Hal Drivers for L4
Dependents: BSP OneHopeOnePrayer FINAL_AUDIO_RECORD AudioDemo
Fork of STM32L4xx_HAL_Driver by
stm32l4xx_hal_rtc.c
00001 /** 00002 ****************************************************************************** 00003 * @file stm32l4xx_hal_rtc.c 00004 * @author MCD Application Team 00005 * @version V1.1.0 00006 * @date 16-September-2015 00007 * @brief RTC HAL module driver. 00008 * This file provides firmware functions to manage the following 00009 * functionalities of the Real-Time Clock (RTC) peripheral: 00010 * + Initialization 00011 * + Calendar (Time and Date) configuration 00012 * + Alarms (Alarm A and Alarm B) configuration 00013 * + WakeUp Timer configuration 00014 * + TimeStamp configuration 00015 * + Tampers configuration 00016 * + Backup Data Registers configuration 00017 * + RTC Tamper and TimeStamp Pins Selection 00018 * + Interrupts and flags management 00019 * 00020 @verbatim 00021 =============================================================================== 00022 ##### RTC Operating Condition ##### 00023 =============================================================================== 00024 [..] The real-time clock (RTC) and the RTC backup registers can be powered 00025 from the VBAT voltage when the main VDD supply is powered off. 00026 To retain the content of the RTC backup registers and supply the RTC 00027 when VDD is turned off, VBAT pin can be connected to an optional 00028 standby voltage supplied by a battery or by another source. 00029 00030 ##### Backup Domain Reset ##### 00031 =============================================================================== 00032 [..] The backup domain reset sets all RTC registers and the RCC_BDCR register 00033 to their reset values. 00034 A backup domain reset is generated when one of the following events occurs: 00035 (#) Software reset, triggered by setting the BDRST bit in the 00036 RCC Backup domain control register (RCC_BDCR). 00037 (#) VDD or VBAT power on, if both supplies have previously been powered off. 00038 (#) Tamper detection event resets all data backup registers. 00039 00040 ##### Backup Domain Access ##### 00041 =================================================================== 00042 [..] After reset, the backup domain (RTC registers, RTC backup data 00043 registers and backup SRAM) is protected against possible unwanted write 00044 accesses. 00045 00046 [..] To enable access to the RTC Domain and RTC registers, proceed as follows: 00047 (#) Call the function HAL_RCCEx_PeriphCLKConfig with RCC_PERIPHCLK_RTC for 00048 PeriphClockSelection and select RTCClockSelection (LSE, LSI or HSEdiv32) 00049 (#) Enable RTC Clock using the __HAL_RCC_RTC_ENABLE() macro. 00050 00051 ##### How to use RTC Driver ##### 00052 =================================================================== 00053 [..] 00054 (#) Enable the RTC domain access (see description in the section above). 00055 (#) Configure the RTC Prescaler (Asynchronous and Synchronous) and RTC hour 00056 format using the HAL_RTC_Init() function. 00057 00058 *** Time and Date configuration *** 00059 =================================== 00060 [..] 00061 (#) To configure the RTC Calendar (Time and Date) use the HAL_RTC_SetTime() 00062 and HAL_RTC_SetDate() functions. 00063 (#) To read the RTC Calendar, use the HAL_RTC_GetTime() and HAL_RTC_GetDate() functions. 00064 00065 *** Alarm configuration *** 00066 =========================== 00067 [..] 00068 (#) To configure the RTC Alarm use the HAL_RTC_SetAlarm() function. 00069 You can also configure the RTC Alarm with interrupt mode using the 00070 HAL_RTC_SetAlarm_IT() function. 00071 (#) To read the RTC Alarm, use the HAL_RTC_GetAlarm() function. 00072 00073 ##### RTC and low power modes ##### 00074 =================================================================== 00075 [..] The MCU can be woken up from a low power mode by an RTC alternate 00076 function. 00077 [..] The RTC alternate functions are the RTC alarms (Alarm A and Alarm B), 00078 RTC wakeup, RTC tamper event detection and RTC time stamp event detection. 00079 These RTC alternate functions can wake up the system from the Stop and 00080 Standby low power modes. 00081 [..] The system can also wake up from low power modes without depending 00082 on an external interrupt (Auto-wakeup mode), by using the RTC alarm 00083 or the RTC wakeup events. 00084 [..] The RTC provides a programmable time base for waking up from the 00085 Stop or Standby mode at regular intervals. 00086 Wakeup from STOP and Standby modes is possible only when the RTC clock source 00087 is LSE or LSI. 00088 00089 @endverbatim 00090 00091 ****************************************************************************** 00092 * @attention 00093 * 00094 * <h2><center>© COPYRIGHT(c) 2015 STMicroelectronics</center></h2> 00095 * 00096 * Redistribution and use in source and binary forms, with or without modification, 00097 * are permitted provided that the following conditions are met: 00098 * 1. Redistributions of source code must retain the above copyright notice, 00099 * this list of conditions and the following disclaimer. 00100 * 2. Redistributions in binary form must reproduce the above copyright notice, 00101 * this list of conditions and the following disclaimer in the documentation 00102 * and/or other materials provided with the distribution. 00103 * 3. Neither the name of STMicroelectronics nor the names of its contributors 00104 * may be used to endorse or promote products derived from this software 00105 * without specific prior written permission. 00106 * 00107 * THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" 00108 * AND ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE 00109 * IMPLIED WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE 00110 * DISCLAIMED. IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE 00111 * FOR ANY DIRECT, INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL 00112 * DAMAGES (INCLUDING, BUT NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR 00113 * SERVICES; LOSS OF USE, DATA, OR PROFITS; OR BUSINESS INTERRUPTION) HOWEVER 00114 * CAUSED AND ON ANY THEORY OF LIABILITY, WHETHER IN CONTRACT, STRICT LIABILITY, 00115 * OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) ARISING IN ANY WAY OUT OF THE USE 00116 * OF THIS SOFTWARE, EVEN IF ADVISED OF THE POSSIBILITY OF SUCH DAMAGE. 00117 * 00118 ****************************************************************************** 00119 */ 00120 00121 /* Includes ------------------------------------------------------------------*/ 00122 #include "stm32l4xx_hal.h" 00123 00124 /** @addtogroup STM32L4xx_HAL_Driver 00125 * @{ 00126 */ 00127 00128 /** @defgroup RTC RTC 00129 * @brief RTC HAL module driver 00130 * @{ 00131 */ 00132 00133 #ifdef HAL_RTC_MODULE_ENABLED 00134 00135 /* Private typedef -----------------------------------------------------------*/ 00136 /* Private define ------------------------------------------------------------*/ 00137 /* Private macro -------------------------------------------------------------*/ 00138 /* Private variables ---------------------------------------------------------*/ 00139 /* Private function prototypes -----------------------------------------------*/ 00140 /* Exported functions --------------------------------------------------------*/ 00141 00142 /** @defgroup RTC_Exported_Functions RTC Exported Functions 00143 * @{ 00144 */ 00145 00146 /** @defgroup RTC_Exported_Functions_Group1 Initialization and de-initialization functions 00147 * @brief Initialization and Configuration functions 00148 * 00149 @verbatim 00150 =============================================================================== 00151 ##### Initialization and de-initialization functions ##### 00152 =============================================================================== 00153 [..] This section provide functions allowing to initialize and configure the 00154 RTC Prescaler (Synchronous and Asynchronous), RTC Hour format, disable 00155 RTC registers Write protection, enter and exit the RTC initialization mode, 00156 RTC registers synchronization check and reference clock detection enable. 00157 (#) The RTC Prescaler is programmed to generate the RTC 1Hz time base. 00158 It is split into 2 programmable prescalers to minimize power consumption. 00159 (++) A 7-bit asynchronous prescaler and a 15-bit synchronous prescaler. 00160 (++) When both prescalers are used, it is recommended to configure the 00161 asynchronous prescaler to a high value to minimize power consumption. 00162 (#) All RTC registers are Write protected. Writing to the RTC registers 00163 is enabled by writing a key into the Write Protection register, RTC_WPR. 00164 (#) To configure the RTC Calendar, user application should enter 00165 initialization mode. In this mode, the calendar counter is stopped 00166 and its value can be updated. When the initialization sequence is 00167 complete, the calendar restarts counting after 4 RTCCLK cycles. 00168 (#) To read the calendar through the shadow registers after Calendar 00169 initialization, calendar update or after wakeup from low power modes 00170 the software must first clear the RSF flag. The software must then 00171 wait until it is set again before reading the calendar, which means 00172 that the calendar registers have been correctly copied into the 00173 RTC_TR and RTC_DR shadow registers. The HAL_RTC_WaitForSynchro() function 00174 implements the above software sequence (RSF clear and RSF check). 00175 00176 @endverbatim 00177 * @{ 00178 */ 00179 00180 /** 00181 * @brief Initialize the RTC according to the specified parameters 00182 * in the RTC_InitTypeDef structure and initialize the associated handle. 00183 * @param hrtc: RTC handle 00184 * @retval HAL status 00185 */ 00186 HAL_StatusTypeDef HAL_RTC_Init(RTC_HandleTypeDef *hrtc) 00187 { 00188 /* Check the RTC peripheral state */ 00189 if(hrtc == NULL) 00190 { 00191 return HAL_ERROR; 00192 } 00193 00194 /* Check the parameters */ 00195 assert_param(IS_RTC_ALL_INSTANCE(hrtc->Instance)); 00196 assert_param(IS_RTC_HOUR_FORMAT(hrtc->Init.HourFormat)); 00197 assert_param(IS_RTC_ASYNCH_PREDIV(hrtc->Init.AsynchPrediv)); 00198 assert_param(IS_RTC_SYNCH_PREDIV(hrtc->Init.SynchPrediv)); 00199 assert_param(IS_RTC_OUTPUT(hrtc->Init.OutPut)); 00200 assert_param(IS_RTC_OUTPUT_REMAP(hrtc->Init.OutPutRemap)); 00201 assert_param(IS_RTC_OUTPUT_POL(hrtc->Init.OutPutPolarity)); 00202 assert_param(IS_RTC_OUTPUT_TYPE(hrtc->Init.OutPutType)); 00203 00204 if(hrtc->State == HAL_RTC_STATE_RESET) 00205 { 00206 /* Allocate lock resource and initialize it */ 00207 hrtc->Lock = HAL_UNLOCKED; 00208 00209 /* Initialize RTC MSP */ 00210 HAL_RTC_MspInit(hrtc); 00211 } 00212 00213 /* Set RTC state */ 00214 hrtc->State = HAL_RTC_STATE_BUSY; 00215 00216 /* Disable the write protection for RTC registers */ 00217 __HAL_RTC_WRITEPROTECTION_DISABLE(hrtc); 00218 00219 /* Set Initialization mode */ 00220 if(RTC_EnterInitMode(hrtc) != HAL_OK) 00221 { 00222 /* Enable the write protection for RTC registers */ 00223 __HAL_RTC_WRITEPROTECTION_ENABLE(hrtc); 00224 00225 /* Set RTC state */ 00226 hrtc->State = HAL_RTC_STATE_ERROR; 00227 00228 return HAL_ERROR; 00229 } 00230 else 00231 { 00232 /* Clear RTC_CR FMT, OSEL and POL Bits */ 00233 hrtc->Instance->CR &= ((uint32_t)~(RTC_CR_FMT | RTC_CR_OSEL | RTC_CR_POL)); 00234 /* Set RTC_CR register */ 00235 hrtc->Instance->CR |= (uint32_t)(hrtc->Init.HourFormat | hrtc->Init.OutPut | hrtc->Init.OutPutPolarity); 00236 00237 /* Configure the RTC PRER */ 00238 hrtc->Instance->PRER = (uint32_t)(hrtc->Init.SynchPrediv); 00239 hrtc->Instance->PRER |= (uint32_t)(hrtc->Init.AsynchPrediv << 16); 00240 00241 /* Exit Initialization mode */ 00242 hrtc->Instance->ISR &= ((uint32_t)~RTC_ISR_INIT); 00243 00244 hrtc->Instance->OR &= (uint32_t)~(RTC_OR_ALARMOUTTYPE | RTC_OR_OUT_RMP); 00245 hrtc->Instance->OR |= (uint32_t)(hrtc->Init.OutPutType | hrtc->Init.OutPutRemap); 00246 00247 /* Enable the write protection for RTC registers */ 00248 __HAL_RTC_WRITEPROTECTION_ENABLE(hrtc); 00249 00250 /* Set RTC state */ 00251 hrtc->State = HAL_RTC_STATE_READY; 00252 00253 return HAL_OK; 00254 } 00255 } 00256 00257 /** 00258 * @brief DeInitialize the RTC peripheral. 00259 * @param hrtc: RTC handle 00260 * @note This function doesn't reset the RTC Backup Data registers. 00261 * @retval HAL status 00262 */ 00263 HAL_StatusTypeDef HAL_RTC_DeInit(RTC_HandleTypeDef *hrtc) 00264 { 00265 uint32_t tickstart = 0; 00266 00267 /* Check the parameters */ 00268 assert_param(IS_RTC_ALL_INSTANCE(hrtc->Instance)); 00269 00270 /* Set RTC state */ 00271 hrtc->State = HAL_RTC_STATE_BUSY; 00272 00273 /* Disable the write protection for RTC registers */ 00274 __HAL_RTC_WRITEPROTECTION_DISABLE(hrtc); 00275 00276 /* Set Initialization mode */ 00277 if(RTC_EnterInitMode(hrtc) != HAL_OK) 00278 { 00279 /* Enable the write protection for RTC registers */ 00280 __HAL_RTC_WRITEPROTECTION_ENABLE(hrtc); 00281 00282 /* Set RTC state */ 00283 hrtc->State = HAL_RTC_STATE_ERROR; 00284 00285 return HAL_ERROR; 00286 } 00287 else 00288 { 00289 /* Reset TR, DR and CR registers */ 00290 hrtc->Instance->TR = (uint32_t)0x00000000; 00291 hrtc->Instance->DR = ((uint32_t)(RTC_DR_WDU_0 | RTC_DR_MU_0 | RTC_DR_DU_0)); 00292 /* Reset All CR bits except CR[2:0] */ 00293 hrtc->Instance->CR &= RTC_CR_WUCKSEL; 00294 00295 tickstart = HAL_GetTick(); 00296 00297 /* Wait till WUTWF flag is set and if Time out is reached exit */ 00298 while(((hrtc->Instance->ISR) & RTC_ISR_WUTWF) == (uint32_t)RESET) 00299 { 00300 if((HAL_GetTick() - tickstart ) > RTC_TIMEOUT_VALUE) 00301 { 00302 /* Enable the write protection for RTC registers */ 00303 __HAL_RTC_WRITEPROTECTION_ENABLE(hrtc); 00304 00305 /* Set RTC state */ 00306 hrtc->State = HAL_RTC_STATE_TIMEOUT; 00307 00308 return HAL_TIMEOUT; 00309 } 00310 } 00311 00312 /* Reset all RTC CR register bits */ 00313 hrtc->Instance->CR &= (uint32_t)0x00000000; 00314 hrtc->Instance->WUTR = RTC_WUTR_WUT; 00315 hrtc->Instance->PRER = ((uint32_t)(RTC_PRER_PREDIV_A | 0x000000FF)); 00316 hrtc->Instance->ALRMAR = (uint32_t)0x00000000; 00317 hrtc->Instance->ALRMBR = (uint32_t)0x00000000; 00318 hrtc->Instance->SHIFTR = (uint32_t)0x00000000; 00319 hrtc->Instance->CALR = (uint32_t)0x00000000; 00320 hrtc->Instance->ALRMASSR = (uint32_t)0x00000000; 00321 hrtc->Instance->ALRMBSSR = (uint32_t)0x00000000; 00322 00323 /* Reset ISR register and exit initialization mode */ 00324 hrtc->Instance->ISR = (uint32_t)0x00000000; 00325 00326 /* Reset Tamper configuration register */ 00327 hrtc->Instance->TAMPCR = 0x00000000; 00328 00329 /* Reset Option register */ 00330 hrtc->Instance->OR = 0x00000000; 00331 00332 /* If RTC_CR_BYPSHAD bit = 0, wait for synchro else this check is not needed */ 00333 if((hrtc->Instance->CR & RTC_CR_BYPSHAD) == RESET) 00334 { 00335 if(HAL_RTC_WaitForSynchro(hrtc) != HAL_OK) 00336 { 00337 /* Enable the write protection for RTC registers */ 00338 __HAL_RTC_WRITEPROTECTION_ENABLE(hrtc); 00339 00340 hrtc->State = HAL_RTC_STATE_ERROR; 00341 00342 return HAL_ERROR; 00343 } 00344 } 00345 } 00346 00347 /* Enable the write protection for RTC registers */ 00348 __HAL_RTC_WRITEPROTECTION_ENABLE(hrtc); 00349 00350 /* De-Initialize RTC MSP */ 00351 HAL_RTC_MspDeInit(hrtc); 00352 00353 hrtc->State = HAL_RTC_STATE_RESET; 00354 00355 /* Release Lock */ 00356 __HAL_UNLOCK(hrtc); 00357 00358 return HAL_OK; 00359 } 00360 00361 /** 00362 * @brief Initialize the RTC MSP. 00363 * @param hrtc: RTC handle 00364 * @retval None 00365 */ 00366 __weak void HAL_RTC_MspInit(RTC_HandleTypeDef* hrtc) 00367 { 00368 /* NOTE : This function should not be modified, when the callback is needed, 00369 the HAL_RTC_MspInit could be implemented in the user file 00370 */ 00371 } 00372 00373 /** 00374 * @brief DeInitialize the RTC MSP. 00375 * @param hrtc: RTC handle 00376 * @retval None 00377 */ 00378 __weak void HAL_RTC_MspDeInit(RTC_HandleTypeDef* hrtc) 00379 { 00380 /* NOTE : This function should not be modified, when the callback is needed, 00381 the HAL_RTC_MspDeInit could be implemented in the user file 00382 */ 00383 } 00384 00385 /** 00386 * @} 00387 */ 00388 00389 /** @defgroup RTC_Exported_Functions_Group2 RTC Time and Date functions 00390 * @brief RTC Time and Date functions 00391 * 00392 @verbatim 00393 =============================================================================== 00394 ##### RTC Time and Date functions ##### 00395 =============================================================================== 00396 00397 [..] This section provides functions allowing to configure Time and Date features 00398 00399 @endverbatim 00400 * @{ 00401 */ 00402 00403 /** 00404 * @brief Set RTC current time. 00405 * @param hrtc: RTC handle 00406 * @param sTime: Pointer to Time structure 00407 * @param Format: Specifies the format of the entered parameters. 00408 * This parameter can be one of the following values: 00409 * @arg RTC_FORMAT_BIN: Binary data format 00410 * @arg RTC_FORMAT_BCD: BCD data format 00411 * @retval HAL status 00412 */ 00413 HAL_StatusTypeDef HAL_RTC_SetTime(RTC_HandleTypeDef *hrtc, RTC_TimeTypeDef *sTime, uint32_t Format) 00414 { 00415 uint32_t tmpreg = 0; 00416 00417 /* Check the parameters */ 00418 assert_param(IS_RTC_FORMAT(Format)); 00419 assert_param(IS_RTC_DAYLIGHT_SAVING(sTime->DayLightSaving)); 00420 assert_param(IS_RTC_STORE_OPERATION(sTime->StoreOperation)); 00421 00422 /* Process Locked */ 00423 __HAL_LOCK(hrtc); 00424 00425 hrtc->State = HAL_RTC_STATE_BUSY; 00426 00427 if(Format == RTC_FORMAT_BIN) 00428 { 00429 if((hrtc->Instance->CR & RTC_CR_FMT) != (uint32_t)RESET) 00430 { 00431 assert_param(IS_RTC_HOUR12(sTime->Hours)); 00432 assert_param(IS_RTC_HOURFORMAT12(sTime->TimeFormat)); 00433 } 00434 else 00435 { 00436 sTime->TimeFormat = 0x00; 00437 assert_param(IS_RTC_HOUR24(sTime->Hours)); 00438 } 00439 assert_param(IS_RTC_MINUTES(sTime->Minutes)); 00440 assert_param(IS_RTC_SECONDS(sTime->Seconds)); 00441 00442 tmpreg = (uint32_t)(((uint32_t)RTC_ByteToBcd2(sTime->Hours) << 16) | \ 00443 ((uint32_t)RTC_ByteToBcd2(sTime->Minutes) << 8) | \ 00444 ((uint32_t)RTC_ByteToBcd2(sTime->Seconds)) | \ 00445 (((uint32_t)sTime->TimeFormat) << 16)); 00446 } 00447 else 00448 { 00449 if((hrtc->Instance->CR & RTC_CR_FMT) != (uint32_t)RESET) 00450 { 00451 tmpreg = RTC_Bcd2ToByte(sTime->Hours); 00452 assert_param(IS_RTC_HOUR12(tmpreg)); 00453 assert_param(IS_RTC_HOURFORMAT12(sTime->TimeFormat)); 00454 } 00455 else 00456 { 00457 sTime->TimeFormat = 0x00; 00458 assert_param(IS_RTC_HOUR24(RTC_Bcd2ToByte(sTime->Hours))); 00459 } 00460 assert_param(IS_RTC_MINUTES(RTC_Bcd2ToByte(sTime->Minutes))); 00461 assert_param(IS_RTC_SECONDS(RTC_Bcd2ToByte(sTime->Seconds))); 00462 tmpreg = (((uint32_t)(sTime->Hours) << 16) | \ 00463 ((uint32_t)(sTime->Minutes) << 8) | \ 00464 ((uint32_t)sTime->Seconds) | \ 00465 ((uint32_t)(sTime->TimeFormat) << 16)); 00466 } 00467 00468 /* Disable the write protection for RTC registers */ 00469 __HAL_RTC_WRITEPROTECTION_DISABLE(hrtc); 00470 00471 /* Set Initialization mode */ 00472 if(RTC_EnterInitMode(hrtc) != HAL_OK) 00473 { 00474 /* Enable the write protection for RTC registers */ 00475 __HAL_RTC_WRITEPROTECTION_ENABLE(hrtc); 00476 00477 /* Set RTC state */ 00478 hrtc->State = HAL_RTC_STATE_ERROR; 00479 00480 /* Process Unlocked */ 00481 __HAL_UNLOCK(hrtc); 00482 00483 return HAL_ERROR; 00484 } 00485 else 00486 { 00487 /* Set the RTC_TR register */ 00488 hrtc->Instance->TR = (uint32_t)(tmpreg & RTC_TR_RESERVED_MASK); 00489 00490 /* Clear the bits to be configured */ 00491 hrtc->Instance->CR &= ((uint32_t)~RTC_CR_BCK); 00492 00493 /* Configure the RTC_CR register */ 00494 hrtc->Instance->CR |= (uint32_t)(sTime->DayLightSaving | sTime->StoreOperation); 00495 00496 /* Exit Initialization mode */ 00497 hrtc->Instance->ISR &= ((uint32_t)~RTC_ISR_INIT); 00498 00499 /* If CR_BYPSHAD bit = 0, wait for synchro else this check is not needed */ 00500 if((hrtc->Instance->CR & RTC_CR_BYPSHAD) == RESET) 00501 { 00502 if(HAL_RTC_WaitForSynchro(hrtc) != HAL_OK) 00503 { 00504 /* Enable the write protection for RTC registers */ 00505 __HAL_RTC_WRITEPROTECTION_ENABLE(hrtc); 00506 00507 hrtc->State = HAL_RTC_STATE_ERROR; 00508 00509 /* Process Unlocked */ 00510 __HAL_UNLOCK(hrtc); 00511 00512 return HAL_ERROR; 00513 } 00514 } 00515 00516 /* Enable the write protection for RTC registers */ 00517 __HAL_RTC_WRITEPROTECTION_ENABLE(hrtc); 00518 00519 hrtc->State = HAL_RTC_STATE_READY; 00520 00521 __HAL_UNLOCK(hrtc); 00522 00523 return HAL_OK; 00524 } 00525 } 00526 00527 /** 00528 * @brief Get RTC current time. 00529 * @param hrtc: RTC handle 00530 * @param sTime: Pointer to Time structure with Hours, Minutes and Seconds fields returned 00531 * with input format (BIN or BCD), also SubSeconds field returning the 00532 * RTC_SSR register content and SecondFraction field the Synchronous pre-scaler 00533 * factor to be used for second fraction ratio computation. 00534 * @param Format: Specifies the format of the entered parameters. 00535 * This parameter can be one of the following values: 00536 * @arg RTC_FORMAT_BIN: Binary data format 00537 * @arg RTC_FORMAT_BCD: BCD data format 00538 * @note You can use SubSeconds and SecondFraction (sTime structure fields returned) to convert SubSeconds 00539 * value in second fraction ratio with time unit following generic formula: 00540 * Second fraction ratio * time_unit= [(SecondFraction-SubSeconds)/(SecondFraction+1)] * time_unit 00541 * This conversion can be performed only if no shift operation is pending (ie. SHFP=0) when PREDIV_S >= SS 00542 * @note You must call HAL_RTC_GetDate() after HAL_RTC_GetTime() to unlock the values 00543 * in the higher-order calendar shadow registers to ensure consistency between the time and date values. 00544 * Reading RTC current time locks the values in calendar shadow registers until Current date is read 00545 * to ensure consistency between the time and date values. 00546 * @retval HAL status 00547 */ 00548 HAL_StatusTypeDef HAL_RTC_GetTime(RTC_HandleTypeDef *hrtc, RTC_TimeTypeDef *sTime, uint32_t Format) 00549 { 00550 uint32_t tmpreg = 0; 00551 00552 /* Check the parameters */ 00553 assert_param(IS_RTC_FORMAT(Format)); 00554 00555 /* Get subseconds structure field from the corresponding register*/ 00556 sTime->SubSeconds = (uint32_t)(hrtc->Instance->SSR); 00557 00558 /* Get SecondFraction structure field from the corresponding register field*/ 00559 sTime->SecondFraction = (uint32_t)(hrtc->Instance->PRER & RTC_PRER_PREDIV_S); 00560 00561 /* Get the TR register */ 00562 tmpreg = (uint32_t)(hrtc->Instance->TR & RTC_TR_RESERVED_MASK); 00563 00564 /* Fill the structure fields with the read parameters */ 00565 sTime->Hours = (uint8_t)((tmpreg & (RTC_TR_HT | RTC_TR_HU)) >> 16); 00566 sTime->Minutes = (uint8_t)((tmpreg & (RTC_TR_MNT | RTC_TR_MNU)) >>8); 00567 sTime->Seconds = (uint8_t)(tmpreg & (RTC_TR_ST | RTC_TR_SU)); 00568 sTime->TimeFormat = (uint8_t)((tmpreg & (RTC_TR_PM)) >> 16); 00569 00570 /* Check the input parameters format */ 00571 if(Format == RTC_FORMAT_BIN) 00572 { 00573 /* Convert the time structure parameters to Binary format */ 00574 sTime->Hours = (uint8_t)RTC_Bcd2ToByte(sTime->Hours); 00575 sTime->Minutes = (uint8_t)RTC_Bcd2ToByte(sTime->Minutes); 00576 sTime->Seconds = (uint8_t)RTC_Bcd2ToByte(sTime->Seconds); 00577 } 00578 00579 return HAL_OK; 00580 } 00581 00582 /** 00583 * @brief Set RTC current date. 00584 * @param hrtc: RTC handle 00585 * @param sDate: Pointer to date structure 00586 * @param Format: specifies the format of the entered parameters. 00587 * This parameter can be one of the following values: 00588 * @arg RTC_FORMAT_BIN: Binary data format 00589 * @arg RTC_FORMAT_BCD: BCD data format 00590 * @retval HAL status 00591 */ 00592 HAL_StatusTypeDef HAL_RTC_SetDate(RTC_HandleTypeDef *hrtc, RTC_DateTypeDef *sDate, uint32_t Format) 00593 { 00594 uint32_t datetmpreg = 0; 00595 00596 /* Check the parameters */ 00597 assert_param(IS_RTC_FORMAT(Format)); 00598 00599 /* Process Locked */ 00600 __HAL_LOCK(hrtc); 00601 00602 hrtc->State = HAL_RTC_STATE_BUSY; 00603 00604 if((Format == RTC_FORMAT_BIN) && ((sDate->Month & 0x10) == 0x10)) 00605 { 00606 sDate->Month = (uint8_t)((sDate->Month & (uint8_t)~(0x10)) + (uint8_t)0x0A); 00607 } 00608 00609 assert_param(IS_RTC_WEEKDAY(sDate->WeekDay)); 00610 00611 if(Format == RTC_FORMAT_BIN) 00612 { 00613 assert_param(IS_RTC_YEAR(sDate->Year)); 00614 assert_param(IS_RTC_MONTH(sDate->Month)); 00615 assert_param(IS_RTC_DATE(sDate->Date)); 00616 00617 datetmpreg = (((uint32_t)RTC_ByteToBcd2(sDate->Year) << 16) | \ 00618 ((uint32_t)RTC_ByteToBcd2(sDate->Month) << 8) | \ 00619 ((uint32_t)RTC_ByteToBcd2(sDate->Date)) | \ 00620 ((uint32_t)sDate->WeekDay << 13)); 00621 } 00622 else 00623 { 00624 assert_param(IS_RTC_YEAR(RTC_Bcd2ToByte(sDate->Year))); 00625 datetmpreg = RTC_Bcd2ToByte(sDate->Month); 00626 assert_param(IS_RTC_MONTH(datetmpreg)); 00627 datetmpreg = RTC_Bcd2ToByte(sDate->Date); 00628 assert_param(IS_RTC_DATE(datetmpreg)); 00629 00630 datetmpreg = ((((uint32_t)sDate->Year) << 16) | \ 00631 (((uint32_t)sDate->Month) << 8) | \ 00632 ((uint32_t)sDate->Date) | \ 00633 (((uint32_t)sDate->WeekDay) << 13)); 00634 } 00635 00636 /* Disable the write protection for RTC registers */ 00637 __HAL_RTC_WRITEPROTECTION_DISABLE(hrtc); 00638 00639 /* Set Initialization mode */ 00640 if(RTC_EnterInitMode(hrtc) != HAL_OK) 00641 { 00642 /* Enable the write protection for RTC registers */ 00643 __HAL_RTC_WRITEPROTECTION_ENABLE(hrtc); 00644 00645 /* Set RTC state*/ 00646 hrtc->State = HAL_RTC_STATE_ERROR; 00647 00648 /* Process Unlocked */ 00649 __HAL_UNLOCK(hrtc); 00650 00651 return HAL_ERROR; 00652 } 00653 else 00654 { 00655 /* Set the RTC_DR register */ 00656 hrtc->Instance->DR = (uint32_t)(datetmpreg & RTC_DR_RESERVED_MASK); 00657 00658 /* Exit Initialization mode */ 00659 hrtc->Instance->ISR &= ((uint32_t)~RTC_ISR_INIT); 00660 00661 /* If CR_BYPSHAD bit = 0, wait for synchro else this check is not needed */ 00662 if((hrtc->Instance->CR & RTC_CR_BYPSHAD) == RESET) 00663 { 00664 if(HAL_RTC_WaitForSynchro(hrtc) != HAL_OK) 00665 { 00666 /* Enable the write protection for RTC registers */ 00667 __HAL_RTC_WRITEPROTECTION_ENABLE(hrtc); 00668 00669 hrtc->State = HAL_RTC_STATE_ERROR; 00670 00671 /* Process Unlocked */ 00672 __HAL_UNLOCK(hrtc); 00673 00674 return HAL_ERROR; 00675 } 00676 } 00677 00678 /* Enable the write protection for RTC registers */ 00679 __HAL_RTC_WRITEPROTECTION_ENABLE(hrtc); 00680 00681 hrtc->State = HAL_RTC_STATE_READY ; 00682 00683 /* Process Unlocked */ 00684 __HAL_UNLOCK(hrtc); 00685 00686 return HAL_OK; 00687 } 00688 } 00689 00690 /** 00691 * @brief Get RTC current date. 00692 * @param hrtc: RTC handle 00693 * @param sDate: Pointer to Date structure 00694 * @param Format: Specifies the format of the entered parameters. 00695 * This parameter can be one of the following values: 00696 * @arg RTC_FORMAT_BIN: Binary data format 00697 * @arg RTC_FORMAT_BCD: BCD data format 00698 * @note You must call HAL_RTC_GetDate() after HAL_RTC_GetTime() to unlock the values 00699 * in the higher-order calendar shadow registers to ensure consistency between the time and date values. 00700 * Reading RTC current time locks the values in calendar shadow registers until Current date is read. 00701 * @retval HAL status 00702 */ 00703 HAL_StatusTypeDef HAL_RTC_GetDate(RTC_HandleTypeDef *hrtc, RTC_DateTypeDef *sDate, uint32_t Format) 00704 { 00705 uint32_t datetmpreg = 0; 00706 00707 /* Check the parameters */ 00708 assert_param(IS_RTC_FORMAT(Format)); 00709 00710 /* Get the DR register */ 00711 datetmpreg = (uint32_t)(hrtc->Instance->DR & RTC_DR_RESERVED_MASK); 00712 00713 /* Fill the structure fields with the read parameters */ 00714 sDate->Year = (uint8_t)((datetmpreg & (RTC_DR_YT | RTC_DR_YU)) >> 16); 00715 sDate->Month = (uint8_t)((datetmpreg & (RTC_DR_MT | RTC_DR_MU)) >> 8); 00716 sDate->Date = (uint8_t)(datetmpreg & (RTC_DR_DT | RTC_DR_DU)); 00717 sDate->WeekDay = (uint8_t)((datetmpreg & (RTC_DR_WDU)) >> 13); 00718 00719 /* Check the input parameters format */ 00720 if(Format == RTC_FORMAT_BIN) 00721 { 00722 /* Convert the date structure parameters to Binary format */ 00723 sDate->Year = (uint8_t)RTC_Bcd2ToByte(sDate->Year); 00724 sDate->Month = (uint8_t)RTC_Bcd2ToByte(sDate->Month); 00725 sDate->Date = (uint8_t)RTC_Bcd2ToByte(sDate->Date); 00726 } 00727 return HAL_OK; 00728 } 00729 00730 /** 00731 * @} 00732 */ 00733 00734 /** @defgroup RTC_Exported_Functions_Group3 RTC Alarm functions 00735 * @brief RTC Alarm functions 00736 * 00737 @verbatim 00738 =============================================================================== 00739 ##### RTC Alarm functions ##### 00740 =============================================================================== 00741 00742 [..] This section provides functions allowing to configure Alarm feature 00743 00744 @endverbatim 00745 * @{ 00746 */ 00747 /** 00748 * @brief Set the specified RTC Alarm. 00749 * @param hrtc: RTC handle 00750 * @param sAlarm: Pointer to Alarm structure 00751 * @param Format: Specifies the format of the entered parameters. 00752 * This parameter can be one of the following values: 00753 * @arg RTC_FORMAT_BIN: Binary data format 00754 * @arg RTC_FORMAT_BCD: BCD data format 00755 * @retval HAL status 00756 */ 00757 HAL_StatusTypeDef HAL_RTC_SetAlarm(RTC_HandleTypeDef *hrtc, RTC_AlarmTypeDef *sAlarm, uint32_t Format) 00758 { 00759 uint32_t tickstart = 0; 00760 uint32_t tmpreg = 0, subsecondtmpreg = 0; 00761 00762 /* Check the parameters */ 00763 assert_param(IS_RTC_FORMAT(Format)); 00764 assert_param(IS_RTC_ALARM(sAlarm->Alarm)); 00765 assert_param(IS_RTC_ALARM_MASK(sAlarm->AlarmMask)); 00766 assert_param(IS_RTC_ALARM_DATE_WEEKDAY_SEL(sAlarm->AlarmDateWeekDaySel)); 00767 assert_param(IS_RTC_ALARM_SUB_SECOND_VALUE(sAlarm->AlarmTime.SubSeconds)); 00768 assert_param(IS_RTC_ALARM_SUB_SECOND_MASK(sAlarm->AlarmSubSecondMask)); 00769 00770 /* Process Locked */ 00771 __HAL_LOCK(hrtc); 00772 00773 hrtc->State = HAL_RTC_STATE_BUSY; 00774 00775 if(Format == RTC_FORMAT_BIN) 00776 { 00777 if((hrtc->Instance->CR & RTC_CR_FMT) != (uint32_t)RESET) 00778 { 00779 assert_param(IS_RTC_HOUR12(sAlarm->AlarmTime.Hours)); 00780 assert_param(IS_RTC_HOURFORMAT12(sAlarm->AlarmTime.TimeFormat)); 00781 } 00782 else 00783 { 00784 sAlarm->AlarmTime.TimeFormat = 0x00; 00785 assert_param(IS_RTC_HOUR24(sAlarm->AlarmTime.Hours)); 00786 } 00787 assert_param(IS_RTC_MINUTES(sAlarm->AlarmTime.Minutes)); 00788 assert_param(IS_RTC_SECONDS(sAlarm->AlarmTime.Seconds)); 00789 00790 if(sAlarm->AlarmDateWeekDaySel == RTC_ALARMDATEWEEKDAYSEL_DATE) 00791 { 00792 assert_param(IS_RTC_ALARM_DATE_WEEKDAY_DATE(sAlarm->AlarmDateWeekDay)); 00793 } 00794 else 00795 { 00796 assert_param(IS_RTC_ALARM_DATE_WEEKDAY_WEEKDAY(sAlarm->AlarmDateWeekDay)); 00797 } 00798 00799 tmpreg = (((uint32_t)RTC_ByteToBcd2(sAlarm->AlarmTime.Hours) << 16) | \ 00800 ((uint32_t)RTC_ByteToBcd2(sAlarm->AlarmTime.Minutes) << 8) | \ 00801 ((uint32_t)RTC_ByteToBcd2(sAlarm->AlarmTime.Seconds)) | \ 00802 ((uint32_t)(sAlarm->AlarmTime.TimeFormat) << 16) | \ 00803 ((uint32_t)RTC_ByteToBcd2(sAlarm->AlarmDateWeekDay) << 24) | \ 00804 ((uint32_t)sAlarm->AlarmDateWeekDaySel) | \ 00805 ((uint32_t)sAlarm->AlarmMask)); 00806 } 00807 else 00808 { 00809 if((hrtc->Instance->CR & RTC_CR_FMT) != (uint32_t)RESET) 00810 { 00811 tmpreg = RTC_Bcd2ToByte(sAlarm->AlarmTime.Hours); 00812 assert_param(IS_RTC_HOUR12(tmpreg)); 00813 assert_param(IS_RTC_HOURFORMAT12(sAlarm->AlarmTime.TimeFormat)); 00814 } 00815 else 00816 { 00817 sAlarm->AlarmTime.TimeFormat = 0x00; 00818 assert_param(IS_RTC_HOUR24(RTC_Bcd2ToByte(sAlarm->AlarmTime.Hours))); 00819 } 00820 00821 assert_param(IS_RTC_MINUTES(RTC_Bcd2ToByte(sAlarm->AlarmTime.Minutes))); 00822 assert_param(IS_RTC_SECONDS(RTC_Bcd2ToByte(sAlarm->AlarmTime.Seconds))); 00823 00824 if(sAlarm->AlarmDateWeekDaySel == RTC_ALARMDATEWEEKDAYSEL_DATE) 00825 { 00826 tmpreg = RTC_Bcd2ToByte(sAlarm->AlarmDateWeekDay); 00827 assert_param(IS_RTC_ALARM_DATE_WEEKDAY_DATE(tmpreg)); 00828 } 00829 else 00830 { 00831 tmpreg = RTC_Bcd2ToByte(sAlarm->AlarmDateWeekDay); 00832 assert_param(IS_RTC_ALARM_DATE_WEEKDAY_WEEKDAY(tmpreg)); 00833 } 00834 00835 tmpreg = (((uint32_t)(sAlarm->AlarmTime.Hours) << 16) | \ 00836 ((uint32_t)(sAlarm->AlarmTime.Minutes) << 8) | \ 00837 ((uint32_t) sAlarm->AlarmTime.Seconds) | \ 00838 ((uint32_t)(sAlarm->AlarmTime.TimeFormat) << 16) | \ 00839 ((uint32_t)(sAlarm->AlarmDateWeekDay) << 24) | \ 00840 ((uint32_t)sAlarm->AlarmDateWeekDaySel) | \ 00841 ((uint32_t)sAlarm->AlarmMask)); 00842 } 00843 00844 /* Configure the Alarm A or Alarm B Sub Second registers */ 00845 subsecondtmpreg = (uint32_t)((uint32_t)(sAlarm->AlarmTime.SubSeconds) | (uint32_t)(sAlarm->AlarmSubSecondMask)); 00846 00847 /* Disable the write protection for RTC registers */ 00848 __HAL_RTC_WRITEPROTECTION_DISABLE(hrtc); 00849 00850 /* Configure the Alarm register */ 00851 if(sAlarm->Alarm == RTC_ALARM_A) 00852 { 00853 /* Disable the Alarm A interrupt */ 00854 __HAL_RTC_ALARMA_DISABLE(hrtc); 00855 00856 /* In case of interrupt mode is used, the interrupt source must disabled */ 00857 __HAL_RTC_ALARM_DISABLE_IT(hrtc, RTC_IT_ALRA); 00858 00859 tickstart = HAL_GetTick(); 00860 /* Wait till RTC ALRAWF flag is set and if Time out is reached exit */ 00861 while(__HAL_RTC_ALARM_GET_FLAG(hrtc, RTC_FLAG_ALRAWF) == RESET) 00862 { 00863 if((HAL_GetTick() - tickstart ) > RTC_TIMEOUT_VALUE) 00864 { 00865 /* Enable the write protection for RTC registers */ 00866 __HAL_RTC_WRITEPROTECTION_ENABLE(hrtc); 00867 00868 hrtc->State = HAL_RTC_STATE_TIMEOUT; 00869 00870 /* Process Unlocked */ 00871 __HAL_UNLOCK(hrtc); 00872 00873 return HAL_TIMEOUT; 00874 } 00875 } 00876 00877 hrtc->Instance->ALRMAR = (uint32_t)tmpreg; 00878 /* Configure the Alarm A Sub Second register */ 00879 hrtc->Instance->ALRMASSR = subsecondtmpreg; 00880 /* Configure the Alarm state: Enable Alarm */ 00881 __HAL_RTC_ALARMA_ENABLE(hrtc); 00882 } 00883 else 00884 { 00885 /* Disable the Alarm B interrupt */ 00886 __HAL_RTC_ALARMB_DISABLE(hrtc); 00887 00888 /* In case of interrupt mode is used, the interrupt source must disabled */ 00889 __HAL_RTC_ALARM_DISABLE_IT(hrtc, RTC_IT_ALRB); 00890 00891 tickstart = HAL_GetTick(); 00892 /* Wait till RTC ALRBWF flag is set and if Time out is reached exit */ 00893 while(__HAL_RTC_ALARM_GET_FLAG(hrtc, RTC_FLAG_ALRBWF) == RESET) 00894 { 00895 if((HAL_GetTick() - tickstart ) > RTC_TIMEOUT_VALUE) 00896 { 00897 /* Enable the write protection for RTC registers */ 00898 __HAL_RTC_WRITEPROTECTION_ENABLE(hrtc); 00899 00900 hrtc->State = HAL_RTC_STATE_TIMEOUT; 00901 00902 /* Process Unlocked */ 00903 __HAL_UNLOCK(hrtc); 00904 00905 return HAL_TIMEOUT; 00906 } 00907 } 00908 00909 hrtc->Instance->ALRMBR = (uint32_t)tmpreg; 00910 /* Configure the Alarm B Sub Second register */ 00911 hrtc->Instance->ALRMBSSR = subsecondtmpreg; 00912 /* Configure the Alarm state: Enable Alarm */ 00913 __HAL_RTC_ALARMB_ENABLE(hrtc); 00914 } 00915 00916 /* Enable the write protection for RTC registers */ 00917 __HAL_RTC_WRITEPROTECTION_ENABLE(hrtc); 00918 00919 /* Change RTC state */ 00920 hrtc->State = HAL_RTC_STATE_READY; 00921 00922 /* Process Unlocked */ 00923 __HAL_UNLOCK(hrtc); 00924 00925 return HAL_OK; 00926 } 00927 00928 /** 00929 * @brief Set the specified RTC Alarm with Interrupt. 00930 * @param hrtc: RTC handle 00931 * @param sAlarm: Pointer to Alarm structure 00932 * @param Format: Specifies the format of the entered parameters. 00933 * This parameter can be one of the following values: 00934 * @arg RTC_FORMAT_BIN: Binary data format 00935 * @arg RTC_FORMAT_BCD: BCD data format 00936 * @note The Alarm register can only be written when the corresponding Alarm 00937 * is disabled (Use the HAL_RTC_DeactivateAlarm()). 00938 * @note The HAL_RTC_SetTime() must be called before enabling the Alarm feature. 00939 * @retval HAL status 00940 */ 00941 HAL_StatusTypeDef HAL_RTC_SetAlarm_IT(RTC_HandleTypeDef *hrtc, RTC_AlarmTypeDef *sAlarm, uint32_t Format) 00942 { 00943 uint32_t tickstart = 0; 00944 uint32_t tmpreg = 0, subsecondtmpreg = 0; 00945 00946 /* Check the parameters */ 00947 assert_param(IS_RTC_FORMAT(Format)); 00948 assert_param(IS_RTC_ALARM(sAlarm->Alarm)); 00949 assert_param(IS_RTC_ALARM_MASK(sAlarm->AlarmMask)); 00950 assert_param(IS_RTC_ALARM_DATE_WEEKDAY_SEL(sAlarm->AlarmDateWeekDaySel)); 00951 assert_param(IS_RTC_ALARM_SUB_SECOND_VALUE(sAlarm->AlarmTime.SubSeconds)); 00952 assert_param(IS_RTC_ALARM_SUB_SECOND_MASK(sAlarm->AlarmSubSecondMask)); 00953 00954 /* Process Locked */ 00955 __HAL_LOCK(hrtc); 00956 00957 hrtc->State = HAL_RTC_STATE_BUSY; 00958 00959 if(Format == RTC_FORMAT_BIN) 00960 { 00961 if((hrtc->Instance->CR & RTC_CR_FMT) != (uint32_t)RESET) 00962 { 00963 assert_param(IS_RTC_HOUR12(sAlarm->AlarmTime.Hours)); 00964 assert_param(IS_RTC_HOURFORMAT12(sAlarm->AlarmTime.TimeFormat)); 00965 } 00966 else 00967 { 00968 sAlarm->AlarmTime.TimeFormat = 0x00; 00969 assert_param(IS_RTC_HOUR24(sAlarm->AlarmTime.Hours)); 00970 } 00971 assert_param(IS_RTC_MINUTES(sAlarm->AlarmTime.Minutes)); 00972 assert_param(IS_RTC_SECONDS(sAlarm->AlarmTime.Seconds)); 00973 00974 if(sAlarm->AlarmDateWeekDaySel == RTC_ALARMDATEWEEKDAYSEL_DATE) 00975 { 00976 assert_param(IS_RTC_ALARM_DATE_WEEKDAY_DATE(sAlarm->AlarmDateWeekDay)); 00977 } 00978 else 00979 { 00980 assert_param(IS_RTC_ALARM_DATE_WEEKDAY_WEEKDAY(sAlarm->AlarmDateWeekDay)); 00981 } 00982 tmpreg = (((uint32_t)RTC_ByteToBcd2(sAlarm->AlarmTime.Hours) << 16) | \ 00983 ((uint32_t)RTC_ByteToBcd2(sAlarm->AlarmTime.Minutes) << 8) | \ 00984 ((uint32_t)RTC_ByteToBcd2(sAlarm->AlarmTime.Seconds)) | \ 00985 ((uint32_t)(sAlarm->AlarmTime.TimeFormat) << 16) | \ 00986 ((uint32_t)RTC_ByteToBcd2(sAlarm->AlarmDateWeekDay) << 24) | \ 00987 ((uint32_t)sAlarm->AlarmDateWeekDaySel) | \ 00988 ((uint32_t)sAlarm->AlarmMask)); 00989 } 00990 else 00991 { 00992 if((hrtc->Instance->CR & RTC_CR_FMT) != (uint32_t)RESET) 00993 { 00994 tmpreg = RTC_Bcd2ToByte(sAlarm->AlarmTime.Hours); 00995 assert_param(IS_RTC_HOUR12(tmpreg)); 00996 assert_param(IS_RTC_HOURFORMAT12(sAlarm->AlarmTime.TimeFormat)); 00997 } 00998 else 00999 { 01000 sAlarm->AlarmTime.TimeFormat = 0x00; 01001 assert_param(IS_RTC_HOUR24(RTC_Bcd2ToByte(sAlarm->AlarmTime.Hours))); 01002 } 01003 01004 assert_param(IS_RTC_MINUTES(RTC_Bcd2ToByte(sAlarm->AlarmTime.Minutes))); 01005 assert_param(IS_RTC_SECONDS(RTC_Bcd2ToByte(sAlarm->AlarmTime.Seconds))); 01006 01007 if(sAlarm->AlarmDateWeekDaySel == RTC_ALARMDATEWEEKDAYSEL_DATE) 01008 { 01009 tmpreg = RTC_Bcd2ToByte(sAlarm->AlarmDateWeekDay); 01010 assert_param(IS_RTC_ALARM_DATE_WEEKDAY_DATE(tmpreg)); 01011 } 01012 else 01013 { 01014 tmpreg = RTC_Bcd2ToByte(sAlarm->AlarmDateWeekDay); 01015 assert_param(IS_RTC_ALARM_DATE_WEEKDAY_WEEKDAY(tmpreg)); 01016 } 01017 tmpreg = (((uint32_t)(sAlarm->AlarmTime.Hours) << 16) | \ 01018 ((uint32_t)(sAlarm->AlarmTime.Minutes) << 8) | \ 01019 ((uint32_t) sAlarm->AlarmTime.Seconds) | \ 01020 ((uint32_t)(sAlarm->AlarmTime.TimeFormat) << 16) | \ 01021 ((uint32_t)(sAlarm->AlarmDateWeekDay) << 24) | \ 01022 ((uint32_t)sAlarm->AlarmDateWeekDaySel) | \ 01023 ((uint32_t)sAlarm->AlarmMask)); 01024 } 01025 /* Configure the Alarm A or Alarm B Sub Second registers */ 01026 subsecondtmpreg = (uint32_t)((uint32_t)(sAlarm->AlarmTime.SubSeconds) | (uint32_t)(sAlarm->AlarmSubSecondMask)); 01027 01028 /* Disable the write protection for RTC registers */ 01029 __HAL_RTC_WRITEPROTECTION_DISABLE(hrtc); 01030 01031 /* Configure the Alarm register */ 01032 if(sAlarm->Alarm == RTC_ALARM_A) 01033 { 01034 /* Disable the Alarm A interrupt */ 01035 __HAL_RTC_ALARMA_DISABLE(hrtc); 01036 01037 /* Clear flag alarm A */ 01038 __HAL_RTC_ALARM_CLEAR_FLAG(hrtc, RTC_FLAG_ALRAF); 01039 01040 tickstart = HAL_GetTick(); 01041 /* Wait till RTC ALRAWF flag is set and if Time out is reached exit */ 01042 while(__HAL_RTC_ALARM_GET_FLAG(hrtc, RTC_FLAG_ALRAWF) == RESET) 01043 { 01044 if((HAL_GetTick() - tickstart ) > RTC_TIMEOUT_VALUE) 01045 { 01046 /* Enable the write protection for RTC registers */ 01047 __HAL_RTC_WRITEPROTECTION_ENABLE(hrtc); 01048 01049 hrtc->State = HAL_RTC_STATE_TIMEOUT; 01050 01051 /* Process Unlocked */ 01052 __HAL_UNLOCK(hrtc); 01053 01054 return HAL_TIMEOUT; 01055 } 01056 } 01057 01058 hrtc->Instance->ALRMAR = (uint32_t)tmpreg; 01059 /* Configure the Alarm A Sub Second register */ 01060 hrtc->Instance->ALRMASSR = subsecondtmpreg; 01061 /* Configure the Alarm state: Enable Alarm */ 01062 __HAL_RTC_ALARMA_ENABLE(hrtc); 01063 /* Configure the Alarm interrupt */ 01064 __HAL_RTC_ALARM_ENABLE_IT(hrtc,RTC_IT_ALRA); 01065 } 01066 else 01067 { 01068 /* Disable the Alarm B interrupt */ 01069 __HAL_RTC_ALARMB_DISABLE(hrtc); 01070 01071 /* Clear flag alarm B */ 01072 __HAL_RTC_ALARM_CLEAR_FLAG(hrtc, RTC_FLAG_ALRBF); 01073 01074 tickstart = HAL_GetTick(); 01075 /* Wait till RTC ALRBWF flag is set and if Time out is reached exit */ 01076 while(__HAL_RTC_ALARM_GET_FLAG(hrtc, RTC_FLAG_ALRBWF) == RESET) 01077 { 01078 if((HAL_GetTick() - tickstart ) > RTC_TIMEOUT_VALUE) 01079 { 01080 /* Enable the write protection for RTC registers */ 01081 __HAL_RTC_WRITEPROTECTION_ENABLE(hrtc); 01082 01083 hrtc->State = HAL_RTC_STATE_TIMEOUT; 01084 01085 /* Process Unlocked */ 01086 __HAL_UNLOCK(hrtc); 01087 01088 return HAL_TIMEOUT; 01089 } 01090 } 01091 01092 hrtc->Instance->ALRMBR = (uint32_t)tmpreg; 01093 /* Configure the Alarm B Sub Second register */ 01094 hrtc->Instance->ALRMBSSR = subsecondtmpreg; 01095 /* Configure the Alarm state: Enable Alarm */ 01096 __HAL_RTC_ALARMB_ENABLE(hrtc); 01097 /* Configure the Alarm interrupt */ 01098 __HAL_RTC_ALARM_ENABLE_IT(hrtc, RTC_IT_ALRB); 01099 } 01100 01101 /* RTC Alarm Interrupt Configuration: EXTI configuration */ 01102 __HAL_RTC_ALARM_EXTI_ENABLE_IT(); 01103 01104 __HAL_RTC_ALARM_EXTI_ENABLE_RISING_EDGE(); 01105 01106 /* Enable the write protection for RTC registers */ 01107 __HAL_RTC_WRITEPROTECTION_ENABLE(hrtc); 01108 01109 hrtc->State = HAL_RTC_STATE_READY; 01110 01111 /* Process Unlocked */ 01112 __HAL_UNLOCK(hrtc); 01113 01114 return HAL_OK; 01115 } 01116 01117 /** 01118 * @brief Deactivate the specified RTC Alarm. 01119 * @param hrtc: RTC handle 01120 * @param Alarm: Specifies the Alarm. 01121 * This parameter can be one of the following values: 01122 * @arg RTC_ALARM_A: AlarmA 01123 * @arg RTC_ALARM_B: AlarmB 01124 * @retval HAL status 01125 */ 01126 HAL_StatusTypeDef HAL_RTC_DeactivateAlarm(RTC_HandleTypeDef *hrtc, uint32_t Alarm) 01127 { 01128 uint32_t tickstart = 0; 01129 01130 /* Check the parameters */ 01131 assert_param(IS_RTC_ALARM(Alarm)); 01132 01133 /* Process Locked */ 01134 __HAL_LOCK(hrtc); 01135 01136 hrtc->State = HAL_RTC_STATE_BUSY; 01137 01138 /* Disable the write protection for RTC registers */ 01139 __HAL_RTC_WRITEPROTECTION_DISABLE(hrtc); 01140 01141 if(Alarm == RTC_ALARM_A) 01142 { 01143 /* AlarmA */ 01144 __HAL_RTC_ALARMA_DISABLE(hrtc); 01145 01146 /* In case of interrupt mode is used, the interrupt source must disabled */ 01147 __HAL_RTC_ALARM_DISABLE_IT(hrtc, RTC_IT_ALRA); 01148 01149 tickstart = HAL_GetTick(); 01150 01151 /* Wait till RTC ALRxWF flag is set and if Time out is reached exit */ 01152 while(__HAL_RTC_ALARM_GET_FLAG(hrtc, RTC_FLAG_ALRAWF) == RESET) 01153 { 01154 if( (HAL_GetTick() - tickstart ) > RTC_TIMEOUT_VALUE) 01155 { 01156 /* Enable the write protection for RTC registers */ 01157 __HAL_RTC_WRITEPROTECTION_ENABLE(hrtc); 01158 01159 hrtc->State = HAL_RTC_STATE_TIMEOUT; 01160 01161 /* Process Unlocked */ 01162 __HAL_UNLOCK(hrtc); 01163 01164 return HAL_TIMEOUT; 01165 } 01166 } 01167 } 01168 else 01169 { 01170 /* AlarmB */ 01171 __HAL_RTC_ALARMB_DISABLE(hrtc); 01172 01173 /* In case of interrupt mode is used, the interrupt source must disabled */ 01174 __HAL_RTC_ALARM_DISABLE_IT(hrtc,RTC_IT_ALRB); 01175 01176 tickstart = HAL_GetTick(); 01177 01178 /* Wait till RTC ALRxWF flag is set and if Time out is reached exit */ 01179 while(__HAL_RTC_ALARM_GET_FLAG(hrtc, RTC_FLAG_ALRBWF) == RESET) 01180 { 01181 if((HAL_GetTick() - tickstart ) > RTC_TIMEOUT_VALUE) 01182 { 01183 /* Enable the write protection for RTC registers */ 01184 __HAL_RTC_WRITEPROTECTION_ENABLE(hrtc); 01185 01186 hrtc->State = HAL_RTC_STATE_TIMEOUT; 01187 01188 /* Process Unlocked */ 01189 __HAL_UNLOCK(hrtc); 01190 01191 return HAL_TIMEOUT; 01192 } 01193 } 01194 } 01195 /* Enable the write protection for RTC registers */ 01196 __HAL_RTC_WRITEPROTECTION_ENABLE(hrtc); 01197 01198 hrtc->State = HAL_RTC_STATE_READY; 01199 01200 /* Process Unlocked */ 01201 __HAL_UNLOCK(hrtc); 01202 01203 return HAL_OK; 01204 } 01205 01206 /** 01207 * @brief Get the RTC Alarm value and masks. 01208 * @param hrtc: RTC handle 01209 * @param sAlarm: Pointer to Date structure 01210 * @param Alarm: Specifies the Alarm. 01211 * This parameter can be one of the following values: 01212 * @arg RTC_ALARM_A: AlarmA 01213 * @arg RTC_ALARM_B: AlarmB 01214 * @param Format: Specifies the format of the entered parameters. 01215 * This parameter can be one of the following values: 01216 * @arg RTC_FORMAT_BIN: Binary data format 01217 * @arg RTC_FORMAT_BCD: BCD data format 01218 * @retval HAL status 01219 */ 01220 HAL_StatusTypeDef HAL_RTC_GetAlarm(RTC_HandleTypeDef *hrtc, RTC_AlarmTypeDef *sAlarm, uint32_t Alarm, uint32_t Format) 01221 { 01222 uint32_t tmpreg = 0, subsecondtmpreg = 0; 01223 01224 /* Check the parameters */ 01225 assert_param(IS_RTC_FORMAT(Format)); 01226 assert_param(IS_RTC_ALARM(Alarm)); 01227 01228 if(Alarm == RTC_ALARM_A) 01229 { 01230 /* AlarmA */ 01231 sAlarm->Alarm = RTC_ALARM_A; 01232 01233 tmpreg = (uint32_t)(hrtc->Instance->ALRMAR); 01234 subsecondtmpreg = (uint32_t)((hrtc->Instance->ALRMASSR ) & RTC_ALRMASSR_SS); 01235 } 01236 else 01237 { 01238 sAlarm->Alarm = RTC_ALARM_B; 01239 01240 tmpreg = (uint32_t)(hrtc->Instance->ALRMBR); 01241 subsecondtmpreg = (uint32_t)((hrtc->Instance->ALRMBSSR) & RTC_ALRMBSSR_SS); 01242 } 01243 01244 /* Fill the structure with the read parameters */ 01245 /* ALRMAR/ALRMBR registers have same mapping) */ 01246 sAlarm->AlarmTime.Hours = (uint32_t)((tmpreg & (RTC_ALRMAR_HT | RTC_ALRMAR_HU)) >> 16); 01247 sAlarm->AlarmTime.Minutes = (uint32_t)((tmpreg & (RTC_ALRMAR_MNT | RTC_ALRMAR_MNU)) >> 8); 01248 sAlarm->AlarmTime.Seconds = (uint32_t)(tmpreg & (RTC_ALRMAR_ST | RTC_ALRMAR_SU)); 01249 sAlarm->AlarmTime.TimeFormat = (uint32_t)((tmpreg & RTC_ALRMAR_PM) >> 16); 01250 sAlarm->AlarmTime.SubSeconds = (uint32_t) subsecondtmpreg; 01251 sAlarm->AlarmDateWeekDay = (uint32_t)((tmpreg & (RTC_ALRMAR_DT | RTC_ALRMAR_DU)) >> 24); 01252 sAlarm->AlarmDateWeekDaySel = (uint32_t)(tmpreg & RTC_ALRMAR_WDSEL); 01253 sAlarm->AlarmMask = (uint32_t)(tmpreg & RTC_ALARMMASK_ALL); 01254 01255 if(Format == RTC_FORMAT_BIN) 01256 { 01257 sAlarm->AlarmTime.Hours = RTC_Bcd2ToByte(sAlarm->AlarmTime.Hours); 01258 sAlarm->AlarmTime.Minutes = RTC_Bcd2ToByte(sAlarm->AlarmTime.Minutes); 01259 sAlarm->AlarmTime.Seconds = RTC_Bcd2ToByte(sAlarm->AlarmTime.Seconds); 01260 sAlarm->AlarmDateWeekDay = RTC_Bcd2ToByte(sAlarm->AlarmDateWeekDay); 01261 } 01262 01263 return HAL_OK; 01264 } 01265 01266 /** 01267 * @brief Handle Alarm interrupt request. 01268 * @param hrtc: RTC handle 01269 * @retval None 01270 */ 01271 void HAL_RTC_AlarmIRQHandler(RTC_HandleTypeDef* hrtc) 01272 { 01273 /* Get the AlarmA interrupt source enable status */ 01274 if(__HAL_RTC_ALARM_GET_IT_SOURCE(hrtc, RTC_IT_ALRA) != RESET) 01275 { 01276 /* Get the pending status of the AlarmA Interrupt */ 01277 if(__HAL_RTC_ALARM_GET_FLAG(hrtc, RTC_FLAG_ALRAF) != RESET) 01278 { 01279 /* AlarmA callback */ 01280 HAL_RTC_AlarmAEventCallback(hrtc); 01281 01282 /* Clear the AlarmA interrupt pending bit */ 01283 __HAL_RTC_ALARM_CLEAR_FLAG(hrtc, RTC_FLAG_ALRAF); 01284 } 01285 } 01286 01287 /* Get the AlarmB interrupt source enable status */ 01288 if(__HAL_RTC_ALARM_GET_IT_SOURCE(hrtc, RTC_IT_ALRB) != RESET) 01289 { 01290 /* Get the pending status of the AlarmB Interrupt */ 01291 if(__HAL_RTC_ALARM_GET_FLAG(hrtc, RTC_FLAG_ALRBF) != RESET) 01292 { 01293 /* AlarmB callback */ 01294 HAL_RTCEx_AlarmBEventCallback(hrtc); 01295 01296 /* Clear the AlarmB interrupt pending bit */ 01297 __HAL_RTC_ALARM_CLEAR_FLAG(hrtc, RTC_FLAG_ALRBF); 01298 } 01299 } 01300 01301 /* Clear the EXTI's line Flag for RTC Alarm */ 01302 __HAL_RTC_ALARM_EXTI_CLEAR_FLAG(); 01303 01304 /* Change RTC state */ 01305 hrtc->State = HAL_RTC_STATE_READY; 01306 } 01307 01308 /** 01309 * @brief Alarm A callback. 01310 * @param hrtc: RTC handle 01311 * @retval None 01312 */ 01313 __weak void HAL_RTC_AlarmAEventCallback(RTC_HandleTypeDef *hrtc) 01314 { 01315 /* NOTE : This function should not be modified, when the callback is needed, 01316 the HAL_RTC_AlarmAEventCallback could be implemented in the user file 01317 */ 01318 } 01319 01320 /** 01321 * @brief Handle AlarmA Polling request. 01322 * @param hrtc: RTC handle 01323 * @param Timeout: Timeout duration 01324 * @retval HAL status 01325 */ 01326 HAL_StatusTypeDef HAL_RTC_PollForAlarmAEvent(RTC_HandleTypeDef *hrtc, uint32_t Timeout) 01327 { 01328 01329 uint32_t tickstart = HAL_GetTick(); 01330 01331 while(__HAL_RTC_ALARM_GET_FLAG(hrtc, RTC_FLAG_ALRAF) == RESET) 01332 { 01333 if(Timeout != HAL_MAX_DELAY) 01334 { 01335 if((Timeout == 0)||((HAL_GetTick() - tickstart ) > Timeout)) 01336 { 01337 hrtc->State = HAL_RTC_STATE_TIMEOUT; 01338 return HAL_TIMEOUT; 01339 } 01340 } 01341 } 01342 01343 /* Clear the Alarm interrupt pending bit */ 01344 __HAL_RTC_ALARM_CLEAR_FLAG(hrtc, RTC_FLAG_ALRAF); 01345 01346 /* Change RTC state */ 01347 hrtc->State = HAL_RTC_STATE_READY; 01348 01349 return HAL_OK; 01350 } 01351 01352 /** 01353 * @} 01354 */ 01355 01356 /** @defgroup RTC_Exported_Functions_Group4 Peripheral Control functions 01357 * @brief Peripheral Control functions 01358 * 01359 @verbatim 01360 =============================================================================== 01361 ##### Peripheral Control functions ##### 01362 =============================================================================== 01363 [..] 01364 This subsection provides functions allowing to 01365 (+) Wait for RTC Time and Date Synchronization 01366 01367 @endverbatim 01368 * @{ 01369 */ 01370 01371 /** 01372 * @brief Wait until the RTC Time and Date registers (RTC_TR and RTC_DR) are 01373 * synchronized with RTC APB clock. 01374 * @note The RTC Resynchronization mode is write protected, use the 01375 * __HAL_RTC_WRITEPROTECTION_DISABLE() before calling this function. 01376 * @note To read the calendar through the shadow registers after Calendar 01377 * initialization, calendar update or after wakeup from low power modes 01378 * the software must first clear the RSF flag. 01379 * The software must then wait until it is set again before reading 01380 * the calendar, which means that the calendar registers have been 01381 * correctly copied into the RTC_TR and RTC_DR shadow registers. 01382 * @param hrtc: RTC handle 01383 * @retval HAL status 01384 */ 01385 HAL_StatusTypeDef HAL_RTC_WaitForSynchro(RTC_HandleTypeDef* hrtc) 01386 { 01387 uint32_t tickstart = 0; 01388 01389 /* Clear RSF flag */ 01390 hrtc->Instance->ISR &= (uint32_t)RTC_RSF_MASK; 01391 01392 tickstart = HAL_GetTick(); 01393 01394 /* Wait the registers to be synchronised */ 01395 while((hrtc->Instance->ISR & RTC_ISR_RSF) == (uint32_t)RESET) 01396 { 01397 if((HAL_GetTick() - tickstart ) > RTC_TIMEOUT_VALUE) 01398 { 01399 return HAL_TIMEOUT; 01400 } 01401 } 01402 01403 return HAL_OK; 01404 } 01405 01406 /** 01407 * @} 01408 */ 01409 01410 /** @defgroup RTC_Exported_Functions_Group5 Peripheral State functions 01411 * @brief Peripheral State functions 01412 * 01413 @verbatim 01414 =============================================================================== 01415 ##### Peripheral State functions ##### 01416 =============================================================================== 01417 [..] 01418 This subsection provides functions allowing to 01419 (+) Get RTC state 01420 01421 @endverbatim 01422 * @{ 01423 */ 01424 /** 01425 * @brief Return the RTC handle state. 01426 * @param hrtc: RTC handle 01427 * @retval HAL state 01428 */ 01429 HAL_RTCStateTypeDef HAL_RTC_GetState(RTC_HandleTypeDef* hrtc) 01430 { 01431 /* Return RTC handle state */ 01432 return hrtc->State; 01433 } 01434 01435 /** 01436 * @} 01437 */ 01438 01439 /** 01440 * @} 01441 */ 01442 01443 /** @defgroup RTC_Private_Functions RTC Private functions 01444 * @{ 01445 */ 01446 /** 01447 * @brief Enter the RTC Initialization mode. 01448 * @note The RTC Initialization mode is write protected, use the 01449 * __HAL_RTC_WRITEPROTECTION_DISABLE() before calling this function. 01450 * @param hrtc: RTC handle 01451 * @retval HAL status 01452 */ 01453 HAL_StatusTypeDef RTC_EnterInitMode(RTC_HandleTypeDef* hrtc) 01454 { 01455 uint32_t tickstart = 0; 01456 01457 /* Check if the Initialization mode is set */ 01458 if((hrtc->Instance->ISR & RTC_ISR_INITF) == (uint32_t)RESET) 01459 { 01460 /* Set the Initialization mode */ 01461 hrtc->Instance->ISR = (uint32_t)RTC_INIT_MASK; 01462 01463 tickstart = HAL_GetTick(); 01464 /* Wait till RTC is in INIT state and if Time out is reached exit */ 01465 while((hrtc->Instance->ISR & RTC_ISR_INITF) == (uint32_t)RESET) 01466 { 01467 if((HAL_GetTick() - tickstart ) > RTC_TIMEOUT_VALUE) 01468 { 01469 return HAL_TIMEOUT; 01470 } 01471 } 01472 } 01473 01474 return HAL_OK; 01475 } 01476 01477 01478 /** 01479 * @brief Convert a 2 digit decimal to BCD format. 01480 * @param Value: Byte to be converted 01481 * @retval Converted byte 01482 */ 01483 uint8_t RTC_ByteToBcd2(uint8_t Value) 01484 { 01485 uint32_t bcdhigh = 0; 01486 01487 while(Value >= 10) 01488 { 01489 bcdhigh++; 01490 Value -= 10; 01491 } 01492 01493 return ((uint8_t)(bcdhigh << 4) | Value); 01494 } 01495 01496 /** 01497 * @brief Convert from 2 digit BCD to Binary. 01498 * @param Value: BCD value to be converted 01499 * @retval Converted word 01500 */ 01501 uint8_t RTC_Bcd2ToByte(uint8_t Value) 01502 { 01503 uint32_t tmp = 0; 01504 tmp = ((uint8_t)(Value & (uint8_t)0xF0) >> (uint8_t)0x4) * 10; 01505 return (tmp + (Value & (uint8_t)0x0F)); 01506 } 01507 01508 /** 01509 * @} 01510 */ 01511 01512 #endif /* HAL_RTC_MODULE_ENABLED */ 01513 /** 01514 * @} 01515 */ 01516 01517 /** 01518 * @} 01519 */ 01520 01521 /************************ (C) COPYRIGHT STMicroelectronics *****END OF FILE****/ 01522
Generated on Tue Jul 12 2022 11:35:15 by
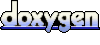