Hal Drivers for L4
Dependents: BSP OneHopeOnePrayer FINAL_AUDIO_RECORD AudioDemo
Fork of STM32L4xx_HAL_Driver by
stm32l4xx_hal_uart_ex.c
00001 /** 00002 ****************************************************************************** 00003 * @file stm32l4xx_hal_uart_ex.c 00004 * @author MCD Application Team 00005 * @version V1.1.0 00006 * @date 16-September-2015 00007 * @brief Extended UART HAL module driver. 00008 * This file provides firmware functions to manage the following extended 00009 * functionalities of the Universal Asynchronous Receiver Transmitter Peripheral (UART). 00010 * + Initialization and de-initialization functions 00011 * + Peripheral Control functions 00012 * 00013 * 00014 @verbatim 00015 ============================================================================== 00016 ##### UART peripheral extended features ##### 00017 ============================================================================== 00018 00019 (#) Declare a UART_HandleTypeDef handle structure. 00020 00021 (#) For the UART RS485 Driver Enable mode, initialize the UART registers 00022 by calling the HAL_RS485Ex_Init() API. 00023 00024 00025 @endverbatim 00026 ****************************************************************************** 00027 * @attention 00028 * 00029 * <h2><center>© COPYRIGHT(c) 2015 STMicroelectronics</center></h2> 00030 * 00031 * Redistribution and use in source and binary forms, with or without modification, 00032 * are permitted provided that the following conditions are met: 00033 * 1. Redistributions of source code must retain the above copyright notice, 00034 * this list of conditions and the following disclaimer. 00035 * 2. Redistributions in binary form must reproduce the above copyright notice, 00036 * this list of conditions and the following disclaimer in the documentation 00037 * and/or other materials provided with the distribution. 00038 * 3. Neither the name of STMicroelectronics nor the names of its contributors 00039 * may be used to endorse or promote products derived from this software 00040 * without specific prior written permission. 00041 * 00042 * THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" 00043 * AND ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE 00044 * IMPLIED WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE 00045 * DISCLAIMED. IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE 00046 * FOR ANY DIRECT, INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL 00047 * DAMAGES (INCLUDING, BUT NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR 00048 * SERVICES; LOSS OF USE, DATA, OR PROFITS; OR BUSINESS INTERRUPTION) HOWEVER 00049 * CAUSED AND ON ANY THEORY OF LIABILITY, WHETHER IN CONTRACT, STRICT LIABILITY, 00050 * OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) ARISING IN ANY WAY OUT OF THE USE 00051 * OF THIS SOFTWARE, EVEN IF ADVISED OF THE POSSIBILITY OF SUCH DAMAGE. 00052 * 00053 ****************************************************************************** 00054 */ 00055 00056 /* Includes ------------------------------------------------------------------*/ 00057 #include "stm32l4xx_hal.h" 00058 00059 /** @addtogroup STM32L4xx_HAL_Driver 00060 * @{ 00061 */ 00062 00063 /** @defgroup UARTEx UARTEx 00064 * @brief UART Extended HAL module driver 00065 * @{ 00066 */ 00067 00068 #ifdef HAL_UART_MODULE_ENABLED 00069 00070 /* Private typedef -----------------------------------------------------------*/ 00071 /* Private define ------------------------------------------------------------*/ 00072 /* Private macros ------------------------------------------------------------*/ 00073 /* Private variables ---------------------------------------------------------*/ 00074 /* Private function prototypes -----------------------------------------------*/ 00075 /** @defgroup UARTEx_Private_Functions UARTEx Private Functions 00076 * @{ 00077 */ 00078 static void UARTEx_Wakeup_AddressConfig(UART_HandleTypeDef *huart, UART_WakeUpTypeDef WakeUpSelection); 00079 /** 00080 * @} 00081 */ 00082 00083 /* Exported functions --------------------------------------------------------*/ 00084 00085 /** @defgroup UARTEx_Exported_Functions UARTEx Exported Functions 00086 * @{ 00087 */ 00088 00089 /** @defgroup UARTEx_Exported_Functions_Group1 Initialization and de-initialization functions 00090 * @brief Extended Initialization and Configuration Functions 00091 * 00092 @verbatim 00093 =============================================================================== 00094 ##### Initialization and Configuration functions ##### 00095 =============================================================================== 00096 [..] 00097 This subsection provides a set of functions allowing to initialize the USARTx or the UARTy 00098 in asynchronous mode. 00099 (+) For the asynchronous mode the parameters below can be configured: 00100 (++) Baud Rate 00101 (++) Word Length 00102 (++) Stop Bit 00103 (++) Parity: If the parity is enabled, then the MSB bit of the data written 00104 in the data register is transmitted but is changed by the parity bit. 00105 Depending on the frame length defined by the M1 and M0 bits (7-bit, 00106 8-bit or 9-bit), 00107 the possible UART frame formats are as listed in the following table: 00108 00109 (+++) Table 1. UART frame format. 00110 (+++) +-----------------------------------------------------------------------+ 00111 (+++) | M1 bit | M0 bit | PCE bit | UART frame | 00112 (+++) |---------|---------|-----------|---------------------------------------| 00113 (+++) | 0 | 0 | 0 | | SB | 8 bit data | STB | | 00114 (+++) |---------|---------|-----------|---------------------------------------| 00115 (+++) | 0 | 0 | 1 | | SB | 7 bit data | PB | STB | | 00116 (+++) |---------|---------|-----------|---------------------------------------| 00117 (+++) | 0 | 1 | 0 | | SB | 9 bit data | STB | | 00118 (+++) |---------|---------|-----------|---------------------------------------| 00119 (+++) | 0 | 1 | 1 | | SB | 8 bit data | PB | STB | | 00120 (+++) |---------|---------|-----------|---------------------------------------| 00121 (+++) | 1 | 0 | 0 | | SB | 7 bit data | STB | | 00122 (+++) |---------|---------|-----------|---------------------------------------| 00123 (+++) | 1 | 0 | 1 | | SB | 6 bit data | PB | STB | | 00124 (+++) +-----------------------------------------------------------------------+ 00125 (++) Hardware flow control 00126 (++) Receiver/transmitter modes 00127 (++) Over Sampling Method 00128 (++) One-Bit Sampling Method 00129 (+) For the asynchronous mode, the following advanced features can be configured as well: 00130 (++) TX and/or RX pin level inversion 00131 (++) data logical level inversion 00132 (++) RX and TX pins swap 00133 (++) RX overrun detection disabling 00134 (++) DMA disabling on RX error 00135 (++) MSB first on communication line 00136 (++) auto Baud rate detection 00137 [..] 00138 The HAL_RS485Ex_Init() API follows the UART RS485 mode configuration 00139 procedures (details for the procedures are available in reference manual). 00140 00141 @endverbatim 00142 * @{ 00143 */ 00144 00145 /** 00146 * @brief Initialize the RS485 Driver enable feature according to the specified 00147 * parameters in the UART_InitTypeDef and creates the associated handle. 00148 * @param huart: UART handle. 00149 * @param Polarity: select the driver enable polarity. 00150 * This parameter can be one of the following values: 00151 * @arg UART_DE_POLARITY_HIGH: DE signal is active high 00152 * @arg UART_DE_POLARITY_LOW: DE signal is active low 00153 * @param AssertionTime: Driver Enable assertion time: 00154 * 5-bit value defining the time between the activation of the DE (Driver Enable) 00155 * signal and the beginning of the start bit. It is expressed in sample time 00156 * units (1/8 or 1/16 bit time, depending on the oversampling rate) 00157 * @param DeassertionTime: Driver Enable deassertion time: 00158 * 5-bit value defining the time between the end of the last stop bit, in a 00159 * transmitted message, and the de-activation of the DE (Driver Enable) signal. 00160 * It is expressed in sample time units (1/8 or 1/16 bit time, depending on the 00161 * oversampling rate). 00162 * @retval HAL status 00163 */ 00164 HAL_StatusTypeDef HAL_RS485Ex_Init(UART_HandleTypeDef *huart, uint32_t Polarity, uint32_t AssertionTime, uint32_t DeassertionTime) 00165 { 00166 uint32_t temp = 0x0; 00167 00168 /* Check the UART handle allocation */ 00169 if(huart == NULL) 00170 { 00171 return HAL_ERROR; 00172 } 00173 /* Check the Driver Enable UART instance */ 00174 assert_param(IS_UART_DRIVER_ENABLE_INSTANCE(huart->Instance)); 00175 00176 /* Check the Driver Enable polarity */ 00177 assert_param(IS_UART_DE_POLARITY(Polarity)); 00178 00179 /* Check the Driver Enable assertion time */ 00180 assert_param(IS_UART_ASSERTIONTIME(AssertionTime)); 00181 00182 /* Check the Driver Enable deassertion time */ 00183 assert_param(IS_UART_DEASSERTIONTIME(DeassertionTime)); 00184 00185 if(huart->State == HAL_UART_STATE_RESET) 00186 { 00187 /* Allocate lock resource and initialize it */ 00188 huart->Lock = HAL_UNLOCKED; 00189 00190 /* Init the low level hardware : GPIO, CLOCK, CORTEX */ 00191 HAL_UART_MspInit(huart); 00192 } 00193 00194 huart->State = HAL_UART_STATE_BUSY; 00195 00196 /* Disable the Peripheral */ 00197 __HAL_UART_DISABLE(huart); 00198 00199 /* Set the UART Communication parameters */ 00200 if (UART_SetConfig(huart) == HAL_ERROR) 00201 { 00202 return HAL_ERROR; 00203 } 00204 00205 if(huart->AdvancedInit.AdvFeatureInit != UART_ADVFEATURE_NO_INIT) 00206 { 00207 UART_AdvFeatureConfig(huart); 00208 } 00209 00210 /* Enable the Driver Enable mode by setting the DEM bit in the CR3 register */ 00211 SET_BIT(huart->Instance->CR3, USART_CR3_DEM); 00212 00213 /* Set the Driver Enable polarity */ 00214 MODIFY_REG(huart->Instance->CR3, USART_CR3_DEP, Polarity); 00215 00216 /* Set the Driver Enable assertion and deassertion times */ 00217 temp = (AssertionTime << UART_CR1_DEAT_ADDRESS_LSB_POS); 00218 temp |= (DeassertionTime << UART_CR1_DEDT_ADDRESS_LSB_POS); 00219 MODIFY_REG(huart->Instance->CR1, (USART_CR1_DEDT|USART_CR1_DEAT), temp); 00220 00221 /* Enable the Peripheral */ 00222 __HAL_UART_ENABLE(huart); 00223 00224 /* TEACK and/or REACK to check before moving huart->State to Ready */ 00225 return (UART_CheckIdleState(huart)); 00226 } 00227 00228 00229 /** 00230 * @} 00231 */ 00232 00233 /** @defgroup UARTEx_Exported_Functions_Group3 Peripheral Control functions 00234 * @brief Extended Peripheral Control functions 00235 * 00236 @verbatim 00237 =============================================================================== 00238 ##### Peripheral Control functions ##### 00239 =============================================================================== 00240 [..] This section provides the following functions: 00241 (+) HAL_UARTEx_EnableClockStopMode() API enables the UART clock (HSI or LSE only) during stop mode 00242 (+) HAL_UARTEx_DisableClockStopMode() API disables the above functionality 00243 (+) HAL_MultiProcessorEx_AddressLength_Set() API optionally sets the UART node address 00244 detection length to more than 4 bits for multiprocessor address mark wake up. 00245 (+) HAL_UARTEx_StopModeWakeUpSourceConfig() API defines the wake-up from stop mode 00246 trigger: address match, Start Bit detection or RXNE bit status. 00247 (+) HAL_UARTEx_EnableStopMode() API enables the UART to wake up the MCU from stop mode 00248 (+) HAL_UARTEx_DisableStopMode() API disables the above functionality 00249 (+) HAL_UARTEx_WakeupCallback() called upon UART wakeup interrupt 00250 00251 00252 @endverbatim 00253 * @{ 00254 */ 00255 00256 00257 00258 00259 /** 00260 * @brief By default in multiprocessor mode, when the wake up method is set 00261 * to address mark, the UART handles only 4-bit long addresses detection; 00262 * this API allows to enable longer addresses detection (6-, 7- or 8-bit 00263 * long). 00264 * @note Addresses detection lengths are: 6-bit address detection in 7-bit data mode, 00265 * 7-bit address detection in 8-bit data mode, 8-bit address detection in 9-bit data mode. 00266 * @param huart: UART handle. 00267 * @param AddressLength: this parameter can be one of the following values: 00268 * @arg UART_ADDRESS_DETECT_4B: 4-bit long address 00269 * @arg UART_ADDRESS_DETECT_7B: 6-, 7- or 8-bit long address 00270 * @retval HAL status 00271 */ 00272 HAL_StatusTypeDef HAL_MultiProcessorEx_AddressLength_Set(UART_HandleTypeDef *huart, uint32_t AddressLength) 00273 { 00274 /* Check the UART handle allocation */ 00275 if(huart == NULL) 00276 { 00277 return HAL_ERROR; 00278 } 00279 00280 /* Check the address length parameter */ 00281 assert_param(IS_UART_ADDRESSLENGTH_DETECT(AddressLength)); 00282 00283 huart->State = HAL_UART_STATE_BUSY; 00284 00285 /* Disable the Peripheral */ 00286 __HAL_UART_DISABLE(huart); 00287 00288 /* Set the address length */ 00289 MODIFY_REG(huart->Instance->CR2, USART_CR2_ADDM7, AddressLength); 00290 00291 /* Enable the Peripheral */ 00292 __HAL_UART_ENABLE(huart); 00293 00294 /* TEACK and/or REACK to check before moving huart->State to Ready */ 00295 return (UART_CheckIdleState(huart)); 00296 } 00297 00298 00299 /** 00300 * @brief Set Wakeup from Stop mode interrupt flag selection. 00301 * @param huart: UART handle. 00302 * @param WakeUpSelection: address match, Start Bit detection or RXNE bit status. 00303 * This parameter can be one of the following values: 00304 * @arg UART_WAKEUP_ON_ADDRESS 00305 * @arg UART_WAKEUP_ON_STARTBIT 00306 * @arg UART_WAKEUP_ON_READDATA_NONEMPTY 00307 * @retval HAL status 00308 */ 00309 HAL_StatusTypeDef HAL_UARTEx_StopModeWakeUpSourceConfig(UART_HandleTypeDef *huart, UART_WakeUpTypeDef WakeUpSelection) 00310 { 00311 HAL_StatusTypeDef status = HAL_OK; 00312 00313 /* check the wake-up from stop mode UART instance */ 00314 assert_param(IS_UART_WAKEUP_FROMSTOP_INSTANCE(huart->Instance)); 00315 /* check the wake-up selection parameter */ 00316 assert_param(IS_UART_WAKEUP_SELECTION(WakeUpSelection.WakeUpEvent)); 00317 00318 /* Process Locked */ 00319 __HAL_LOCK(huart); 00320 00321 huart->State = HAL_UART_STATE_BUSY; 00322 00323 /* Disable the Peripheral */ 00324 __HAL_UART_DISABLE(huart); 00325 00326 /* Set the wake-up selection scheme */ 00327 MODIFY_REG(huart->Instance->CR3, USART_CR3_WUS, WakeUpSelection.WakeUpEvent); 00328 00329 if (WakeUpSelection.WakeUpEvent == UART_WAKEUP_ON_ADDRESS) 00330 { 00331 UARTEx_Wakeup_AddressConfig(huart, WakeUpSelection); 00332 } 00333 00334 /* Enable the Peripheral */ 00335 __HAL_UART_ENABLE(huart); 00336 00337 /* Wait until REACK flag is set */ 00338 if(UART_WaitOnFlagUntilTimeout(huart, USART_ISR_REACK, RESET, HAL_UART_TIMEOUT_VALUE) != HAL_OK) 00339 { 00340 status = HAL_TIMEOUT; 00341 } 00342 else 00343 { 00344 /* Initialize the UART State */ 00345 huart->State = HAL_UART_STATE_READY; 00346 } 00347 00348 /* Process Unlocked */ 00349 __HAL_UNLOCK(huart); 00350 00351 return status; 00352 } 00353 00354 00355 /** 00356 * @brief Enable UART Stop Mode. 00357 * @note The UART is able to wake up the MCU from Stop 1 mode as long as UART clock is HSI or LSE. 00358 * @param huart: UART handle. 00359 * @retval HAL status 00360 */ 00361 HAL_StatusTypeDef HAL_UARTEx_EnableStopMode(UART_HandleTypeDef *huart) 00362 { 00363 /* Process Locked */ 00364 __HAL_LOCK(huart); 00365 00366 huart->State = HAL_UART_STATE_BUSY; 00367 00368 /* Set UESM bit */ 00369 SET_BIT(huart->Instance->CR1, USART_CR1_UESM); 00370 00371 huart->State = HAL_UART_STATE_READY; 00372 00373 /* Process Unlocked */ 00374 __HAL_UNLOCK(huart); 00375 00376 return HAL_OK; 00377 } 00378 00379 /** 00380 * @brief Disable UART Stop Mode. 00381 * @param huart: UART handle. 00382 * @retval HAL status 00383 */ 00384 HAL_StatusTypeDef HAL_UARTEx_DisableStopMode(UART_HandleTypeDef *huart) 00385 { 00386 /* Process Locked */ 00387 __HAL_LOCK(huart); 00388 00389 huart->State = HAL_UART_STATE_BUSY; 00390 00391 /* Clear UESM bit */ 00392 CLEAR_BIT(huart->Instance->CR1, USART_CR1_UESM); 00393 00394 huart->State = HAL_UART_STATE_READY; 00395 00396 /* Process Unlocked */ 00397 __HAL_UNLOCK(huart); 00398 00399 return HAL_OK; 00400 } 00401 00402 /** 00403 * @brief UART wakeup from Stop mode callback. 00404 * @param huart: UART handle. 00405 * @retval None 00406 */ 00407 __weak void HAL_UARTEx_WakeupCallback(UART_HandleTypeDef *huart) 00408 { 00409 /* NOTE : This function should not be modified, when the callback is needed, 00410 the HAL_UARTEx_WakeupCallback can be implemented in the user file. 00411 */ 00412 } 00413 00414 /** 00415 * @} 00416 */ 00417 00418 /** 00419 * @} 00420 */ 00421 00422 /** @addtogroup UARTEx_Private_Functions 00423 * @{ 00424 */ 00425 00426 /** 00427 * @brief Initialize the UART wake-up from stop mode parameters when triggered by address detection. 00428 * @param huart: UART handle. 00429 * @param WakeUpSelection: UART wake up from stop mode parameters. 00430 * @retval None 00431 */ 00432 static void UARTEx_Wakeup_AddressConfig(UART_HandleTypeDef *huart, UART_WakeUpTypeDef WakeUpSelection) 00433 { 00434 assert_param(IS_UART_ADDRESSLENGTH_DETECT(WakeUpSelection.AddressLength)); 00435 00436 /* Set the USART address length */ 00437 MODIFY_REG(huart->Instance->CR2, USART_CR2_ADDM7, WakeUpSelection.AddressLength); 00438 00439 /* Set the USART address node */ 00440 MODIFY_REG(huart->Instance->CR2, USART_CR2_ADD, ((uint32_t)WakeUpSelection.Address << UART_CR2_ADDRESS_LSB_POS)); 00441 } 00442 00443 /** 00444 * @} 00445 */ 00446 00447 #endif /* HAL_UART_MODULE_ENABLED */ 00448 00449 /** 00450 * @} 00451 */ 00452 00453 /** 00454 * @} 00455 */ 00456 00457 /************************ (C) COPYRIGHT STMicroelectronics *****END OF FILE****/ 00458
Generated on Tue Jul 12 2022 11:35:15 by
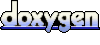