Hal Drivers for L4
Dependents: BSP OneHopeOnePrayer FINAL_AUDIO_RECORD AudioDemo
Fork of STM32L4xx_HAL_Driver by
stm32l4xx_hal_uart.h
00001 /** 00002 ****************************************************************************** 00003 * @file stm32l4xx_hal_uart.h 00004 * @author MCD Application Team 00005 * @version V1.1.0 00006 * @date 16-September-2015 00007 * @brief Header file of UART HAL module. 00008 ****************************************************************************** 00009 * @attention 00010 * 00011 * <h2><center>© COPYRIGHT(c) 2015 STMicroelectronics</center></h2> 00012 * 00013 * Redistribution and use in source and binary forms, with or without modification, 00014 * are permitted provided that the following conditions are met: 00015 * 1. Redistributions of source code must retain the above copyright notice, 00016 * this list of conditions and the following disclaimer. 00017 * 2. Redistributions in binary form must reproduce the above copyright notice, 00018 * this list of conditions and the following disclaimer in the documentation 00019 * and/or other materials provided with the distribution. 00020 * 3. Neither the name of STMicroelectronics nor the names of its contributors 00021 * may be used to endorse or promote products derived from this software 00022 * without specific prior written permission. 00023 * 00024 * THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" 00025 * AND ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE 00026 * IMPLIED WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE 00027 * DISCLAIMED. IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE 00028 * FOR ANY DIRECT, INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL 00029 * DAMAGES (INCLUDING, BUT NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR 00030 * SERVICES; LOSS OF USE, DATA, OR PROFITS; OR BUSINESS INTERRUPTION) HOWEVER 00031 * CAUSED AND ON ANY THEORY OF LIABILITY, WHETHER IN CONTRACT, STRICT LIABILITY, 00032 * OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) ARISING IN ANY WAY OUT OF THE USE 00033 * OF THIS SOFTWARE, EVEN IF ADVISED OF THE POSSIBILITY OF SUCH DAMAGE. 00034 * 00035 ****************************************************************************** 00036 */ 00037 00038 /* Define to prevent recursive inclusion -------------------------------------*/ 00039 #ifndef __STM32L4xx_HAL_UART_H 00040 #define __STM32L4xx_HAL_UART_H 00041 00042 #ifdef __cplusplus 00043 extern "C" { 00044 #endif 00045 00046 /* Includes ------------------------------------------------------------------*/ 00047 #include "stm32l4xx_hal_def.h" 00048 00049 /** @addtogroup STM32L4xx_HAL_Driver 00050 * @{ 00051 */ 00052 00053 /** @addtogroup UART 00054 * @{ 00055 */ 00056 00057 /* Exported types ------------------------------------------------------------*/ 00058 /** @defgroup UART_Exported_Types UART Exported Types 00059 * @{ 00060 */ 00061 00062 /** 00063 * @brief UART Init Structure definition 00064 */ 00065 typedef struct 00066 { 00067 uint32_t BaudRate; /*!< This member configures the UART communication baud rate. 00068 The baud rate register is computed using the following formula: 00069 - If oversampling is 16 or in LIN mode, 00070 Baud Rate Register = ((PCLKx) / ((huart->Init.BaudRate))) 00071 - If oversampling is 8, 00072 - - Baud Rate Register[15:4] = ((2 * PCLKx) / ((huart->Init.BaudRate)))[15:4] 00073 - - Baud Rate Register[3] = 0 00074 - - Baud Rate Register[2:0] = (((2 * PCLKx) / ((huart->Init.BaudRate)))[3:0]) >> 1 */ 00075 00076 uint32_t WordLength; /*!< Specifies the number of data bits transmitted or received in a frame. 00077 This parameter can be a value of @ref UARTEx_Word_Length. */ 00078 00079 uint32_t StopBits; /*!< Specifies the number of stop bits transmitted. 00080 This parameter can be a value of @ref UART_Stop_Bits. */ 00081 00082 uint32_t Parity; /*!< Specifies the parity mode. 00083 This parameter can be a value of @ref UART_Parity 00084 @note When parity is enabled, the computed parity is inserted 00085 at the MSB position of the transmitted data (9th bit when 00086 the word length is set to 9 data bits; 8th bit when the 00087 word length is set to 8 data bits). */ 00088 00089 uint32_t Mode; /*!< Specifies whether the Receive or Transmit mode is enabled or disabled. 00090 This parameter can be a value of @ref UART_Mode. */ 00091 00092 uint32_t HwFlowCtl; /*!< Specifies whether the hardware flow control mode is enabled 00093 or disabled. 00094 This parameter can be a value of @ref UART_Hardware_Flow_Control. */ 00095 00096 uint32_t OverSampling; /*!< Specifies whether the Over sampling 8 is enabled or disabled, to achieve higher speed (up to f_PCLK/8). 00097 This parameter can be a value of @ref UART_Over_Sampling. */ 00098 00099 uint32_t OneBitSampling; /*!< Specifies whether a single sample or three samples' majority vote is selected. 00100 Selecting the single sample method increases the receiver tolerance to clock 00101 deviations. This parameter can be a value of @ref UART_OneBit_Sampling. */ 00102 }UART_InitTypeDef; 00103 00104 /** 00105 * @brief UART Advanced Features initalization structure definition 00106 */ 00107 typedef struct 00108 { 00109 uint32_t AdvFeatureInit; /*!< Specifies which advanced UART features is initialized. Several 00110 Advanced Features may be initialized at the same time . 00111 This parameter can be a value of @ref UART_Advanced_Features_Initialization_Type. */ 00112 00113 uint32_t TxPinLevelInvert; /*!< Specifies whether the TX pin active level is inverted. 00114 This parameter can be a value of @ref UART_Tx_Inv. */ 00115 00116 uint32_t RxPinLevelInvert; /*!< Specifies whether the RX pin active level is inverted. 00117 This parameter can be a value of @ref UART_Rx_Inv. */ 00118 00119 uint32_t DataInvert; /*!< Specifies whether data are inverted (positive/direct logic 00120 vs negative/inverted logic). 00121 This parameter can be a value of @ref UART_Data_Inv. */ 00122 00123 uint32_t Swap; /*!< Specifies whether TX and RX pins are swapped. 00124 This parameter can be a value of @ref UART_Rx_Tx_Swap. */ 00125 00126 uint32_t OverrunDisable; /*!< Specifies whether the reception overrun detection is disabled. 00127 This parameter can be a value of @ref UART_Overrun_Disable. */ 00128 00129 uint32_t DMADisableonRxError; /*!< Specifies whether the DMA is disabled in case of reception error. 00130 This parameter can be a value of @ref UART_DMA_Disable_on_Rx_Error. */ 00131 00132 uint32_t AutoBaudRateEnable; /*!< Specifies whether auto Baud rate detection is enabled. 00133 This parameter can be a value of @ref UART_AutoBaudRate_Enable */ 00134 00135 uint32_t AutoBaudRateMode; /*!< If auto Baud rate detection is enabled, specifies how the rate 00136 detection is carried out. 00137 This parameter can be a value of @ref UART_AutoBaud_Rate_Mode. */ 00138 00139 uint32_t MSBFirst; /*!< Specifies whether MSB is sent first on UART line. 00140 This parameter can be a value of @ref UART_MSB_First. */ 00141 } UART_AdvFeatureInitTypeDef; 00142 00143 00144 00145 /** 00146 * @brief HAL UART State structures definition 00147 */ 00148 typedef enum 00149 { 00150 HAL_UART_STATE_RESET = 0x00, /*!< Peripheral is not initialized */ 00151 HAL_UART_STATE_READY = 0x01, /*!< Peripheral Initialized and ready for use */ 00152 HAL_UART_STATE_BUSY = 0x02, /*!< an internal process is ongoing */ 00153 HAL_UART_STATE_BUSY_TX = 0x12, /*!< Data Transmission process is ongoing */ 00154 HAL_UART_STATE_BUSY_RX = 0x22, /*!< Data Reception process is ongoing */ 00155 HAL_UART_STATE_BUSY_TX_RX = 0x32, /*!< Data Transmission and Reception process is ongoing */ 00156 HAL_UART_STATE_TIMEOUT = 0x03, /*!< Timeout state */ 00157 HAL_UART_STATE_ERROR = 0x04 /*!< Error */ 00158 }HAL_UART_StateTypeDef; 00159 00160 /** 00161 * @brief HAL UART Error Code structure definition 00162 */ 00163 typedef enum 00164 { 00165 HAL_UART_ERROR_NONE = 0x00, /*!< No error */ 00166 HAL_UART_ERROR_PE = 0x01, /*!< Parity error */ 00167 HAL_UART_ERROR_NE = 0x02, /*!< Noise error */ 00168 HAL_UART_ERROR_FE = 0x04, /*!< frame error */ 00169 HAL_UART_ERROR_ORE = 0x08, /*!< Overrun error */ 00170 HAL_UART_ERROR_DMA = 0x10 /*!< DMA transfer error */ 00171 }HAL_UART_ErrorTypeDef; 00172 00173 /** 00174 * @brief UART clock sources definition 00175 */ 00176 typedef enum 00177 { 00178 UART_CLOCKSOURCE_PCLK1 = 0x00, /*!< PCLK1 clock source */ 00179 UART_CLOCKSOURCE_PCLK2 = 0x01, /*!< PCLK2 clock source */ 00180 UART_CLOCKSOURCE_HSI = 0x02, /*!< HSI clock source */ 00181 UART_CLOCKSOURCE_SYSCLK = 0x04, /*!< SYSCLK clock source */ 00182 UART_CLOCKSOURCE_LSE = 0x08, /*!< LSE clock source */ 00183 UART_CLOCKSOURCE_UNDEFINED = 0x10 /*!< Undefined clock source */ 00184 }UART_ClockSourceTypeDef; 00185 00186 /** 00187 * @brief UART handle Structure definition 00188 */ 00189 typedef struct 00190 { 00191 USART_TypeDef *Instance; /*!< UART registers base address */ 00192 00193 UART_InitTypeDef Init; /*!< UART communication parameters */ 00194 00195 UART_AdvFeatureInitTypeDef AdvancedInit; /*!< UART Advanced Features initialization parameters */ 00196 00197 uint8_t *pTxBuffPtr; /*!< Pointer to UART Tx transfer Buffer */ 00198 00199 uint16_t TxXferSize; /*!< UART Tx Transfer size */ 00200 00201 uint16_t TxXferCount; /*!< UART Tx Transfer Counter */ 00202 00203 uint8_t *pRxBuffPtr; /*!< Pointer to UART Rx transfer Buffer */ 00204 00205 uint16_t RxXferSize; /*!< UART Rx Transfer size */ 00206 00207 uint16_t RxXferCount; /*!< UART Rx Transfer Counter */ 00208 00209 uint16_t Mask; /*!< UART Rx RDR register mask */ 00210 00211 DMA_HandleTypeDef *hdmatx; /*!< UART Tx DMA Handle parameters */ 00212 00213 DMA_HandleTypeDef *hdmarx; /*!< UART Rx DMA Handle parameters */ 00214 00215 HAL_LockTypeDef Lock; /*!< Locking object */ 00216 00217 __IO HAL_UART_StateTypeDef State; /*!< UART communication state */ 00218 00219 __IO uint32_t ErrorCode; /*!< UART Error code */ 00220 00221 }UART_HandleTypeDef; 00222 00223 /** 00224 * @} 00225 */ 00226 00227 /* Exported constants --------------------------------------------------------*/ 00228 /** @defgroup UART_Exported_Constants UART Exported Constants 00229 * @{ 00230 */ 00231 00232 /** @defgroup UART_Stop_Bits UART Number of Stop Bits 00233 * @{ 00234 */ 00235 #define UART_STOPBITS_1 ((uint32_t)0x00000000) /*!< UART frame with 1 stop bit */ 00236 #define UART_STOPBITS_1_5 ((uint32_t)(USART_CR2_STOP_0 | USART_CR2_STOP_1)) /*!< UART frame with 1.5 stop bits */ 00237 #define UART_STOPBITS_2 ((uint32_t)USART_CR2_STOP_1) /*!< UART frame with 2 stop bits */ 00238 /** 00239 * @} 00240 */ 00241 00242 /** @defgroup UART_Parity UART Parity 00243 * @{ 00244 */ 00245 #define UART_PARITY_NONE ((uint32_t)0x00000000) /*!< No parity */ 00246 #define UART_PARITY_EVEN ((uint32_t)USART_CR1_PCE) /*!< Even parity */ 00247 #define UART_PARITY_ODD ((uint32_t)(USART_CR1_PCE | USART_CR1_PS)) /*!< Odd parity */ 00248 /** 00249 * @} 00250 */ 00251 00252 /** @defgroup UART_Hardware_Flow_Control UART Hardware Flow Control 00253 * @{ 00254 */ 00255 #define UART_HWCONTROL_NONE ((uint32_t)0x00000000) /*!< No hardware control */ 00256 #define UART_HWCONTROL_RTS ((uint32_t)USART_CR3_RTSE) /*!< Request To Send */ 00257 #define UART_HWCONTROL_CTS ((uint32_t)USART_CR3_CTSE) /*!< Clear To Send */ 00258 #define UART_HWCONTROL_RTS_CTS ((uint32_t)(USART_CR3_RTSE | USART_CR3_CTSE)) /*!< Request and Clear To Send */ 00259 /** 00260 * @} 00261 */ 00262 00263 /** @defgroup UART_Mode UART Transfer Mode 00264 * @{ 00265 */ 00266 #define UART_MODE_RX ((uint32_t)USART_CR1_RE) /*!< RX mode */ 00267 #define UART_MODE_TX ((uint32_t)USART_CR1_TE) /*!< TX mode */ 00268 #define UART_MODE_TX_RX ((uint32_t)(USART_CR1_TE |USART_CR1_RE)) /*!< RX and TX mode */ 00269 /** 00270 * @} 00271 */ 00272 00273 /** @defgroup UART_State UART State 00274 * @{ 00275 */ 00276 #define UART_STATE_DISABLE ((uint32_t)0x00000000) /*!< UART disabled */ 00277 #define UART_STATE_ENABLE ((uint32_t)USART_CR1_UE) /*!< UART enabled */ 00278 /** 00279 * @} 00280 */ 00281 00282 /** @defgroup UART_Over_Sampling UART Over Sampling 00283 * @{ 00284 */ 00285 #define UART_OVERSAMPLING_16 ((uint32_t)0x00000000) /*!< Oversampling by 16 */ 00286 #define UART_OVERSAMPLING_8 ((uint32_t)USART_CR1_OVER8) /*!< Oversampling by 8 */ 00287 /** 00288 * @} 00289 */ 00290 00291 /** @defgroup UART_OneBit_Sampling UART One Bit Sampling Method 00292 * @{ 00293 */ 00294 #define UART_ONE_BIT_SAMPLE_DISABLE ((uint32_t)0x00000000) /*!< One-bit sampling disable */ 00295 #define UART_ONE_BIT_SAMPLE_ENABLE ((uint32_t)USART_CR3_ONEBIT) /*!< One-bit sampling enable */ 00296 /** 00297 * @} 00298 */ 00299 00300 /** @defgroup UART_AutoBaud_Rate_Mode UART Advanced Feature AutoBaud Rate Mode 00301 * @{ 00302 */ 00303 #define UART_ADVFEATURE_AUTOBAUDRATE_ONSTARTBIT ((uint32_t)0x0000) /*!< Auto Baud rate detection on start bit */ 00304 #define UART_ADVFEATURE_AUTOBAUDRATE_ONFALLINGEDGE ((uint32_t)USART_CR2_ABRMODE_0) /*!< Auto Baud rate detection on falling edge */ 00305 #define UART_ADVFEATURE_AUTOBAUDRATE_ON0X7FFRAME ((uint32_t)USART_CR2_ABRMODE_1) /*!< Auto Baud rate detection on 0x7F frame detection */ 00306 #define UART_ADVFEATURE_AUTOBAUDRATE_ON0X55FRAME ((uint32_t)USART_CR2_ABRMODE) /*!< Auto Baud rate detection on 0x55 frame detection */ 00307 /** 00308 * @} 00309 */ 00310 00311 /** @defgroup UART_Receiver_TimeOut UART Receiver TimeOut 00312 * @{ 00313 */ 00314 #define UART_RECEIVER_TIMEOUT_DISABLE ((uint32_t)0x00000000) /*!< UART receiver timeout disable */ 00315 #define UART_RECEIVER_TIMEOUT_ENABLE ((uint32_t)USART_CR2_RTOEN) /*!< UART receiver timeout enable */ 00316 /** 00317 * @} 00318 */ 00319 00320 /** @defgroup UART_LIN UART Local Interconnection Network mode 00321 * @{ 00322 */ 00323 #define UART_LIN_DISABLE ((uint32_t)0x00000000) /*!< Local Interconnect Network disable */ 00324 #define UART_LIN_ENABLE ((uint32_t)USART_CR2_LINEN) /*!< Local Interconnect Network enable */ 00325 /** 00326 * @} 00327 */ 00328 00329 /** @defgroup UART_LIN_Break_Detection UART LIN Break Detection 00330 * @{ 00331 */ 00332 #define UART_LINBREAKDETECTLENGTH_10B ((uint32_t)0x00000000) /*!< LIN 10-bit break detection length */ 00333 #define UART_LINBREAKDETECTLENGTH_11B ((uint32_t)USART_CR2_LBDL) /*!< LIN 11-bit break detection length */ 00334 /** 00335 * @} 00336 */ 00337 00338 /** @defgroup UART_DMA_Tx UART DMA Tx 00339 * @{ 00340 */ 00341 #define UART_DMA_TX_DISABLE ((uint32_t)0x00000000) /*!< UART DMA TX disabled */ 00342 #define UART_DMA_TX_ENABLE ((uint32_t)USART_CR3_DMAT) /*!< UART DMA TX enabled */ 00343 /** 00344 * @} 00345 */ 00346 00347 /** @defgroup UART_DMA_Rx UART DMA Rx 00348 * @{ 00349 */ 00350 #define UART_DMA_RX_DISABLE ((uint32_t)0x0000) /*!< UART DMA RX disabled */ 00351 #define UART_DMA_RX_ENABLE ((uint32_t)USART_CR3_DMAR) /*!< UART DMA RX enabled */ 00352 /** 00353 * @} 00354 */ 00355 00356 /** @defgroup UART_Half_Duplex_Selection UART Half Duplex Selection 00357 * @{ 00358 */ 00359 #define UART_HALF_DUPLEX_DISABLE ((uint32_t)0x0000) /*!< UART half-duplex disabled */ 00360 #define UART_HALF_DUPLEX_ENABLE ((uint32_t)USART_CR3_HDSEL) /*!< UART half-duplex enabled */ 00361 /** 00362 * @} 00363 */ 00364 00365 /** @defgroup UART_WakeUp_Methods UART WakeUp Methods 00366 * @{ 00367 */ 00368 #define UART_WAKEUPMETHOD_IDLELINE ((uint32_t)0x00000000) /*!< UART wake-up on idle line */ 00369 #define UART_WAKEUPMETHOD_ADDRESSMARK ((uint32_t)USART_CR1_WAKE) /*!< UART wake-up on address mark */ 00370 /** 00371 * @} 00372 */ 00373 00374 /** @defgroup UART_Request_Parameters UART Request Parameters 00375 * @{ 00376 */ 00377 #define UART_AUTOBAUD_REQUEST ((uint32_t)USART_RQR_ABRRQ) /*!< Auto-Baud Rate Request */ 00378 #define UART_SENDBREAK_REQUEST ((uint32_t)USART_RQR_SBKRQ) /*!< Send Break Request */ 00379 #define UART_MUTE_MODE_REQUEST ((uint32_t)USART_RQR_MMRQ) /*!< Mute Mode Request */ 00380 #define UART_RXDATA_FLUSH_REQUEST ((uint32_t)USART_RQR_RXFRQ) /*!< Receive Data flush Request */ 00381 #define UART_TXDATA_FLUSH_REQUEST ((uint32_t)USART_RQR_TXFRQ) /*!< Transmit data flush Request */ 00382 /** 00383 * @} 00384 */ 00385 00386 /** @defgroup UART_Advanced_Features_Initialization_Type UART Advanced Feature Initialization Type 00387 * @{ 00388 */ 00389 #define UART_ADVFEATURE_NO_INIT ((uint32_t)0x00000000) /*!< No advanced feature initialization */ 00390 #define UART_ADVFEATURE_TXINVERT_INIT ((uint32_t)0x00000001) /*!< TX pin active level inversion */ 00391 #define UART_ADVFEATURE_RXINVERT_INIT ((uint32_t)0x00000002) /*!< RX pin active level inversion */ 00392 #define UART_ADVFEATURE_DATAINVERT_INIT ((uint32_t)0x00000004) /*!< Binary data inversion */ 00393 #define UART_ADVFEATURE_SWAP_INIT ((uint32_t)0x00000008) /*!< TX/RX pins swap */ 00394 #define UART_ADVFEATURE_RXOVERRUNDISABLE_INIT ((uint32_t)0x00000010) /*!< RX overrun disable */ 00395 #define UART_ADVFEATURE_DMADISABLEONERROR_INIT ((uint32_t)0x00000020) /*!< DMA disable on Reception Error */ 00396 #define UART_ADVFEATURE_AUTOBAUDRATE_INIT ((uint32_t)0x00000040) /*!< Auto Baud rate detection initialization */ 00397 #define UART_ADVFEATURE_MSBFIRST_INIT ((uint32_t)0x00000080) /*!< Most significant bit sent/received first */ 00398 /** 00399 * @} 00400 */ 00401 00402 /** @defgroup UART_Tx_Inv UART Advanced Feature TX Pin Active Level Inversion 00403 * @{ 00404 */ 00405 #define UART_ADVFEATURE_TXINV_DISABLE ((uint32_t)0x00000000) /*!< TX pin active level inversion disable */ 00406 #define UART_ADVFEATURE_TXINV_ENABLE ((uint32_t)USART_CR2_TXINV) /*!< TX pin active level inversion enable */ 00407 /** 00408 * @} 00409 */ 00410 00411 /** @defgroup UART_Rx_Inv UART Advanced Feature RX Pin Active Level Inversion 00412 * @{ 00413 */ 00414 #define UART_ADVFEATURE_RXINV_DISABLE ((uint32_t)0x00000000) /*!< RX pin active level inversion disable */ 00415 #define UART_ADVFEATURE_RXINV_ENABLE ((uint32_t)USART_CR2_RXINV) /*!< RX pin active level inversion enable */ 00416 /** 00417 * @} 00418 */ 00419 00420 /** @defgroup UART_Data_Inv UART Advanced Feature Binary Data Inversion 00421 * @{ 00422 */ 00423 #define UART_ADVFEATURE_DATAINV_DISABLE ((uint32_t)0x00000000) /*!< Binary data inversion disable */ 00424 #define UART_ADVFEATURE_DATAINV_ENABLE ((uint32_t)USART_CR2_DATAINV) /*!< Binary data inversion enable */ 00425 /** 00426 * @} 00427 */ 00428 00429 /** @defgroup UART_Rx_Tx_Swap UART Advanced Feature RX TX Pins Swap 00430 * @{ 00431 */ 00432 #define UART_ADVFEATURE_SWAP_DISABLE ((uint32_t)0x00000000) /*!< TX/RX pins swap disable */ 00433 #define UART_ADVFEATURE_SWAP_ENABLE ((uint32_t)USART_CR2_SWAP) /*!< TX/RX pins swap enable */ 00434 /** 00435 * @} 00436 */ 00437 00438 /** @defgroup UART_Overrun_Disable UART Advanced Feature Overrun Disable 00439 * @{ 00440 */ 00441 #define UART_ADVFEATURE_OVERRUN_ENABLE ((uint32_t)0x00000000) /*!< RX overrun enable */ 00442 #define UART_ADVFEATURE_OVERRUN_DISABLE ((uint32_t)USART_CR3_OVRDIS) /*!< RX overrun disable */ 00443 /** 00444 * @} 00445 */ 00446 00447 /** @defgroup UART_AutoBaudRate_Enable UART Advanced Feature Auto BaudRate Enable 00448 * @{ 00449 */ 00450 #define UART_ADVFEATURE_AUTOBAUDRATE_DISABLE ((uint32_t)0x00000000) /*!< RX Auto Baud rate detection enable */ 00451 #define UART_ADVFEATURE_AUTOBAUDRATE_ENABLE ((uint32_t)USART_CR2_ABREN) /*!< RX Auto Baud rate detection disable */ 00452 /** 00453 * @} 00454 */ 00455 00456 /** @defgroup UART_DMA_Disable_on_Rx_Error UART Advanced Feature DMA Disable On Rx Error 00457 * @{ 00458 */ 00459 #define UART_ADVFEATURE_DMA_ENABLEONRXERROR ((uint32_t)0x00000000) /*!< DMA enable on Reception Error */ 00460 #define UART_ADVFEATURE_DMA_DISABLEONRXERROR ((uint32_t)USART_CR3_DDRE) /*!< DMA disable on Reception Error */ 00461 /** 00462 * @} 00463 */ 00464 00465 /** @defgroup UART_MSB_First UART Advanced Feature MSB First 00466 * @{ 00467 */ 00468 #define UART_ADVFEATURE_MSBFIRST_DISABLE ((uint32_t)0x00000000) /*!< Most significant bit sent/received first disable */ 00469 #define UART_ADVFEATURE_MSBFIRST_ENABLE ((uint32_t)USART_CR2_MSBFIRST) /*!< Most significant bit sent/received first enable */ 00470 /** 00471 * @} 00472 */ 00473 00474 /** @defgroup UART_Stop_Mode_Enable UART Advanced Feature Stop Mode Enable 00475 * @{ 00476 */ 00477 #define UART_ADVFEATURE_STOPMODE_DISABLE ((uint32_t)0x00000000) /*!< UART stop mode disable */ 00478 #define UART_ADVFEATURE_STOPMODE_ENABLE ((uint32_t)USART_CR1_UESM) /*!< UART stop mode enable */ 00479 /** 00480 * @} 00481 */ 00482 00483 /** @defgroup UART_Mute_Mode UART Advanced Feature Mute Mode Enable 00484 * @{ 00485 */ 00486 #define UART_ADVFEATURE_MUTEMODE_DISABLE ((uint32_t)0x00000000) /*!< UART mute mode disable */ 00487 #define UART_ADVFEATURE_MUTEMODE_ENABLE ((uint32_t)USART_CR1_MME) /*!< UART mute mode enable */ 00488 /** 00489 * @} 00490 */ 00491 00492 /** @defgroup UART_CR2_ADDRESS_LSB_POS UART Address-matching LSB Position In CR2 Register 00493 * @{ 00494 */ 00495 #define UART_CR2_ADDRESS_LSB_POS ((uint32_t) 24) /*!< UART address-matching LSB position in CR2 register */ 00496 /** 00497 * @} 00498 */ 00499 00500 /** @defgroup UART_WakeUp_from_Stop_Selection UART WakeUp From Stop Selection 00501 * @{ 00502 */ 00503 #define UART_WAKEUP_ON_ADDRESS ((uint32_t)0x00000000) /*!< UART wake-up on address */ 00504 #define UART_WAKEUP_ON_STARTBIT ((uint32_t)USART_CR3_WUS_1) /*!< UART wake-up on start bit */ 00505 #define UART_WAKEUP_ON_READDATA_NONEMPTY ((uint32_t)USART_CR3_WUS) /*!< UART wake-up on receive data register not empty */ 00506 /** 00507 * @} 00508 */ 00509 00510 /** @defgroup UART_DriverEnable_Polarity UART DriverEnable Polarity 00511 * @{ 00512 */ 00513 #define UART_DE_POLARITY_HIGH ((uint32_t)0x00000000) /*!< Driver enable signal is active high */ 00514 #define UART_DE_POLARITY_LOW ((uint32_t)USART_CR3_DEP) /*!< Driver enable signal is active low */ 00515 /** 00516 * @} 00517 */ 00518 00519 /** @defgroup UART_CR1_DEAT_ADDRESS_LSB_POS UART Driver Enable Assertion Time LSB Position In CR1 Register 00520 * @{ 00521 */ 00522 #define UART_CR1_DEAT_ADDRESS_LSB_POS ((uint32_t) 21) /*!< UART Driver Enable assertion time LSB position in CR1 register */ 00523 /** 00524 * @} 00525 */ 00526 00527 /** @defgroup UART_CR1_DEDT_ADDRESS_LSB_POS UART Driver Enable DeAssertion Time LSB Position In CR1 Register 00528 * @{ 00529 */ 00530 #define UART_CR1_DEDT_ADDRESS_LSB_POS ((uint32_t) 16) /*!< UART Driver Enable de-assertion time LSB position in CR1 register */ 00531 /** 00532 * @} 00533 */ 00534 00535 /** @defgroup UART_Interruption_Mask UART Interruptions Flag Mask 00536 * @{ 00537 */ 00538 #define UART_IT_MASK ((uint32_t)0x001F) /*!< UART interruptions flags mask */ 00539 /** 00540 * @} 00541 */ 00542 00543 /** @defgroup UART_TimeOut_Value UART polling-based communications time-out value 00544 * @{ 00545 */ 00546 #define HAL_UART_TIMEOUT_VALUE 0x1FFFFFF /*!< UART polling-based communications time-out value */ 00547 /** 00548 * @} 00549 */ 00550 00551 /** @defgroup UART_Flags UART Status Flags 00552 * Elements values convention: 0xXXXX 00553 * - 0xXXXX : Flag mask in the ISR register 00554 * @{ 00555 */ 00556 #define UART_FLAG_REACK ((uint32_t)0x00400000) /*!< UART receive enable acknowledge flag */ 00557 #define UART_FLAG_TEACK ((uint32_t)0x00200000) /*!< UART transmit enable acknowledge flag */ 00558 #define UART_FLAG_WUF ((uint32_t)0x00100000) /*!< UART wake-up from stop mode flag */ 00559 #define UART_FLAG_RWU ((uint32_t)0x00080000) /*!< UART receiver wake-up from mute mode flag */ 00560 #define UART_FLAG_SBKF ((uint32_t)0x00040000) /*!< UART send break flag */ 00561 #define UART_FLAG_CMF ((uint32_t)0x00020000) /*!< UART character match flag */ 00562 #define UART_FLAG_BUSY ((uint32_t)0x00010000) /*!< UART busy flag */ 00563 #define UART_FLAG_ABRF ((uint32_t)0x00008000) /*!< UART auto Baud rate flag */ 00564 #define UART_FLAG_ABRE ((uint32_t)0x00004000) /*!< UART uto Baud rate error */ 00565 #define UART_FLAG_EOBF ((uint32_t)0x00001000) /*!< UART end of block flag */ 00566 #define UART_FLAG_RTOF ((uint32_t)0x00000800) /*!< UART receiver timeout flag */ 00567 #define UART_FLAG_CTS ((uint32_t)0x00000400) /*!< UART clear to send flag */ 00568 #define UART_FLAG_CTSIF ((uint32_t)0x00000200) /*!< UART clear to send interrupt flag */ 00569 #define UART_FLAG_LBDF ((uint32_t)0x00000100) /*!< UART LIN break detection flag */ 00570 #define UART_FLAG_TXE ((uint32_t)0x00000080) /*!< UART transmit data register empty */ 00571 #define UART_FLAG_TC ((uint32_t)0x00000040) /*!< UART transmission complete */ 00572 #define UART_FLAG_RXNE ((uint32_t)0x00000020) /*!< UART read data register not empty */ 00573 #define UART_FLAG_IDLE ((uint32_t)0x00000010) /*!< UART idle flag */ 00574 #define UART_FLAG_ORE ((uint32_t)0x00000008) /*!< UART overrun error */ 00575 #define UART_FLAG_NE ((uint32_t)0x00000004) /*!< UART noise error */ 00576 #define UART_FLAG_FE ((uint32_t)0x00000002) /*!< UART frame error */ 00577 #define UART_FLAG_PE ((uint32_t)0x00000001) /*!< UART parity error */ 00578 /** 00579 * @} 00580 */ 00581 00582 /** @defgroup UART_Interrupt_definition UART Interrupts Definition 00583 * Elements values convention: 000ZZZZZ0XXYYYYYb 00584 * - YYYYY : Interrupt source position in the XX register (5bits) 00585 * - XX : Interrupt source register (2bits) 00586 * - 01: CR1 register 00587 * - 10: CR2 register 00588 * - 11: CR3 register 00589 * - ZZZZZ : Flag position in the ISR register(5bits) 00590 * @{ 00591 */ 00592 #define UART_IT_PE ((uint32_t)0x0028) /*!< UART parity error interruption */ 00593 #define UART_IT_TXE ((uint32_t)0x0727) /*!< UART transmit data register empty interruption */ 00594 #define UART_IT_TC ((uint32_t)0x0626) /*!< UART transmission complete interruption */ 00595 #define UART_IT_RXNE ((uint32_t)0x0525) /*!< UART read data register not empty interruption */ 00596 #define UART_IT_IDLE ((uint32_t)0x0424) /*!< UART idle interruption */ 00597 #define UART_IT_LBD ((uint32_t)0x0846) /*!< UART LIN break detection interruption */ 00598 #define UART_IT_CTS ((uint32_t)0x096A) /*!< UART CTS interruption */ 00599 #define UART_IT_CM ((uint32_t)0x112E) /*!< UART character match interruption */ 00600 #define UART_IT_WUF ((uint32_t)0x1476) /*!< UART wake-up from stop mode interruption */ 00601 00602 /* Elements values convention: 000000000XXYYYYYb 00603 - YYYYY : Interrupt source position in the XX register (5bits) 00604 - XX : Interrupt source register (2bits) 00605 - 01: CR1 register 00606 - 10: CR2 register 00607 - 11: CR3 register */ 00608 #define UART_IT_ERR ((uint32_t)0x0060) /*!< UART error interruption */ 00609 00610 /* Elements values convention: 0000ZZZZ00000000b 00611 - ZZZZ : Flag position in the ISR register(4bits) */ 00612 #define UART_IT_ORE ((uint32_t)0x0300) /*!< UART overrun error interruption */ 00613 #define UART_IT_NE ((uint32_t)0x0200) /*!< UART noise error interruption */ 00614 #define UART_IT_FE ((uint32_t)0x0100) /*!< UART frame error interruption */ 00615 /** 00616 * @} 00617 */ 00618 00619 /** @defgroup UART_IT_CLEAR_Flags UART Interruption Clear Flags 00620 * @{ 00621 */ 00622 #define UART_CLEAR_PEF USART_ICR_PECF /*!< Parity Error Clear Flag */ 00623 #define UART_CLEAR_FEF USART_ICR_FECF /*!< Framing Error Clear Flag */ 00624 #define UART_CLEAR_NEF USART_ICR_NCF /*!< Noise detected Clear Flag */ 00625 #define UART_CLEAR_OREF USART_ICR_ORECF /*!< OverRun Error Clear Flag */ 00626 #define UART_CLEAR_IDLEF USART_ICR_IDLECF /*!< IDLE line detected Clear Flag */ 00627 #define UART_CLEAR_TCF USART_ICR_TCCF /*!< Transmission Complete Clear Flag */ 00628 #define UART_CLEAR_LBDF USART_ICR_LBDCF /*!< LIN Break Detection Clear Flag */ 00629 #define UART_CLEAR_CTSF USART_ICR_CTSCF /*!< CTS Interrupt Clear Flag */ 00630 #define UART_CLEAR_RTOF USART_ICR_RTOCF /*!< Receiver Time Out Clear Flag */ 00631 #define UART_CLEAR_EOBF USART_ICR_EOBCF /*!< End Of Block Clear Flag */ 00632 #define UART_CLEAR_CMF USART_ICR_CMCF /*!< Character Match Clear Flag */ 00633 #define UART_CLEAR_WUF USART_ICR_WUCF /*!< Wake Up from stop mode Clear Flag */ 00634 /** 00635 * @} 00636 */ 00637 00638 00639 /** 00640 * @} 00641 */ 00642 00643 /* Exported macros -----------------------------------------------------------*/ 00644 /** @defgroup UART_Exported_Macros UART Exported Macros 00645 * @{ 00646 */ 00647 00648 /** @brief Reset UART handle state. 00649 * @param __HANDLE__: UART handle. 00650 * @retval None 00651 */ 00652 #define __HAL_UART_RESET_HANDLE_STATE(__HANDLE__) ((__HANDLE__)->State = HAL_UART_STATE_RESET) 00653 00654 /** @brief Flush the UART Data registers. 00655 * @param __HANDLE__: specifies the UART Handle. 00656 * @retval None 00657 */ 00658 #define __HAL_UART_FLUSH_DRREGISTER(__HANDLE__) \ 00659 do{ \ 00660 SET_BIT((__HANDLE__)->Instance->RQR, UART_RXDATA_FLUSH_REQUEST); \ 00661 SET_BIT((__HANDLE__)->Instance->RQR, UART_TXDATA_FLUSH_REQUEST); \ 00662 } while(0) 00663 00664 /** @brief Clear the specified UART pending flag. 00665 * @param __HANDLE__: specifies the UART Handle. 00666 * @param __FLAG__: specifies the flag to check. 00667 * This parameter can be any combination of the following values: 00668 * @arg UART_CLEAR_PEF, Parity Error Clear Flag 00669 * @arg UART_CLEAR_FEF, Framing Error Clear Flag 00670 * @arg UART_CLEAR_NEF, Noise detected Clear Flag 00671 * @arg UART_CLEAR_OREF, OverRun Error Clear Flag 00672 * @arg UART_CLEAR_IDLEF, IDLE line detected Clear Flag 00673 * @arg UART_CLEAR_TCF, Transmission Complete Clear Flag 00674 * @arg UART_CLEAR_LBDF, LIN Break Detection Clear Flag 00675 * @arg UART_CLEAR_CTSF, CTS Interrupt Clear Flag 00676 * @arg UART_CLEAR_RTOF, Receiver Time Out Clear Flag 00677 * @arg UART_CLEAR_EOBF, End Of Block Clear Flag 00678 * @arg UART_CLEAR_CMF, Character Match Clear Flag 00679 * @arg UART_CLEAR_WUF, Wake Up from stop mode Clear Flag 00680 * @retval None 00681 */ 00682 #define __HAL_UART_CLEAR_FLAG(__HANDLE__, __FLAG__) ((__HANDLE__)->Instance->ICR = (__FLAG__)) 00683 00684 /** @brief Clear the UART PE pending flag. 00685 * @param __HANDLE__: specifies the UART Handle. 00686 * @retval None 00687 */ 00688 #define __HAL_UART_CLEAR_PEFLAG(__HANDLE__) __HAL_UART_CLEAR_FLAG((__HANDLE__), UART_CLEAR_PEF) 00689 00690 /** @brief Clear the UART FE pending flag. 00691 * @param __HANDLE__: specifies the UART Handle. 00692 * @retval None 00693 */ 00694 #define __HAL_UART_CLEAR_FEFLAG(__HANDLE__) __HAL_UART_CLEAR_FLAG((__HANDLE__), UART_CLEAR_FEF) 00695 00696 /** @brief Clear the UART NE pending flag. 00697 * @param __HANDLE__: specifies the UART Handle. 00698 * @retval None 00699 */ 00700 #define __HAL_UART_CLEAR_NEFLAG(__HANDLE__) __HAL_UART_CLEAR_FLAG((__HANDLE__), UART_CLEAR_NEF) 00701 00702 /** @brief Clear the UART ORE pending flag. 00703 * @param __HANDLE__: specifies the UART Handle. 00704 * @retval None 00705 */ 00706 #define __HAL_UART_CLEAR_OREFLAG(__HANDLE__) __HAL_UART_CLEAR_FLAG((__HANDLE__), UART_CLEAR_OREF) 00707 00708 /** @brief Clear the UART IDLE pending flag. 00709 * @param __HANDLE__: specifies the UART Handle. 00710 * @retval None 00711 */ 00712 #define __HAL_UART_CLEAR_IDLEFLAG(__HANDLE__) __HAL_UART_CLEAR_FLAG((__HANDLE__), UART_CLEAR_IDLEF) 00713 00714 /** @brief Check whether the specified UART flag is set or not. 00715 * @param __HANDLE__: specifies the UART Handle. 00716 * @param __FLAG__: specifies the flag to check. 00717 * This parameter can be one of the following values: 00718 * @arg UART_FLAG_REACK: Receive enable acknowledge flag 00719 * @arg UART_FLAG_TEACK: Transmit enable acknowledge flag 00720 * @arg UART_FLAG_WUF: Wake up from stop mode flag 00721 * @arg UART_FLAG_RWU: Receiver wake up flag (if the UART in mute mode) 00722 * @arg UART_FLAG_SBKF: Send Break flag 00723 * @arg UART_FLAG_CMF: Character match flag 00724 * @arg UART_FLAG_BUSY: Busy flag 00725 * @arg UART_FLAG_ABRF: Auto Baud rate detection flag 00726 * @arg UART_FLAG_ABRE: Auto Baud rate detection error flag 00727 * @arg UART_FLAG_EOBF: End of block flag 00728 * @arg UART_FLAG_RTOF: Receiver timeout flag 00729 * @arg UART_FLAG_CTS: CTS Change flag 00730 * @arg UART_FLAG_LBD: LIN Break detection flag 00731 * @arg UART_FLAG_TXE: Transmit data register empty flag 00732 * @arg UART_FLAG_TC: Transmission Complete flag 00733 * @arg UART_FLAG_RXNE: Receive data register not empty flag 00734 * @arg UART_FLAG_IDLE: Idle Line detection flag 00735 * @arg UART_FLAG_ORE: OverRun Error flag 00736 * @arg UART_FLAG_NE: Noise Error flag 00737 * @arg UART_FLAG_FE: Framing Error flag 00738 * @arg UART_FLAG_PE: Parity Error flag 00739 * @retval The new state of __FLAG__ (TRUE or FALSE). 00740 */ 00741 #define __HAL_UART_GET_FLAG(__HANDLE__, __FLAG__) (((__HANDLE__)->Instance->ISR & (__FLAG__)) == (__FLAG__)) 00742 00743 /** @brief Enable the specified UART interrupt. 00744 * @param __HANDLE__: specifies the UART Handle. 00745 * @param __INTERRUPT__: specifies the UART interrupt source to enable. 00746 * This parameter can be one of the following values: 00747 * @arg UART_IT_WUF: Wakeup from stop mode interrupt 00748 * @arg UART_IT_CM: Character match interrupt 00749 * @arg UART_IT_CTS: CTS change interrupt 00750 * @arg UART_IT_LBD: LIN Break detection interrupt 00751 * @arg UART_IT_TXE: Transmit Data Register empty interrupt 00752 * @arg UART_IT_TC: Transmission complete interrupt 00753 * @arg UART_IT_RXNE: Receive Data register not empty interrupt 00754 * @arg UART_IT_IDLE: Idle line detection interrupt 00755 * @arg UART_IT_PE: Parity Error interrupt 00756 * @arg UART_IT_ERR: Error interrupt (Frame error, noise error, overrun error) 00757 * @retval None 00758 */ 00759 #define __HAL_UART_ENABLE_IT(__HANDLE__, __INTERRUPT__) (((((uint8_t)(__INTERRUPT__)) >> 5U) == 1)? ((__HANDLE__)->Instance->CR1 |= (1U << ((__INTERRUPT__) & UART_IT_MASK))): \ 00760 ((((uint8_t)(__INTERRUPT__)) >> 5U) == 2)? ((__HANDLE__)->Instance->CR2 |= (1U << ((__INTERRUPT__) & UART_IT_MASK))): \ 00761 ((__HANDLE__)->Instance->CR3 |= (1U << ((__INTERRUPT__) & UART_IT_MASK)))) 00762 00763 00764 /** @brief Disable the specified UART interrupt. 00765 * @param __HANDLE__: specifies the UART Handle. 00766 * @param __INTERRUPT__: specifies the UART interrupt source to disable. 00767 * This parameter can be one of the following values: 00768 * @arg UART_IT_WUF: Wakeup from stop mode interrupt 00769 * @arg UART_IT_CM: Character match interrupt 00770 * @arg UART_IT_CTS: CTS change interrupt 00771 * @arg UART_IT_LBD: LIN Break detection interrupt 00772 * @arg UART_IT_TXE: Transmit Data Register empty interrupt 00773 * @arg UART_IT_TC: Transmission complete interrupt 00774 * @arg UART_IT_RXNE: Receive Data register not empty interrupt 00775 * @arg UART_IT_IDLE: Idle line detection interrupt 00776 * @arg UART_IT_PE: Parity Error interrupt 00777 * @arg UART_IT_ERR: Error interrupt (Frame error, noise error, overrun error) 00778 * @retval None 00779 */ 00780 #define __HAL_UART_DISABLE_IT(__HANDLE__, __INTERRUPT__) (((((uint8_t)(__INTERRUPT__)) >> 5U) == 1)? ((__HANDLE__)->Instance->CR1 &= ~ (1U << ((__INTERRUPT__) & UART_IT_MASK))): \ 00781 ((((uint8_t)(__INTERRUPT__)) >> 5U) == 2)? ((__HANDLE__)->Instance->CR2 &= ~ (1U << ((__INTERRUPT__) & UART_IT_MASK))): \ 00782 ((__HANDLE__)->Instance->CR3 &= ~ (1U << ((__INTERRUPT__) & UART_IT_MASK)))) 00783 00784 /** @brief Check whether the specified UART interrupt has occurred or not. 00785 * @param __HANDLE__: specifies the UART Handle. 00786 * @param __IT__: specifies the UART interrupt to check. 00787 * This parameter can be one of the following values: 00788 * @arg UART_IT_WUF: Wakeup from stop mode interrupt 00789 * @arg UART_IT_CM: Character match interrupt 00790 * @arg UART_IT_CTS: CTS change interrupt 00791 * @arg UART_IT_LBD: LIN Break detection interrupt 00792 * @arg UART_IT_TXE: Transmit Data Register empty interrupt 00793 * @arg UART_IT_TC: Transmission complete interrupt 00794 * @arg UART_IT_RXNE: Receive Data register not empty interrupt 00795 * @arg UART_IT_IDLE: Idle line detection interrupt 00796 * @arg UART_IT_ORE: OverRun Error interrupt 00797 * @arg UART_IT_NE: Noise Error interrupt 00798 * @arg UART_IT_FE: Framing Error interrupt 00799 * @arg UART_IT_PE: Parity Error interrupt 00800 * @retval The new state of __IT__ (TRUE or FALSE). 00801 */ 00802 #define __HAL_UART_GET_IT(__HANDLE__, __IT__) ((__HANDLE__)->Instance->ISR & ((uint32_t)1 << ((__IT__)>> 0x08))) 00803 00804 /** @brief Check whether the specified UART interrupt source is enabled or not. 00805 * @param __HANDLE__: specifies the UART Handle. 00806 * @param __IT__: specifies the UART interrupt source to check. 00807 * This parameter can be one of the following values: 00808 * @arg UART_IT_WUF: Wakeup from stop mode interrupt 00809 * @arg UART_IT_CM: Character match interrupt 00810 * @arg UART_IT_CTS: CTS change interrupt 00811 * @arg UART_IT_LBD: LIN Break detection interrupt 00812 * @arg UART_IT_TXE: Transmit Data Register empty interrupt 00813 * @arg UART_IT_TC: Transmission complete interrupt 00814 * @arg UART_IT_RXNE: Receive Data register not empty interrupt 00815 * @arg UART_IT_IDLE: Idle line detection interrupt 00816 * @arg UART_IT_ORE: OverRun Error interrupt 00817 * @arg UART_IT_NE: Noise Error interrupt 00818 * @arg UART_IT_FE: Framing Error interrupt 00819 * @arg UART_IT_PE: Parity Error interrupt 00820 * @retval The new state of __IT__ (TRUE or FALSE). 00821 */ 00822 #define __HAL_UART_GET_IT_SOURCE(__HANDLE__, __IT__) ((((((uint8_t)(__IT__)) >> 5U) == 1)? (__HANDLE__)->Instance->CR1:(((((uint8_t)(__IT__)) >> 5U) == 2)? \ 00823 (__HANDLE__)->Instance->CR2 : (__HANDLE__)->Instance->CR3)) & ((uint32_t)1 << (((uint16_t)(__IT__)) & UART_IT_MASK))) 00824 00825 /** @brief Clear the specified UART ISR flag, in setting the proper ICR register flag. 00826 * @param __HANDLE__: specifies the UART Handle. 00827 * @param __IT_CLEAR__: specifies the interrupt clear register flag that needs to be set 00828 * to clear the corresponding interrupt 00829 * This parameter can be one of the following values: 00830 * @arg UART_CLEAR_PEF: Parity Error Clear Flag 00831 * @arg UART_CLEAR_FEF: Framing Error Clear Flag 00832 * @arg UART_CLEAR_NEF: Noise detected Clear Flag 00833 * @arg UART_CLEAR_OREF: OverRun Error Clear Flag 00834 * @arg UART_CLEAR_IDLEF: IDLE line detected Clear Flag 00835 * @arg UART_CLEAR_TCF: Transmission Complete Clear Flag 00836 * @arg UART_CLEAR_LBDF: LIN Break Detection Clear Flag 00837 * @arg UART_CLEAR_CTSF: CTS Interrupt Clear Flag 00838 * @arg UART_CLEAR_RTOF: Receiver Time Out Clear Flag 00839 * @arg UART_CLEAR_EOBF: End Of Block Clear Flag 00840 * @arg UART_CLEAR_CMF: Character Match Clear Flag 00841 * @arg UART_CLEAR_WUF: Wake Up from stop mode Clear Flag 00842 * @retval None 00843 */ 00844 #define __HAL_UART_CLEAR_IT(__HANDLE__, __IT_CLEAR__) ((__HANDLE__)->Instance->ICR = (uint32_t)(__IT_CLEAR__)) 00845 00846 /** @brief Set a specific UART request flag. 00847 * @param __HANDLE__: specifies the UART Handle. 00848 * @param __REQ__: specifies the request flag to set 00849 * This parameter can be one of the following values: 00850 * @arg UART_AUTOBAUD_REQUEST: Auto-Baud Rate Request 00851 * @arg UART_SENDBREAK_REQUEST: Send Break Request 00852 * @arg UART_MUTE_MODE_REQUEST: Mute Mode Request 00853 * @arg UART_RXDATA_FLUSH_REQUEST: Receive Data flush Request 00854 * @arg UART_TXDATA_FLUSH_REQUEST: Transmit data flush Request 00855 * @retval None 00856 */ 00857 #define __HAL_UART_SEND_REQ(__HANDLE__, __REQ__) ((__HANDLE__)->Instance->RQR |= (uint32_t)(__REQ__)) 00858 00859 /** @brief Enable the UART one bit sample method. 00860 * @param __HANDLE__: specifies the UART Handle. 00861 * @retval None 00862 */ 00863 #define __HAL_UART_ONE_BIT_SAMPLE_ENABLE(__HANDLE__) ((__HANDLE__)->Instance->CR3|= USART_CR3_ONEBIT) 00864 00865 /** @brief Disable the UART one bit sample method. 00866 * @param __HANDLE__: specifies the UART Handle. 00867 * @retval None 00868 */ 00869 #define __HAL_UART_ONE_BIT_SAMPLE_DISABLE(__HANDLE__) ((__HANDLE__)->Instance->CR3 &= (uint32_t)~((uint32_t)USART_CR3_ONEBIT)) 00870 00871 /** @brief Enable UART. 00872 * @param __HANDLE__: specifies the UART Handle. 00873 * @retval None 00874 */ 00875 #define __HAL_UART_ENABLE(__HANDLE__) ((__HANDLE__)->Instance->CR1 |= USART_CR1_UE) 00876 00877 /** @brief Disable UART. 00878 * @param __HANDLE__: specifies the UART Handle. 00879 * @retval None 00880 */ 00881 #define __HAL_UART_DISABLE(__HANDLE__) ((__HANDLE__)->Instance->CR1 &= ~USART_CR1_UE) 00882 00883 /** @brief Enable CTS flow control. 00884 * @note This macro allows to enable CTS hardware flow control for a given UART instance, 00885 * without need to call HAL_UART_Init() function. 00886 * As involving direct access to UART registers, usage of this macro should be fully endorsed by user. 00887 * @note As macro is expected to be used for modifying CTS Hw flow control feature activation, without need 00888 * for USART instance Deinit/Init, following conditions for macro call should be fulfilled : 00889 * - UART instance should have already been initialised (through call of HAL_UART_Init() ) 00890 * - macro could only be called when corresponding UART instance is disabled (i.e. __HAL_UART_DISABLE(__HANDLE__)) 00891 * and should be followed by an Enable macro (i.e. __HAL_UART_ENABLE(__HANDLE__)). 00892 * @param __HANDLE__: specifies the UART Handle. 00893 * @retval None 00894 */ 00895 #define __HAL_UART_HWCONTROL_CTS_ENABLE(__HANDLE__) \ 00896 do{ \ 00897 SET_BIT((__HANDLE__)->Instance->CR3, USART_CR3_CTSE); \ 00898 (__HANDLE__)->Init.HwFlowCtl |= USART_CR3_CTSE; \ 00899 } while(0) 00900 00901 /** @brief Disable CTS flow control. 00902 * @note This macro allows to disable CTS hardware flow control for a given UART instance, 00903 * without need to call HAL_UART_Init() function. 00904 * As involving direct access to UART registers, usage of this macro should be fully endorsed by user. 00905 * @note As macro is expected to be used for modifying CTS Hw flow control feature activation, without need 00906 * for USART instance Deinit/Init, following conditions for macro call should be fulfilled : 00907 * - UART instance should have already been initialised (through call of HAL_UART_Init() ) 00908 * - macro could only be called when corresponding UART instance is disabled (i.e. __HAL_UART_DISABLE(__HANDLE__)) 00909 * and should be followed by an Enable macro (i.e. __HAL_UART_ENABLE(__HANDLE__)). 00910 * @param __HANDLE__: specifies the UART Handle. 00911 * @retval None 00912 */ 00913 #define __HAL_UART_HWCONTROL_CTS_DISABLE(__HANDLE__) \ 00914 do{ \ 00915 CLEAR_BIT((__HANDLE__)->Instance->CR3, USART_CR3_CTSE); \ 00916 (__HANDLE__)->Init.HwFlowCtl &= ~(USART_CR3_CTSE); \ 00917 } while(0) 00918 00919 /** @brief Enable RTS flow control. 00920 * @note This macro allows to enable RTS hardware flow control for a given UART instance, 00921 * without need to call HAL_UART_Init() function. 00922 * As involving direct access to UART registers, usage of this macro should be fully endorsed by user. 00923 * @note As macro is expected to be used for modifying RTS Hw flow control feature activation, without need 00924 * for USART instance Deinit/Init, following conditions for macro call should be fulfilled : 00925 * - UART instance should have already been initialised (through call of HAL_UART_Init() ) 00926 * - macro could only be called when corresponding UART instance is disabled (i.e. __HAL_UART_DISABLE(__HANDLE__)) 00927 * and should be followed by an Enable macro (i.e. __HAL_UART_ENABLE(__HANDLE__)). 00928 * @param __HANDLE__: specifies the UART Handle. 00929 * @retval None 00930 */ 00931 #define __HAL_UART_HWCONTROL_RTS_ENABLE(__HANDLE__) \ 00932 do{ \ 00933 SET_BIT((__HANDLE__)->Instance->CR3, USART_CR3_RTSE); \ 00934 (__HANDLE__)->Init.HwFlowCtl |= USART_CR3_RTSE; \ 00935 } while(0) 00936 00937 /** @brief Disable RTS flow control. 00938 * @note This macro allows to disable RTS hardware flow control for a given UART instance, 00939 * without need to call HAL_UART_Init() function. 00940 * As involving direct access to UART registers, usage of this macro should be fully endorsed by user. 00941 * @note As macro is expected to be used for modifying RTS Hw flow control feature activation, without need 00942 * for USART instance Deinit/Init, following conditions for macro call should be fulfilled : 00943 * - UART instance should have already been initialised (through call of HAL_UART_Init() ) 00944 * - macro could only be called when corresponding UART instance is disabled (i.e. __HAL_UART_DISABLE(__HANDLE__)) 00945 * and should be followed by an Enable macro (i.e. __HAL_UART_ENABLE(__HANDLE__)). 00946 * @param __HANDLE__: specifies the UART Handle. 00947 * @retval None 00948 */ 00949 #define __HAL_UART_HWCONTROL_RTS_DISABLE(__HANDLE__) \ 00950 do{ \ 00951 CLEAR_BIT((__HANDLE__)->Instance->CR3, USART_CR3_RTSE);\ 00952 (__HANDLE__)->Init.HwFlowCtl &= ~(USART_CR3_RTSE); \ 00953 } while(0) 00954 00955 /** 00956 * @} 00957 */ 00958 00959 /* Private macros --------------------------------------------------------*/ 00960 /** @defgroup UART_Private_Macros UART Private Macros 00961 * @{ 00962 */ 00963 /** @brief BRR division operation to set BRR register with LPUART. 00964 * @param __PCLK__: LPUART clock. 00965 * @param __BAUD__: Baud rate set by the user. 00966 * @retval Division result 00967 */ 00968 #define UART_DIV_LPUART(__PCLK__, __BAUD__) (((uint64_t)(__PCLK__)*256)/((__BAUD__))) 00969 00970 /** @brief BRR division operation to set BRR register in 8-bit oversampling mode. 00971 * @param __PCLK__: UART clock. 00972 * @param __BAUD__: Baud rate set by the user. 00973 * @retval Division result 00974 */ 00975 #define UART_DIV_SAMPLING8(__PCLK__, __BAUD__) (((__PCLK__)*2)/((__BAUD__))) 00976 00977 /** @brief BRR division operation to set BRR register in 16-bit oversampling mode. 00978 * @param __PCLK__: UART clock. 00979 * @param __BAUD__: Baud rate set by the user. 00980 * @retval Division result 00981 */ 00982 #define UART_DIV_SAMPLING16(__PCLK__, __BAUD__) (((__PCLK__))/((__BAUD__))) 00983 00984 /** @brief Check whether or not UART instance is Low Power UART. 00985 * @param __HANDLE__: specifies the UART Handle. 00986 * @retval SET (instance is LPUART) or RESET (instance isn't LPUART) 00987 */ 00988 #define UART_INSTANCE_LOWPOWER(__HANDLE__) (((__HANDLE__)->Instance == LPUART1) ? SET : RESET ) 00989 00990 /** @brief Check UART Baud rate. 00991 * @param __BAUDRATE__: Baudrate specified by the user. 00992 * The maximum Baud Rate is derived from the maximum clock on L4 (i.e. 80 MHz) 00993 * divided by the smallest oversampling used on the USART (i.e. 8) 00994 * @retval SET (__BAUDRATE__ is valid) or RESET (__BAUDRATE__ is invalid) 00995 */ 00996 #define IS_UART_BAUDRATE(__BAUDRATE__) ((__BAUDRATE__) < 10000001) 00997 00998 /** @brief Check UART assertion time. 00999 * @param __TIME__: 5-bit value assertion time. 01000 * @retval Test result (TRUE or FALSE). 01001 */ 01002 #define IS_UART_ASSERTIONTIME(__TIME__) ((__TIME__) <= 0x1F) 01003 01004 /** @brief Check UART deassertion time. 01005 * @param __TIME__: 5-bit value deassertion time. 01006 * @retval Test result (TRUE or FALSE). 01007 */ 01008 #define IS_UART_DEASSERTIONTIME(__TIME__) ((__TIME__) <= 0x1F) 01009 01010 /** 01011 * @brief Ensure that UART frame number of stop bits is valid. 01012 * @param __STOPBITS__: UART frame number of stop bits. 01013 * @retval SET (__STOPBITS__ is valid) or RESET (__STOPBITS__ is invalid) 01014 */ 01015 #define IS_UART_STOPBITS(__STOPBITS__) (((__STOPBITS__) == UART_STOPBITS_1) || \ 01016 ((__STOPBITS__) == UART_STOPBITS_2) || \ 01017 ((__STOPBITS__) == UART_STOPBITS_1_5)) 01018 01019 /** 01020 * @brief Ensure that UART frame parity is valid. 01021 * @param __PARITY__: UART frame parity. 01022 * @retval SET (__PARITY__ is valid) or RESET (__PARITY__ is invalid) 01023 */ 01024 #define IS_UART_PARITY(__PARITY__) (((__PARITY__) == UART_PARITY_NONE) || \ 01025 ((__PARITY__) == UART_PARITY_EVEN) || \ 01026 ((__PARITY__) == UART_PARITY_ODD)) 01027 01028 /** 01029 * @brief Ensure that UART hardware flow control is valid. 01030 * @param __CONTROL__: UART hardware flow control. 01031 * @retval SET (__CONTROL__ is valid) or RESET (__CONTROL__ is invalid) 01032 */ 01033 #define IS_UART_HARDWARE_FLOW_CONTROL(__CONTROL__)\ 01034 (((__CONTROL__) == UART_HWCONTROL_NONE) || \ 01035 ((__CONTROL__) == UART_HWCONTROL_RTS) || \ 01036 ((__CONTROL__) == UART_HWCONTROL_CTS) || \ 01037 ((__CONTROL__) == UART_HWCONTROL_RTS_CTS)) 01038 01039 /** 01040 * @brief Ensure that UART communication mode is valid. 01041 * @param __MODE__: UART communication mode. 01042 * @retval SET (__MODE__ is valid) or RESET (__MODE__ is invalid) 01043 */ 01044 #define IS_UART_MODE(__MODE__) ((((__MODE__) & (~((uint32_t)(UART_MODE_TX_RX)))) == (uint32_t)0x00) && ((__MODE__) != (uint32_t)0x00)) 01045 01046 /** 01047 * @brief Ensure that UART state is valid. 01048 * @param __STATE__: UART state. 01049 * @retval SET (__STATE__ is valid) or RESET (__STATE__ is invalid) 01050 */ 01051 #define IS_UART_STATE(__STATE__) (((__STATE__) == UART_STATE_DISABLE) || \ 01052 ((__STATE__) == UART_STATE_ENABLE)) 01053 01054 /** 01055 * @brief Ensure that UART oversampling is valid. 01056 * @param __SAMPLING__: UART oversampling. 01057 * @retval SET (__SAMPLING__ is valid) or RESET (__SAMPLING__ is invalid) 01058 */ 01059 #define IS_UART_OVERSAMPLING(__SAMPLING__) (((__SAMPLING__) == UART_OVERSAMPLING_16) || \ 01060 ((__SAMPLING__) == UART_OVERSAMPLING_8)) 01061 01062 /** 01063 * @brief Ensure that UART frame sampling is valid. 01064 * @param __ONEBIT__: UART frame sampling. 01065 * @retval SET (__ONEBIT__ is valid) or RESET (__ONEBIT__ is invalid) 01066 */ 01067 #define IS_UART_ONE_BIT_SAMPLE(__ONEBIT__) (((__ONEBIT__) == UART_ONE_BIT_SAMPLE_DISABLE) || \ 01068 ((__ONEBIT__) == UART_ONE_BIT_SAMPLE_ENABLE)) 01069 01070 /** 01071 * @brief Ensure that UART auto Baud rate detection mode is valid. 01072 * @param __MODE__: UART auto Baud rate detection mode. 01073 * @retval SET (__MODE__ is valid) or RESET (__MODE__ is invalid) 01074 */ 01075 #define IS_UART_ADVFEATURE_AUTOBAUDRATEMODE(__MODE__) (((__MODE__) == UART_ADVFEATURE_AUTOBAUDRATE_ONSTARTBIT) || \ 01076 ((__MODE__) == UART_ADVFEATURE_AUTOBAUDRATE_ONFALLINGEDGE) || \ 01077 ((__MODE__) == UART_ADVFEATURE_AUTOBAUDRATE_ON0X7FFRAME) || \ 01078 ((__MODE__) == UART_ADVFEATURE_AUTOBAUDRATE_ON0X55FRAME)) 01079 01080 /** 01081 * @brief Ensure that UART receiver timeout setting is valid. 01082 * @param __TIMEOUT__: UART receiver timeout setting. 01083 * @retval SET (__TIMEOUT__ is valid) or RESET (__TIMEOUT__ is invalid) 01084 */ 01085 #define IS_UART_RECEIVER_TIMEOUT(__TIMEOUT__) (((__TIMEOUT__) == UART_RECEIVER_TIMEOUT_DISABLE) || \ 01086 ((__TIMEOUT__) == UART_RECEIVER_TIMEOUT_ENABLE)) 01087 01088 /** 01089 * @brief Ensure that UART LIN state is valid. 01090 * @param __LIN__: UART LIN state. 01091 * @retval SET (__LIN__ is valid) or RESET (__LIN__ is invalid) 01092 */ 01093 #define IS_UART_LIN(__LIN__) (((__LIN__) == UART_LIN_DISABLE) || \ 01094 ((__LIN__) == UART_LIN_ENABLE)) 01095 01096 /** 01097 * @brief Ensure that UART LIN break detection length is valid. 01098 * @param __LENGTH__: UART LIN break detection length. 01099 * @retval SET (__LENGTH__ is valid) or RESET (__LENGTH__ is invalid) 01100 */ 01101 #define IS_UART_LIN_BREAK_DETECT_LENGTH(__LENGTH__) (((__LENGTH__) == UART_LINBREAKDETECTLENGTH_10B) || \ 01102 ((__LENGTH__) == UART_LINBREAKDETECTLENGTH_11B)) 01103 01104 /** 01105 * @brief Ensure that UART DMA TX state is valid. 01106 * @param __DMATX__: UART DMA TX state. 01107 * @retval SET (__DMATX__ is valid) or RESET (__DMATX__ is invalid) 01108 */ 01109 #define IS_UART_DMA_TX(__DMATX__) (((__DMATX__) == UART_DMA_TX_DISABLE) || \ 01110 ((__DMATX__) == UART_DMA_TX_ENABLE)) 01111 01112 /** 01113 * @brief Ensure that UART DMA RX state is valid. 01114 * @param __DMARX__: UART DMA RX state. 01115 * @retval SET (__DMARX__ is valid) or RESET (__DMARX__ is invalid) 01116 */ 01117 #define IS_UART_DMA_RX(__DMARX__) (((__DMARX__) == UART_DMA_RX_DISABLE) || \ 01118 ((__DMARX__) == UART_DMA_RX_ENABLE)) 01119 01120 /** 01121 * @brief Ensure that UART half-duplex state is valid. 01122 * @param __HDSEL__: UART half-duplex state. 01123 * @retval SET (__HDSEL__ is valid) or RESET (__HDSEL__ is invalid) 01124 */ 01125 #define IS_UART_HALF_DUPLEX(__HDSEL__) (((__HDSEL__) == UART_HALF_DUPLEX_DISABLE) || \ 01126 ((__HDSEL__) == UART_HALF_DUPLEX_ENABLE)) 01127 01128 /** 01129 * @brief Ensure that UART wake-up method is valid. 01130 * @param __WAKEUP__: UART wake-up method . 01131 * @retval SET (__WAKEUP__ is valid) or RESET (__WAKEUP__ is invalid) 01132 */ 01133 #define IS_UART_WAKEUPMETHOD(__WAKEUP__) (((__WAKEUP__) == UART_WAKEUPMETHOD_IDLELINE) || \ 01134 ((__WAKEUP__) == UART_WAKEUPMETHOD_ADDRESSMARK)) 01135 01136 /** 01137 * @brief Ensure that UART request parameter is valid. 01138 * @param __PARAM__: UART request parameter. 01139 * @retval SET (__PARAM__ is valid) or RESET (__PARAM__ is invalid) 01140 */ 01141 #define IS_UART_REQUEST_PARAMETER(__PARAM__) (((__PARAM__) == UART_AUTOBAUD_REQUEST) || \ 01142 ((__PARAM__) == UART_SENDBREAK_REQUEST) || \ 01143 ((__PARAM__) == UART_MUTE_MODE_REQUEST) || \ 01144 ((__PARAM__) == UART_RXDATA_FLUSH_REQUEST) || \ 01145 ((__PARAM__) == UART_TXDATA_FLUSH_REQUEST)) 01146 01147 /** 01148 * @brief Ensure that UART advanced features initialization is valid. 01149 * @param __INIT__: UART advanced features initialization. 01150 * @retval SET (__INIT__ is valid) or RESET (__INIT__ is invalid) 01151 */ 01152 #define IS_UART_ADVFEATURE_INIT(__INIT__) ((__INIT__) <= (UART_ADVFEATURE_NO_INIT | \ 01153 UART_ADVFEATURE_TXINVERT_INIT | \ 01154 UART_ADVFEATURE_RXINVERT_INIT | \ 01155 UART_ADVFEATURE_DATAINVERT_INIT | \ 01156 UART_ADVFEATURE_SWAP_INIT | \ 01157 UART_ADVFEATURE_RXOVERRUNDISABLE_INIT | \ 01158 UART_ADVFEATURE_DMADISABLEONERROR_INIT | \ 01159 UART_ADVFEATURE_AUTOBAUDRATE_INIT | \ 01160 UART_ADVFEATURE_MSBFIRST_INIT)) 01161 01162 /** 01163 * @brief Ensure that UART frame TX inversion setting is valid. 01164 * @param __TXINV__: UART frame TX inversion setting. 01165 * @retval SET (__TXINV__ is valid) or RESET (__TXINV__ is invalid) 01166 */ 01167 #define IS_UART_ADVFEATURE_TXINV(__TXINV__) (((__TXINV__) == UART_ADVFEATURE_TXINV_DISABLE) || \ 01168 ((__TXINV__) == UART_ADVFEATURE_TXINV_ENABLE)) 01169 01170 /** 01171 * @brief Ensure that UART frame RX inversion setting is valid. 01172 * @param __RXINV__: UART frame RX inversion setting. 01173 * @retval SET (__RXINV__ is valid) or RESET (__RXINV__ is invalid) 01174 */ 01175 #define IS_UART_ADVFEATURE_RXINV(__RXINV__) (((__RXINV__) == UART_ADVFEATURE_RXINV_DISABLE) || \ 01176 ((__RXINV__) == UART_ADVFEATURE_RXINV_ENABLE)) 01177 01178 /** 01179 * @brief Ensure that UART frame data inversion setting is valid. 01180 * @param __DATAINV__: UART frame data inversion setting. 01181 * @retval SET (__DATAINV__ is valid) or RESET (__DATAINV__ is invalid) 01182 */ 01183 #define IS_UART_ADVFEATURE_DATAINV(__DATAINV__) (((__DATAINV__) == UART_ADVFEATURE_DATAINV_DISABLE) || \ 01184 ((__DATAINV__) == UART_ADVFEATURE_DATAINV_ENABLE)) 01185 01186 /** 01187 * @brief Ensure that UART frame RX/TX pins swap setting is valid. 01188 * @param __SWAP__: UART frame RX/TX pins swap setting. 01189 * @retval SET (__SWAP__ is valid) or RESET (__SWAP__ is invalid) 01190 */ 01191 #define IS_UART_ADVFEATURE_SWAP(__SWAP__) (((__SWAP__) == UART_ADVFEATURE_SWAP_DISABLE) || \ 01192 ((__SWAP__) == UART_ADVFEATURE_SWAP_ENABLE)) 01193 01194 /** 01195 * @brief Ensure that UART frame overrun setting is valid. 01196 * @param __OVERRUN__: UART frame overrun setting. 01197 * @retval SET (__OVERRUN__ is valid) or RESET (__OVERRUN__ is invalid) 01198 */ 01199 #define IS_UART_OVERRUN(__OVERRUN__) (((__OVERRUN__) == UART_ADVFEATURE_OVERRUN_ENABLE) || \ 01200 ((__OVERRUN__) == UART_ADVFEATURE_OVERRUN_DISABLE)) 01201 01202 /** 01203 * @brief Ensure that UART auto Baud rate state is valid. 01204 * @param __AUTOBAUDRATE__: UART auto Baud rate state. 01205 * @retval SET (__AUTOBAUDRATE__ is valid) or RESET (__AUTOBAUDRATE__ is invalid) 01206 */ 01207 #define IS_UART_ADVFEATURE_AUTOBAUDRATE(__AUTOBAUDRATE__) (((__AUTOBAUDRATE__) == UART_ADVFEATURE_AUTOBAUDRATE_DISABLE) || \ 01208 ((__AUTOBAUDRATE__) == UART_ADVFEATURE_AUTOBAUDRATE_ENABLE)) 01209 01210 /** 01211 * @brief Ensure that UART DMA enabling or disabling on error setting is valid. 01212 * @param __DMA__: UART DMA enabling or disabling on error setting. 01213 * @retval SET (__DMA__ is valid) or RESET (__DMA__ is invalid) 01214 */ 01215 #define IS_UART_ADVFEATURE_DMAONRXERROR(__DMA__) (((__DMA__) == UART_ADVFEATURE_DMA_ENABLEONRXERROR) || \ 01216 ((__DMA__) == UART_ADVFEATURE_DMA_DISABLEONRXERROR)) 01217 01218 /** 01219 * @brief Ensure that UART frame MSB first setting is valid. 01220 * @param __MSBFIRST__: UART frame MSB first setting. 01221 * @retval SET (__MSBFIRST__ is valid) or RESET (__MSBFIRST__ is invalid) 01222 */ 01223 #define IS_UART_ADVFEATURE_MSBFIRST(__MSBFIRST__) (((__MSBFIRST__) == UART_ADVFEATURE_MSBFIRST_DISABLE) || \ 01224 ((__MSBFIRST__) == UART_ADVFEATURE_MSBFIRST_ENABLE)) 01225 01226 /** 01227 * @brief Ensure that UART stop mode state is valid. 01228 * @param __STOPMODE__: UART stop mode state. 01229 * @retval SET (__STOPMODE__ is valid) or RESET (__STOPMODE__ is invalid) 01230 */ 01231 #define IS_UART_ADVFEATURE_STOPMODE(__STOPMODE__) (((__STOPMODE__) == UART_ADVFEATURE_STOPMODE_DISABLE) || \ 01232 ((__STOPMODE__) == UART_ADVFEATURE_STOPMODE_ENABLE)) 01233 01234 /** 01235 * @brief Ensure that UART mute mode state is valid. 01236 * @param __MUTE__: UART mute mode state. 01237 * @retval SET (__MUTE__ is valid) or RESET (__MUTE__ is invalid) 01238 */ 01239 #define IS_UART_MUTE_MODE(__MUTE__) (((__MUTE__) == UART_ADVFEATURE_MUTEMODE_DISABLE) || \ 01240 ((__MUTE__) == UART_ADVFEATURE_MUTEMODE_ENABLE)) 01241 01242 /** 01243 * @brief Ensure that UART wake-up selection is valid. 01244 * @param __WAKE__: UART wake-up selection. 01245 * @retval SET (__WAKE__ is valid) or RESET (__WAKE__ is invalid) 01246 */ 01247 #define IS_UART_WAKEUP_SELECTION(__WAKE__) (((__WAKE__) == UART_WAKEUP_ON_ADDRESS) || \ 01248 ((__WAKE__) == UART_WAKEUP_ON_STARTBIT) || \ 01249 ((__WAKE__) == UART_WAKEUP_ON_READDATA_NONEMPTY)) 01250 01251 /** 01252 * @brief Ensure that UART driver enable polarity is valid. 01253 * @param __POLARITY__: UART driver enable polarity. 01254 * @retval SET (__POLARITY__ is valid) or RESET (__POLARITY__ is invalid) 01255 */ 01256 #define IS_UART_DE_POLARITY(__POLARITY__) (((__POLARITY__) == UART_DE_POLARITY_HIGH) || \ 01257 ((__POLARITY__) == UART_DE_POLARITY_LOW)) 01258 01259 /** 01260 * @brief Ensure that LPUART frame number of stop bits is valid. 01261 * @param __STOPBITS__: LPUART frame number of stop bits. 01262 * @retval SET (__STOPBITS__ is valid) or RESET (__STOPBITS__ is invalid) 01263 */ 01264 #define IS_LPUART_STOPBITS(__STOPBITS__) (((__STOPBITS__) == UART_STOPBITS_1) || \ 01265 ((__STOPBITS__) == UART_STOPBITS_2)) 01266 01267 /** 01268 * @} 01269 */ 01270 01271 /* Include UART HAL Extended module */ 01272 #include "stm32l4xx_hal_uart_ex.h" 01273 01274 /* Exported functions --------------------------------------------------------*/ 01275 /** @addtogroup UART_Exported_Functions UART Exported Functions 01276 * @{ 01277 */ 01278 01279 /** @addtogroup UART_Exported_Functions_Group1 Initialization and de-initialization functions 01280 * @{ 01281 */ 01282 01283 /* Initialization and de-initialization functions ****************************/ 01284 HAL_StatusTypeDef HAL_UART_Init(UART_HandleTypeDef *huart); 01285 HAL_StatusTypeDef HAL_HalfDuplex_Init(UART_HandleTypeDef *huart); 01286 HAL_StatusTypeDef HAL_LIN_Init(UART_HandleTypeDef *huart, uint32_t BreakDetectLength); 01287 HAL_StatusTypeDef HAL_MultiProcessor_Init(UART_HandleTypeDef *huart, uint8_t Address, uint32_t WakeUpMethod); 01288 HAL_StatusTypeDef HAL_UART_DeInit (UART_HandleTypeDef *huart); 01289 void HAL_UART_MspInit(UART_HandleTypeDef *huart); 01290 void HAL_UART_MspDeInit(UART_HandleTypeDef *huart); 01291 01292 /** 01293 * @} 01294 */ 01295 01296 /** @addtogroup UART_Exported_Functions_Group2 IO operation functions 01297 * @{ 01298 */ 01299 01300 /* IO operation functions *****************************************************/ 01301 HAL_StatusTypeDef HAL_UART_Transmit(UART_HandleTypeDef *huart, uint8_t *pData, uint16_t Size, uint32_t Timeout); 01302 HAL_StatusTypeDef HAL_UART_Receive(UART_HandleTypeDef *huart, uint8_t *pData, uint16_t Size, uint32_t Timeout); 01303 HAL_StatusTypeDef HAL_UART_Transmit_IT(UART_HandleTypeDef *huart, uint8_t *pData, uint16_t Size); 01304 HAL_StatusTypeDef HAL_UART_Receive_IT(UART_HandleTypeDef *huart, uint8_t *pData, uint16_t Size); 01305 HAL_StatusTypeDef HAL_UART_Transmit_DMA(UART_HandleTypeDef *huart, uint8_t *pData, uint16_t Size); 01306 HAL_StatusTypeDef HAL_UART_Receive_DMA(UART_HandleTypeDef *huart, uint8_t *pData, uint16_t Size); 01307 HAL_StatusTypeDef HAL_UART_DMAPause(UART_HandleTypeDef *huart); 01308 HAL_StatusTypeDef HAL_UART_DMAResume(UART_HandleTypeDef *huart); 01309 HAL_StatusTypeDef HAL_UART_DMAStop(UART_HandleTypeDef *huart); 01310 void HAL_UART_IRQHandler(UART_HandleTypeDef *huart); 01311 void HAL_UART_TxHalfCpltCallback(UART_HandleTypeDef *huart); 01312 void HAL_UART_TxCpltCallback(UART_HandleTypeDef *huart); 01313 void HAL_UART_RxHalfCpltCallback(UART_HandleTypeDef *huart); 01314 void HAL_UART_RxCpltCallback(UART_HandleTypeDef *huart); 01315 void HAL_UART_ErrorCallback(UART_HandleTypeDef *huart); 01316 01317 /** 01318 * @} 01319 */ 01320 01321 /** @addtogroup UART_Exported_Functions_Group3 Peripheral Control functions 01322 * @{ 01323 */ 01324 01325 /* Peripheral Control functions ************************************************/ 01326 HAL_StatusTypeDef HAL_LIN_SendBreak(UART_HandleTypeDef *huart); 01327 HAL_StatusTypeDef HAL_MultiProcessor_EnableMuteMode(UART_HandleTypeDef *huart); 01328 HAL_StatusTypeDef HAL_MultiProcessor_DisableMuteMode(UART_HandleTypeDef *huart); 01329 void HAL_MultiProcessor_EnterMuteMode(UART_HandleTypeDef *huart); 01330 HAL_StatusTypeDef HAL_HalfDuplex_EnableTransmitter(UART_HandleTypeDef *huart); 01331 HAL_StatusTypeDef HAL_HalfDuplex_EnableReceiver(UART_HandleTypeDef *huart); 01332 01333 /** 01334 * @} 01335 */ 01336 01337 /** @addtogroup UART_Exported_Functions_Group4 Peripheral State and Error functions 01338 * @{ 01339 */ 01340 01341 /* Peripheral State and Errors functions **************************************************/ 01342 HAL_UART_StateTypeDef HAL_UART_GetState(UART_HandleTypeDef *huart); 01343 uint32_t HAL_UART_GetError(UART_HandleTypeDef *huart); 01344 01345 /** 01346 * @} 01347 */ 01348 01349 /** 01350 * @} 01351 */ 01352 01353 /* Private functions -----------------------------------------------------------*/ 01354 /** @addtogroup UART_Private_Functions UART Private Functions 01355 * @{ 01356 */ 01357 01358 HAL_StatusTypeDef UART_SetConfig(UART_HandleTypeDef *huart); 01359 HAL_StatusTypeDef UART_CheckIdleState(UART_HandleTypeDef *huart); 01360 HAL_StatusTypeDef UART_WaitOnFlagUntilTimeout(UART_HandleTypeDef *huart, uint32_t Flag, FlagStatus Status, uint32_t Timeout); 01361 void UART_AdvFeatureConfig(UART_HandleTypeDef *huart); 01362 01363 /** 01364 * @} 01365 */ 01366 01367 /** 01368 * @} 01369 */ 01370 01371 /** 01372 * @} 01373 */ 01374 01375 #ifdef __cplusplus 01376 } 01377 #endif 01378 01379 #endif /* __STM32L4xx_HAL_UART_H */ 01380 01381 /************************ (C) COPYRIGHT STMicroelectronics *****END OF FILE****/ 01382
Generated on Tue Jul 12 2022 11:35:15 by
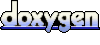