Hal Drivers for L4
Dependents: BSP OneHopeOnePrayer FINAL_AUDIO_RECORD AudioDemo
Fork of STM32L4xx_HAL_Driver by
stm32l4xx_hal_sram.c
00001 /** 00002 ****************************************************************************** 00003 * @file stm32l4xx_hal_sram.c 00004 * @author MCD Application Team 00005 * @version V1.1.0 00006 * @date 16-September-2015 00007 * @brief SRAM HAL module driver. 00008 * This file provides a generic firmware to drive SRAM memories 00009 * mounted as external device. 00010 * 00011 @verbatim 00012 ============================================================================== 00013 ##### How to use this driver ##### 00014 ============================================================================== 00015 [..] 00016 This driver is a generic layered driver which contains a set of APIs used to 00017 control SRAM memories. It uses the FMC layer functions to interface 00018 with SRAM devices. 00019 The following sequence should be followed to configure the FMC to interface 00020 with SRAM/PSRAM memories: 00021 00022 (#) Declare a SRAM_HandleTypeDef handle structure, for example: 00023 SRAM_HandleTypeDef hsram; and: 00024 00025 (++) Fill the SRAM_HandleTypeDef handle "Init" field with the allowed 00026 values of the structure member. 00027 00028 (++) Fill the SRAM_HandleTypeDef handle "Instance" field with a predefined 00029 base register instance for NOR or SRAM device 00030 00031 (++) Fill the SRAM_HandleTypeDef handle "Extended" field with a predefined 00032 base register instance for NOR or SRAM extended mode 00033 00034 (#) Declare two FMC_NORSRAM_TimingTypeDef structures, for both normal and extended 00035 mode timings; for example: 00036 FMC_NORSRAM_TimingTypeDef Timing and FMC_NORSRAM_TimingTypeDef ExTiming; 00037 and fill its fields with the allowed values of the structure member. 00038 00039 (#) Initialize the SRAM Controller by calling the function HAL_SRAM_Init(). This function 00040 performs the following sequence: 00041 00042 (##) MSP hardware layer configuration using the function HAL_SRAM_MspInit() 00043 (##) Control register configuration using the FMC NORSRAM interface function 00044 FMC_NORSRAM_Init() 00045 (##) Timing register configuration using the FMC NORSRAM interface function 00046 FMC_NORSRAM_Timing_Init() 00047 (##) Extended mode Timing register configuration using the FMC NORSRAM interface function 00048 FMC_NORSRAM_Extended_Timing_Init() 00049 (##) Enable the SRAM device using the macro __FMC_NORSRAM_ENABLE() 00050 00051 (#) At this stage you can perform read/write accesses from/to the memory connected 00052 to the NOR/SRAM Bank. You can perform either polling or DMA transfer using the 00053 following APIs: 00054 (++) HAL_SRAM_Read()/HAL_SRAM_Write() for polling read/write access 00055 (++) HAL_SRAM_Read_DMA()/HAL_SRAM_Write_DMA() for DMA read/write transfer 00056 00057 (#) You can also control the SRAM device by calling the control APIs HAL_SRAM_WriteOperation_Enable()/ 00058 HAL_SRAM_WriteOperation_Disable() to respectively enable/disable the SRAM write operation 00059 00060 (#) You can continuously monitor the SRAM device HAL state by calling the function 00061 HAL_SRAM_GetState() 00062 00063 @endverbatim 00064 ****************************************************************************** 00065 * @attention 00066 * 00067 * <h2><center>© COPYRIGHT(c) 2015 STMicroelectronics</center></h2> 00068 * 00069 * Redistribution and use in source and binary forms, with or without modification, 00070 * are permitted provided that the following conditions are met: 00071 * 1. Redistributions of source code must retain the above copyright notice, 00072 * this list of conditions and the following disclaimer. 00073 * 2. Redistributions in binary form must reproduce the above copyright notice, 00074 * this list of conditions and the following disclaimer in the documentation 00075 * and/or other materials provided with the distribution. 00076 * 3. Neither the name of STMicroelectronics nor the names of its contributors 00077 * may be used to endorse or promote products derived from this software 00078 * without specific prior written permission. 00079 * 00080 * THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" 00081 * AND ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE 00082 * IMPLIED WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE 00083 * DISCLAIMED. IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE 00084 * FOR ANY DIRECT, INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL 00085 * DAMAGES (INCLUDING, BUT NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR 00086 * SERVICES; LOSS OF USE, DATA, OR PROFITS; OR BUSINESS INTERRUPTION) HOWEVER 00087 * CAUSED AND ON ANY THEORY OF LIABILITY, WHETHER IN CONTRACT, STRICT LIABILITY, 00088 * OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) ARISING IN ANY WAY OUT OF THE USE 00089 * OF THIS SOFTWARE, EVEN IF ADVISED OF THE POSSIBILITY OF SUCH DAMAGE. 00090 * 00091 ****************************************************************************** 00092 */ 00093 00094 /* Includes ------------------------------------------------------------------*/ 00095 #include "stm32l4xx_hal.h" 00096 00097 /** @addtogroup STM32L4xx_HAL_Driver 00098 * @{ 00099 */ 00100 00101 #ifdef HAL_SRAM_MODULE_ENABLED 00102 00103 /** @defgroup SRAM SRAM 00104 * @brief SRAM HAL module driver. 00105 * @{ 00106 */ 00107 /* Private typedef -----------------------------------------------------------*/ 00108 /* Private define ------------------------------------------------------------*/ 00109 /* Private macro -------------------------------------------------------------*/ 00110 /* Private variables ---------------------------------------------------------*/ 00111 /* Private function prototypes -----------------------------------------------*/ 00112 /* Exported functions --------------------------------------------------------*/ 00113 00114 /** @defgroup SRAM_Exported_Functions SRAM Exported Functions 00115 * @{ 00116 */ 00117 00118 /** @defgroup SRAM_Exported_Functions_Group1 Initialization and de-initialization functions 00119 * @brief Initialization and Configuration functions. 00120 * 00121 @verbatim 00122 ============================================================================== 00123 ##### SRAM Initialization and de-initialization functions ##### 00124 ============================================================================== 00125 [..] This section provides functions allowing to initialize/de-initialize 00126 the SRAM memory. 00127 00128 @endverbatim 00129 * @{ 00130 */ 00131 00132 /** 00133 * @brief Perform the SRAM device initialization sequence. 00134 * @param hsram: pointer to a SRAM_HandleTypeDef structure that contains 00135 * the configuration information for SRAM module. 00136 * @param Timing: Pointer to SRAM control timing structure 00137 * @param ExtTiming: Pointer to SRAM extended mode timing structure 00138 * @retval HAL status 00139 */ 00140 HAL_StatusTypeDef HAL_SRAM_Init(SRAM_HandleTypeDef *hsram, FMC_NORSRAM_TimingTypeDef *Timing, FMC_NORSRAM_TimingTypeDef *ExtTiming) 00141 { 00142 /* Check the SRAM handle parameter */ 00143 if(hsram == NULL) 00144 { 00145 return HAL_ERROR; 00146 } 00147 00148 if(hsram->State == HAL_SRAM_STATE_RESET) 00149 { 00150 /* Allocate lock resource and initialize it */ 00151 hsram->Lock = HAL_UNLOCKED; 00152 00153 /* Initialize the low level hardware (MSP) */ 00154 HAL_SRAM_MspInit(hsram); 00155 } 00156 00157 /* Initialize SRAM control Interface */ 00158 FMC_NORSRAM_Init(hsram->Instance, &(hsram->Init)); 00159 00160 /* Initialize SRAM timing Interface */ 00161 FMC_NORSRAM_Timing_Init(hsram->Instance, Timing, hsram->Init.NSBank); 00162 00163 /* Initialize SRAM extended mode timing Interface */ 00164 FMC_NORSRAM_Extended_Timing_Init(hsram->Extended, ExtTiming, hsram->Init.NSBank, hsram->Init.ExtendedMode); 00165 00166 /* Enable the NORSRAM device */ 00167 __FMC_NORSRAM_ENABLE(hsram->Instance, hsram->Init.NSBank); 00168 00169 return HAL_OK; 00170 } 00171 00172 /** 00173 * @brief Perform the SRAM device de-initialization sequence. 00174 * @param hsram: pointer to a SRAM_HandleTypeDef structure that contains 00175 * the configuration information for SRAM module. 00176 * @retval HAL status 00177 */ 00178 HAL_StatusTypeDef HAL_SRAM_DeInit(SRAM_HandleTypeDef *hsram) 00179 { 00180 /* De-Initialize the low level hardware (MSP) */ 00181 HAL_SRAM_MspDeInit(hsram); 00182 00183 /* Configure the SRAM registers with their reset values */ 00184 FMC_NORSRAM_DeInit(hsram->Instance, hsram->Extended, hsram->Init.NSBank); 00185 00186 hsram->State = HAL_SRAM_STATE_RESET; 00187 00188 /* Release Lock */ 00189 __HAL_UNLOCK(hsram); 00190 00191 return HAL_OK; 00192 } 00193 00194 /** 00195 * @brief Initialize the SRAM MSP. 00196 * @param hsram: pointer to a SRAM_HandleTypeDef structure that contains 00197 * the configuration information for SRAM module. 00198 * @retval None 00199 */ 00200 __weak void HAL_SRAM_MspInit(SRAM_HandleTypeDef *hsram) 00201 { 00202 /* NOTE : This function should not be modified, when the callback is needed, 00203 the HAL_SRAM_MspInit could be implemented in the user file 00204 */ 00205 } 00206 00207 /** 00208 * @brief DeInitialize the SRAM MSP. 00209 * @param hsram: pointer to a SRAM_HandleTypeDef structure that contains 00210 * the configuration information for SRAM module. 00211 * @retval None 00212 */ 00213 __weak void HAL_SRAM_MspDeInit(SRAM_HandleTypeDef *hsram) 00214 { 00215 /* NOTE : This function should not be modified, when the callback is needed, 00216 the HAL_SRAM_MspDeInit could be implemented in the user file 00217 */ 00218 } 00219 00220 /** 00221 * @brief DMA transfer complete callback. 00222 * @param hdma: pointer to a SRAM_HandleTypeDef structure that contains 00223 * the configuration information for SRAM module. 00224 * @retval None 00225 */ 00226 __weak void HAL_SRAM_DMA_XferCpltCallback(DMA_HandleTypeDef *hdma) 00227 { 00228 /* NOTE : This function should not be modified, when the callback is needed, 00229 the HAL_SRAM_DMA_XferCpltCallback could be implemented in the user file 00230 */ 00231 } 00232 00233 /** 00234 * @brief DMA transfer complete error callback. 00235 * @param hdma: pointer to a SRAM_HandleTypeDef structure that contains 00236 * the configuration information for SRAM module. 00237 * @retval None 00238 */ 00239 __weak void HAL_SRAM_DMA_XferErrorCallback(DMA_HandleTypeDef *hdma) 00240 { 00241 /* NOTE : This function should not be modified, when the callback is needed, 00242 the HAL_SRAM_DMA_XferErrorCallback could be implemented in the user file 00243 */ 00244 } 00245 00246 /** 00247 * @} 00248 */ 00249 00250 /** @defgroup SRAM_Exported_Functions_Group2 Input Output and memory control functions 00251 * @brief Input Output and memory control functions 00252 * 00253 @verbatim 00254 ============================================================================== 00255 ##### SRAM Input and Output functions ##### 00256 ============================================================================== 00257 [..] 00258 This section provides functions allowing to use and control the SRAM memory 00259 00260 @endverbatim 00261 * @{ 00262 */ 00263 00264 /** 00265 * @brief Read 8-bit buffer from SRAM memory. 00266 * @param hsram: pointer to a SRAM_HandleTypeDef structure that contains 00267 * the configuration information for SRAM module. 00268 * @param pAddress: Pointer to read start address 00269 * @param pDstBuffer: Pointer to destination buffer 00270 * @param BufferSize: Size of the buffer to read from memory 00271 * @retval HAL status 00272 */ 00273 HAL_StatusTypeDef HAL_SRAM_Read_8b(SRAM_HandleTypeDef *hsram, uint32_t *pAddress, uint8_t *pDstBuffer, uint32_t BufferSize) 00274 { 00275 __IO uint8_t * psramaddress = (uint8_t *)pAddress; 00276 00277 /* Process Locked */ 00278 __HAL_LOCK(hsram); 00279 00280 /* Update the SRAM controller state */ 00281 hsram->State = HAL_SRAM_STATE_BUSY; 00282 00283 /* Read data from memory */ 00284 for(; BufferSize != 0; BufferSize--) 00285 { 00286 *pDstBuffer = *(__IO uint8_t *)psramaddress; 00287 pDstBuffer++; 00288 psramaddress++; 00289 } 00290 00291 /* Update the SRAM controller state */ 00292 hsram->State = HAL_SRAM_STATE_READY; 00293 00294 /* Process unlocked */ 00295 __HAL_UNLOCK(hsram); 00296 00297 return HAL_OK; 00298 } 00299 00300 /** 00301 * @brief Write 8-bit buffer to SRAM memory. 00302 * @param hsram: pointer to a SRAM_HandleTypeDef structure that contains 00303 * the configuration information for SRAM module. 00304 * @param pAddress: Pointer to write start address 00305 * @param pSrcBuffer: Pointer to source buffer to write 00306 * @param BufferSize: Size of the buffer to write to memory 00307 * @retval HAL status 00308 */ 00309 HAL_StatusTypeDef HAL_SRAM_Write_8b(SRAM_HandleTypeDef *hsram, uint32_t *pAddress, uint8_t *pSrcBuffer, uint32_t BufferSize) 00310 { 00311 __IO uint8_t * psramaddress = (uint8_t *)pAddress; 00312 00313 /* Check the SRAM controller state */ 00314 if(hsram->State == HAL_SRAM_STATE_PROTECTED) 00315 { 00316 return HAL_ERROR; 00317 } 00318 00319 /* Process Locked */ 00320 __HAL_LOCK(hsram); 00321 00322 /* Update the SRAM controller state */ 00323 hsram->State = HAL_SRAM_STATE_BUSY; 00324 00325 /* Write data to memory */ 00326 for(; BufferSize != 0; BufferSize--) 00327 { 00328 *(__IO uint8_t *)psramaddress = *pSrcBuffer; 00329 pSrcBuffer++; 00330 psramaddress++; 00331 } 00332 00333 /* Update the SRAM controller state */ 00334 hsram->State = HAL_SRAM_STATE_READY; 00335 00336 /* Process unlocked */ 00337 __HAL_UNLOCK(hsram); 00338 00339 return HAL_OK; 00340 } 00341 00342 /** 00343 * @brief Read 16-bit buffer from SRAM memory. 00344 * @param hsram: pointer to a SRAM_HandleTypeDef structure that contains 00345 * the configuration information for SRAM module. 00346 * @param pAddress: Pointer to read start address 00347 * @param pDstBuffer: Pointer to destination buffer 00348 * @param BufferSize: Size of the buffer to read from memory 00349 * @retval HAL status 00350 */ 00351 HAL_StatusTypeDef HAL_SRAM_Read_16b(SRAM_HandleTypeDef *hsram, uint32_t *pAddress, uint16_t *pDstBuffer, uint32_t BufferSize) 00352 { 00353 __IO uint16_t * psramaddress = (uint16_t *)pAddress; 00354 00355 /* Process Locked */ 00356 __HAL_LOCK(hsram); 00357 00358 /* Update the SRAM controller state */ 00359 hsram->State = HAL_SRAM_STATE_BUSY; 00360 00361 /* Read data from memory */ 00362 for(; BufferSize != 0; BufferSize--) 00363 { 00364 *pDstBuffer = *(__IO uint16_t *)psramaddress; 00365 pDstBuffer++; 00366 psramaddress++; 00367 } 00368 00369 /* Update the SRAM controller state */ 00370 hsram->State = HAL_SRAM_STATE_READY; 00371 00372 /* Process unlocked */ 00373 __HAL_UNLOCK(hsram); 00374 00375 return HAL_OK; 00376 } 00377 00378 /** 00379 * @brief Write 16-bit buffer to SRAM memory. 00380 * @param hsram: pointer to a SRAM_HandleTypeDef structure that contains 00381 * the configuration information for SRAM module. 00382 * @param pAddress: Pointer to write start address 00383 * @param pSrcBuffer: Pointer to source buffer to write 00384 * @param BufferSize: Size of the buffer to write to memory 00385 * @retval HAL status 00386 */ 00387 HAL_StatusTypeDef HAL_SRAM_Write_16b(SRAM_HandleTypeDef *hsram, uint32_t *pAddress, uint16_t *pSrcBuffer, uint32_t BufferSize) 00388 { 00389 __IO uint16_t * psramaddress = (uint16_t *)pAddress; 00390 00391 /* Check the SRAM controller state */ 00392 if(hsram->State == HAL_SRAM_STATE_PROTECTED) 00393 { 00394 return HAL_ERROR; 00395 } 00396 00397 /* Process Locked */ 00398 __HAL_LOCK(hsram); 00399 00400 /* Update the SRAM controller state */ 00401 hsram->State = HAL_SRAM_STATE_BUSY; 00402 00403 /* Write data to memory */ 00404 for(; BufferSize != 0; BufferSize--) 00405 { 00406 *(__IO uint16_t *)psramaddress = *pSrcBuffer; 00407 pSrcBuffer++; 00408 psramaddress++; 00409 } 00410 00411 /* Update the SRAM controller state */ 00412 hsram->State = HAL_SRAM_STATE_READY; 00413 00414 /* Process unlocked */ 00415 __HAL_UNLOCK(hsram); 00416 00417 return HAL_OK; 00418 } 00419 00420 /** 00421 * @brief Read 32-bit buffer from SRAM memory. 00422 * @param hsram: pointer to a SRAM_HandleTypeDef structure that contains 00423 * the configuration information for SRAM module. 00424 * @param pAddress: Pointer to read start address 00425 * @param pDstBuffer: Pointer to destination buffer 00426 * @param BufferSize: Size of the buffer to read from memory 00427 * @retval HAL status 00428 */ 00429 HAL_StatusTypeDef HAL_SRAM_Read_32b(SRAM_HandleTypeDef *hsram, uint32_t *pAddress, uint32_t *pDstBuffer, uint32_t BufferSize) 00430 { 00431 /* Process Locked */ 00432 __HAL_LOCK(hsram); 00433 00434 /* Update the SRAM controller state */ 00435 hsram->State = HAL_SRAM_STATE_BUSY; 00436 00437 /* Read data from memory */ 00438 for(; BufferSize != 0; BufferSize--) 00439 { 00440 *pDstBuffer = *(__IO uint32_t *)pAddress; 00441 pDstBuffer++; 00442 pAddress++; 00443 } 00444 00445 /* Update the SRAM controller state */ 00446 hsram->State = HAL_SRAM_STATE_READY; 00447 00448 /* Process unlocked */ 00449 __HAL_UNLOCK(hsram); 00450 00451 return HAL_OK; 00452 } 00453 00454 /** 00455 * @brief Write 32-bit buffer to SRAM memory. 00456 * @param hsram: pointer to a SRAM_HandleTypeDef structure that contains 00457 * the configuration information for SRAM module. 00458 * @param pAddress: Pointer to write start address 00459 * @param pSrcBuffer: Pointer to source buffer to write 00460 * @param BufferSize: Size of the buffer to write to memory 00461 * @retval HAL status 00462 */ 00463 HAL_StatusTypeDef HAL_SRAM_Write_32b(SRAM_HandleTypeDef *hsram, uint32_t *pAddress, uint32_t *pSrcBuffer, uint32_t BufferSize) 00464 { 00465 /* Check the SRAM controller state */ 00466 if(hsram->State == HAL_SRAM_STATE_PROTECTED) 00467 { 00468 return HAL_ERROR; 00469 } 00470 00471 /* Process Locked */ 00472 __HAL_LOCK(hsram); 00473 00474 /* Update the SRAM controller state */ 00475 hsram->State = HAL_SRAM_STATE_BUSY; 00476 00477 /* Write data to memory */ 00478 for(; BufferSize != 0; BufferSize--) 00479 { 00480 *(__IO uint32_t *)pAddress = *pSrcBuffer; 00481 pSrcBuffer++; 00482 pAddress++; 00483 } 00484 00485 /* Update the SRAM controller state */ 00486 hsram->State = HAL_SRAM_STATE_READY; 00487 00488 /* Process unlocked */ 00489 __HAL_UNLOCK(hsram); 00490 00491 return HAL_OK; 00492 } 00493 00494 /** 00495 * @brief Read a Word data buffer from the SRAM memory using DMA transfer. 00496 * @param hsram: pointer to a SRAM_HandleTypeDef structure that contains 00497 * the configuration information for SRAM module. 00498 * @param pAddress: Pointer to read start address 00499 * @param pDstBuffer: Pointer to destination buffer 00500 * @param BufferSize: Size of the buffer to read from memory 00501 * @retval HAL status 00502 */ 00503 HAL_StatusTypeDef HAL_SRAM_Read_DMA(SRAM_HandleTypeDef *hsram, uint32_t *pAddress, uint32_t *pDstBuffer, uint32_t BufferSize) 00504 { 00505 /* Process Locked */ 00506 __HAL_LOCK(hsram); 00507 00508 /* Update the SRAM controller state */ 00509 hsram->State = HAL_SRAM_STATE_BUSY; 00510 00511 /* Configure DMA user callbacks */ 00512 hsram->hdma->XferCpltCallback = HAL_SRAM_DMA_XferCpltCallback; 00513 hsram->hdma->XferErrorCallback = HAL_SRAM_DMA_XferErrorCallback; 00514 00515 /* Enable the DMA Channel */ 00516 HAL_DMA_Start_IT(hsram->hdma, (uint32_t)pAddress, (uint32_t)pDstBuffer, (uint32_t)BufferSize); 00517 00518 /* Update the SRAM controller state */ 00519 hsram->State = HAL_SRAM_STATE_READY; 00520 00521 /* Process unlocked */ 00522 __HAL_UNLOCK(hsram); 00523 00524 return HAL_OK; 00525 } 00526 00527 /** 00528 * @brief Write a Word data buffer to SRAM memory using DMA transfer. 00529 * @param hsram: pointer to a SRAM_HandleTypeDef structure that contains 00530 * the configuration information for SRAM module. 00531 * @param pAddress: Pointer to write start address 00532 * @param pSrcBuffer: Pointer to source buffer to write 00533 * @param BufferSize: Size of the buffer to write to memory 00534 * @retval HAL status 00535 */ 00536 HAL_StatusTypeDef HAL_SRAM_Write_DMA(SRAM_HandleTypeDef *hsram, uint32_t *pAddress, uint32_t *pSrcBuffer, uint32_t BufferSize) 00537 { 00538 /* Check the SRAM controller state */ 00539 if(hsram->State == HAL_SRAM_STATE_PROTECTED) 00540 { 00541 return HAL_ERROR; 00542 } 00543 00544 /* Process Locked */ 00545 __HAL_LOCK(hsram); 00546 00547 /* Update the SRAM controller state */ 00548 hsram->State = HAL_SRAM_STATE_BUSY; 00549 00550 /* Configure DMA user callbacks */ 00551 hsram->hdma->XferCpltCallback = HAL_SRAM_DMA_XferCpltCallback; 00552 hsram->hdma->XferErrorCallback = HAL_SRAM_DMA_XferErrorCallback; 00553 00554 /* Enable the DMA Channel */ 00555 HAL_DMA_Start_IT(hsram->hdma, (uint32_t)pSrcBuffer, (uint32_t)pAddress, (uint32_t)BufferSize); 00556 00557 /* Update the SRAM controller state */ 00558 hsram->State = HAL_SRAM_STATE_READY; 00559 00560 /* Process unlocked */ 00561 __HAL_UNLOCK(hsram); 00562 00563 return HAL_OK; 00564 } 00565 00566 /** 00567 * @} 00568 */ 00569 00570 /** @defgroup SRAM_Exported_Functions_Group3 Control functions 00571 * @brief Control functions 00572 * 00573 @verbatim 00574 ============================================================================== 00575 ##### SRAM Control functions ##### 00576 ============================================================================== 00577 [..] 00578 This subsection provides a set of functions allowing to control dynamically 00579 the SRAM interface. 00580 00581 @endverbatim 00582 * @{ 00583 */ 00584 00585 /** 00586 * @brief Enable dynamically SRAM write operation. 00587 * @param hsram: pointer to a SRAM_HandleTypeDef structure that contains 00588 * the configuration information for SRAM module. 00589 * @retval HAL status 00590 */ 00591 HAL_StatusTypeDef HAL_SRAM_WriteOperation_Enable(SRAM_HandleTypeDef *hsram) 00592 { 00593 /* Process Locked */ 00594 __HAL_LOCK(hsram); 00595 00596 /* Enable write operation */ 00597 FMC_NORSRAM_WriteOperation_Enable(hsram->Instance, hsram->Init.NSBank); 00598 00599 /* Update the SRAM controller state */ 00600 hsram->State = HAL_SRAM_STATE_READY; 00601 00602 /* Process unlocked */ 00603 __HAL_UNLOCK(hsram); 00604 00605 return HAL_OK; 00606 } 00607 00608 /** 00609 * @brief Disable dynamically SRAM write operation. 00610 * @param hsram: pointer to a SRAM_HandleTypeDef structure that contains 00611 * the configuration information for SRAM module. 00612 * @retval HAL status 00613 */ 00614 HAL_StatusTypeDef HAL_SRAM_WriteOperation_Disable(SRAM_HandleTypeDef *hsram) 00615 { 00616 /* Process Locked */ 00617 __HAL_LOCK(hsram); 00618 00619 /* Update the SRAM controller state */ 00620 hsram->State = HAL_SRAM_STATE_BUSY; 00621 00622 /* Disable write operation */ 00623 FMC_NORSRAM_WriteOperation_Disable(hsram->Instance, hsram->Init.NSBank); 00624 00625 /* Update the SRAM controller state */ 00626 hsram->State = HAL_SRAM_STATE_PROTECTED; 00627 00628 /* Process unlocked */ 00629 __HAL_UNLOCK(hsram); 00630 00631 return HAL_OK; 00632 } 00633 00634 /** 00635 * @} 00636 */ 00637 00638 /** @defgroup SRAM_Exported_Functions_Group4 Peripheral State functions 00639 * @brief Peripheral State functions 00640 * 00641 @verbatim 00642 ============================================================================== 00643 ##### SRAM State functions ##### 00644 ============================================================================== 00645 [..] 00646 This subsection permits to get in run-time the status of the SRAM controller 00647 and the data flow. 00648 00649 @endverbatim 00650 * @{ 00651 */ 00652 00653 /** 00654 * @brief Return the SRAM controller handle state. 00655 * @param hsram: pointer to a SRAM_HandleTypeDef structure that contains 00656 * the configuration information for SRAM module. 00657 * @retval HAL state 00658 */ 00659 HAL_SRAM_StateTypeDef HAL_SRAM_GetState(SRAM_HandleTypeDef *hsram) 00660 { 00661 /* Return SRAM handle state */ 00662 return hsram->State; 00663 } 00664 00665 /** 00666 * @} 00667 */ 00668 00669 /** 00670 * @} 00671 */ 00672 /** 00673 * @} 00674 */ 00675 #endif /* HAL_SRAM_MODULE_ENABLED */ 00676 00677 /** 00678 * @} 00679 */ 00680 00681 /************************ (C) COPYRIGHT STMicroelectronics *****END OF FILE****/ 00682
Generated on Tue Jul 12 2022 11:35:15 by
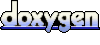