Hal Drivers for L4
Dependents: BSP OneHopeOnePrayer FINAL_AUDIO_RECORD AudioDemo
Fork of STM32L4xx_HAL_Driver by
stm32l4xx_hal_rtc.h
00001 /** 00002 ****************************************************************************** 00003 * @file stm32l4xx_hal_rtc.h 00004 * @author MCD Application Team 00005 * @version V1.1.0 00006 * @date 16-September-2015 00007 * @brief Header file of RTC HAL module. 00008 ****************************************************************************** 00009 * @attention 00010 * 00011 * <h2><center>© COPYRIGHT(c) 2015 STMicroelectronics</center></h2> 00012 * 00013 * Redistribution and use in source and binary forms, with or without modification, 00014 * are permitted provided that the following conditions are met: 00015 * 1. Redistributions of source code must retain the above copyright notice, 00016 * this list of conditions and the following disclaimer. 00017 * 2. Redistributions in binary form must reproduce the above copyright notice, 00018 * this list of conditions and the following disclaimer in the documentation 00019 * and/or other materials provided with the distribution. 00020 * 3. Neither the name of STMicroelectronics nor the names of its contributors 00021 * may be used to endorse or promote products derived from this software 00022 * without specific prior written permission. 00023 * 00024 * THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" 00025 * AND ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE 00026 * IMPLIED WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE 00027 * DISCLAIMED. IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE 00028 * FOR ANY DIRECT, INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL 00029 * DAMAGES (INCLUDING, BUT NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR 00030 * SERVICES; LOSS OF USE, DATA, OR PROFITS; OR BUSINESS INTERRUPTION) HOWEVER 00031 * CAUSED AND ON ANY THEORY OF LIABILITY, WHETHER IN CONTRACT, STRICT LIABILITY, 00032 * OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) ARISING IN ANY WAY OUT OF THE USE 00033 * OF THIS SOFTWARE, EVEN IF ADVISED OF THE POSSIBILITY OF SUCH DAMAGE. 00034 * 00035 ****************************************************************************** 00036 */ 00037 00038 /* Define to prevent recursive inclusion -------------------------------------*/ 00039 #ifndef __STM32L4xx_HAL_RTC_H 00040 #define __STM32L4xx_HAL_RTC_H 00041 00042 #ifdef __cplusplus 00043 extern "C" { 00044 #endif 00045 00046 /* Includes ------------------------------------------------------------------*/ 00047 #include "stm32l4xx_hal_def.h" 00048 00049 /** @addtogroup STM32L4xx_HAL_Driver 00050 * @{ 00051 */ 00052 00053 /** @addtogroup RTC 00054 * @{ 00055 */ 00056 00057 /* Exported types ------------------------------------------------------------*/ 00058 /** @defgroup RTC_Exported_Types RTC Exported Types 00059 * @{ 00060 */ 00061 /** 00062 * @brief HAL State structures definition 00063 */ 00064 typedef enum 00065 { 00066 HAL_RTC_STATE_RESET = 0x00, /*!< RTC not yet initialized or disabled */ 00067 HAL_RTC_STATE_READY = 0x01, /*!< RTC initialized and ready for use */ 00068 HAL_RTC_STATE_BUSY = 0x02, /*!< RTC process is ongoing */ 00069 HAL_RTC_STATE_TIMEOUT = 0x03, /*!< RTC timeout state */ 00070 HAL_RTC_STATE_ERROR = 0x04 /*!< RTC error state */ 00071 00072 }HAL_RTCStateTypeDef; 00073 00074 /** 00075 * @brief RTC Configuration Structure definition 00076 */ 00077 typedef struct 00078 { 00079 uint32_t HourFormat; /*!< Specifies the RTC Hour Format. 00080 This parameter can be a value of @ref RTC_Hour_Formats */ 00081 00082 uint32_t AsynchPrediv; /*!< Specifies the RTC Asynchronous Predivider value. 00083 This parameter must be a number between Min_Data = 0x00 and Max_Data = 0x7F */ 00084 00085 uint32_t SynchPrediv; /*!< Specifies the RTC Synchronous Predivider value. 00086 This parameter must be a number between Min_Data = 0x00 and Max_Data = 0x7FFF */ 00087 00088 uint32_t OutPut; /*!< Specifies which signal will be routed to the RTC output. 00089 This parameter can be a value of @ref RTCEx_Output_selection_Definitions */ 00090 00091 uint32_t OutPutRemap; /*!< Specifies the remap for RTC output. 00092 This parameter can be a value of @ref RTC_Output_ALARM_OUT_Remap */ 00093 00094 uint32_t OutPutPolarity; /*!< Specifies the polarity of the output signal. 00095 This parameter can be a value of @ref RTC_Output_Polarity_Definitions */ 00096 00097 uint32_t OutPutType; /*!< Specifies the RTC Output Pin mode. 00098 This parameter can be a value of @ref RTC_Output_Type_ALARM_OUT */ 00099 }RTC_InitTypeDef; 00100 00101 /** 00102 * @brief RTC Time structure definition 00103 */ 00104 typedef struct 00105 { 00106 uint8_t Hours; /*!< Specifies the RTC Time Hour. 00107 This parameter must be a number between Min_Data = 0 and Max_Data = 12 if the RTC_HourFormat_12 is selected. 00108 This parameter must be a number between Min_Data = 0 and Max_Data = 23 if the RTC_HourFormat_24 is selected */ 00109 00110 uint8_t Minutes; /*!< Specifies the RTC Time Minutes. 00111 This parameter must be a number between Min_Data = 0 and Max_Data = 59 */ 00112 00113 uint8_t Seconds; /*!< Specifies the RTC Time Seconds. 00114 This parameter must be a number between Min_Data = 0 and Max_Data = 59 */ 00115 00116 uint8_t TimeFormat; /*!< Specifies the RTC AM/PM Time. 00117 This parameter can be a value of @ref RTC_AM_PM_Definitions */ 00118 00119 uint32_t SubSeconds; /*!< Specifies the RTC_SSR RTC Sub Second register content. 00120 This parameter corresponds to a time unit range between [0-1] Second 00121 with [1 Sec / SecondFraction +1] granularity */ 00122 00123 uint32_t SecondFraction; /*!< Specifies the range or granularity of Sub Second register content 00124 corresponding to Synchronous pre-scaler factor value (PREDIV_S) 00125 This parameter corresponds to a time unit range between [0-1] Second 00126 with [1 Sec / SecondFraction +1] granularity. 00127 This field will be used only by HAL_RTC_GetTime function */ 00128 00129 uint32_t DayLightSaving; /*!< Specifies RTC_DayLightSaveOperation: the value of hour adjustment. 00130 This parameter can be a value of @ref RTC_DayLightSaving_Definitions */ 00131 00132 uint32_t StoreOperation; /*!< Specifies RTC_StoreOperation value to be written in the BCK bit 00133 in CR register to store the operation. 00134 This parameter can be a value of @ref RTC_StoreOperation_Definitions */ 00135 }RTC_TimeTypeDef; 00136 00137 /** 00138 * @brief RTC Date structure definition 00139 */ 00140 typedef struct 00141 { 00142 uint8_t WeekDay; /*!< Specifies the RTC Date WeekDay. 00143 This parameter can be a value of @ref RTC_WeekDay_Definitions */ 00144 00145 uint8_t Month; /*!< Specifies the RTC Date Month (in BCD format). 00146 This parameter can be a value of @ref RTC_Month_Date_Definitions */ 00147 00148 uint8_t Date; /*!< Specifies the RTC Date. 00149 This parameter must be a number between Min_Data = 1 and Max_Data = 31 */ 00150 00151 uint8_t Year; /*!< Specifies the RTC Date Year. 00152 This parameter must be a number between Min_Data = 0 and Max_Data = 99 */ 00153 00154 }RTC_DateTypeDef; 00155 00156 /** 00157 * @brief RTC Alarm structure definition 00158 */ 00159 typedef struct 00160 { 00161 RTC_TimeTypeDef AlarmTime; /*!< Specifies the RTC Alarm Time members */ 00162 00163 uint32_t AlarmMask; /*!< Specifies the RTC Alarm Masks. 00164 This parameter can be a value of @ref RTC_AlarmMask_Definitions */ 00165 00166 uint32_t AlarmSubSecondMask; /*!< Specifies the RTC Alarm SubSeconds Masks. 00167 This parameter can be a value of @ref RTC_Alarm_Sub_Seconds_Masks_Definitions */ 00168 00169 uint32_t AlarmDateWeekDaySel; /*!< Specifies the RTC Alarm is on Date or WeekDay. 00170 This parameter can be a value of @ref RTC_AlarmDateWeekDay_Definitions */ 00171 00172 uint8_t AlarmDateWeekDay; /*!< Specifies the RTC Alarm Date/WeekDay. 00173 If the Alarm Date is selected, this parameter must be set to a value in the 1-31 range. 00174 If the Alarm WeekDay is selected, this parameter can be a value of @ref RTC_WeekDay_Definitions */ 00175 00176 uint32_t Alarm; /*!< Specifies the alarm . 00177 This parameter can be a value of @ref RTC_Alarms_Definitions */ 00178 }RTC_AlarmTypeDef; 00179 00180 /** 00181 * @brief Time Handle Structure definition 00182 */ 00183 typedef struct 00184 { 00185 RTC_TypeDef *Instance; /*!< Register base address */ 00186 00187 RTC_InitTypeDef Init; /*!< RTC required parameters */ 00188 00189 HAL_LockTypeDef Lock; /*!< RTC locking object */ 00190 00191 __IO HAL_RTCStateTypeDef State; /*!< Time communication state */ 00192 00193 }RTC_HandleTypeDef; 00194 00195 /** 00196 * @} 00197 */ 00198 00199 /* Exported constants --------------------------------------------------------*/ 00200 /** @defgroup RTC_Exported_Constants RTC Exported Constants 00201 * @{ 00202 */ 00203 00204 /** @defgroup RTC_Hour_Formats RTC Hour Formats 00205 * @{ 00206 */ 00207 #define RTC_HOURFORMAT_24 ((uint32_t)0x00000000) 00208 #define RTC_HOURFORMAT_12 ((uint32_t)0x00000040) 00209 /** 00210 * @} 00211 */ 00212 00213 /** @defgroup RTC_Output_Polarity_Definitions RTC Output Polarity Definitions 00214 * @{ 00215 */ 00216 #define RTC_OUTPUT_POLARITY_HIGH ((uint32_t)0x00000000) 00217 #define RTC_OUTPUT_POLARITY_LOW ((uint32_t)0x00100000) 00218 /** 00219 * @} 00220 */ 00221 00222 /** @defgroup RTC_Output_Type_ALARM_OUT RTC Output Type ALARM OUT 00223 * @{ 00224 */ 00225 #define RTC_OUTPUT_TYPE_OPENDRAIN ((uint32_t)0x00000000) 00226 #define RTC_OUTPUT_TYPE_PUSHPULL ((uint32_t)RTC_OR_ALARMOUTTYPE) 00227 /** 00228 * @} 00229 */ 00230 00231 /** @defgroup RTC_Output_ALARM_OUT_Remap RTC Output ALARM OUT Remap 00232 * @{ 00233 */ 00234 #define RTC_OUTPUT_REMAP_NONE ((uint32_t)0x00000000) 00235 #define RTC_OUTPUT_REMAP_POS1 ((uint32_t)RTC_OR_OUT_RMP) 00236 /** 00237 * @} 00238 */ 00239 00240 /** @defgroup RTC_AM_PM_Definitions RTC AM PM Definitions 00241 * @{ 00242 */ 00243 #define RTC_HOURFORMAT12_AM ((uint8_t)0x00) 00244 #define RTC_HOURFORMAT12_PM ((uint8_t)0x40) 00245 /** 00246 * @} 00247 */ 00248 00249 /** @defgroup RTC_DayLightSaving_Definitions RTC DayLight Saving Definitions 00250 * @{ 00251 */ 00252 #define RTC_DAYLIGHTSAVING_SUB1H ((uint32_t)0x00020000) 00253 #define RTC_DAYLIGHTSAVING_ADD1H ((uint32_t)0x00010000) 00254 #define RTC_DAYLIGHTSAVING_NONE ((uint32_t)0x00000000) 00255 /** 00256 * @} 00257 */ 00258 00259 /** @defgroup RTC_StoreOperation_Definitions RTC Store Operation Definitions 00260 * @{ 00261 */ 00262 #define RTC_STOREOPERATION_RESET ((uint32_t)0x00000000) 00263 #define RTC_STOREOPERATION_SET ((uint32_t)0x00040000) 00264 /** 00265 * @} 00266 */ 00267 00268 /** @defgroup RTC_Input_parameter_format_definitions RTC Input Parameter Format Definitions 00269 * @{ 00270 */ 00271 #define RTC_FORMAT_BIN ((uint32_t)0x000000000) 00272 #define RTC_FORMAT_BCD ((uint32_t)0x000000001) 00273 /** 00274 * @} 00275 */ 00276 00277 /** @defgroup RTC_Month_Date_Definitions RTC Month Date Definitions 00278 * @{ 00279 */ 00280 00281 /* Coded in BCD format */ 00282 #define RTC_MONTH_JANUARY ((uint8_t)0x01) 00283 #define RTC_MONTH_FEBRUARY ((uint8_t)0x02) 00284 #define RTC_MONTH_MARCH ((uint8_t)0x03) 00285 #define RTC_MONTH_APRIL ((uint8_t)0x04) 00286 #define RTC_MONTH_MAY ((uint8_t)0x05) 00287 #define RTC_MONTH_JUNE ((uint8_t)0x06) 00288 #define RTC_MONTH_JULY ((uint8_t)0x07) 00289 #define RTC_MONTH_AUGUST ((uint8_t)0x08) 00290 #define RTC_MONTH_SEPTEMBER ((uint8_t)0x09) 00291 #define RTC_MONTH_OCTOBER ((uint8_t)0x10) 00292 #define RTC_MONTH_NOVEMBER ((uint8_t)0x11) 00293 #define RTC_MONTH_DECEMBER ((uint8_t)0x12) 00294 /** 00295 * @} 00296 */ 00297 00298 /** @defgroup RTC_WeekDay_Definitions RTC WeekDay Definitions 00299 * @{ 00300 */ 00301 #define RTC_WEEKDAY_MONDAY ((uint8_t)0x01) 00302 #define RTC_WEEKDAY_TUESDAY ((uint8_t)0x02) 00303 #define RTC_WEEKDAY_WEDNESDAY ((uint8_t)0x03) 00304 #define RTC_WEEKDAY_THURSDAY ((uint8_t)0x04) 00305 #define RTC_WEEKDAY_FRIDAY ((uint8_t)0x05) 00306 #define RTC_WEEKDAY_SATURDAY ((uint8_t)0x06) 00307 #define RTC_WEEKDAY_SUNDAY ((uint8_t)0x07) 00308 /** 00309 * @} 00310 */ 00311 00312 /** @defgroup RTC_AlarmDateWeekDay_Definitions RTC Alarm Date WeekDay Definitions 00313 * @{ 00314 */ 00315 #define RTC_ALARMDATEWEEKDAYSEL_DATE ((uint32_t)0x00000000) 00316 #define RTC_ALARMDATEWEEKDAYSEL_WEEKDAY ((uint32_t)0x40000000) 00317 /** 00318 * @} 00319 */ 00320 00321 00322 /** @defgroup RTC_AlarmMask_Definitions RTC Alarm Mask Definitions 00323 * @{ 00324 */ 00325 #define RTC_ALARMMASK_NONE ((uint32_t)0x00000000) 00326 #define RTC_ALARMMASK_DATEWEEKDAY RTC_ALRMAR_MSK4 00327 #define RTC_ALARMMASK_HOURS RTC_ALRMAR_MSK3 00328 #define RTC_ALARMMASK_MINUTES RTC_ALRMAR_MSK2 00329 #define RTC_ALARMMASK_SECONDS RTC_ALRMAR_MSK1 00330 #define RTC_ALARMMASK_ALL ((uint32_t)0x80808080) 00331 /** 00332 * @} 00333 */ 00334 00335 /** @defgroup RTC_Alarms_Definitions RTC Alarms Definitions 00336 * @{ 00337 */ 00338 #define RTC_ALARM_A RTC_CR_ALRAE 00339 #define RTC_ALARM_B RTC_CR_ALRBE 00340 /** 00341 * @} 00342 */ 00343 00344 /** @defgroup RTC_Alarm_Sub_Seconds_Masks_Definitions RTC Alarm Sub Seconds Masks Definitions 00345 * @{ 00346 */ 00347 #define RTC_ALARMSUBSECONDMASK_ALL ((uint32_t)0x00000000) /*!< All Alarm SS fields are masked. 00348 There is no comparison on sub seconds 00349 for Alarm */ 00350 #define RTC_ALARMSUBSECONDMASK_SS14_1 ((uint32_t)0x01000000) /*!< SS[14:1] are don't care in Alarm 00351 comparison. Only SS[0] is compared. */ 00352 #define RTC_ALARMSUBSECONDMASK_SS14_2 ((uint32_t)0x02000000) /*!< SS[14:2] are don't care in Alarm 00353 comparison. Only SS[1:0] are compared */ 00354 #define RTC_ALARMSUBSECONDMASK_SS14_3 ((uint32_t)0x03000000) /*!< SS[14:3] are don't care in Alarm 00355 comparison. Only SS[2:0] are compared */ 00356 #define RTC_ALARMSUBSECONDMASK_SS14_4 ((uint32_t)0x04000000) /*!< SS[14:4] are don't care in Alarm 00357 comparison. Only SS[3:0] are compared */ 00358 #define RTC_ALARMSUBSECONDMASK_SS14_5 ((uint32_t)0x05000000) /*!< SS[14:5] are don't care in Alarm 00359 comparison. Only SS[4:0] are compared */ 00360 #define RTC_ALARMSUBSECONDMASK_SS14_6 ((uint32_t)0x06000000) /*!< SS[14:6] are don't care in Alarm 00361 comparison. Only SS[5:0] are compared */ 00362 #define RTC_ALARMSUBSECONDMASK_SS14_7 ((uint32_t)0x07000000) /*!< SS[14:7] are don't care in Alarm 00363 comparison. Only SS[6:0] are compared */ 00364 #define RTC_ALARMSUBSECONDMASK_SS14_8 ((uint32_t)0x08000000) /*!< SS[14:8] are don't care in Alarm 00365 comparison. Only SS[7:0] are compared */ 00366 #define RTC_ALARMSUBSECONDMASK_SS14_9 ((uint32_t)0x09000000) /*!< SS[14:9] are don't care in Alarm 00367 comparison. Only SS[8:0] are compared */ 00368 #define RTC_ALARMSUBSECONDMASK_SS14_10 ((uint32_t)0x0A000000) /*!< SS[14:10] are don't care in Alarm 00369 comparison. Only SS[9:0] are compared */ 00370 #define RTC_ALARMSUBSECONDMASK_SS14_11 ((uint32_t)0x0B000000) /*!< SS[14:11] are don't care in Alarm 00371 comparison. Only SS[10:0] are compared */ 00372 #define RTC_ALARMSUBSECONDMASK_SS14_12 ((uint32_t)0x0C000000) /*!< SS[14:12] are don't care in Alarm 00373 comparison. Only SS[11:0] are compared */ 00374 #define RTC_ALARMSUBSECONDMASK_SS14_13 ((uint32_t)0x0D000000) /*!< SS[14:13] are don't care in Alarm 00375 comparison. Only SS[12:0] are compared */ 00376 #define RTC_ALARMSUBSECONDMASK_SS14 ((uint32_t)0x0E000000) /*!< SS[14] is don't care in Alarm 00377 comparison. Only SS[13:0] are compared */ 00378 #define RTC_ALARMSUBSECONDMASK_NONE ((uint32_t)0x0F000000) /*!< SS[14:0] are compared and must match 00379 to activate alarm. */ 00380 /** 00381 * @} 00382 */ 00383 00384 /** @defgroup RTC_Interrupts_Definitions RTC Interrupts Definitions 00385 * @{ 00386 */ 00387 #define RTC_IT_TS ((uint32_t)RTC_CR_TSIE) /*!< Enable Timestamp Interrupt */ 00388 #define RTC_IT_WUT ((uint32_t)RTC_CR_WUTIE) /*!< Enable Wakeup timer Interrupt */ 00389 #define RTC_IT_ALRA ((uint32_t)RTC_CR_ALRAIE) /*!< Enable Alarm A Interrupt */ 00390 #define RTC_IT_ALRB ((uint32_t)RTC_CR_ALRBIE) /*!< Enable Alarm B Interrupt */ 00391 #define RTC_IT_TAMP ((uint32_t)RTC_TAMPCR_TAMPIE) /*!< Enable all Tamper Interrupt */ 00392 #define RTC_IT_TAMP1 ((uint32_t)RTC_TAMPCR_TAMP1IE) /*!< Enable Tamper 1 Interrupt */ 00393 #define RTC_IT_TAMP2 ((uint32_t)RTC_TAMPCR_TAMP2IE) /*!< Enable Tamper 2 Interrupt */ 00394 #define RTC_IT_TAMP3 ((uint32_t)RTC_TAMPCR_TAMP3IE) /*!< Enable Tamper 3 Interrupt */ 00395 /** 00396 * @} 00397 */ 00398 00399 /** @defgroup RTC_Flags_Definitions RTC Flags Definitions 00400 * @{ 00401 */ 00402 #define RTC_FLAG_RECALPF ((uint32_t)RTC_ISR_RECALPF) 00403 #define RTC_FLAG_TAMP3F ((uint32_t)RTC_ISR_TAMP3F) 00404 #define RTC_FLAG_TAMP2F ((uint32_t)RTC_ISR_TAMP2F) 00405 #define RTC_FLAG_TAMP1F ((uint32_t)RTC_ISR_TAMP1F) 00406 #define RTC_FLAG_TSOVF ((uint32_t)RTC_ISR_TSOVF) 00407 #define RTC_FLAG_TSF ((uint32_t)RTC_ISR_TSF) 00408 #define RTC_FLAG_ITSF ((uint32_t)RTC_ISR_ITSF) 00409 #define RTC_FLAG_WUTF ((uint32_t)RTC_ISR_WUTF) 00410 #define RTC_FLAG_ALRBF ((uint32_t)RTC_ISR_ALRBF) 00411 #define RTC_FLAG_ALRAF ((uint32_t)RTC_ISR_ALRAF) 00412 #define RTC_FLAG_INITF ((uint32_t)RTC_ISR_INITF) 00413 #define RTC_FLAG_RSF ((uint32_t)RTC_ISR_RSF) 00414 #define RTC_FLAG_INITS ((uint32_t)RTC_ISR_INITS) 00415 #define RTC_FLAG_SHPF ((uint32_t)RTC_ISR_SHPF) 00416 #define RTC_FLAG_WUTWF ((uint32_t)RTC_ISR_WUTWF) 00417 #define RTC_FLAG_ALRBWF ((uint32_t)RTC_ISR_ALRBWF) 00418 #define RTC_FLAG_ALRAWF ((uint32_t)RTC_ISR_ALRAWF) 00419 /** 00420 * @} 00421 */ 00422 00423 /** 00424 * @} 00425 */ 00426 00427 /* Exported macros -----------------------------------------------------------*/ 00428 /** @defgroup RTC_Exported_Macros RTC Exported Macros 00429 * @{ 00430 */ 00431 00432 /** @brief Reset RTC handle state. 00433 * @param __HANDLE__: RTC handle. 00434 * @retval None 00435 */ 00436 #define __HAL_RTC_RESET_HANDLE_STATE(__HANDLE__) ((__HANDLE__)->State = HAL_RTC_STATE_RESET) 00437 00438 /** 00439 * @brief Disable the write protection for RTC registers. 00440 * @param __HANDLE__: specifies the RTC handle. 00441 * @retval None 00442 */ 00443 #define __HAL_RTC_WRITEPROTECTION_DISABLE(__HANDLE__) \ 00444 do{ \ 00445 (__HANDLE__)->Instance->WPR = 0xCA; \ 00446 (__HANDLE__)->Instance->WPR = 0x53; \ 00447 } while(0) 00448 00449 /** 00450 * @brief Enable the write protection for RTC registers. 00451 * @param __HANDLE__: specifies the RTC handle. 00452 * @retval None 00453 */ 00454 #define __HAL_RTC_WRITEPROTECTION_ENABLE(__HANDLE__) \ 00455 do{ \ 00456 (__HANDLE__)->Instance->WPR = 0xFF; \ 00457 } while(0) 00458 00459 00460 /** 00461 * @brief Enable the RTC ALARMA peripheral. 00462 * @param __HANDLE__: specifies the RTC handle. 00463 * @retval None 00464 */ 00465 #define __HAL_RTC_ALARMA_ENABLE(__HANDLE__) ((__HANDLE__)->Instance->CR |= (RTC_CR_ALRAE)) 00466 00467 /** 00468 * @brief Disable the RTC ALARMA peripheral. 00469 * @param __HANDLE__: specifies the RTC handle. 00470 * @retval None 00471 */ 00472 #define __HAL_RTC_ALARMA_DISABLE(__HANDLE__) ((__HANDLE__)->Instance->CR &= ~(RTC_CR_ALRAE)) 00473 00474 /** 00475 * @brief Enable the RTC ALARMB peripheral. 00476 * @param __HANDLE__: specifies the RTC handle. 00477 * @retval None 00478 */ 00479 #define __HAL_RTC_ALARMB_ENABLE(__HANDLE__) ((__HANDLE__)->Instance->CR |= (RTC_CR_ALRBE)) 00480 00481 /** 00482 * @brief Disable the RTC ALARMB peripheral. 00483 * @param __HANDLE__: specifies the RTC handle. 00484 * @retval None 00485 */ 00486 #define __HAL_RTC_ALARMB_DISABLE(__HANDLE__) ((__HANDLE__)->Instance->CR &= ~(RTC_CR_ALRBE)) 00487 00488 /** 00489 * @brief Enable the RTC Alarm interrupt. 00490 * @param __HANDLE__: specifies the RTC handle. 00491 * @param __INTERRUPT__: specifies the RTC Alarm interrupt sources to be enabled or disabled. 00492 * This parameter can be any combination of the following values: 00493 * @arg RTC_IT_ALRA: Alarm A interrupt 00494 * @arg RTC_IT_ALRB: Alarm B interrupt 00495 * @retval None 00496 */ 00497 #define __HAL_RTC_ALARM_ENABLE_IT(__HANDLE__, __INTERRUPT__) ((__HANDLE__)->Instance->CR |= (__INTERRUPT__)) 00498 00499 /** 00500 * @brief Disable the RTC Alarm interrupt. 00501 * @param __HANDLE__: specifies the RTC handle. 00502 * @param __INTERRUPT__: specifies the RTC Alarm interrupt sources to be enabled or disabled. 00503 * This parameter can be any combination of the following values: 00504 * @arg RTC_IT_ALRA: Alarm A interrupt 00505 * @arg RTC_IT_ALRB: Alarm B interrupt 00506 * @retval None 00507 */ 00508 #define __HAL_RTC_ALARM_DISABLE_IT(__HANDLE__, __INTERRUPT__) ((__HANDLE__)->Instance->CR &= ~(__INTERRUPT__)) 00509 00510 /** 00511 * @brief Check whether the specified RTC Alarm interrupt has occurred or not. 00512 * @param __HANDLE__: specifies the RTC handle. 00513 * @param __INTERRUPT__: specifies the RTC Alarm interrupt sources to check. 00514 * This parameter can be: 00515 * @arg RTC_IT_ALRA: Alarm A interrupt 00516 * @arg RTC_IT_ALRB: Alarm B interrupt 00517 * @retval None 00518 */ 00519 #define __HAL_RTC_ALARM_GET_IT(__HANDLE__, __INTERRUPT__) (((((__HANDLE__)->Instance->ISR)& ((__INTERRUPT__)>> 4)) != RESET) ? SET : RESET) 00520 00521 /** 00522 * @brief Get the selected RTC Alarm's flag status. 00523 * @param __HANDLE__: specifies the RTC handle. 00524 * @param __FLAG__: specifies the RTC Alarm Flag sources to check. 00525 * This parameter can be: 00526 * @arg RTC_FLAG_ALRAF 00527 * @arg RTC_FLAG_ALRBF 00528 * @arg RTC_FLAG_ALRAWF 00529 * @arg RTC_FLAG_ALRBWF 00530 * @retval None 00531 */ 00532 #define __HAL_RTC_ALARM_GET_FLAG(__HANDLE__, __FLAG__) (((((__HANDLE__)->Instance->ISR) & (__FLAG__)) != RESET) ? SET : RESET) 00533 00534 /** 00535 * @brief Clear the RTC Alarm's pending flags. 00536 * @param __HANDLE__: specifies the RTC handle. 00537 * @param __FLAG__: specifies the RTC Alarm Flag sources to clear. 00538 * This parameter can be: 00539 * @arg RTC_FLAG_ALRAF 00540 * @arg RTC_FLAG_ALRBF 00541 * @retval None 00542 */ 00543 #define __HAL_RTC_ALARM_CLEAR_FLAG(__HANDLE__, __FLAG__) ((__HANDLE__)->Instance->ISR) = (~((__FLAG__) | RTC_ISR_INIT)|((__HANDLE__)->Instance->ISR & RTC_ISR_INIT)) 00544 00545 /** 00546 * @brief Check whether the specified RTC Alarm interrupt is enabled or not. 00547 * @param __HANDLE__: specifies the RTC handle. 00548 * @param __INTERRUPT__: specifies the RTC Alarm interrupt sources to check. 00549 * This parameter can be: 00550 * @arg RTC_IT_ALRA: Alarm A interrupt 00551 * @arg RTC_IT_ALRB: Alarm B interrupt 00552 * @retval None 00553 */ 00554 #define __HAL_RTC_ALARM_GET_IT_SOURCE(__HANDLE__, __INTERRUPT__) (((((__HANDLE__)->Instance->CR) & (__INTERRUPT__)) != RESET) ? SET : RESET) 00555 00556 /** 00557 * @brief Enable interrupt on the RTC Alarm associated Exti line. 00558 * @retval None 00559 */ 00560 #define __HAL_RTC_ALARM_EXTI_ENABLE_IT() (EXTI->IMR1 |= RTC_EXTI_LINE_ALARM_EVENT) 00561 00562 /** 00563 * @brief Disable interrupt on the RTC Alarm associated Exti line. 00564 * @retval None 00565 */ 00566 #define __HAL_RTC_ALARM_EXTI_DISABLE_IT() (EXTI->IMR1 &= ~(RTC_EXTI_LINE_ALARM_EVENT)) 00567 00568 /** 00569 * @brief Enable event on the RTC Alarm associated Exti line. 00570 * @retval None 00571 */ 00572 #define __HAL_RTC_ALARM_EXTI_ENABLE_EVENT() (EXTI->EMR1 |= RTC_EXTI_LINE_ALARM_EVENT) 00573 00574 /** 00575 * @brief Disable event on the RTC Alarm associated Exti line. 00576 * @retval None 00577 */ 00578 #define __HAL_RTC_ALARM_EXTI_DISABLE_EVENT() (EXTI->EMR1 &= ~(RTC_EXTI_LINE_ALARM_EVENT)) 00579 00580 /** 00581 * @brief Enable falling edge trigger on the RTC Alarm associated Exti line. 00582 * @retval None 00583 */ 00584 #define __HAL_RTC_ALARM_EXTI_ENABLE_FALLING_EDGE() (EXTI->FTSR1 |= RTC_EXTI_LINE_ALARM_EVENT) 00585 00586 /** 00587 * @brief Disable falling edge trigger on the RTC Alarm associated Exti line. 00588 * @retval None 00589 */ 00590 #define __HAL_RTC_ALARM_EXTI_DISABLE_FALLING_EDGE() (EXTI->FTSR1 &= ~(RTC_EXTI_LINE_ALARM_EVENT)) 00591 00592 /** 00593 * @brief Enable rising edge trigger on the RTC Alarm associated Exti line. 00594 * @retval None 00595 */ 00596 #define __HAL_RTC_ALARM_EXTI_ENABLE_RISING_EDGE() (EXTI->RTSR1 |= RTC_EXTI_LINE_ALARM_EVENT) 00597 00598 /** 00599 * @brief Disable rising edge trigger on the RTC Alarm associated Exti line. 00600 * @retval None 00601 */ 00602 #define __HAL_RTC_ALARM_EXTI_DISABLE_RISING_EDGE() (EXTI->RTSR1 &= ~(RTC_EXTI_LINE_ALARM_EVENT)) 00603 00604 /** 00605 * @brief Enable rising & falling edge trigger on the RTC Alarm associated Exti line. 00606 * @retval None 00607 */ 00608 #define __HAL_RTC_ALARM_EXTI_ENABLE_RISING_FALLING_EDGE() do { \ 00609 __HAL_RTC_ALARM_EXTI_ENABLE_RISING_EDGE(); \ 00610 __HAL_RTC_ALARM_EXTI_ENABLE_FALLING_EDGE(); \ 00611 } while(0) 00612 00613 /** 00614 * @brief Disable rising & falling edge trigger on the RTC Alarm associated Exti line. 00615 * @retval None 00616 */ 00617 #define __HAL_RTC_ALARM_EXTI_DISABLE_RISING_FALLING_EDGE() do { \ 00618 __HAL_RTC_ALARM_EXTI_DISABLE_RISING_EDGE(); \ 00619 __HAL_RTC_ALARM_EXTI_DISABLE_FALLING_EDGE(); \ 00620 } while(0) 00621 00622 /** 00623 * @brief Check whether the RTC Alarm associated Exti line interrupt flag is set or not. 00624 * @retval Line Status. 00625 */ 00626 #define __HAL_RTC_ALARM_EXTI_GET_FLAG() (EXTI->PR1 & RTC_EXTI_LINE_ALARM_EVENT) 00627 00628 /** 00629 * @brief Clear the RTC Alarm associated Exti line flag. 00630 * @retval None 00631 */ 00632 #define __HAL_RTC_ALARM_EXTI_CLEAR_FLAG() (EXTI->PR1 = RTC_EXTI_LINE_ALARM_EVENT) 00633 00634 /** 00635 * @brief Generate a Software interrupt on RTC Alarm associated Exti line. 00636 * @retval None 00637 */ 00638 #define __HAL_RTC_ALARM_EXTI_GENERATE_SWIT() (EXTI->SWIER1 |= RTC_EXTI_LINE_ALARM_EVENT) 00639 00640 /** 00641 * @} 00642 */ 00643 00644 /* Include RTC HAL Extended module */ 00645 #include "stm32l4xx_hal_rtc_ex.h" 00646 00647 /* Exported functions --------------------------------------------------------*/ 00648 /** @addtogroup RTC_Exported_Functions 00649 * @{ 00650 */ 00651 00652 /** @addtogroup RTC_Exported_Functions_Group1 00653 * @{ 00654 */ 00655 /* Initialization and de-initialization functions ****************************/ 00656 HAL_StatusTypeDef HAL_RTC_Init(RTC_HandleTypeDef *hrtc); 00657 HAL_StatusTypeDef HAL_RTC_DeInit(RTC_HandleTypeDef *hrtc); 00658 void HAL_RTC_MspInit(RTC_HandleTypeDef *hrtc); 00659 void HAL_RTC_MspDeInit(RTC_HandleTypeDef *hrtc); 00660 /** 00661 * @} 00662 */ 00663 00664 /** @addtogroup RTC_Exported_Functions_Group2 00665 * @{ 00666 */ 00667 /* RTC Time and Date functions ************************************************/ 00668 HAL_StatusTypeDef HAL_RTC_SetTime(RTC_HandleTypeDef *hrtc, RTC_TimeTypeDef *sTime, uint32_t Format); 00669 HAL_StatusTypeDef HAL_RTC_GetTime(RTC_HandleTypeDef *hrtc, RTC_TimeTypeDef *sTime, uint32_t Format); 00670 HAL_StatusTypeDef HAL_RTC_SetDate(RTC_HandleTypeDef *hrtc, RTC_DateTypeDef *sDate, uint32_t Format); 00671 HAL_StatusTypeDef HAL_RTC_GetDate(RTC_HandleTypeDef *hrtc, RTC_DateTypeDef *sDate, uint32_t Format); 00672 /** 00673 * @} 00674 */ 00675 00676 /** @addtogroup RTC_Exported_Functions_Group3 00677 * @{ 00678 */ 00679 /* RTC Alarm functions ********************************************************/ 00680 HAL_StatusTypeDef HAL_RTC_SetAlarm(RTC_HandleTypeDef *hrtc, RTC_AlarmTypeDef *sAlarm, uint32_t Format); 00681 HAL_StatusTypeDef HAL_RTC_SetAlarm_IT(RTC_HandleTypeDef *hrtc, RTC_AlarmTypeDef *sAlarm, uint32_t Format); 00682 HAL_StatusTypeDef HAL_RTC_DeactivateAlarm(RTC_HandleTypeDef *hrtc, uint32_t Alarm); 00683 HAL_StatusTypeDef HAL_RTC_GetAlarm(RTC_HandleTypeDef *hrtc, RTC_AlarmTypeDef *sAlarm, uint32_t Alarm, uint32_t Format); 00684 void HAL_RTC_AlarmIRQHandler(RTC_HandleTypeDef *hrtc); 00685 HAL_StatusTypeDef HAL_RTC_PollForAlarmAEvent(RTC_HandleTypeDef *hrtc, uint32_t Timeout); 00686 void HAL_RTC_AlarmAEventCallback(RTC_HandleTypeDef *hrtc); 00687 /** 00688 * @} 00689 */ 00690 00691 /** @addtogroup RTC_Exported_Functions_Group4 00692 * @{ 00693 */ 00694 /* Peripheral Control functions ***********************************************/ 00695 HAL_StatusTypeDef HAL_RTC_WaitForSynchro(RTC_HandleTypeDef* hrtc); 00696 /** 00697 * @} 00698 */ 00699 00700 /** @addtogroup RTC_Exported_Functions_Group5 00701 * @{ 00702 */ 00703 /* Peripheral State functions *************************************************/ 00704 HAL_RTCStateTypeDef HAL_RTC_GetState(RTC_HandleTypeDef *hrtc); 00705 00706 /** 00707 * @} 00708 */ 00709 00710 /** 00711 * @} 00712 */ 00713 00714 /* Private types -------------------------------------------------------------*/ 00715 /* Private variables ---------------------------------------------------------*/ 00716 /* Private constants ---------------------------------------------------------*/ 00717 /** @defgroup RTC_Private_Constants RTC Private Constants 00718 * @{ 00719 */ 00720 /* Masks Definition */ 00721 #define RTC_TR_RESERVED_MASK ((uint32_t)0x007F7F7F) 00722 #define RTC_DR_RESERVED_MASK ((uint32_t)0x00FFFF3F) 00723 #define RTC_INIT_MASK ((uint32_t)0xFFFFFFFF) 00724 #define RTC_RSF_MASK ((uint32_t)0xFFFFFF5F) 00725 00726 #define RTC_TIMEOUT_VALUE 1000 00727 00728 #define RTC_EXTI_LINE_ALARM_EVENT ((uint32_t)0x00040000) /*!< External interrupt line 18 Connected to the RTC Alarm event */ 00729 00730 /** 00731 * @} 00732 */ 00733 00734 /* Private macros ------------------------------------------------------------*/ 00735 /** @defgroup RTC_Private_Macros RTC Private Macros 00736 * @{ 00737 */ 00738 00739 /** @defgroup RTC_IS_RTC_Definitions RTC Private macros to check input parameters 00740 * @{ 00741 */ 00742 00743 #define IS_RTC_HOUR_FORMAT(FORMAT) (((FORMAT) == RTC_HOURFORMAT_12) || \ 00744 ((FORMAT) == RTC_HOURFORMAT_24)) 00745 00746 #define IS_RTC_OUTPUT_POL(POL) (((POL) == RTC_OUTPUT_POLARITY_HIGH) || \ 00747 ((POL) == RTC_OUTPUT_POLARITY_LOW)) 00748 00749 #define IS_RTC_OUTPUT_TYPE(TYPE) (((TYPE) == RTC_OUTPUT_TYPE_OPENDRAIN) || \ 00750 ((TYPE) == RTC_OUTPUT_TYPE_PUSHPULL)) 00751 00752 #define IS_RTC_OUTPUT_REMAP(REMAP) (((REMAP) == RTC_OUTPUT_REMAP_NONE) || \ 00753 ((REMAP) == RTC_OUTPUT_REMAP_POS1)) 00754 00755 #define IS_RTC_HOURFORMAT12(PM) (((PM) == RTC_HOURFORMAT12_AM) || ((PM) == RTC_HOURFORMAT12_PM)) 00756 00757 #define IS_RTC_DAYLIGHT_SAVING(SAVE) (((SAVE) == RTC_DAYLIGHTSAVING_SUB1H) || \ 00758 ((SAVE) == RTC_DAYLIGHTSAVING_ADD1H) || \ 00759 ((SAVE) == RTC_DAYLIGHTSAVING_NONE)) 00760 00761 #define IS_RTC_STORE_OPERATION(OPERATION) (((OPERATION) == RTC_STOREOPERATION_RESET) || \ 00762 ((OPERATION) == RTC_STOREOPERATION_SET)) 00763 00764 #define IS_RTC_FORMAT(FORMAT) (((FORMAT) == RTC_FORMAT_BIN) || ((FORMAT) == RTC_FORMAT_BCD)) 00765 00766 #define IS_RTC_YEAR(YEAR) ((YEAR) <= (uint32_t)99) 00767 00768 #define IS_RTC_MONTH(MONTH) (((MONTH) >= (uint32_t)1) && ((MONTH) <= (uint32_t)12)) 00769 00770 #define IS_RTC_DATE(DATE) (((DATE) >= (uint32_t)1) && ((DATE) <= (uint32_t)31)) 00771 00772 #define IS_RTC_WEEKDAY(WEEKDAY) (((WEEKDAY) == RTC_WEEKDAY_MONDAY) || \ 00773 ((WEEKDAY) == RTC_WEEKDAY_TUESDAY) || \ 00774 ((WEEKDAY) == RTC_WEEKDAY_WEDNESDAY) || \ 00775 ((WEEKDAY) == RTC_WEEKDAY_THURSDAY) || \ 00776 ((WEEKDAY) == RTC_WEEKDAY_FRIDAY) || \ 00777 ((WEEKDAY) == RTC_WEEKDAY_SATURDAY) || \ 00778 ((WEEKDAY) == RTC_WEEKDAY_SUNDAY)) 00779 00780 #define IS_RTC_ALARM_DATE_WEEKDAY_DATE(DATE) (((DATE) >(uint32_t) 0) && ((DATE) <= (uint32_t)31)) 00781 00782 #define IS_RTC_ALARM_DATE_WEEKDAY_WEEKDAY(WEEKDAY) (((WEEKDAY) == RTC_WEEKDAY_MONDAY) || \ 00783 ((WEEKDAY) == RTC_WEEKDAY_TUESDAY) || \ 00784 ((WEEKDAY) == RTC_WEEKDAY_WEDNESDAY) || \ 00785 ((WEEKDAY) == RTC_WEEKDAY_THURSDAY) || \ 00786 ((WEEKDAY) == RTC_WEEKDAY_FRIDAY) || \ 00787 ((WEEKDAY) == RTC_WEEKDAY_SATURDAY) || \ 00788 ((WEEKDAY) == RTC_WEEKDAY_SUNDAY)) 00789 00790 #define IS_RTC_ALARM_DATE_WEEKDAY_SEL(SEL) (((SEL) == RTC_ALARMDATEWEEKDAYSEL_DATE) || \ 00791 ((SEL) == RTC_ALARMDATEWEEKDAYSEL_WEEKDAY)) 00792 00793 #define IS_RTC_ALARM_MASK(MASK) (((MASK) & 0x7F7F7F7F) == (uint32_t)RESET) 00794 00795 #define IS_RTC_ALARM(ALARM) (((ALARM) == RTC_ALARM_A) || ((ALARM) == RTC_ALARM_B)) 00796 00797 #define IS_RTC_ALARM_SUB_SECOND_VALUE(VALUE) ((VALUE) <= (uint32_t)0x00007FFF) 00798 00799 #define IS_RTC_ALARM_SUB_SECOND_MASK(MASK) (((MASK) == RTC_ALARMSUBSECONDMASK_ALL) || \ 00800 ((MASK) == RTC_ALARMSUBSECONDMASK_SS14_1) || \ 00801 ((MASK) == RTC_ALARMSUBSECONDMASK_SS14_2) || \ 00802 ((MASK) == RTC_ALARMSUBSECONDMASK_SS14_3) || \ 00803 ((MASK) == RTC_ALARMSUBSECONDMASK_SS14_4) || \ 00804 ((MASK) == RTC_ALARMSUBSECONDMASK_SS14_5) || \ 00805 ((MASK) == RTC_ALARMSUBSECONDMASK_SS14_6) || \ 00806 ((MASK) == RTC_ALARMSUBSECONDMASK_SS14_7) || \ 00807 ((MASK) == RTC_ALARMSUBSECONDMASK_SS14_8) || \ 00808 ((MASK) == RTC_ALARMSUBSECONDMASK_SS14_9) || \ 00809 ((MASK) == RTC_ALARMSUBSECONDMASK_SS14_10) || \ 00810 ((MASK) == RTC_ALARMSUBSECONDMASK_SS14_11) || \ 00811 ((MASK) == RTC_ALARMSUBSECONDMASK_SS14_12) || \ 00812 ((MASK) == RTC_ALARMSUBSECONDMASK_SS14_13) || \ 00813 ((MASK) == RTC_ALARMSUBSECONDMASK_SS14) || \ 00814 ((MASK) == RTC_ALARMSUBSECONDMASK_NONE)) 00815 00816 #define IS_RTC_ASYNCH_PREDIV(PREDIV) ((PREDIV) <= (uint32_t)0x7F) 00817 00818 #define IS_RTC_SYNCH_PREDIV(PREDIV) ((PREDIV) <= (uint32_t)0x7FFF) 00819 00820 #define IS_RTC_HOUR12(HOUR) (((HOUR) > (uint32_t)0) && ((HOUR) <= (uint32_t)12)) 00821 00822 #define IS_RTC_HOUR24(HOUR) ((HOUR) <= (uint32_t)23) 00823 00824 #define IS_RTC_MINUTES(MINUTES) ((MINUTES) <= (uint32_t)59) 00825 00826 #define IS_RTC_SECONDS(SECONDS) ((SECONDS) <= (uint32_t)59) 00827 00828 /** 00829 * @} 00830 */ 00831 00832 /** 00833 * @} 00834 */ 00835 00836 /* Private functions ---------------------------------------------------------*/ 00837 /** @addtogroup RTC_Private_Functions 00838 * @{ 00839 */ 00840 00841 HAL_StatusTypeDef RTC_EnterInitMode(RTC_HandleTypeDef* hrtc); 00842 uint8_t RTC_ByteToBcd2(uint8_t Value); 00843 uint8_t RTC_Bcd2ToByte(uint8_t Value); 00844 00845 /** 00846 * @} 00847 */ 00848 00849 /** 00850 * @} 00851 */ 00852 00853 /** 00854 * @} 00855 */ 00856 00857 #ifdef __cplusplus 00858 } 00859 #endif 00860 00861 #endif /* __STM32L4xx_HAL_RTC_H */ 00862 00863 /************************ (C) COPYRIGHT STMicroelectronics *****END OF FILE****/ 00864
Generated on Tue Jul 12 2022 11:35:15 by
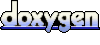