Hal Drivers for L4
Dependents: BSP OneHopeOnePrayer FINAL_AUDIO_RECORD AudioDemo
Fork of STM32L4xx_HAL_Driver by
stm32l4xx_hal_rtc_ex.c
00001 /** 00002 ****************************************************************************** 00003 * @file stm32l4xx_hal_rtc_ex.c 00004 * @author MCD Application Team 00005 * @version V1.1.0 00006 * @date 16-September-2015 00007 * @brief Extended RTC HAL module driver. 00008 * This file provides firmware functions to manage the following 00009 * functionalities of the Real Time Clock (RTC) Extended peripheral: 00010 * + RTC Time Stamp functions 00011 * + RTC Tamper functions 00012 * + RTC Wake-up functions 00013 * + Extended Control functions 00014 * + Extended RTC features functions 00015 * 00016 @verbatim 00017 ============================================================================== 00018 ##### How to use this driver ##### 00019 ============================================================================== 00020 [..] 00021 (+) Enable the RTC domain access. 00022 (+) Configure the RTC Prescaler (Asynchronous and Synchronous) and RTC hour 00023 format using the HAL_RTC_Init() function. 00024 00025 *** RTC Wakeup configuration *** 00026 ================================ 00027 [..] 00028 (+) To configure the RTC Wakeup Clock source and Counter use the HAL_RTCEx_SetWakeUpTimer() 00029 function. You can also configure the RTC Wakeup timer with interrupt mode 00030 using the HAL_RTCEx_SetWakeUpTimer_IT() function. 00031 (+) To read the RTC WakeUp Counter register, use the HAL_RTCEx_GetWakeUpTimer() 00032 function. 00033 00034 *** Outputs configuration *** 00035 ============================= 00036 [..] The RTC has 2 different outputs: 00037 (+) RTC_ALARM: this output is used to manage the RTC Alarm A, Alarm B 00038 and WaKeUp signals. 00039 To output the selected RTC signal, use the HAL_RTC_Init() function. 00040 (+) RTC_CALIB: this output is 512Hz signal or 1Hz. 00041 To enable the RTC_CALIB, use the HAL_RTCEx_SetCalibrationOutPut() function. 00042 (+) Two pins can be used as RTC_ALARM or RTC_CALIB (PC13, PB2) managed on 00043 the RTC_OR register. 00044 (+) When the RTC_CALIB or RTC_ALARM output is selected, the RTC_OUT pin is 00045 automatically configured in output alternate function. 00046 00047 *** Smooth digital Calibration configuration *** 00048 ================================================ 00049 [..] 00050 (+) Configure the RTC Original Digital Calibration Value and the corresponding 00051 calibration cycle period (32s,16s and 8s) using the HAL_RTCEx_SetSmoothCalib() 00052 function. 00053 00054 *** TimeStamp configuration *** 00055 =============================== 00056 [..] 00057 (+) Enable the RTC TimeStamp using the HAL_RTCEx_SetTimeStamp() function. 00058 You can also configure the RTC TimeStamp with interrupt mode using the 00059 HAL_RTCEx_SetTimeStamp_IT() function. 00060 (+) To read the RTC TimeStamp Time and Date register, use the HAL_RTCEx_GetTimeStamp() 00061 function. 00062 00063 *** Internal TimeStamp configuration *** 00064 =============================== 00065 [..] 00066 (+) Enable the RTC internal TimeStamp using the HAL_RTCEx_SetInternalTimeStamp() function. 00067 User has to check internal timestamp occurrence using __HAL_RTC_INTERNAL_TIMESTAMP_GET_FLAG. 00068 (+) To read the RTC TimeStamp Time and Date register, use the HAL_RTCEx_GetTimeStamp() 00069 function. 00070 00071 *** Tamper configuration *** 00072 ============================ 00073 [..] 00074 (+) Enable the RTC Tamper and configure the Tamper filter count, trigger Edge 00075 or Level according to the Tamper filter (if equal to 0 Edge else Level) 00076 value, sampling frequency, NoErase, MaskFlag, precharge or discharge and 00077 Pull-UP using the HAL_RTCEx_SetTamper() function. You can configure RTC Tamper 00078 with interrupt mode using HAL_RTCEx_SetTamper_IT() function. 00079 (+) The default configuration of the Tamper erases the backup registers. To avoid 00080 erase, enable the NoErase field on the RTC_TAMPCR register. 00081 00082 *** Backup Data Registers configuration *** 00083 =========================================== 00084 [..] 00085 (+) To write to the RTC Backup Data registers, use the HAL_RTCEx_BKUPWrite() 00086 function. 00087 (+) To read the RTC Backup Data registers, use the HAL_RTCEx_BKUPRead() 00088 function. 00089 00090 @endverbatim 00091 ****************************************************************************** 00092 * @attention 00093 * 00094 * <h2><center>© COPYRIGHT(c) 2015 STMicroelectronics</center></h2> 00095 * 00096 * Redistribution and use in source and binary forms, with or without modification, 00097 * are permitted provided that the following conditions are met: 00098 * 1. Redistributions of source code must retain the above copyright notice, 00099 * this list of conditions and the following disclaimer. 00100 * 2. Redistributions in binary form must reproduce the above copyright notice, 00101 * this list of conditions and the following disclaimer in the documentation 00102 * and/or other materials provided with the distribution. 00103 * 3. Neither the name of STMicroelectronics nor the names of its contributors 00104 * may be used to endorse or promote products derived from this software 00105 * without specific prior written permission. 00106 * 00107 * THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" 00108 * AND ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE 00109 * IMPLIED WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE 00110 * DISCLAIMED. IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE 00111 * FOR ANY DIRECT, INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL 00112 * DAMAGES (INCLUDING, BUT NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR 00113 * SERVICES; LOSS OF USE, DATA, OR PROFITS; OR BUSINESS INTERRUPTION) HOWEVER 00114 * CAUSED AND ON ANY THEORY OF LIABILITY, WHETHER IN CONTRACT, STRICT LIABILITY, 00115 * OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) ARISING IN ANY WAY OUT OF THE USE 00116 * OF THIS SOFTWARE, EVEN IF ADVISED OF THE POSSIBILITY OF SUCH DAMAGE. 00117 * 00118 ****************************************************************************** 00119 */ 00120 00121 /* Includes ------------------------------------------------------------------*/ 00122 #include "stm32l4xx_hal.h" 00123 00124 /** @addtogroup STM32L4xx_HAL_Driver 00125 * @{ 00126 */ 00127 00128 /** @defgroup RTCEx RTCEx 00129 * @brief RTC Extended HAL module driver 00130 * @{ 00131 */ 00132 00133 #ifdef HAL_RTC_MODULE_ENABLED 00134 00135 /* Private typedef -----------------------------------------------------------*/ 00136 /* Private define ------------------------------------------------------------*/ 00137 /* Private macro -------------------------------------------------------------*/ 00138 /* Private variables ---------------------------------------------------------*/ 00139 /* Private function prototypes -----------------------------------------------*/ 00140 /* Exported functions --------------------------------------------------------*/ 00141 00142 /** @defgroup RTCEx_Exported_Functions RTCEx Exported Functions 00143 * @{ 00144 */ 00145 00146 00147 /** @defgroup RTCEx_Exported_Functions_Group1 RTC TimeStamp and Tamper functions 00148 * @brief RTC TimeStamp and Tamper functions 00149 * 00150 @verbatim 00151 =============================================================================== 00152 ##### RTC TimeStamp and Tamper functions ##### 00153 =============================================================================== 00154 00155 [..] This section provide functions allowing to configure TimeStamp feature 00156 00157 @endverbatim 00158 * @{ 00159 */ 00160 00161 /** 00162 * @brief Set TimeStamp. 00163 * @note This API must be called before enabling the TimeStamp feature. 00164 * @param hrtc: RTC handle 00165 * @param TimeStampEdge: Specifies the pin edge on which the TimeStamp is 00166 * activated. 00167 * This parameter can be one of the following values: 00168 * @arg RTC_TIMESTAMPEDGE_RISING: the Time stamp event occurs on the 00169 * rising edge of the related pin. 00170 * @arg RTC_TIMESTAMPEDGE_FALLING: the Time stamp event occurs on the 00171 * falling edge of the related pin. 00172 * @param RTC_TimeStampPin: specifies the RTC TimeStamp Pin. 00173 * This parameter can be one of the following values: 00174 * @arg RTC_TIMESTAMPPIN_DEFAULT: PC13 is selected as RTC TimeStamp Pin. 00175 * The RTC TimeStamp Pin is per default PC13, but for reasons of 00176 * compatibility, this parameter is required. 00177 * @retval HAL status 00178 */ 00179 HAL_StatusTypeDef HAL_RTCEx_SetTimeStamp(RTC_HandleTypeDef *hrtc, uint32_t TimeStampEdge, uint32_t RTC_TimeStampPin) 00180 { 00181 uint32_t tmpreg = 0; 00182 00183 /* Check the parameters */ 00184 assert_param(IS_TIMESTAMP_EDGE(TimeStampEdge)); 00185 assert_param(IS_RTC_TIMESTAMP_PIN(RTC_TimeStampPin)); 00186 00187 /* Process Locked */ 00188 __HAL_LOCK(hrtc); 00189 00190 hrtc->State = HAL_RTC_STATE_BUSY; 00191 00192 /* Get the RTC_CR register and clear the bits to be configured */ 00193 tmpreg = (uint32_t)(hrtc->Instance->CR & (uint32_t)~(RTC_CR_TSEDGE | RTC_CR_TSE)); 00194 00195 tmpreg|= TimeStampEdge; 00196 00197 /* Disable the write protection for RTC registers */ 00198 __HAL_RTC_WRITEPROTECTION_DISABLE(hrtc); 00199 00200 /* Configure the Time Stamp TSEDGE and Enable bits */ 00201 hrtc->Instance->CR = (uint32_t)tmpreg; 00202 00203 __HAL_RTC_TIMESTAMP_ENABLE(hrtc); 00204 00205 /* Enable the write protection for RTC registers */ 00206 __HAL_RTC_WRITEPROTECTION_ENABLE(hrtc); 00207 00208 /* Change RTC state */ 00209 hrtc->State = HAL_RTC_STATE_READY; 00210 00211 /* Process Unlocked */ 00212 __HAL_UNLOCK(hrtc); 00213 00214 return HAL_OK; 00215 } 00216 00217 /** 00218 * @brief Set TimeStamp with Interrupt. 00219 * @param hrtc: RTC handle 00220 * @note This API must be called before enabling the TimeStamp feature. 00221 * @param TimeStampEdge: Specifies the pin edge on which the TimeStamp is 00222 * activated. 00223 * This parameter can be one of the following values: 00224 * @arg RTC_TIMESTAMPEDGE_RISING: the Time stamp event occurs on the 00225 * rising edge of the related pin. 00226 * @arg RTC_TIMESTAMPEDGE_FALLING: the Time stamp event occurs on the 00227 * falling edge of the related pin. 00228 * @param RTC_TimeStampPin: Specifies the RTC TimeStamp Pin. 00229 * This parameter can be one of the following values: 00230 * @arg RTC_TIMESTAMPPIN_DEFAULT: PC13 is selected as RTC TimeStamp Pin. 00231 * The RTC TimeStamp Pin is per default PC13, but for reasons of 00232 * compatibility, this parameter is required. 00233 * @retval HAL status 00234 */ 00235 HAL_StatusTypeDef HAL_RTCEx_SetTimeStamp_IT(RTC_HandleTypeDef *hrtc, uint32_t TimeStampEdge, uint32_t RTC_TimeStampPin) 00236 { 00237 uint32_t tmpreg = 0; 00238 00239 /* Check the parameters */ 00240 assert_param(IS_TIMESTAMP_EDGE(TimeStampEdge)); 00241 assert_param(IS_RTC_TIMESTAMP_PIN(RTC_TimeStampPin)); 00242 00243 /* Process Locked */ 00244 __HAL_LOCK(hrtc); 00245 00246 hrtc->State = HAL_RTC_STATE_BUSY; 00247 00248 /* Get the RTC_CR register and clear the bits to be configured */ 00249 tmpreg = (uint32_t)(hrtc->Instance->CR & (uint32_t)~(RTC_CR_TSEDGE | RTC_CR_TSE)); 00250 00251 tmpreg |= TimeStampEdge; 00252 00253 /* Disable the write protection for RTC registers */ 00254 __HAL_RTC_WRITEPROTECTION_DISABLE(hrtc); 00255 00256 /* Configure the Time Stamp TSEDGE and Enable bits */ 00257 hrtc->Instance->CR = (uint32_t)tmpreg; 00258 00259 __HAL_RTC_TIMESTAMP_ENABLE(hrtc); 00260 00261 /* Enable IT timestamp */ 00262 __HAL_RTC_TIMESTAMP_ENABLE_IT(hrtc,RTC_IT_TS); 00263 00264 /* RTC timestamp Interrupt Configuration: EXTI configuration */ 00265 __HAL_RTC_TAMPER_TIMESTAMP_EXTI_ENABLE_IT(); 00266 00267 __HAL_RTC_TAMPER_TIMESTAMP_EXTI_ENABLE_RISING_EDGE(); 00268 00269 /* Enable the write protection for RTC registers */ 00270 __HAL_RTC_WRITEPROTECTION_ENABLE(hrtc); 00271 00272 hrtc->State = HAL_RTC_STATE_READY; 00273 00274 /* Process Unlocked */ 00275 __HAL_UNLOCK(hrtc); 00276 00277 return HAL_OK; 00278 } 00279 00280 /** 00281 * @brief Deactivate TimeStamp. 00282 * @param hrtc: RTC handle 00283 * @retval HAL status 00284 */ 00285 HAL_StatusTypeDef HAL_RTCEx_DeactivateTimeStamp(RTC_HandleTypeDef *hrtc) 00286 { 00287 uint32_t tmpreg = 0; 00288 00289 /* Process Locked */ 00290 __HAL_LOCK(hrtc); 00291 00292 hrtc->State = HAL_RTC_STATE_BUSY; 00293 00294 /* Disable the write protection for RTC registers */ 00295 __HAL_RTC_WRITEPROTECTION_DISABLE(hrtc); 00296 00297 /* In case of interrupt mode is used, the interrupt source must disabled */ 00298 __HAL_RTC_TIMESTAMP_DISABLE_IT(hrtc, RTC_IT_TS); 00299 00300 /* Get the RTC_CR register and clear the bits to be configured */ 00301 tmpreg = (uint32_t)(hrtc->Instance->CR & (uint32_t)~(RTC_CR_TSEDGE | RTC_CR_TSE)); 00302 00303 /* Configure the Time Stamp TSEDGE and Enable bits */ 00304 hrtc->Instance->CR = (uint32_t)tmpreg; 00305 00306 /* Enable the write protection for RTC registers */ 00307 __HAL_RTC_WRITEPROTECTION_ENABLE(hrtc); 00308 00309 hrtc->State = HAL_RTC_STATE_READY; 00310 00311 /* Process Unlocked */ 00312 __HAL_UNLOCK(hrtc); 00313 00314 return HAL_OK; 00315 } 00316 00317 /** 00318 * @brief Set Internal TimeStamp. 00319 * @note This API must be called before enabling the internal TimeStamp feature. 00320 * @param hrtc: pointer to a RTC_HandleTypeDef structure that contains 00321 * the configuration information for RTC. 00322 * @retval HAL status 00323 */ 00324 HAL_StatusTypeDef HAL_RTCEx_SetInternalTimeStamp(RTC_HandleTypeDef *hrtc) 00325 { 00326 /* Process Locked */ 00327 __HAL_LOCK(hrtc); 00328 00329 hrtc->State = HAL_RTC_STATE_BUSY; 00330 00331 /* Disable the write protection for RTC registers */ 00332 __HAL_RTC_WRITEPROTECTION_DISABLE(hrtc); 00333 00334 /* Configure the internal Time Stamp Enable bits */ 00335 __HAL_RTC_INTERNAL_TIMESTAMP_ENABLE(hrtc); 00336 00337 /* Enable the write protection for RTC registers */ 00338 __HAL_RTC_WRITEPROTECTION_ENABLE(hrtc); 00339 00340 /* Change RTC state */ 00341 hrtc->State = HAL_RTC_STATE_READY; 00342 00343 /* Process Unlocked */ 00344 __HAL_UNLOCK(hrtc); 00345 00346 return HAL_OK; 00347 } 00348 00349 /** 00350 * @brief Deactivate Internal TimeStamp. 00351 * @param hrtc: pointer to a RTC_HandleTypeDef structure that contains 00352 * the configuration information for RTC. 00353 * @retval HAL status 00354 */ 00355 HAL_StatusTypeDef HAL_RTCEx_DeactivateInternalTimeStamp(RTC_HandleTypeDef *hrtc) 00356 { 00357 /* Process Locked */ 00358 __HAL_LOCK(hrtc); 00359 00360 hrtc->State = HAL_RTC_STATE_BUSY; 00361 00362 /* Disable the write protection for RTC registers */ 00363 __HAL_RTC_WRITEPROTECTION_DISABLE(hrtc); 00364 00365 /* Configure the internal Time Stamp Enable bits */ 00366 __HAL_RTC_INTERNAL_TIMESTAMP_DISABLE(hrtc); 00367 00368 /* Enable the write protection for RTC registers */ 00369 __HAL_RTC_WRITEPROTECTION_ENABLE(hrtc); 00370 00371 hrtc->State = HAL_RTC_STATE_READY; 00372 00373 /* Process Unlocked */ 00374 __HAL_UNLOCK(hrtc); 00375 00376 return HAL_OK; 00377 } 00378 00379 /** 00380 * @brief Get the RTC TimeStamp value. 00381 * @param hrtc: RTC handle 00382 * @param sTimeStamp: Pointer to Time structure 00383 * @param sTimeStampDate: Pointer to Date structure 00384 * @param Format: specifies the format of the entered parameters. 00385 * This parameter can be one of the following values: 00386 * @arg RTC_FORMAT_BIN: Binary data format 00387 * @arg RTC_FORMAT_BCD: BCD data format 00388 * @retval HAL status 00389 */ 00390 HAL_StatusTypeDef HAL_RTCEx_GetTimeStamp(RTC_HandleTypeDef *hrtc, RTC_TimeTypeDef* sTimeStamp, RTC_DateTypeDef* sTimeStampDate, uint32_t Format) 00391 { 00392 uint32_t tmptime = 0, tmpdate = 0; 00393 00394 /* Check the parameters */ 00395 assert_param(IS_RTC_FORMAT(Format)); 00396 00397 /* Get the TimeStamp time and date registers values */ 00398 tmptime = (uint32_t)(hrtc->Instance->TSTR & RTC_TR_RESERVED_MASK); 00399 tmpdate = (uint32_t)(hrtc->Instance->TSDR & RTC_DR_RESERVED_MASK); 00400 00401 /* Fill the Time structure fields with the read parameters */ 00402 sTimeStamp->Hours = (uint8_t)((tmptime & (RTC_TR_HT | RTC_TR_HU)) >> 16); 00403 sTimeStamp->Minutes = (uint8_t)((tmptime & (RTC_TR_MNT | RTC_TR_MNU)) >> 8); 00404 sTimeStamp->Seconds = (uint8_t)(tmptime & (RTC_TR_ST | RTC_TR_SU)); 00405 sTimeStamp->TimeFormat = (uint8_t)((tmptime & (RTC_TR_PM)) >> 16); 00406 sTimeStamp->SubSeconds = (uint32_t) hrtc->Instance->TSSSR; 00407 00408 /* Fill the Date structure fields with the read parameters */ 00409 sTimeStampDate->Year = 0; 00410 sTimeStampDate->Month = (uint8_t)((tmpdate & (RTC_DR_MT | RTC_DR_MU)) >> 8); 00411 sTimeStampDate->Date = (uint8_t)(tmpdate & (RTC_DR_DT | RTC_DR_DU)); 00412 sTimeStampDate->WeekDay = (uint8_t)((tmpdate & (RTC_DR_WDU)) >> 13); 00413 00414 /* Check the input parameters format */ 00415 if(Format == RTC_FORMAT_BIN) 00416 { 00417 /* Convert the TimeStamp structure parameters to Binary format */ 00418 sTimeStamp->Hours = (uint8_t)RTC_Bcd2ToByte(sTimeStamp->Hours); 00419 sTimeStamp->Minutes = (uint8_t)RTC_Bcd2ToByte(sTimeStamp->Minutes); 00420 sTimeStamp->Seconds = (uint8_t)RTC_Bcd2ToByte(sTimeStamp->Seconds); 00421 00422 /* Convert the DateTimeStamp structure parameters to Binary format */ 00423 sTimeStampDate->Month = (uint8_t)RTC_Bcd2ToByte(sTimeStampDate->Month); 00424 sTimeStampDate->Date = (uint8_t)RTC_Bcd2ToByte(sTimeStampDate->Date); 00425 sTimeStampDate->WeekDay = (uint8_t)RTC_Bcd2ToByte(sTimeStampDate->WeekDay); 00426 } 00427 00428 /* Clear the TIMESTAMP Flags */ 00429 __HAL_RTC_INTERNAL_TIMESTAMP_CLEAR_FLAG(hrtc, RTC_FLAG_ITSF); 00430 __HAL_RTC_TIMESTAMP_CLEAR_FLAG(hrtc, RTC_FLAG_TSF); 00431 00432 return HAL_OK; 00433 } 00434 00435 /** 00436 * @brief Set Tamper. 00437 * @note By calling this API we disable the tamper interrupt for all tampers. 00438 * @param hrtc: RTC handle 00439 * @param sTamper: Pointer to Tamper Structure. 00440 * @retval HAL status 00441 */ 00442 HAL_StatusTypeDef HAL_RTCEx_SetTamper(RTC_HandleTypeDef *hrtc, RTC_TamperTypeDef* sTamper) 00443 { 00444 uint32_t tmpreg = 0; 00445 00446 /* Check the parameters */ 00447 assert_param(IS_RTC_TAMPER(sTamper->Tamper)); 00448 assert_param(IS_RTC_TAMPER_TRIGGER(sTamper->Trigger)); 00449 assert_param(IS_RTC_TAMPER_ERASE_MODE(sTamper->NoErase)); 00450 assert_param(IS_RTC_TAMPER_MASKFLAG_STATE(sTamper->MaskFlag)); 00451 assert_param(IS_RTC_TAMPER_FILTER(sTamper->Filter)); 00452 assert_param(IS_RTC_TAMPER_SAMPLING_FREQ(sTamper->SamplingFrequency)); 00453 assert_param(IS_RTC_TAMPER_PRECHARGE_DURATION(sTamper->PrechargeDuration)); 00454 assert_param(IS_RTC_TAMPER_PULLUP_STATE(sTamper->TamperPullUp)); 00455 assert_param(IS_RTC_TAMPER_TIMESTAMPONTAMPER_DETECTION(sTamper->TimeStampOnTamperDetection)); 00456 00457 /* Process Locked */ 00458 __HAL_LOCK(hrtc); 00459 00460 hrtc->State = HAL_RTC_STATE_BUSY; 00461 00462 /* Configure the tamper trigger */ 00463 if(sTamper->Trigger != RTC_TAMPERTRIGGER_RISINGEDGE) 00464 { 00465 sTamper->Trigger = (uint32_t)(sTamper->Tamper << 1); 00466 } 00467 00468 if(sTamper->NoErase != RTC_TAMPER_ERASE_BACKUP_ENABLE) 00469 { 00470 sTamper->NoErase = 0; 00471 if((sTamper->Tamper & RTC_TAMPER_1) != 0) 00472 { 00473 sTamper->NoErase |= RTC_TAMPCR_TAMP1NOERASE; 00474 } 00475 if((sTamper->Tamper & RTC_TAMPER_2) != 0) 00476 { 00477 sTamper->NoErase |= RTC_TAMPCR_TAMP2NOERASE; 00478 } 00479 if((sTamper->Tamper & RTC_TAMPER_3) != 0) 00480 { 00481 sTamper->NoErase |= RTC_TAMPCR_TAMP3NOERASE; 00482 } 00483 } 00484 00485 if(sTamper->MaskFlag != RTC_TAMPERMASK_FLAG_DISABLE) 00486 { 00487 sTamper->MaskFlag = 0; 00488 if((sTamper->Tamper & RTC_TAMPER_1) != 0) 00489 { 00490 sTamper->MaskFlag |= RTC_TAMPCR_TAMP1MF; 00491 } 00492 if((sTamper->Tamper & RTC_TAMPER_2) != 0) 00493 { 00494 sTamper->MaskFlag |= RTC_TAMPCR_TAMP2MF; 00495 } 00496 if((sTamper->Tamper & RTC_TAMPER_3) != 0) 00497 { 00498 sTamper->MaskFlag |= RTC_TAMPCR_TAMP3MF; 00499 } 00500 } 00501 00502 tmpreg = ((uint32_t)sTamper->Tamper | (uint32_t)sTamper->Trigger | (uint32_t)sTamper->NoErase |\ 00503 (uint32_t)sTamper->MaskFlag | (uint32_t)sTamper->Filter | (uint32_t)sTamper->SamplingFrequency |\ 00504 (uint32_t)sTamper->PrechargeDuration | (uint32_t)sTamper->TamperPullUp | sTamper->TimeStampOnTamperDetection); 00505 00506 hrtc->Instance->TAMPCR &= (uint32_t)~((uint32_t)sTamper->Tamper | (uint32_t)(sTamper->Tamper << 1) | (uint32_t)RTC_TAMPCR_TAMPTS |\ 00507 (uint32_t)RTC_TAMPCR_TAMPFREQ | (uint32_t)RTC_TAMPCR_TAMPFLT | (uint32_t)RTC_TAMPCR_TAMPPRCH |\ 00508 (uint32_t)RTC_TAMPCR_TAMPPUDIS | (uint32_t)RTC_TAMPCR_TAMPIE | (uint32_t)RTC_TAMPCR_TAMP1IE |\ 00509 (uint32_t)RTC_TAMPCR_TAMP2IE | (uint32_t)RTC_TAMPCR_TAMP3IE | (uint32_t)RTC_TAMPCR_TAMP1NOERASE |\ 00510 (uint32_t)RTC_TAMPCR_TAMP2NOERASE | (uint32_t)RTC_TAMPCR_TAMP3NOERASE | (uint32_t)RTC_TAMPCR_TAMP1MF |\ 00511 (uint32_t)RTC_TAMPCR_TAMP2MF | (uint32_t)RTC_TAMPCR_TAMP3MF); 00512 00513 hrtc->Instance->TAMPCR |= tmpreg; 00514 00515 hrtc->State = HAL_RTC_STATE_READY; 00516 00517 /* Process Unlocked */ 00518 __HAL_UNLOCK(hrtc); 00519 00520 return HAL_OK; 00521 } 00522 00523 /** 00524 * @brief Set Tamper with interrupt. 00525 * @note By calling this API we force the tamper interrupt for all tampers. 00526 * @param hrtc: RTC handle 00527 * @param sTamper: Pointer to RTC Tamper. 00528 * @retval HAL status 00529 */ 00530 HAL_StatusTypeDef HAL_RTCEx_SetTamper_IT(RTC_HandleTypeDef *hrtc, RTC_TamperTypeDef* sTamper) 00531 { 00532 uint32_t tmpreg = 0; 00533 00534 /* Check the parameters */ 00535 assert_param(IS_RTC_TAMPER(sTamper->Tamper)); 00536 assert_param(IS_RTC_TAMPER_INTERRUPT(sTamper->Interrupt)); 00537 assert_param(IS_RTC_TAMPER_TRIGGER(sTamper->Trigger)); 00538 assert_param(IS_RTC_TAMPER_ERASE_MODE(sTamper->NoErase)); 00539 assert_param(IS_RTC_TAMPER_MASKFLAG_STATE(sTamper->MaskFlag)); 00540 assert_param(IS_RTC_TAMPER_FILTER(sTamper->Filter)); 00541 assert_param(IS_RTC_TAMPER_SAMPLING_FREQ(sTamper->SamplingFrequency)); 00542 assert_param(IS_RTC_TAMPER_PRECHARGE_DURATION(sTamper->PrechargeDuration)); 00543 assert_param(IS_RTC_TAMPER_PULLUP_STATE(sTamper->TamperPullUp)); 00544 assert_param(IS_RTC_TAMPER_TIMESTAMPONTAMPER_DETECTION(sTamper->TimeStampOnTamperDetection)); 00545 00546 /* Process Locked */ 00547 __HAL_LOCK(hrtc); 00548 00549 hrtc->State = HAL_RTC_STATE_BUSY; 00550 00551 /* Configure the tamper trigger */ 00552 if(sTamper->Trigger != RTC_TAMPERTRIGGER_RISINGEDGE) 00553 { 00554 sTamper->Trigger = (uint32_t)(sTamper->Tamper << 1); 00555 } 00556 00557 if(sTamper->NoErase != RTC_TAMPER_ERASE_BACKUP_ENABLE) 00558 { 00559 sTamper->NoErase = 0; 00560 if((sTamper->Tamper & RTC_TAMPER_1) != 0) 00561 { 00562 sTamper->NoErase |= RTC_TAMPCR_TAMP1NOERASE; 00563 } 00564 if((sTamper->Tamper & RTC_TAMPER_2) != 0) 00565 { 00566 sTamper->NoErase |= RTC_TAMPCR_TAMP2NOERASE; 00567 } 00568 if((sTamper->Tamper & RTC_TAMPER_3) != 0) 00569 { 00570 sTamper->NoErase |= RTC_TAMPCR_TAMP3NOERASE; 00571 } 00572 } 00573 00574 if(sTamper->MaskFlag != RTC_TAMPERMASK_FLAG_DISABLE) 00575 { 00576 sTamper->MaskFlag = 0; 00577 if((sTamper->Tamper & RTC_TAMPER_1) != 0) 00578 { 00579 sTamper->MaskFlag |= RTC_TAMPCR_TAMP1MF; 00580 } 00581 if((sTamper->Tamper & RTC_TAMPER_2) != 0) 00582 { 00583 sTamper->MaskFlag |= RTC_TAMPCR_TAMP2MF; 00584 } 00585 if((sTamper->Tamper & RTC_TAMPER_3) != 0) 00586 { 00587 sTamper->MaskFlag |= RTC_TAMPCR_TAMP3MF; 00588 } 00589 } 00590 00591 tmpreg = ((uint32_t)sTamper->Tamper | (uint32_t)sTamper->Interrupt | (uint32_t)sTamper->Trigger | (uint32_t)sTamper->NoErase |\ 00592 (uint32_t)sTamper->MaskFlag | (uint32_t)sTamper->Filter | (uint32_t)sTamper->SamplingFrequency |\ 00593 (uint32_t)sTamper->PrechargeDuration | (uint32_t)sTamper->TamperPullUp | sTamper->TimeStampOnTamperDetection); 00594 00595 hrtc->Instance->TAMPCR &= (uint32_t)~((uint32_t)sTamper->Tamper | (uint32_t)(sTamper->Tamper << 1) | (uint32_t)RTC_TAMPCR_TAMPTS |\ 00596 (uint32_t)RTC_TAMPCR_TAMPFREQ | (uint32_t)RTC_TAMPCR_TAMPFLT | (uint32_t)RTC_TAMPCR_TAMPPRCH |\ 00597 (uint32_t)RTC_TAMPCR_TAMPPUDIS | (uint32_t)RTC_TAMPCR_TAMPIE | (uint32_t)RTC_TAMPCR_TAMP1IE |\ 00598 (uint32_t)RTC_TAMPCR_TAMP2IE | (uint32_t)RTC_TAMPCR_TAMP3IE | (uint32_t)RTC_TAMPCR_TAMP1NOERASE |\ 00599 (uint32_t)RTC_TAMPCR_TAMP2NOERASE | (uint32_t)RTC_TAMPCR_TAMP3NOERASE | (uint32_t)RTC_TAMPCR_TAMP1MF |\ 00600 (uint32_t)RTC_TAMPCR_TAMP2MF | (uint32_t)RTC_TAMPCR_TAMP3MF); 00601 00602 hrtc->Instance->TAMPCR |= tmpreg; 00603 00604 /* RTC Tamper Interrupt Configuration: EXTI configuration */ 00605 __HAL_RTC_TAMPER_TIMESTAMP_EXTI_ENABLE_IT(); 00606 00607 __HAL_RTC_TAMPER_TIMESTAMP_EXTI_ENABLE_RISING_EDGE(); 00608 00609 hrtc->State = HAL_RTC_STATE_READY; 00610 00611 /* Process Unlocked */ 00612 __HAL_UNLOCK(hrtc); 00613 00614 return HAL_OK; 00615 } 00616 00617 /** 00618 * @brief Deactivate Tamper. 00619 * @param hrtc: RTC handle 00620 * @param Tamper: Selected tamper pin. 00621 * This parameter can be any combination of RTC_TAMPER_1, RTC_TAMPER_2 and RTC_TAMPER_3. 00622 * @retval HAL status 00623 */ 00624 HAL_StatusTypeDef HAL_RTCEx_DeactivateTamper(RTC_HandleTypeDef *hrtc, uint32_t Tamper) 00625 { 00626 assert_param(IS_RTC_TAMPER(Tamper)); 00627 00628 /* Process Locked */ 00629 __HAL_LOCK(hrtc); 00630 00631 hrtc->State = HAL_RTC_STATE_BUSY; 00632 00633 /* Disable the selected Tamper pin */ 00634 hrtc->Instance->TAMPCR &= ((uint32_t)~Tamper); 00635 00636 if ((Tamper & RTC_TAMPER_1) != 0) 00637 { 00638 /* Disable the Tamper1 interrupt */ 00639 hrtc->Instance->TAMPCR &= ((uint32_t)~(RTC_IT_TAMP | RTC_IT_TAMP1)); 00640 } 00641 if ((Tamper & RTC_TAMPER_2) != 0) 00642 { 00643 /* Disable the Tamper2 interrupt */ 00644 hrtc->Instance->TAMPCR &= ((uint32_t)~(RTC_IT_TAMP | RTC_IT_TAMP2)); 00645 } 00646 if ((Tamper & RTC_TAMPER_3) != 0) 00647 { 00648 /* Disable the Tamper3 interrupt */ 00649 hrtc->Instance->TAMPCR &= ((uint32_t)~(RTC_IT_TAMP | RTC_IT_TAMP3)); 00650 } 00651 00652 hrtc->State = HAL_RTC_STATE_READY; 00653 00654 /* Process Unlocked */ 00655 __HAL_UNLOCK(hrtc); 00656 00657 return HAL_OK; 00658 } 00659 00660 /** 00661 * @brief Handle TimeStamp interrupt request. 00662 * @param hrtc: RTC handle 00663 * @retval None 00664 */ 00665 void HAL_RTCEx_TamperTimeStampIRQHandler(RTC_HandleTypeDef *hrtc) 00666 { 00667 /* Get the TimeStamp interrupt source enable status */ 00668 if(__HAL_RTC_TIMESTAMP_GET_IT_SOURCE(hrtc, RTC_IT_TS) != RESET) 00669 { 00670 /* Get the pending status of the TIMESTAMP Interrupt */ 00671 if(__HAL_RTC_TIMESTAMP_GET_FLAG(hrtc, RTC_FLAG_TSF) != RESET) 00672 { 00673 /* TIMESTAMP callback */ 00674 HAL_RTCEx_TimeStampEventCallback(hrtc); 00675 00676 /* Clear the TIMESTAMP interrupt pending bit */ 00677 __HAL_RTC_TIMESTAMP_CLEAR_FLAG(hrtc, RTC_FLAG_TSF); 00678 } 00679 } 00680 00681 /* Get the Tamper1 interrupts source enable status */ 00682 if(__HAL_RTC_TAMPER_GET_IT_SOURCE(hrtc, RTC_IT_TAMP | RTC_IT_TAMP1) != RESET) 00683 { 00684 /* Get the pending status of the Tamper1 Interrupt */ 00685 if(__HAL_RTC_TAMPER_GET_FLAG(hrtc, RTC_FLAG_TAMP1F) != RESET) 00686 { 00687 /* Tamper1 callback */ 00688 HAL_RTCEx_Tamper1EventCallback(hrtc); 00689 00690 /* Clear the Tamper1 interrupt pending bit */ 00691 __HAL_RTC_TAMPER_CLEAR_FLAG(hrtc, RTC_FLAG_TAMP1F); 00692 } 00693 } 00694 00695 /* Get the Tamper2 interrupts source enable status */ 00696 if(__HAL_RTC_TAMPER_GET_IT_SOURCE(hrtc, RTC_IT_TAMP | RTC_IT_TAMP2) != RESET) 00697 { 00698 /* Get the pending status of the Tamper2 Interrupt */ 00699 if(__HAL_RTC_TAMPER_GET_FLAG(hrtc, RTC_FLAG_TAMP2F) != RESET) 00700 { 00701 /* Tamper2 callback */ 00702 HAL_RTCEx_Tamper2EventCallback(hrtc); 00703 00704 /* Clear the Tamper2 interrupt pending bit */ 00705 __HAL_RTC_TAMPER_CLEAR_FLAG(hrtc, RTC_FLAG_TAMP2F); 00706 } 00707 } 00708 00709 /* Get the Tamper3 interrupts source enable status */ 00710 if(__HAL_RTC_TAMPER_GET_IT_SOURCE(hrtc, RTC_IT_TAMP | RTC_IT_TAMP3) != RESET) 00711 { 00712 /* Get the pending status of the Tamper3 Interrupt */ 00713 if(__HAL_RTC_TAMPER_GET_FLAG(hrtc, RTC_FLAG_TAMP3F) != RESET) 00714 { 00715 /* Tamper3 callback */ 00716 HAL_RTCEx_Tamper3EventCallback(hrtc); 00717 00718 /* Clear the Tamper3 interrupt pending bit */ 00719 __HAL_RTC_TAMPER_CLEAR_FLAG(hrtc, RTC_FLAG_TAMP3F); 00720 } 00721 } 00722 00723 /* Clear the EXTI's Flag for RTC TimeStamp and Tamper */ 00724 __HAL_RTC_TAMPER_TIMESTAMP_EXTI_CLEAR_FLAG(); 00725 00726 /* Change RTC state */ 00727 hrtc->State = HAL_RTC_STATE_READY; 00728 } 00729 00730 /** 00731 * @brief TimeStamp callback. 00732 * @param hrtc: RTC handle 00733 * @retval None 00734 */ 00735 __weak void HAL_RTCEx_TimeStampEventCallback(RTC_HandleTypeDef *hrtc) 00736 { 00737 /* NOTE : This function should not be modified, when the callback is needed, 00738 the HAL_RTCEx_TimeStampEventCallback could be implemented in the user file 00739 */ 00740 } 00741 00742 /** 00743 * @brief Tamper 1 callback. 00744 * @param hrtc: RTC handle 00745 * @retval None 00746 */ 00747 __weak void HAL_RTCEx_Tamper1EventCallback(RTC_HandleTypeDef *hrtc) 00748 { 00749 /* NOTE : This function should not be modified, when the callback is needed, 00750 the HAL_RTCEx_Tamper1EventCallback could be implemented in the user file 00751 */ 00752 } 00753 00754 /** 00755 * @brief Tamper 2 callback. 00756 * @param hrtc: RTC handle 00757 * @retval None 00758 */ 00759 __weak void HAL_RTCEx_Tamper2EventCallback(RTC_HandleTypeDef *hrtc) 00760 { 00761 /* NOTE : This function should not be modified, when the callback is needed, 00762 the HAL_RTCEx_Tamper2EventCallback could be implemented in the user file 00763 */ 00764 } 00765 00766 /** 00767 * @brief Tamper 3 callback. 00768 * @param hrtc: RTC handle 00769 * @retval None 00770 */ 00771 __weak void HAL_RTCEx_Tamper3EventCallback(RTC_HandleTypeDef *hrtc) 00772 { 00773 /* NOTE : This function should not be modified, when the callback is needed, 00774 the HAL_RTCEx_Tamper3EventCallback could be implemented in the user file 00775 */ 00776 } 00777 00778 /** 00779 * @brief Handle TimeStamp polling request. 00780 * @param hrtc: RTC handle 00781 * @param Timeout: Timeout duration 00782 * @retval HAL status 00783 */ 00784 HAL_StatusTypeDef HAL_RTCEx_PollForTimeStampEvent(RTC_HandleTypeDef *hrtc, uint32_t Timeout) 00785 { 00786 uint32_t tickstart = HAL_GetTick(); 00787 00788 while(__HAL_RTC_TIMESTAMP_GET_FLAG(hrtc, RTC_FLAG_TSF) == RESET) 00789 { 00790 if(__HAL_RTC_TIMESTAMP_GET_FLAG(hrtc, RTC_FLAG_TSOVF) != RESET) 00791 { 00792 /* Clear the TIMESTAMP OverRun Flag */ 00793 __HAL_RTC_TIMESTAMP_CLEAR_FLAG(hrtc, RTC_FLAG_TSOVF); 00794 00795 /* Change TIMESTAMP state */ 00796 hrtc->State = HAL_RTC_STATE_ERROR; 00797 00798 return HAL_ERROR; 00799 } 00800 00801 if(Timeout != HAL_MAX_DELAY) 00802 { 00803 if((Timeout == 0)||((HAL_GetTick() - tickstart ) > Timeout)) 00804 { 00805 hrtc->State = HAL_RTC_STATE_TIMEOUT; 00806 return HAL_TIMEOUT; 00807 } 00808 } 00809 } 00810 00811 /* Change RTC state */ 00812 hrtc->State = HAL_RTC_STATE_READY; 00813 00814 return HAL_OK; 00815 } 00816 00817 /** 00818 * @brief Handle Tamper 1 Polling. 00819 * @param hrtc: RTC handle 00820 * @param Timeout: Timeout duration 00821 * @retval HAL status 00822 */ 00823 HAL_StatusTypeDef HAL_RTCEx_PollForTamper1Event(RTC_HandleTypeDef *hrtc, uint32_t Timeout) 00824 { 00825 uint32_t tickstart = HAL_GetTick(); 00826 00827 /* Get the status of the Interrupt */ 00828 while(__HAL_RTC_TAMPER_GET_FLAG(hrtc, RTC_FLAG_TAMP1F)== RESET) 00829 { 00830 if(Timeout != HAL_MAX_DELAY) 00831 { 00832 if((Timeout == 0)||((HAL_GetTick() - tickstart ) > Timeout)) 00833 { 00834 hrtc->State = HAL_RTC_STATE_TIMEOUT; 00835 return HAL_TIMEOUT; 00836 } 00837 } 00838 } 00839 00840 /* Clear the Tamper Flag */ 00841 __HAL_RTC_TAMPER_CLEAR_FLAG(hrtc, RTC_FLAG_TAMP1F); 00842 00843 /* Change RTC state */ 00844 hrtc->State = HAL_RTC_STATE_READY; 00845 00846 return HAL_OK; 00847 } 00848 00849 /** 00850 * @brief Handle Tamper 2 Polling. 00851 * @param hrtc: RTC handle 00852 * @param Timeout: Timeout duration 00853 * @retval HAL status 00854 */ 00855 HAL_StatusTypeDef HAL_RTCEx_PollForTamper2Event(RTC_HandleTypeDef *hrtc, uint32_t Timeout) 00856 { 00857 uint32_t tickstart = HAL_GetTick(); 00858 00859 /* Get the status of the Interrupt */ 00860 while(__HAL_RTC_TAMPER_GET_FLAG(hrtc, RTC_FLAG_TAMP2F) == RESET) 00861 { 00862 if(Timeout != HAL_MAX_DELAY) 00863 { 00864 if((Timeout == 0)||((HAL_GetTick() - tickstart ) > Timeout)) 00865 { 00866 hrtc->State = HAL_RTC_STATE_TIMEOUT; 00867 return HAL_TIMEOUT; 00868 } 00869 } 00870 } 00871 00872 /* Clear the Tamper Flag */ 00873 __HAL_RTC_TAMPER_CLEAR_FLAG(hrtc, RTC_FLAG_TAMP2F); 00874 00875 /* Change RTC state */ 00876 hrtc->State = HAL_RTC_STATE_READY; 00877 00878 return HAL_OK; 00879 } 00880 00881 /** 00882 * @brief Handle Tamper 3 Polling. 00883 * @param hrtc: RTC handle 00884 * @param Timeout: Timeout duration 00885 * @retval HAL status 00886 */ 00887 HAL_StatusTypeDef HAL_RTCEx_PollForTamper3Event(RTC_HandleTypeDef *hrtc, uint32_t Timeout) 00888 { 00889 uint32_t tickstart = HAL_GetTick(); 00890 00891 /* Get the status of the Interrupt */ 00892 while(__HAL_RTC_TAMPER_GET_FLAG(hrtc, RTC_FLAG_TAMP3F) == RESET) 00893 { 00894 if(Timeout != HAL_MAX_DELAY) 00895 { 00896 if((Timeout == 0)||((HAL_GetTick() - tickstart ) > Timeout)) 00897 { 00898 hrtc->State = HAL_RTC_STATE_TIMEOUT; 00899 return HAL_TIMEOUT; 00900 } 00901 } 00902 } 00903 00904 /* Clear the Tamper Flag */ 00905 __HAL_RTC_TAMPER_CLEAR_FLAG(hrtc, RTC_FLAG_TAMP3F); 00906 00907 /* Change RTC state */ 00908 hrtc->State = HAL_RTC_STATE_READY; 00909 00910 return HAL_OK; 00911 } 00912 00913 /** 00914 * @} 00915 */ 00916 00917 /** @defgroup RTCEx_Exported_Functions_Group2 RTC Wake-up functions 00918 * @brief RTC Wake-up functions 00919 * 00920 @verbatim 00921 =============================================================================== 00922 ##### RTC Wake-up functions ##### 00923 =============================================================================== 00924 00925 [..] This section provide functions allowing to configure Wake-up feature 00926 00927 @endverbatim 00928 * @{ 00929 */ 00930 00931 /** 00932 * @brief Set wake up timer. 00933 * @param hrtc: RTC handle 00934 * @param WakeUpCounter: Wake up counter 00935 * @param WakeUpClock: Wake up clock 00936 * @retval HAL status 00937 */ 00938 HAL_StatusTypeDef HAL_RTCEx_SetWakeUpTimer(RTC_HandleTypeDef *hrtc, uint32_t WakeUpCounter, uint32_t WakeUpClock) 00939 { 00940 uint32_t tickstart = 0; 00941 00942 /* Check the parameters */ 00943 assert_param(IS_RTC_WAKEUP_CLOCK(WakeUpClock)); 00944 assert_param(IS_RTC_WAKEUP_COUNTER(WakeUpCounter)); 00945 00946 /* Process Locked */ 00947 __HAL_LOCK(hrtc); 00948 00949 hrtc->State = HAL_RTC_STATE_BUSY; 00950 00951 /* Disable the write protection for RTC registers */ 00952 __HAL_RTC_WRITEPROTECTION_DISABLE(hrtc); 00953 00954 /*Check RTC WUTWF flag is reset only when wake up timer enabled*/ 00955 if((hrtc->Instance->CR & RTC_CR_WUTE) != RESET) 00956 { 00957 tickstart = HAL_GetTick(); 00958 00959 /* Wait till RTC WUTWF flag is reset and if Time out is reached exit */ 00960 while(__HAL_RTC_WAKEUPTIMER_GET_FLAG(hrtc, RTC_FLAG_WUTWF) == SET) 00961 { 00962 if((HAL_GetTick() - tickstart ) > RTC_TIMEOUT_VALUE) 00963 { 00964 /* Enable the write protection for RTC registers */ 00965 __HAL_RTC_WRITEPROTECTION_ENABLE(hrtc); 00966 00967 hrtc->State = HAL_RTC_STATE_TIMEOUT; 00968 00969 /* Process Unlocked */ 00970 __HAL_UNLOCK(hrtc); 00971 00972 return HAL_TIMEOUT; 00973 } 00974 } 00975 } 00976 00977 __HAL_RTC_WAKEUPTIMER_DISABLE(hrtc); 00978 00979 tickstart = HAL_GetTick(); 00980 00981 /* Wait till RTC WUTWF flag is set and if Time out is reached exit */ 00982 while(__HAL_RTC_WAKEUPTIMER_GET_FLAG(hrtc, RTC_FLAG_WUTWF) == RESET) 00983 { 00984 if((HAL_GetTick() - tickstart ) > RTC_TIMEOUT_VALUE) 00985 { 00986 /* Enable the write protection for RTC registers */ 00987 __HAL_RTC_WRITEPROTECTION_ENABLE(hrtc); 00988 00989 hrtc->State = HAL_RTC_STATE_TIMEOUT; 00990 00991 /* Process Unlocked */ 00992 __HAL_UNLOCK(hrtc); 00993 00994 return HAL_TIMEOUT; 00995 } 00996 } 00997 00998 /* Clear the Wakeup Timer clock source bits in CR register */ 00999 hrtc->Instance->CR &= (uint32_t)~RTC_CR_WUCKSEL; 01000 01001 /* Configure the clock source */ 01002 hrtc->Instance->CR |= (uint32_t)WakeUpClock; 01003 01004 /* Configure the Wakeup Timer counter */ 01005 hrtc->Instance->WUTR = (uint32_t)WakeUpCounter; 01006 01007 /* Enable the Wakeup Timer */ 01008 __HAL_RTC_WAKEUPTIMER_ENABLE(hrtc); 01009 01010 /* Enable the write protection for RTC registers */ 01011 __HAL_RTC_WRITEPROTECTION_ENABLE(hrtc); 01012 01013 hrtc->State = HAL_RTC_STATE_READY; 01014 01015 /* Process Unlocked */ 01016 __HAL_UNLOCK(hrtc); 01017 01018 return HAL_OK; 01019 } 01020 01021 /** 01022 * @brief Set wake up timer with interrupt. 01023 * @param hrtc: RTC handle 01024 * @param WakeUpCounter: Wake up counter 01025 * @param WakeUpClock: Wake up clock 01026 * @retval HAL status 01027 */ 01028 HAL_StatusTypeDef HAL_RTCEx_SetWakeUpTimer_IT(RTC_HandleTypeDef *hrtc, uint32_t WakeUpCounter, uint32_t WakeUpClock) 01029 { 01030 uint32_t tickstart = 0; 01031 01032 /* Check the parameters */ 01033 assert_param(IS_RTC_WAKEUP_CLOCK(WakeUpClock)); 01034 assert_param(IS_RTC_WAKEUP_COUNTER(WakeUpCounter)); 01035 01036 /* Process Locked */ 01037 __HAL_LOCK(hrtc); 01038 01039 hrtc->State = HAL_RTC_STATE_BUSY; 01040 01041 /* Disable the write protection for RTC registers */ 01042 __HAL_RTC_WRITEPROTECTION_DISABLE(hrtc); 01043 01044 /*Check RTC WUTWF flag is reset only when wake up timer enabled*/ 01045 if((hrtc->Instance->CR & RTC_CR_WUTE) != RESET) 01046 { 01047 tickstart = HAL_GetTick(); 01048 01049 /* Wait till RTC WUTWF flag is reset and if Time out is reached exit */ 01050 while(__HAL_RTC_WAKEUPTIMER_GET_FLAG(hrtc, RTC_FLAG_WUTWF) == SET) 01051 { 01052 if((HAL_GetTick() - tickstart ) > RTC_TIMEOUT_VALUE) 01053 { 01054 /* Enable the write protection for RTC registers */ 01055 __HAL_RTC_WRITEPROTECTION_ENABLE(hrtc); 01056 01057 hrtc->State = HAL_RTC_STATE_TIMEOUT; 01058 01059 /* Process Unlocked */ 01060 __HAL_UNLOCK(hrtc); 01061 01062 return HAL_TIMEOUT; 01063 } 01064 } 01065 } 01066 01067 __HAL_RTC_WAKEUPTIMER_DISABLE(hrtc); 01068 01069 tickstart = HAL_GetTick(); 01070 01071 /* Wait till RTC WUTWF flag is set and if Time out is reached exit */ 01072 while(__HAL_RTC_WAKEUPTIMER_GET_FLAG(hrtc, RTC_FLAG_WUTWF) == RESET) 01073 { 01074 if((HAL_GetTick() - tickstart ) > RTC_TIMEOUT_VALUE) 01075 { 01076 /* Enable the write protection for RTC registers */ 01077 __HAL_RTC_WRITEPROTECTION_ENABLE(hrtc); 01078 01079 hrtc->State = HAL_RTC_STATE_TIMEOUT; 01080 01081 /* Process Unlocked */ 01082 __HAL_UNLOCK(hrtc); 01083 01084 return HAL_TIMEOUT; 01085 } 01086 } 01087 01088 /* Configure the Wakeup Timer counter */ 01089 hrtc->Instance->WUTR = (uint32_t)WakeUpCounter; 01090 01091 /* Clear the Wakeup Timer clock source bits in CR register */ 01092 hrtc->Instance->CR &= (uint32_t)~RTC_CR_WUCKSEL; 01093 01094 /* Configure the clock source */ 01095 hrtc->Instance->CR |= (uint32_t)WakeUpClock; 01096 01097 /* RTC WakeUpTimer Interrupt Configuration: EXTI configuration */ 01098 __HAL_RTC_WAKEUPTIMER_EXTI_ENABLE_IT(); 01099 01100 __HAL_RTC_WAKEUPTIMER_EXTI_ENABLE_RISING_EDGE(); 01101 01102 /* Configure the Interrupt in the RTC_CR register */ 01103 __HAL_RTC_WAKEUPTIMER_ENABLE_IT(hrtc,RTC_IT_WUT); 01104 01105 /* Enable the Wakeup Timer */ 01106 __HAL_RTC_WAKEUPTIMER_ENABLE(hrtc); 01107 01108 /* Enable the write protection for RTC registers */ 01109 __HAL_RTC_WRITEPROTECTION_ENABLE(hrtc); 01110 01111 hrtc->State = HAL_RTC_STATE_READY; 01112 01113 /* Process Unlocked */ 01114 __HAL_UNLOCK(hrtc); 01115 01116 return HAL_OK; 01117 } 01118 01119 /** 01120 * @brief Deactivate wake up timer counter. 01121 * @param hrtc: RTC handle 01122 * @retval HAL status 01123 */ 01124 uint32_t HAL_RTCEx_DeactivateWakeUpTimer(RTC_HandleTypeDef *hrtc) 01125 { 01126 uint32_t tickstart = 0; 01127 01128 /* Process Locked */ 01129 __HAL_LOCK(hrtc); 01130 01131 hrtc->State = HAL_RTC_STATE_BUSY; 01132 01133 /* Disable the write protection for RTC registers */ 01134 __HAL_RTC_WRITEPROTECTION_DISABLE(hrtc); 01135 01136 /* Disable the Wakeup Timer */ 01137 __HAL_RTC_WAKEUPTIMER_DISABLE(hrtc); 01138 01139 /* In case of interrupt mode is used, the interrupt source must disabled */ 01140 __HAL_RTC_WAKEUPTIMER_DISABLE_IT(hrtc,RTC_IT_WUT); 01141 01142 tickstart = HAL_GetTick(); 01143 /* Wait till RTC WUTWF flag is set and if Time out is reached exit */ 01144 while(__HAL_RTC_WAKEUPTIMER_GET_FLAG(hrtc, RTC_FLAG_WUTWF) == RESET) 01145 { 01146 if((HAL_GetTick() - tickstart ) > RTC_TIMEOUT_VALUE) 01147 { 01148 /* Enable the write protection for RTC registers */ 01149 __HAL_RTC_WRITEPROTECTION_ENABLE(hrtc); 01150 01151 hrtc->State = HAL_RTC_STATE_TIMEOUT; 01152 01153 /* Process Unlocked */ 01154 __HAL_UNLOCK(hrtc); 01155 01156 return HAL_TIMEOUT; 01157 } 01158 } 01159 01160 /* Enable the write protection for RTC registers */ 01161 __HAL_RTC_WRITEPROTECTION_ENABLE(hrtc); 01162 01163 hrtc->State = HAL_RTC_STATE_READY; 01164 01165 /* Process Unlocked */ 01166 __HAL_UNLOCK(hrtc); 01167 01168 return HAL_OK; 01169 } 01170 01171 /** 01172 * @brief Get wake up timer counter. 01173 * @param hrtc: RTC handle 01174 * @retval Counter value 01175 */ 01176 uint32_t HAL_RTCEx_GetWakeUpTimer(RTC_HandleTypeDef *hrtc) 01177 { 01178 /* Get the counter value */ 01179 return ((uint32_t)(hrtc->Instance->WUTR & RTC_WUTR_WUT)); 01180 } 01181 01182 /** 01183 * @brief Handle Wake Up Timer interrupt request. 01184 * @param hrtc: RTC handle 01185 * @retval None 01186 */ 01187 void HAL_RTCEx_WakeUpTimerIRQHandler(RTC_HandleTypeDef *hrtc) 01188 { 01189 /* Get the pending status of the WAKEUPTIMER Interrupt */ 01190 if(__HAL_RTC_WAKEUPTIMER_GET_FLAG(hrtc, RTC_FLAG_WUTF) != RESET) 01191 { 01192 /* WAKEUPTIMER callback */ 01193 HAL_RTCEx_WakeUpTimerEventCallback(hrtc); 01194 01195 /* Clear the WAKEUPTIMER interrupt pending bit */ 01196 __HAL_RTC_WAKEUPTIMER_CLEAR_FLAG(hrtc, RTC_FLAG_WUTF); 01197 } 01198 01199 01200 /* Clear the EXTI's line Flag for RTC WakeUpTimer */ 01201 __HAL_RTC_WAKEUPTIMER_EXTI_CLEAR_FLAG(); 01202 01203 /* Change RTC state */ 01204 hrtc->State = HAL_RTC_STATE_READY; 01205 } 01206 01207 /** 01208 * @brief Wake Up Timer callback. 01209 * @param hrtc: RTC handle 01210 * @retval None 01211 */ 01212 __weak void HAL_RTCEx_WakeUpTimerEventCallback(RTC_HandleTypeDef *hrtc) 01213 { 01214 /* NOTE : This function should not be modified, when the callback is needed, 01215 the HAL_RTCEx_WakeUpTimerEventCallback could be implemented in the user file 01216 */ 01217 } 01218 01219 /** 01220 * @brief Handle Wake Up Timer Polling. 01221 * @param hrtc: RTC handle 01222 * @param Timeout: Timeout duration 01223 * @retval HAL status 01224 */ 01225 HAL_StatusTypeDef HAL_RTCEx_PollForWakeUpTimerEvent(RTC_HandleTypeDef *hrtc, uint32_t Timeout) 01226 { 01227 uint32_t tickstart = HAL_GetTick(); 01228 01229 while(__HAL_RTC_WAKEUPTIMER_GET_FLAG(hrtc, RTC_FLAG_WUTF) == RESET) 01230 { 01231 if(Timeout != HAL_MAX_DELAY) 01232 { 01233 if((Timeout == 0)||((HAL_GetTick() - tickstart ) > Timeout)) 01234 { 01235 hrtc->State = HAL_RTC_STATE_TIMEOUT; 01236 01237 return HAL_TIMEOUT; 01238 } 01239 } 01240 } 01241 01242 /* Clear the WAKEUPTIMER Flag */ 01243 __HAL_RTC_WAKEUPTIMER_CLEAR_FLAG(hrtc, RTC_FLAG_WUTF); 01244 01245 /* Change RTC state */ 01246 hrtc->State = HAL_RTC_STATE_READY; 01247 01248 return HAL_OK; 01249 } 01250 01251 /** 01252 * @} 01253 */ 01254 01255 01256 /** @defgroup RTCEx_Exported_Functions_Group3 Extended Peripheral Control functions 01257 * @brief Extended Peripheral Control functions 01258 * 01259 @verbatim 01260 =============================================================================== 01261 ##### Extended Peripheral Control functions ##### 01262 =============================================================================== 01263 [..] 01264 This subsection provides functions allowing to 01265 (+) Write a data in a specified RTC Backup data register 01266 (+) Read a data in a specified RTC Backup data register 01267 (+) Set the Coarse calibration parameters. 01268 (+) Deactivate the Coarse calibration parameters 01269 (+) Set the Smooth calibration parameters. 01270 (+) Configure the Synchronization Shift Control Settings. 01271 (+) Configure the Calibration Pinout (RTC_CALIB) Selection (1Hz or 512Hz). 01272 (+) Deactivate the Calibration Pinout (RTC_CALIB) Selection (1Hz or 512Hz). 01273 (+) Enable the RTC reference clock detection. 01274 (+) Disable the RTC reference clock detection. 01275 (+) Enable the Bypass Shadow feature. 01276 (+) Disable the Bypass Shadow feature. 01277 01278 @endverbatim 01279 * @{ 01280 */ 01281 01282 /** 01283 * @brief Write a data in a specified RTC Backup data register. 01284 * @param hrtc: RTC handle 01285 * @param BackupRegister: RTC Backup data Register number. 01286 * This parameter can be: RTC_BKP_DRx where x can be from 0 to 19 to 01287 * specify the register. 01288 * @param Data: Data to be written in the specified RTC Backup data register. 01289 * @retval None 01290 */ 01291 void HAL_RTCEx_BKUPWrite(RTC_HandleTypeDef *hrtc, uint32_t BackupRegister, uint32_t Data) 01292 { 01293 uint32_t tmp = 0; 01294 01295 /* Check the parameters */ 01296 assert_param(IS_RTC_BKP(BackupRegister)); 01297 01298 tmp = (uint32_t)&(hrtc->Instance->BKP0R); 01299 tmp += (BackupRegister * 4); 01300 01301 /* Write the specified register */ 01302 *(__IO uint32_t *)tmp = (uint32_t)Data; 01303 } 01304 01305 /** 01306 * @brief Read data from the specified RTC Backup data Register. 01307 * @param hrtc: RTC handle 01308 * @param BackupRegister: RTC Backup data Register number. 01309 * This parameter can be: RTC_BKP_DRx where x can be from 0 to 19 to 01310 * specify the register. 01311 * @retval Read value 01312 */ 01313 uint32_t HAL_RTCEx_BKUPRead(RTC_HandleTypeDef *hrtc, uint32_t BackupRegister) 01314 { 01315 uint32_t tmp = 0; 01316 01317 /* Check the parameters */ 01318 assert_param(IS_RTC_BKP(BackupRegister)); 01319 01320 tmp = (uint32_t)&(hrtc->Instance->BKP0R); 01321 tmp += (BackupRegister * 4); 01322 01323 /* Read the specified register */ 01324 return (*(__IO uint32_t *)tmp); 01325 } 01326 01327 /** 01328 * @brief Set the Smooth calibration parameters. 01329 * @param hrtc: RTC handle 01330 * @param SmoothCalibPeriod: Select the Smooth Calibration Period. 01331 * This parameter can be can be one of the following values : 01332 * @arg RTC_SMOOTHCALIB_PERIOD_32SEC: The smooth calibration period is 32s. 01333 * @arg RTC_SMOOTHCALIB_PERIOD_16SEC: The smooth calibration period is 16s. 01334 * @arg RTC_SMOOTHCALIB_PERIOD_8SEC: The smooth calibration period is 8s. 01335 * @param SmoothCalibPlusPulses: Select to Set or reset the CALP bit. 01336 * This parameter can be one of the following values: 01337 * @arg RTC_SMOOTHCALIB_PLUSPULSES_SET: Add one RTCCLK pulse every 2*11 pulses. 01338 * @arg RTC_SMOOTHCALIB_PLUSPULSES_RESET: No RTCCLK pulses are added. 01339 * @param SmoothCalibMinusPulsesValue: Select the value of CALM[8:0] bits. 01340 * This parameter can be one any value from 0 to 0x000001FF. 01341 * @note To deactivate the smooth calibration, the field SmoothCalibPlusPulses 01342 * must be equal to SMOOTHCALIB_PLUSPULSES_RESET and the field 01343 * SmoothCalibMinusPulsesValue must be equal to 0. 01344 * @retval HAL status 01345 */ 01346 HAL_StatusTypeDef HAL_RTCEx_SetSmoothCalib(RTC_HandleTypeDef* hrtc, uint32_t SmoothCalibPeriod, uint32_t SmoothCalibPlusPulses, uint32_t SmoothCalibMinusPulsesValue) 01347 { 01348 uint32_t tickstart = 0; 01349 01350 /* Check the parameters */ 01351 assert_param(IS_RTC_SMOOTH_CALIB_PERIOD(SmoothCalibPeriod)); 01352 assert_param(IS_RTC_SMOOTH_CALIB_PLUS(SmoothCalibPlusPulses)); 01353 assert_param(IS_RTC_SMOOTH_CALIB_MINUS(SmoothCalibMinusPulsesValue)); 01354 01355 /* Process Locked */ 01356 __HAL_LOCK(hrtc); 01357 01358 hrtc->State = HAL_RTC_STATE_BUSY; 01359 01360 /* Disable the write protection for RTC registers */ 01361 __HAL_RTC_WRITEPROTECTION_DISABLE(hrtc); 01362 01363 /* check if a calibration is pending*/ 01364 if((hrtc->Instance->ISR & RTC_ISR_RECALPF) != RESET) 01365 { 01366 tickstart = HAL_GetTick(); 01367 01368 /* check if a calibration is pending*/ 01369 while((hrtc->Instance->ISR & RTC_ISR_RECALPF) != RESET) 01370 { 01371 if((HAL_GetTick() - tickstart ) > RTC_TIMEOUT_VALUE) 01372 { 01373 /* Enable the write protection for RTC registers */ 01374 __HAL_RTC_WRITEPROTECTION_ENABLE(hrtc); 01375 01376 /* Change RTC state */ 01377 hrtc->State = HAL_RTC_STATE_TIMEOUT; 01378 01379 /* Process Unlocked */ 01380 __HAL_UNLOCK(hrtc); 01381 01382 return HAL_TIMEOUT; 01383 } 01384 } 01385 } 01386 01387 /* Configure the Smooth calibration settings */ 01388 hrtc->Instance->CALR = (uint32_t)((uint32_t)SmoothCalibPeriod | (uint32_t)SmoothCalibPlusPulses | (uint32_t)SmoothCalibMinusPulsesValue); 01389 01390 /* Enable the write protection for RTC registers */ 01391 __HAL_RTC_WRITEPROTECTION_ENABLE(hrtc); 01392 01393 /* Change RTC state */ 01394 hrtc->State = HAL_RTC_STATE_READY; 01395 01396 /* Process Unlocked */ 01397 __HAL_UNLOCK(hrtc); 01398 01399 return HAL_OK; 01400 } 01401 01402 /** 01403 * @brief Configure the Synchronization Shift Control Settings. 01404 * @note When REFCKON is set, firmware must not write to Shift control register. 01405 * @param hrtc: RTC handle 01406 * @param ShiftAdd1S: Select to add or not 1 second to the time calendar. 01407 * This parameter can be one of the following values : 01408 * @arg RTC_SHIFTADD1S_SET: Add one second to the clock calendar. 01409 * @arg RTC_SHIFTADD1S_RESET: No effect. 01410 * @param ShiftSubFS: Select the number of Second Fractions to substitute. 01411 * This parameter can be one any value from 0 to 0x7FFF. 01412 * @retval HAL status 01413 */ 01414 HAL_StatusTypeDef HAL_RTCEx_SetSynchroShift(RTC_HandleTypeDef* hrtc, uint32_t ShiftAdd1S, uint32_t ShiftSubFS) 01415 { 01416 uint32_t tickstart = 0; 01417 01418 /* Check the parameters */ 01419 assert_param(IS_RTC_SHIFT_ADD1S(ShiftAdd1S)); 01420 assert_param(IS_RTC_SHIFT_SUBFS(ShiftSubFS)); 01421 01422 /* Process Locked */ 01423 __HAL_LOCK(hrtc); 01424 01425 hrtc->State = HAL_RTC_STATE_BUSY; 01426 01427 /* Disable the write protection for RTC registers */ 01428 __HAL_RTC_WRITEPROTECTION_DISABLE(hrtc); 01429 01430 tickstart = HAL_GetTick(); 01431 01432 /* Wait until the shift is completed*/ 01433 while((hrtc->Instance->ISR & RTC_ISR_SHPF) != RESET) 01434 { 01435 if((HAL_GetTick() - tickstart ) > RTC_TIMEOUT_VALUE) 01436 { 01437 /* Enable the write protection for RTC registers */ 01438 __HAL_RTC_WRITEPROTECTION_ENABLE(hrtc); 01439 01440 hrtc->State = HAL_RTC_STATE_TIMEOUT; 01441 01442 /* Process Unlocked */ 01443 __HAL_UNLOCK(hrtc); 01444 01445 return HAL_TIMEOUT; 01446 } 01447 } 01448 01449 /* Check if the reference clock detection is disabled */ 01450 if((hrtc->Instance->CR & RTC_CR_REFCKON) == RESET) 01451 { 01452 /* Configure the Shift settings */ 01453 hrtc->Instance->SHIFTR = (uint32_t)(uint32_t)(ShiftSubFS) | (uint32_t)(ShiftAdd1S); 01454 01455 /* If RTC_CR_BYPSHAD bit = 0, wait for synchro else this check is not needed */ 01456 if((hrtc->Instance->CR & RTC_CR_BYPSHAD) == RESET) 01457 { 01458 if(HAL_RTC_WaitForSynchro(hrtc) != HAL_OK) 01459 { 01460 /* Enable the write protection for RTC registers */ 01461 __HAL_RTC_WRITEPROTECTION_ENABLE(hrtc); 01462 01463 hrtc->State = HAL_RTC_STATE_ERROR; 01464 01465 /* Process Unlocked */ 01466 __HAL_UNLOCK(hrtc); 01467 01468 return HAL_ERROR; 01469 } 01470 } 01471 } 01472 else 01473 { 01474 /* Enable the write protection for RTC registers */ 01475 __HAL_RTC_WRITEPROTECTION_ENABLE(hrtc); 01476 01477 /* Change RTC state */ 01478 hrtc->State = HAL_RTC_STATE_ERROR; 01479 01480 /* Process Unlocked */ 01481 __HAL_UNLOCK(hrtc); 01482 01483 return HAL_ERROR; 01484 } 01485 01486 /* Enable the write protection for RTC registers */ 01487 __HAL_RTC_WRITEPROTECTION_ENABLE(hrtc); 01488 01489 /* Change RTC state */ 01490 hrtc->State = HAL_RTC_STATE_READY; 01491 01492 /* Process Unlocked */ 01493 __HAL_UNLOCK(hrtc); 01494 01495 return HAL_OK; 01496 } 01497 01498 /** 01499 * @brief Configure the Calibration Pinout (RTC_CALIB) Selection (1Hz or 512Hz). 01500 * @param hrtc: RTC handle 01501 * @param CalibOutput : Select the Calibration output Selection . 01502 * This parameter can be one of the following values: 01503 * @arg RTC_CALIBOUTPUT_512HZ: A signal has a regular waveform at 512Hz. 01504 * @arg RTC_CALIBOUTPUT_1HZ: A signal has a regular waveform at 1Hz. 01505 * @retval HAL status 01506 */ 01507 HAL_StatusTypeDef HAL_RTCEx_SetCalibrationOutPut(RTC_HandleTypeDef* hrtc, uint32_t CalibOutput) 01508 { 01509 /* Check the parameters */ 01510 assert_param(IS_RTC_CALIB_OUTPUT(CalibOutput)); 01511 01512 /* Process Locked */ 01513 __HAL_LOCK(hrtc); 01514 01515 hrtc->State = HAL_RTC_STATE_BUSY; 01516 01517 /* Disable the write protection for RTC registers */ 01518 __HAL_RTC_WRITEPROTECTION_DISABLE(hrtc); 01519 01520 /* Clear flags before config */ 01521 hrtc->Instance->CR &= (uint32_t)~RTC_CR_COSEL; 01522 01523 /* Configure the RTC_CR register */ 01524 hrtc->Instance->CR |= (uint32_t)CalibOutput; 01525 01526 __HAL_RTC_CALIBRATION_OUTPUT_ENABLE(hrtc); 01527 01528 /* Enable the write protection for RTC registers */ 01529 __HAL_RTC_WRITEPROTECTION_ENABLE(hrtc); 01530 01531 /* Change RTC state */ 01532 hrtc->State = HAL_RTC_STATE_READY; 01533 01534 /* Process Unlocked */ 01535 __HAL_UNLOCK(hrtc); 01536 01537 return HAL_OK; 01538 } 01539 01540 /** 01541 * @brief Deactivate the Calibration Pinout (RTC_CALIB) Selection (1Hz or 512Hz). 01542 * @param hrtc: RTC handle 01543 * @retval HAL status 01544 */ 01545 HAL_StatusTypeDef HAL_RTCEx_DeactivateCalibrationOutPut(RTC_HandleTypeDef* hrtc) 01546 { 01547 /* Process Locked */ 01548 __HAL_LOCK(hrtc); 01549 01550 hrtc->State = HAL_RTC_STATE_BUSY; 01551 01552 /* Disable the write protection for RTC registers */ 01553 __HAL_RTC_WRITEPROTECTION_DISABLE(hrtc); 01554 01555 __HAL_RTC_CALIBRATION_OUTPUT_DISABLE(hrtc); 01556 01557 /* Enable the write protection for RTC registers */ 01558 __HAL_RTC_WRITEPROTECTION_ENABLE(hrtc); 01559 01560 /* Change RTC state */ 01561 hrtc->State = HAL_RTC_STATE_READY; 01562 01563 /* Process Unlocked */ 01564 __HAL_UNLOCK(hrtc); 01565 01566 return HAL_OK; 01567 } 01568 01569 /** 01570 * @brief Enable the RTC reference clock detection. 01571 * @param hrtc: RTC handle 01572 * @retval HAL status 01573 */ 01574 HAL_StatusTypeDef HAL_RTCEx_SetRefClock(RTC_HandleTypeDef* hrtc) 01575 { 01576 /* Process Locked */ 01577 __HAL_LOCK(hrtc); 01578 01579 hrtc->State = HAL_RTC_STATE_BUSY; 01580 01581 /* Disable the write protection for RTC registers */ 01582 __HAL_RTC_WRITEPROTECTION_DISABLE(hrtc); 01583 01584 /* Set Initialization mode */ 01585 if(RTC_EnterInitMode(hrtc) != HAL_OK) 01586 { 01587 /* Enable the write protection for RTC registers */ 01588 __HAL_RTC_WRITEPROTECTION_ENABLE(hrtc); 01589 01590 /* Set RTC state*/ 01591 hrtc->State = HAL_RTC_STATE_ERROR; 01592 01593 /* Process Unlocked */ 01594 __HAL_UNLOCK(hrtc); 01595 01596 return HAL_ERROR; 01597 } 01598 else 01599 { 01600 __HAL_RTC_CLOCKREF_DETECTION_ENABLE(hrtc); 01601 01602 /* Exit Initialization mode */ 01603 hrtc->Instance->ISR &= (uint32_t)~RTC_ISR_INIT; 01604 } 01605 01606 /* Enable the write protection for RTC registers */ 01607 __HAL_RTC_WRITEPROTECTION_ENABLE(hrtc); 01608 01609 /* Change RTC state */ 01610 hrtc->State = HAL_RTC_STATE_READY; 01611 01612 /* Process Unlocked */ 01613 __HAL_UNLOCK(hrtc); 01614 01615 return HAL_OK; 01616 } 01617 01618 /** 01619 * @brief Disable the RTC reference clock detection. 01620 * @param hrtc: RTC handle 01621 * @retval HAL status 01622 */ 01623 HAL_StatusTypeDef HAL_RTCEx_DeactivateRefClock(RTC_HandleTypeDef* hrtc) 01624 { 01625 /* Process Locked */ 01626 __HAL_LOCK(hrtc); 01627 01628 hrtc->State = HAL_RTC_STATE_BUSY; 01629 01630 /* Disable the write protection for RTC registers */ 01631 __HAL_RTC_WRITEPROTECTION_DISABLE(hrtc); 01632 01633 /* Set Initialization mode */ 01634 if(RTC_EnterInitMode(hrtc) != HAL_OK) 01635 { 01636 /* Enable the write protection for RTC registers */ 01637 __HAL_RTC_WRITEPROTECTION_ENABLE(hrtc); 01638 01639 /* Set RTC state*/ 01640 hrtc->State = HAL_RTC_STATE_ERROR; 01641 01642 /* Process Unlocked */ 01643 __HAL_UNLOCK(hrtc); 01644 01645 return HAL_ERROR; 01646 } 01647 else 01648 { 01649 __HAL_RTC_CLOCKREF_DETECTION_DISABLE(hrtc); 01650 01651 /* Exit Initialization mode */ 01652 hrtc->Instance->ISR &= (uint32_t)~RTC_ISR_INIT; 01653 } 01654 01655 /* Enable the write protection for RTC registers */ 01656 __HAL_RTC_WRITEPROTECTION_ENABLE(hrtc); 01657 01658 /* Change RTC state */ 01659 hrtc->State = HAL_RTC_STATE_READY; 01660 01661 /* Process Unlocked */ 01662 __HAL_UNLOCK(hrtc); 01663 01664 return HAL_OK; 01665 } 01666 01667 /** 01668 * @brief Enable the Bypass Shadow feature. 01669 * @param hrtc: RTC handle 01670 * @note When the Bypass Shadow is enabled the calendar value are taken 01671 * directly from the Calendar counter. 01672 * @retval HAL status 01673 */ 01674 HAL_StatusTypeDef HAL_RTCEx_EnableBypassShadow(RTC_HandleTypeDef* hrtc) 01675 { 01676 /* Process Locked */ 01677 __HAL_LOCK(hrtc); 01678 01679 hrtc->State = HAL_RTC_STATE_BUSY; 01680 01681 /* Disable the write protection for RTC registers */ 01682 __HAL_RTC_WRITEPROTECTION_DISABLE(hrtc); 01683 01684 /* Set the BYPSHAD bit */ 01685 hrtc->Instance->CR |= (uint8_t)RTC_CR_BYPSHAD; 01686 01687 /* Enable the write protection for RTC registers */ 01688 __HAL_RTC_WRITEPROTECTION_ENABLE(hrtc); 01689 01690 /* Change RTC state */ 01691 hrtc->State = HAL_RTC_STATE_READY; 01692 01693 /* Process Unlocked */ 01694 __HAL_UNLOCK(hrtc); 01695 01696 return HAL_OK; 01697 } 01698 01699 /** 01700 * @brief Disable the Bypass Shadow feature. 01701 * @param hrtc: RTC handle 01702 * @note When the Bypass Shadow is enabled the calendar value are taken 01703 * directly from the Calendar counter. 01704 * @retval HAL status 01705 */ 01706 HAL_StatusTypeDef HAL_RTCEx_DisableBypassShadow(RTC_HandleTypeDef* hrtc) 01707 { 01708 /* Process Locked */ 01709 __HAL_LOCK(hrtc); 01710 01711 hrtc->State = HAL_RTC_STATE_BUSY; 01712 01713 /* Disable the write protection for RTC registers */ 01714 __HAL_RTC_WRITEPROTECTION_DISABLE(hrtc); 01715 01716 /* Reset the BYPSHAD bit */ 01717 hrtc->Instance->CR &= ((uint8_t)~RTC_CR_BYPSHAD); 01718 01719 /* Enable the write protection for RTC registers */ 01720 __HAL_RTC_WRITEPROTECTION_ENABLE(hrtc); 01721 01722 /* Change RTC state */ 01723 hrtc->State = HAL_RTC_STATE_READY; 01724 01725 /* Process Unlocked */ 01726 __HAL_UNLOCK(hrtc); 01727 01728 return HAL_OK; 01729 } 01730 01731 /** 01732 * @} 01733 */ 01734 01735 /** @defgroup RTCEx_Exported_Functions_Group4 Extended features functions 01736 * @brief Extended features functions 01737 * 01738 @verbatim 01739 =============================================================================== 01740 ##### Extended features functions ##### 01741 =============================================================================== 01742 [..] This section provides functions allowing to: 01743 (+) RTC Alarm B callback 01744 (+) RTC Poll for Alarm B request 01745 01746 @endverbatim 01747 * @{ 01748 */ 01749 01750 /** 01751 * @brief Alarm B callback. 01752 * @param hrtc: RTC handle 01753 * @retval None 01754 */ 01755 __weak void HAL_RTCEx_AlarmBEventCallback(RTC_HandleTypeDef *hrtc) 01756 { 01757 /* NOTE : This function should not be modified, when the callback is needed, 01758 the HAL_RTCEx_AlarmBEventCallback could be implemented in the user file 01759 */ 01760 } 01761 01762 /** 01763 * @brief Handle Alarm B Polling request. 01764 * @param hrtc: RTC handle 01765 * @param Timeout: Timeout duration 01766 * @retval HAL status 01767 */ 01768 HAL_StatusTypeDef HAL_RTCEx_PollForAlarmBEvent(RTC_HandleTypeDef *hrtc, uint32_t Timeout) 01769 { 01770 uint32_t tickstart = HAL_GetTick(); 01771 01772 while(__HAL_RTC_ALARM_GET_FLAG(hrtc, RTC_FLAG_ALRBF) == RESET) 01773 { 01774 if(Timeout != HAL_MAX_DELAY) 01775 { 01776 if((Timeout == 0)||((HAL_GetTick() - tickstart ) > Timeout)) 01777 { 01778 hrtc->State = HAL_RTC_STATE_TIMEOUT; 01779 return HAL_TIMEOUT; 01780 } 01781 } 01782 } 01783 01784 /* Clear the Alarm Flag */ 01785 __HAL_RTC_ALARM_CLEAR_FLAG(hrtc, RTC_FLAG_ALRBF); 01786 01787 /* Change RTC state */ 01788 hrtc->State = HAL_RTC_STATE_READY; 01789 01790 return HAL_OK; 01791 } 01792 01793 /** 01794 * @} 01795 */ 01796 01797 /** 01798 * @} 01799 */ 01800 01801 #endif /* HAL_RTC_MODULE_ENABLED */ 01802 /** 01803 * @} 01804 */ 01805 01806 /** 01807 * @} 01808 */ 01809 01810 /************************ (C) COPYRIGHT STMicroelectronics *****END OF FILE****/ 01811
Generated on Tue Jul 12 2022 11:35:15 by
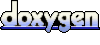