Hal Drivers for L4
Dependents: BSP OneHopeOnePrayer FINAL_AUDIO_RECORD AudioDemo
Fork of STM32L4xx_HAL_Driver by
stm32l4xx_hal_rcc_ex.h
00001 /** 00002 ****************************************************************************** 00003 * @file stm32l4xx_hal_rcc_ex.h 00004 * @author MCD Application Team 00005 * @version V1.1.0 00006 * @date 16-September-2015 00007 * @brief Header file of RCC HAL Extended module. 00008 ****************************************************************************** 00009 * @attention 00010 * 00011 * <h2><center>© COPYRIGHT(c) 2015 STMicroelectronics</center></h2> 00012 * 00013 * Redistribution and use in source and binary forms, with or without modification, 00014 * are permitted provided that the following conditions are met: 00015 * 1. Redistributions of source code must retain the above copyright notice, 00016 * this list of conditions and the following disclaimer. 00017 * 2. Redistributions in binary form must reproduce the above copyright notice, 00018 * this list of conditions and the following disclaimer in the documentation 00019 * and/or other materials provided with the distribution. 00020 * 3. Neither the name of STMicroelectronics nor the names of its contributors 00021 * may be used to endorse or promote products derived from this software 00022 * without specific prior written permission. 00023 * 00024 * THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" 00025 * AND ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE 00026 * IMPLIED WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE 00027 * DISCLAIMED. IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE 00028 * FOR ANY DIRECT, INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL 00029 * DAMAGES (INCLUDING, BUT NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR 00030 * SERVICES; LOSS OF USE, DATA, OR PROFITS; OR BUSINESS INTERRUPTION) HOWEVER 00031 * CAUSED AND ON ANY THEORY OF LIABILITY, WHETHER IN CONTRACT, STRICT LIABILITY, 00032 * OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) ARISING IN ANY WAY OUT OF THE USE 00033 * OF THIS SOFTWARE, EVEN IF ADVISED OF THE POSSIBILITY OF SUCH DAMAGE. 00034 * 00035 ****************************************************************************** 00036 */ 00037 00038 /* Define to prevent recursive inclusion -------------------------------------*/ 00039 #ifndef __STM32L4xx_HAL_RCC_EX_H 00040 #define __STM32L4xx_HAL_RCC_EX_H 00041 00042 #ifdef __cplusplus 00043 extern "C" { 00044 #endif 00045 00046 /* Includes ------------------------------------------------------------------*/ 00047 #include "stm32l4xx_hal_def.h" 00048 00049 /** @addtogroup STM32L4xx_HAL_Driver 00050 * @{ 00051 */ 00052 00053 /** @addtogroup RCCEx 00054 * @{ 00055 */ 00056 00057 /* Exported types ------------------------------------------------------------*/ 00058 00059 /** @defgroup RCCEx_Exported_Types RCCEx Exported Types 00060 * @{ 00061 */ 00062 00063 /** 00064 * @brief PLLSAI1 Clock structure definition 00065 */ 00066 typedef struct 00067 { 00068 00069 uint32_t PLLSAI1N; /*!< PLLSAI1N: specifies the multiplication factor for PLLSAI1 VCO output clock. 00070 This parameter must be a number between 8 and 86. */ 00071 00072 uint32_t PLLSAI1P; /*!< PLLSAI1P: specifies the division factor for SAI clock. 00073 This parameter must be a value of @ref RCC_PLLP_Clock_Divider */ 00074 00075 uint32_t PLLSAI1Q; /*!< PLLSAI1Q: specifies the division factor for USB/RNG/SDMMC1 clock. 00076 This parameter must be a value of @ref RCC_PLLQ_Clock_Divider */ 00077 00078 uint32_t PLLSAI1R; /*!< PLLSAI1R: specifies the division factor for ADC clock. 00079 This parameter must be a value of @ref RCC_PLLR_Clock_Divider */ 00080 00081 uint32_t PLLSAI1ClockOut; /*!< PLLSAIClockOut: specifies PLLSAI1 output clock to be enabled. 00082 This parameter must be a value of @ref RCC_PLLSAI1_Clock_Output */ 00083 }RCC_PLLSAI1InitTypeDef; 00084 00085 /** 00086 * @brief PLLSAI2 Clock structure definition 00087 */ 00088 typedef struct 00089 { 00090 00091 uint32_t PLLSAI2N; /*!< PLLSAI2N: specifies the multiplication factor for PLLSAI2 VCO output clock. 00092 This parameter must be a number between 8 and 86. */ 00093 00094 uint32_t PLLSAI2P; /*!< PLLSAI2P: specifies the division factor for SAI clock. 00095 This parameter must be a value of @ref RCC_PLLP_Clock_Divider */ 00096 00097 uint32_t PLLSAI2R; /*!< PLLSAI2R: specifies the division factor for ADC clock. 00098 This parameter must be a value of @ref RCC_PLLR_Clock_Divider */ 00099 00100 uint32_t PLLSAI2ClockOut; /*!< PLLSAIClockOut: specifies PLLSAI2 output clock to be enabled. 00101 This parameter must be a value of @ref RCC_PLLSAI2_Clock_Output */ 00102 }RCC_PLLSAI2InitTypeDef; 00103 00104 /** 00105 * @brief RCC extended clocks structure definition 00106 */ 00107 typedef struct 00108 { 00109 uint32_t PeriphClockSelection; /*!< The Extended Clock to be configured. 00110 This parameter can be a value of @ref RCCEx_Periph_Clock_Selection */ 00111 00112 RCC_PLLSAI1InitTypeDef PLLSAI1; /*!< PLLSAI1 structure parameters. 00113 This parameter will be used only when PLLSAI1 is selected as Clock Source for SAI1, USB/RNG/SDMMC1 or ADC */ 00114 00115 RCC_PLLSAI2InitTypeDef PLLSAI2; /*!< PLLSAI2 structure parameters. 00116 This parameter will be used only when PLLSAI2 is selected as Clock Source for SAI2 or ADC */ 00117 00118 uint32_t Usart1ClockSelection; /*!< Specifies USART1 clock source. 00119 This parameter can be a value of @ref RCCEx_USART1_Clock_Source */ 00120 00121 uint32_t Usart2ClockSelection; /*!< Specifies USART2 clock source. 00122 This parameter can be a value of @ref RCCEx_USART2_Clock_Source */ 00123 00124 uint32_t Usart3ClockSelection; /*!< Specifies USART3 clock source. 00125 This parameter can be a value of @ref RCCEx_USART3_Clock_Source */ 00126 00127 uint32_t Uart4ClockSelection; /*!< Specifies UART4 clock source. 00128 This parameter can be a value of @ref RCCEx_UART4_Clock_Source */ 00129 00130 uint32_t Uart5ClockSelection; /*!< Specifies UART5 clock source. 00131 This parameter can be a value of @ref RCCEx_UART5_Clock_Source */ 00132 00133 uint32_t Lpuart1ClockSelection; /*!< Specifies LPUART1 clock source. 00134 This parameter can be a value of @ref RCCEx_LPUART1_Clock_Source */ 00135 00136 uint32_t I2c1ClockSelection; /*!< Specifies I2C1 clock source. 00137 This parameter can be a value of @ref RCCEx_I2C1_Clock_Source */ 00138 00139 uint32_t I2c2ClockSelection; /*!< Specifies I2C2 clock source. 00140 This parameter can be a value of @ref RCCEx_I2C2_Clock_Source */ 00141 00142 uint32_t I2c3ClockSelection; /*!< Specifies I2C3 clock source. 00143 This parameter can be a value of @ref RCCEx_I2C3_Clock_Source */ 00144 00145 uint32_t Lptim1ClockSelection; /*!< Specifies LPTIM1 clock source. 00146 This parameter can be a value of @ref RCCEx_LPTIM1_Clock_Source */ 00147 00148 uint32_t Lptim2ClockSelection; /*!< Specifies LPTIM2 clock source. 00149 This parameter can be a value of @ref RCCEx_LPTIM2_Clock_Source */ 00150 00151 uint32_t Sai1ClockSelection; /*!< Specifies SAI1 clock source. 00152 This parameter can be a value of @ref RCCEx_SAI1_Clock_Source */ 00153 00154 uint32_t Sai2ClockSelection; /*!< Specifies SAI2 clock source. 00155 This parameter can be a value of @ref RCCEx_SAI2_Clock_Source */ 00156 00157 #if defined(STM32L475xx) || defined(STM32L476xx) || defined(STM32L485xx) || defined(STM32L486xx) 00158 00159 uint32_t UsbClockSelection; /*!< Specifies USB clock source (warning: same source for SDMMC1 and RNG). 00160 This parameter can be a value of @ref RCCEx_USB_Clock_Source */ 00161 00162 #endif /* STM32L475xx || STM32L476xx || STM32L485xx || STM32L486xx */ 00163 00164 uint32_t Sdmmc1ClockSelection; /*!< Specifies SDMMC1 clock source (warning: same source for USB and RNG). 00165 This parameter can be a value of @ref RCCEx_SDMMC1_Clock_Source */ 00166 00167 uint32_t RngClockSelection; /*!< Specifies RNG clock source (warning: same source for USB and SDMMC1). 00168 This parameter can be a value of @ref RCCEx_RNG_Clock_Source */ 00169 00170 uint32_t AdcClockSelection; /*!< Specifies ADC interface clock source. 00171 This parameter can be a value of @ref RCCEx_ADC_Clock_Source */ 00172 00173 uint32_t Swpmi1ClockSelection; /*!< Specifies SWPMI1 clock source. 00174 This parameter can be a value of @ref RCCEx_SWPMI1_Clock_Source */ 00175 00176 uint32_t DfsdmClockSelection; /*!< Specifies DFSDM clock source. 00177 This parameter can be a value of @ref RCCEx_DFSDM_Clock_Source */ 00178 00179 uint32_t RTCClockSelection; /*!< Specifies RTC clock source. 00180 This parameter can be a value of @ref RCC_RTC_Clock_Source */ 00181 }RCC_PeriphCLKInitTypeDef; 00182 00183 /** 00184 * @} 00185 */ 00186 00187 /* Exported constants --------------------------------------------------------*/ 00188 /** @defgroup RCCEx_Exported_Constants RCCEx Exported Constants 00189 * @{ 00190 */ 00191 00192 /** @defgroup RCCEx_LSCO_Clock_Source Low Speed Clock Source 00193 * @{ 00194 */ 00195 #define RCC_LSCOSOURCE_LSI (uint32_t)0x00000000 /*!< LSI selection for low speed clock output */ 00196 #define RCC_LSCOSOURCE_LSE RCC_BDCR_LSCOSEL /*!< LSE selection for low speed clock output */ 00197 /** 00198 * @} 00199 */ 00200 00201 /** @defgroup RCCEx_Periph_Clock_Selection Periph Clock Selection 00202 * @{ 00203 */ 00204 #define RCC_PERIPHCLK_USART1 ((uint32_t)0x00000001) 00205 #define RCC_PERIPHCLK_USART2 ((uint32_t)0x00000002) 00206 #define RCC_PERIPHCLK_USART3 ((uint32_t)0x00000004) 00207 #define RCC_PERIPHCLK_UART4 ((uint32_t)0x00000008) 00208 #define RCC_PERIPHCLK_UART5 ((uint32_t)0x00000010) 00209 #define RCC_PERIPHCLK_LPUART1 ((uint32_t)0x00000020) 00210 #define RCC_PERIPHCLK_I2C1 ((uint32_t)0x00000040) 00211 #define RCC_PERIPHCLK_I2C2 ((uint32_t)0x00000080) 00212 #define RCC_PERIPHCLK_I2C3 ((uint32_t)0x00000100) 00213 #define RCC_PERIPHCLK_LPTIM1 ((uint32_t)0x00000200) 00214 #define RCC_PERIPHCLK_LPTIM2 ((uint32_t)0x00000400) 00215 #define RCC_PERIPHCLK_SAI1 ((uint32_t)0x00000800) 00216 #define RCC_PERIPHCLK_SAI2 ((uint32_t)0x00001000) 00217 #if defined(STM32L475xx) || defined(STM32L476xx) || defined(STM32L485xx) || defined(STM32L486xx) 00218 #define RCC_PERIPHCLK_USB ((uint32_t)0x00002000) 00219 #endif /* STM32L475xx || STM32L476xx || STM32L485xx || STM32L486xx */ 00220 #define RCC_PERIPHCLK_ADC ((uint32_t)0x00004000) 00221 #define RCC_PERIPHCLK_SWPMI1 ((uint32_t)0x00008000) 00222 #define RCC_PERIPHCLK_DFSDM ((uint32_t)0x00010000) 00223 #define RCC_PERIPHCLK_RTC ((uint32_t)0x00020000) 00224 #define RCC_PERIPHCLK_RNG ((uint32_t)0x00040000) 00225 #define RCC_PERIPHCLK_SDMMC1 ((uint32_t)0x00080000) 00226 /** 00227 * @} 00228 */ 00229 00230 00231 /** @defgroup RCCEx_USART1_Clock_Source USART1 Clock Source 00232 * @{ 00233 */ 00234 #define RCC_USART1CLKSOURCE_PCLK2 ((uint32_t)0x00000000) 00235 #define RCC_USART1CLKSOURCE_SYSCLK RCC_CCIPR_USART1SEL_0 00236 #define RCC_USART1CLKSOURCE_HSI RCC_CCIPR_USART1SEL_1 00237 #define RCC_USART1CLKSOURCE_LSE (RCC_CCIPR_USART1SEL_0 | RCC_CCIPR_USART1SEL_1) 00238 /** 00239 * @} 00240 */ 00241 00242 /** @defgroup RCCEx_USART2_Clock_Source USART2 Clock Source 00243 * @{ 00244 */ 00245 #define RCC_USART2CLKSOURCE_PCLK1 ((uint32_t)0x00000000) 00246 #define RCC_USART2CLKSOURCE_SYSCLK RCC_CCIPR_USART2SEL_0 00247 #define RCC_USART2CLKSOURCE_HSI RCC_CCIPR_USART2SEL_1 00248 #define RCC_USART2CLKSOURCE_LSE (RCC_CCIPR_USART2SEL_0 | RCC_CCIPR_USART2SEL_1) 00249 /** 00250 * @} 00251 */ 00252 00253 /** @defgroup RCCEx_USART3_Clock_Source USART3 Clock Source 00254 * @{ 00255 */ 00256 #define RCC_USART3CLKSOURCE_PCLK1 ((uint32_t)0x00000000) 00257 #define RCC_USART3CLKSOURCE_SYSCLK RCC_CCIPR_USART3SEL_0 00258 #define RCC_USART3CLKSOURCE_HSI RCC_CCIPR_USART3SEL_1 00259 #define RCC_USART3CLKSOURCE_LSE (RCC_CCIPR_USART3SEL_0 | RCC_CCIPR_USART3SEL_1) 00260 /** 00261 * @} 00262 */ 00263 00264 /** @defgroup RCCEx_UART4_Clock_Source UART4 Clock Source 00265 * @{ 00266 */ 00267 #define RCC_UART4CLKSOURCE_PCLK1 ((uint32_t)0x00000000) 00268 #define RCC_UART4CLKSOURCE_SYSCLK RCC_CCIPR_UART4SEL_0 00269 #define RCC_UART4CLKSOURCE_HSI RCC_CCIPR_UART4SEL_1 00270 #define RCC_UART4CLKSOURCE_LSE (RCC_CCIPR_UART4SEL_0 | RCC_CCIPR_UART4SEL_1) 00271 /** 00272 * @} 00273 */ 00274 00275 /** @defgroup RCCEx_UART5_Clock_Source UART5 Clock Source 00276 * @{ 00277 */ 00278 #define RCC_UART5CLKSOURCE_PCLK1 ((uint32_t)0x00000000) 00279 #define RCC_UART5CLKSOURCE_SYSCLK RCC_CCIPR_UART5SEL_0 00280 #define RCC_UART5CLKSOURCE_HSI RCC_CCIPR_UART5SEL_1 00281 #define RCC_UART5CLKSOURCE_LSE (RCC_CCIPR_UART5SEL_0 | RCC_CCIPR_UART5SEL_1) 00282 /** 00283 * @} 00284 */ 00285 00286 /** @defgroup RCCEx_LPUART1_Clock_Source LPUART1 Clock Source 00287 * @{ 00288 */ 00289 #define RCC_LPUART1CLKSOURCE_PCLK1 ((uint32_t)0x00000000) 00290 #define RCC_LPUART1CLKSOURCE_SYSCLK RCC_CCIPR_LPUART1SEL_0 00291 #define RCC_LPUART1CLKSOURCE_HSI RCC_CCIPR_LPUART1SEL_1 00292 #define RCC_LPUART1CLKSOURCE_LSE (RCC_CCIPR_LPUART1SEL_0 | RCC_CCIPR_LPUART1SEL_1) 00293 /** 00294 * @} 00295 */ 00296 00297 /** @defgroup RCCEx_I2C1_Clock_Source I2C1 Clock Source 00298 * @{ 00299 */ 00300 #define RCC_I2C1CLKSOURCE_PCLK1 ((uint32_t)0x00000000) 00301 #define RCC_I2C1CLKSOURCE_SYSCLK RCC_CCIPR_I2C1SEL_0 00302 #define RCC_I2C1CLKSOURCE_HSI RCC_CCIPR_I2C1SEL_1 00303 /** 00304 * @} 00305 */ 00306 00307 /** @defgroup RCCEx_I2C2_Clock_Source I2C2 Clock Source 00308 * @{ 00309 */ 00310 #define RCC_I2C2CLKSOURCE_PCLK1 ((uint32_t)0x00000000) 00311 #define RCC_I2C2CLKSOURCE_SYSCLK RCC_CCIPR_I2C2SEL_0 00312 #define RCC_I2C2CLKSOURCE_HSI RCC_CCIPR_I2C2SEL_1 00313 /** 00314 * @} 00315 */ 00316 00317 /** @defgroup RCCEx_I2C3_Clock_Source I2C3 Clock Source 00318 * @{ 00319 */ 00320 #define RCC_I2C3CLKSOURCE_PCLK1 ((uint32_t)0x00000000) 00321 #define RCC_I2C3CLKSOURCE_SYSCLK RCC_CCIPR_I2C3SEL_0 00322 #define RCC_I2C3CLKSOURCE_HSI RCC_CCIPR_I2C3SEL_1 00323 /** 00324 * @} 00325 */ 00326 00327 /** @defgroup RCCEx_SAI1_Clock_Source SAI1 Clock Source 00328 * @{ 00329 */ 00330 #define RCC_SAI1CLKSOURCE_PLLSAI1 ((uint32_t)0x00000000) 00331 #define RCC_SAI1CLKSOURCE_PLLSAI2 RCC_CCIPR_SAI1SEL_0 00332 #define RCC_SAI1CLKSOURCE_PLL RCC_CCIPR_SAI1SEL_1 00333 #define RCC_SAI1CLKSOURCE_PIN RCC_CCIPR_SAI1SEL 00334 /** 00335 * @} 00336 */ 00337 00338 /** @defgroup RCCEx_SAI2_Clock_Source SAI2 Clock Source 00339 * @{ 00340 */ 00341 #define RCC_SAI2CLKSOURCE_PLLSAI1 ((uint32_t)0x00000000) 00342 #define RCC_SAI2CLKSOURCE_PLLSAI2 RCC_CCIPR_SAI2SEL_0 00343 #define RCC_SAI2CLKSOURCE_PLL RCC_CCIPR_SAI2SEL_1 00344 #define RCC_SAI2CLKSOURCE_PIN RCC_CCIPR_SAI2SEL 00345 /** 00346 * @} 00347 */ 00348 00349 /** @defgroup RCCEx_LPTIM1_Clock_Source LPTIM1 Clock Source 00350 * @{ 00351 */ 00352 #define RCC_LPTIM1CLKSOURCE_PCLK ((uint32_t)0x00000000) 00353 #define RCC_LPTIM1CLKSOURCE_LSI RCC_CCIPR_LPTIM1SEL_0 00354 #define RCC_LPTIM1CLKSOURCE_HSI RCC_CCIPR_LPTIM1SEL_1 00355 #define RCC_LPTIM1CLKSOURCE_LSE RCC_CCIPR_LPTIM1SEL 00356 /** 00357 * @} 00358 */ 00359 00360 /** @defgroup RCCEx_LPTIM2_Clock_Source LPTIM2 Clock Source 00361 * @{ 00362 */ 00363 #define RCC_LPTIM2CLKSOURCE_PCLK ((uint32_t)0x00000000) 00364 #define RCC_LPTIM2CLKSOURCE_LSI RCC_CCIPR_LPTIM2SEL_0 00365 #define RCC_LPTIM2CLKSOURCE_HSI RCC_CCIPR_LPTIM2SEL_1 00366 #define RCC_LPTIM2CLKSOURCE_LSE RCC_CCIPR_LPTIM2SEL 00367 /** 00368 * @} 00369 */ 00370 00371 /** @defgroup RCCEx_SDMMC1_Clock_Source SDMMC1 Clock Source 00372 * @{ 00373 */ 00374 #define RCC_SDMMC1CLKSOURCE_NONE ((uint32_t)0x00000000) 00375 #define RCC_SDMMC1CLKSOURCE_PLLSAI1 RCC_CCIPR_CLK48SEL_0 00376 #define RCC_SDMMC1CLKSOURCE_PLL RCC_CCIPR_CLK48SEL_1 00377 #define RCC_SDMMC1CLKSOURCE_MSI RCC_CCIPR_CLK48SEL 00378 /** 00379 * @} 00380 */ 00381 00382 /** @defgroup RCCEx_RNG_Clock_Source RNG Clock Source 00383 * @{ 00384 */ 00385 #define RCC_RNGCLKSOURCE_NONE ((uint32_t)0x00000000) 00386 #define RCC_RNGCLKSOURCE_PLLSAI1 RCC_CCIPR_CLK48SEL_0 00387 #define RCC_RNGCLKSOURCE_PLL RCC_CCIPR_CLK48SEL_1 00388 #define RCC_RNGCLKSOURCE_MSI RCC_CCIPR_CLK48SEL 00389 /** 00390 * @} 00391 */ 00392 00393 #if defined(STM32L475xx) || defined(STM32L476xx) || defined(STM32L485xx) || defined(STM32L486xx) 00394 /** @defgroup RCCEx_USB_Clock_Source USB Clock Source 00395 * @{ 00396 */ 00397 #define RCC_USBCLKSOURCE_NONE ((uint32_t)0x00000000) 00398 #define RCC_USBCLKSOURCE_PLLSAI1 RCC_CCIPR_CLK48SEL_0 00399 #define RCC_USBCLKSOURCE_PLL RCC_CCIPR_CLK48SEL_1 00400 #define RCC_USBCLKSOURCE_MSI RCC_CCIPR_CLK48SEL 00401 /** 00402 * @} 00403 */ 00404 #endif /* STM32L475xx || STM32L476xx || STM32L485xx || STM32L486xx */ 00405 00406 /** @defgroup RCCEx_ADC_Clock_Source ADC Clock Source 00407 * @{ 00408 */ 00409 #define RCC_ADCCLKSOURCE_NONE ((uint32_t)0x00000000) 00410 #define RCC_ADCCLKSOURCE_PLLSAI1 RCC_CCIPR_ADCSEL_0 00411 #define RCC_ADCCLKSOURCE_PLLSAI2 RCC_CCIPR_ADCSEL_1 00412 #define RCC_ADCCLKSOURCE_SYSCLK RCC_CCIPR_ADCSEL 00413 /** 00414 * @} 00415 */ 00416 00417 /** @defgroup RCCEx_SWPMI1_Clock_Source SWPMI1 Clock Source 00418 * @{ 00419 */ 00420 #define RCC_SWPMI1CLKSOURCE_PCLK ((uint32_t)0x00000000) 00421 #define RCC_SWPMI1CLKSOURCE_HSI RCC_CCIPR_SWPMI1SEL 00422 /** 00423 * @} 00424 */ 00425 00426 /** @defgroup RCCEx_DFSDM_Clock_Source DFSDM Clock Source 00427 * @{ 00428 */ 00429 #define RCC_DFSDMCLKSOURCE_PCLK ((uint32_t)0x00000000) 00430 #define RCC_DFSDMCLKSOURCE_SYSCLK RCC_CCIPR_DFSDMSEL 00431 /** 00432 * @} 00433 */ 00434 00435 /** @defgroup RCCEx_EXTI_LINE_LSECSS RCC LSE CSS external interrupt line 00436 * @{ 00437 */ 00438 #define RCC_EXTI_LINE_LSECSS EXTI_IMR1_IM19 /*!< External interrupt line 19 connected to the LSE CSS EXTI Line */ 00439 /** 00440 * @} 00441 */ 00442 00443 /** 00444 * @} 00445 */ 00446 00447 /* Exported macros -----------------------------------------------------------*/ 00448 /** @defgroup RCCEx_Exported_Macros RCCEx Exported Macros 00449 * @{ 00450 */ 00451 00452 00453 /** 00454 * @brief Macro to configure the PLLSAI1 clock multiplication and division factors. 00455 * 00456 * @note This function must be used only when the PLLSAI1 is disabled. 00457 * @note PLLSAI1 clock source is common with the main PLL (configured through 00458 * __HAL_RCC_PLL_CONFIG() macro) 00459 * 00460 * @param __PLLSAI1N__ specifies the multiplication factor for PLLSAI1 VCO output clock. 00461 * This parameter must be a number between 8 and 86. 00462 * @note You have to set the PLLSAI1N parameter correctly to ensure that the VCO 00463 * output frequency is between 64 and 344 MHz. 00464 * PLLSAI1 clock frequency = f(PLLSAI1) multiplied by PLLSAI1N 00465 * 00466 * @param __PLLSAI1P__ specifies the division factor for SAI clock. 00467 * This parameter must be a number in the range (7 or 17). 00468 * SAI1 clock frequency = f(PLLSAI1) / PLLSAI1P 00469 * 00470 * @param __PLLSAI1Q__ specifies the division factor for USB/RNG/SDMMC1 clock. 00471 * This parameter must be in the range (2, 4, 6 or 8). 00472 * USB/RNG/SDMMC1 clock frequency = f(PLLSAI1) / PLLSAI1Q 00473 * 00474 * @param __PLLSAI1R__ specifies the division factor for SAR ADC clock. 00475 * This parameter must be in the range (2, 4, 6 or 8). 00476 * ADC clock frequency = f(PLLSAI1) / PLLSAI1R 00477 * 00478 * @retval None 00479 */ 00480 #define __HAL_RCC_PLLSAI1_CONFIG(__PLLSAI1N__, __PLLSAI1P__, __PLLSAI1Q__, __PLLSAI1R__) \ 00481 WRITE_REG(RCC->PLLSAI1CFGR, ((__PLLSAI1N__) << 8U) | (((__PLLSAI1P__) >> 4U) << 17U) | \ 00482 ((((__PLLSAI1Q__) >> 1U) - 1) << 21U) | ((((__PLLSAI1R__) >> 1U) - 1) << 25U)) 00483 00484 /** 00485 * @brief Macro to configure the PLLSAI1 clock multiplication factor N. 00486 * 00487 * @note This function must be used only when the PLLSAI1 is disabled. 00488 * @note PLLSAI1 clock source is common with the main PLL (configured through 00489 * __HAL_RCC_PLL_CONFIG() macro) 00490 * 00491 * @param __PLLSAI1N__ specifies the multiplication factor for PLLSAI1 VCO output clock. 00492 * This parameter must be a number between 8 and 86. 00493 * @note You have to set the PLLSAI1N parameter correctly to ensure that the VCO 00494 * output frequency is between 64 and 344 MHz. 00495 * Use to set PLLSAI1 clock frequency = f(PLLSAI1) multiplied by PLLSAI1N 00496 * 00497 * @retval None 00498 */ 00499 #define __HAL_RCC_PLLSAI1_MULN_CONFIG(__PLLSAI1N__) \ 00500 MODIFY_REG(RCC->PLLSAI1CFGR, RCC_PLLSAI1CFGR_PLLSAI1N, (__PLLSAI1N__) << 8U) 00501 00502 /** @brief Macro to configure the PLLSAI1 clock division factor P. 00503 * 00504 * @note This function must be used only when the PLLSAI1 is disabled. 00505 * @note PLLSAI1 clock source is common with the main PLL (configured through 00506 * __HAL_RCC_PLL_CONFIG() macro) 00507 * 00508 * @param __PLLSAI1P__ specifies the division factor for SAI clock. 00509 * This parameter must be a number in the range (7 or 17). 00510 * Use to set SAI1 clock frequency = f(PLLSAI1) / PLLSAI1P 00511 * 00512 * @retval None 00513 */ 00514 #define __HAL_RCC_PLLSAI1_DIVP_CONFIG(__PLLSAI1P__) \ 00515 MODIFY_REG(RCC->PLLSAI1CFGR, RCC_PLLSAI1CFGR_PLLSAI1P, ((__PLLSAI1P__) >> 4U) << 17U) 00516 00517 /** @brief Macro to configure the PLLSAI1 clock division factor Q. 00518 * 00519 * @note This function must be used only when the PLLSAI1 is disabled. 00520 * @note PLLSAI1 clock source is common with the main PLL (configured through 00521 * __HAL_RCC_PLL_CONFIG() macro) 00522 * 00523 * @param __PLLSAI1Q__ specifies the division factor for USB/RNG/SDMMC1 clock. 00524 * This parameter must be in the range (2, 4, 6 or 8). 00525 * Use to set USB/RNG/SDMMC1 clock frequency = f(PLLSAI1) / PLLSAI1Q 00526 * 00527 * @retval None 00528 */ 00529 #define __HAL_RCC_PLLSAI1_DIVQ_CONFIG(__PLLSAI1Q__) \ 00530 MODIFY_REG(RCC->PLLSAI1CFGR, RCC_PLLSAI1CFGR_PLLSAI1Q, (((__PLLSAI1Q__) >> 1U) - 1) << 21U) 00531 00532 /** @brief Macro to configure the PLLSAI1 clock division factor R. 00533 * 00534 * @note This function must be used only when the PLLSAI1 is disabled. 00535 * @note PLLSAI1 clock source is common with the main PLL (configured through 00536 * __HAL_RCC_PLL_CONFIG() macro) 00537 * 00538 * @param __PLLSAI1R__ specifies the division factor for ADC clock. 00539 * This parameter must be in the range (2, 4, 6 or 8) 00540 * Use to set ADC clock frequency = f(PLLSAI1) / PLLSAI1R 00541 * 00542 * @retval None 00543 */ 00544 #define __HAL_RCC_PLLSAI1_DIVR_CONFIG(__PLLSAI1R__) \ 00545 MODIFY_REG(RCC->PLLSAI1CFGR, RCC_PLLSAI1CFGR_PLLSAI1R, (((__PLLSAI1R__) >> 1U) - 1) << 25U) 00546 00547 /** 00548 * @brief Macros to enable or disable the PLLSAI1. 00549 * @note The PLLSAI1 is disabled by hardware when entering STOP and STANDBY modes. 00550 * @retval None 00551 */ 00552 00553 #define __HAL_RCC_PLLSAI1_ENABLE() SET_BIT(RCC->CR, RCC_CR_PLLSAI1ON) 00554 00555 #define __HAL_RCC_PLLSAI1_DISABLE() CLEAR_BIT(RCC->CR, RCC_CR_PLLSAI1ON) 00556 00557 /** 00558 * @brief Macros to enable or disable each clock output (PLLSAI1_SAI1, PLLSAI1_USB2 and PLLSAI1_ADC1). 00559 * @note Enabling and disabling those clocks can be done without the need to stop the PLL. 00560 * This is mainly used to save Power. 00561 * @param __PLLSAI1_CLOCKOUT__ specifies the PLLSAI1 clock to be output. 00562 * This parameter can be one or a combination of the following values: 00563 * @arg @ref RCC_PLLSAI1_SAI1CLK This clock is used to generate an accurate clock to achieve 00564 * high-quality audio performance on SAI interface in case. 00565 * @arg @ref RCC_PLLSAI1_48M2CLK This clock is used to generate the clock for the USB OTG FS (48 MHz), 00566 * the random number generator (<=48 MHz) and the SDIO (<= 48 MHz). 00567 * @arg @ref RCC_PLLSAI1_ADC1CLK Clock used to clock ADC peripheral. 00568 * @retval None 00569 */ 00570 00571 #define __HAL_RCC_PLLSAI1CLKOUT_ENABLE(__PLLSAI1_CLOCKOUT__) SET_BIT(RCC->PLLSAI1CFGR, (__PLLSAI1_CLOCKOUT__)) 00572 00573 #define __HAL_RCC_PLLSAI1CLKOUT_DISABLE(__PLLSAI1_CLOCKOUT__) CLEAR_BIT(RCC->PLLSAI1CFGR, (__PLLSAI1_CLOCKOUT__)) 00574 00575 /** 00576 * @brief Macro to get clock output enable status (PLLSAI1_SAI1, PLLSAI1_USB2 and PLLSAI1_ADC1). 00577 * @param __PLLSAI1_CLOCKOUT__ specifies the PLLSAI1 clock to be output. 00578 * This parameter can be one of the following values: 00579 * @arg @ref RCC_PLLSAI1_SAI1CLK This clock is used to generate an accurate clock to achieve 00580 * high-quality audio performance on SAI interface in case. 00581 * @arg @ref RCC_PLLSAI1_48M2CLK This clock is used to generate the clock for the USB OTG FS (48 MHz), 00582 * the random number generator (<=48 MHz) and the SDIO (<= 48 MHz). 00583 * @arg @ref RCC_PLLSAI1_ADC1CLK Clock used to clock ADC peripheral. 00584 * @retval SET / RESET 00585 */ 00586 #define __HAL_RCC_GET_PLLSAI1CLKOUT_CONFIG(__PLLSAI1_CLOCKOUT__) READ_BIT(RCC->PLLSAI1CFGR, (__PLLSAI1_CLOCKOUT__)) 00587 00588 /** 00589 * @brief Macro to configure the PLLSAI2 clock multiplication and division factors. 00590 * 00591 * @note This function must be used only when the PLLSAI2 is disabled. 00592 * @note PLLSAI2 clock source is common with the main PLL (configured through 00593 * __HAL_RCC_PLL_CONFIG() macro) 00594 * 00595 * @param __PLLSAI2N__ specifies the multiplication factor for PLLSAI2 VCO output clock. 00596 * This parameter must be a number between 8 and 86. 00597 * @note You have to set the PLLSAI2N parameter correctly to ensure that the VCO 00598 * output frequency is between 64 and 344 MHz. 00599 * 00600 * @param __PLLSAI2P__ specifies the division factor for SAI clock. 00601 * This parameter must be a number in the range (7 or 17). 00602 * 00603 * 00604 * @param __PLLSAI2R__ specifies the division factor for SAR ADC clock. 00605 * This parameter must be in the range (2, 4, 6 or 8) 00606 * 00607 * @retval None 00608 */ 00609 00610 #define __HAL_RCC_PLLSAI2_CONFIG(__PLLSAI2N__, __PLLSAI2P__, __PLLSAI2R__) \ 00611 WRITE_REG(RCC->PLLSAI2CFGR, ((__PLLSAI2N__) << 8U) | (((__PLLSAI2P__) >> 4U) << 17U) | \ 00612 ((((__PLLSAI2R__) >> 1U) - 1) << 25U)) 00613 00614 /** 00615 * @brief Macro to configure the PLLSAI2 clock multiplication factor N. 00616 * 00617 * @note This function must be used only when the PLLSAI2 is disabled. 00618 * @note PLLSAI2 clock source is common with the main PLL (configured through 00619 * __HAL_RCC_PLL_CONFIG() macro) 00620 * 00621 * @param __PLLSAI2N__ specifies the multiplication factor for PLLSAI2 VCO output clock. 00622 * This parameter must be a number between 8 and 86. 00623 * @note You have to set the PLLSAI2N parameter correctly to ensure that the VCO 00624 * output frequency is between 64 and 344 MHz. 00625 * PLLSAI1 clock frequency = f(PLLSAI1) multiplied by PLLSAI2N 00626 * 00627 * @retval None 00628 */ 00629 #define __HAL_RCC_PLLSAI2_MULN_CONFIG(__PLLSAI2N__) \ 00630 MODIFY_REG(RCC->PLLSAI2CFGR, RCC_PLLSAI2CFGR_PLLSAI2N, (__PLLSAI2N__) << 8U) 00631 00632 /** @brief Macro to configure the PLLSAI2 clock division factor P. 00633 * 00634 * @note This function must be used only when the PLLSAI2 is disabled. 00635 * @note PLLSAI2 clock source is common with the main PLL (configured through 00636 * __HAL_RCC_PLL_CONFIG() macro) 00637 * 00638 * @param __PLLSAI2P__ specifies the division factor. 00639 * This parameter must be a number in the range (7 or 17). 00640 * Use to set SAI2 clock frequency = f(PLLSAI2) / __PLLSAI2P__ 00641 * 00642 * @retval None 00643 */ 00644 #define __HAL_RCC_PLLSAI2_DIVP_CONFIG(__PLLSAI2P__) \ 00645 MODIFY_REG(RCC->PLLSAI2CFGR, RCC_PLLSAI2CFGR_PLLSAI2P, ((__PLLSAI2P__) >> 4U) << 17U) 00646 00647 /** @brief Macro to configure the PLLSAI2 clock division factor R. 00648 * 00649 * @note This function must be used only when the PLLSAI2 is disabled. 00650 * @note PLLSAI1 clock source is common with the main PLL (configured through 00651 * __HAL_RCC_PLL_CONFIG() macro) 00652 * 00653 * @param __PLLSAI2R__ specifies the division factor. 00654 * This parameter must be in the range (2, 4, 6 or 8). 00655 * Use to set ADC clock frequency = f(PLLSAI2) / __PLLSAI2Q__ 00656 * 00657 * @retval None 00658 */ 00659 #define __HAL_RCC_PLLSAI2_DIVR_CONFIG(__PLLSAI2R__) \ 00660 MODIFY_REG(RCC->PLLSAI2CFGR, RCC_PLLSAI2CFGR_PLLSAI2R, (((__PLLSAI2R__) >> 1U) - 1) << 25U) 00661 00662 /** 00663 * @brief Macros to enable or disable the PLLSAI2. 00664 * @note The PLLSAI2 is disabled by hardware when entering STOP and STANDBY modes. 00665 * @retval None 00666 */ 00667 00668 #define __HAL_RCC_PLLSAI2_ENABLE() SET_BIT(RCC->CR, RCC_CR_PLLSAI2ON) 00669 00670 #define __HAL_RCC_PLLSAI2_DISABLE() CLEAR_BIT(RCC->CR, RCC_CR_PLLSAI2ON) 00671 00672 /** 00673 * @brief Macros to enable or disable each clock output (PLLSAI2_SAI2 and PLLSAI2_ADC2). 00674 * @note Enabling and disabling those clocks can be done without the need to stop the PLL. 00675 * This is mainly used to save Power. 00676 * @param __PLLSAI2_CLOCKOUT__ specifies the PLLSAI2 clock to be output. 00677 * This parameter can be one or a combination of the following values: 00678 * @arg @ref RCC_PLLSAI2_SAI2CLK This clock is used to generate an accurate clock to achieve 00679 * high-quality audio performance on SAI interface in case. 00680 * @arg @ref RCC_PLLSAI2_ADC2CLK Clock used to clock ADC peripheral. 00681 * @retval None 00682 */ 00683 00684 #define __HAL_RCC_PLLSAI2CLKOUT_ENABLE(__PLLSAI2_CLOCKOUT__) SET_BIT(RCC->PLLSAI2CFGR, (__PLLSAI2_CLOCKOUT__)) 00685 00686 #define __HAL_RCC_PLLSAI2CLKOUT_DISABLE(__PLLSAI2_CLOCKOUT__) CLEAR_BIT(RCC->PLLSAI2CFGR, (__PLLSAI2_CLOCKOUT__)) 00687 00688 /** 00689 * @brief Macro to get clock output enable status (PLLSAI2_SAI2 and PLLSAI2_ADC2). 00690 * @param __PLLSAI2_CLOCKOUT__ specifies the PLLSAI2 clock to be output. 00691 * This parameter can be one of the following values: 00692 * @arg @ref RCC_PLLSAI2_SAI2CLK This clock is used to generate an accurate clock to achieve 00693 * high-quality audio performance on SAI interface in case. 00694 * @arg @ref RCC_PLLSAI2_ADC2CLK Clock used to clock ADC peripheral. 00695 * @retval SET / RESET 00696 */ 00697 #define __HAL_RCC_GET_PLLSAI2CLKOUT_CONFIG(__PLLSAI2_CLOCKOUT__) READ_BIT(RCC->PLLSAI2CFGR, (__PLLSAI2_CLOCKOUT__)) 00698 00699 /** 00700 * @brief Macro to configure the SAI1 clock source. 00701 * @param __SAI1_CLKSOURCE__ defines the SAI1 clock source. This clock is derived 00702 * from the PLLSAI1, system PLL or external clock (through a dedicated pin). 00703 * This parameter can be one of the following values: 00704 * @arg @ref RCC_SAI1CLKSOURCE_PLLSAI1 SAI1 clock = PLLSAI1 "P" clock (PLLSAI1CLK) 00705 * @arg @ref RCC_SAI1CLKSOURCE_PLLSAI2 SAI1 clock = PLLSAI2 "P" clock (PLLSAI2CLK) 00706 * @arg @ref RCC_SAI1CLKSOURCE_PLL SAI1 clock = PLL "P" clock (PLLSAI3CLK) 00707 * @arg @ref RCC_SAI1CLKSOURCE_PIN SAI1 clock = External Clock (SAI1_EXTCLK) 00708 * 00709 * @retval None 00710 */ 00711 #define __HAL_RCC_SAI1_CONFIG(__SAI1_CLKSOURCE__)\ 00712 MODIFY_REG(RCC->CCIPR, RCC_CCIPR_SAI1SEL, (uint32_t)(__SAI1_CLKSOURCE__)) 00713 00714 /** @brief Macro to get the SAI1 clock source. 00715 * @retval The clock source can be one of the following values: 00716 * @arg @ref RCC_SAI1CLKSOURCE_PLLSAI1 SAI1 clock = PLLSAI1 "P" clock (PLLSAI1CLK) 00717 * @arg @ref RCC_SAI1CLKSOURCE_PLLSAI2 SAI1 clock = PLLSAI2 "P" clock (PLLSAI2CLK) 00718 * @arg @ref RCC_SAI1CLKSOURCE_PLL SAI1 clock = PLL "P" clock (PLLSAI3CLK) 00719 * @arg @ref RCC_SAI1CLKSOURCE_PIN SAI1 clock = External Clock (SAI1_EXTCLK) 00720 */ 00721 #define __HAL_RCC_GET_SAI1_SOURCE() ((uint32_t)(READ_BIT(RCC->CCIPR, RCC_CCIPR_SAI1SEL))) 00722 00723 /** 00724 * @brief Macro to configure the SAI2 clock source. 00725 * @param __SAI2_CLKSOURCE__ defines the SAI2 clock source. This clock is derived 00726 * from the PLLSAI2, system PLL or external clock (through a dedicated pin). 00727 * This parameter can be one of the following values: 00728 * @arg @ref RCC_SAI2CLKSOURCE_PLLSAI1 SAI2 clock = PLLSAI1 "P" clock (PLLSAI1CLK) 00729 * @arg @ref RCC_SAI2CLKSOURCE_PLLSAI2 SAI2 clock = PLLSAI2 "P" clock (PLLSAI2CLK) 00730 * @arg @ref RCC_SAI2CLKSOURCE_PLL SAI2 clock = PLL "P" clock (PLLSAI3CLK) 00731 * @arg @ref RCC_SAI2CLKSOURCE_PIN SAI2 clock = External Clock (SAI2_EXTCLK) 00732 * @retval None 00733 */ 00734 #define __HAL_RCC_SAI2_CONFIG(__SAI2_CLKSOURCE__ )\ 00735 MODIFY_REG(RCC->CCIPR, RCC_CCIPR_SAI2SEL, (uint32_t)(__SAI2_CLKSOURCE__)) 00736 00737 /** @brief Macro to get the SAI2 clock source. 00738 * @retval The clock source can be one of the following values: 00739 * @arg @ref RCC_SAI2CLKSOURCE_PLLSAI1 SAI2 clock = PLLSAI1 "P" clock (PLLSAI1CLK) 00740 * @arg @ref RCC_SAI2CLKSOURCE_PLLSAI2 SAI2 clock = PLLSAI2 "P" clock (PLLSAI2CLK) 00741 * @arg @ref RCC_SAI2CLKSOURCE_PLL SAI2 clock = PLL "P" clock (PLLSAI3CLK) 00742 * @arg @ref RCC_SAI2CLKSOURCE_PIN SAI2 clock = External Clock (SAI2_EXTCLK) 00743 */ 00744 #define __HAL_RCC_GET_SAI2_SOURCE() ((uint32_t)(READ_BIT(RCC->CCIPR, RCC_CCIPR_SAI2SEL))) 00745 00746 /** @brief Macro to configure the I2C1 clock (I2C1CLK). 00747 * 00748 * @param __I2C1_CLKSOURCE__ specifies the I2C1 clock source. 00749 * This parameter can be one of the following values: 00750 * @arg @ref RCC_I2C1CLKSOURCE_PCLK1 PCLK1 selected as I2C1 clock 00751 * @arg @ref RCC_I2C1CLKSOURCE_HSI HSI selected as I2C1 clock 00752 * @arg @ref RCC_I2C1CLKSOURCE_SYSCLK System Clock selected as I2C1 clock 00753 * @retval None 00754 */ 00755 #define __HAL_RCC_I2C1_CONFIG(__I2C1_CLKSOURCE__) \ 00756 MODIFY_REG(RCC->CCIPR, RCC_CCIPR_I2C1SEL, (uint32_t)(__I2C1_CLKSOURCE__)) 00757 00758 /** @brief Macro to get the I2C1 clock source. 00759 * @retval The clock source can be one of the following values: 00760 * @arg @ref RCC_I2C1CLKSOURCE_PCLK1 PCLK1 selected as I2C1 clock 00761 * @arg @ref RCC_I2C1CLKSOURCE_HSI HSI selected as I2C1 clock 00762 * @arg @ref RCC_I2C1CLKSOURCE_SYSCLK System Clock selected as I2C1 clock 00763 */ 00764 #define __HAL_RCC_GET_I2C1_SOURCE() ((uint32_t)(READ_BIT(RCC->CCIPR, RCC_CCIPR_I2C1SEL))) 00765 00766 /** @brief Macro to configure the I2C2 clock (I2C2CLK). 00767 * 00768 * @param __I2C2_CLKSOURCE__ specifies the I2C2 clock source. 00769 * This parameter can be one of the following values: 00770 * @arg @ref RCC_I2C2CLKSOURCE_PCLK1 PCLK1 selected as I2C2 clock 00771 * @arg @ref RCC_I2C2CLKSOURCE_HSI HSI selected as I2C2 clock 00772 * @arg @ref RCC_I2C2CLKSOURCE_SYSCLK System Clock selected as I2C2 clock 00773 * @retval None 00774 */ 00775 #define __HAL_RCC_I2C2_CONFIG(__I2C2_CLKSOURCE__) \ 00776 MODIFY_REG(RCC->CCIPR, RCC_CCIPR_I2C2SEL, (uint32_t)(__I2C2_CLKSOURCE__)) 00777 00778 /** @brief Macro to get the I2C2 clock source. 00779 * @retval The clock source can be one of the following values: 00780 * @arg @ref RCC_I2C2CLKSOURCE_PCLK1 PCLK1 selected as I2C2 clock 00781 * @arg @ref RCC_I2C2CLKSOURCE_HSI HSI selected as I2C2 clock 00782 * @arg @ref RCC_I2C2CLKSOURCE_SYSCLK System Clock selected as I2C2 clock 00783 */ 00784 #define __HAL_RCC_GET_I2C2_SOURCE() ((uint32_t)(READ_BIT(RCC->CCIPR, RCC_CCIPR_I2C2SEL))) 00785 00786 /** @brief Macro to configure the I2C3 clock (I2C3CLK). 00787 * 00788 * @param __I2C3_CLKSOURCE__ specifies the I2C3 clock source. 00789 * This parameter can be one of the following values: 00790 * @arg @ref RCC_I2C3CLKSOURCE_PCLK1 PCLK1 selected as I2C3 clock 00791 * @arg @ref RCC_I2C3CLKSOURCE_HSI HSI selected as I2C3 clock 00792 * @arg @ref RCC_I2C3CLKSOURCE_SYSCLK System Clock selected as I2C3 clock 00793 * @retval None 00794 */ 00795 #define __HAL_RCC_I2C3_CONFIG(__I2C3_CLKSOURCE__) \ 00796 MODIFY_REG(RCC->CCIPR, RCC_CCIPR_I2C3SEL, (uint32_t)(__I2C3_CLKSOURCE__)) 00797 00798 /** @brief Macro to get the I2C3 clock source. 00799 * @retval The clock source can be one of the following values: 00800 * @arg @ref RCC_I2C3CLKSOURCE_PCLK1 PCLK1 selected as I2C3 clock 00801 * @arg @ref RCC_I2C3CLKSOURCE_HSI HSI selected as I2C3 clock 00802 * @arg @ref RCC_I2C3CLKSOURCE_SYSCLK System Clock selected as I2C3 clock 00803 */ 00804 #define __HAL_RCC_GET_I2C3_SOURCE() ((uint32_t)(READ_BIT(RCC->CCIPR, RCC_CCIPR_I2C3SEL))) 00805 00806 /** @brief Macro to configure the USART1 clock (USART1CLK). 00807 * 00808 * @param __USART1_CLKSOURCE__ specifies the USART1 clock source. 00809 * This parameter can be one of the following values: 00810 * @arg @ref RCC_USART1CLKSOURCE_PCLK2 PCLK2 selected as USART1 clock 00811 * @arg @ref RCC_USART1CLKSOURCE_HSI HSI selected as USART1 clock 00812 * @arg @ref RCC_USART1CLKSOURCE_SYSCLK System Clock selected as USART1 clock 00813 * @arg @ref RCC_USART1CLKSOURCE_LSE LSE selected as USART1 clock 00814 * @retval None 00815 */ 00816 #define __HAL_RCC_USART1_CONFIG(__USART1_CLKSOURCE__) \ 00817 MODIFY_REG(RCC->CCIPR, RCC_CCIPR_USART1SEL, (uint32_t)(__USART1_CLKSOURCE__)) 00818 00819 /** @brief Macro to get the USART1 clock source. 00820 * @retval The clock source can be one of the following values: 00821 * @arg @ref RCC_USART1CLKSOURCE_PCLK2 PCLK2 selected as USART1 clock 00822 * @arg @ref RCC_USART1CLKSOURCE_HSI HSI selected as USART1 clock 00823 * @arg @ref RCC_USART1CLKSOURCE_SYSCLK System Clock selected as USART1 clock 00824 * @arg @ref RCC_USART1CLKSOURCE_LSE LSE selected as USART1 clock 00825 */ 00826 #define __HAL_RCC_GET_USART1_SOURCE() ((uint32_t)(READ_BIT(RCC->CCIPR, RCC_CCIPR_USART1SEL))) 00827 00828 /** @brief Macro to configure the USART2 clock (USART2CLK). 00829 * 00830 * @param __USART2_CLKSOURCE__ specifies the USART2 clock source. 00831 * This parameter can be one of the following values: 00832 * @arg @ref RCC_USART2CLKSOURCE_PCLK1 PCLK1 selected as USART2 clock 00833 * @arg @ref RCC_USART2CLKSOURCE_HSI HSI selected as USART2 clock 00834 * @arg @ref RCC_USART2CLKSOURCE_SYSCLK System Clock selected as USART2 clock 00835 * @arg @ref RCC_USART2CLKSOURCE_LSE LSE selected as USART2 clock 00836 * @retval None 00837 */ 00838 #define __HAL_RCC_USART2_CONFIG(__USART2_CLKSOURCE__) \ 00839 MODIFY_REG(RCC->CCIPR, RCC_CCIPR_USART2SEL, (uint32_t)(__USART2_CLKSOURCE__)) 00840 00841 /** @brief Macro to get the USART2 clock source. 00842 * @retval The clock source can be one of the following values: 00843 * @arg @ref RCC_USART2CLKSOURCE_PCLK1 PCLK1 selected as USART2 clock 00844 * @arg @ref RCC_USART2CLKSOURCE_HSI HSI selected as USART2 clock 00845 * @arg @ref RCC_USART2CLKSOURCE_SYSCLK System Clock selected as USART2 clock 00846 * @arg @ref RCC_USART2CLKSOURCE_LSE LSE selected as USART2 clock 00847 */ 00848 #define __HAL_RCC_GET_USART2_SOURCE() ((uint32_t)(READ_BIT(RCC->CCIPR, RCC_CCIPR_USART2SEL))) 00849 00850 /** @brief Macro to configure the USART3 clock (USART3CLK). 00851 * 00852 * @param __USART3_CLKSOURCE__ specifies the USART3 clock source. 00853 * This parameter can be one of the following values: 00854 * @arg @ref RCC_USART3CLKSOURCE_PCLK1 PCLK1 selected as USART3 clock 00855 * @arg @ref RCC_USART3CLKSOURCE_HSI HSI selected as USART3 clock 00856 * @arg @ref RCC_USART3CLKSOURCE_SYSCLK System Clock selected as USART3 clock 00857 * @arg @ref RCC_USART3CLKSOURCE_LSE LSE selected as USART3 clock 00858 * @retval None 00859 */ 00860 #define __HAL_RCC_USART3_CONFIG(__USART3_CLKSOURCE__) \ 00861 MODIFY_REG(RCC->CCIPR, RCC_CCIPR_USART3SEL, (uint32_t)(__USART3_CLKSOURCE__)) 00862 00863 /** @brief Macro to get the USART3 clock source. 00864 * @retval The clock source can be one of the following values: 00865 * @arg @ref RCC_USART3CLKSOURCE_PCLK1 PCLK1 selected as USART3 clock 00866 * @arg @ref RCC_USART3CLKSOURCE_HSI HSI selected as USART3 clock 00867 * @arg @ref RCC_USART3CLKSOURCE_SYSCLK System Clock selected as USART3 clock 00868 * @arg @ref RCC_USART3CLKSOURCE_LSE LSE selected as USART3 clock 00869 */ 00870 #define __HAL_RCC_GET_USART3_SOURCE() ((uint32_t)(READ_BIT(RCC->CCIPR, RCC_CCIPR_USART3SEL))) 00871 00872 /** @brief Macro to configure the UART4 clock (UART4CLK). 00873 * 00874 * @param __UART4_CLKSOURCE__ specifies the UART4 clock source. 00875 * This parameter can be one of the following values: 00876 * @arg @ref RCC_UART4CLKSOURCE_PCLK1 PCLK1 selected as UART4 clock 00877 * @arg @ref RCC_UART4CLKSOURCE_HSI HSI selected as UART4 clock 00878 * @arg @ref RCC_UART4CLKSOURCE_SYSCLK System Clock selected as UART4 clock 00879 * @arg @ref RCC_UART4CLKSOURCE_LSE LSE selected as UART4 clock 00880 * @retval None 00881 */ 00882 #define __HAL_RCC_UART4_CONFIG(__UART4_CLKSOURCE__) \ 00883 MODIFY_REG(RCC->CCIPR, RCC_CCIPR_UART4SEL, (uint32_t)(__UART4_CLKSOURCE__)) 00884 00885 /** @brief Macro to get the UART4 clock source. 00886 * @retval The clock source can be one of the following values: 00887 * @arg @ref RCC_UART4CLKSOURCE_PCLK1 PCLK1 selected as UART4 clock 00888 * @arg @ref RCC_UART4CLKSOURCE_HSI HSI selected as UART4 clock 00889 * @arg @ref RCC_UART4CLKSOURCE_SYSCLK System Clock selected as UART4 clock 00890 * @arg @ref RCC_UART4CLKSOURCE_LSE LSE selected as UART4 clock 00891 */ 00892 #define __HAL_RCC_GET_UART4_SOURCE() ((uint32_t)(READ_BIT(RCC->CCIPR, RCC_CCIPR_UART4SEL))) 00893 00894 /** @brief Macro to configure the UART5 clock (UART5CLK). 00895 * 00896 * @param __UART5_CLKSOURCE__ specifies the UART5 clock source. 00897 * This parameter can be one of the following values: 00898 * @arg @ref RCC_UART5CLKSOURCE_PCLK1 PCLK1 selected as UART5 clock 00899 * @arg @ref RCC_UART5CLKSOURCE_HSI HSI selected as UART5 clock 00900 * @arg @ref RCC_UART5CLKSOURCE_SYSCLK System Clock selected as UART5 clock 00901 * @arg @ref RCC_UART5CLKSOURCE_LSE LSE selected as UART5 clock 00902 * @retval None 00903 */ 00904 #define __HAL_RCC_UART5_CONFIG(__UART5_CLKSOURCE__) \ 00905 MODIFY_REG(RCC->CCIPR, RCC_CCIPR_UART5SEL, (uint32_t)(__UART5_CLKSOURCE__)) 00906 00907 /** @brief Macro to get the UART5 clock source. 00908 * @retval The clock source can be one of the following values: 00909 * @arg @ref RCC_UART5CLKSOURCE_PCLK1 PCLK1 selected as UART5 clock 00910 * @arg @ref RCC_UART5CLKSOURCE_HSI HSI selected as UART5 clock 00911 * @arg @ref RCC_UART5CLKSOURCE_SYSCLK System Clock selected as UART5 clock 00912 * @arg @ref RCC_UART5CLKSOURCE_LSE LSE selected as UART5 clock 00913 */ 00914 #define __HAL_RCC_GET_UART5_SOURCE() ((uint32_t)(READ_BIT(RCC->CCIPR, RCC_CCIPR_UART5SEL))) 00915 00916 /** @brief Macro to configure the LPUART1 clock (LPUART1CLK). 00917 * 00918 * @param __LPUART1_CLKSOURCE__ specifies the LPUART1 clock source. 00919 * This parameter can be one of the following values: 00920 * @arg @ref RCC_LPUART1CLKSOURCE_PCLK1 PCLK1 selected as LPUART1 clock 00921 * @arg @ref RCC_LPUART1CLKSOURCE_HSI HSI selected as LPUART1 clock 00922 * @arg @ref RCC_LPUART1CLKSOURCE_SYSCLK System Clock selected as LPUART1 clock 00923 * @arg @ref RCC_LPUART1CLKSOURCE_LSE LSE selected as LPUART1 clock 00924 * @retval None 00925 */ 00926 #define __HAL_RCC_LPUART1_CONFIG(__LPUART1_CLKSOURCE__) \ 00927 MODIFY_REG(RCC->CCIPR, RCC_CCIPR_LPUART1SEL, (uint32_t)(__LPUART1_CLKSOURCE__)) 00928 00929 /** @brief Macro to get the LPUART1 clock source. 00930 * @retval The clock source can be one of the following values: 00931 * @arg @ref RCC_LPUART1CLKSOURCE_PCLK1 PCLK1 selected as LPUART1 clock 00932 * @arg @ref RCC_LPUART1CLKSOURCE_HSI HSI selected as LPUART1 clock 00933 * @arg @ref RCC_LPUART1CLKSOURCE_SYSCLK System Clock selected as LPUART1 clock 00934 * @arg @ref RCC_LPUART1CLKSOURCE_LSE LSE selected as LPUART1 clock 00935 */ 00936 #define __HAL_RCC_GET_LPUART1_SOURCE() ((uint32_t)(READ_BIT(RCC->CCIPR, RCC_CCIPR_LPUART1SEL))) 00937 00938 /** @brief Macro to configure the LPTIM1 clock (LPTIM1CLK). 00939 * 00940 * @param __LPTIM1_CLKSOURCE__ specifies the LPTIM1 clock source. 00941 * This parameter can be one of the following values: 00942 * @arg @ref RCC_LPTIM1CLKSOURCE_PCLK PCLK selected as LPTIM1 clock 00943 * @arg @ref RCC_LPTIM1CLKSOURCE_LSI HSI selected as LPTIM1 clock 00944 * @arg @ref RCC_LPTIM1CLKSOURCE_HSI LSI selected as LPTIM1 clock 00945 * @arg @ref RCC_LPTIM1CLKSOURCE_LSE LSE selected as LPTIM1 clock 00946 * @retval None 00947 */ 00948 #define __HAL_RCC_LPTIM1_CONFIG(__LPTIM1_CLKSOURCE__) \ 00949 MODIFY_REG(RCC->CCIPR, RCC_CCIPR_LPTIM1SEL, (uint32_t)(__LPTIM1_CLKSOURCE__)) 00950 00951 /** @brief Macro to get the LPTIM1 clock source. 00952 * @retval The clock source can be one of the following values: 00953 * @arg @ref RCC_LPTIM1CLKSOURCE_PCLK PCLK selected as LPUART1 clock 00954 * @arg @ref RCC_LPTIM1CLKSOURCE_LSI HSI selected as LPUART1 clock 00955 * @arg @ref RCC_LPTIM1CLKSOURCE_HSI System Clock selected as LPUART1 clock 00956 * @arg @ref RCC_LPTIM1CLKSOURCE_LSE LSE selected as LPUART1 clock 00957 */ 00958 #define __HAL_RCC_GET_LPTIM1_SOURCE() ((uint32_t)(READ_BIT(RCC->CCIPR, RCC_CCIPR_LPTIM1SEL))) 00959 00960 /** @brief Macro to configure the LPTIM2 clock (LPTIM2CLK). 00961 * 00962 * @param __LPTIM2_CLKSOURCE__ specifies the LPTIM2 clock source. 00963 * This parameter can be one of the following values: 00964 * @arg @ref RCC_LPTIM2CLKSOURCE_PCLK PCLK selected as LPTIM2 clock 00965 * @arg @ref RCC_LPTIM2CLKSOURCE_LSI HSI selected as LPTIM2 clock 00966 * @arg @ref RCC_LPTIM2CLKSOURCE_HSI LSI selected as LPTIM2 clock 00967 * @arg @ref RCC_LPTIM2CLKSOURCE_LSE LSE selected as LPTIM2 clock 00968 * @retval None 00969 */ 00970 #define __HAL_RCC_LPTIM2_CONFIG(__LPTIM2_CLKSOURCE__) \ 00971 MODIFY_REG(RCC->CCIPR, RCC_CCIPR_LPTIM2SEL, (uint32_t)(__LPTIM2_CLKSOURCE__)) 00972 00973 /** @brief Macro to get the LPTIM2 clock source. 00974 * @retval The clock source can be one of the following values: 00975 * @arg @ref RCC_LPTIM2CLKSOURCE_PCLK PCLK selected as LPUART1 clock 00976 * @arg @ref RCC_LPTIM2CLKSOURCE_LSI HSI selected as LPUART1 clock 00977 * @arg @ref RCC_LPTIM2CLKSOURCE_HSI System Clock selected as LPUART1 clock 00978 * @arg @ref RCC_LPTIM2CLKSOURCE_LSE LSE selected as LPUART1 clock 00979 */ 00980 #define __HAL_RCC_GET_LPTIM2_SOURCE() ((uint32_t)(READ_BIT(RCC->CCIPR, RCC_CCIPR_LPTIM2SEL))) 00981 00982 /** @brief Macro to configure the SDMMC1 clock. 00983 * 00984 * @note USB, RNG and SDMMC1 peripherals share the same 48MHz clock source. 00985 * 00986 * @param __SDMMC1_CLKSOURCE__ specifies the SDMMC1 clock source. 00987 * This parameter can be one of the following values: 00988 * @arg @ref RCC_SDMMC1CLKSOURCE_NONE No clock selected as SDMMC1 clock 00989 * @arg @ref RCC_SDMMC1CLKSOURCE_MSI MSI selected as SDMMC1 clock 00990 * @arg @ref RCC_SDMMC1CLKSOURCE_PLLSAI1 PLLSAI1 Clock selected as SDMMC1 clock 00991 * @arg @ref RCC_SDMMC1CLKSOURCE_PLL PLL Clock selected as SDMMC1 clock 00992 * @retval None 00993 */ 00994 #define __HAL_RCC_SDMMC1_CONFIG(__SDMMC1_CLKSOURCE__) \ 00995 MODIFY_REG(RCC->CCIPR, RCC_CCIPR_CLK48SEL, (uint32_t)(__SDMMC1_CLKSOURCE__)) 00996 00997 /** @brief Macro to get the SDMMC1 clock. 00998 * @retval The clock source can be one of the following values: 00999 * @arg @ref RCC_SDMMC1CLKSOURCE_NONE No clock selected as SDMMC1 clock 01000 * @arg @ref RCC_SDMMC1CLKSOURCE_MSI MSI selected as SDMMC1 clock 01001 * @arg @ref RCC_SDMMC1CLKSOURCE_PLLSAI1 PLLSAI1 "Q" clock (PLL48M2CLK) selected as SDMMC1 clock 01002 * @arg @ref RCC_SDMMC1CLKSOURCE_PLL PLL "Q" clock (PLL48M1CLK) selected as SDMMC1 clock 01003 */ 01004 #define __HAL_RCC_GET_SDMMC1_SOURCE() ((uint32_t)(READ_BIT(RCC->CCIPR, RCC_CCIPR_CLK48SEL))) 01005 01006 /** @brief Macro to configure the RNG clock. 01007 * 01008 * @note USB, RNG and SDMMC1 peripherals share the same 48MHz clock source. 01009 * 01010 * @param __RNG_CLKSOURCE__ specifies the RNG clock source. 01011 * This parameter can be one of the following values: 01012 * @arg @ref RCC_RNGCLKSOURCE_NONE No clock selected as RNG clock 01013 * @arg @ref RCC_RNGCLKSOURCE_MSI MSI selected as RNG clock 01014 * @arg @ref RCC_RNGCLKSOURCE_PLLSAI1 PLLSAI1 Clock selected as RNG clock 01015 * @arg @ref RCC_RNGCLKSOURCE_PLL PLL Clock selected as RNG clock 01016 * @retval None 01017 */ 01018 #define __HAL_RCC_RNG_CONFIG(__RNG_CLKSOURCE__) \ 01019 MODIFY_REG(RCC->CCIPR, RCC_CCIPR_CLK48SEL, (uint32_t)(__RNG_CLKSOURCE__)) 01020 01021 /** @brief Macro to get the RNG clock. 01022 * @retval The clock source can be one of the following values: 01023 * @arg @ref RCC_RNGCLKSOURCE_NONE No clock selected as RNG clock 01024 * @arg @ref RCC_RNGCLKSOURCE_MSI MSI selected as RNG clock 01025 * @arg @ref RCC_RNGCLKSOURCE_PLLSAI1 PLLSAI1 "Q" clock (PLL48M2CLK) selected as RNG clock 01026 * @arg @ref RCC_RNGCLKSOURCE_PLL PLL "Q" clock (PLL48M1CLK) selected as RNG clock 01027 */ 01028 #define __HAL_RCC_GET_RNG_SOURCE() ((uint32_t)(READ_BIT(RCC->CCIPR, RCC_CCIPR_CLK48SEL))) 01029 01030 #if defined(STM32L475xx) || defined(STM32L476xx) || defined(STM32L485xx) || defined(STM32L486xx) 01031 /** @brief Macro to configure the USB clock (USBCLK). 01032 * 01033 * @note USB, RNG and SDMMC1 peripherals share the same 48MHz clock source. 01034 * 01035 * @param __USB_CLKSOURCE__ specifies the USB clock source. 01036 * This parameter can be one of the following values: 01037 * @arg @ref RCC_USBCLKSOURCE_NONE No clock selected 01038 * @arg @ref RCC_USBCLKSOURCE_MSI MSI selected as USB clock 01039 * @arg @ref RCC_USBCLKSOURCE_PLLSAI1 PLLSAI1 "Q" clock (PLL48M2CLK) selected as USB clock 01040 * @arg @ref RCC_USBCLKSOURCE_PLL PLL "Q" clock (PLL48M1CLK) selected as USB clock 01041 * @retval None 01042 */ 01043 #define __HAL_RCC_USB_CONFIG(__USB_CLKSOURCE__) \ 01044 MODIFY_REG(RCC->CCIPR, RCC_CCIPR_CLK48SEL, (uint32_t)(__USB_CLKSOURCE__)) 01045 01046 /** @brief Macro to get the USB clock source. 01047 * @retval The clock source can be one of the following values: 01048 * @arg @ref RCC_USBCLKSOURCE_NONE No clock selected 01049 * @arg @ref RCC_USBCLKSOURCE_MSI MSI selected as USB clock 01050 * @arg @ref RCC_USBCLKSOURCE_PLLSAI1 PLLSAI1 "Q" clock (PLL48M2CLK) selected as USB clock 01051 * @arg @ref RCC_USBCLKSOURCE_PLL PLL "Q" clock (PLL48M1CLK) selected as USB clock 01052 */ 01053 #define __HAL_RCC_GET_USB_SOURCE() ((uint32_t)(READ_BIT(RCC->CCIPR, RCC_CCIPR_CLK48SEL))) 01054 01055 #endif /* STM32L475xx || STM32L476xx || STM32L485xx || STM32L486xx */ 01056 01057 /** @brief Macro to configure the ADC interface clock. 01058 * @param __ADC_CLKSOURCE__ specifies the ADC digital interface clock source. 01059 * This parameter can be one of the following values: 01060 * @arg @ref RCC_ADCCLKSOURCE_NONE No clock selected as ADC clock 01061 * @arg @ref RCC_ADCCLKSOURCE_PLLSAI1 PLLSAI1 Clock selected as ADC clock 01062 * @arg @ref RCC_ADCCLKSOURCE_PLLSAI2 PLLSAI2 Clock selected as ADC clock 01063 * @arg @ref RCC_ADCCLKSOURCE_SYSCLK System Clock selected as ADC clock 01064 * @retval None 01065 */ 01066 #define __HAL_RCC_ADC_CONFIG(__ADC_CLKSOURCE__) \ 01067 MODIFY_REG(RCC->CCIPR, RCC_CCIPR_ADCSEL, (uint32_t)(__ADC_CLKSOURCE__)) 01068 01069 /** @brief Macro to get the ADC clock source. 01070 * @retval The clock source can be one of the following values: 01071 * @arg @ref RCC_ADCCLKSOURCE_NONE No clock selected as ADC clock 01072 * @arg @ref RCC_ADCCLKSOURCE_PLLSAI1 PLLSAI1 Clock selected as ADC clock 01073 * @arg @ref RCC_ADCCLKSOURCE_PLLSAI2 PLLSAI2 Clock selected as ADC clock 01074 * @arg @ref RCC_ADCCLKSOURCE_SYSCLK System Clock selected as ADC clock 01075 */ 01076 #define __HAL_RCC_GET_ADC_SOURCE() ((uint32_t)(READ_BIT(RCC->CCIPR, RCC_CCIPR_ADCSEL))) 01077 01078 /** @brief Macro to configure the SWPMI1 clock. 01079 * @param __SWPMI1_CLKSOURCE__ specifies the SWPMI1 clock source. 01080 * This parameter can be one of the following values: 01081 * @arg @ref RCC_SWPMI1CLKSOURCE_PCLK PCLK Clock selected as SWPMI1 clock 01082 * @arg @ref RCC_SWPMI1CLKSOURCE_HSI HSI Clock selected as SWPMI1 clock 01083 * @retval None 01084 */ 01085 #define __HAL_RCC_SWPMI1_CONFIG(__SWPMI1_CLKSOURCE__) \ 01086 MODIFY_REG(RCC->CCIPR, RCC_CCIPR_SWPMI1SEL, (uint32_t)(__SWPMI1_CLKSOURCE__)) 01087 01088 /** @brief Macro to get the SWPMI1 clock source. 01089 * @retval The clock source can be one of the following values: 01090 * @arg @ref RCC_SWPMI1CLKSOURCE_PCLK PCLK Clock selected as SWPMI1 clock 01091 * @arg @ref RCC_SWPMI1CLKSOURCE_HSI HSI Clock selected as SWPMI1 clock 01092 */ 01093 #define __HAL_RCC_GET_SWPMI1_SOURCE() ((uint32_t)(READ_BIT(RCC->CCIPR, RCC_CCIPR_SWPMI1SEL))) 01094 01095 /** @brief Macro to configure the DFSDM clock. 01096 * @param __DFSDM_CLKSOURCE__ specifies the DFSDM clock source. 01097 * This parameter can be one of the following values: 01098 * @arg @ref RCC_DFSDMCLKSOURCE_PCLK PCLK Clock selected as DFSDM clock 01099 * @arg @ref RCC_DFSDMCLKSOURCE_SYSCLK System Clock selected as DFSDM clock 01100 * @retval None 01101 */ 01102 #define __HAL_RCC_DFSDM_CONFIG(__DFSDM_CLKSOURCE__) \ 01103 MODIFY_REG(RCC->CCIPR, RCC_CCIPR_DFSDMSEL, (uint32_t)(__DFSDM_CLKSOURCE__)) 01104 01105 /** @brief Macro to get the DFSDM clock source. 01106 * @retval The clock source can be one of the following values: 01107 * @arg @ref RCC_DFSDMCLKSOURCE_PCLK PCLK Clock selected as DFSDM clock 01108 * @arg @ref RCC_DFSDMCLKSOURCE_SYSCLK System Clock selected as DFSDM clock 01109 */ 01110 #define __HAL_RCC_GET_DFSDM_SOURCE() ((uint32_t)(READ_BIT(RCC->CCIPR, RCC_CCIPR_DFSDMSEL))) 01111 01112 /** @defgroup RCCEx_Flags_Interrupts_Management Flags Interrupts Management 01113 * @brief macros to manage the specified RCC Flags and interrupts. 01114 * @{ 01115 */ 01116 01117 /** @brief Enable PLLSAI1RDY interrupt. 01118 * @retval None 01119 */ 01120 #define __HAL_RCC_PLLSAI1_ENABLE_IT() SET_BIT(RCC->CIER, RCC_CIER_PLLSAI1RDYIE) 01121 01122 /** @brief Disable PLLSAI1RDY interrupt. 01123 * @retval None 01124 */ 01125 #define __HAL_RCC_PLLSAI1_DISABLE_IT() CLEAR_BIT(RCC->CIER, RCC_CIER_PLLSAI1RDYIE) 01126 01127 /** @brief Clear the PLLSAI1RDY interrupt pending bit. 01128 * @retval None 01129 */ 01130 #define __HAL_RCC_PLLSAI1_CLEAR_IT() WRITE_REG(RCC->CICR, RCC_CICR_PLLSAI1RDYC) 01131 01132 /** @brief Check whether PLLSAI1RDY interrupt has occurred or not. 01133 * @retval TRUE or FALSE. 01134 */ 01135 #define __HAL_RCC_PLLSAI1_GET_IT_SOURCE() (READ_BIT(RCC->CIFR, RCC_CIFR_PLLSAI1RDYF) == RCC_CIFR_PLLSAI1RDYF) 01136 01137 /** @brief Check whether the PLLSAI1RDY flag is set or not. 01138 * @retval TRUE or FALSE. 01139 */ 01140 #define __HAL_RCC_PLLSAI1_GET_FLAG() (READ_BIT(RCC->CR, RCC_CR_PLLSAI1RDY) == (RCC_CR_PLLSAI1RDY)) 01141 01142 /** @brief Enable PLLSAI2RDY interrupt. 01143 * @retval None 01144 */ 01145 #define __HAL_RCC_PLLSAI2_ENABLE_IT() SET_BIT(RCC->CIER, RCC_CIER_PLLSAI2RDYIE) 01146 01147 /** @brief Disable PLLSAI2RDY interrupt. 01148 * @retval None 01149 */ 01150 #define __HAL_RCC_PLLSAI2_DISABLE_IT() CLEAR_BIT(RCC->CIER, RCC_CIER_PLLSAI2RDYIE) 01151 01152 /** @brief Clear the PLLSAI2RDY interrupt pending bit. 01153 * @retval None 01154 */ 01155 #define __HAL_RCC_PLLSAI2_CLEAR_IT() WRITE_REG(RCC->CICR, RCC_CICR_PLLSAI2RDYC) 01156 01157 /** @brief Check whether the PLLSAI2RDY interrupt has occurred or not. 01158 * @retval TRUE or FALSE. 01159 */ 01160 #define __HAL_RCC_PLLSAI2_GET_IT_SOURCE() (READ_BIT(RCC->CIFR, RCC_CIFR_PLLSAI2RDYF) == RCC_CIFR_PLLSAI2RDYF) 01161 01162 /** @brief Check whether the PLLSAI2RDY flag is set or not. 01163 * @retval TRUE or FALSE. 01164 */ 01165 #define __HAL_RCC_PLLSAI2_GET_FLAG() (READ_BIT(RCC->CR, RCC_CR_PLLSAI2RDY) == (RCC_CR_PLLSAI2RDY)) 01166 01167 01168 /** 01169 * @brief Enable the RCC LSE CSS Extended Interrupt Line. 01170 * @retval None 01171 */ 01172 #define __HAL_RCC_LSECSS_EXTI_ENABLE_IT() SET_BIT(EXTI->IMR1, RCC_EXTI_LINE_LSECSS) 01173 01174 /** 01175 * @brief Disable the RCC LSE CSS Extended Interrupt Line. 01176 * @retval None 01177 */ 01178 #define __HAL_RCC_LSECSS_EXTI_DISABLE_IT() CLEAR_BIT(EXTI->IMR1, RCC_EXTI_LINE_LSECSS) 01179 01180 /** 01181 * @brief Enable the RCC LSE CSS Event Line. 01182 * @retval None. 01183 */ 01184 #define __HAL_RCC_LSECSS_EXTI_ENABLE_EVENT() SET_BIT(EXTI->EMR1, RCC_EXTI_LINE_LSECSS) 01185 01186 /** 01187 * @brief Disable the RCC LSE CSS Event Line. 01188 * @retval None. 01189 */ 01190 #define __HAL_RCC_LSECSS_EXTI_DISABLE_EVENT() CLEAR_BIT(EXTI->EMR1, RCC_EXTI_LINE_LSECSS) 01191 01192 01193 /** 01194 * @brief Enable the RCC LSE CSS Extended Interrupt Falling Trigger. 01195 * @retval None. 01196 */ 01197 #define __HAL_RCC_LSECSS_EXTI_ENABLE_FALLING_EDGE() SET_BIT(EXTI->FTSR1, RCC_EXTI_LINE_LSECSS) 01198 01199 01200 /** 01201 * @brief Disable the RCC LSE CSS Extended Interrupt Falling Trigger. 01202 * @retval None. 01203 */ 01204 #define __HAL_RCC_LSECSS_EXTI_DISABLE_FALLING_EDGE() CLEAR_BIT(EXTI->FTSR1, RCC_EXTI_LINE_LSECSS) 01205 01206 01207 /** 01208 * @brief Enable the RCC LSE CSS Extended Interrupt Rising Trigger. 01209 * @retval None. 01210 */ 01211 #define __HAL_RCC_LSECSS_EXTI_ENABLE_RISING_EDGE() SET_BIT(EXTI->RTSR1, RCC_EXTI_LINE_LSECSS) 01212 01213 /** 01214 * @brief Disable the RCC LSE CSS Extended Interrupt Rising Trigger. 01215 * @retval None. 01216 */ 01217 #define __HAL_RCC_LSECSS_EXTI_DISABLE_RISING_EDGE() CLEAR_BIT(EXTI->RTSR1, RCC_EXTI_LINE_LSECSS) 01218 01219 /** 01220 * @brief Enable the RCC LSE CSS Extended Interrupt Rising & Falling Trigger. 01221 * @retval None. 01222 */ 01223 #define __HAL_RCC_LSECSS_EXTI_ENABLE_RISING_FALLING_EDGE() \ 01224 do { \ 01225 __HAL_RCC_LSECSS_EXTI_ENABLE_RISING_EDGE(); \ 01226 __HAL_RCC_LSECSS_EXTI_ENABLE_FALLING_EDGE(); \ 01227 } while(0) 01228 01229 /** 01230 * @brief Disable the RCC LSE CSS Extended Interrupt Rising & Falling Trigger. 01231 * @retval None. 01232 */ 01233 #define __HAL_RCC_LSECSS_EXTI_DISABLE_RISING_FALLING_EDGE() \ 01234 do { \ 01235 __HAL_RCC_LSECSS_EXTI_DISABLE_RISING_EDGE(); \ 01236 __HAL_RCC_LSECSS_EXTI_DISABLE_FALLING_EDGE(); \ 01237 } while(0) 01238 01239 /** 01240 * @brief Check whether the specified RCC LSE CSS EXTI interrupt flag is set or not. 01241 * @retval EXTI RCC LSE CSS Line Status. 01242 */ 01243 #define __HAL_RCC_LSECSS_EXTI_GET_FLAG() (READ_BIT(EXTI->PR1, RCC_EXTI_LINE_LSECSS) == RCC_EXTI_LINE_LSECSS) 01244 01245 /** 01246 * @brief Clear the RCC LSE CSS EXTI flag. 01247 * @retval None. 01248 */ 01249 #define __HAL_RCC_LSECSS_EXTI_CLEAR_FLAG() WRITE_REG(EXTI->PR1, RCC_EXTI_LINE_LSECSS) 01250 01251 /** 01252 * @brief Generate a Software interrupt on the RCC LSE CSS EXTI line. 01253 * @retval None. 01254 */ 01255 #define __HAL_RCC_LSECSS_EXTI_GENERATE_SWIT() SET_BIT(EXTI->SWIER1, RCC_EXTI_LINE_LSECSS) 01256 01257 /** 01258 * @} 01259 */ 01260 01261 /** 01262 * @} 01263 */ 01264 01265 /* Exported functions --------------------------------------------------------*/ 01266 /** @addtogroup RCCEx_Exported_Functions 01267 * @{ 01268 */ 01269 01270 /** @addtogroup RCCEx_Exported_Functions_Group1 01271 * @{ 01272 */ 01273 01274 HAL_StatusTypeDef HAL_RCCEx_PeriphCLKConfig(RCC_PeriphCLKInitTypeDef *PeriphClkInit); 01275 void HAL_RCCEx_GetPeriphCLKConfig(RCC_PeriphCLKInitTypeDef *PeriphClkInit); 01276 uint32_t HAL_RCCEx_GetPeriphCLKFreq(uint32_t PeriphClk); 01277 01278 /** 01279 * @} 01280 */ 01281 01282 /** @addtogroup RCCEx_Exported_Functions_Group2 01283 * @{ 01284 */ 01285 01286 HAL_StatusTypeDef HAL_RCCEx_EnablePLLSAI1(RCC_PLLSAI1InitTypeDef *PLLSAI1Init); 01287 HAL_StatusTypeDef HAL_RCCEx_DisablePLLSAI1(void); 01288 01289 #if defined(STM32L471xx) || defined(STM32L475xx) || defined(STM32L476xx) || defined(STM32L485xx) || defined(STM32L486xx) 01290 01291 HAL_StatusTypeDef HAL_RCCEx_EnablePLLSAI2(RCC_PLLSAI2InitTypeDef *PLLSAI2Init); 01292 HAL_StatusTypeDef HAL_RCCEx_DisablePLLSAI2(void); 01293 01294 #endif /* STM32L471xx || STM32L475xx || STM32L476xx || STM32L485xx || STM32L486xx */ 01295 01296 void HAL_RCCEx_WakeUpStopCLKConfig(uint32_t WakeUpClk); 01297 void HAL_RCCEx_StandbyMSIRangeConfig(uint32_t MSIRange); 01298 void HAL_RCCEx_EnableLSECSS(void); 01299 void HAL_RCCEx_DisableLSECSS(void); 01300 void HAL_RCCEx_EnableLSECSS_IT(void); 01301 void HAL_RCCEx_LSECSS_IRQHandler(void); 01302 void HAL_RCCEx_LSECSS_Callback(void); 01303 void HAL_RCCEx_EnableLSCO(uint32_t LSCOSource); 01304 void HAL_RCCEx_DisableLSCO(void); 01305 void HAL_RCCEx_EnableMSIPLLMode(void); 01306 void HAL_RCCEx_DisableMSIPLLMode(void); 01307 01308 /** 01309 * @} 01310 */ 01311 01312 /** 01313 * @} 01314 */ 01315 01316 /* Private macros ------------------------------------------------------------*/ 01317 /** @addtogroup RCCEx_Private_Macros 01318 * @{ 01319 */ 01320 01321 #define IS_RCC_LSCOSOURCE(__SOURCE__) (((__SOURCE__) == RCC_LSCOSOURCE_LSI) || \ 01322 ((__SOURCE__) == RCC_LSCOSOURCE_LSE)) 01323 01324 #if defined(STM32L471xx) 01325 01326 #define IS_RCC_PERIPHCLOCK(__SELECTION__) \ 01327 ((((__SELECTION__) & RCC_PERIPHCLK_USART1) == RCC_PERIPHCLK_USART1) || \ 01328 (((__SELECTION__) & RCC_PERIPHCLK_USART2) == RCC_PERIPHCLK_USART2) || \ 01329 (((__SELECTION__) & RCC_PERIPHCLK_USART3) == RCC_PERIPHCLK_USART3) || \ 01330 (((__SELECTION__) & RCC_PERIPHCLK_UART4) == RCC_PERIPHCLK_UART4) || \ 01331 (((__SELECTION__) & RCC_PERIPHCLK_UART5) == RCC_PERIPHCLK_UART5) || \ 01332 (((__SELECTION__) & RCC_PERIPHCLK_LPUART1) == RCC_PERIPHCLK_LPUART1) || \ 01333 (((__SELECTION__) & RCC_PERIPHCLK_I2C1) == RCC_PERIPHCLK_I2C1) || \ 01334 (((__SELECTION__) & RCC_PERIPHCLK_I2C2) == RCC_PERIPHCLK_I2C2) || \ 01335 (((__SELECTION__) & RCC_PERIPHCLK_I2C3) == RCC_PERIPHCLK_I2C3) || \ 01336 (((__SELECTION__) & RCC_PERIPHCLK_LPTIM1) == RCC_PERIPHCLK_LPTIM1) || \ 01337 (((__SELECTION__) & RCC_PERIPHCLK_LPTIM2) == RCC_PERIPHCLK_LPTIM2) || \ 01338 (((__SELECTION__) & RCC_PERIPHCLK_SAI1) == RCC_PERIPHCLK_SAI1) || \ 01339 (((__SELECTION__) & RCC_PERIPHCLK_SAI2) == RCC_PERIPHCLK_SAI2) || \ 01340 (((__SELECTION__) & RCC_PERIPHCLK_ADC) == RCC_PERIPHCLK_ADC) || \ 01341 (((__SELECTION__) & RCC_PERIPHCLK_SWPMI1) == RCC_PERIPHCLK_SWPMI1) || \ 01342 (((__SELECTION__) & RCC_PERIPHCLK_DFSDM) == RCC_PERIPHCLK_DFSDM) || \ 01343 (((__SELECTION__) & RCC_PERIPHCLK_RTC) == RCC_PERIPHCLK_RTC) || \ 01344 (((__SELECTION__) & RCC_PERIPHCLK_RNG) == RCC_PERIPHCLK_RNG) || \ 01345 (((__SELECTION__) & RCC_PERIPHCLK_SDMMC1) == RCC_PERIPHCLK_SDMMC1)) 01346 01347 #else /* STM32L475xx || STM32L476xx || STM32L485xx || STM32L486xx */ 01348 01349 #define IS_RCC_PERIPHCLOCK(__SELECTION__) \ 01350 ((((__SELECTION__) & RCC_PERIPHCLK_USART1) == RCC_PERIPHCLK_USART1) || \ 01351 (((__SELECTION__) & RCC_PERIPHCLK_USART2) == RCC_PERIPHCLK_USART2) || \ 01352 (((__SELECTION__) & RCC_PERIPHCLK_USART3) == RCC_PERIPHCLK_USART3) || \ 01353 (((__SELECTION__) & RCC_PERIPHCLK_UART4) == RCC_PERIPHCLK_UART4) || \ 01354 (((__SELECTION__) & RCC_PERIPHCLK_UART5) == RCC_PERIPHCLK_UART5) || \ 01355 (((__SELECTION__) & RCC_PERIPHCLK_LPUART1) == RCC_PERIPHCLK_LPUART1) || \ 01356 (((__SELECTION__) & RCC_PERIPHCLK_I2C1) == RCC_PERIPHCLK_I2C1) || \ 01357 (((__SELECTION__) & RCC_PERIPHCLK_I2C2) == RCC_PERIPHCLK_I2C2) || \ 01358 (((__SELECTION__) & RCC_PERIPHCLK_I2C3) == RCC_PERIPHCLK_I2C3) || \ 01359 (((__SELECTION__) & RCC_PERIPHCLK_LPTIM1) == RCC_PERIPHCLK_LPTIM1) || \ 01360 (((__SELECTION__) & RCC_PERIPHCLK_LPTIM2) == RCC_PERIPHCLK_LPTIM2) || \ 01361 (((__SELECTION__) & RCC_PERIPHCLK_SAI1) == RCC_PERIPHCLK_SAI1) || \ 01362 (((__SELECTION__) & RCC_PERIPHCLK_SAI2) == RCC_PERIPHCLK_SAI2) || \ 01363 (((__SELECTION__) & RCC_PERIPHCLK_USB) == RCC_PERIPHCLK_USB) || \ 01364 (((__SELECTION__) & RCC_PERIPHCLK_ADC) == RCC_PERIPHCLK_ADC) || \ 01365 (((__SELECTION__) & RCC_PERIPHCLK_SWPMI1) == RCC_PERIPHCLK_SWPMI1) || \ 01366 (((__SELECTION__) & RCC_PERIPHCLK_DFSDM) == RCC_PERIPHCLK_DFSDM) || \ 01367 (((__SELECTION__) & RCC_PERIPHCLK_RTC) == RCC_PERIPHCLK_RTC) || \ 01368 (((__SELECTION__) & RCC_PERIPHCLK_RNG) == RCC_PERIPHCLK_RNG) || \ 01369 (((__SELECTION__) & RCC_PERIPHCLK_SDMMC1) == RCC_PERIPHCLK_SDMMC1)) 01370 01371 #endif /* STM32L471xx */ 01372 01373 #define IS_RCC_USART1CLKSOURCE(__SOURCE__) \ 01374 (((__SOURCE__) == RCC_USART1CLKSOURCE_PCLK2) || \ 01375 ((__SOURCE__) == RCC_USART1CLKSOURCE_SYSCLK) || \ 01376 ((__SOURCE__) == RCC_USART1CLKSOURCE_LSE) || \ 01377 ((__SOURCE__) == RCC_USART1CLKSOURCE_HSI)) 01378 01379 #define IS_RCC_USART2CLKSOURCE(__SOURCE__) \ 01380 (((__SOURCE__) == RCC_USART2CLKSOURCE_PCLK1) || \ 01381 ((__SOURCE__) == RCC_USART2CLKSOURCE_SYSCLK) || \ 01382 ((__SOURCE__) == RCC_USART2CLKSOURCE_LSE) || \ 01383 ((__SOURCE__) == RCC_USART2CLKSOURCE_HSI)) 01384 01385 #define IS_RCC_USART3CLKSOURCE(__SOURCE__) \ 01386 (((__SOURCE__) == RCC_USART3CLKSOURCE_PCLK1) || \ 01387 ((__SOURCE__) == RCC_USART3CLKSOURCE_SYSCLK) || \ 01388 ((__SOURCE__) == RCC_USART3CLKSOURCE_LSE) || \ 01389 ((__SOURCE__) == RCC_USART3CLKSOURCE_HSI)) 01390 01391 #define IS_RCC_UART4CLKSOURCE(__SOURCE__) \ 01392 (((__SOURCE__) == RCC_UART4CLKSOURCE_PCLK1) || \ 01393 ((__SOURCE__) == RCC_UART4CLKSOURCE_SYSCLK) || \ 01394 ((__SOURCE__) == RCC_UART4CLKSOURCE_LSE) || \ 01395 ((__SOURCE__) == RCC_UART4CLKSOURCE_HSI)) 01396 01397 #define IS_RCC_UART5CLKSOURCE(__SOURCE__) \ 01398 (((__SOURCE__) == RCC_UART5CLKSOURCE_PCLK1) || \ 01399 ((__SOURCE__) == RCC_UART5CLKSOURCE_SYSCLK) || \ 01400 ((__SOURCE__) == RCC_UART5CLKSOURCE_LSE) || \ 01401 ((__SOURCE__) == RCC_UART5CLKSOURCE_HSI)) 01402 01403 #define IS_RCC_LPUART1CLKSOURCE(__SOURCE__) \ 01404 (((__SOURCE__) == RCC_LPUART1CLKSOURCE_PCLK1) || \ 01405 ((__SOURCE__) == RCC_LPUART1CLKSOURCE_SYSCLK) || \ 01406 ((__SOURCE__) == RCC_LPUART1CLKSOURCE_LSE) || \ 01407 ((__SOURCE__) == RCC_LPUART1CLKSOURCE_HSI)) 01408 01409 #define IS_RCC_I2C1CLKSOURCE(__SOURCE__) \ 01410 (((__SOURCE__) == RCC_I2C1CLKSOURCE_PCLK1) || \ 01411 ((__SOURCE__) == RCC_I2C1CLKSOURCE_SYSCLK)|| \ 01412 ((__SOURCE__) == RCC_I2C1CLKSOURCE_HSI)) 01413 01414 #define IS_RCC_I2C2CLKSOURCE(__SOURCE__) \ 01415 (((__SOURCE__) == RCC_I2C2CLKSOURCE_PCLK1) || \ 01416 ((__SOURCE__) == RCC_I2C2CLKSOURCE_SYSCLK)|| \ 01417 ((__SOURCE__) == RCC_I2C2CLKSOURCE_HSI)) 01418 01419 #define IS_RCC_I2C3CLKSOURCE(__SOURCE__) \ 01420 (((__SOURCE__) == RCC_I2C3CLKSOURCE_PCLK1) || \ 01421 ((__SOURCE__) == RCC_I2C3CLKSOURCE_SYSCLK)|| \ 01422 ((__SOURCE__) == RCC_I2C3CLKSOURCE_HSI)) 01423 01424 #define IS_RCC_SAI1CLK(__SOURCE__) \ 01425 (((__SOURCE__) == RCC_SAI1CLKSOURCE_PLLSAI1) || \ 01426 ((__SOURCE__) == RCC_SAI1CLKSOURCE_PLLSAI2) || \ 01427 ((__SOURCE__) == RCC_SAI1CLKSOURCE_PLL) || \ 01428 ((__SOURCE__) == RCC_SAI1CLKSOURCE_PIN)) 01429 01430 #define IS_RCC_SAI2CLK(__SOURCE__) \ 01431 (((__SOURCE__) == RCC_SAI2CLKSOURCE_PLLSAI1) || \ 01432 ((__SOURCE__) == RCC_SAI2CLKSOURCE_PLLSAI2) || \ 01433 ((__SOURCE__) == RCC_SAI2CLKSOURCE_PLL) || \ 01434 ((__SOURCE__) == RCC_SAI2CLKSOURCE_PIN)) 01435 01436 #define IS_RCC_LPTIM1CLK(__SOURCE__) \ 01437 (((__SOURCE__) == RCC_LPTIM1CLKSOURCE_PCLK) || \ 01438 ((__SOURCE__) == RCC_LPTIM1CLKSOURCE_LSI) || \ 01439 ((__SOURCE__) == RCC_LPTIM1CLKSOURCE_HSI) || \ 01440 ((__SOURCE__) == RCC_LPTIM1CLKSOURCE_LSE)) 01441 01442 #define IS_RCC_LPTIM2CLK(__SOURCE__) \ 01443 (((__SOURCE__) == RCC_LPTIM2CLKSOURCE_PCLK) || \ 01444 ((__SOURCE__) == RCC_LPTIM2CLKSOURCE_LSI) || \ 01445 ((__SOURCE__) == RCC_LPTIM2CLKSOURCE_HSI) || \ 01446 ((__SOURCE__) == RCC_LPTIM2CLKSOURCE_LSE)) 01447 01448 #define IS_RCC_SDMMC1CLKSOURCE(__SOURCE__) \ 01449 (((__SOURCE__) == RCC_SDMMC1CLKSOURCE_NONE) || \ 01450 ((__SOURCE__) == RCC_SDMMC1CLKSOURCE_PLLSAI1) || \ 01451 ((__SOURCE__) == RCC_SDMMC1CLKSOURCE_PLL) || \ 01452 ((__SOURCE__) == RCC_SDMMC1CLKSOURCE_MSI)) 01453 01454 #define IS_RCC_RNGCLKSOURCE(__SOURCE__) \ 01455 (((__SOURCE__) == RCC_RNGCLKSOURCE_NONE) || \ 01456 ((__SOURCE__) == RCC_RNGCLKSOURCE_PLLSAI1) || \ 01457 ((__SOURCE__) == RCC_RNGCLKSOURCE_PLL) || \ 01458 ((__SOURCE__) == RCC_RNGCLKSOURCE_MSI)) 01459 01460 #if defined(STM32L475xx) || defined(STM32L476xx) || defined(STM32L485xx) || defined(STM32L486xx) 01461 01462 #define IS_RCC_USBCLKSOURCE(__SOURCE__) \ 01463 (((__SOURCE__) == RCC_USBCLKSOURCE_NONE) || \ 01464 ((__SOURCE__) == RCC_USBCLKSOURCE_PLLSAI1) || \ 01465 ((__SOURCE__) == RCC_USBCLKSOURCE_PLL) || \ 01466 ((__SOURCE__) == RCC_USBCLKSOURCE_MSI)) 01467 01468 #endif /* STM32L475xx || STM32L476xx || STM32L485xx || STM32L486xx */ 01469 01470 #define IS_RCC_ADCCLKSOURCE(__SOURCE__) \ 01471 (((__SOURCE__) == RCC_ADCCLKSOURCE_NONE) || \ 01472 ((__SOURCE__) == RCC_ADCCLKSOURCE_PLLSAI1) || \ 01473 ((__SOURCE__) == RCC_ADCCLKSOURCE_PLLSAI2) || \ 01474 ((__SOURCE__) == RCC_ADCCLKSOURCE_SYSCLK)) 01475 01476 #define IS_RCC_SWPMI1CLKSOURCE(__SOURCE__) \ 01477 (((__SOURCE__) == RCC_SWPMI1CLKSOURCE_PCLK) || \ 01478 ((__SOURCE__) == RCC_SWPMI1CLKSOURCE_HSI)) 01479 01480 #define IS_RCC_DFSDMCLKSOURCE(__SOURCE__) \ 01481 (((__SOURCE__) == RCC_DFSDMCLKSOURCE_PCLK) || \ 01482 ((__SOURCE__) == RCC_DFSDMCLKSOURCE_SYSCLK)) 01483 01484 #define IS_RCC_PLLSAI1N_VALUE(__VALUE__) ((8 <= (__VALUE__)) && ((__VALUE__) <= 86)) 01485 01486 #define IS_RCC_PLLSAI1P_VALUE(__VALUE__) (((__VALUE__) == 7) || ((__VALUE__) == 17)) 01487 01488 #define IS_RCC_PLLSAI1Q_VALUE(__VALUE__) (((__VALUE__) == 2 ) || ((__VALUE__) == 4) || \ 01489 ((__VALUE__) == 6) || ((__VALUE__) == 8)) 01490 01491 #define IS_RCC_PLLSAI1R_VALUE(__VALUE__) (((__VALUE__) == 2 ) || ((__VALUE__) == 4) || \ 01492 ((__VALUE__) == 6) || ((__VALUE__) == 8)) 01493 01494 #define IS_RCC_PLLSAI2N_VALUE(__VALUE__) ((8 <= (__VALUE__)) && ((__VALUE__) <= 86)) 01495 01496 #define IS_RCC_PLLSAI2P_VALUE(__VALUE__) (((__VALUE__) == 7) || ((__VALUE__) == 17)) 01497 01498 #define IS_RCC_PLLSAI2R_VALUE(__VALUE__) (((__VALUE__) == 2 ) || ((__VALUE__) == 4) || \ 01499 ((__VALUE__) == 6) || ((__VALUE__) == 8)) 01500 01501 01502 /** 01503 * @} 01504 */ 01505 01506 /** 01507 * @} 01508 */ 01509 01510 /** 01511 * @} 01512 */ 01513 01514 #ifdef __cplusplus 01515 } 01516 #endif 01517 01518 #endif /* __STM32L4xx_HAL_RCC_EX_H */ 01519 01520 /************************ (C) COPYRIGHT STMicroelectronics *****END OF FILE****/ 01521
Generated on Tue Jul 12 2022 11:35:15 by
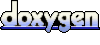