Hal Drivers for L4
Dependents: BSP OneHopeOnePrayer FINAL_AUDIO_RECORD AudioDemo
Fork of STM32L4xx_HAL_Driver by
stm32l4xx_hal_rtc_ex.h
00001 /** 00002 ****************************************************************************** 00003 * @file stm32l4xx_hal_rtc_ex.h 00004 * @author MCD Application Team 00005 * @version V1.1.0 00006 * @date 16-September-2015 00007 * @brief Header file of RTC HAL Extended module. 00008 ****************************************************************************** 00009 * @attention 00010 * 00011 * <h2><center>© COPYRIGHT(c) 2015 STMicroelectronics</center></h2> 00012 * 00013 * Redistribution and use in source and binary forms, with or without modification, 00014 * are permitted provided that the following conditions are met: 00015 * 1. Redistributions of source code must retain the above copyright notice, 00016 * this list of conditions and the following disclaimer. 00017 * 2. Redistributions in binary form must reproduce the above copyright notice, 00018 * this list of conditions and the following disclaimer in the documentation 00019 * and/or other materials provided with the distribution. 00020 * 3. Neither the name of STMicroelectronics nor the names of its contributors 00021 * may be used to endorse or promote products derived from this software 00022 * without specific prior written permission. 00023 * 00024 * THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" 00025 * AND ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE 00026 * IMPLIED WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE 00027 * DISCLAIMED. IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE 00028 * FOR ANY DIRECT, INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL 00029 * DAMAGES (INCLUDING, BUT NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR 00030 * SERVICES; LOSS OF USE, DATA, OR PROFITS; OR BUSINESS INTERRUPTION) HOWEVER 00031 * CAUSED AND ON ANY THEORY OF LIABILITY, WHETHER IN CONTRACT, STRICT LIABILITY, 00032 * OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) ARISING IN ANY WAY OUT OF THE USE 00033 * OF THIS SOFTWARE, EVEN IF ADVISED OF THE POSSIBILITY OF SUCH DAMAGE. 00034 * 00035 ****************************************************************************** 00036 */ 00037 00038 /* Define to prevent recursive inclusion -------------------------------------*/ 00039 #ifndef __STM32L4xx_HAL_RTC_EX_H 00040 #define __STM32L4xx_HAL_RTC_EX_H 00041 00042 #ifdef __cplusplus 00043 extern "C" { 00044 #endif 00045 00046 /* Includes ------------------------------------------------------------------*/ 00047 #include "stm32l4xx_hal_def.h" 00048 00049 /** @addtogroup STM32L4xx_HAL_Driver 00050 * @{ 00051 */ 00052 00053 /** @addtogroup RTCEx 00054 * @{ 00055 */ 00056 00057 /* Exported types ------------------------------------------------------------*/ 00058 /** @defgroup RTCEx_Exported_Types RTCEx Exported Types 00059 * @{ 00060 */ 00061 /** 00062 * @brief RTC Tamper structure definition 00063 */ 00064 typedef struct 00065 { 00066 uint32_t Tamper; /*!< Specifies the Tamper Pin. 00067 This parameter can be a value of @ref RTCEx_Tamper_Pins_Definitions */ 00068 00069 uint32_t Interrupt; /*!< Specifies the Tamper Interrupt. 00070 This parameter can be a value of @ref RTCEx_Tamper_Interrupt_Definitions */ 00071 00072 uint32_t Trigger; /*!< Specifies the Tamper Trigger. 00073 This parameter can be a value of @ref RTCEx_Tamper_Trigger_Definitions */ 00074 00075 uint32_t NoErase; /*!< Specifies the Tamper no erase mode. 00076 This parameter can be a value of @ref RTCEx_Tamper_EraseBackUp_Definitions */ 00077 00078 uint32_t MaskFlag; /*!< Specifies the Tamper Flag masking. 00079 This parameter can be a value of @ref RTCEx_Tamper_MaskFlag_Definitions */ 00080 00081 uint32_t Filter; /*!< Specifies the RTC Filter Tamper. 00082 This parameter can be a value of @ref RTCEx_Tamper_Filter_Definitions */ 00083 00084 uint32_t SamplingFrequency; /*!< Specifies the sampling frequency. 00085 This parameter can be a value of @ref RTCEx_Tamper_Sampling_Frequencies_Definitions */ 00086 00087 uint32_t PrechargeDuration; /*!< Specifies the Precharge Duration . 00088 This parameter can be a value of @ref RTCEx_Tamper_Pin_Precharge_Duration_Definitions */ 00089 00090 uint32_t TamperPullUp; /*!< Specifies the Tamper PullUp . 00091 This parameter can be a value of @ref RTCEx_Tamper_Pull_UP_Definitions */ 00092 00093 uint32_t TimeStampOnTamperDetection; /*!< Specifies the TimeStampOnTamperDetection. 00094 This parameter can be a value of @ref RTCEx_Tamper_TimeStampOnTamperDetection_Definitions */ 00095 }RTC_TamperTypeDef; 00096 00097 /** 00098 * @} 00099 */ 00100 00101 /* Exported constants --------------------------------------------------------*/ 00102 /** @defgroup RTCEx_Exported_Constants RTCEx Exported Constants 00103 * @{ 00104 */ 00105 00106 /** @defgroup RTCEx_Output_selection_Definitions RTC Output Selection Definitions 00107 * @{ 00108 */ 00109 #define RTC_OUTPUT_DISABLE ((uint32_t)0x00000000) 00110 #define RTC_OUTPUT_ALARMA ((uint32_t)0x00200000) 00111 #define RTC_OUTPUT_ALARMB ((uint32_t)0x00400000) 00112 #define RTC_OUTPUT_WAKEUP ((uint32_t)0x00600000) 00113 /** 00114 * @} 00115 */ 00116 00117 /** @defgroup RTCEx_Backup_Registers_Definitions RTC Backup Registers Definitions 00118 * @{ 00119 */ 00120 #define RTC_BKP_DR0 ((uint32_t)0x00000000) 00121 #define RTC_BKP_DR1 ((uint32_t)0x00000001) 00122 #define RTC_BKP_DR2 ((uint32_t)0x00000002) 00123 #define RTC_BKP_DR3 ((uint32_t)0x00000003) 00124 #define RTC_BKP_DR4 ((uint32_t)0x00000004) 00125 #define RTC_BKP_DR5 ((uint32_t)0x00000005) 00126 #define RTC_BKP_DR6 ((uint32_t)0x00000006) 00127 #define RTC_BKP_DR7 ((uint32_t)0x00000007) 00128 #define RTC_BKP_DR8 ((uint32_t)0x00000008) 00129 #define RTC_BKP_DR9 ((uint32_t)0x00000009) 00130 #define RTC_BKP_DR10 ((uint32_t)0x0000000A) 00131 #define RTC_BKP_DR11 ((uint32_t)0x0000000B) 00132 #define RTC_BKP_DR12 ((uint32_t)0x0000000C) 00133 #define RTC_BKP_DR13 ((uint32_t)0x0000000D) 00134 #define RTC_BKP_DR14 ((uint32_t)0x0000000E) 00135 #define RTC_BKP_DR15 ((uint32_t)0x0000000F) 00136 #define RTC_BKP_DR16 ((uint32_t)0x00000010) 00137 #define RTC_BKP_DR17 ((uint32_t)0x00000011) 00138 #define RTC_BKP_DR18 ((uint32_t)0x00000012) 00139 #define RTC_BKP_DR19 ((uint32_t)0x00000013) 00140 #define RTC_BKP_DR20 ((uint32_t)0x00000014) 00141 #define RTC_BKP_DR21 ((uint32_t)0x00000015) 00142 #define RTC_BKP_DR22 ((uint32_t)0x00000016) 00143 #define RTC_BKP_DR23 ((uint32_t)0x00000017) 00144 #define RTC_BKP_DR24 ((uint32_t)0x00000018) 00145 #define RTC_BKP_DR25 ((uint32_t)0x00000019) 00146 #define RTC_BKP_DR26 ((uint32_t)0x0000001A) 00147 #define RTC_BKP_DR27 ((uint32_t)0x0000001B) 00148 #define RTC_BKP_DR28 ((uint32_t)0x0000001C) 00149 #define RTC_BKP_DR29 ((uint32_t)0x0000001D) 00150 #define RTC_BKP_DR30 ((uint32_t)0x0000001E) 00151 #define RTC_BKP_DR31 ((uint32_t)0x0000001F) 00152 /** 00153 * @} 00154 */ 00155 00156 /** @defgroup RTCEx_TimeStamp_Edges_definitions RTC TimeStamp Edges Definitions 00157 * @{ 00158 */ 00159 #define RTC_TIMESTAMPEDGE_RISING ((uint32_t)0x00000000) 00160 #define RTC_TIMESTAMPEDGE_FALLING ((uint32_t)0x00000008) 00161 /** 00162 * @} 00163 */ 00164 00165 /** @defgroup RTCEx_TimeStamp_Pin_Selection RTC TimeStamp Pins Selection 00166 * @{ 00167 */ 00168 #define RTC_TIMESTAMPPIN_DEFAULT ((uint32_t)0x00000000) 00169 /** 00170 * @} 00171 */ 00172 00173 /** @defgroup RTCEx_Tamper_Pins_Definitions RTC Tamper Pins Definitions 00174 * @{ 00175 */ 00176 #define RTC_TAMPER_1 RTC_TAMPCR_TAMP1E 00177 #define RTC_TAMPER_2 RTC_TAMPCR_TAMP2E 00178 #define RTC_TAMPER_3 RTC_TAMPCR_TAMP3E 00179 /** 00180 * @} 00181 */ 00182 00183 /** @defgroup RTCEx_Tamper_Interrupt_Definitions RTC Tamper Interrupts Definitions 00184 * @{ 00185 */ 00186 #define RTC_TAMPER1_INTERRUPT RTC_TAMPCR_TAMP1IE 00187 #define RTC_TAMPER2_INTERRUPT RTC_TAMPCR_TAMP2IE 00188 #define RTC_TAMPER3_INTERRUPT RTC_TAMPCR_TAMP3IE 00189 #define RTC_ALL_TAMPER_INTERRUPT RTC_TAMPCR_TAMPIE 00190 /** 00191 * @} 00192 */ 00193 00194 /** @defgroup RTCEx_Tamper_Trigger_Definitions RTC Tamper Triggers Definitions 00195 * @{ 00196 */ 00197 #define RTC_TAMPERTRIGGER_RISINGEDGE ((uint32_t)0x00000000) 00198 #define RTC_TAMPERTRIGGER_FALLINGEDGE ((uint32_t)0x00000002) 00199 #define RTC_TAMPERTRIGGER_LOWLEVEL RTC_TAMPERTRIGGER_RISINGEDGE 00200 #define RTC_TAMPERTRIGGER_HIGHLEVEL RTC_TAMPERTRIGGER_FALLINGEDGE 00201 /** 00202 * @} 00203 */ 00204 00205 /** @defgroup RTCEx_Tamper_EraseBackUp_Definitions RTC Tamper EraseBackUp Definitions 00206 * @{ 00207 */ 00208 #define RTC_TAMPER_ERASE_BACKUP_ENABLE ((uint32_t)0x00000000) 00209 #define RTC_TAMPER_ERASE_BACKUP_DISABLE ((uint32_t)0x00020000) 00210 /** 00211 * @} 00212 */ 00213 00214 /** @defgroup RTCEx_Tamper_MaskFlag_Definitions RTC Tamper Mask Flag Definitions 00215 * @{ 00216 */ 00217 #define RTC_TAMPERMASK_FLAG_DISABLE ((uint32_t)0x00000000) 00218 #define RTC_TAMPERMASK_FLAG_ENABLE ((uint32_t)0x00040000) 00219 /** 00220 * @} 00221 */ 00222 00223 /** @defgroup RTCEx_Tamper_Filter_Definitions RTC Tamper Filter Definitions 00224 * @{ 00225 */ 00226 #define RTC_TAMPERFILTER_DISABLE ((uint32_t)0x00000000) /*!< Tamper filter is disabled */ 00227 00228 #define RTC_TAMPERFILTER_2SAMPLE ((uint32_t)0x00000800) /*!< Tamper is activated after 2 00229 consecutive samples at the active level */ 00230 #define RTC_TAMPERFILTER_4SAMPLE ((uint32_t)0x00001000) /*!< Tamper is activated after 4 00231 consecutive samples at the active level */ 00232 #define RTC_TAMPERFILTER_8SAMPLE ((uint32_t)0x00001800) /*!< Tamper is activated after 8 00233 consecutive samples at the active level. */ 00234 /** 00235 * @} 00236 */ 00237 00238 /** @defgroup RTCEx_Tamper_Sampling_Frequencies_Definitions RTC Tamper Sampling Frequencies Definitions 00239 * @{ 00240 */ 00241 #define RTC_TAMPERSAMPLINGFREQ_RTCCLK_DIV32768 ((uint32_t)0x00000000) /*!< Each of the tamper inputs are sampled 00242 with a frequency = RTCCLK / 32768 */ 00243 #define RTC_TAMPERSAMPLINGFREQ_RTCCLK_DIV16384 ((uint32_t)0x00000100) /*!< Each of the tamper inputs are sampled 00244 with a frequency = RTCCLK / 16384 */ 00245 #define RTC_TAMPERSAMPLINGFREQ_RTCCLK_DIV8192 ((uint32_t)0x00000200) /*!< Each of the tamper inputs are sampled 00246 with a frequency = RTCCLK / 8192 */ 00247 #define RTC_TAMPERSAMPLINGFREQ_RTCCLK_DIV4096 ((uint32_t)0x00000300) /*!< Each of the tamper inputs are sampled 00248 with a frequency = RTCCLK / 4096 */ 00249 #define RTC_TAMPERSAMPLINGFREQ_RTCCLK_DIV2048 ((uint32_t)0x00000400) /*!< Each of the tamper inputs are sampled 00250 with a frequency = RTCCLK / 2048 */ 00251 #define RTC_TAMPERSAMPLINGFREQ_RTCCLK_DIV1024 ((uint32_t)0x00000500) /*!< Each of the tamper inputs are sampled 00252 with a frequency = RTCCLK / 1024 */ 00253 #define RTC_TAMPERSAMPLINGFREQ_RTCCLK_DIV512 ((uint32_t)0x00000600) /*!< Each of the tamper inputs are sampled 00254 with a frequency = RTCCLK / 512 */ 00255 #define RTC_TAMPERSAMPLINGFREQ_RTCCLK_DIV256 ((uint32_t)0x00000700) /*!< Each of the tamper inputs are sampled 00256 with a frequency = RTCCLK / 256 */ 00257 /** 00258 * @} 00259 */ 00260 00261 /** @defgroup RTCEx_Tamper_Pin_Precharge_Duration_Definitions RTC Tamper Pin Precharge Duration Definitions 00262 * @{ 00263 */ 00264 #define RTC_TAMPERPRECHARGEDURATION_1RTCCLK ((uint32_t)0x00000000) /*!< Tamper pins are pre-charged before 00265 sampling during 1 RTCCLK cycle */ 00266 #define RTC_TAMPERPRECHARGEDURATION_2RTCCLK ((uint32_t)0x00002000) /*!< Tamper pins are pre-charged before 00267 sampling during 2 RTCCLK cycles */ 00268 #define RTC_TAMPERPRECHARGEDURATION_4RTCCLK ((uint32_t)0x00004000) /*!< Tamper pins are pre-charged before 00269 sampling during 4 RTCCLK cycles */ 00270 #define RTC_TAMPERPRECHARGEDURATION_8RTCCLK ((uint32_t)0x00006000) /*!< Tamper pins are pre-charged before 00271 sampling during 8 RTCCLK cycles */ 00272 /** 00273 * @} 00274 */ 00275 00276 /** @defgroup RTCEx_Tamper_TimeStampOnTamperDetection_Definitions RTC Tamper TimeStamp On Tamper Detection Definitions 00277 * @{ 00278 */ 00279 #define RTC_TIMESTAMPONTAMPERDETECTION_ENABLE ((uint32_t)RTC_TAMPCR_TAMPTS) /*!< TimeStamp on Tamper Detection event saved */ 00280 #define RTC_TIMESTAMPONTAMPERDETECTION_DISABLE ((uint32_t)0x00000000) /*!< TimeStamp on Tamper Detection event is not saved */ 00281 /** 00282 * @} 00283 */ 00284 00285 /** @defgroup RTCEx_Tamper_Pull_UP_Definitions RTC Tamper Pull Up Definitions 00286 * @{ 00287 */ 00288 #define RTC_TAMPER_PULLUP_ENABLE ((uint32_t)0x00000000) /*!< TimeStamp on Tamper Detection event saved */ 00289 #define RTC_TAMPER_PULLUP_DISABLE ((uint32_t)RTC_TAMPCR_TAMPPUDIS) /*!< TimeStamp on Tamper Detection event is not saved */ 00290 /** 00291 * @} 00292 */ 00293 00294 /** @defgroup RTCEx_Wakeup_Timer_Definitions RTC Wakeup Timer Definitions 00295 * @{ 00296 */ 00297 #define RTC_WAKEUPCLOCK_RTCCLK_DIV16 ((uint32_t)0x00000000) 00298 #define RTC_WAKEUPCLOCK_RTCCLK_DIV8 ((uint32_t)0x00000001) 00299 #define RTC_WAKEUPCLOCK_RTCCLK_DIV4 ((uint32_t)0x00000002) 00300 #define RTC_WAKEUPCLOCK_RTCCLK_DIV2 ((uint32_t)0x00000003) 00301 #define RTC_WAKEUPCLOCK_CK_SPRE_16BITS ((uint32_t)0x00000004) 00302 #define RTC_WAKEUPCLOCK_CK_SPRE_17BITS ((uint32_t)0x00000006) 00303 /** 00304 * @} 00305 */ 00306 00307 /** @defgroup RTCEx_Smooth_calib_period_Definitions RTC Smooth Calib Period Definitions 00308 * @{ 00309 */ 00310 #define RTC_SMOOTHCALIB_PERIOD_32SEC ((uint32_t)0x00000000) /*!< If RTCCLK = 32768 Hz, Smooth calibration 00311 period is 32s, else 2exp20 RTCCLK seconds */ 00312 #define RTC_SMOOTHCALIB_PERIOD_16SEC ((uint32_t)0x00002000) /*!< If RTCCLK = 32768 Hz, Smooth calibration 00313 period is 16s, else 2exp19 RTCCLK seconds */ 00314 #define RTC_SMOOTHCALIB_PERIOD_8SEC ((uint32_t)0x00004000) /*!< If RTCCLK = 32768 Hz, Smooth calibration 00315 period is 8s, else 2exp18 RTCCLK seconds */ 00316 /** 00317 * @} 00318 */ 00319 00320 /** @defgroup RTCEx_Smooth_calib_Plus_pulses_Definitions RTC Smooth Calib Plus Pulses Definitions 00321 * @{ 00322 */ 00323 #define RTC_SMOOTHCALIB_PLUSPULSES_SET ((uint32_t)0x00008000) /*!< The number of RTCCLK pulses added 00324 during a X -second window = Y - CALM[8:0] 00325 with Y = 512, 256, 128 when X = 32, 16, 8 */ 00326 #define RTC_SMOOTHCALIB_PLUSPULSES_RESET ((uint32_t)0x00000000) /*!< The number of RTCCLK pulses subbstited 00327 during a 32-second window = CALM[8:0] */ 00328 /** 00329 * @} 00330 */ 00331 00332 /** @defgroup RTCEx_Calib_Output_selection_Definitions RTC Calib Output Selection Definitions 00333 * @{ 00334 */ 00335 #define RTC_CALIBOUTPUT_512HZ ((uint32_t)0x00000000) 00336 #define RTC_CALIBOUTPUT_1HZ ((uint32_t)0x00080000) 00337 /** 00338 * @} 00339 */ 00340 00341 /** @defgroup RTCEx_Add_1_Second_Parameter_Definitions RTC Add 1 Second Parameter Definitions 00342 * @{ 00343 */ 00344 #define RTC_SHIFTADD1S_RESET ((uint32_t)0x00000000) 00345 #define RTC_SHIFTADD1S_SET ((uint32_t)0x80000000) 00346 /** 00347 * @} 00348 */ 00349 00350 /** 00351 * @} 00352 */ 00353 00354 /* Exported macros -----------------------------------------------------------*/ 00355 /** @defgroup RTCEx_Exported_Macros RTCEx Exported Macros 00356 * @{ 00357 */ 00358 00359 /** 00360 * @brief Enable the RTC WakeUp Timer peripheral. 00361 * @param __HANDLE__: specifies the RTC handle. 00362 * @retval None 00363 */ 00364 #define __HAL_RTC_WAKEUPTIMER_ENABLE(__HANDLE__) ((__HANDLE__)->Instance->CR |= (RTC_CR_WUTE)) 00365 00366 /** 00367 * @brief Disable the RTC WakeUp Timer peripheral. 00368 * @param __HANDLE__: specifies the RTC handle. 00369 * @retval None 00370 */ 00371 #define __HAL_RTC_WAKEUPTIMER_DISABLE(__HANDLE__) ((__HANDLE__)->Instance->CR &= ~(RTC_CR_WUTE)) 00372 00373 /** 00374 * @brief Enable the RTC WakeUpTimer interrupt. 00375 * @param __HANDLE__: specifies the RTC handle. 00376 * @param __INTERRUPT__: specifies the RTC WakeUpTimer interrupt sources to be enabled. 00377 * This parameter can be: 00378 * @arg RTC_IT_WUT: WakeUpTimer interrupt 00379 * @retval None 00380 */ 00381 #define __HAL_RTC_WAKEUPTIMER_ENABLE_IT(__HANDLE__, __INTERRUPT__) ((__HANDLE__)->Instance->CR |= (__INTERRUPT__)) 00382 00383 /** 00384 * @brief Disable the RTC WakeUpTimer interrupt. 00385 * @param __HANDLE__: specifies the RTC handle. 00386 * @param __INTERRUPT__: specifies the RTC WakeUpTimer interrupt sources to be disabled. 00387 * This parameter can be: 00388 * @arg RTC_IT_WUT: WakeUpTimer interrupt 00389 * @retval None 00390 */ 00391 #define __HAL_RTC_WAKEUPTIMER_DISABLE_IT(__HANDLE__, __INTERRUPT__) ((__HANDLE__)->Instance->CR &= ~(__INTERRUPT__)) 00392 00393 /** 00394 * @brief Check whether the specified RTC WakeUpTimer interrupt has occurred or not. 00395 * @param __HANDLE__: specifies the RTC handle. 00396 * @param __INTERRUPT__: specifies the RTC WakeUpTimer interrupt sources to check. 00397 * This parameter can be: 00398 * @arg RTC_IT_WUT: WakeUpTimer interrupt 00399 * @retval None 00400 */ 00401 #define __HAL_RTC_WAKEUPTIMER_GET_IT(__HANDLE__, __INTERRUPT__) (((((__HANDLE__)->Instance->ISR) & ((__INTERRUPT__)>> 4)) != RESET) ? SET : RESET) 00402 00403 /** 00404 * @brief Check whether the specified RTC Wake Up timer interrupt is enabled or not. 00405 * @param __HANDLE__: specifies the RTC handle. 00406 * @param __INTERRUPT__: specifies the RTC Wake Up timer interrupt sources to check. 00407 * This parameter can be: 00408 * @arg RTC_IT_WUT: WakeUpTimer interrupt 00409 * @retval None 00410 */ 00411 #define __HAL_RTC_WAKEUPTIMER_GET_IT_SOURCE(__HANDLE__, __INTERRUPT__) (((((__HANDLE__)->Instance->CR) & (__INTERRUPT__)) != RESET) ? SET : RESET) 00412 00413 /** 00414 * @brief Get the selected RTC WakeUpTimer's flag status. 00415 * @param __HANDLE__: specifies the RTC handle. 00416 * @param __FLAG__: specifies the RTC WakeUpTimer Flag is pending or not. 00417 * This parameter can be: 00418 * @arg RTC_FLAG_WUTF 00419 * @arg RTC_FLAG_WUTWF 00420 * @retval None 00421 */ 00422 #define __HAL_RTC_WAKEUPTIMER_GET_FLAG(__HANDLE__, __FLAG__) (((((__HANDLE__)->Instance->ISR) & (__FLAG__)) != RESET) ? SET : RESET) 00423 00424 /** 00425 * @brief Clear the RTC Wake Up timer's pending flags. 00426 * @param __HANDLE__: specifies the RTC handle. 00427 * @param __FLAG__: specifies the RTC WakeUpTimer Flag to clear. 00428 * This parameter can be: 00429 * @arg RTC_FLAG_WUTF 00430 * @retval None 00431 */ 00432 #define __HAL_RTC_WAKEUPTIMER_CLEAR_FLAG(__HANDLE__, __FLAG__) ((__HANDLE__)->Instance->ISR) = (~((__FLAG__) | RTC_ISR_INIT)|((__HANDLE__)->Instance->ISR & RTC_ISR_INIT)) 00433 00434 /** 00435 * @brief Enable the RTC Tamper1 input detection. 00436 * @param __HANDLE__: specifies the RTC handle. 00437 * @retval None 00438 */ 00439 #define __HAL_RTC_TAMPER1_ENABLE(__HANDLE__) ((__HANDLE__)->Instance->TAMPCR |= (RTC_TAMPCR_TAMP1E)) 00440 00441 /** 00442 * @brief Disable the RTC Tamper1 input detection. 00443 * @param __HANDLE__: specifies the RTC handle. 00444 * @retval None 00445 */ 00446 #define __HAL_RTC_TAMPER1_DISABLE(__HANDLE__) ((__HANDLE__)->Instance->TAMPCR &= ~(RTC_TAMPCR_TAMP1E)) 00447 00448 /** 00449 * @brief Enable the RTC Tamper2 input detection. 00450 * @param __HANDLE__: specifies the RTC handle. 00451 * @retval None 00452 */ 00453 #define __HAL_RTC_TAMPER2_ENABLE(__HANDLE__) ((__HANDLE__)->Instance->TAMPCR |= (RTC_TAMPCR_TAMP2E)) 00454 00455 /** 00456 * @brief Disable the RTC Tamper2 input detection. 00457 * @param __HANDLE__: specifies the RTC handle. 00458 * @retval None 00459 */ 00460 #define __HAL_RTC_TAMPER2_DISABLE(__HANDLE__) ((__HANDLE__)->Instance->TAMPCR &= ~(RTC_TAMPCR_TAMP2E)) 00461 00462 /** 00463 * @brief Enable the RTC Tamper3 input detection. 00464 * @param __HANDLE__: specifies the RTC handle. 00465 * @retval None 00466 */ 00467 #define __HAL_RTC_TAMPER3_ENABLE(__HANDLE__) ((__HANDLE__)->Instance->TAMPCR |= (RTC_TAMPCR_TAMP3E)) 00468 00469 /** 00470 * @brief Disable the RTC Tamper3 input detection. 00471 * @param __HANDLE__: specifies the RTC handle. 00472 * @retval None 00473 */ 00474 #define __HAL_RTC_TAMPER3_DISABLE(__HANDLE__) ((__HANDLE__)->Instance->TAMPCR &= ~(RTC_TAMPCR_TAMP3E)) 00475 00476 /** 00477 * @brief Enable the RTC Tamper interrupt. 00478 * @param __HANDLE__: specifies the RTC handle. 00479 * @param __INTERRUPT__: specifies the RTC Tamper interrupt sources to be enabled. 00480 * This parameter can be any combination of the following values: 00481 * @arg RTC_IT_TAMP: All tampers interrupts 00482 * @arg RTC_IT_TAMP1: Tamper1 interrupt 00483 * @arg RTC_IT_TAMP2: Tamper2 interrupt 00484 * @arg RTC_IT_TAMP3: Tamper3 interrupt 00485 * @retval None 00486 */ 00487 #define __HAL_RTC_TAMPER_ENABLE_IT(__HANDLE__, __INTERRUPT__) ((__HANDLE__)->Instance->TAMPCR |= (__INTERRUPT__)) 00488 00489 /** 00490 * @brief Disable the RTC Tamper interrupt. 00491 * @param __HANDLE__: specifies the RTC handle. 00492 * @param __INTERRUPT__: specifies the RTC Tamper interrupt sources to be disabled. 00493 * This parameter can be any combination of the following values: 00494 * @arg RTC_IT_TAMP: All tampers interrupts 00495 * @arg RTC_IT_TAMP1: Tamper1 interrupt 00496 * @arg RTC_IT_TAMP2: Tamper2 interrupt 00497 * @arg RTC_IT_TAMP3: Tamper3 interrupt 00498 * @retval None 00499 */ 00500 #define __HAL_RTC_TAMPER_DISABLE_IT(__HANDLE__, __INTERRUPT__) ((__HANDLE__)->Instance->TAMPCR &= ~(__INTERRUPT__)) 00501 00502 /** 00503 * @brief Check whether the specified RTC Tamper interrupt has occurred or not. 00504 * @param __HANDLE__: specifies the RTC handle. 00505 * @param __INTERRUPT__: specifies the RTC Tamper interrupt to check. 00506 * This parameter can be: 00507 * @arg RTC_IT_TAMP1: Tamper1 interrupt 00508 * @arg RTC_IT_TAMP2: Tamper2 interrupt 00509 * @arg RTC_IT_TAMP3: Tamper3 interrupt 00510 * @retval None 00511 */ 00512 #define __HAL_RTC_TAMPER_GET_IT(__HANDLE__, __INTERRUPT__) (((__INTERRUPT__) == RTC_IT_TAMP1) ? (((((__HANDLE__)->Instance->ISR) & ((__INTERRUPT__)>> 3)) != RESET) ? SET : RESET) : \ 00513 ((__INTERRUPT__) == RTC_IT_TAMP2) ? (((((__HANDLE__)->Instance->ISR) & ((__INTERRUPT__)>> 5)) != RESET) ? SET : RESET) : \ 00514 (((((__HANDLE__)->Instance->ISR) & ((__INTERRUPT__)>> 7)) != RESET) ? SET : RESET)) 00515 00516 /** 00517 * @brief Check whether the specified RTC Tamper interrupt is enabled or not. 00518 * @param __HANDLE__: specifies the RTC handle. 00519 * @param __INTERRUPT__: specifies the RTC Tamper interrupt source to check. 00520 * This parameter can be: 00521 * @arg RTC_IT_TAMP: All tampers interrupts 00522 * @arg RTC_IT_TAMP1: Tamper1 interrupt 00523 * @arg RTC_IT_TAMP2: Tamper2 interrupt 00524 * @arg RTC_IT_TAMP3: Tamper3 interrupt 00525 * @retval None 00526 */ 00527 #define __HAL_RTC_TAMPER_GET_IT_SOURCE(__HANDLE__, __INTERRUPT__) (((((__HANDLE__)->Instance->TAMPCR) & (__INTERRUPT__)) != RESET) ? SET : RESET) 00528 00529 /** 00530 * @brief Get the selected RTC Tamper's flag status. 00531 * @param __HANDLE__: specifies the RTC handle. 00532 * @param __FLAG__: specifies the RTC Tamper Flag is pending or not. 00533 * This parameter can be: 00534 * @arg RTC_FLAG_TAMP1F: Tamper1 flag 00535 * @arg RTC_FLAG_TAMP2F: Tamper2 flag 00536 * @arg RTC_FLAG_TAMP3F: Tamper3 flag 00537 * @retval None 00538 */ 00539 #define __HAL_RTC_TAMPER_GET_FLAG(__HANDLE__, __FLAG__) (((((__HANDLE__)->Instance->ISR) & (__FLAG__)) != RESET) ? SET : RESET) 00540 00541 /** 00542 * @brief Clear the RTC Tamper's pending flags. 00543 * @param __HANDLE__: specifies the RTC handle. 00544 * @param __FLAG__: specifies the RTC Tamper Flag sources to clear. 00545 * This parameter can be: 00546 * @arg RTC_FLAG_TAMP1F: Tamper1 flag 00547 * @arg RTC_FLAG_TAMP2F: Tamper2 flag 00548 * @arg RTC_FLAG_TAMP3F: Tamper3 flag 00549 * @retval None 00550 */ 00551 #define __HAL_RTC_TAMPER_CLEAR_FLAG(__HANDLE__, __FLAG__) ((__HANDLE__)->Instance->ISR) = (~((__FLAG__) | RTC_ISR_INIT)|((__HANDLE__)->Instance->ISR & RTC_ISR_INIT)) 00552 00553 /** 00554 * @brief Enable the RTC TimeStamp peripheral. 00555 * @param __HANDLE__: specifies the RTC handle. 00556 * @retval None 00557 */ 00558 #define __HAL_RTC_TIMESTAMP_ENABLE(__HANDLE__) ((__HANDLE__)->Instance->CR |= (RTC_CR_TSE)) 00559 00560 /** 00561 * @brief Disable the RTC TimeStamp peripheral. 00562 * @param __HANDLE__: specifies the RTC handle. 00563 * @retval None 00564 */ 00565 #define __HAL_RTC_TIMESTAMP_DISABLE(__HANDLE__) ((__HANDLE__)->Instance->CR &= ~(RTC_CR_TSE)) 00566 00567 /** 00568 * @brief Enable the RTC TimeStamp interrupt. 00569 * @param __HANDLE__: specifies the RTC handle. 00570 * @param __INTERRUPT__: specifies the RTC TimeStamp interrupt source to be enabled. 00571 * This parameter can be: 00572 * @arg RTC_IT_TS: TimeStamp interrupt 00573 * @retval None 00574 */ 00575 #define __HAL_RTC_TIMESTAMP_ENABLE_IT(__HANDLE__, __INTERRUPT__) ((__HANDLE__)->Instance->CR |= (__INTERRUPT__)) 00576 00577 /** 00578 * @brief Disable the RTC TimeStamp interrupt. 00579 * @param __HANDLE__: specifies the RTC handle. 00580 * @param __INTERRUPT__: specifies the RTC TimeStamp interrupt source to be disabled. 00581 * This parameter can be: 00582 * @arg RTC_IT_TS: TimeStamp interrupt 00583 * @retval None 00584 */ 00585 #define __HAL_RTC_TIMESTAMP_DISABLE_IT(__HANDLE__, __INTERRUPT__) ((__HANDLE__)->Instance->CR &= ~(__INTERRUPT__)) 00586 00587 /** 00588 * @brief Check whether the specified RTC TimeStamp interrupt has occurred or not. 00589 * @param __HANDLE__: specifies the RTC handle. 00590 * @param __INTERRUPT__: specifies the RTC TimeStamp interrupt source to check. 00591 * This parameter can be: 00592 * @arg RTC_IT_TS: TimeStamp interrupt 00593 * @retval None 00594 */ 00595 #define __HAL_RTC_TIMESTAMP_GET_IT(__HANDLE__, __INTERRUPT__) (((((__HANDLE__)->Instance->ISR) & ((__INTERRUPT__)>> 4)) != RESET) ? SET : RESET) 00596 00597 /** 00598 * @brief Check whether the specified RTC Time Stamp interrupt is enabled or not. 00599 * @param __HANDLE__: specifies the RTC handle. 00600 * @param __INTERRUPT__: specifies the RTC Time Stamp interrupt source to check. 00601 * This parameter can be: 00602 * @arg RTC_IT_TS: TimeStamp interrupt 00603 * @retval None 00604 */ 00605 #define __HAL_RTC_TIMESTAMP_GET_IT_SOURCE(__HANDLE__, __INTERRUPT__) (((((__HANDLE__)->Instance->CR) & (__INTERRUPT__)) != RESET) ? SET : RESET) 00606 00607 /** 00608 * @brief Get the selected RTC TimeStamp's flag status. 00609 * @param __HANDLE__: specifies the RTC handle. 00610 * @param __FLAG__: specifies the RTC TimeStamp Flag is pending or not. 00611 * This parameter can be: 00612 * @arg RTC_FLAG_TSF 00613 * @arg RTC_FLAG_TSOVF 00614 * @retval None 00615 */ 00616 #define __HAL_RTC_TIMESTAMP_GET_FLAG(__HANDLE__, __FLAG__) (((((__HANDLE__)->Instance->ISR) & (__FLAG__)) != RESET) ? SET : RESET) 00617 00618 /** 00619 * @brief Clear the RTC Time Stamp's pending flags. 00620 * @param __HANDLE__: specifies the RTC handle. 00621 * @param __FLAG__: specifies the RTC Alarm Flag sources to clear. 00622 * This parameter can be: 00623 * @arg RTC_FLAG_TSF 00624 * @arg RTC_FLAG_TSOVF 00625 * @retval None 00626 */ 00627 #define __HAL_RTC_TIMESTAMP_CLEAR_FLAG(__HANDLE__, __FLAG__) ((__HANDLE__)->Instance->ISR) = (~((__FLAG__) | RTC_ISR_INIT)|((__HANDLE__)->Instance->ISR & RTC_ISR_INIT)) 00628 00629 /** 00630 * @brief Enable the RTC internal TimeStamp peripheral. 00631 * @param __HANDLE__: specifies the RTC handle. 00632 * @retval None 00633 */ 00634 #define __HAL_RTC_INTERNAL_TIMESTAMP_ENABLE(__HANDLE__) ((__HANDLE__)->Instance->CR |= (RTC_CR_ITSE)) 00635 00636 /** 00637 * @brief Disable the RTC internal TimeStamp peripheral. 00638 * @param __HANDLE__: specifies the RTC handle. 00639 * @retval None 00640 */ 00641 #define __HAL_RTC_INTERNAL_TIMESTAMP_DISABLE(__HANDLE__) ((__HANDLE__)->Instance->CR &= ~(RTC_CR_ITSE)) 00642 00643 /** 00644 * @brief Get the selected RTC Internal Time Stamp's flag status. 00645 * @param __HANDLE__: specifies the RTC handle. 00646 * @param __FLAG__: specifies the RTC Internal Time Stamp Flag is pending or not. 00647 * This parameter can be: 00648 * @arg RTC_FLAG_ITSF 00649 * @retval None 00650 */ 00651 #define __HAL_RTC_INTERNAL_TIMESTAMP_GET_FLAG(__HANDLE__, __FLAG__) (((((__HANDLE__)->Instance->ISR) & (__FLAG__)) != RESET) ? SET : RESET) 00652 00653 /** 00654 * @brief Clear the RTC Internal Time Stamp's pending flags. 00655 * @param __HANDLE__: specifies the RTC handle. 00656 * @param __FLAG__: specifies the RTC Internal Time Stamp Flag source to clear. 00657 * This parameter can be: 00658 * @arg RTC_FLAG_ITSF 00659 * @retval None 00660 */ 00661 #define __HAL_RTC_INTERNAL_TIMESTAMP_CLEAR_FLAG(__HANDLE__, __FLAG__) ((__HANDLE__)->Instance->ISR) = (~((__FLAG__) | RTC_ISR_INIT)|((__HANDLE__)->Instance->ISR & RTC_ISR_INIT)) 00662 00663 /** 00664 * @brief Enable the RTC calibration output. 00665 * @param __HANDLE__: specifies the RTC handle. 00666 * @retval None 00667 */ 00668 #define __HAL_RTC_CALIBRATION_OUTPUT_ENABLE(__HANDLE__) ((__HANDLE__)->Instance->CR |= (RTC_CR_COE)) 00669 00670 /** 00671 * @brief Disable the calibration output. 00672 * @param __HANDLE__: specifies the RTC handle. 00673 * @retval None 00674 */ 00675 #define __HAL_RTC_CALIBRATION_OUTPUT_DISABLE(__HANDLE__) ((__HANDLE__)->Instance->CR &= ~(RTC_CR_COE)) 00676 00677 /** 00678 * @brief Enable the clock reference detection. 00679 * @param __HANDLE__: specifies the RTC handle. 00680 * @retval None 00681 */ 00682 #define __HAL_RTC_CLOCKREF_DETECTION_ENABLE(__HANDLE__) ((__HANDLE__)->Instance->CR |= (RTC_CR_REFCKON)) 00683 00684 /** 00685 * @brief Disable the clock reference detection. 00686 * @param __HANDLE__: specifies the RTC handle. 00687 * @retval None 00688 */ 00689 #define __HAL_RTC_CLOCKREF_DETECTION_DISABLE(__HANDLE__) ((__HANDLE__)->Instance->CR &= ~(RTC_CR_REFCKON)) 00690 00691 /** 00692 * @brief Get the selected RTC shift operation's flag status. 00693 * @param __HANDLE__: specifies the RTC handle. 00694 * @param __FLAG__: specifies the RTC shift operation Flag is pending or not. 00695 * This parameter can be: 00696 * @arg RTC_FLAG_SHPF 00697 * @retval None 00698 */ 00699 #define __HAL_RTC_SHIFT_GET_FLAG(__HANDLE__, __FLAG__) (((((__HANDLE__)->Instance->ISR) & (__FLAG__)) != RESET) ? SET : RESET) 00700 00701 /** 00702 * @brief Enable interrupt on the RTC WakeUp Timer associated Exti line. 00703 * @retval None 00704 */ 00705 #define __HAL_RTC_WAKEUPTIMER_EXTI_ENABLE_IT() (EXTI->IMR1 |= RTC_EXTI_LINE_WAKEUPTIMER_EVENT) 00706 00707 /** 00708 * @brief Disable interrupt on the RTC WakeUp Timer associated Exti line. 00709 * @retval None 00710 */ 00711 #define __HAL_RTC_WAKEUPTIMER_EXTI_DISABLE_IT() (EXTI->IMR1 &= ~(RTC_EXTI_LINE_WAKEUPTIMER_EVENT)) 00712 00713 /** 00714 * @brief Enable event on the RTC WakeUp Timer associated Exti line. 00715 * @retval None 00716 */ 00717 #define __HAL_RTC_WAKEUPTIMER_EXTI_ENABLE_EVENT() (EXTI->EMR1 |= RTC_EXTI_LINE_WAKEUPTIMER_EVENT) 00718 00719 /** 00720 * @brief Disable event on the RTC WakeUp Timer associated Exti line. 00721 * @retval None 00722 */ 00723 #define __HAL_RTC_WAKEUPTIMER_EXTI_DISABLE_EVENT() (EXTI->EMR1 &= ~(RTC_EXTI_LINE_WAKEUPTIMER_EVENT)) 00724 00725 /** 00726 * @brief Enable falling edge trigger on the RTC WakeUp Timer associated Exti line. 00727 * @retval None 00728 */ 00729 #define __HAL_RTC_WAKEUPTIMER_EXTI_ENABLE_FALLING_EDGE() (EXTI->FTSR1 |= RTC_EXTI_LINE_WAKEUPTIMER_EVENT) 00730 00731 /** 00732 * @brief Disable falling edge trigger on the RTC WakeUp Timer associated Exti line. 00733 * @retval None 00734 */ 00735 #define __HAL_RTC_WAKEUPTIMER_EXTI_DISABLE_FALLING_EDGE() (EXTI->FTSR1 &= ~(RTC_EXTI_LINE_WAKEUPTIMER_EVENT)) 00736 00737 /** 00738 * @brief Enable rising edge trigger on the RTC WakeUp Timer associated Exti line. 00739 * @retval None 00740 */ 00741 #define __HAL_RTC_WAKEUPTIMER_EXTI_ENABLE_RISING_EDGE() (EXTI->RTSR1 |= RTC_EXTI_LINE_WAKEUPTIMER_EVENT) 00742 00743 /** 00744 * @brief Disable rising edge trigger on the RTC WakeUp Timer associated Exti line. 00745 * @retval None 00746 */ 00747 #define __HAL_RTC_WAKEUPTIMER_EXTI_DISABLE_RISING_EDGE() (EXTI->RTSR1 &= ~(RTC_EXTI_LINE_WAKEUPTIMER_EVENT)) 00748 00749 /** 00750 * @brief Enable rising & falling edge trigger on the RTC WakeUp Timer associated Exti line. 00751 * @retval None 00752 */ 00753 #define __HAL_RTC_WAKEUPTIMER_EXTI_ENABLE_RISING_FALLING_EDGE() do { \ 00754 __HAL_RTC_WAKEUPTIMER_EXTI_ENABLE_RISING_EDGE(); \ 00755 __HAL_RTC_WAKEUPTIMER_EXTI_ENABLE_FALLING_EDGE(); \ 00756 } while(0) 00757 00758 /** 00759 * @brief Disable rising & falling edge trigger on the RTC WakeUp Timer associated Exti line. 00760 * This parameter can be: 00761 * @retval None 00762 */ 00763 #define __HAL_RTC_WAKEUPTIMER_EXTI_DISABLE_RISING_FALLING_EDGE() do { \ 00764 __HAL_RTC_WAKEUPTIMER_EXTI_DISABLE_RISING_EDGE(); \ 00765 __HAL_RTC_WAKEUPTIMER_EXTI_DISABLE_FALLING_EDGE(); \ 00766 } while(0) 00767 00768 /** 00769 * @brief Check whether the RTC WakeUp Timer associated Exti line interrupt flag is set or not. 00770 * @retval Line Status. 00771 */ 00772 #define __HAL_RTC_WAKEUPTIMER_EXTI_GET_FLAG() (EXTI->PR1 & RTC_EXTI_LINE_WAKEUPTIMER_EVENT) 00773 00774 /** 00775 * @brief Clear the RTC WakeUp Timer associated Exti line flag. 00776 * @retval None 00777 */ 00778 #define __HAL_RTC_WAKEUPTIMER_EXTI_CLEAR_FLAG() (EXTI->PR1 = RTC_EXTI_LINE_WAKEUPTIMER_EVENT) 00779 00780 /** 00781 * @brief Generate a Software interrupt on the RTC WakeUp Timer associated Exti line. 00782 * @retval None 00783 */ 00784 #define __HAL_RTC_WAKEUPTIMER_EXTI_GENERATE_SWIT() (EXTI->SWIER1 |= RTC_EXTI_LINE_WAKEUPTIMER_EVENT) 00785 00786 /** 00787 * @brief Enable interrupt on the RTC Tamper and Timestamp associated Exti line. 00788 * @retval None 00789 */ 00790 #define __HAL_RTC_TAMPER_TIMESTAMP_EXTI_ENABLE_IT() (EXTI->IMR1 |= RTC_EXTI_LINE_TAMPER_TIMESTAMP_EVENT) 00791 00792 /** 00793 * @brief Disable interrupt on the RTC Tamper and Timestamp associated Exti line. 00794 * @retval None 00795 */ 00796 #define __HAL_RTC_TAMPER_TIMESTAMP_EXTI_DISABLE_IT() (EXTI->IMR1 &= ~(RTC_EXTI_LINE_TAMPER_TIMESTAMP_EVENT)) 00797 00798 /** 00799 * @brief Enable event on the RTC Tamper and Timestamp associated Exti line. 00800 * @retval None 00801 */ 00802 #define __HAL_RTC_TAMPER_TIMESTAMP_EXTI_ENABLE_EVENT() (EXTI->EMR1 |= RTC_EXTI_LINE_TAMPER_TIMESTAMP_EVENT) 00803 00804 /** 00805 * @brief Disable event on the RTC Tamper and Timestamp associated Exti line. 00806 * @retval None 00807 */ 00808 #define __HAL_RTC_TAMPER_TIMESTAMP_EXTI_DISABLE_EVENT() (EXTI->EMR1 &= ~(RTC_EXTI_LINE_TAMPER_TIMESTAMP_EVENT)) 00809 00810 /** 00811 * @brief Enable falling edge trigger on the RTC Tamper and Timestamp associated Exti line. 00812 * @retval None 00813 */ 00814 #define __HAL_RTC_TAMPER_TIMESTAMP_EXTI_ENABLE_FALLING_EDGE() (EXTI->FTSR1 |= RTC_EXTI_LINE_TAMPER_TIMESTAMP_EVENT) 00815 00816 /** 00817 * @brief Disable falling edge trigger on the RTC Tamper and Timestamp associated Exti line. 00818 * @retval None 00819 */ 00820 #define __HAL_RTC_TAMPER_TIMESTAMP_EXTI_DISABLE_FALLING_EDGE() (EXTI->FTSR1 &= ~(RTC_EXTI_LINE_TAMPER_TIMESTAMP_EVENT)) 00821 00822 /** 00823 * @brief Enable rising edge trigger on the RTC Tamper and Timestamp associated Exti line. 00824 * @retval None 00825 */ 00826 #define __HAL_RTC_TAMPER_TIMESTAMP_EXTI_ENABLE_RISING_EDGE() (EXTI->RTSR1 |= RTC_EXTI_LINE_TAMPER_TIMESTAMP_EVENT) 00827 00828 /** 00829 * @brief Disable rising edge trigger on the RTC Tamper and Timestamp associated Exti line. 00830 * @retval None 00831 */ 00832 #define __HAL_RTC_TAMPER_TIMESTAMP_EXTI_DISABLE_RISING_EDGE() (EXTI->RTSR1 &= ~(RTC_EXTI_LINE_TAMPER_TIMESTAMP_EVENT)) 00833 00834 /** 00835 * @brief Enable rising & falling edge trigger on the RTC Tamper and Timestamp associated Exti line. 00836 * @retval None 00837 */ 00838 #define __HAL_RTC_TAMPER_TIMESTAMP_EXTI_ENABLE_RISING_FALLING_EDGE() do { \ 00839 __HAL_RTC_TAMPER_TIMESTAMP_EXTI_ENABLE_RISING_EDGE(); \ 00840 __HAL_RTC_TAMPER_TIMESTAMP_EXTI_ENABLE_FALLING_EDGE(); \ 00841 } while(0) 00842 00843 /** 00844 * @brief Disable rising & falling edge trigger on the RTC Tamper and Timestamp associated Exti line. 00845 * This parameter can be: 00846 * @retval None 00847 */ 00848 #define __HAL_RTC_TAMPER_TIMESTAMP_EXTI_DISABLE_RISING_FALLING_EDGE() do { \ 00849 __HAL_RTC_TAMPER_TIMESTAMP_EXTI_DISABLE_RISING_EDGE(); \ 00850 __HAL_RTC_TAMPER_TIMESTAMP_EXTI_DISABLE_FALLING_EDGE(); \ 00851 } while(0) 00852 00853 /** 00854 * @brief Check whether the RTC Tamper and Timestamp associated Exti line interrupt flag is set or not. 00855 * @retval Line Status. 00856 */ 00857 #define __HAL_RTC_TAMPER_TIMESTAMP_EXTI_GET_FLAG() (EXTI->PR1 & RTC_EXTI_LINE_TAMPER_TIMESTAMP_EVENT) 00858 00859 /** 00860 * @brief Clear the RTC Tamper and Timestamp associated Exti line flag. 00861 * @retval None 00862 */ 00863 #define __HAL_RTC_TAMPER_TIMESTAMP_EXTI_CLEAR_FLAG() (EXTI->PR1 = RTC_EXTI_LINE_TAMPER_TIMESTAMP_EVENT) 00864 00865 /** 00866 * @brief Generate a Software interrupt on the RTC Tamper and Timestamp associated Exti line 00867 * @retval None 00868 */ 00869 #define __HAL_RTC_TAMPER_TIMESTAMP_EXTI_GENERATE_SWIT() (EXTI->SWIER1 |= RTC_EXTI_LINE_TAMPER_TIMESTAMP_EVENT) 00870 00871 /** 00872 * @} 00873 */ 00874 00875 /* Exported functions --------------------------------------------------------*/ 00876 /** @addtogroup RTCEx_Exported_Functions 00877 * @{ 00878 */ 00879 00880 /* RTC TimeStamp and Tamper functions *****************************************/ 00881 /** @addtogroup RTCEx_Exported_Functions_Group1 00882 * @{ 00883 */ 00884 HAL_StatusTypeDef HAL_RTCEx_SetTimeStamp(RTC_HandleTypeDef *hrtc, uint32_t TimeStampEdge, uint32_t RTC_TimeStampPin); 00885 HAL_StatusTypeDef HAL_RTCEx_SetTimeStamp_IT(RTC_HandleTypeDef *hrtc, uint32_t TimeStampEdge, uint32_t RTC_TimeStampPin); 00886 HAL_StatusTypeDef HAL_RTCEx_DeactivateTimeStamp(RTC_HandleTypeDef *hrtc); 00887 HAL_StatusTypeDef HAL_RTCEx_SetInternalTimeStamp(RTC_HandleTypeDef *hrtc); 00888 HAL_StatusTypeDef HAL_RTCEx_DeactivateInternalTimeStamp(RTC_HandleTypeDef *hrtc); 00889 HAL_StatusTypeDef HAL_RTCEx_GetTimeStamp(RTC_HandleTypeDef *hrtc, RTC_TimeTypeDef *sTimeStamp, RTC_DateTypeDef *sTimeStampDate, uint32_t Format); 00890 00891 HAL_StatusTypeDef HAL_RTCEx_SetTamper(RTC_HandleTypeDef *hrtc, RTC_TamperTypeDef* sTamper); 00892 HAL_StatusTypeDef HAL_RTCEx_SetTamper_IT(RTC_HandleTypeDef *hrtc, RTC_TamperTypeDef* sTamper); 00893 HAL_StatusTypeDef HAL_RTCEx_DeactivateTamper(RTC_HandleTypeDef *hrtc, uint32_t Tamper); 00894 void HAL_RTCEx_TamperTimeStampIRQHandler(RTC_HandleTypeDef *hrtc); 00895 00896 void HAL_RTCEx_Tamper1EventCallback(RTC_HandleTypeDef *hrtc); 00897 void HAL_RTCEx_Tamper2EventCallback(RTC_HandleTypeDef *hrtc); 00898 void HAL_RTCEx_Tamper3EventCallback(RTC_HandleTypeDef *hrtc); 00899 void HAL_RTCEx_TimeStampEventCallback(RTC_HandleTypeDef *hrtc); 00900 HAL_StatusTypeDef HAL_RTCEx_PollForTimeStampEvent(RTC_HandleTypeDef *hrtc, uint32_t Timeout); 00901 HAL_StatusTypeDef HAL_RTCEx_PollForTamper1Event(RTC_HandleTypeDef *hrtc, uint32_t Timeout); 00902 HAL_StatusTypeDef HAL_RTCEx_PollForTamper2Event(RTC_HandleTypeDef *hrtc, uint32_t Timeout); 00903 HAL_StatusTypeDef HAL_RTCEx_PollForTamper3Event(RTC_HandleTypeDef *hrtc, uint32_t Timeout); 00904 /** 00905 * @} 00906 */ 00907 00908 /* RTC Wake-up functions ******************************************************/ 00909 /** @addtogroup RTCEx_Exported_Functions_Group2 00910 * @{ 00911 */ 00912 HAL_StatusTypeDef HAL_RTCEx_SetWakeUpTimer(RTC_HandleTypeDef *hrtc, uint32_t WakeUpCounter, uint32_t WakeUpClock); 00913 HAL_StatusTypeDef HAL_RTCEx_SetWakeUpTimer_IT(RTC_HandleTypeDef *hrtc, uint32_t WakeUpCounter, uint32_t WakeUpClock); 00914 uint32_t HAL_RTCEx_DeactivateWakeUpTimer(RTC_HandleTypeDef *hrtc); 00915 uint32_t HAL_RTCEx_GetWakeUpTimer(RTC_HandleTypeDef *hrtc); 00916 void HAL_RTCEx_WakeUpTimerIRQHandler(RTC_HandleTypeDef *hrtc); 00917 void HAL_RTCEx_WakeUpTimerEventCallback(RTC_HandleTypeDef *hrtc); 00918 HAL_StatusTypeDef HAL_RTCEx_PollForWakeUpTimerEvent(RTC_HandleTypeDef *hrtc, uint32_t Timeout); 00919 /** 00920 * @} 00921 */ 00922 00923 /* Extended Control functions ************************************************/ 00924 /** @addtogroup RTCEx_Exported_Functions_Group3 00925 * @{ 00926 */ 00927 void HAL_RTCEx_BKUPWrite(RTC_HandleTypeDef *hrtc, uint32_t BackupRegister, uint32_t Data); 00928 uint32_t HAL_RTCEx_BKUPRead(RTC_HandleTypeDef *hrtc, uint32_t BackupRegister); 00929 00930 HAL_StatusTypeDef HAL_RTCEx_SetSmoothCalib(RTC_HandleTypeDef *hrtc, uint32_t SmoothCalibPeriod, uint32_t SmoothCalibPlusPulses, uint32_t SmouthCalibMinusPulsesValue); 00931 HAL_StatusTypeDef HAL_RTCEx_SetSynchroShift(RTC_HandleTypeDef *hrtc, uint32_t ShiftAdd1S, uint32_t ShiftSubFS); 00932 HAL_StatusTypeDef HAL_RTCEx_SetCalibrationOutPut(RTC_HandleTypeDef *hrtc, uint32_t CalibOutput); 00933 HAL_StatusTypeDef HAL_RTCEx_DeactivateCalibrationOutPut(RTC_HandleTypeDef *hrtc); 00934 HAL_StatusTypeDef HAL_RTCEx_SetRefClock(RTC_HandleTypeDef *hrtc); 00935 HAL_StatusTypeDef HAL_RTCEx_DeactivateRefClock(RTC_HandleTypeDef *hrtc); 00936 HAL_StatusTypeDef HAL_RTCEx_EnableBypassShadow(RTC_HandleTypeDef *hrtc); 00937 HAL_StatusTypeDef HAL_RTCEx_DisableBypassShadow(RTC_HandleTypeDef *hrtc); 00938 /** 00939 * @} 00940 */ 00941 00942 /* Extended RTC features functions *******************************************/ 00943 /** @addtogroup RTCEx_Exported_Functions_Group4 00944 * @{ 00945 */ 00946 void HAL_RTCEx_AlarmBEventCallback(RTC_HandleTypeDef *hrtc); 00947 HAL_StatusTypeDef HAL_RTCEx_PollForAlarmBEvent(RTC_HandleTypeDef *hrtc, uint32_t Timeout); 00948 /** 00949 * @} 00950 */ 00951 00952 /** 00953 * @} 00954 */ 00955 00956 /* Private types -------------------------------------------------------------*/ 00957 /* Private variables ---------------------------------------------------------*/ 00958 /* Private constants ---------------------------------------------------------*/ 00959 /** @defgroup RTCEx_Private_Constants RTCEx Private Constants 00960 * @{ 00961 */ 00962 #define RTC_EXTI_LINE_TAMPER_TIMESTAMP_EVENT ((uint32_t)0x00080000) /*!< External interrupt line 19 Connected to the RTC Tamper and Time Stamp events */ 00963 #define RTC_EXTI_LINE_WAKEUPTIMER_EVENT ((uint32_t)0x00100000) /*!< External interrupt line 20 Connected to the RTC Wakeup event */ 00964 00965 /** 00966 * @} 00967 */ 00968 00969 /* Private macros ------------------------------------------------------------*/ 00970 /** @defgroup RTCEx_Private_Macros RTCEx Private Macros 00971 * @{ 00972 */ 00973 00974 /** @defgroup RTCEx_IS_RTC_Definitions Private macros to check input parameters 00975 * @{ 00976 */ 00977 00978 #define IS_RTC_OUTPUT(OUTPUT) (((OUTPUT) == RTC_OUTPUT_DISABLE) || \ 00979 ((OUTPUT) == RTC_OUTPUT_ALARMA) || \ 00980 ((OUTPUT) == RTC_OUTPUT_ALARMB) || \ 00981 ((OUTPUT) == RTC_OUTPUT_WAKEUP)) 00982 00983 #define IS_RTC_BKP(BKP) ((BKP) < (uint32_t) RTC_BKP_NUMBER) 00984 00985 #define IS_TIMESTAMP_EDGE(EDGE) (((EDGE) == RTC_TIMESTAMPEDGE_RISING) || \ 00986 ((EDGE) == RTC_TIMESTAMPEDGE_FALLING)) 00987 00988 #define IS_RTC_TAMPER(TAMPER) ((((TAMPER) & (uint32_t)0xFFFFFFD6) == 0x00) && ((TAMPER) != (uint32_t)RESET)) 00989 00990 #define IS_RTC_TAMPER_INTERRUPT(INTERRUPT) ((((INTERRUPT) & (uint32_t)0xFFB6FFFB) == 0x00) && ((INTERRUPT) != (uint32_t)RESET)) 00991 00992 #define IS_RTC_TIMESTAMP_PIN(PIN) (((PIN) == RTC_TIMESTAMPPIN_DEFAULT)) 00993 00994 #define IS_RTC_TAMPER_TRIGGER(TRIGGER) (((TRIGGER) == RTC_TAMPERTRIGGER_RISINGEDGE) || \ 00995 ((TRIGGER) == RTC_TAMPERTRIGGER_FALLINGEDGE) || \ 00996 ((TRIGGER) == RTC_TAMPERTRIGGER_LOWLEVEL) || \ 00997 ((TRIGGER) == RTC_TAMPERTRIGGER_HIGHLEVEL)) 00998 00999 #define IS_RTC_TAMPER_ERASE_MODE(MODE) (((MODE) == RTC_TAMPER_ERASE_BACKUP_ENABLE) || \ 01000 ((MODE) == RTC_TAMPER_ERASE_BACKUP_DISABLE)) 01001 01002 #define IS_RTC_TAMPER_MASKFLAG_STATE(STATE) (((STATE) == RTC_TAMPERMASK_FLAG_ENABLE) || \ 01003 ((STATE) == RTC_TAMPERMASK_FLAG_DISABLE)) 01004 01005 #define IS_RTC_TAMPER_FILTER(FILTER) (((FILTER) == RTC_TAMPERFILTER_DISABLE) || \ 01006 ((FILTER) == RTC_TAMPERFILTER_2SAMPLE) || \ 01007 ((FILTER) == RTC_TAMPERFILTER_4SAMPLE) || \ 01008 ((FILTER) == RTC_TAMPERFILTER_8SAMPLE)) 01009 01010 #define IS_RTC_TAMPER_SAMPLING_FREQ(FREQ) (((FREQ) == RTC_TAMPERSAMPLINGFREQ_RTCCLK_DIV32768)|| \ 01011 ((FREQ) == RTC_TAMPERSAMPLINGFREQ_RTCCLK_DIV16384)|| \ 01012 ((FREQ) == RTC_TAMPERSAMPLINGFREQ_RTCCLK_DIV8192) || \ 01013 ((FREQ) == RTC_TAMPERSAMPLINGFREQ_RTCCLK_DIV4096) || \ 01014 ((FREQ) == RTC_TAMPERSAMPLINGFREQ_RTCCLK_DIV2048) || \ 01015 ((FREQ) == RTC_TAMPERSAMPLINGFREQ_RTCCLK_DIV1024) || \ 01016 ((FREQ) == RTC_TAMPERSAMPLINGFREQ_RTCCLK_DIV512) || \ 01017 ((FREQ) == RTC_TAMPERSAMPLINGFREQ_RTCCLK_DIV256)) 01018 01019 #define IS_RTC_TAMPER_PRECHARGE_DURATION(DURATION) (((DURATION) == RTC_TAMPERPRECHARGEDURATION_1RTCCLK) || \ 01020 ((DURATION) == RTC_TAMPERPRECHARGEDURATION_2RTCCLK) || \ 01021 ((DURATION) == RTC_TAMPERPRECHARGEDURATION_4RTCCLK) || \ 01022 ((DURATION) == RTC_TAMPERPRECHARGEDURATION_8RTCCLK)) 01023 01024 #define IS_RTC_TAMPER_TIMESTAMPONTAMPER_DETECTION(DETECTION) (((DETECTION) == RTC_TIMESTAMPONTAMPERDETECTION_ENABLE) || \ 01025 ((DETECTION) == RTC_TIMESTAMPONTAMPERDETECTION_DISABLE)) 01026 01027 #define IS_RTC_TAMPER_PULLUP_STATE(STATE) (((STATE) == RTC_TAMPER_PULLUP_ENABLE) || \ 01028 ((STATE) == RTC_TAMPER_PULLUP_DISABLE)) 01029 01030 #define IS_RTC_WAKEUP_CLOCK(CLOCK) (((CLOCK) == RTC_WAKEUPCLOCK_RTCCLK_DIV16) || \ 01031 ((CLOCK) == RTC_WAKEUPCLOCK_RTCCLK_DIV8) || \ 01032 ((CLOCK) == RTC_WAKEUPCLOCK_RTCCLK_DIV4) || \ 01033 ((CLOCK) == RTC_WAKEUPCLOCK_RTCCLK_DIV2) || \ 01034 ((CLOCK) == RTC_WAKEUPCLOCK_CK_SPRE_16BITS) || \ 01035 ((CLOCK) == RTC_WAKEUPCLOCK_CK_SPRE_17BITS)) 01036 01037 #define IS_RTC_WAKEUP_COUNTER(COUNTER) ((COUNTER) <= 0xFFFF) 01038 01039 #define IS_RTC_SMOOTH_CALIB_PERIOD(PERIOD) (((PERIOD) == RTC_SMOOTHCALIB_PERIOD_32SEC) || \ 01040 ((PERIOD) == RTC_SMOOTHCALIB_PERIOD_16SEC) || \ 01041 ((PERIOD) == RTC_SMOOTHCALIB_PERIOD_8SEC)) 01042 01043 #define IS_RTC_SMOOTH_CALIB_PLUS(PLUS) (((PLUS) == RTC_SMOOTHCALIB_PLUSPULSES_SET) || \ 01044 ((PLUS) == RTC_SMOOTHCALIB_PLUSPULSES_RESET)) 01045 01046 #define IS_RTC_SMOOTH_CALIB_MINUS(VALUE) ((VALUE) <= 0x000001FF) 01047 01048 #define IS_RTC_SHIFT_ADD1S(SEL) (((SEL) == RTC_SHIFTADD1S_RESET) || \ 01049 ((SEL) == RTC_SHIFTADD1S_SET)) 01050 01051 #define IS_RTC_SHIFT_SUBFS(FS) ((FS) <= 0x00007FFF) 01052 01053 #define IS_RTC_CALIB_OUTPUT(OUTPUT) (((OUTPUT) == RTC_CALIBOUTPUT_512HZ) || \ 01054 ((OUTPUT) == RTC_CALIBOUTPUT_1HZ)) 01055 01056 /** 01057 * @} 01058 */ 01059 01060 /** 01061 * @} 01062 */ 01063 01064 /** 01065 * @} 01066 */ 01067 01068 /** 01069 * @} 01070 */ 01071 01072 #ifdef __cplusplus 01073 } 01074 #endif 01075 01076 #endif /* __STM32L4xx_HAL_RTC_EX_H */ 01077 01078 /************************ (C) COPYRIGHT STMicroelectronics *****END OF FILE****/ 01079
Generated on Tue Jul 12 2022 11:35:15 by
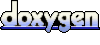