Hal Drivers for L4
Dependents: BSP OneHopeOnePrayer FINAL_AUDIO_RECORD AudioDemo
Fork of STM32L4xx_HAL_Driver by
stm32l4xx_hal_rcc_ex.c
00001 /** 00002 ****************************************************************************** 00003 * @file stm32l4xx_hal_rcc_ex.c 00004 * @author MCD Application Team 00005 * @version V1.1.0 00006 * @date 16-September-2015 00007 * @brief Extended RCC HAL module driver. 00008 * This file provides firmware functions to manage the following 00009 * functionalities RCC extended peripheral: 00010 * + Extended Peripheral Control functions 00011 * 00012 ****************************************************************************** 00013 * @attention 00014 * 00015 * <h2><center>© COPYRIGHT(c) 2015 STMicroelectronics</center></h2> 00016 * 00017 * Redistribution and use in source and binary forms, with or without modification, 00018 * are permitted provided that the following conditions are met: 00019 * 1. Redistributions of source code must retain the above copyright notice, 00020 * this list of conditions and the following disclaimer. 00021 * 2. Redistributions in binary form must reproduce the above copyright notice, 00022 * this list of conditions and the following disclaimer in the documentation 00023 * and/or other materials provided with the distribution. 00024 * 3. Neither the name of STMicroelectronics nor the names of its contributors 00025 * may be used to endorse or promote products derived from this software 00026 * without specific prior written permission. 00027 * 00028 * THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" 00029 * AND ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE 00030 * IMPLIED WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE 00031 * DISCLAIMED. IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE 00032 * FOR ANY DIRECT, INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL 00033 * DAMAGES (INCLUDING, BUT NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR 00034 * SERVICES; LOSS OF USE, DATA, OR PROFITS; OR BUSINESS INTERRUPTION) HOWEVER 00035 * CAUSED AND ON ANY THEORY OF LIABILITY, WHETHER IN CONTRACT, STRICT LIABILITY, 00036 * OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) ARISING IN ANY WAY OUT OF THE USE 00037 * OF THIS SOFTWARE, EVEN IF ADVISED OF THE POSSIBILITY OF SUCH DAMAGE. 00038 * 00039 ****************************************************************************** 00040 */ 00041 00042 /* Includes ------------------------------------------------------------------*/ 00043 #include "stm32l4xx_hal.h" 00044 00045 /** @addtogroup STM32L4xx_HAL_Driver 00046 * @{ 00047 */ 00048 00049 /** @defgroup RCCEx RCCEx 00050 * @brief RCC Extended HAL module driver 00051 * @{ 00052 */ 00053 00054 #ifdef HAL_RCC_MODULE_ENABLED 00055 00056 /* Private typedef -----------------------------------------------------------*/ 00057 /* Private defines -----------------------------------------------------------*/ 00058 /** @defgroup RCCEx_Private_Constants RCCEx Private Constants 00059 * @{ 00060 */ 00061 #define PLLSAI1_TIMEOUT_VALUE 1000U /* Timeout value fixed to 100 ms */ 00062 #define PLLSAI2_TIMEOUT_VALUE 1000U /* Timeout value fixed to 100 ms */ 00063 #define PLL_TIMEOUT_VALUE 100U /* Timeout value fixed to 100 ms */ 00064 00065 #define __LSCO_CLK_ENABLE() __HAL_RCC_GPIOA_CLK_ENABLE() 00066 #define LSCO_GPIO_PORT GPIOA 00067 #define LSCO_PIN GPIO_PIN_2 00068 /** 00069 * @} 00070 */ 00071 00072 /* Private macros ------------------------------------------------------------*/ 00073 /* Private variables ---------------------------------------------------------*/ 00074 /* Private function prototypes -----------------------------------------------*/ 00075 /** @defgroup RCCEx_Private_Functions RCCEx Private Functions 00076 * @{ 00077 */ 00078 static HAL_StatusTypeDef RCCEx_PLLSAI1_ConfigNP(RCC_PLLSAI1InitTypeDef *PllSai1); 00079 static HAL_StatusTypeDef RCCEx_PLLSAI1_ConfigNQ(RCC_PLLSAI1InitTypeDef *PllSai1); 00080 static HAL_StatusTypeDef RCCEx_PLLSAI1_ConfigNR(RCC_PLLSAI1InitTypeDef *PllSai1); 00081 static HAL_StatusTypeDef RCCEx_PLLSAI2_ConfigNP(RCC_PLLSAI2InitTypeDef *PllSai2); 00082 static HAL_StatusTypeDef RCCEx_PLLSAI2_ConfigNR(RCC_PLLSAI2InitTypeDef *PllSai2); 00083 /** 00084 * @} 00085 */ 00086 00087 /* Exported functions --------------------------------------------------------*/ 00088 00089 /** @defgroup RCCEx_Exported_Functions RCCEx Exported Functions 00090 * @{ 00091 */ 00092 00093 /** @defgroup RCCEx_Exported_Functions_Group1 Extended Peripheral Control functions 00094 * @brief Extended Peripheral Control functions 00095 * 00096 @verbatim 00097 =============================================================================== 00098 ##### Extended Peripheral Control functions ##### 00099 =============================================================================== 00100 [..] 00101 This subsection provides a set of functions allowing to control the RCC Clocks 00102 frequencies. 00103 [..] 00104 (@) Important note: Care must be taken when HAL_RCCEx_PeriphCLKConfig() is used to 00105 select the RTC clock source; in this case the Backup domain will be reset in 00106 order to modify the RTC Clock source, as consequence RTC registers (including 00107 the backup registers) and RCC_BDCR register are set to their reset values. 00108 00109 @endverbatim 00110 * @{ 00111 */ 00112 /** 00113 * @brief Initialize the RCC extended peripherals clocks according to the specified 00114 * parameters in the RCC_PeriphCLKInitTypeDef. 00115 * @param PeriphClkInit pointer to an RCC_PeriphCLKInitTypeDef structure that 00116 * contains a field PeriphClockSelection which can be a combination of the following values: 00117 * @arg @ref RCC_PERIPHCLK_RTC RTC peripheral clock 00118 * @arg @ref RCC_PERIPHCLK_ADC ADC peripheral clock 00119 * @arg @ref RCC_PERIPHCLK_DFSDM DFSDM peripheral clock 00120 * @arg @ref RCC_PERIPHCLK_I2C1 I2C1 peripheral clock 00121 * @arg @ref RCC_PERIPHCLK_I2C2 I2C2 peripheral clock 00122 * @arg @ref RCC_PERIPHCLK_I2C3 I2C3 peripheral clock 00123 * @arg @ref RCC_PERIPHCLK_LPTIM1 LPTIM1 peripheral clock 00124 * @arg @ref RCC_PERIPHCLK_LPTIM2 LPTIM2 peripheral clock 00125 * @arg @ref RCC_PERIPHCLK_LPUART1 LPUART1 peripheral clock 00126 * @arg @ref RCC_PERIPHCLK_RNG RNG peripheral clock 00127 * @arg @ref RCC_PERIPHCLK_SAI1 SAI1 peripheral clock 00128 * @arg @ref RCC_PERIPHCLK_SAI2 SAI2 peripheral clock 00129 * @arg @ref RCC_PERIPHCLK_SDMMC1 SDMMC1 peripheral clock 00130 * @arg @ref RCC_PERIPHCLK_SWPMI1 SWPMI1 peripheral clock 00131 * @arg @ref RCC_PERIPHCLK_USART1 USART1 peripheral clock 00132 * @arg @ref RCC_PERIPHCLK_USART2 USART1 peripheral clock 00133 * @arg @ref RCC_PERIPHCLK_USART3 USART1 peripheral clock 00134 * @arg @ref RCC_PERIPHCLK_UART4 USART1 peripheral clock 00135 * @arg @ref RCC_PERIPHCLK_UART5 USART1 peripheral clock 00136 * @arg @ref RCC_PERIPHCLK_USB USB peripheral clock (only for devices with USB) 00137 * 00138 * @note Care must be taken when HAL_RCCEx_PeriphCLKConfig() is used to select 00139 * the RTC clock source: in this case the access to Backup domain is enabled. 00140 * 00141 * @retval HAL status 00142 */ 00143 HAL_StatusTypeDef HAL_RCCEx_PeriphCLKConfig(RCC_PeriphCLKInitTypeDef *PeriphClkInit) 00144 { 00145 uint32_t tmpregister = 0; 00146 uint32_t tickstart = 0; 00147 HAL_StatusTypeDef ret = HAL_OK; /* Intermediate status */ 00148 HAL_StatusTypeDef status = HAL_OK; /* Final status */ 00149 00150 /* Check the parameters */ 00151 assert_param(IS_RCC_PERIPHCLOCK(PeriphClkInit->PeriphClockSelection)); 00152 00153 /*-------------------------- SAI1 clock source configuration ---------------------*/ 00154 if((((PeriphClkInit->PeriphClockSelection) & RCC_PERIPHCLK_SAI1) == RCC_PERIPHCLK_SAI1)) 00155 { 00156 /* Check the parameters */ 00157 assert_param(IS_RCC_SAI1CLK(PeriphClkInit->Sai1ClockSelection)); 00158 00159 switch(PeriphClkInit->Sai1ClockSelection) 00160 { 00161 case RCC_SAI1CLKSOURCE_PLL: /* PLL is used as clock source for SAI1*/ 00162 /* Enable SAI Clock output generated form System PLL . */ 00163 __HAL_RCC_PLLCLKOUT_ENABLE(RCC_PLL_SAI3CLK); 00164 /* SAI1 clock source config set later after clock selection check */ 00165 break; 00166 00167 case RCC_SAI1CLKSOURCE_PLLSAI1: /* PLLSAI1 is used as clock source for SAI1*/ 00168 /* PLLSAI1 parameters N & P configuration and clock output (PLLSAI1ClockOut) */ 00169 ret = RCCEx_PLLSAI1_ConfigNP(&(PeriphClkInit->PLLSAI1)); 00170 /* SAI1 clock source config set later after clock selection check */ 00171 break; 00172 00173 case RCC_SAI1CLKSOURCE_PLLSAI2: /* PLLSAI2 is used as clock source for SAI1*/ 00174 /* PLLSAI2 parameters N & P configuration and clock output (PLLSAI2ClockOut) */ 00175 ret = RCCEx_PLLSAI2_ConfigNP(&(PeriphClkInit->PLLSAI2)); 00176 /* SAI1 clock source config set later after clock selection check */ 00177 break; 00178 00179 case RCC_SAI1CLKSOURCE_PIN: /* External clock is used as source of SAI1 clock*/ 00180 /* SAI1 clock source config set later after clock selection check */ 00181 break; 00182 00183 default: 00184 ret = HAL_ERROR; 00185 break; 00186 } 00187 00188 if(ret == HAL_OK) 00189 { 00190 /* Set the source of SAI1 clock*/ 00191 __HAL_RCC_SAI1_CONFIG(PeriphClkInit->Sai1ClockSelection); 00192 } 00193 else 00194 { 00195 /* set overall return value */ 00196 status = ret; 00197 } 00198 } 00199 00200 /*-------------------------- SAI2 clock source configuration ---------------------*/ 00201 if((((PeriphClkInit->PeriphClockSelection) & RCC_PERIPHCLK_SAI2) == RCC_PERIPHCLK_SAI2)) 00202 { 00203 /* Check the parameters */ 00204 assert_param(IS_RCC_SAI2CLK(PeriphClkInit->Sai2ClockSelection)); 00205 00206 switch(PeriphClkInit->Sai2ClockSelection) 00207 { 00208 case RCC_SAI2CLKSOURCE_PLL: /* PLL is used as clock source for SAI2*/ 00209 /* Enable SAI Clock output generated form System PLL . */ 00210 __HAL_RCC_PLLCLKOUT_ENABLE(RCC_PLL_SAI3CLK); 00211 /* SAI2 clock source config set later after clock selection check */ 00212 break; 00213 00214 case RCC_SAI2CLKSOURCE_PLLSAI1: /* PLLSAI1 is used as clock source for SAI2*/ 00215 /* PLLSAI1 parameters N & P configuration and clock output (PLLSAI1ClockOut) */ 00216 ret = RCCEx_PLLSAI1_ConfigNP(&(PeriphClkInit->PLLSAI1)); 00217 /* SAI2 clock source config set later after clock selection check */ 00218 break; 00219 00220 case RCC_SAI2CLKSOURCE_PLLSAI2: /* PLLSAI2 is used as clock source for SAI2*/ 00221 /* PLLSAI2 parameters N & P configuration and clock output (PLLSAI2ClockOut) */ 00222 ret = RCCEx_PLLSAI2_ConfigNP(&(PeriphClkInit->PLLSAI2)); 00223 /* SAI2 clock source config set later after clock selection check */ 00224 break; 00225 00226 case RCC_SAI2CLKSOURCE_PIN: /* External clock is used as source of SAI2 clock*/ 00227 /* SAI2 clock source config set later after clock selection check */ 00228 break; 00229 00230 default: 00231 ret = HAL_ERROR; 00232 break; 00233 } 00234 00235 if(ret == HAL_OK) 00236 { 00237 /* Set the source of SAI2 clock*/ 00238 __HAL_RCC_SAI2_CONFIG(PeriphClkInit->Sai2ClockSelection); 00239 } 00240 else 00241 { 00242 /* set overall return value */ 00243 status = ret; 00244 } 00245 } 00246 00247 /*-------------------------- RTC clock source configuration ----------------------*/ 00248 if((PeriphClkInit->PeriphClockSelection & RCC_PERIPHCLK_RTC) == RCC_PERIPHCLK_RTC) 00249 { 00250 FlagStatus pwrclkchanged = RESET; 00251 00252 /* Check for RTC Parameters used to output RTCCLK */ 00253 assert_param(IS_RCC_RTCCLKSOURCE(PeriphClkInit->RTCClockSelection)); 00254 00255 /* Enable Power Clock */ 00256 if(__HAL_RCC_PWR_IS_CLK_DISABLED()) 00257 { 00258 __HAL_RCC_PWR_CLK_ENABLE(); 00259 pwrclkchanged = SET; 00260 } 00261 00262 /* Enable write access to Backup domain */ 00263 SET_BIT(PWR->CR1, PWR_CR1_DBP); 00264 00265 /* Wait for Backup domain Write protection disable */ 00266 tickstart = HAL_GetTick(); 00267 00268 while((PWR->CR1 & PWR_CR1_DBP) == RESET) 00269 { 00270 if((HAL_GetTick() - tickstart) > RCC_DBP_TIMEOUT_VALUE) 00271 { 00272 ret = HAL_TIMEOUT; 00273 break; 00274 } 00275 } 00276 00277 if(ret == HAL_OK) 00278 { 00279 /* Reset the Backup domain only if the RTC Clock source selection is modified */ 00280 if(READ_BIT(RCC->BDCR, RCC_BDCR_RTCSEL) != PeriphClkInit->RTCClockSelection) 00281 { 00282 /* Store the content of BDCR register before the reset of Backup Domain */ 00283 tmpregister = READ_BIT(RCC->BDCR, ~(RCC_BDCR_RTCSEL)); 00284 /* RTC Clock selection can be changed only if the Backup Domain is reset */ 00285 __HAL_RCC_BACKUPRESET_FORCE(); 00286 __HAL_RCC_BACKUPRESET_RELEASE(); 00287 /* Restore the Content of BDCR register */ 00288 RCC->BDCR = tmpregister; 00289 } 00290 00291 /* Wait for LSE reactivation if LSE was enable prior to Backup Domain reset */ 00292 if (HAL_IS_BIT_SET(tmpregister, RCC_BDCR_LSERDY)) 00293 { 00294 /* Get Start Tick*/ 00295 tickstart = HAL_GetTick(); 00296 00297 /* Wait till LSE is ready */ 00298 while(__HAL_RCC_GET_FLAG(RCC_FLAG_LSERDY) == RESET) 00299 { 00300 if((HAL_GetTick() - tickstart) > RCC_LSE_TIMEOUT_VALUE) 00301 { 00302 ret = HAL_TIMEOUT; 00303 break; 00304 } 00305 } 00306 } 00307 00308 if(ret == HAL_OK) 00309 { 00310 /* Apply new RTC clock source selection */ 00311 __HAL_RCC_RTC_CONFIG(PeriphClkInit->RTCClockSelection); 00312 } 00313 else 00314 { 00315 /* set overall return value */ 00316 status = ret; 00317 } 00318 } 00319 else 00320 { 00321 /* set overall return value */ 00322 status = ret; 00323 } 00324 00325 /* Restore clock configuration if changed */ 00326 if(pwrclkchanged == SET) 00327 { 00328 __HAL_RCC_PWR_CLK_DISABLE(); 00329 } 00330 } 00331 00332 /*-------------------------- USART1 clock source configuration -------------------*/ 00333 if(((PeriphClkInit->PeriphClockSelection) & RCC_PERIPHCLK_USART1) == RCC_PERIPHCLK_USART1) 00334 { 00335 /* Check the parameters */ 00336 assert_param(IS_RCC_USART1CLKSOURCE(PeriphClkInit->Usart1ClockSelection)); 00337 00338 /* Configure the USART1 clock source */ 00339 __HAL_RCC_USART1_CONFIG(PeriphClkInit->Usart1ClockSelection); 00340 } 00341 00342 /*-------------------------- USART2 clock source configuration -------------------*/ 00343 if(((PeriphClkInit->PeriphClockSelection) & RCC_PERIPHCLK_USART2) == RCC_PERIPHCLK_USART2) 00344 { 00345 /* Check the parameters */ 00346 assert_param(IS_RCC_USART2CLKSOURCE(PeriphClkInit->Usart2ClockSelection)); 00347 00348 /* Configure the USART2 clock source */ 00349 __HAL_RCC_USART2_CONFIG(PeriphClkInit->Usart2ClockSelection); 00350 } 00351 00352 /*-------------------------- USART3 clock source configuration -------------------*/ 00353 if(((PeriphClkInit->PeriphClockSelection) & RCC_PERIPHCLK_USART3) == RCC_PERIPHCLK_USART3) 00354 { 00355 /* Check the parameters */ 00356 assert_param(IS_RCC_USART3CLKSOURCE(PeriphClkInit->Usart3ClockSelection)); 00357 00358 /* Configure the USART3 clock source */ 00359 __HAL_RCC_USART3_CONFIG(PeriphClkInit->Usart3ClockSelection); 00360 } 00361 00362 /*-------------------------- UART4 clock source configuration --------------------*/ 00363 if(((PeriphClkInit->PeriphClockSelection) & RCC_PERIPHCLK_UART4) == RCC_PERIPHCLK_UART4) 00364 { 00365 /* Check the parameters */ 00366 assert_param(IS_RCC_UART4CLKSOURCE(PeriphClkInit->Uart4ClockSelection)); 00367 00368 /* Configure the UART4 clock source */ 00369 __HAL_RCC_UART4_CONFIG(PeriphClkInit->Uart4ClockSelection); 00370 } 00371 00372 /*-------------------------- UART5 clock source configuration --------------------*/ 00373 if(((PeriphClkInit->PeriphClockSelection) & RCC_PERIPHCLK_UART5) == RCC_PERIPHCLK_UART5) 00374 { 00375 /* Check the parameters */ 00376 assert_param(IS_RCC_UART5CLKSOURCE(PeriphClkInit->Uart5ClockSelection)); 00377 00378 /* Configure the UART5 clock source */ 00379 __HAL_RCC_UART5_CONFIG(PeriphClkInit->Uart5ClockSelection); 00380 } 00381 00382 /*-------------------------- LPUART1 clock source configuration ------------------*/ 00383 if(((PeriphClkInit->PeriphClockSelection) & RCC_PERIPHCLK_LPUART1) == RCC_PERIPHCLK_LPUART1) 00384 { 00385 /* Check the parameters */ 00386 assert_param(IS_RCC_LPUART1CLKSOURCE(PeriphClkInit->Lpuart1ClockSelection)); 00387 00388 /* Configure the LPUAR1 clock source */ 00389 __HAL_RCC_LPUART1_CONFIG(PeriphClkInit->Lpuart1ClockSelection); 00390 } 00391 00392 /*-------------------------- LPTIM1 clock source configuration -------------------*/ 00393 if(((PeriphClkInit->PeriphClockSelection) & RCC_PERIPHCLK_LPTIM1) == (RCC_PERIPHCLK_LPTIM1)) 00394 { 00395 assert_param(IS_RCC_LPTIM1CLK(PeriphClkInit->Lptim1ClockSelection)); 00396 __HAL_RCC_LPTIM1_CONFIG(PeriphClkInit->Lptim1ClockSelection); 00397 } 00398 00399 /*-------------------------- LPTIM2 clock source configuration -------------------*/ 00400 if(((PeriphClkInit->PeriphClockSelection) & RCC_PERIPHCLK_LPTIM2) == (RCC_PERIPHCLK_LPTIM2)) 00401 { 00402 assert_param(IS_RCC_LPTIM2CLK(PeriphClkInit->Lptim2ClockSelection)); 00403 __HAL_RCC_LPTIM2_CONFIG(PeriphClkInit->Lptim2ClockSelection); 00404 } 00405 00406 /*-------------------------- I2C1 clock source configuration ---------------------*/ 00407 if(((PeriphClkInit->PeriphClockSelection) & RCC_PERIPHCLK_I2C1) == RCC_PERIPHCLK_I2C1) 00408 { 00409 /* Check the parameters */ 00410 assert_param(IS_RCC_I2C1CLKSOURCE(PeriphClkInit->I2c1ClockSelection)); 00411 00412 /* Configure the I2C1 clock source */ 00413 __HAL_RCC_I2C1_CONFIG(PeriphClkInit->I2c1ClockSelection); 00414 } 00415 00416 /*-------------------------- I2C2 clock source configuration ---------------------*/ 00417 if(((PeriphClkInit->PeriphClockSelection) & RCC_PERIPHCLK_I2C2) == RCC_PERIPHCLK_I2C2) 00418 { 00419 /* Check the parameters */ 00420 assert_param(IS_RCC_I2C2CLKSOURCE(PeriphClkInit->I2c2ClockSelection)); 00421 00422 /* Configure the I2C2 clock source */ 00423 __HAL_RCC_I2C2_CONFIG(PeriphClkInit->I2c2ClockSelection); 00424 } 00425 00426 /*-------------------------- I2C3 clock source configuration ---------------------*/ 00427 if(((PeriphClkInit->PeriphClockSelection) & RCC_PERIPHCLK_I2C3) == RCC_PERIPHCLK_I2C3) 00428 { 00429 /* Check the parameters */ 00430 assert_param(IS_RCC_I2C3CLKSOURCE(PeriphClkInit->I2c3ClockSelection)); 00431 00432 /* Configure the I2C3 clock source */ 00433 __HAL_RCC_I2C3_CONFIG(PeriphClkInit->I2c3ClockSelection); 00434 } 00435 00436 #if defined(STM32L475xx) || defined(STM32L476xx) || defined(STM32L485xx) || defined(STM32L486xx) 00437 /*-------------------------- USB clock source configuration ----------------------*/ 00438 if(((PeriphClkInit->PeriphClockSelection) & RCC_PERIPHCLK_USB) == (RCC_PERIPHCLK_USB)) 00439 { 00440 assert_param(IS_RCC_USBCLKSOURCE(PeriphClkInit->UsbClockSelection)); 00441 __HAL_RCC_USB_CONFIG(PeriphClkInit->UsbClockSelection); 00442 00443 if(PeriphClkInit->UsbClockSelection == RCC_USBCLKSOURCE_PLL) 00444 { 00445 /* Enable PLL48M1CLK output */ 00446 __HAL_RCC_PLLCLKOUT_ENABLE(RCC_PLL_48M1CLK); 00447 } 00448 else if(PeriphClkInit->UsbClockSelection == RCC_USBCLKSOURCE_PLLSAI1) 00449 { 00450 /* PLLSAI1 parameters N & Q configuration and clock output (PLLSAI1ClockOut) */ 00451 ret = RCCEx_PLLSAI1_ConfigNQ(&(PeriphClkInit->PLLSAI1)); 00452 00453 if(ret != HAL_OK) 00454 { 00455 /* set overall return value */ 00456 status = ret; 00457 } 00458 } 00459 } 00460 #endif /* STM32L475xx || STM32L476xx || STM32L485xx || STM32L486xx */ 00461 00462 /*-------------------------- SDMMC1 clock source configuration -------------------*/ 00463 if(((PeriphClkInit->PeriphClockSelection) & RCC_PERIPHCLK_SDMMC1) == (RCC_PERIPHCLK_SDMMC1)) 00464 { 00465 assert_param(IS_RCC_SDMMC1CLKSOURCE(PeriphClkInit->Sdmmc1ClockSelection)); 00466 __HAL_RCC_SDMMC1_CONFIG(PeriphClkInit->Sdmmc1ClockSelection); 00467 00468 if(PeriphClkInit->Sdmmc1ClockSelection == RCC_SDMMC1CLKSOURCE_PLL) 00469 { 00470 /* Enable PLL48M1CLK output */ 00471 __HAL_RCC_PLLCLKOUT_ENABLE(RCC_PLL_48M1CLK); 00472 } 00473 else if(PeriphClkInit->Sdmmc1ClockSelection == RCC_SDMMC1CLKSOURCE_PLLSAI1) 00474 { 00475 /* PLLSAI1 parameters N & Q configuration and clock output (PLLSAI1ClockOut) */ 00476 ret = RCCEx_PLLSAI1_ConfigNQ(&(PeriphClkInit->PLLSAI1)); 00477 00478 if(ret != HAL_OK) 00479 { 00480 /* set overall return value */ 00481 status = ret; 00482 } 00483 } 00484 } 00485 00486 /*-------------------------- RNG clock source configuration ----------------------*/ 00487 if(((PeriphClkInit->PeriphClockSelection) & RCC_PERIPHCLK_RNG) == (RCC_PERIPHCLK_RNG)) 00488 { 00489 assert_param(IS_RCC_RNGCLKSOURCE(PeriphClkInit->RngClockSelection)); 00490 __HAL_RCC_RNG_CONFIG(PeriphClkInit->RngClockSelection); 00491 00492 if(PeriphClkInit->RngClockSelection == RCC_RNGCLKSOURCE_PLL) 00493 { 00494 /* Enable PLL48M1CLK output */ 00495 __HAL_RCC_PLLCLKOUT_ENABLE(RCC_PLL_48M1CLK); 00496 } 00497 else if(PeriphClkInit->RngClockSelection == RCC_RNGCLKSOURCE_PLLSAI1) 00498 { 00499 /* PLLSAI1 parameters N & Q configuration and clock output (PLLSAI1ClockOut) */ 00500 ret = RCCEx_PLLSAI1_ConfigNQ(&(PeriphClkInit->PLLSAI1)); 00501 00502 if(ret != HAL_OK) 00503 { 00504 /* set overall return value */ 00505 status = ret; 00506 } 00507 } 00508 } 00509 00510 /*-------------------------- ADC clock source configuration ----------------------*/ 00511 if(((PeriphClkInit->PeriphClockSelection) & RCC_PERIPHCLK_ADC) == RCC_PERIPHCLK_ADC) 00512 { 00513 /* Check the parameters */ 00514 assert_param(IS_RCC_ADCCLKSOURCE(PeriphClkInit->AdcClockSelection)); 00515 00516 /* Configure the ADC interface clock source */ 00517 __HAL_RCC_ADC_CONFIG(PeriphClkInit->AdcClockSelection); 00518 00519 if(PeriphClkInit->AdcClockSelection == RCC_ADCCLKSOURCE_PLLSAI1) 00520 { 00521 /* PLLSAI1 parameters N & R configuration and clock output (PLLSAI1ClockOut) */ 00522 ret = RCCEx_PLLSAI1_ConfigNR(&(PeriphClkInit->PLLSAI1)); 00523 00524 if(ret != HAL_OK) 00525 { 00526 /* set overall return value */ 00527 status = ret; 00528 } 00529 } 00530 else if(PeriphClkInit->AdcClockSelection == RCC_ADCCLKSOURCE_PLLSAI2) 00531 { 00532 /* PLLSAI2 parameters N & R configuration and clock output (PLLSAI2ClockOut) */ 00533 ret = RCCEx_PLLSAI2_ConfigNR(&(PeriphClkInit->PLLSAI2)); 00534 00535 if(ret != HAL_OK) 00536 { 00537 /* set overall return value */ 00538 status = ret; 00539 } 00540 } 00541 } 00542 00543 /*-------------------------- SWPMI1 clock source configuration -------------------*/ 00544 if(((PeriphClkInit->PeriphClockSelection) & RCC_PERIPHCLK_SWPMI1) == RCC_PERIPHCLK_SWPMI1) 00545 { 00546 /* Check the parameters */ 00547 assert_param(IS_RCC_SWPMI1CLKSOURCE(PeriphClkInit->Swpmi1ClockSelection)); 00548 00549 /* Configure the SWPMI1 clock source */ 00550 __HAL_RCC_SWPMI1_CONFIG(PeriphClkInit->Swpmi1ClockSelection); 00551 } 00552 00553 /*-------------------------- DFSDM clock source configuration --------------------*/ 00554 if(((PeriphClkInit->PeriphClockSelection) & RCC_PERIPHCLK_DFSDM) == RCC_PERIPHCLK_DFSDM) 00555 { 00556 /* Check the parameters */ 00557 assert_param(IS_RCC_DFSDMCLKSOURCE(PeriphClkInit->DfsdmClockSelection)); 00558 00559 /* Configure the DFSDM interface clock source */ 00560 __HAL_RCC_DFSDM_CONFIG(PeriphClkInit->DfsdmClockSelection); 00561 } 00562 00563 return status; 00564 } 00565 00566 /** 00567 * @brief Get the RCC_ClkInitStruct according to the internal RCC configuration registers. 00568 * @param PeriphClkInit pointer to an RCC_PeriphCLKInitTypeDef structure that 00569 * returns the configuration information for the Extended Peripherals 00570 * clocks(SAI1, SAI2, LPTIM1, LPTIM2, I2C1, I2C2, I2C3, LPUART, 00571 * USART1, USART2, USART3, UART4, UART5, RTC, ADCx, DFSDMx, SWPMI1, USB, SDMMC1 and RNG). 00572 * @retval None 00573 */ 00574 void HAL_RCCEx_GetPeriphCLKConfig(RCC_PeriphCLKInitTypeDef *PeriphClkInit) 00575 { 00576 /* Set all possible values for the extended clock type parameter------------*/ 00577 00578 #if defined(STM32L471xx) 00579 00580 PeriphClkInit->PeriphClockSelection = RCC_PERIPHCLK_USART1 | RCC_PERIPHCLK_USART2 | RCC_PERIPHCLK_USART3 | RCC_PERIPHCLK_UART4 | RCC_PERIPHCLK_UART5 | \ 00581 RCC_PERIPHCLK_LPUART1 | RCC_PERIPHCLK_I2C1 | RCC_PERIPHCLK_I2C2 | RCC_PERIPHCLK_I2C3 | RCC_PERIPHCLK_LPTIM1 | \ 00582 RCC_PERIPHCLK_LPTIM2 | RCC_PERIPHCLK_SAI1 | RCC_PERIPHCLK_SAI2 | RCC_PERIPHCLK_SDMMC1 | \ 00583 RCC_PERIPHCLK_RNG | RCC_PERIPHCLK_ADC | RCC_PERIPHCLK_SWPMI1 | RCC_PERIPHCLK_DFSDM | RCC_PERIPHCLK_RTC ; 00584 00585 #else /* defined(STM32L475xx) || defined(STM32L476xx) || defined(STM32L485xx) || defined(STM32L486xx) */ 00586 00587 PeriphClkInit->PeriphClockSelection = RCC_PERIPHCLK_USART1 | RCC_PERIPHCLK_USART2 | RCC_PERIPHCLK_USART3 | RCC_PERIPHCLK_UART4 | RCC_PERIPHCLK_UART5 | \ 00588 RCC_PERIPHCLK_LPUART1 | RCC_PERIPHCLK_I2C1 | RCC_PERIPHCLK_I2C2 | RCC_PERIPHCLK_I2C3 | RCC_PERIPHCLK_LPTIM1 | \ 00589 RCC_PERIPHCLK_LPTIM2 | RCC_PERIPHCLK_SAI1 | RCC_PERIPHCLK_SAI2 | RCC_PERIPHCLK_USB | RCC_PERIPHCLK_SDMMC1 | \ 00590 RCC_PERIPHCLK_RNG | RCC_PERIPHCLK_ADC | RCC_PERIPHCLK_SWPMI1 | RCC_PERIPHCLK_DFSDM | RCC_PERIPHCLK_RTC ; 00591 00592 #endif /* STM32L471xx */ 00593 00594 /* Get the PLLSAI1 Clock configuration -----------------------------------------------*/ 00595 PeriphClkInit->PLLSAI1.PLLSAI1N = (uint32_t)((RCC->PLLSAI1CFGR & RCC_PLLSAI1CFGR_PLLSAI1N) >> POSITION_VAL(RCC_PLLSAI1CFGR_PLLSAI1N)); 00596 PeriphClkInit->PLLSAI1.PLLSAI1P = (uint32_t)(((RCC->PLLSAI1CFGR & RCC_PLLSAI1CFGR_PLLSAI1P) >> POSITION_VAL(RCC_PLLSAI1CFGR_PLLSAI1P)) << 4)+7; 00597 PeriphClkInit->PLLSAI1.PLLSAI1R = (uint32_t)(((RCC->PLLSAI1CFGR & RCC_PLLSAI1CFGR_PLLSAI1R) >> POSITION_VAL(RCC_PLLSAI1CFGR_PLLSAI1R))+1)* 2; 00598 PeriphClkInit->PLLSAI1.PLLSAI1Q = (uint32_t)(((RCC->PLLSAI1CFGR & RCC_PLLSAI1CFGR_PLLSAI1Q) >> POSITION_VAL(RCC_PLLSAI1CFGR_PLLSAI1Q))+1)* 2; 00599 /* Get the PLLSAI2 Clock configuration -----------------------------------------------*/ 00600 PeriphClkInit->PLLSAI2.PLLSAI2N = (uint32_t)((RCC->PLLSAI2CFGR & RCC_PLLSAI2CFGR_PLLSAI2N) >> POSITION_VAL(RCC_PLLSAI2CFGR_PLLSAI2N)); 00601 PeriphClkInit->PLLSAI2.PLLSAI2P = (uint32_t)(((RCC->PLLSAI2CFGR & RCC_PLLSAI2CFGR_PLLSAI2P) >> POSITION_VAL(RCC_PLLSAI2CFGR_PLLSAI2P)) << 4)+7; 00602 PeriphClkInit->PLLSAI2.PLLSAI2R = (uint32_t)(((RCC->PLLSAI2CFGR & RCC_PLLSAI2CFGR_PLLSAI2R)>> POSITION_VAL(RCC_PLLSAI2CFGR_PLLSAI2R))+1)* 2; 00603 00604 /* Get the USART1 clock source ---------------------------------------------*/ 00605 PeriphClkInit->Usart1ClockSelection = __HAL_RCC_GET_USART1_SOURCE(); 00606 /* Get the USART2 clock source ---------------------------------------------*/ 00607 PeriphClkInit->Usart2ClockSelection = __HAL_RCC_GET_USART2_SOURCE(); 00608 /* Get the USART3 clock source ---------------------------------------------*/ 00609 PeriphClkInit->Usart3ClockSelection = __HAL_RCC_GET_USART3_SOURCE(); 00610 /* Get the UART4 clock source ----------------------------------------------*/ 00611 PeriphClkInit->Uart4ClockSelection = __HAL_RCC_GET_UART4_SOURCE(); 00612 /* Get the UART5 clock source ----------------------------------------------*/ 00613 PeriphClkInit->Uart5ClockSelection = __HAL_RCC_GET_UART5_SOURCE(); 00614 /* Get the LPUART1 clock source --------------------------------------------*/ 00615 PeriphClkInit->Lpuart1ClockSelection = __HAL_RCC_GET_LPUART1_SOURCE(); 00616 /* Get the I2C1 clock source -----------------------------------------------*/ 00617 PeriphClkInit->I2c1ClockSelection = __HAL_RCC_GET_I2C1_SOURCE(); 00618 /* Get the I2C2 clock source ----------------------------------------------*/ 00619 PeriphClkInit->I2c2ClockSelection = __HAL_RCC_GET_I2C2_SOURCE(); 00620 /* Get the I2C3 clock source -----------------------------------------------*/ 00621 PeriphClkInit->I2c3ClockSelection = __HAL_RCC_GET_I2C3_SOURCE(); 00622 /* Get the LPTIM1 clock source ---------------------------------------------*/ 00623 PeriphClkInit->Lptim1ClockSelection = __HAL_RCC_GET_LPTIM1_SOURCE(); 00624 /* Get the LPTIM2 clock source ---------------------------------------------*/ 00625 PeriphClkInit->Lptim2ClockSelection = __HAL_RCC_GET_LPTIM2_SOURCE(); 00626 /* Get the SAI1 clock source -----------------------------------------------*/ 00627 PeriphClkInit->Sai1ClockSelection = __HAL_RCC_GET_SAI1_SOURCE(); 00628 /* Get the SAI2 clock source -----------------------------------------------*/ 00629 PeriphClkInit->Sai2ClockSelection = __HAL_RCC_GET_SAI2_SOURCE(); 00630 /* Get the RTC clock source ------------------------------------------------*/ 00631 PeriphClkInit->RTCClockSelection = __HAL_RCC_GET_RTC_SOURCE(); 00632 00633 #if defined(STM32L475xx) || defined(STM32L476xx) || defined(STM32L485xx) || defined(STM32L486xx) 00634 /* Get the USB clock source ------------------------------------------------*/ 00635 PeriphClkInit->UsbClockSelection = __HAL_RCC_GET_USB_SOURCE(); 00636 #endif /* STM32L475xx || STM32L476xx || STM32L485xx || STM32L486xx */ 00637 00638 /* Get the SDMMC1 clock source ---------------------------------------------*/ 00639 PeriphClkInit->Sdmmc1ClockSelection = __HAL_RCC_GET_SDMMC1_SOURCE(); 00640 /* Get the RNG clock source ------------------------------------------------*/ 00641 PeriphClkInit->RngClockSelection = __HAL_RCC_GET_RNG_SOURCE(); 00642 /* Get the ADC clock source -----------------------------------------------*/ 00643 PeriphClkInit->AdcClockSelection = __HAL_RCC_GET_ADC_SOURCE(); 00644 /* Get the SWPMI1 clock source ----------------------------------------------*/ 00645 PeriphClkInit->Swpmi1ClockSelection = __HAL_RCC_GET_SWPMI1_SOURCE(); 00646 /* Get the DFSDM clock source -------------------------------------------*/ 00647 PeriphClkInit->DfsdmClockSelection = __HAL_RCC_GET_DFSDM_SOURCE(); 00648 } 00649 00650 /** 00651 * @brief Return the peripheral clock frequency for peripherals with clock source from PLLSAIs 00652 * @note Return 0 if peripheral clock identifier not managed by this API 00653 * @param PeriphClk Peripheral clock identifier 00654 * This parameter can be one of the following values: 00655 * @arg @ref RCC_PERIPHCLK_RTC RTC peripheral clock 00656 * @arg @ref RCC_PERIPHCLK_ADC ADC peripheral clock 00657 * @arg @ref RCC_PERIPHCLK_DFSDM DFSDM peripheral clock 00658 * @arg @ref RCC_PERIPHCLK_I2C1 I2C1 peripheral clock 00659 * @arg @ref RCC_PERIPHCLK_I2C2 I2C2 peripheral clock 00660 * @arg @ref RCC_PERIPHCLK_I2C3 I2C3 peripheral clock 00661 * @arg @ref RCC_PERIPHCLK_LPTIM1 LPTIM1 peripheral clock 00662 * @arg @ref RCC_PERIPHCLK_LPTIM2 LPTIM2 peripheral clock 00663 * @arg @ref RCC_PERIPHCLK_LPUART1 LPUART1 peripheral clock 00664 * @arg @ref RCC_PERIPHCLK_RNG RNG peripheral clock 00665 * @arg @ref RCC_PERIPHCLK_SAI1 SAI1 peripheral clock 00666 * @arg @ref RCC_PERIPHCLK_SAI2 SAI2 peripheral clock 00667 * @arg @ref RCC_PERIPHCLK_SDMMC1 SDMMC1 peripheral clock 00668 * @arg @ref RCC_PERIPHCLK_SWPMI1 SWPMI1 peripheral clock 00669 * @arg @ref RCC_PERIPHCLK_USART1 USART1 peripheral clock 00670 * @arg @ref RCC_PERIPHCLK_USART2 USART1 peripheral clock 00671 * @arg @ref RCC_PERIPHCLK_USART3 USART1 peripheral clock 00672 * @arg @ref RCC_PERIPHCLK_UART4 USART1 peripheral clock 00673 * @arg @ref RCC_PERIPHCLK_UART5 USART1 peripheral clock 00674 * @arg @ref RCC_PERIPHCLK_USB USB peripheral clock (only for devices with USB) 00675 * @retval Frequency in Hz 00676 */ 00677 uint32_t HAL_RCCEx_GetPeriphCLKFreq(uint32_t PeriphClk) 00678 { 00679 uint32_t frequency = 0; 00680 uint32_t srcclk = 0; 00681 uint32_t pllvco = 0, plln = 0, pllp = 0; 00682 00683 /* Check the parameters */ 00684 assert_param(IS_RCC_PERIPHCLOCK(PeriphClk)); 00685 00686 if(PeriphClk == RCC_PERIPHCLK_RTC) 00687 { 00688 /* Get the current RTC source */ 00689 srcclk = __HAL_RCC_GET_RTC_SOURCE(); 00690 00691 /* Check if LSE is ready and if RTC clock selection is LSE */ 00692 if ((srcclk == RCC_RTCCLKSOURCE_LSE) && (HAL_IS_BIT_SET(RCC->BDCR, RCC_BDCR_LSERDY))) 00693 { 00694 frequency = LSE_VALUE; 00695 } 00696 /* Check if LSI is ready and if RTC clock selection is LSI */ 00697 else if ((srcclk == RCC_RTCCLKSOURCE_LSI) && (HAL_IS_BIT_SET(RCC->CSR, RCC_CSR_LSIRDY))) 00698 { 00699 frequency = LSI_VALUE; 00700 } 00701 /* Check if HSE is ready and if RTC clock selection is HSI_DIV32*/ 00702 else if ((srcclk == RCC_RTCCLKSOURCE_HSE_DIV32) && (HAL_IS_BIT_SET(RCC->CR, RCC_CR_HSERDY))) 00703 { 00704 frequency = HSE_VALUE / 32; 00705 } 00706 /* Clock not enabled for RTC*/ 00707 else 00708 { 00709 frequency = 0; 00710 } 00711 } 00712 else 00713 { 00714 /* Other external peripheral clock source than RTC */ 00715 00716 /* Compute PLL clock input */ 00717 if(__HAL_RCC_GET_PLL_OSCSOURCE() == RCC_PLLSOURCE_MSI) /* MSI ? */ 00718 { 00719 pllvco = (1 << ((__HAL_RCC_GET_MSI_RANGE() >> 4) - 4)) * 1000000; 00720 } 00721 else if(__HAL_RCC_GET_PLL_OSCSOURCE() == RCC_PLLSOURCE_HSI) /* HSI ? */ 00722 { 00723 pllvco = HSI_VALUE; 00724 } 00725 else if(__HAL_RCC_GET_PLL_OSCSOURCE() == RCC_PLLSOURCE_HSE) /* HSE ? */ 00726 { 00727 pllvco = HSE_VALUE; 00728 } 00729 else /* No source */ 00730 { 00731 pllvco = 0; 00732 } 00733 00734 /* f(PLL Source) / PLLM */ 00735 pllvco = (pllvco / ((READ_BIT(RCC->PLLCFGR, RCC_PLLCFGR_PLLM) >> 4) + 1)); 00736 00737 switch(PeriphClk) 00738 { 00739 case RCC_PERIPHCLK_SAI1: 00740 case RCC_PERIPHCLK_SAI2: 00741 00742 if(PeriphClk == RCC_PERIPHCLK_SAI1) 00743 { 00744 srcclk = READ_BIT(RCC->CCIPR, RCC_CCIPR_SAI1SEL); 00745 00746 if(srcclk == RCC_SAI1CLKSOURCE_PIN) 00747 { 00748 frequency = EXTERNAL_SAI1_CLOCK_VALUE; 00749 } 00750 /* Else, PLL clock output to check below */ 00751 } 00752 else /* RCC_PERIPHCLK_SAI2 */ 00753 { 00754 srcclk = READ_BIT(RCC->CCIPR, RCC_CCIPR_SAI2SEL); 00755 00756 if(srcclk == RCC_SAI2CLKSOURCE_PIN) 00757 { 00758 frequency = EXTERNAL_SAI2_CLOCK_VALUE; 00759 } 00760 /* Else, PLL clock output to check below */ 00761 } 00762 00763 if(frequency == 0) 00764 { 00765 if((srcclk == RCC_SAI1CLKSOURCE_PLL) || (srcclk == RCC_SAI2CLKSOURCE_PLL)) 00766 { 00767 if(__HAL_RCC_GET_PLLCLKOUT_CONFIG(RCC_PLL_SAI3CLK) != RESET) 00768 { 00769 /* f(PLLSAI3CLK) = f(VCO input) * PLLN / PLLP */ 00770 plln = READ_BIT(RCC->PLLCFGR, RCC_PLLCFGR_PLLN) >> 8; 00771 if(READ_BIT(RCC->PLLCFGR, RCC_PLLCFGR_PLLP) != RESET) 00772 { 00773 pllp = 17; 00774 } 00775 else 00776 { 00777 pllp = 7; 00778 } 00779 frequency = (pllvco * plln) / pllp; 00780 } 00781 } 00782 else if(srcclk == 0) /* RCC_SAI1CLKSOURCE_PLLSAI1 || RCC_SAI2CLKSOURCE_PLLSAI1 */ 00783 { 00784 if(__HAL_RCC_GET_PLLSAI1CLKOUT_CONFIG(RCC_PLLSAI1_SAI1CLK) != RESET) 00785 { 00786 /* f(PLLSAI1CLK) = f(VCOSAI1 input) * PLLSAI1N / PLLSAI1P */ 00787 plln = READ_BIT(RCC->PLLSAI1CFGR, RCC_PLLSAI1CFGR_PLLSAI1N) >> 8; 00788 if(READ_BIT(RCC->PLLSAI1CFGR, RCC_PLLSAI1CFGR_PLLSAI1P) != RESET) 00789 { 00790 pllp = 17; 00791 } 00792 else 00793 { 00794 pllp = 7; 00795 } 00796 frequency = (pllvco * plln) / pllp; 00797 } 00798 } 00799 else if((srcclk == RCC_SAI1CLKSOURCE_PLLSAI2) || (srcclk == RCC_SAI2CLKSOURCE_PLLSAI2)) 00800 { 00801 if(__HAL_RCC_GET_PLLSAI2CLKOUT_CONFIG(RCC_PLLSAI2_SAI2CLK) != RESET) 00802 { 00803 /* f(PLLSAI2CLK) = f(VCOSAI2 input) * PLLSAI2N / PLLSAI2P */ 00804 plln = READ_BIT(RCC->PLLSAI2CFGR, RCC_PLLSAI2CFGR_PLLSAI2N) >> 8; 00805 if(READ_BIT(RCC->PLLSAI2CFGR, RCC_PLLSAI2CFGR_PLLSAI2P) != RESET) 00806 { 00807 pllp = 17; 00808 } 00809 else 00810 { 00811 pllp = 7; 00812 } 00813 frequency = (pllvco * plln) / pllp; 00814 } 00815 } 00816 else 00817 { 00818 /* No clock source */ 00819 frequency = 0; 00820 } 00821 } 00822 break; 00823 00824 #if defined(STM32L475xx) || defined(STM32L476xx) || defined(STM32L485xx) || defined(STM32L486xx) 00825 00826 case RCC_PERIPHCLK_USB: 00827 00828 #endif /* STM32L475xx || STM32L476xx || STM32L485xx || STM32L486xx */ 00829 00830 case RCC_PERIPHCLK_RNG: 00831 case RCC_PERIPHCLK_SDMMC1: 00832 00833 srcclk = READ_BIT(RCC->CCIPR, RCC_CCIPR_CLK48SEL); 00834 00835 if(srcclk == RCC_CCIPR_CLK48SEL) /* MSI ? */ 00836 { 00837 frequency = (1 << ((__HAL_RCC_GET_MSI_RANGE() >> 4) - 4)) * 1000000; 00838 } 00839 else if(srcclk == RCC_CCIPR_CLK48SEL_1) /* PLL ? */ 00840 { 00841 /* f(PLL48M1CLK) = f(VCO input) * PLLN / PLLQ */ 00842 plln = READ_BIT(RCC->PLLCFGR, RCC_PLLCFGR_PLLN) >> 8; 00843 frequency = (pllvco * plln) / (((READ_BIT(RCC->PLLCFGR, RCC_PLLCFGR_PLLQ) >> 21) + 1) << 1); 00844 } 00845 else if(srcclk == RCC_CCIPR_CLK48SEL_0) /* PLLSAI1 ? */ 00846 { 00847 /* f(PLL48M2CLK) = f(VCOSAI1 input) * PLLSAI1N / PLLSAI1Q */ 00848 plln = READ_BIT(RCC->PLLSAI1CFGR, RCC_PLLSAI1CFGR_PLLSAI1N) >> 8; 00849 frequency = (pllvco * plln) / (((READ_BIT(RCC->PLLSAI1CFGR, RCC_PLLSAI1CFGR_PLLSAI1Q) >> 21) + 1) << 1); 00850 } 00851 else /* No clock source */ 00852 { 00853 frequency = 0; 00854 } 00855 break; 00856 00857 case RCC_PERIPHCLK_USART1: 00858 /* Get the current USART1 source */ 00859 srcclk = __HAL_RCC_GET_USART1_SOURCE(); 00860 00861 if(srcclk == RCC_USART1CLKSOURCE_PCLK2) 00862 { 00863 frequency = HAL_RCC_GetPCLK2Freq(); 00864 } 00865 else if(srcclk == RCC_USART1CLKSOURCE_SYSCLK) 00866 { 00867 frequency = HAL_RCC_GetSysClockFreq(); 00868 } 00869 else if((srcclk == RCC_USART1CLKSOURCE_HSI) && (HAL_IS_BIT_SET(RCC->CR, RCC_CR_HSIRDY))) 00870 { 00871 frequency = HSI_VALUE; 00872 } 00873 else if((srcclk == RCC_USART1CLKSOURCE_LSE) && (HAL_IS_BIT_SET(RCC->BDCR, RCC_BDCR_LSERDY))) 00874 { 00875 frequency = LSE_VALUE; 00876 } 00877 /* Clock not enabled for USART1 */ 00878 else 00879 { 00880 frequency = 0; 00881 } 00882 break; 00883 00884 case RCC_PERIPHCLK_USART2: 00885 /* Get the current USART2 source */ 00886 srcclk = __HAL_RCC_GET_USART2_SOURCE(); 00887 00888 if(srcclk == RCC_USART2CLKSOURCE_PCLK1) 00889 { 00890 frequency = HAL_RCC_GetPCLK1Freq(); 00891 } 00892 else if(srcclk == RCC_USART2CLKSOURCE_SYSCLK) 00893 { 00894 frequency = HAL_RCC_GetSysClockFreq(); 00895 } 00896 else if((srcclk == RCC_USART2CLKSOURCE_HSI) && (HAL_IS_BIT_SET(RCC->CR, RCC_CR_HSIRDY))) 00897 { 00898 frequency = HSI_VALUE; 00899 } 00900 else if((srcclk == RCC_USART2CLKSOURCE_LSE) && (HAL_IS_BIT_SET(RCC->BDCR, RCC_BDCR_LSERDY))) 00901 { 00902 frequency = LSE_VALUE; 00903 } 00904 /* Clock not enabled for USART2 */ 00905 else 00906 { 00907 frequency = 0; 00908 } 00909 break; 00910 00911 case RCC_PERIPHCLK_USART3: 00912 /* Get the current USART3 source */ 00913 srcclk = __HAL_RCC_GET_USART3_SOURCE(); 00914 00915 if(srcclk == RCC_USART3CLKSOURCE_PCLK1) 00916 { 00917 frequency = HAL_RCC_GetPCLK1Freq(); 00918 } 00919 else if(srcclk == RCC_USART3CLKSOURCE_SYSCLK) 00920 { 00921 frequency = HAL_RCC_GetSysClockFreq(); 00922 } 00923 else if((srcclk == RCC_USART3CLKSOURCE_HSI) && (HAL_IS_BIT_SET(RCC->CR, RCC_CR_HSIRDY))) 00924 { 00925 frequency = HSI_VALUE; 00926 } 00927 else if((srcclk == RCC_USART3CLKSOURCE_LSE) && (HAL_IS_BIT_SET(RCC->BDCR, RCC_BDCR_LSERDY))) 00928 { 00929 frequency = LSE_VALUE; 00930 } 00931 /* Clock not enabled for USART3 */ 00932 else 00933 { 00934 frequency = 0; 00935 } 00936 break; 00937 00938 case RCC_PERIPHCLK_UART4: 00939 /* Get the current UART4 source */ 00940 srcclk = __HAL_RCC_GET_UART4_SOURCE(); 00941 00942 if(srcclk == RCC_UART4CLKSOURCE_PCLK1) 00943 { 00944 frequency = HAL_RCC_GetPCLK1Freq(); 00945 } 00946 else if(srcclk == RCC_UART4CLKSOURCE_SYSCLK) 00947 { 00948 frequency = HAL_RCC_GetSysClockFreq(); 00949 } 00950 else if((srcclk == RCC_UART4CLKSOURCE_HSI) && (HAL_IS_BIT_SET(RCC->CR, RCC_CR_HSIRDY))) 00951 { 00952 frequency = HSI_VALUE; 00953 } 00954 else if((srcclk == RCC_UART4CLKSOURCE_LSE) && (HAL_IS_BIT_SET(RCC->BDCR, RCC_BDCR_LSERDY))) 00955 { 00956 frequency = LSE_VALUE; 00957 } 00958 /* Clock not enabled for UART4 */ 00959 else 00960 { 00961 frequency = 0; 00962 } 00963 break; 00964 00965 case RCC_PERIPHCLK_UART5: 00966 /* Get the current UART5 source */ 00967 srcclk = __HAL_RCC_GET_UART5_SOURCE(); 00968 00969 if(srcclk == RCC_UART5CLKSOURCE_PCLK1) 00970 { 00971 frequency = HAL_RCC_GetPCLK1Freq(); 00972 } 00973 else if(srcclk == RCC_UART5CLKSOURCE_SYSCLK) 00974 { 00975 frequency = HAL_RCC_GetSysClockFreq(); 00976 } 00977 else if((srcclk == RCC_UART5CLKSOURCE_HSI) && (HAL_IS_BIT_SET(RCC->CR, RCC_CR_HSIRDY))) 00978 { 00979 frequency = HSI_VALUE; 00980 } 00981 else if((srcclk == RCC_UART5CLKSOURCE_LSE) && (HAL_IS_BIT_SET(RCC->BDCR, RCC_BDCR_LSERDY))) 00982 { 00983 frequency = LSE_VALUE; 00984 } 00985 /* Clock not enabled for UART5 */ 00986 else 00987 { 00988 frequency = 0; 00989 } 00990 break; 00991 00992 case RCC_PERIPHCLK_LPUART1: 00993 /* Get the current LPUART1 source */ 00994 srcclk = __HAL_RCC_GET_LPUART1_SOURCE(); 00995 00996 if(srcclk == RCC_LPUART1CLKSOURCE_PCLK1) 00997 { 00998 frequency = HAL_RCC_GetPCLK1Freq(); 00999 } 01000 else if(srcclk == RCC_LPUART1CLKSOURCE_SYSCLK) 01001 { 01002 frequency = HAL_RCC_GetSysClockFreq(); 01003 } 01004 else if((srcclk == RCC_LPUART1CLKSOURCE_HSI) && (HAL_IS_BIT_SET(RCC->CR, RCC_CR_HSIRDY))) 01005 { 01006 frequency = HSI_VALUE; 01007 } 01008 else if((srcclk == RCC_LPUART1CLKSOURCE_LSE) && (HAL_IS_BIT_SET(RCC->BDCR, RCC_BDCR_LSERDY))) 01009 { 01010 frequency = LSE_VALUE; 01011 } 01012 /* Clock not enabled for LPUART1 */ 01013 else 01014 { 01015 frequency = 0; 01016 } 01017 break; 01018 01019 case RCC_PERIPHCLK_ADC: 01020 01021 srcclk = __HAL_RCC_GET_ADC_SOURCE(); 01022 01023 if(srcclk == RCC_ADCCLKSOURCE_SYSCLK) 01024 { 01025 frequency = HAL_RCC_GetSysClockFreq(); 01026 } 01027 else if(srcclk == RCC_ADCCLKSOURCE_PLLSAI1) 01028 { 01029 if(__HAL_RCC_GET_PLLSAI1CLKOUT_CONFIG(RCC_PLLSAI1_ADC1CLK) != RESET) 01030 { 01031 /* f(PLLADC1CLK) = f(VCOSAI1 input) * PLLSAI1N / PLLSAI1R */ 01032 plln = READ_BIT(RCC->PLLSAI1CFGR, RCC_PLLSAI1CFGR_PLLSAI1N) >> 8; 01033 frequency = (pllvco * plln) / (((READ_BIT(RCC->PLLSAI1CFGR, RCC_PLLSAI1CFGR_PLLSAI1R) >> 24) + 1) << 1); 01034 } 01035 } 01036 else if(srcclk == RCC_ADCCLKSOURCE_PLLSAI2) 01037 { 01038 if(__HAL_RCC_GET_PLLSAI2CLKOUT_CONFIG(RCC_PLLSAI2_ADC2CLK) != RESET) 01039 { 01040 /* f(PLLADC2CLK) = f(VCOSAI2 input) * PLLSAI2N / PLLSAI2R */ 01041 plln = READ_BIT(RCC->PLLSAI2CFGR, RCC_PLLSAI2CFGR_PLLSAI2N) >> 8; 01042 frequency = (pllvco * plln) / (((READ_BIT(RCC->PLLSAI2CFGR, RCC_PLLSAI2CFGR_PLLSAI2R) >> 24) + 1) << 1); 01043 } 01044 } 01045 /* Clock not enabled for ADC */ 01046 else 01047 { 01048 frequency = 0; 01049 } 01050 break; 01051 01052 case RCC_PERIPHCLK_DFSDM: 01053 /* Get the current DFSDM source */ 01054 srcclk = __HAL_RCC_GET_DFSDM_SOURCE(); 01055 01056 if(srcclk == RCC_DFSDMCLKSOURCE_PCLK) 01057 { 01058 frequency = HAL_RCC_GetPCLK1Freq(); 01059 } 01060 else 01061 { 01062 frequency = HAL_RCC_GetSysClockFreq(); 01063 } 01064 break; 01065 01066 case RCC_PERIPHCLK_I2C1: 01067 /* Get the current I2C1 source */ 01068 srcclk = __HAL_RCC_GET_I2C1_SOURCE(); 01069 01070 if(srcclk == RCC_I2C1CLKSOURCE_PCLK1) 01071 { 01072 frequency = HAL_RCC_GetPCLK1Freq(); 01073 } 01074 else if(srcclk == RCC_I2C1CLKSOURCE_SYSCLK) 01075 { 01076 frequency = HAL_RCC_GetSysClockFreq(); 01077 } 01078 else if((srcclk == RCC_I2C1CLKSOURCE_HSI) && (HAL_IS_BIT_SET(RCC->CR, RCC_CR_HSIRDY))) 01079 { 01080 frequency = HSI_VALUE; 01081 } 01082 /* Clock not enabled for I2C1 */ 01083 else 01084 { 01085 frequency = 0; 01086 } 01087 break; 01088 01089 case RCC_PERIPHCLK_I2C2: 01090 /* Get the current I2C2 source */ 01091 srcclk = __HAL_RCC_GET_I2C2_SOURCE(); 01092 01093 if(srcclk == RCC_I2C2CLKSOURCE_PCLK1) 01094 { 01095 frequency = HAL_RCC_GetPCLK1Freq(); 01096 } 01097 else if(srcclk == RCC_I2C2CLKSOURCE_SYSCLK) 01098 { 01099 frequency = HAL_RCC_GetSysClockFreq(); 01100 } 01101 else if((srcclk == RCC_I2C2CLKSOURCE_HSI) && (HAL_IS_BIT_SET(RCC->CR, RCC_CR_HSIRDY))) 01102 { 01103 frequency = HSI_VALUE; 01104 } 01105 /* Clock not enabled for I2C2 */ 01106 else 01107 { 01108 frequency = 0; 01109 } 01110 break; 01111 01112 case RCC_PERIPHCLK_I2C3: 01113 /* Get the current I2C3 source */ 01114 srcclk = __HAL_RCC_GET_I2C3_SOURCE(); 01115 01116 if(srcclk == RCC_I2C3CLKSOURCE_PCLK1) 01117 { 01118 frequency = HAL_RCC_GetPCLK1Freq(); 01119 } 01120 else if(srcclk == RCC_I2C3CLKSOURCE_SYSCLK) 01121 { 01122 frequency = HAL_RCC_GetSysClockFreq(); 01123 } 01124 else if((srcclk == RCC_I2C3CLKSOURCE_HSI) && (HAL_IS_BIT_SET(RCC->CR, RCC_CR_HSIRDY))) 01125 { 01126 frequency = HSI_VALUE; 01127 } 01128 /* Clock not enabled for I2C3 */ 01129 else 01130 { 01131 frequency = 0; 01132 } 01133 break; 01134 01135 case RCC_PERIPHCLK_LPTIM1: 01136 /* Get the current LPTIM1 source */ 01137 srcclk = __HAL_RCC_GET_LPTIM1_SOURCE(); 01138 01139 if(srcclk == RCC_LPTIM1CLKSOURCE_PCLK) 01140 { 01141 frequency = HAL_RCC_GetPCLK1Freq(); 01142 } 01143 else if((srcclk == RCC_LPTIM1CLKSOURCE_LSI) && (HAL_IS_BIT_SET(RCC->CSR, RCC_CSR_LSIRDY))) 01144 { 01145 frequency = LSI_VALUE; 01146 } 01147 else if((srcclk == RCC_LPTIM1CLKSOURCE_HSI) && (HAL_IS_BIT_SET(RCC->CR, RCC_CR_HSIRDY))) 01148 { 01149 frequency = HSI_VALUE; 01150 } 01151 else if ((srcclk == RCC_LPTIM1CLKSOURCE_LSE) && (HAL_IS_BIT_SET(RCC->BDCR, RCC_BDCR_LSERDY))) 01152 { 01153 frequency = LSE_VALUE; 01154 } 01155 /* Clock not enabled for LPTIM1 */ 01156 else 01157 { 01158 frequency = 0; 01159 } 01160 break; 01161 01162 case RCC_PERIPHCLK_LPTIM2: 01163 /* Get the current LPTIM2 source */ 01164 srcclk = __HAL_RCC_GET_LPTIM2_SOURCE(); 01165 01166 if(srcclk == RCC_LPTIM2CLKSOURCE_PCLK) 01167 { 01168 frequency = HAL_RCC_GetPCLK1Freq(); 01169 } 01170 else if((srcclk == RCC_LPTIM2CLKSOURCE_LSI) && (HAL_IS_BIT_SET(RCC->CSR, RCC_CSR_LSIRDY))) 01171 { 01172 frequency = LSI_VALUE; 01173 } 01174 else if((srcclk == RCC_LPTIM2CLKSOURCE_HSI) && (HAL_IS_BIT_SET(RCC->CR, RCC_CR_HSIRDY))) 01175 { 01176 frequency = HSI_VALUE; 01177 } 01178 else if ((srcclk == RCC_LPTIM2CLKSOURCE_LSE) && (HAL_IS_BIT_SET(RCC->BDCR, RCC_BDCR_LSERDY))) 01179 { 01180 frequency = LSE_VALUE; 01181 } 01182 /* Clock not enabled for LPTIM2 */ 01183 else 01184 { 01185 frequency = 0; 01186 } 01187 break; 01188 01189 case RCC_PERIPHCLK_SWPMI1: 01190 /* Get the current SWPMI1 source */ 01191 srcclk = __HAL_RCC_GET_SWPMI1_SOURCE(); 01192 01193 if(srcclk == RCC_SWPMI1CLKSOURCE_PCLK) 01194 { 01195 frequency = HAL_RCC_GetPCLK1Freq(); 01196 } 01197 else if((srcclk == RCC_SWPMI1CLKSOURCE_HSI) && (HAL_IS_BIT_SET(RCC->CR, RCC_CR_HSIRDY))) 01198 { 01199 frequency = HSI_VALUE; 01200 } 01201 /* Clock not enabled for SWPMI1 */ 01202 else 01203 { 01204 frequency = 0; 01205 } 01206 break; 01207 01208 default: 01209 break; 01210 } 01211 } 01212 01213 return(frequency); 01214 } 01215 01216 /** 01217 * @} 01218 */ 01219 01220 /** @defgroup RCCEx_Exported_Functions_Group2 Extended clock management functions 01221 * @brief Extended clock management functions 01222 * 01223 @verbatim 01224 =============================================================================== 01225 ##### Extended clock management functions ##### 01226 =============================================================================== 01227 [..] 01228 This subsection provides a set of functions allowing to control the 01229 activation or deactivation of MSI PLL-mode, PLLSAI1, PLLSAI2, LSE CSS, 01230 Low speed clock output and clock after wake-up from STOP mode. 01231 @endverbatim 01232 * @{ 01233 */ 01234 01235 /** 01236 * @brief Enable PLLSAI1. 01237 * @param PLLSAI1Init pointer to an RCC_PLLSAI1InitTypeDef structure that 01238 * contains the configuration information for the PLLSAI1 01239 * @retval HAL status 01240 */ 01241 HAL_StatusTypeDef HAL_RCCEx_EnablePLLSAI1(RCC_PLLSAI1InitTypeDef *PLLSAI1Init) 01242 { 01243 uint32_t tickstart = 0; 01244 HAL_StatusTypeDef status = HAL_OK; 01245 01246 /* check for PLLSAI1 Parameters used to output PLLSAI1CLK */ 01247 assert_param(IS_RCC_PLLSAI1N_VALUE(PLLSAI1Init->PLLSAI1N)); 01248 assert_param(IS_RCC_PLLSAI1P_VALUE(PLLSAI1Init->PLLSAI1P)); 01249 assert_param(IS_RCC_PLLSAI1Q_VALUE(PLLSAI1Init->PLLSAI1Q)); 01250 assert_param(IS_RCC_PLLSAI1R_VALUE(PLLSAI1Init->PLLSAI1R)); 01251 assert_param(IS_RCC_PLLSAI1CLOCKOUT_VALUE(PLLSAI1Init->PLLSAI1ClockOut)); 01252 01253 /* Disable the PLLSAI1 */ 01254 __HAL_RCC_PLLSAI1_DISABLE(); 01255 01256 /* Get Start Tick*/ 01257 tickstart = HAL_GetTick(); 01258 01259 /* Wait till PLLSAI1 is ready to be updated */ 01260 while(__HAL_RCC_GET_FLAG(RCC_FLAG_PLLSAI1RDY) != RESET) 01261 { 01262 if((HAL_GetTick() - tickstart) > PLLSAI1_TIMEOUT_VALUE) 01263 { 01264 status = HAL_TIMEOUT; 01265 break; 01266 } 01267 } 01268 01269 if(status == HAL_OK) 01270 { 01271 /* Configure the PLLSAI1 Multiplication factor N */ 01272 /* Configure the PLLSAI1 Division factors P, Q and R */ 01273 __HAL_RCC_PLLSAI1_CONFIG(PLLSAI1Init->PLLSAI1N, PLLSAI1Init->PLLSAI1P, PLLSAI1Init->PLLSAI1Q, PLLSAI1Init->PLLSAI1R); 01274 /* Configure the PLLSAI1 Clock output(s) */ 01275 __HAL_RCC_PLLSAI1CLKOUT_ENABLE(PLLSAI1Init->PLLSAI1ClockOut); 01276 01277 /* Enable the PLLSAI1 again by setting PLLSAI1ON to 1*/ 01278 __HAL_RCC_PLLSAI1_ENABLE(); 01279 01280 /* Get Start Tick*/ 01281 tickstart = HAL_GetTick(); 01282 01283 /* Wait till PLLSAI1 is ready */ 01284 while(__HAL_RCC_GET_FLAG(RCC_FLAG_PLLSAI1RDY) == RESET) 01285 { 01286 if((HAL_GetTick() - tickstart) > PLLSAI1_TIMEOUT_VALUE) 01287 { 01288 status = HAL_TIMEOUT; 01289 break; 01290 } 01291 } 01292 } 01293 01294 return status; 01295 } 01296 01297 /** 01298 * @brief Disable PLLSAI1. 01299 * @retval HAL status 01300 */ 01301 HAL_StatusTypeDef HAL_RCCEx_DisablePLLSAI1(void) 01302 { 01303 uint32_t tickstart = 0; 01304 HAL_StatusTypeDef status = HAL_OK; 01305 01306 /* Disable the PLLSAI1 */ 01307 __HAL_RCC_PLLSAI1_DISABLE(); 01308 01309 /* Get Start Tick*/ 01310 tickstart = HAL_GetTick(); 01311 01312 /* Wait till PLLSAI1 is ready */ 01313 while(__HAL_RCC_GET_FLAG(RCC_FLAG_PLLSAI1RDY) != RESET) 01314 { 01315 if((HAL_GetTick() - tickstart) > PLLSAI1_TIMEOUT_VALUE) 01316 { 01317 status = HAL_TIMEOUT; 01318 break; 01319 } 01320 } 01321 01322 /* Disable the PLLSAI1 Clock outputs */ 01323 __HAL_RCC_PLLSAI1CLKOUT_DISABLE(RCC_PLLSAI1_SAI1CLK|RCC_PLLSAI1_48M2CLK|RCC_PLLSAI1_ADC1CLK); 01324 01325 return status; 01326 } 01327 01328 /** 01329 * @brief Enable PLLSAI2. 01330 * @param PLLSAI2Init pointer to an RCC_PLLSAI2InitTypeDef structure that 01331 * contains the configuration information for the PLLSAI2 01332 * @retval HAL status 01333 */ 01334 HAL_StatusTypeDef HAL_RCCEx_EnablePLLSAI2(RCC_PLLSAI2InitTypeDef *PLLSAI2Init) 01335 { 01336 uint32_t tickstart = 0; 01337 HAL_StatusTypeDef status = HAL_OK; 01338 01339 /* check for PLLSAI2 Parameters used to output PLLSAI2CLK */ 01340 assert_param(IS_RCC_PLLSAI2N_VALUE(PLLSAI2Init->PLLSAI2N)); 01341 assert_param(IS_RCC_PLLSAI2P_VALUE(PLLSAI2Init->PLLSAI2P)); 01342 assert_param(IS_RCC_PLLSAI2R_VALUE(PLLSAI2Init->PLLSAI2R)); 01343 assert_param(IS_RCC_PLLSAI2CLOCKOUT_VALUE(PLLSAI2Init->PLLSAI2ClockOut)); 01344 01345 /* Disable the PLLSAI2 */ 01346 __HAL_RCC_PLLSAI2_DISABLE(); 01347 01348 /* Get Start Tick*/ 01349 tickstart = HAL_GetTick(); 01350 01351 /* Wait till PLLSAI2 is ready to be updated */ 01352 while(__HAL_RCC_GET_FLAG(RCC_FLAG_PLLSAI2RDY) != RESET) 01353 { 01354 if((HAL_GetTick() - tickstart) > PLLSAI2_TIMEOUT_VALUE) 01355 { 01356 status = HAL_TIMEOUT; 01357 break; 01358 } 01359 } 01360 01361 if(status == HAL_OK) 01362 { 01363 /* Configure the PLLSAI2 Multiplication factor N */ 01364 /* Configure the PLLSAI2 Division factors P and R */ 01365 __HAL_RCC_PLLSAI2_CONFIG(PLLSAI2Init->PLLSAI2N, PLLSAI2Init->PLLSAI2P, PLLSAI2Init->PLLSAI2R); 01366 /* Configure the PLLSAI2 Clock output(s) */ 01367 __HAL_RCC_PLLSAI2CLKOUT_ENABLE(PLLSAI2Init->PLLSAI2ClockOut); 01368 01369 /* Enable the PLLSAI2 again by setting PLLSAI2ON to 1*/ 01370 __HAL_RCC_PLLSAI2_ENABLE(); 01371 01372 /* Get Start Tick*/ 01373 tickstart = HAL_GetTick(); 01374 01375 /* Wait till PLLSAI2 is ready */ 01376 while(__HAL_RCC_GET_FLAG(RCC_FLAG_PLLSAI2RDY) == RESET) 01377 { 01378 if((HAL_GetTick() - tickstart) > PLLSAI2_TIMEOUT_VALUE) 01379 { 01380 status = HAL_TIMEOUT; 01381 break; 01382 } 01383 } 01384 } 01385 01386 return status; 01387 } 01388 01389 /** 01390 * @brief Disable PLLISAI2. 01391 * @retval HAL status 01392 */ 01393 HAL_StatusTypeDef HAL_RCCEx_DisablePLLSAI2(void) 01394 { 01395 uint32_t tickstart = 0; 01396 HAL_StatusTypeDef status = HAL_OK; 01397 01398 /* Disable the PLLSAI2 */ 01399 __HAL_RCC_PLLSAI2_DISABLE(); 01400 01401 /* Get Start Tick*/ 01402 tickstart = HAL_GetTick(); 01403 01404 /* Wait till PLLSAI2 is ready */ 01405 while(__HAL_RCC_GET_FLAG(RCC_FLAG_PLLSAI2RDY) != RESET) 01406 { 01407 if((HAL_GetTick() - tickstart) > PLLSAI2_TIMEOUT_VALUE) 01408 { 01409 status = HAL_TIMEOUT; 01410 break; 01411 } 01412 } 01413 01414 /* Disable the PLLSAI2 Clock outputs */ 01415 __HAL_RCC_PLLSAI2CLKOUT_DISABLE(RCC_PLLSAI2_SAI2CLK|RCC_PLLSAI2_ADC2CLK); 01416 01417 return status; 01418 } 01419 01420 /** 01421 * @brief Configure the oscillator clock source for wakeup from Stop and CSS backup clock. 01422 * @param WakeUpClk Wakeup clock 01423 * This parameter can be one of the following values: 01424 * @arg @ref RCC_STOP_WAKEUPCLOCK_MSI MSI oscillator selection 01425 * @arg @ref RCC_STOP_WAKEUPCLOCK_HSI HSI oscillator selection 01426 * @note This function shall not be called after the Clock Security System on HSE has been 01427 * enabled. 01428 * @retval None 01429 */ 01430 void HAL_RCCEx_WakeUpStopCLKConfig(uint32_t WakeUpClk) 01431 { 01432 assert_param(IS_RCC_STOP_WAKEUPCLOCK(WakeUpClk)); 01433 01434 __HAL_RCC_WAKEUPSTOP_CLK_CONFIG(WakeUpClk); 01435 } 01436 01437 /** 01438 * @brief Configure the MSI range after standby mode. 01439 * @note After Standby its frequency can be selected between 4 possible values (1, 2, 4 or 8 MHz). 01440 * @param MSIRange MSI range 01441 * This parameter can be one of the following values: 01442 * @arg @ref RCC_MSIRANGE_4 Range 4 around 1 MHz 01443 * @arg @ref RCC_MSIRANGE_5 Range 5 around 2 MHz 01444 * @arg @ref RCC_MSIRANGE_6 Range 6 around 4 MHz (reset value) 01445 * @arg @ref RCC_MSIRANGE_7 Range 7 around 8 MHz 01446 * @retval None 01447 */ 01448 void HAL_RCCEx_StandbyMSIRangeConfig(uint32_t MSIRange) 01449 { 01450 assert_param(IS_RCC_MSI_STANDBY_CLOCK_RANGE(MSIRange)); 01451 01452 __HAL_RCC_MSI_STANDBY_RANGE_CONFIG(MSIRange); 01453 } 01454 01455 /** 01456 * @brief Enable the LSE Clock Security System. 01457 * @note Prior to enable the LSE Clock Security System, LSE oscillator is to be enabled 01458 * with HAL_RCC_OscConfig() and the LSE oscillator clock is to be selected as RTC 01459 * clock with HAL_RCCEx_PeriphCLKConfig(). 01460 * @retval None 01461 */ 01462 void HAL_RCCEx_EnableLSECSS(void) 01463 { 01464 SET_BIT(RCC->BDCR, RCC_BDCR_LSECSSON) ; 01465 } 01466 01467 /** 01468 * @brief Disable the LSE Clock Security System. 01469 * @note LSE Clock Security System can only be disabled after a LSE failure detection. 01470 * @retval None 01471 */ 01472 void HAL_RCCEx_DisableLSECSS(void) 01473 { 01474 CLEAR_BIT(RCC->BDCR, RCC_BDCR_LSECSSON) ; 01475 01476 /* Disable LSE CSS IT if any */ 01477 __HAL_RCC_DISABLE_IT(RCC_IT_LSECSS); 01478 } 01479 01480 /** 01481 * @brief Enable the LSE Clock Security System Interrupt & corresponding EXTI line. 01482 * @note LSE Clock Security System Interrupt is mapped on RTC EXTI line 19 01483 * @retval None 01484 */ 01485 void HAL_RCCEx_EnableLSECSS_IT(void) 01486 { 01487 /* Enable LSE CSS */ 01488 SET_BIT(RCC->BDCR, RCC_BDCR_LSECSSON) ; 01489 01490 /* Enable LSE CSS IT */ 01491 __HAL_RCC_ENABLE_IT(RCC_IT_LSECSS); 01492 01493 /* Enable IT on EXTI Line 19 */ 01494 __HAL_RCC_LSECSS_EXTI_ENABLE_IT(); 01495 __HAL_RCC_LSECSS_EXTI_ENABLE_RISING_EDGE(); 01496 } 01497 01498 /** 01499 * @brief Handle the RCC LSE Clock Security System interrupt request. 01500 * @retval None 01501 */ 01502 void HAL_RCCEx_LSECSS_IRQHandler(void) 01503 { 01504 /* Check RCC LSE CSSF flag */ 01505 if(__HAL_RCC_GET_IT(RCC_IT_LSECSS)) 01506 { 01507 /* RCC LSE Clock Security System interrupt user callback */ 01508 HAL_RCCEx_LSECSS_Callback(); 01509 01510 /* Clear RCC LSE CSS pending bit */ 01511 __HAL_RCC_CLEAR_IT(RCC_IT_LSECSS); 01512 } 01513 } 01514 01515 /** 01516 * @brief RCCEx LSE Clock Security System interrupt callback. 01517 * @retval none 01518 */ 01519 __weak void HAL_RCCEx_LSECSS_Callback(void) 01520 { 01521 /* NOTE : This function should not be modified, when the callback is needed, 01522 the @ref HAL_RCCEx_LSECSS_Callback should be implemented in the user file 01523 */ 01524 } 01525 01526 /** 01527 * @brief Select the Low Speed clock source to output on LSCO pin (PA2). 01528 * @param LSCOSource specifies the Low Speed clock source to output. 01529 * This parameter can be one of the following values: 01530 * @arg @ref RCC_LSCOSOURCE_LSI LSI clock selected as LSCO source 01531 * @arg @ref RCC_LSCOSOURCE_LSE LSE clock selected as LSCO source 01532 * @retval None 01533 */ 01534 void HAL_RCCEx_EnableLSCO(uint32_t LSCOSource) 01535 { 01536 GPIO_InitTypeDef GPIO_InitStruct; 01537 FlagStatus pwrclkchanged = RESET; 01538 FlagStatus backupchanged = RESET; 01539 01540 /* Check the parameters */ 01541 assert_param(IS_RCC_LSCOSOURCE(LSCOSource)); 01542 01543 /* LSCO Pin Clock Enable */ 01544 __LSCO_CLK_ENABLE(); 01545 01546 /* Configue the LSCO pin in analog mode */ 01547 GPIO_InitStruct.Pin = LSCO_PIN; 01548 GPIO_InitStruct.Mode = GPIO_MODE_ANALOG; 01549 GPIO_InitStruct.Speed = GPIO_SPEED_FREQ_HIGH; 01550 GPIO_InitStruct.Pull = GPIO_NOPULL; 01551 HAL_GPIO_Init(LSCO_GPIO_PORT, &GPIO_InitStruct); 01552 01553 /* Update LSCOSEL clock source in Backup Domain control register */ 01554 if(__HAL_RCC_PWR_IS_CLK_DISABLED()) 01555 { 01556 __HAL_RCC_PWR_CLK_ENABLE(); 01557 pwrclkchanged = SET; 01558 } 01559 if(HAL_IS_BIT_CLR(PWR->CR1, PWR_CR1_DBP)) 01560 { 01561 HAL_PWR_EnableBkUpAccess(); 01562 backupchanged = SET; 01563 } 01564 01565 MODIFY_REG(RCC->BDCR, RCC_BDCR_LSCOSEL | RCC_BDCR_LSCOEN, LSCOSource | RCC_BDCR_LSCOEN); 01566 01567 if(backupchanged == SET) 01568 { 01569 HAL_PWR_DisableBkUpAccess(); 01570 } 01571 if(pwrclkchanged == SET) 01572 { 01573 __HAL_RCC_PWR_CLK_DISABLE(); 01574 } 01575 } 01576 01577 /** 01578 * @brief Disable the Low Speed clock output. 01579 * @retval None 01580 */ 01581 void HAL_RCCEx_DisableLSCO(void) 01582 { 01583 FlagStatus pwrclkchanged = RESET; 01584 FlagStatus backupchanged = RESET; 01585 01586 /* Update LSCOEN bit in Backup Domain control register */ 01587 if(__HAL_RCC_PWR_IS_CLK_DISABLED()) 01588 { 01589 __HAL_RCC_PWR_CLK_ENABLE(); 01590 pwrclkchanged = SET; 01591 } 01592 if(HAL_IS_BIT_CLR(PWR->CR1, PWR_CR1_DBP)) 01593 { 01594 /* Enable access to the backup domain */ 01595 HAL_PWR_EnableBkUpAccess(); 01596 backupchanged = SET; 01597 } 01598 01599 CLEAR_BIT(RCC->BDCR, RCC_BDCR_LSCOEN); 01600 01601 /* Restore previous configuration */ 01602 if(backupchanged == SET) 01603 { 01604 /* Disable access to the backup domain */ 01605 HAL_PWR_DisableBkUpAccess(); 01606 } 01607 if(pwrclkchanged == SET) 01608 { 01609 __HAL_RCC_PWR_CLK_DISABLE(); 01610 } 01611 } 01612 01613 /** 01614 * @brief Enable the PLL-mode of the MSI. 01615 * @note Prior to enable the PLL-mode of the MSI for automatic hardware 01616 * calibration LSE oscillator is to be enabled with HAL_RCC_OscConfig(). 01617 * @retval None 01618 */ 01619 void HAL_RCCEx_EnableMSIPLLMode(void) 01620 { 01621 SET_BIT(RCC->CR, RCC_CR_MSIPLLEN) ; 01622 } 01623 01624 /** 01625 * @brief Disable the PLL-mode of the MSI. 01626 * @note PLL-mode of the MSI is automatically reset when LSE oscillator is disabled. 01627 * @retval None 01628 */ 01629 void HAL_RCCEx_DisableMSIPLLMode(void) 01630 { 01631 CLEAR_BIT(RCC->CR, RCC_CR_MSIPLLEN) ; 01632 } 01633 01634 /** 01635 * @} 01636 */ 01637 01638 /** 01639 * @} 01640 */ 01641 01642 /** @addtogroup RCCEx_Private_Functions 01643 * @{ 01644 */ 01645 01646 /** 01647 * @brief Configure the parameters N & P of PLLSAI1 and enable PLLSAI1 output clock(s). 01648 * @param PllSai1 pointer to an RCC_PLLSAI1InitTypeDef structure that 01649 * contains the configuration parameters N & P as well as PLLSAI1 output clock(s) 01650 * 01651 * @note PLLSAI1 is temporary disable to apply new parameters 01652 * 01653 * @retval HAL status 01654 */ 01655 static HAL_StatusTypeDef RCCEx_PLLSAI1_ConfigNP(RCC_PLLSAI1InitTypeDef *PllSai1) 01656 { 01657 uint32_t tickstart = 0; 01658 HAL_StatusTypeDef status = HAL_OK; 01659 01660 /* check for PLLSAI1 Parameters used to output PLLSAI1CLK */ 01661 assert_param(IS_RCC_PLLSAI1N_VALUE(PllSai1->PLLSAI1N)); 01662 assert_param(IS_RCC_PLLSAI1P_VALUE(PllSai1->PLLSAI1P)); 01663 assert_param(IS_RCC_PLLSAI1CLOCKOUT_VALUE(PllSai1->PLLSAI1ClockOut)); 01664 01665 /* Disable the PLLSAI1 */ 01666 __HAL_RCC_PLLSAI1_DISABLE(); 01667 01668 /* Get Start Tick*/ 01669 tickstart = HAL_GetTick(); 01670 01671 /* Wait till PLLSAI1 is ready to be updated */ 01672 while(__HAL_RCC_GET_FLAG(RCC_FLAG_PLLSAI1RDY) != RESET) 01673 { 01674 if((HAL_GetTick() - tickstart) > PLLSAI1_TIMEOUT_VALUE) 01675 { 01676 status = HAL_TIMEOUT; 01677 break; 01678 } 01679 } 01680 01681 if(status == HAL_OK) 01682 { 01683 /* Configure the PLLSAI1 Multiplication factor N */ 01684 __HAL_RCC_PLLSAI1_MULN_CONFIG(PllSai1->PLLSAI1N); 01685 /* Configure the PLLSAI1 Division factor P */ 01686 __HAL_RCC_PLLSAI1_DIVP_CONFIG(PllSai1->PLLSAI1P); 01687 01688 /* Enable the PLLSAI1 again by setting PLLSAI1ON to 1*/ 01689 __HAL_RCC_PLLSAI1_ENABLE(); 01690 01691 /* Get Start Tick*/ 01692 tickstart = HAL_GetTick(); 01693 01694 /* Wait till PLLSAI1 is ready */ 01695 while(__HAL_RCC_GET_FLAG(RCC_FLAG_PLLSAI1RDY) == RESET) 01696 { 01697 if((HAL_GetTick() - tickstart) > PLLSAI1_TIMEOUT_VALUE) 01698 { 01699 status = HAL_TIMEOUT; 01700 break; 01701 } 01702 } 01703 01704 if(status == HAL_OK) 01705 { 01706 /* Configure the PLLSAI1 Clock output(s) */ 01707 __HAL_RCC_PLLSAI1CLKOUT_ENABLE(PllSai1->PLLSAI1ClockOut); 01708 } 01709 } 01710 01711 return status; 01712 } 01713 01714 /** 01715 * @brief Configure the parameters N & Q of PLLSAI1 and enable PLLSAI1 output clock(s). 01716 * @param PllSai1 pointer to an RCC_PLLSAI1InitTypeDef structure that 01717 * contains the configuration parameters N & Q as well as PLLSAI1 output clock(s) 01718 * 01719 * @note PLLSAI1 is temporary disable to apply new parameters 01720 * 01721 * @retval HAL status 01722 */ 01723 static HAL_StatusTypeDef RCCEx_PLLSAI1_ConfigNQ(RCC_PLLSAI1InitTypeDef *PllSai1) 01724 { 01725 uint32_t tickstart = 0; 01726 HAL_StatusTypeDef status = HAL_OK; 01727 01728 /* check for PLLSAI1 Parameters used to output PLLSAI1CLK */ 01729 assert_param(IS_RCC_PLLSAI1N_VALUE(PllSai1->PLLSAI1N)); 01730 assert_param(IS_RCC_PLLSAI1Q_VALUE(PllSai1->PLLSAI1Q)); 01731 assert_param(IS_RCC_PLLSAI1CLOCKOUT_VALUE(PllSai1->PLLSAI1ClockOut)); 01732 01733 /* Disable the PLLSAI1 */ 01734 __HAL_RCC_PLLSAI1_DISABLE(); 01735 01736 /* Get Start Tick*/ 01737 tickstart = HAL_GetTick(); 01738 01739 /* Wait till PLLSAI1 is ready to be updated */ 01740 while(__HAL_RCC_GET_FLAG(RCC_FLAG_PLLSAI1RDY) != RESET) 01741 { 01742 if((HAL_GetTick() - tickstart) > PLLSAI1_TIMEOUT_VALUE) 01743 { 01744 status = HAL_TIMEOUT; 01745 break; 01746 } 01747 } 01748 01749 if(status == HAL_OK) 01750 { 01751 /* Configure the PLLSAI1 Multiplication factor N */ 01752 __HAL_RCC_PLLSAI1_MULN_CONFIG(PllSai1->PLLSAI1N); 01753 /* Configure the PLLSAI1 Division factor Q */ 01754 __HAL_RCC_PLLSAI1_DIVQ_CONFIG(PllSai1->PLLSAI1Q); 01755 01756 /* Enable the PLLSAI1 again by setting PLLSAI1ON to 1*/ 01757 __HAL_RCC_PLLSAI1_ENABLE(); 01758 01759 /* Get Start Tick*/ 01760 tickstart = HAL_GetTick(); 01761 01762 /* Wait till PLLSAI1 is ready */ 01763 while(__HAL_RCC_GET_FLAG(RCC_FLAG_PLLSAI1RDY) == RESET) 01764 { 01765 if((HAL_GetTick() - tickstart) > PLLSAI1_TIMEOUT_VALUE) 01766 { 01767 status = HAL_TIMEOUT; 01768 break; 01769 } 01770 } 01771 01772 if(status == HAL_OK) 01773 { 01774 /* Configure the PLLSAI1 Clock output(s) */ 01775 __HAL_RCC_PLLSAI1CLKOUT_ENABLE(PllSai1->PLLSAI1ClockOut); 01776 } 01777 } 01778 01779 return status; 01780 } 01781 01782 /** 01783 * @brief Configure the parameters N & R of PLLSAI1 and enable PLLSAI1 output clock(s). 01784 * @param PllSai1 pointer to an RCC_PLLSAI1InitTypeDef structure that 01785 * contains the configuration parameters N & R as well as PLLSAI1 output clock(s) 01786 * 01787 * @note PLLSAI1 is temporary disable to apply new parameters 01788 * 01789 * @retval HAL status 01790 */ 01791 static HAL_StatusTypeDef RCCEx_PLLSAI1_ConfigNR(RCC_PLLSAI1InitTypeDef *PllSai1) 01792 { 01793 uint32_t tickstart = 0; 01794 HAL_StatusTypeDef status = HAL_OK; 01795 01796 /* check for PLLSAI1 Parameters used to output PLLSAI1CLK */ 01797 assert_param(IS_RCC_PLLSAI1N_VALUE(PllSai1->PLLSAI1N)); 01798 assert_param(IS_RCC_PLLSAI1R_VALUE(PllSai1->PLLSAI1R)); 01799 assert_param(IS_RCC_PLLSAI1CLOCKOUT_VALUE(PllSai1->PLLSAI1ClockOut)); 01800 01801 /* Disable the PLLSAI1 */ 01802 __HAL_RCC_PLLSAI1_DISABLE(); 01803 01804 /* Get Start Tick*/ 01805 tickstart = HAL_GetTick(); 01806 01807 /* Wait till PLLSAI1 is ready to be updated */ 01808 while(__HAL_RCC_GET_FLAG(RCC_FLAG_PLLSAI1RDY) != RESET) 01809 { 01810 if((HAL_GetTick() - tickstart) > PLLSAI1_TIMEOUT_VALUE) 01811 { 01812 status = HAL_TIMEOUT; 01813 break; 01814 } 01815 } 01816 01817 if(status == HAL_OK) 01818 { 01819 /* Configure the PLLSAI1 Multiplication factor N */ 01820 __HAL_RCC_PLLSAI1_MULN_CONFIG(PllSai1->PLLSAI1N); 01821 /* Configure the PLLSAI1 Division factor R */ 01822 __HAL_RCC_PLLSAI1_DIVR_CONFIG(PllSai1->PLLSAI1R); 01823 01824 /* Enable the PLLSAI1 again by setting PLLSAI1ON to 1*/ 01825 __HAL_RCC_PLLSAI1_ENABLE(); 01826 01827 /* Get Start Tick*/ 01828 tickstart = HAL_GetTick(); 01829 01830 /* Wait till PLLSAI1 is ready */ 01831 while(__HAL_RCC_GET_FLAG(RCC_FLAG_PLLSAI1RDY) == RESET) 01832 { 01833 if((HAL_GetTick() - tickstart) > PLLSAI1_TIMEOUT_VALUE) 01834 { 01835 status = HAL_TIMEOUT; 01836 break; 01837 } 01838 } 01839 01840 if(status == HAL_OK) 01841 { 01842 /* Configure the PLLSAI1 Clock output(s) */ 01843 __HAL_RCC_PLLSAI1CLKOUT_ENABLE(PllSai1->PLLSAI1ClockOut); 01844 } 01845 } 01846 01847 return status; 01848 } 01849 01850 /** 01851 * @brief Configure the parameters N & P of PLLSAI2 and enable PLLSAI2 output clock(s). 01852 * @param PllSai2 pointer to an RCC_PLLSAI2InitTypeDef structure that 01853 * contains the configuration parameters N & P as well as PLLSAI2 output clock(s) 01854 * 01855 * @note PLLSAI2 is temporary disable to apply new parameters 01856 * 01857 * @retval HAL status 01858 */ 01859 static HAL_StatusTypeDef RCCEx_PLLSAI2_ConfigNP(RCC_PLLSAI2InitTypeDef *PllSai2) 01860 { 01861 uint32_t tickstart = 0; 01862 HAL_StatusTypeDef status = HAL_OK; 01863 01864 /* check for PLLSAI2 Parameters */ 01865 assert_param(IS_RCC_PLLSAI2N_VALUE(PllSai2->PLLSAI2N)); 01866 assert_param(IS_RCC_PLLSAI2P_VALUE(PllSai2->PLLSAI2P)); 01867 assert_param(IS_RCC_PLLSAI2CLOCKOUT_VALUE(PllSai2->PLLSAI2ClockOut)); 01868 01869 /* Disable the PLLSAI2 */ 01870 __HAL_RCC_PLLSAI2_DISABLE(); 01871 01872 /* Get Start Tick*/ 01873 tickstart = HAL_GetTick(); 01874 01875 /* Wait till PLLSAI2 is ready */ 01876 while(__HAL_RCC_GET_FLAG(RCC_FLAG_PLLSAI2RDY) != RESET) 01877 { 01878 if((HAL_GetTick() - tickstart) > PLLSAI2_TIMEOUT_VALUE) 01879 { 01880 status = HAL_TIMEOUT; 01881 break; 01882 } 01883 } 01884 01885 if(status == HAL_OK) 01886 { 01887 /* Configure the PLLSAI2 Multiplication factor N */ 01888 __HAL_RCC_PLLSAI2_MULN_CONFIG(PllSai2->PLLSAI2N); 01889 /* Configure the PLLSAI2 Division factor P */ 01890 __HAL_RCC_PLLSAI2_DIVP_CONFIG(PllSai2->PLLSAI2P); 01891 01892 /* Enable the PLLSAI2 again by setting PLLSAI2ON to 1*/ 01893 __HAL_RCC_PLLSAI2_ENABLE(); 01894 01895 /* Get Start Tick*/ 01896 tickstart = HAL_GetTick(); 01897 01898 /* Wait till PLLSAI2 is ready */ 01899 while(__HAL_RCC_GET_FLAG(RCC_FLAG_PLLSAI2RDY) == RESET) 01900 { 01901 if((HAL_GetTick() - tickstart) > PLLSAI2_TIMEOUT_VALUE) 01902 { 01903 status = HAL_TIMEOUT; 01904 break; 01905 } 01906 } 01907 01908 if(status == HAL_OK) 01909 { 01910 /* Configure the PLLSAI2 Clock output(s) */ 01911 __HAL_RCC_PLLSAI2CLKOUT_ENABLE(PllSai2->PLLSAI2ClockOut); 01912 } 01913 } 01914 01915 return status; 01916 } 01917 01918 /** 01919 * @brief Configure the parameters N & R of PLLSAI2 and enable PLLSAI2 output clock(s). 01920 * @param PllSai2 pointer to an RCC_PLLSAI2InitTypeDef structure that 01921 * contains the configuration parameters N & R as well as PLLSAI2 output clock(s) 01922 * 01923 * @note PLLSAI2 is temporary disable to apply new parameters 01924 * 01925 * @retval HAL status 01926 */ 01927 static HAL_StatusTypeDef RCCEx_PLLSAI2_ConfigNR(RCC_PLLSAI2InitTypeDef *PllSai2) 01928 { 01929 uint32_t tickstart = 0; 01930 HAL_StatusTypeDef status = HAL_OK; 01931 01932 /* check for PLLSAI2 Parameters */ 01933 assert_param(IS_RCC_PLLSAI2N_VALUE(PllSai2->PLLSAI2N)); 01934 assert_param(IS_RCC_PLLSAI2R_VALUE(PllSai2->PLLSAI2R)); 01935 assert_param(IS_RCC_PLLSAI2CLOCKOUT_VALUE(PllSai2->PLLSAI2ClockOut)); 01936 01937 /* Disable the PLLSAI2 */ 01938 __HAL_RCC_PLLSAI2_DISABLE(); 01939 01940 /* Get Start Tick*/ 01941 tickstart = HAL_GetTick(); 01942 01943 /* Wait till PLLSAI2 is ready */ 01944 while(__HAL_RCC_GET_FLAG(RCC_FLAG_PLLSAI2RDY) != RESET) 01945 { 01946 if((HAL_GetTick() - tickstart) > PLLSAI2_TIMEOUT_VALUE) 01947 { 01948 status = HAL_TIMEOUT; 01949 break; 01950 } 01951 } 01952 01953 if(status == HAL_OK) 01954 { 01955 /* Configure the PLLSAI2 Multiplication factor N */ 01956 __HAL_RCC_PLLSAI2_MULN_CONFIG(PllSai2->PLLSAI2N); 01957 /* Configure the PLLSAI2 Division factor R */ 01958 __HAL_RCC_PLLSAI2_DIVR_CONFIG(PllSai2->PLLSAI2R); 01959 01960 /* Enable the PLLSAI2 again by setting PLLSAI2ON to 1*/ 01961 __HAL_RCC_PLLSAI2_ENABLE(); 01962 01963 /* Get Start Tick*/ 01964 tickstart = HAL_GetTick(); 01965 01966 /* Wait till PLLSAI2 is ready */ 01967 while(__HAL_RCC_GET_FLAG(RCC_FLAG_PLLSAI2RDY) == RESET) 01968 { 01969 if((HAL_GetTick() - tickstart) > PLLSAI2_TIMEOUT_VALUE) 01970 { 01971 status = HAL_TIMEOUT; 01972 break; 01973 } 01974 } 01975 01976 if(status == HAL_OK) 01977 { 01978 /* Configure the PLLSAI2 Clock output(s) */ 01979 __HAL_RCC_PLLSAI2CLKOUT_ENABLE(PllSai2->PLLSAI2ClockOut); 01980 } 01981 } 01982 01983 return status; 01984 } 01985 01986 /** 01987 * @} 01988 */ 01989 01990 /** 01991 * @} 01992 */ 01993 01994 #endif /* HAL_RCC_MODULE_ENABLED */ 01995 /** 01996 * @} 01997 */ 01998 01999 /** 02000 * @} 02001 */ 02002 02003 /************************ (C) COPYRIGHT STMicroelectronics *****END OF FILE****/ 02004 02005
Generated on Tue Jul 12 2022 11:35:15 by
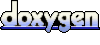