_TO_DECIMAL_NB(LL_DAC_CHANNEL_1) 00336 * will return decimal number "1". 00337 * @note The input can be a value from functions where a channel 00338 * number is returned. 00339 * @param __CHANNEL__ This parameter can be one of the following values: 00340 * @arg @ref LL_DAC_CHANNEL_1 00341 * @arg @ref LL_DAC_CHANNEL_2 (1) 00342 * (1) On this STM32 family, parameter not available on all devices. 00343 * Refer to device datasheet for channels availability. 00344 * @retval 1...2 (value "2" depending on DAC channel 2 availability) 00345 */ 00346 #define __LL_DAC_CHANNEL_TO_DECIMAL_NB(__CHANNEL__) \ 00347 ((__CHANNEL__) & DAC_SWTR_CHX_MASK) 00348 00349 /** 00350 * @brief Helper macro to get DAC channel in literal format LL_DAC_CHANNEL_x 00351 * from number in decimal format. 00352 * Example: 00353 * __LL_DAC_DECIMAL_NB_TO_CHANNEL(1) 00354 * will return a data equivalent to "LL_DAC_CHANNEL_1". 00355 * @param __DECIMAL_NB__ 1...2 (value "2" depending on DAC channel 2 availability) 00356 * @retval Returned value can be one of the following values: 00357 * @arg @ref LL_DAC_CHANNEL_1 00358 * @arg @ref LL_DAC_CHANNEL_2 (1) 00359 * (1) On this STM32 family, parameter not available on all devices. 00360 * Refer to device datasheet for channels availability. 00361 */ 00362 #define __LL_DAC_DECIMAL_NB_TO_CHANNEL(__DECIMAL_NB__) \ 00363 (((__DECIMAL_NB__) == 1) \ 00364 ? ( \ 00365 LL_DAC_CHANNEL_1 \ 00366 ) \ 00367 : \ 00368 ( \ 00369 LL_DAC_CHANNEL_2 \ 00370 ) \ 00371 ) 00372 00373 /** 00374 * @brief Helper macro to calculate the DAC conversion data (unit: digital 00375 * value) corresponding to a voltage (unit: mVolt). 00376 * @note DAC conversion data is set with a resolution of 12bits 00377 * (full scale digital value 4095), right aligned. 00378 * The data is formatted to be used with function 00379 * @ref LL_DAC_ConvertData12RightAligned(). 00380 * @note Analog reference voltage (Vref+) must be either known from 00381 * user board environment or can be calculated using ADC measurement 00382 * and ADC helper macro "__LL_ADC_CALC_VREF_VOLTAGE()". 00383 * @param __VREF_VOLTAGE__ Analog reference voltage (unit: mV) 00384 * @param __DAC_VOLTAGE__ Voltage to be generated by DAC channel 00385 * (unit: mVolt). 00386 * @retval DAC conversion data (unit: digital value) 00387 */ 00388 #define __LL_DAC_CALC_DATA_VOLTAGE(__VREF_VOLTAGE__, __DAC_VOLTAGE__) \ 00389 ((__DAC_VOLTAGE__) * DAC_DIGITAL_SCALE_12BITS / (__VREF_VOLTAGE__)) 00390 00391 /** 00392 * @} 00393 */ 00394 00395 /** 00396 * @} 00397 */ 00398 00399 00400 /* Exported functions --------------------------------------------------------*/ 00401 /** @defgroup DAC_LL_Exported_Functions DAC Exported Functions 00402 * @{ 00403 */ 00404 /** @defgroup DAC_LL_EF_Configuration Configuration of DAC channels 00405 * @{ 00406 */ 00407 00408 /** 00409 * @brief Set the mode for the selected DAC channel: calibration or normal mode. 00410 * @rmtoll CR CEN1 LL_DAC_SetMode\n 00411 * CR CEN2 LL_DAC_SetMode 00412 * @param DACx DAC instance 00413 * @param DAC_Channel This parameter can be one of the following values: 00414 * @arg @ref LL_DAC_CHANNEL_1 00415 * @arg @ref LL_DAC_CHANNEL_2 (1) 00416 * (1) On this STM32 family, parameter not available on all devices. 00417 * Refer to device datasheet for channels availability. 00418 * @param ChannelMode This parameter can be one of the following values: 00419 * @arg @ref LL_DAC_MODE_NORMAL_OPERATION 00420 * @arg @ref LL_DAC_MODE_CALIBRATION 00421 * @retval None 00422 */ 00423 __STATIC_INLINE void LL_DAC_SetMode(DAC_TypeDef *DACx, uint32_t DAC_Channel, uint32_t ChannelMode) 00424 { 00425 MODIFY_REG(DACx->CR, 00426 DAC_CR_CEN1 << (DAC_Channel & DAC_CR_CHx_BITOFFSET_MASK), 00427 ChannelMode << (DAC_Channel & DAC_CR_CHx_BITOFFSET_MASK)); 00428 } 00429 00430 /** 00431 * @brief Get the mode for the selected DAC channel: calibration or normal mode. 00432 * @rmtoll CR CEN1 LL_DAC_GetMode\n 00433 * CR CEN2 LL_DAC_GetMode 00434 * @param DACx DAC instance 00435 * @param DAC_Channel This parameter can be one of the following values: 00436 * @arg @ref LL_DAC_CHANNEL_1 00437 * @arg @ref LL_DAC_CHANNEL_2 (1) 00438 * (1) On this STM32 family, parameter not available on all devices. 00439 * Refer to device datasheet for channels availability. 00440 * @retval Returned value can be one of the following values: 00441 * @arg @ref LL_DAC_MODE_NORMAL_OPERATION 00442 * @arg @ref LL_DAC_MODE_CALIBRATION 00443 */ 00444 __STATIC_INLINE uint32_t LL_DAC_GetMode(DAC_TypeDef *DACx, uint32_t DAC_Channel) 00445 { 00446 return (uint32_t)(READ_BIT(DACx->CR, DAC_CR_CEN1 << (DAC_Channel & DAC_CR_CHx_BITOFFSET_MASK)) 00447 >> (DAC_Channel & DAC_CR_CHx_BITOFFSET_MASK) 00448 ); 00449 } 00450 00451 /** 00452 * @brief Set the offset trimming value for the selected DAC channel. 00453 * Trimming has an impact when output buffer is enabled 00454 * and is intended to replace factory calibration default values. 00455 * @rmtoll CCR OTRIM1 LL_DAC_SetTrimmingValue\n 00456 * CCR OTRIM2 LL_DAC_SetTrimmingValue 00457 * @param DACx DAC instance 00458 * @param DAC_Channel This parameter can be one of the following values: 00459 * @arg @ref LL_DAC_CHANNEL_1 00460 * @arg @ref LL_DAC_CHANNEL_2 (1) 00461 * (1) On this STM32 family, parameter not available on all devices. 00462 * Refer to device datasheet for channels availability. 00463 * @param TrimmingValue 0x00...0x1F 00464 * @retval None 00465 */ 00466 __STATIC_INLINE void LL_DAC_SetTrimmingValue(DAC_TypeDef *DACx, uint32_t DAC_Channel, uint32_t TrimmingValue) 00467 { 00468 MODIFY_REG(DACx->CCR, 00469 DAC_CCR_OTRIM1 << (DAC_Channel & DAC_CR_CHx_BITOFFSET_MASK), 00470 TrimmingValue << (DAC_Channel & DAC_CR_CHx_BITOFFSET_MASK)); 00471 } 00472 00473 /** 00474 * @brief Get the offset trimming value for the selected DAC channel. 00475 * Trimming has an impact when output buffer is enabled 00476 * and is intended to replace factory calibration default values. 00477 * @rmtoll CCR OTRIM1 LL_DAC_GetTrimmingValue\n 00478 * CCR OTRIM2 LL_DAC_GetTrimmingValue 00479 * @param DACx DAC instance 00480 * @param DAC_Channel This parameter can be one of the following values: 00481 * @arg @ref LL_DAC_CHANNEL_1 00482 * @arg @ref LL_DAC_CHANNEL_2 (1) 00483 * (1) On this STM32 family, parameter not available on all devices. 00484 * Refer to device datasheet for channels availability. 00485 * @retval TrimmingValue 0x00...0x1F 00486 */ 00487 __STATIC_INLINE uint32_t LL_DAC_GetTrimmingValue(DAC_TypeDef *DACx, uint32_t DAC_Channel) 00488 { 00489 return (uint32_t)(READ_BIT(DACx->CCR, DAC_CCR_OTRIM1 << (DAC_Channel & DAC_CR_CHx_BITOFFSET_MASK)) 00490 >> (DAC_Channel & DAC_CR_CHx_BITOFFSET_MASK) 00491 ); 00492 } 00493 00494 /** 00495 * @brief Set the conversion trigger source for the selected DAC channel. 00496 * @note For conversion trigger source to be effective, DAC trigger 00497 * must be enabled using function @ref LL_DAC_EnableTrigger(). 00498 * @note To set conversion trigger source, DAC channel must be disabled. 00499 * Otherwise, the setting is discarded. 00500 * @rmtoll CR TSEL1 LL_DAC_SetTriggerSource\n 00501 * CR TSEL2 LL_DAC_SetTriggerSource 00502 * @param DACx DAC instance 00503 * @param DAC_Channel This parameter can be one of the following values: 00504 * @arg @ref LL_DAC_CHANNEL_1 00505 * @arg @ref LL_DAC_CHANNEL_2 (1) 00506 * (1) On this STM32 family, parameter not available on all devices. 00507 * Refer to device datasheet for channels availability. 00508 * @param DAC_Trigger This parameter can be one of the following values: 00509 * @arg @ref LL_DAC_TRIGGER_TIM2_TRGO 00510 * @arg @ref LL_DAC_TRIGGER_TIM4_TRGO 00511 * @arg @ref LL_DAC_TRIGGER_TIM5_TRGO 00512 * @arg @ref LL_DAC_TRIGGER_TIM6_TRGO 00513 * @arg @ref LL_DAC_TRIGGER_TIM7_TRGO 00514 * @arg @ref LL_DAC_TRIGGER_TIM8_TRGO 00515 * @arg @ref LL_DAC_TRIGGER_EXT_IT9 00516 * @arg @ref LL_DAC_TRIGGER_SOFTWARE 00517 * @retval None 00518 */ 00519 __STATIC_INLINE void LL_DAC_SetTriggerSource(DAC_TypeDef *DACx, uint32_t DAC_Channel, uint32_t DAC_Trigger) 00520 { 00521 MODIFY_REG(DACx->CR, 00522 DAC_CR_TSEL1 << (DAC_Channel & DAC_CR_CHx_BITOFFSET_MASK), 00523 DAC_Trigger << (DAC_Channel & DAC_CR_CHx_BITOFFSET_MASK)); 00524 } 00525 00526 /** 00527 * @brief Get the conversion trigger source for the selected DAC channel. 00528 * @note For conversion trigger source to be effective, DAC trigger 00529 * must be enabled using function @ref LL_DAC_EnableTrigger(). 00530 * @rmtoll CR TSEL1 LL_DAC_GetTriggerSource\n 00531 * CR TSEL2 LL_DAC_GetTriggerSource 00532 * @param DACx DAC instance 00533 * @param DAC_Channel This parameter can be one of the following values: 00534 * @arg @ref LL_DAC_CHANNEL_1 00535 * @arg @ref LL_DAC_CHANNEL_2 (1) 00536 * (1) On this STM32 family, parameter not available on all devices. 00537 * Refer to device datasheet for channels availability. 00538 * @retval Returned value can be one of the following values: 00539 * @arg @ref LL_DAC_TRIGGER_TIM2_TRGO 00540 * @arg @ref LL_DAC_TRIGGER_TIM4_TRGO 00541 * @arg @ref LL_DAC_TRIGGER_TIM5_TRGO 00542 * @arg @ref LL_DAC_TRIGGER_TIM6_TRGO 00543 * @arg @ref LL_DAC_TRIGGER_TIM7_TRGO 00544 * @arg @ref LL_DAC_TRIGGER_TIM8_TRGO 00545 * @arg @ref LL_DAC_TRIGGER_EXT_IT9 00546 * @arg @ref LL_DAC_TRIGGER_SOFTWARE 00547 */ 00548 __STATIC_INLINE uint32_t LL_DAC_GetTriggerSource(DAC_TypeDef *DACx, uint32_t DAC_Channel) 00549 { 00550 return (uint32_t)(READ_BIT(DACx->CR, DAC_CR_TSEL1 << (DAC_Channel & DAC_CR_CHx_BITOFFSET_MASK)) 00551 >> (DAC_Channel & DAC_CR_CHx_BITOFFSET_MASK) 00552 ); 00553 } 00554 00555 /** 00556 * @brief Set the wave generation mode for the selected DAC channel. 00557 * @rmtoll CR WAVE1 LL_DAC_SetWaveMode\n 00558 * CR WAVE2 LL_DAC_SetWaveMode 00559 * @param DACx DAC instance 00560 * @param DAC_Channel This parameter can be one of the following values: 00561 * @arg @ref LL_DAC_CHANNEL_1 00562 * @arg @ref LL_DAC_CHANNEL_2 (1) 00563 * (1) On this STM32 family, parameter not available on all devices. 00564 * Refer to device datasheet for channels availability. 00565 * @param WaveMode This parameter can be one of the following values: 00566 * @arg @ref LL_DAC_WAVEGENERATION_NONE 00567 * @arg @ref LL_DAC_WAVEGENERATION_NOISE 00568 * @arg @ref LL_DAC_WAVEGENERATION_TRIANGLE 00569 * @retval None 00570 */ 00571 __STATIC_INLINE void LL_DAC_SetWaveMode(DAC_TypeDef *DACx, uint32_t DAC_Channel, uint32_t WaveMode) 00572 { 00573 MODIFY_REG(DACx->CR, 00574 DAC_CR_WAVE1 << (DAC_Channel & DAC_CR_CHx_BITOFFSET_MASK), 00575 WaveMode << (DAC_Channel & DAC_CR_CHx_BITOFFSET_MASK)); 00576 } 00577 00578 /** 00579 * @brief Get the wave generation mode for the selected DAC channel. 00580 * @rmtoll CR WAVE1 LL_DAC_GetWaveMode\n 00581 * CR WAVE2 LL_DAC_GetWaveMode 00582 * @param DACx DAC instance 00583 * @param DAC_Channel This parameter can be one of the following values: 00584 * @arg @ref LL_DAC_CHANNEL_1 00585 * @arg @ref LL_DAC_CHANNEL_2 (1) 00586 * (1) On this STM32 family, parameter not available on all devices. 00587 * Refer to device datasheet for channels availability. 00588 * @retval Returned value can be one of the following values: 00589 * @arg @ref LL_DAC_WAVEGENERATION_NONE 00590 * @arg @ref LL_DAC_WAVEGENERATION_NOISE 00591 * @arg @ref LL_DAC_WAVEGENERATION_TRIANGLE 00592 */ 00593 __STATIC_INLINE uint32_t LL_DAC_GetWaveMode(DAC_TypeDef *DACx, uint32_t DAC_Channel) 00594 { 00595 return (uint32_t)(READ_BIT(DACx->CR, DAC_CR_WAVE1 << (DAC_Channel & DAC_CR_CHx_BITOFFSET_MASK)) 00596 >> (DAC_Channel & DAC_CR_CHx_BITOFFSET_MASK) 00597 ); 00598 } 00599 00600 /** 00601 * @brief Set the noise generation for the selected DAC channel: 00602 * Noise mode and parameters LFSR (linear feedback shift register). 00603 * @note For wave generation to be effective, DAC channel wave generation 00604 * mode must be enabled using function @ref LL_DAC_SetWaveMode(). 00605 * @note This setting can be set when the selected DAC channel is disabled 00606 * (otherwise, the setting operation is ignored). 00607 * @rmtoll CR MAMP1 LL_DAC_SetWaveNoiseLFSR\n 00608 * CR MAMP2 LL_DAC_SetWaveNoiseLFSR 00609 * @param DACx DAC instance 00610 * @param DAC_Channel This parameter can be one of the following values: 00611 * @arg @ref LL_DAC_CHANNEL_1 00612 * @arg @ref LL_DAC_CHANNEL_2 (1) 00613 * (1) On this STM32 family, parameter not available on all devices. 00614 * Refer to device datasheet for channels availability. 00615 * @param NoiseLFSRMask This parameter can be one of the following values: 00616 * @arg @ref LL_DAC_NOISE_LFSR_UNMASK_BIT0 00617 * @arg @ref LL_DAC_NOISE_LFSR_UNMASK_BITS1_0 00618 * @arg @ref LL_DAC_NOISE_LFSR_UNMASK_BITS2_0 00619 * @arg @ref LL_DAC_NOISE_LFSR_UNMASK_BITS3_0 00620 * @arg @ref LL_DAC_NOISE_LFSR_UNMASK_BITS4_0 00621 * @arg @ref LL_DAC_NOISE_LFSR_UNMASK_BITS5_0 00622 * @arg @ref LL_DAC_NOISE_LFSR_UNMASK_BITS6_0 00623 * @arg @ref LL_DAC_NOISE_LFSR_UNMASK_BITS7_0 00624 * @arg @ref LL_DAC_NOISE_LFSR_UNMASK_BITS8_0 00625 * @arg @ref LL_DAC_NOISE_LFSR_UNMASK_BITS9_0 00626 * @arg @ref LL_DAC_NOISE_LFSR_UNMASK_BITS10_0 00627 * @arg @ref LL_DAC_NOISE_LFSR_UNMASK_BITS11_0 00628 * @retval None 00629 */ 00630 __STATIC_INLINE void LL_DAC_SetWaveNoiseLFSR(DAC_TypeDef *DACx, uint32_t DAC_Channel, uint32_t NoiseLFSRMask) 00631 { 00632 MODIFY_REG(DACx->CR, 00633 DAC_CR_MAMP1 << (DAC_Channel & DAC_CR_CHx_BITOFFSET_MASK), 00634 NoiseLFSRMask << (DAC_Channel & DAC_CR_CHx_BITOFFSET_MASK)); 00635 } 00636 00637 /** 00638 * @brief Set the noise generation for the selected DAC channel: 00639 * Noise mode and parameters LFSR (linear feedback shift register). 00640 * @rmtoll CR MAMP1 LL_DAC_GetWaveNoiseLFSR\n 00641 * CR MAMP2 LL_DAC_GetWaveNoiseLFSR 00642 * @param DACx DAC instance 00643 * @param DAC_Channel This parameter can be one of the following values: 00644 * @arg @ref LL_DAC_CHANNEL_1 00645 * @arg @ref LL_DAC_CHANNEL_2 (1) 00646 * (1) On this STM32 family, parameter not available on all devices. 00647 * Refer to device datasheet for channels availability. 00648 * @retval Returned value can be one of the following values: 00649 * @arg @ref LL_DAC_NOISE_LFSR_UNMASK_BIT0 00650 * @arg @ref LL_DAC_NOISE_LFSR_UNMASK_BITS1_0 00651 * @arg @ref LL_DAC_NOISE_LFSR_UNMASK_BITS2_0 00652 * @arg @ref LL_DAC_NOISE_LFSR_UNMASK_BITS3_0 00653 * @arg @ref LL_DAC_NOISE_LFSR_UNMASK_BITS4_0 00654 * @arg @ref LL_DAC_NOISE_LFSR_UNMASK_BITS5_0 00655 * @arg @ref LL_DAC_NOISE_LFSR_UNMASK_BITS6_0 00656 * @arg @ref LL_DAC_NOISE_LFSR_UNMASK_BITS7_0 00657 * @arg @ref LL_DAC_NOISE_LFSR_UNMASK_BITS8_0 00658 * @arg @ref LL_DAC_NOISE_LFSR_UNMASK_BITS9_0 00659 * @arg @ref LL_DAC_NOISE_LFSR_UNMASK_BITS10_0 00660 * @arg @ref LL_DAC_NOISE_LFSR_UNMASK_BITS11_0 00661 */ 00662 __STATIC_INLINE uint32_t LL_DAC_GetWaveNoiseLFSR(DAC_TypeDef *DACx, uint32_t DAC_Channel) 00663 { 00664 return (uint32_t)(READ_BIT(DACx->CR, DAC_CR_MAMP1 << (DAC_Channel & DAC_CR_CHx_BITOFFSET_MASK)) 00665 >> (DAC_Channel & DAC_CR_CHx_BITOFFSET_MASK) 00666 ); 00667 } 00668 00669 /** 00670 * @brief Set the triangle generation for the selected DAC channel: 00671 * triangle mode and amplitude. 00672 * @note For wave generation to be effective, DAC channel wave generation 00673 * mode must be enabled using function @ref LL_DAC_SetWaveMode(). 00674 * @note This setting can be set when the selected DAC channel is disabled 00675 * (otherwise, the setting operation is ignored). 00676 * @rmtoll CR MAMP1 LL_DAC_SetWaveTriangleAmplitude\n 00677 * CR MAMP2 LL_DAC_SetWaveTriangleAmplitude 00678 * @param DACx DAC instance 00679 * @param DAC_Channel This parameter can be one of the following values: 00680 * @arg @ref LL_DAC_CHANNEL_1 00681 * @arg @ref LL_DAC_CHANNEL_2 (1) 00682 * (1) On this STM32 family, parameter not available on all devices. 00683 * Refer to device datasheet for channels availability. 00684 * @param TriangleAmplitude This parameter can be one of the following values: 00685 * @arg @ref LL_DAC_TRIANGLE_AMPLITUDE_1 00686 * @arg @ref LL_DAC_TRIANGLE_AMPLITUDE_3 00687 * @arg @ref LL_DAC_TRIANGLE_AMPLITUDE_7 00688 * @arg @ref LL_DAC_TRIANGLE_AMPLITUDE_15 00689 * @arg @ref LL_DAC_TRIANGLE_AMPLITUDE_31 00690 * @arg @ref LL_DAC_TRIANGLE_AMPLITUDE_63 00691 * @arg @ref LL_DAC_TRIANGLE_AMPLITUDE_127 00692 * @arg @ref LL_DAC_TRIANGLE_AMPLITUDE_255 00693 * @arg @ref LL_DAC_TRIANGLE_AMPLITUDE_511 00694 * @arg @ref LL_DAC_TRIANGLE_AMPLITUDE_1023 00695 * @arg @ref LL_DAC_TRIANGLE_AMPLITUDE_2047 00696 * @arg @ref LL_DAC_TRIANGLE_AMPLITUDE_4095 00697 * @retval None 00698 */ 00699 __STATIC_INLINE void LL_DAC_SetWaveTriangleAmplitude(DAC_TypeDef *DACx, uint32_t DAC_Channel, uint32_t TriangleAmplitude) 00700 { 00701 MODIFY_REG(DACx->CR, 00702 DAC_CR_MAMP1 << (DAC_Channel & DAC_CR_CHx_BITOFFSET_MASK), 00703 TriangleAmplitude << (DAC_Channel & DAC_CR_CHx_BITOFFSET_MASK)); 00704 } 00705 00706 /** 00707 * @brief Set the triangle generation for the selected DAC channel: 00708 * triangle mode and amplitude. 00709 * @rmtoll CR MAMP1 LL_DAC_GetWaveTriangleAmplitude\n 00710 * CR MAMP2 LL_DAC_GetWaveTriangleAmplitude 00711 * @param DACx DAC instance 00712 * @param DAC_Channel This parameter can be one of the following values: 00713 * @arg @ref LL_DAC_CHANNEL_1 00714 * @arg @ref LL_DAC_CHANNEL_2 (1) 00715 * (1) On this STM32 family, parameter not available on all devices. 00716 * Refer to device datasheet for channels availability. 00717 * @retval Returned value can be one of the following values: 00718 * @arg @ref LL_DAC_TRIANGLE_AMPLITUDE_1 00719 * @arg @ref LL_DAC_TRIANGLE_AMPLITUDE_3 00720 * @arg @ref LL_DAC_TRIANGLE_AMPLITUDE_7 00721 * @arg @ref LL_DAC_TRIANGLE_AMPLITUDE_15 00722 * @arg @ref LL_DAC_TRIANGLE_AMPLITUDE_31 00723 * @arg @ref LL_DAC_TRIANGLE_AMPLITUDE_63 00724 * @arg @ref LL_DAC_TRIANGLE_AMPLITUDE_127 00725 * @arg @ref LL_DAC_TRIANGLE_AMPLITUDE_255 00726 * @arg @ref LL_DAC_TRIANGLE_AMPLITUDE_511 00727 * @arg @ref LL_DAC_TRIANGLE_AMPLITUDE_1023 00728 * @arg @ref LL_DAC_TRIANGLE_AMPLITUDE_2047 00729 * @arg @ref LL_DAC_TRIANGLE_AMPLITUDE_4095 00730 */ 00731 __STATIC_INLINE uint32_t LL_DAC_GetWaveTriangleAmplitude(DAC_TypeDef *DACx, uint32_t DAC_Channel) 00732 { 00733 return (uint32_t)(READ_BIT(DACx->CR, DAC_CR_MAMP1 << (DAC_Channel & DAC_CR_CHx_BITOFFSET_MASK)) 00734 >> (DAC_Channel & DAC_CR_CHx_BITOFFSET_MASK) 00735 ); 00736 } 00737 00738 /** 00739 * @brief Set the output for the selected DAC channel: 00740 * * mode normal or sample-and-hold 00741 * * buffer 00742 * * connection to GPIO or internal path. 00743 * @note these settings can also be set individually using 00744 * dedicated functions: 00745 * - @ref LL_DAC_SetOutputBuffer() 00746 * - @ref LL_DAC_SetOutputMode() 00747 * - @ref LL_DAC_SetOutputConnection() 00748 * @note On this STM32 family, output connection depends on output mode (normal or 00749 * sample and hold) and output buffer state. 00750 * - if output connection is set to internal path and output buffer 00751 * is enabled (whatever output mode): 00752 * output connection is also connected to GPIO pin 00753 * (both connections to GPIO pin and internal path). 00754 * - if output connection is set to GPIO pin, output buffer 00755 * is disabled, output mode set to sample and hold: 00756 * output connection is also connected to internal path 00757 * (both connections to GPIO pin and internal path). 00758 * @rmtoll CR MODE1 LL_DAC_ConfigOutput\n 00759 * CR MODE2 LL_DAC_ConfigOutput 00760 * @param DACx DAC instance 00761 * @param DAC_Channel This parameter can be one of the following values: 00762 * @arg @ref LL_DAC_CHANNEL_1 00763 * @arg @ref LL_DAC_CHANNEL_2 (1) 00764 * (1) On this STM32 family, parameter not available on all devices. 00765 * Refer to device datasheet for channels availability. 00766 * @param OutputMode This parameter can be one of the following values: 00767 * @arg @ref LL_DAC_OUTPUT_MODE_NORMAL 00768 * @arg @ref LL_DAC_OUTPUT_MODE_SAMPLE_AND_HOLD 00769 * @param OutputBuffer This parameter can be one of the following values: 00770 * @arg @ref LL_DAC_OUTPUT_BUFFER_ENABLE 00771 * @arg @ref LL_DAC_OUTPUT_BUFFER_DISABLE 00772 * @param OutputConnection This parameter can be one of the following values: 00773 * @arg @ref LL_DAC_CONNECT_GPIO 00774 * @arg @ref LL_DAC_CONNECT_INTERNAL 00775 * @retval None 00776 */ 00777 __STATIC_INLINE void LL_DAC_ConfigOutput(DAC_TypeDef *DACx, uint32_t DAC_Channel, uint32_t OutputMode, uint32_t OutputBuffer, uint32_t OutputConnection) 00778 { 00779 MODIFY_REG(DACx->MCR, 00780 (DAC_MCR_MODE1_2 | DAC_MCR_MODE1_1 | DAC_MCR_MODE1_0) << (DAC_Channel & DAC_CR_CHx_BITOFFSET_MASK), 00781 (OutputMode | OutputBuffer | OutputConnection) << (DAC_Channel & DAC_CR_CHx_BITOFFSET_MASK)); 00782 } 00783 00784 /** 00785 * @brief Set the output mode normal or sample-and-hold for the selected DAC channel. 00786 * @rmtoll CR MODE1 LL_DAC_SetOutputMode\n 00787 * CR MODE2 LL_DAC_SetOutputMode 00788 * @param DACx DAC instance 00789 * @param DAC_Channel This parameter can be one of the following values: 00790 * @arg @ref LL_DAC_CHANNEL_1 00791 * @arg @ref LL_DAC_CHANNEL_2 (1) 00792 * (1) On this STM32 family, parameter not available on all devices. 00793 * Refer to device datasheet for channels availability. 00794 * @param OutputMode This parameter can be one of the following values: 00795 * @arg @ref LL_DAC_OUTPUT_MODE_NORMAL 00796 * @arg @ref LL_DAC_OUTPUT_MODE_SAMPLE_AND_HOLD 00797 * @retval None 00798 */ 00799 __STATIC_INLINE void LL_DAC_SetOutputMode(DAC_TypeDef *DACx, uint32_t DAC_Channel, uint32_t OutputMode) 00800 { 00801 MODIFY_REG(DACx->MCR, 00802 DAC_MCR_MODE1_2 << (DAC_Channel & DAC_CR_CHx_BITOFFSET_MASK), 00803 OutputMode << (DAC_Channel & DAC_CR_CHx_BITOFFSET_MASK)); 00804 } 00805 00806 /** 00807 * @brief Get the output mode normal or sample-and-hold for the selected DAC channel. 00808 * @rmtoll CR MODE1 LL_DAC_GetOutputMode\n 00809 * CR MODE2 LL_DAC_GetOutputMode 00810 * @param DACx DAC instance 00811 * @param DAC_Channel This parameter can be one of the following values: 00812 * @arg @ref LL_DAC_CHANNEL_1 00813 * @arg @ref LL_DAC_CHANNEL_2 (1) 00814 * (1) On this STM32 family, parameter not available on all devices. 00815 * Refer to device datasheet for channels availability. 00816 * @retval Returned value can be one of the following values: 00817 * @arg @ref LL_DAC_OUTPUT_MODE_NORMAL 00818 * @arg @ref LL_DAC_OUTPUT_MODE_SAMPLE_AND_HOLD 00819 */ 00820 __STATIC_INLINE uint32_t LL_DAC_GetOutputMode(DAC_TypeDef *DACx, uint32_t DAC_Channel) 00821 { 00822 return (uint32_t)(READ_BIT(DACx->MCR, DAC_MCR_MODE1_2 << (DAC_Channel & DAC_CR_CHx_BITOFFSET_MASK)) 00823 >> (DAC_Channel & DAC_CR_CHx_BITOFFSET_MASK) 00824 ); 00825 } 00826 00827 /** 00828 * @brief Set the output buffer for the selected DAC channel. 00829 * @note On this STM32 family, when buffer is enabled, its offset can be 00830 * trimmed: factory calibration default values can be 00831 * replaced by user trimming values, using function 00832 * @ref LL_DAC_SetTrimmingValue(). 00833 * @rmtoll CR MODE1 LL_DAC_SetOutputBuffer\n 00834 * CR MODE2 LL_DAC_SetOutputBuffer 00835 * @param DACx DAC instance 00836 * @param DAC_Channel This parameter can be one of the following values: 00837 * @arg @ref LL_DAC_CHANNEL_1 00838 * @arg @ref LL_DAC_CHANNEL_2 (1) 00839 * (1) On this STM32 family, parameter not available on all devices. 00840 * Refer to device datasheet for channels availability. 00841 * @param OutputBuffer This parameter can be one of the following values: 00842 * @arg @ref LL_DAC_OUTPUT_BUFFER_ENABLE 00843 * @arg @ref LL_DAC_OUTPUT_BUFFER_DISABLE 00844 * @retval None 00845 */ 00846 __STATIC_INLINE void LL_DAC_SetOutputBuffer(DAC_TypeDef *DACx, uint32_t DAC_Channel, uint32_t OutputBuffer) 00847 { 00848 MODIFY_REG(DACx->MCR, 00849 DAC_MCR_MODE1_1 << (DAC_Channel & DAC_CR_CHx_BITOFFSET_MASK), 00850 OutputBuffer << (DAC_Channel & DAC_CR_CHx_BITOFFSET_MASK)); 00851 } 00852 00853 /** 00854 * @brief Get the output buffer state for the selected DAC channel. 00855 * @rmtoll CR MODE1 LL_DAC_GetOutputBuffer\n 00856 * CR MODE2 LL_DAC_GetOutputBuffer 00857 * @param DACx DAC instance 00858 * @param DAC_Channel This parameter can be one of the following values: 00859 * @arg @ref LL_DAC_CHANNEL_1 00860 * @arg @ref LL_DAC_CHANNEL_2 (1) 00861 * (1) On this STM32 family, parameter not available on all devices. 00862 * Refer to device datasheet for channels availability. 00863 * @retval Returned value can be one of the following values: 00864 * @arg @ref LL_DAC_OUTPUT_BUFFER_ENABLE 00865 * @arg @ref LL_DAC_OUTPUT_BUFFER_DISABLE 00866 */ 00867 __STATIC_INLINE uint32_t LL_DAC_GetOutputBuffer(DAC_TypeDef *DACx, uint32_t DAC_Channel) 00868 { 00869 return (uint32_t)(READ_BIT(DACx->MCR, DAC_MCR_MODE1_1 << (DAC_Channel & DAC_CR_CHx_BITOFFSET_MASK)) 00870 >> (DAC_Channel & DAC_CR_CHx_BITOFFSET_MASK) 00871 ); 00872 } 00873 00874 /** 00875 * @brief Set the output connection for the selected DAC channel. 00876 * @note On this STM32 family, output connection depends on output mode (normal or 00877 * sample and hold) and output buffer state. 00878 * - if output connection is set to internal path and output buffer 00879 * is enabled (whatever output mode): 00880 * output connection is also connected to GPIO pin 00881 * (both connections to GPIO pin and internal path). 00882 * - if output connection is set to GPIO pin, output buffer 00883 * is disabled, output mode set to sample and hold: 00884 * output connection is also connected to internal path 00885 * (both connections to GPIO pin and internal path). 00886 * @rmtoll CR MODE1 LL_DAC_SetOutputConnection\n 00887 * CR MODE2 LL_DAC_SetOutputConnection 00888 * @param DACx DAC instance 00889 * @param DAC_Channel This parameter can be one of the following values: 00890 * @arg @ref LL_DAC_CHANNEL_1 00891 * @arg @ref LL_DAC_CHANNEL_2 (1) 00892 * (1) On this STM32 family, parameter not available on all devices. 00893 * Refer to device datasheet for channels availability. 00894 * @param OutputConnection This parameter can be one of the following values: 00895 * @arg @ref LL_DAC_CONNECT_GPIO 00896 * @arg @ref LL_DAC_CONNECT_INTERNAL 00897 * @retval None 00898 */ 00899 __STATIC_INLINE void LL_DAC_SetOutputConnection(DAC_TypeDef *DACx, uint32_t DAC_Channel, uint32_t OutputConnection) 00900 { 00901 MODIFY_REG(DACx->MCR, 00902 DAC_MCR_MODE1_0 << (DAC_Channel & DAC_CR_CHx_BITOFFSET_MASK), 00903 OutputConnection << (DAC_Channel & DAC_CR_CHx_BITOFFSET_MASK)); 00904 } 00905 00906 /** 00907 * @brief Get the output connection for the selected DAC channel. 00908 * @note On this STM32 family, output connection depends on output mode (normal or 00909 * sample and hold) and output buffer state. 00910 * - if output connection is set to internal path and output buffer 00911 * is enabled (whatever output mode): 00912 * output connection is also connected to GPIO pin 00913 * (both connections to GPIO pin and internal path). 00914 * - if output connection is set to GPIO pin, output buffer 00915 * is disabled, output mode set to sample and hold: 00916 * output connection is also connected to internal path 00917 * (both connections to GPIO pin and internal path). 00918 * @rmtoll CR MODE1 LL_DAC_GetOutputConnection\n 00919 * CR MODE2 LL_DAC_GetOutputConnection 00920 * @param DACx DAC instance 00921 * @param DAC_Channel This parameter can be one of the following values: 00922 * @arg @ref LL_DAC_CHANNEL_1 00923 * @arg @ref LL_DAC_CHANNEL_2 (1) 00924 * (1) On this STM32 family, parameter not available on all devices. 00925 * Refer to device datasheet for channels availability. 00926 * @retval Returned value can be one of the following values: 00927 * @arg @ref LL_DAC_CONNECT_GPIO 00928 * @arg @ref LL_DAC_CONNECT_INTERNAL 00929 */ 00930 __STATIC_INLINE uint32_t LL_DAC_GetOutputConnection(DAC_TypeDef *DACx, uint32_t DAC_Channel) 00931 { 00932 return (uint32_t)(READ_BIT(DACx->MCR, DAC_MCR_MODE1_0 << (DAC_Channel & DAC_CR_CHx_BITOFFSET_MASK)) 00933 >> (DAC_Channel & DAC_CR_CHx_BITOFFSET_MASK) 00934 ); 00935 } 00936 00937 /** 00938 * @brief Set the sample-and-hold timing for the selected DAC channel: 00939 * sample time 00940 * @note Sample time must be set when DAC channel is disabled 00941 * or during DAC operation when DAC channel flag BWSTx is reset, 00942 * otherwise the setting is ignored. 00943 * Check BWSTx flag state using function "LL_DAC_IsActiveFlag_BWSTx()". 00944 * @rmtoll SHSR1 TSAMPLE1 LL_DAC_SetSampleAndHoldSampleTime\n 00945 * SHSR2 TSAMPLE2 LL_DAC_SetSampleAndHoldSampleTime 00946 * @param DACx DAC instance 00947 * @param DAC_Channel This parameter can be one of the following values: 00948 * @arg @ref LL_DAC_CHANNEL_1 00949 * @arg @ref LL_DAC_CHANNEL_2 (1) 00950 * (1) On this STM32 family, parameter not available on all devices. 00951 * Refer to device datasheet for channels availability. 00952 * @param SampleTime 0x000...0x3FF 00953 * @retval None 00954 */ 00955 __STATIC_INLINE void LL_DAC_SetSampleAndHoldSampleTime(DAC_TypeDef *DACx, uint32_t DAC_Channel, uint32_t SampleTime) 00956 { 00957 register uint32_t *preg = __DAC_PTR_REG_OFFSET(DACx->SHSR1, __DAC_MASK_SHIFT(DAC_Channel, DAC_REG_SHSRx_REGOFFSET_MASK)); 00958 00959 MODIFY_REG(*preg, 00960 DAC_SHSR1_TSAMPLE1, 00961 SampleTime); 00962 } 00963 00964 /** 00965 * @brief Get the sample-and-hold timing for the selected DAC channel: 00966 * sample time 00967 * @rmtoll SHSR1 TSAMPLE1 LL_DAC_GetSampleAndHoldSampleTime\n 00968 * SHSR2 TSAMPLE2 LL_DAC_GetSampleAndHoldSampleTime 00969 * @param DACx DAC instance 00970 * @param DAC_Channel This parameter can be one of the following values: 00971 * @arg @ref LL_DAC_CHANNEL_1 00972 * @arg @ref LL_DAC_CHANNEL_2 (1) 00973 * (1) On this STM32 family, parameter not available on all devices. 00974 * Refer to device datasheet for channels availability. 00975 * @retval 0x000...0x3FF 00976 */ 00977 __STATIC_INLINE uint32_t LL_DAC_GetSampleAndHoldSampleTime(DAC_TypeDef *DACx, uint32_t DAC_Channel) 00978 { 00979 register uint32_t *preg = __DAC_PTR_REG_OFFSET(DACx->SHSR1, __DAC_MASK_SHIFT(DAC_Channel, DAC_REG_SHSRx_REGOFFSET_MASK)); 00980 00981 return (uint32_t) READ_BIT(*preg, DAC_SHSR1_TSAMPLE1); 00982 } 00983 00984 /** 00985 * @brief Set the sample-and-hold timing for the selected DAC channel: 00986 * hold time 00987 * @rmtoll SHHR THOLD1 LL_DAC_SetSampleAndHoldHoldTime\n 00988 * SHHR THOLD2 LL_DAC_SetSampleAndHoldHoldTime 00989 * @param DACx DAC instance 00990 * @param DAC_Channel This parameter can be one of the following values: 00991 * @arg @ref LL_DAC_CHANNEL_1 00992 * @arg @ref LL_DAC_CHANNEL_2 (1) 00993 * (1) On this STM32 family, parameter not available on all devices. 00994 * Refer to device datasheet for channels availability. 00995 * @param HoldTime 0x000...0x3FF 00996 * @retval None 00997 */ 00998 __STATIC_INLINE void LL_DAC_SetSampleAndHoldHoldTime(DAC_TypeDef *DACx, uint32_t DAC_Channel, uint32_t HoldTime) 00999 { 01000 MODIFY_REG(DACx->SHHR, 01001 DAC_SHHR_THOLD1 << (DAC_Channel & DAC_CR_CHx_BITOFFSET_MASK), 01002 HoldTime << (DAC_Channel & DAC_CR_CHx_BITOFFSET_MASK)); 01003 } 01004 01005 /** 01006 * @brief Get the sample-and-hold timing for the selected DAC channel: 01007 * hold time 01008 * @rmtoll SHHR THOLD1 LL_DAC_GetSampleAndHoldHoldTime\n 01009 * SHHR THOLD2 LL_DAC_GetSampleAndHoldHoldTime 01010 * @param DACx DAC instance 01011 * @param DAC_Channel This parameter can be one of the following values: 01012 * @arg @ref LL_DAC_CHANNEL_1 01013 * @arg @ref LL_DAC_CHANNEL_2 (1) 01014 * (1) On this STM32 family, parameter not available on all devices. 01015 * Refer to device datasheet for channels availability. 01016 * @retval 0x000...0x3FF 01017 */ 01018 __STATIC_INLINE uint32_t LL_DAC_GetSampleAndHoldHoldTime(DAC_TypeDef *DACx, uint32_t DAC_Channel) 01019 { 01020 return (uint32_t)(READ_BIT(DACx->SHHR, DAC_SHHR_THOLD1 << (DAC_Channel & DAC_CR_CHx_BITOFFSET_MASK)) 01021 >> (DAC_Channel & DAC_CR_CHx_BITOFFSET_MASK) 01022 ); 01023 } 01024 01025 /** 01026 * @brief Set the sample-and-hold timing for the selected DAC channel: 01027 * refresh time 01028 * @rmtoll SHRR TREFRESH1 LL_DAC_SetSampleAndHoldRefreshTime\n 01029 * SHRR TREFRESH2 LL_DAC_SetSampleAndHoldRefreshTime 01030 * @param DACx DAC instance 01031 * @param DAC_Channel This parameter can be one of the following values: 01032 * @arg @ref LL_DAC_CHANNEL_1 01033 * @arg @ref LL_DAC_CHANNEL_2 (1) 01034 * (1) On this STM32 family, parameter not available on all devices. 01035 * Refer to device datasheet for channels availability. 01036 * @param RefreshTime 0x00...0xFF 01037 * @retval None 01038 */ 01039 __STATIC_INLINE void LL_DAC_SetSampleAndHoldRefreshTime(DAC_TypeDef *DACx, uint32_t DAC_Channel, uint32_t RefreshTime) 01040 { 01041 MODIFY_REG(DACx->SHRR, 01042 DAC_SHRR_TREFRESH1 << (DAC_Channel & DAC_CR_CHx_BITOFFSET_MASK), 01043 RefreshTime << (DAC_Channel & DAC_CR_CHx_BITOFFSET_MASK)); 01044 } 01045 01046 /** 01047 * @brief Get the sample-and-hold timing for the selected DAC channel: 01048 * refresh time 01049 * @rmtoll SHRR TREFRESH1 LL_DAC_GetSampleAndHoldRefreshTime\n 01050 * SHRR TREFRESH2 LL_DAC_GetSampleAndHoldRefreshTime 01051 * @param DACx DAC instance 01052 * @param DAC_Channel This parameter can be one of the following values: 01053 * @arg @ref LL_DAC_CHANNEL_1 01054 * @arg @ref LL_DAC_CHANNEL_2 (1) 01055 * (1) On this STM32 family, parameter not available on all devices. 01056 * Refer to device datasheet for channels availability. 01057 * @retval 0x00...0xFF 01058 */ 01059 __STATIC_INLINE uint32_t LL_DAC_GetSampleAndHoldRefreshTime(DAC_TypeDef *DACx, uint32_t DAC_Channel) 01060 { 01061 return (uint32_t)(READ_BIT(DACx->SHRR, DAC_SHRR_TREFRESH1 << (DAC_Channel & DAC_CR_CHx_BITOFFSET_MASK)) 01062 >> (DAC_Channel & DAC_CR_CHx_BITOFFSET_MASK) 01063 ); 01064 } 01065 01066 /** 01067 * @} 01068 */ 01069 01070 /** @defgroup DAC_LL_EF_DMA_Management DMA_Management 01071 * @{ 01072 */ 01073 01074 /** 01075 * @brief Enable DAC DMA transfer request of the selected channel. 01076 * @note To configure DMA source address (peripheral address), 01077 * use function @ref LL_DAC_DMA_GetRegAddr(). 01078 * @rmtoll CR DMAEN1 LL_DAC_EnableDMAReq\n 01079 * CR DMAEN2 LL_DAC_EnableDMAReq 01080 * @param DACx DAC instance 01081 * @param DAC_Channel This parameter can be one of the following values: 01082 * @arg @ref LL_DAC_CHANNEL_1 01083 * @arg @ref LL_DAC_CHANNEL_2 (1) 01084 * (1) On this STM32 family, parameter not available on all devices. 01085 * Refer to device datasheet for channels availability. 01086 * @retval None 01087 */ 01088 __STATIC_INLINE void LL_DAC_EnableDMAReq(DAC_TypeDef *DACx, uint32_t DAC_Channel) 01089 { 01090 SET_BIT(DACx->CR, 01091 DAC_CR_DMAEN1 << (DAC_Channel & DAC_CR_CHx_BITOFFSET_MASK)); 01092 } 01093 01094 /** 01095 * @brief Disable DAC DMA transfer request of the selected channel. 01096 * @note To configure DMA source address (peripheral address), 01097 * use function @ref LL_DAC_DMA_GetRegAddr(). 01098 * @rmtoll CR DMAEN1 LL_DAC_DisableDMAReq\n 01099 * CR DMAEN2 LL_DAC_DisableDMAReq 01100 * @param DACx DAC instance 01101 * @param DAC_Channel This parameter can be one of the following values: 01102 * @arg @ref LL_DAC_CHANNEL_1 01103 * @arg @ref LL_DAC_CHANNEL_2 (1) 01104 * (1) On this STM32 family, parameter not available on all devices. 01105 * Refer to device datasheet for channels availability. 01106 * @retval None 01107 */ 01108 __STATIC_INLINE void LL_DAC_DisableDMAReq(DAC_TypeDef *DACx, uint32_t DAC_Channel) 01109 { 01110 CLEAR_BIT(DACx->CR, 01111 DAC_CR_DMAEN1 << (DAC_Channel & DAC_CR_CHx_BITOFFSET_MASK)); 01112 } 01113 01114 /** 01115 * @brief Get DAC DMA transfer request state of the selected channel. 01116 * (0: DAC DMA transfer request is disabled, 1: DAC DMA transfer request is enabled) 01117 * @rmtoll CR DMAEN1 LL_DAC_IsDMAReqEnabled\n 01118 * CR DMAEN2 LL_DAC_IsDMAReqEnabled 01119 * @param DACx DAC instance 01120 * @param DAC_Channel This parameter can be one of the following values: 01121 * @arg @ref LL_DAC_CHANNEL_1 01122 * @arg @ref LL_DAC_CHANNEL_2 (1) 01123 * (1) On this STM32 family, parameter not available on all devices. 01124 * Refer to device datasheet for channels availability. 01125 * @retval State of bit (1 or 0). 01126 */ 01127 __STATIC_INLINE uint32_t LL_DAC_IsDMAReqEnabled(DAC_TypeDef *DACx, uint32_t DAC_Channel) 01128 { 01129 return (READ_BIT(DACx->CR, 01130 DAC_CR_DMAEN1 << (DAC_Channel & DAC_CR_CHx_BITOFFSET_MASK)) 01131 == (DAC_CR_DMAEN1 << (DAC_Channel & DAC_CR_CHx_BITOFFSET_MASK))); 01132 } 01133 01134 /** 01135 * @brief Function to help to configure DMA transfer to DAC: retrieve the 01136 * DAC register address from DAC instance and a list of DAC registers 01137 * intended to be used (most commonly) with DMA transfer. 01138 * These DAC registers are data holding registers: 01139 * when DAC conversion is requested, DAC generates a DMA transfer 01140 * request to have data available in DAC data holding registers. 01141 * @note This macro is intended to be used with LL DMA driver, refer to 01142 * function "LL_DMA_ConfigAddresses()". 01143 * Example: 01144 * LL_DMA_ConfigAddresses(DMA1, 01145 * LL_DMA_CHANNEL_1, 01146 * (uint32_t)&< array or variable >, 01147 * LL_DAC_DMA_GetRegAddr(DAC1, LL_DAC_CHANNEL_1, LL_DAC_DMA_REG_DATA_12BITS_RIGHT_ALIGNED), 01148 * LL_DMA_DIRECTION_MEMORY_TO_PERIPH); 01149 * @rmtoll DHR12R1 DACC1DHR LL_DAC_DMA_GetRegAddr\n 01150 * DHR12L1 DACC1DHR LL_DAC_DMA_GetRegAddr\n 01151 * DHR8R1 DACC1DHR LL_DAC_DMA_GetRegAddr\n 01152 * DHR12R2 DACC2DHR LL_DAC_DMA_GetRegAddr\n 01153 * DHR12L2 DACC2DHR LL_DAC_DMA_GetRegAddr\n 01154 * DHR8R2 DACC2DHR LL_DAC_DMA_GetRegAddr 01155 * @param DACx DAC instance 01156 * @param DAC_Channel This parameter can be one of the following values: 01157 * @arg @ref LL_DAC_CHANNEL_1 01158 * @arg @ref LL_DAC_CHANNEL_2 (1) 01159 * (1) On this STM32 family, parameter not available on all devices. 01160 * Refer to device datasheet for channels availability. 01161 * @param Register This parameter can be one of the following values: 01162 * @arg @ref LL_DAC_DMA_REG_DATA_12BITS_RIGHT_ALIGNED 01163 * @arg @ref LL_DAC_DMA_REG_DATA_12BITS_LEFT_ALIGNED 01164 * @arg @ref LL_DAC_DMA_REG_DATA_8BITS_RIGHT_ALIGNED 01165 * @retval DAC register address 01166 */ 01167 __STATIC_INLINE uint32_t LL_DAC_DMA_GetRegAddr(DAC_TypeDef *DACx, uint32_t DAC_Channel, uint32_t Register) 01168 { 01169 /* Retrieve address of register DHR12Rx, DHR12Lx or DHR8Rx depending on */ 01170 /* DAC channel selected. */ 01171 return ((uint32_t)(__DAC_PTR_REG_OFFSET((DACx)->DHR12R1, __DAC_MASK_SHIFT(DAC_Channel, Register)))); 01172 } 01173 /** 01174 * @} 01175 */ 01176 01177 /** @defgroup DAC_LL_EF_Operation Operation on DAC channels 01178 * @{ 01179 */ 01180 01181 /** 01182 * @brief Enable DAC selected channel. 01183 * @rmtoll CR EN1 LL_DAC_Enable\n 01184 * CR EN2 LL_DAC_Enable 01185 * @param DACx DAC instance 01186 * @param DAC_Channel This parameter can be one of the following values: 01187 * @arg @ref LL_DAC_CHANNEL_1 01188 * @arg @ref LL_DAC_CHANNEL_2 (1) 01189 * (1) On this STM32 family, parameter not available on all devices. 01190 * Refer to device datasheet for channels availability. 01191 * @retval None 01192 */ 01193 __STATIC_INLINE void LL_DAC_Enable(DAC_TypeDef *DACx, uint32_t DAC_Channel) 01194 { 01195 SET_BIT(DACx->CR, 01196 DAC_CR_EN1 << (DAC_Channel & DAC_CR_CHx_BITOFFSET_MASK)); 01197 } 01198 01199 /** 01200 * @brief Disable DAC selected channel. 01201 * @rmtoll CR EN1 LL_DAC_Disable\n 01202 * CR EN2 LL_DAC_Disable 01203 * @param DACx DAC instance 01204 * @param DAC_Channel This parameter can be one of the following values: 01205 * @arg @ref LL_DAC_CHANNEL_1 01206 * @arg @ref LL_DAC_CHANNEL_2 (1) 01207 * (1) On this STM32 family, parameter not available on all devices. 01208 * Refer to device datasheet for channels availability. 01209 * @retval None 01210 */ 01211 __STATIC_INLINE void LL_DAC_Disable(DAC_TypeDef *DACx, uint32_t DAC_Channel) 01212 { 01213 CLEAR_BIT(DACx->CR, 01214 DAC_CR_EN1 << (DAC_Channel & DAC_CR_CHx_BITOFFSET_MASK)); 01215 } 01216 01217 /** 01218 * @brief Get DAC enable state of the selected channel. 01219 * (0: DAC channel is disabled, 1: DAC channel is enabled) 01220 * @rmtoll CR EN1 LL_DAC_IsEnabled\n 01221 * CR EN2 LL_DAC_IsEnabled 01222 * @param DACx DAC instance 01223 * @param DAC_Channel This parameter can be one of the following values: 01224 * @arg @ref LL_DAC_CHANNEL_1 01225 * @arg @ref LL_DAC_CHANNEL_2 (1) 01226 * (1) On this STM32 family, parameter not available on all devices. 01227 * Refer to device datasheet for channels availability. 01228 * @retval State of bit (1 or 0). 01229 */ 01230 __STATIC_INLINE uint32_t LL_DAC_IsEnabled(DAC_TypeDef *DACx, uint32_t DAC_Channel) 01231 { 01232 return (READ_BIT(DACx->CR, 01233 DAC_CR_EN1 << (DAC_Channel & DAC_CR_CHx_BITOFFSET_MASK)) 01234 == (DAC_CR_EN1 << (DAC_Channel & DAC_CR_CHx_BITOFFSET_MASK))); 01235 } 01236 01237 /** 01238 * @brief Enable DAC trigger of the selected channel. 01239 * - If DAC trigger is disabled, DAC conversion is performed 01240 * automatically once the data holding register is updated, 01241 * using functions "LL_DAC_ConvertData{8; 12}{Right; Left} Aligned()": 01242 * @ref LL_DAC_ConvertData12RightAligned(), ... 01243 * - If DAC trigger is enabled, DAC conversion is performed 01244 * only when a hardware of software trigger event is occurring. 01245 * Select trigger source using 01246 * function @ref LL_DAC_SetTriggerSource(). 01247 * @rmtoll CR TEN1 LL_DAC_EnableTrigger\n 01248 * CR TEN2 LL_DAC_EnableTrigger 01249 * @param DACx DAC instance 01250 * @param DAC_Channel This parameter can be one of the following values: 01251 * @arg @ref LL_DAC_CHANNEL_1 01252 * @arg @ref LL_DAC_CHANNEL_2 (1) 01253 * (1) On this STM32 family, parameter not available on all devices. 01254 * Refer to device datasheet for channels availability. 01255 * @retval None 01256 */ 01257 __STATIC_INLINE void LL_DAC_EnableTrigger(DAC_TypeDef *DACx, uint32_t DAC_Channel) 01258 { 01259 SET_BIT(DACx->CR, 01260 DAC_CR_TEN1 << (DAC_Channel & DAC_CR_CHx_BITOFFSET_MASK)); 01261 } 01262 01263 /** 01264 * @brief Disable DAC trigger of the selected channel. 01265 * @rmtoll CR TEN1 LL_DAC_DisableTrigger\n 01266 * CR TEN2 LL_DAC_DisableTrigger 01267 * @param DACx DAC instance 01268 * @param DAC_Channel This parameter can be one of the following values: 01269 * @arg @ref LL_DAC_CHANNEL_1 01270 * @arg @ref LL_DAC_CHANNEL_2 (1) 01271 * (1) On this STM32 family, parameter not available on all devices. 01272 * Refer to device datasheet for channels availability. 01273 * @retval None 01274 */ 01275 __STATIC_INLINE void LL_DAC_DisableTrigger(DAC_TypeDef *DACx, uint32_t DAC_Channel) 01276 { 01277 CLEAR_BIT(DACx->CR, 01278 DAC_CR_TEN1 << (DAC_Channel & DAC_CR_CHx_BITOFFSET_MASK)); 01279 } 01280 01281 /** 01282 * @brief Get DAC trigger state of the selected channel. 01283 * (0: DAC trigger is disabled, 1: DAC trigger is enabled) 01284 * @rmtoll CR TEN1 LL_DAC_IsTriggerEnabled\n 01285 * CR TEN2 LL_DAC_IsTriggerEnabled 01286 * @param DACx DAC instance 01287 * @param DAC_Channel This parameter can be one of the following values: 01288 * @arg @ref LL_DAC_CHANNEL_1 01289 * @arg @ref LL_DAC_CHANNEL_2 (1) 01290 * (1) On this STM32 family, parameter not available on all devices. 01291 * Refer to device datasheet for channels availability. 01292 * @retval State of bit (1 or 0). 01293 */ 01294 __STATIC_INLINE uint32_t LL_DAC_IsTriggerEnabled(DAC_TypeDef *DACx, uint32_t DAC_Channel) 01295 { 01296 return (READ_BIT(DACx->CR, 01297 DAC_CR_TEN1 << (DAC_Channel & DAC_CR_CHx_BITOFFSET_MASK)) 01298 == (DAC_CR_TEN1 << (DAC_Channel & DAC_CR_CHx_BITOFFSET_MASK))); 01299 } 01300 01301 /** 01302 * @brief Trig DAC conversion by software for the selected DAC channel. 01303 * @note Preliminarily, DAC trigger must be set to software trigger 01304 * using function @ref LL_DAC_SetTriggerSource() 01305 * with parameter "LL_DAC_TRIGGER_SOFTWARE". 01306 * and DAC trigger must be enabled using 01307 * function @ref LL_DAC_EnableTrigger(). 01308 * @note For devices featuring DAC with 2 channels: this function 01309 * can perform a SW start of both DAC channels simultaneously. 01310 * Two channels can be selected as parameter. 01311 * Example: (LL_DAC_CHANNEL_1 | LL_DAC_CHANNEL_2) 01312 * @rmtoll SWTRIGR SWTRIG1 LL_DAC_TrigSWConversion\n 01313 * SWTRIGR SWTRIG2 LL_DAC_TrigSWConversion 01314 * @param DACx DAC instance 01315 * @param DAC_Channel This parameter can a combination of the following values: 01316 * @arg @ref LL_DAC_CHANNEL_1 01317 * @arg @ref LL_DAC_CHANNEL_2 (1) 01318 * (1) On this STM32 family, parameter not available on all devices. 01319 * Refer to device datasheet for channels availability. 01320 * @retval None 01321 */ 01322 __STATIC_INLINE void LL_DAC_TrigSWConversion(DAC_TypeDef *DACx, uint32_t DAC_Channel) 01323 { 01324 SET_BIT(DACx->SWTRIGR, 01325 (DAC_Channel & DAC_SWTR_CHX_MASK)); 01326 } 01327 01328 /** 01329 * @brief Set the data to be loaded in the data holding register 01330 * in format 12 bits left alignment (LSB aligned on bit 0), 01331 * for the selected DAC channel. 01332 * @rmtoll DHR12R1 DACC1DHR LL_DAC_ConvertData12RightAligned\n 01333 * DHR12R2 DACC2DHR LL_DAC_ConvertData12RightAligned 01334 * @param DACx DAC instance 01335 * @param DAC_Channel This parameter can be one of the following values: 01336 * @arg @ref LL_DAC_CHANNEL_1 01337 * @arg @ref LL_DAC_CHANNEL_2 (1) 01338 * (1) On this STM32 family, parameter not available on all devices. 01339 * Refer to device datasheet for channels availability. 01340 * @param Data 0x000...0xFFF 01341 * @retval None 01342 */ 01343 __STATIC_INLINE void LL_DAC_ConvertData12RightAligned(DAC_TypeDef *DACx, uint32_t DAC_Channel, uint32_t Data) 01344 { 01345 register uint32_t *preg = __DAC_PTR_REG_OFFSET(DACx->DHR12R1, __DAC_MASK_SHIFT(DAC_Channel, DAC_REG_DHR12Rx_REGOFFSET_MASK)); 01346 01347 MODIFY_REG(*preg, 01348 DAC_DHR12R1_DACC1DHR, 01349 Data); 01350 } 01351 01352 /** 01353 * @brief Set the data to be loaded in the data holding register 01354 * in format 12 bits left alignment (MSB aligned on bit 15), 01355 * for the selected DAC channel. 01356 * @rmtoll DHR12L1 DACC1DHR LL_DAC_ConvertData12LeftAligned\n 01357 * DHR12L2 DACC2DHR LL_DAC_ConvertData12LeftAligned 01358 * @param DACx DAC instance 01359 * @param DAC_Channel This parameter can be one of the following values: 01360 * @arg @ref LL_DAC_CHANNEL_1 01361 * @arg @ref LL_DAC_CHANNEL_2 (1) 01362 * (1) On this STM32 family, parameter not available on all devices. 01363 * Refer to device datasheet for channels availability. 01364 * @param Data 0x0000...0xFFFF 01365 * @retval None 01366 */ 01367 __STATIC_INLINE void LL_DAC_ConvertData12LeftAligned(DAC_TypeDef *DACx, uint32_t DAC_Channel, uint32_t Data) 01368 { 01369 register uint32_t *preg = __DAC_PTR_REG_OFFSET(DACx->DHR12R1, __DAC_MASK_SHIFT(DAC_Channel, DAC_REG_DHR12Lx_REGOFFSET_MASK)); 01370 01371 MODIFY_REG(*preg, 01372 DAC_DHR12L1_DACC1DHR, 01373 Data); 01374 } 01375 01376 /** 01377 * @brief Set the data to be loaded in the data holding register 01378 * in format 8 bits left alignment (LSB aligned on bit 0), 01379 * for the selected DAC channel. 01380 * @rmtoll DHR8R1 DACC1DHR LL_DAC_ConvertData8RightAligned\n 01381 * DHR8R2 DACC2DHR LL_DAC_ConvertData8RightAligned 01382 * @param DACx DAC instance 01383 * @param DAC_Channel This parameter can be one of the following values: 01384 * @arg @ref LL_DAC_CHANNEL_1 01385 * @arg @ref LL_DAC_CHANNEL_2 (1) 01386 * (1) On this STM32 family, parameter not available on all devices. 01387 * Refer to device datasheet for channels availability. 01388 * @param Data 0x00...0xFF 01389 * @retval None 01390 */ 01391 __STATIC_INLINE void LL_DAC_ConvertData8RightAligned(DAC_TypeDef *DACx, uint32_t DAC_Channel, uint32_t Data) 01392 { 01393 register uint32_t *preg = __DAC_PTR_REG_OFFSET(DACx->DHR12R1, __DAC_MASK_SHIFT(DAC_Channel, DAC_REG_DHR8Rx_REGOFFSET_MASK)); 01394 01395 MODIFY_REG(*preg, 01396 DAC_DHR8R1_DACC1DHR, 01397 Data); 01398 } 01399 01400 /** 01401 * @brief Set the data to be loaded in the data holding register 01402 * in format 12 bits left alignment (LSB aligned on bit 0), 01403 * for both DAC channels. 01404 * @rmtoll DHR12RD DACC1DHR LL_DAC_ConvertDualData12RightAligned\n 01405 * DHR12RD DACC2DHR LL_DAC_ConvertDualData12RightAligned 01406 * @param DACx DAC instance 01407 * @param DataChannel1 0x000...0xFFF 01408 * @param DataChannel2 0x000...0xFFF 01409 * @retval None 01410 */ 01411 __STATIC_INLINE void LL_DAC_ConvertDualData12RightAligned(DAC_TypeDef *DACx, uint32_t DataChannel1, uint32_t DataChannel2) 01412 { 01413 MODIFY_REG(DACx->DHR12RD, 01414 (DAC_DHR12RD_DACC2DHR | DAC_DHR12RD_DACC1DHR), 01415 ((DataChannel2 << POSITION_VAL(DAC_DHR12RD_DACC2DHR)) | DataChannel1)); 01416 } 01417 01418 /** 01419 * @brief Set the data to be loaded in the data holding register 01420 * in format 12 bits left alignment (MSB aligned on bit 15), 01421 * for both DAC channels. 01422 * @rmtoll DHR12LD DACC1DHR LL_DAC_ConvertDualData12LeftAligned\n 01423 * DHR12LD DACC2DHR LL_DAC_ConvertDualData12LeftAligned 01424 * @param DACx DAC instance 01425 * @param DataChannel1 0x000...0xFFFF 01426 * @param DataChannel2 0x000...0xFFFF 01427 * @retval None 01428 */ 01429 __STATIC_INLINE void LL_DAC_ConvertDualData12LeftAligned(DAC_TypeDef *DACx, uint32_t DataChannel1, uint32_t DataChannel2) 01430 { 01431 /* Note: Data of DAC channel 2 shift value subtracted of 4 because */ 01432 /* data on 16 bits and DAC channel 2 bits field is on the 12 MSB, */ 01433 /* the 4 LSB must be taken into account for the shift value. */ 01434 MODIFY_REG(DACx->DHR12LD, 01435 (DAC_DHR12LD_DACC2DHR | DAC_DHR12LD_DACC1DHR), 01436 ((DataChannel2 << (POSITION_VAL(DAC_DHR12LD_DACC2DHR) - 4)) | DataChannel1)); 01437 } 01438 01439 /** 01440 * @brief Set the data to be loaded in the data holding register 01441 * in format 8 bits left alignment (LSB aligned on bit 0), 01442 * for both DAC channels. 01443 * @rmtoll DHR8RD DACC1DHR LL_DAC_ConvertDualData8RightAligned\n 01444 * DHR8RD DACC2DHR LL_DAC_ConvertDualData8RightAligned 01445 * @param DACx DAC instance 01446 * @param DataChannel1 0x00...0xFF 01447 * @param DataChannel2 0x00...0xFF 01448 * @retval None 01449 */ 01450 __STATIC_INLINE void LL_DAC_ConvertDualData8RightAligned(DAC_TypeDef *DACx, uint32_t DataChannel1, uint32_t DataChannel2) 01451 { 01452 MODIFY_REG(DACx->DHR8RD, 01453 (DAC_DHR8RD_DACC2DHR | DAC_DHR8RD_DACC1DHR), 01454 ((DataChannel2 << POSITION_VAL(DAC_DHR8RD_DACC2DHR)) | DataChannel1)); 01455 } 01456 01457 /** 01458 * @brief Retrieve output data currently generated for the selected DAC channel. 01459 * @note Whatever alignment and resolution settings 01460 * (using functions "LL_DAC_ConvertData{8; 12}{Right; Left} Aligned()": 01461 * @ref LL_DAC_ConvertData12RightAligned(), ...), 01462 * output data format is 12 bits right aligned (LSB aligned on bit 0). 01463 * @rmtoll DOR1 DACC1DOR LL_DAC_RetrieveOutputData\n 01464 * DOR2 DACC2DOR LL_DAC_RetrieveOutputData 01465 * @param DACx DAC instance 01466 * @param DAC_Channel This parameter can be one of the following values: 01467 * @arg @ref LL_DAC_CHANNEL_1 01468 * @arg @ref LL_DAC_CHANNEL_2 (1) 01469 * (1) On this STM32 family, parameter not available on all devices. 01470 * Refer to device datasheet for channels availability. 01471 * @retval 0x000...0xFFF 01472 */ 01473 __STATIC_INLINE uint32_t LL_DAC_RetrieveOutputData(DAC_TypeDef *DACx, uint32_t DAC_Channel) 01474 { 01475 register uint32_t *preg = __DAC_PTR_REG_OFFSET(DACx->DOR1, __DAC_MASK_SHIFT(DAC_Channel, DAC_REG_SHSRx_REGOFFSET_MASK)); 01476 01477 return (uint16_t) READ_BIT(*preg, DAC_DOR1_DACC1DOR); 01478 } 01479 01480 /** 01481 * @} 01482 */ 01483 01484 /** @defgroup DAC_LL_EF_FLAG_Management FLAG_Management 01485 * @{ 01486 */ 01487 /** 01488 * @brief Get DAC calibration offset flag for DAC channel 1 01489 * @rmtoll SR CAL_FLAG1 LL_DAC_IsActiveFlag_CAL1 01490 * @param DACx DAC instance 01491 * @retval State of bit (1 or 0). 01492 */ 01493 __STATIC_INLINE uint32_t LL_DAC_IsActiveFlag_CAL1(DAC_TypeDef *DACx) 01494 { 01495 return (READ_BIT(DACx->SR, DAC_SR_CAL_FLAG1) == (DAC_SR_CAL_FLAG1)); 01496 } 01497 01498 /** 01499 * @brief Get DAC calibration offset flag for DAC channel 2 01500 * @rmtoll SR CAL_FLAG2 LL_DAC_IsActiveFlag_CAL2 01501 * @param DACx DAC instance 01502 * @retval State of bit (1 or 0). 01503 */ 01504 __STATIC_INLINE uint32_t LL_DAC_IsActiveFlag_CAL2(DAC_TypeDef *DACx) 01505 { 01506 return (READ_BIT(DACx->SR, DAC_SR_CAL_FLAG2) == (DAC_SR_CAL_FLAG2)); 01507 } 01508 01509 /** 01510 * @brief Get DAC busy writing sample time flag for DAC channel 1 01511 * @rmtoll SR BWST1 LL_DAC_IsActiveFlag_BWST1 01512 * @param DACx DAC instance 01513 * @retval State of bit (1 or 0). 01514 */ 01515 __STATIC_INLINE uint32_t LL_DAC_IsActiveFlag_BWST1(DAC_TypeDef *DACx) 01516 { 01517 return (READ_BIT(DACx->SR, DAC_SR_BWST1) == (DAC_SR_BWST1)); 01518 } 01519 01520 /** 01521 * @brief Get DAC busy writing sample time flag for DAC channel 2 01522 * @rmtoll SR BWST2 LL_DAC_IsActiveFlag_BWST2 01523 * @param DACx DAC instance 01524 * @retval State of bit (1 or 0). 01525 */ 01526 __STATIC_INLINE uint32_t LL_DAC_IsActiveFlag_BWST2(DAC_TypeDef *DACx) 01527 { 01528 return (READ_BIT(DACx->SR, DAC_SR_BWST2) == (DAC_SR_BWST2)); 01529 } 01530 01531 /** 01532 * @brief Get DAC underrun flag for DAC channel 1 01533 * @rmtoll SR DMAUDR1 LL_DAC_IsActiveFlag_DMAUDR1 01534 * @param DACx DAC instance 01535 * @retval State of bit (1 or 0). 01536 */ 01537 __STATIC_INLINE uint32_t LL_DAC_IsActiveFlag_DMAUDR1(DAC_TypeDef *DACx) 01538 { 01539 return (READ_BIT(DACx->SR, DAC_SR_DMAUDR1) == (DAC_SR_DMAUDR1)); 01540 } 01541 01542 /** 01543 * @brief Get DAC underrun flag for DAC channel 2 01544 * @rmtoll SR DMAUDR2 LL_DAC_IsActiveFlag_DMAUDR2 01545 * @param DACx DAC instance 01546 * @retval State of bit (1 or 0). 01547 */ 01548 __STATIC_INLINE uint32_t LL_DAC_IsActiveFlag_DMAUDR2(DAC_TypeDef *DACx) 01549 { 01550 return (READ_BIT(DACx->SR, DAC_SR_DMAUDR2) == (DAC_SR_DMAUDR2)); 01551 } 01552 01553 /** 01554 * @brief Clear DAC underrun flag for DAC channel 1 01555 * @rmtoll SR DMAUDR1 LL_DAC_ClearFlag_DMAUDR1 01556 * @param DACx DAC instance 01557 * @retval None 01558 */ 01559 __STATIC_INLINE void LL_DAC_ClearFlag_DMAUDR1(DAC_TypeDef *DACx) 01560 { 01561 WRITE_REG(DACx->SR, DAC_SR_DMAUDR1); 01562 } 01563 01564 /** 01565 * @brief Clear DAC underrun flag for DAC channel 2 01566 * @rmtoll SR DMAUDR2 LL_DAC_ClearFlag_DMAUDR2 01567 * @param DACx DAC instance 01568 * @retval None 01569 */ 01570 __STATIC_INLINE void LL_DAC_ClearFlag_DMAUDR2(DAC_TypeDef *DACx) 01571 { 01572 WRITE_REG(DACx->SR, DAC_SR_DMAUDR2); 01573 } 01574 01575 /** 01576 * @} 01577 */ 01578 01579 /** @defgroup DAC_LL_EF_IT_Management IT_Management 01580 * @{ 01581 */ 01582 01583 /** 01584 * @brief Enable DMA underrun interrupt for DAC channel 1 01585 * @rmtoll CR DMAUDRIE1 LL_DAC_EnableIT_DMAUDR1 01586 * @param DACx DAC instance 01587 * @retval None 01588 */ 01589 __STATIC_INLINE void LL_DAC_EnableIT_DMAUDR1(DAC_TypeDef *DACx) 01590 { 01591 SET_BIT(DACx->CR, DAC_CR_DMAUDRIE1); 01592 } 01593 01594 /** 01595 * @brief Enable DMA underrun interrupt for DAC channel 2 01596 * @rmtoll CR DMAUDRIE2 LL_DAC_EnableIT_DMAUDR2 01597 * @param DACx DAC instance 01598 * @retval None 01599 */ 01600 __STATIC_INLINE void LL_DAC_EnableIT_DMAUDR2(DAC_TypeDef *DACx) 01601 { 01602 SET_BIT(DACx->CR, DAC_CR_DMAUDRIE2); 01603 } 01604 01605 /** 01606 * @brief Disable DMA underrun interrupt for DAC channel 1 01607 * @rmtoll CR DMAUDRIE1 LL_DAC_DisableIT_DMAUDR1 01608 * @param DACx DAC instance 01609 * @retval None 01610 */ 01611 __STATIC_INLINE void LL_DAC_DisableIT_DMAUDR1(DAC_TypeDef *DACx) 01612 { 01613 CLEAR_BIT(DACx->CR, DAC_CR_DMAUDRIE1); 01614 } 01615 01616 /** 01617 * @brief Disable DMA underrun interrupt for DAC channel 2 01618 * @rmtoll CR DMAUDRIE2 LL_DAC_DisableIT_DMAUDR2 01619 * @param DACx DAC instance 01620 * @retval None 01621 */ 01622 __STATIC_INLINE void LL_DAC_DisableIT_DMAUDR2(DAC_TypeDef *DACx) 01623 { 01624 CLEAR_BIT(DACx->CR, DAC_CR_DMAUDRIE2); 01625 } 01626 01627 /** 01628 * @brief Get DMA underrun interrupt for DAC channel 1 01629 * @rmtoll CR DMAUDRIE1 LL_DAC_IsEnabledIT_DMAUDR1 01630 * @param DACx DAC instance 01631 * @retval State of bit (1 or 0). 01632 */ 01633 __STATIC_INLINE uint32_t LL_DAC_IsEnabledIT_DMAUDR1(DAC_TypeDef *DACx) 01634 { 01635 return (READ_BIT(DACx->CR, DAC_CR_DMAUDRIE1) == (DAC_CR_DMAUDRIE1)); 01636 } 01637 01638 /** 01639 * @brief Get DMA underrun interrupt for DAC channel 2 01640 * @rmtoll CR DMAUDRIE2 LL_DAC_IsEnabledIT_DMAUDR2 01641 * @param DACx DAC instance 01642 * @retval State of bit (1 or 0). 01643 */ 01644 __STATIC_INLINE uint32_t LL_DAC_IsEnabledIT_DMAUDR2(DAC_TypeDef *DACx) 01645 { 01646 return (READ_BIT(DACx->CR, DAC_CR_DMAUDRIE2) == (DAC_CR_DMAUDRIE2)); 01647 } 01648 01649 /** 01650 * @} 01651 */ 01652 01653 01654 /** 01655 * @} 01656 */ 01657 01658 /** 01659 * @} 01660 */ 01661 01662 #endif /* DAC1 */ 01663 01664 /** 01665 * @} 01666 */ 01667 01668 #ifdef __cplusplus 01669 } 01670 #endif 01671 01672 #endif /* __STM32L4xx_LL_DAC_H */ 01673 01674 /************************ (C) COPYRIGHT STMicroelectronics *****END OF FILE****/ 01675
Generated on Tue Jul 12 2022 11:35:16 by
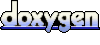