Hal Drivers for L4
Dependents: BSP OneHopeOnePrayer FINAL_AUDIO_RECORD AudioDemo
Fork of STM32L4xx_HAL_Driver by
stm32l4xx_hal_rcc.h
00001 /** 00002 ****************************************************************************** 00003 * @file stm32l4xx_hal_rcc.h 00004 * @author MCD Application Team 00005 * @version V1.1.0 00006 * @date 16-September-2015 00007 * @brief Header file of RCC HAL module. 00008 ****************************************************************************** 00009 * @attention 00010 * 00011 * <h2><center>© COPYRIGHT(c) 2015 STMicroelectronics</center></h2> 00012 * 00013 * Redistribution and use in source and binary forms, with or without modification, 00014 * are permitted provided that the following conditions are met: 00015 * 1. Redistributions of source code must retain the above copyright notice, 00016 * this list of conditions and the following disclaimer. 00017 * 2. Redistributions in binary form must reproduce the above copyright notice, 00018 * this list of conditions and the following disclaimer in the documentation 00019 * and/or other materials provided with the distribution. 00020 * 3. Neither the name of STMicroelectronics nor the names of its contributors 00021 * may be used to endorse or promote products derived from this software 00022 * without specific prior written permission. 00023 * 00024 * THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" 00025 * AND ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE 00026 * IMPLIED WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE 00027 * DISCLAIMED. IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE 00028 * FOR ANY DIRECT, INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL 00029 * DAMAGES (INCLUDING, BUT NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR 00030 * SERVICES; LOSS OF USE, DATA, OR PROFITS; OR BUSINESS INTERRUPTION) HOWEVER 00031 * CAUSED AND ON ANY THEORY OF LIABILITY, WHETHER IN CONTRACT, STRICT LIABILITY, 00032 * OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) ARISING IN ANY WAY OUT OF THE USE 00033 * OF THIS SOFTWARE, EVEN IF ADVISED OF THE POSSIBILITY OF SUCH DAMAGE. 00034 * 00035 ****************************************************************************** 00036 */ 00037 00038 /* Define to prevent recursive inclusion -------------------------------------*/ 00039 #ifndef __STM32L4xx_HAL_RCC_H 00040 #define __STM32L4xx_HAL_RCC_H 00041 00042 #ifdef __cplusplus 00043 extern "C" { 00044 #endif 00045 00046 /* Includes ------------------------------------------------------------------*/ 00047 #include "stm32l4xx_hal_def.h" 00048 00049 /** @addtogroup STM32L4xx_HAL_Driver 00050 * @{ 00051 */ 00052 00053 /** @addtogroup RCC 00054 * @{ 00055 */ 00056 00057 /* Exported types ------------------------------------------------------------*/ 00058 /** @defgroup RCC_Exported_Types RCC Exported Types 00059 * @{ 00060 */ 00061 00062 /** 00063 * @brief RCC PLL configuration structure definition 00064 */ 00065 typedef struct 00066 { 00067 uint32_t PLLState; /*!< The new state of the PLL. 00068 This parameter can be a value of @ref RCC_PLL_Config */ 00069 00070 uint32_t PLLSource; /*!< RCC_PLLSource: PLL entry clock source. 00071 This parameter must be a value of @ref RCC_PLL_Clock_Source */ 00072 00073 uint32_t PLLM; /*!< PLLM: Division factor for PLL VCO input clock. 00074 This parameter must be a number between Min_Data = 0 and Max_Data = 8 */ 00075 00076 uint32_t PLLN; /*!< PLLN: Multiplication factor for PLL VCO output clock. 00077 This parameter must be a number between Min_Data = 8 and Max_Data = 86 */ 00078 00079 uint32_t PLLP; /*!< PLLP: Division factor for SAI clock. 00080 This parameter must be a value of @ref RCC_PLLP_Clock_Divider */ 00081 00082 uint32_t PLLQ; /*!< PLLQ: Division factor for SDMMC1, RNG and USB clocks. 00083 This parameter must be a value of @ref RCC_PLLQ_Clock_Divider */ 00084 00085 uint32_t PLLR; /*!< PLLR: Division for the main system clock. 00086 User have to set the PLLR parameter correctly to not exceed max frequency 80MHZ. 00087 This parameter must be a value of @ref RCC_PLLR_Clock_Divider */ 00088 00089 }RCC_PLLInitTypeDef; 00090 00091 /** 00092 * @brief RCC Internal/External Oscillator (HSE, HSI, MSI, LSE and LSI) configuration structure definition 00093 */ 00094 typedef struct 00095 { 00096 uint32_t OscillatorType; /*!< The oscillators to be configured. 00097 This parameter can be a value of @ref RCC_Oscillator_Type */ 00098 00099 uint32_t HSEState; /*!< The new state of the HSE. 00100 This parameter can be a value of @ref RCC_HSE_Config */ 00101 00102 uint32_t LSEState; /*!< The new state of the LSE. 00103 This parameter can be a value of @ref RCC_LSE_Config */ 00104 00105 uint32_t HSIState; /*!< The new state of the HSI. 00106 This parameter can be a value of @ref RCC_HSI_Config */ 00107 00108 uint32_t HSICalibrationValue; /*!< The calibration trimming value (default is RCC_HSICALIBRATION_DEFAULT). 00109 This parameter must be a number between Min_Data = 0x00 and Max_Data = 0x1F */ 00110 00111 uint32_t LSIState; /*!< The new state of the LSI. 00112 This parameter can be a value of @ref RCC_LSI_Config */ 00113 00114 uint32_t MSIState; /*!< The new state of the MSI. 00115 This parameter can be a value of @ref RCC_MSI_Config */ 00116 00117 uint32_t MSICalibrationValue; /*!< The calibration trimming value (default is RCC_MSICALIBRATION_DEFAULT). 00118 This parameter must be a number between Min_Data = 0x00 and Max_Data = 0xFF */ 00119 00120 uint32_t MSIClockRange; /*!< The MSI frequency range. 00121 This parameter can be a value of @ref RCC_MSI_Clock_Range */ 00122 00123 RCC_PLLInitTypeDef PLL; /*!< Main PLL structure parameters */ 00124 00125 }RCC_OscInitTypeDef; 00126 00127 /** 00128 * @brief RCC System, AHB and APB busses clock configuration structure definition 00129 */ 00130 typedef struct 00131 { 00132 uint32_t ClockType; /*!< The clock to be configured. 00133 This parameter can be a value of @ref RCC_System_Clock_Type */ 00134 00135 uint32_t SYSCLKSource; /*!< The clock source used as system clock (SYSCLK). 00136 This parameter can be a value of @ref RCC_System_Clock_Source */ 00137 00138 uint32_t AHBCLKDivider; /*!< The AHB clock (HCLK) divider. This clock is derived from the system clock (SYSCLK). 00139 This parameter can be a value of @ref RCC_AHB_Clock_Source */ 00140 00141 uint32_t APB1CLKDivider; /*!< The APB1 clock (PCLK1) divider. This clock is derived from the AHB clock (HCLK). 00142 This parameter can be a value of @ref RCC_APB1_APB2_Clock_Source */ 00143 00144 uint32_t APB2CLKDivider; /*!< The APB2 clock (PCLK2) divider. This clock is derived from the AHB clock (HCLK). 00145 This parameter can be a value of @ref RCC_APB1_APB2_Clock_Source */ 00146 00147 }RCC_ClkInitTypeDef; 00148 00149 /** 00150 * @} 00151 */ 00152 00153 /* Exported constants --------------------------------------------------------*/ 00154 /** @defgroup RCC_Exported_Constants RCC Exported Constants 00155 * @{ 00156 */ 00157 00158 /** @defgroup RCC_Timeout_Value Timeout Values 00159 * @{ 00160 */ 00161 #define RCC_DBP_TIMEOUT_VALUE ((uint32_t)100) 00162 #define RCC_LSE_TIMEOUT_VALUE ((uint32_t)5000) 00163 /** 00164 * @} 00165 */ 00166 00167 /** @defgroup RCC_Oscillator_Type Oscillator Type 00168 * @{ 00169 */ 00170 #define RCC_OSCILLATORTYPE_NONE ((uint32_t)0x00000000) /*!< Oscillator configuration unchanged */ 00171 #define RCC_OSCILLATORTYPE_HSE ((uint32_t)0x00000001) /*!< HSE to configure */ 00172 #define RCC_OSCILLATORTYPE_HSI ((uint32_t)0x00000002) /*!< HSI to configure */ 00173 #define RCC_OSCILLATORTYPE_LSE ((uint32_t)0x00000004) /*!< LSE to configure */ 00174 #define RCC_OSCILLATORTYPE_LSI ((uint32_t)0x00000008) /*!< LSI to configure */ 00175 #define RCC_OSCILLATORTYPE_MSI ((uint32_t)0x00000010) /*!< MSI to configure */ 00176 /** 00177 * @} 00178 */ 00179 00180 /** @defgroup RCC_HSE_Config HSE Config 00181 * @{ 00182 */ 00183 #define RCC_HSE_OFF ((uint32_t)0x00000000) /*!< HSE clock deactivation */ 00184 #define RCC_HSE_ON RCC_CR_HSEON /*!< HSE clock activation */ 00185 #define RCC_HSE_BYPASS ((uint32_t)(RCC_CR_HSEBYP | RCC_CR_HSEON)) /*!< External clock source for HSE clock */ 00186 /** 00187 * @} 00188 */ 00189 00190 /** @defgroup RCC_LSE_Config LSE Config 00191 * @{ 00192 */ 00193 #define RCC_LSE_OFF ((uint32_t)0x00000000) /*!< LSE clock deactivation */ 00194 #define RCC_LSE_ON RCC_BDCR_LSEON /*!< LSE clock activation */ 00195 #define RCC_LSE_BYPASS ((uint32_t)(RCC_BDCR_LSEBYP | RCC_BDCR_LSEON)) /*!< External clock source for LSE clock */ 00196 /** 00197 * @} 00198 */ 00199 00200 /** @defgroup RCC_HSI_Config HSI Config 00201 * @{ 00202 */ 00203 #define RCC_HSI_OFF ((uint32_t)0x00000000) /*!< HSI clock deactivation */ 00204 #define RCC_HSI_ON RCC_CR_HSION /*!< HSI clock activation */ 00205 00206 #define RCC_HSICALIBRATION_DEFAULT ((uint32_t)16) /*!< Default HSI calibration trimming value */ 00207 /** 00208 * @} 00209 */ 00210 00211 /** @defgroup RCC_LSI_Config LSI Config 00212 * @{ 00213 */ 00214 #define RCC_LSI_OFF ((uint32_t)0x00000000) /*!< LSI clock deactivation */ 00215 #define RCC_LSI_ON RCC_CSR_LSION /*!< LSI clock activation */ 00216 /** 00217 * @} 00218 */ 00219 00220 /** @defgroup RCC_MSI_Config MSI Config 00221 * @{ 00222 */ 00223 #define RCC_MSI_OFF ((uint32_t)0x00000000) /*!< MSI clock deactivation */ 00224 #define RCC_MSI_ON RCC_CR_MSION /*!< MSI clock activation */ 00225 00226 #define RCC_MSICALIBRATION_DEFAULT ((uint32_t)0) /*!< Default MSI calibration trimming value */ 00227 /** 00228 * @} 00229 */ 00230 00231 /** @defgroup RCC_PLL_Config PLL Config 00232 * @{ 00233 */ 00234 #define RCC_PLL_NONE ((uint32_t)0x00000000) /*!< PLL configuration unchanged */ 00235 #define RCC_PLL_OFF ((uint32_t)0x00000001) /*!< PLL deactivation */ 00236 #define RCC_PLL_ON ((uint32_t)0x00000002) /*!< PLL activation */ 00237 /** 00238 * @} 00239 */ 00240 00241 /** @defgroup RCC_PLLP_Clock_Divider PLLP Clock Divider 00242 * @{ 00243 */ 00244 #define RCC_PLLP_DIV7 ((uint32_t)0x00000007) /*!< PLLP division factor = 7 */ 00245 #define RCC_PLLP_DIV17 ((uint32_t)0x00000011) /*!< PLLP division factor = 17 */ 00246 /** 00247 * @} 00248 */ 00249 00250 /** @defgroup RCC_PLLQ_Clock_Divider PLLQ Clock Divider 00251 * @{ 00252 */ 00253 #define RCC_PLLQ_DIV2 ((uint32_t)0x00000002) /*!< PLLQ division factor = 2 */ 00254 #define RCC_PLLQ_DIV4 ((uint32_t)0x00000004) /*!< PLLQ division factor = 4 */ 00255 #define RCC_PLLQ_DIV6 ((uint32_t)0x00000006) /*!< PLLQ division factor = 6 */ 00256 #define RCC_PLLQ_DIV8 ((uint32_t)0x00000008) /*!< PLLQ division factor = 8 */ 00257 /** 00258 * @} 00259 */ 00260 00261 /** @defgroup RCC_PLLR_Clock_Divider PLLR Clock Divider 00262 * @{ 00263 */ 00264 #define RCC_PLLR_DIV2 ((uint32_t)0x00000002) /*!< PLLR division factor = 2 */ 00265 #define RCC_PLLR_DIV4 ((uint32_t)0x00000004) /*!< PLLR division factor = 4 */ 00266 #define RCC_PLLR_DIV6 ((uint32_t)0x00000006) /*!< PLLR division factor = 6 */ 00267 #define RCC_PLLR_DIV8 ((uint32_t)0x00000008) /*!< PLLR division factor = 8 */ 00268 /** 00269 * @} 00270 */ 00271 00272 /** @defgroup RCC_PLL_Clock_Source PLL Clock Source 00273 * @{ 00274 */ 00275 #define RCC_PLLSOURCE_NONE ((uint32_t)0x00000000) /*!< No clock selected as PLL entry clock source */ 00276 #define RCC_PLLSOURCE_MSI RCC_PLLCFGR_PLLSRC_MSI /*!< MSI clock selected as PLL entry clock source */ 00277 #define RCC_PLLSOURCE_HSI RCC_PLLCFGR_PLLSRC_HSI /*!< HSI clock selected as PLL entry clock source */ 00278 #define RCC_PLLSOURCE_HSE RCC_PLLCFGR_PLLSRC_HSE /*!< HSE clock selected as PLL entry clock source */ 00279 /** 00280 * @} 00281 */ 00282 00283 /** @defgroup RCC_PLL_Clock_Output PLL Clock Output 00284 * @{ 00285 */ 00286 #define RCC_PLL_SAI3CLK RCC_PLLCFGR_PLLPEN /*!< PLLSAI3CLK selection from main PLL */ 00287 #define RCC_PLL_48M1CLK RCC_PLLCFGR_PLLQEN /*!< PLL48M1CLK selection from main PLL */ 00288 #define RCC_PLL_SYSCLK RCC_PLLCFGR_PLLREN /*!< PLLCLK selection from main PLL */ 00289 /** 00290 * @} 00291 */ 00292 00293 /** @defgroup RCC_PLLSAI1_Clock_Output PLLSAI1 Clock Output 00294 * @{ 00295 */ 00296 #define RCC_PLLSAI1_SAI1CLK RCC_PLLSAI1CFGR_PLLSAI1PEN /*!< PLLSAI1CLK selection from PLLSAI1 */ 00297 #define RCC_PLLSAI1_48M2CLK RCC_PLLSAI1CFGR_PLLSAI1QEN /*!< PLL48M2CLK selection from PLLSAI1 */ 00298 #define RCC_PLLSAI1_ADC1CLK RCC_PLLSAI1CFGR_PLLSAI1REN /*!< PLLADC1CLK selection from PLLSAI1 */ 00299 /** 00300 * @} 00301 */ 00302 00303 /** @defgroup RCC_PLLSAI2_Clock_Output PLLSAI2 Clock Output 00304 * @{ 00305 */ 00306 #define RCC_PLLSAI2_SAI2CLK RCC_PLLSAI2CFGR_PLLSAI2PEN /*!< PLLSAI2CLK selection from PLLSAI2 */ 00307 #define RCC_PLLSAI2_ADC2CLK RCC_PLLSAI2CFGR_PLLSAI2REN /*!< PLLADC2CLK selection from PLLSAI2 */ 00308 /** 00309 * @} 00310 */ 00311 00312 /** @defgroup RCC_MSI_Clock_Range MSI Clock Range 00313 * @{ 00314 */ 00315 #define RCC_MSIRANGE_0 RCC_CR_MSIRANGE_0 /*!< MSI = 100 KHz */ 00316 #define RCC_MSIRANGE_1 RCC_CR_MSIRANGE_1 /*!< MSI = 200 KHz */ 00317 #define RCC_MSIRANGE_2 RCC_CR_MSIRANGE_2 /*!< MSI = 400 KHz */ 00318 #define RCC_MSIRANGE_3 RCC_CR_MSIRANGE_3 /*!< MSI = 800 KHz */ 00319 #define RCC_MSIRANGE_4 RCC_CR_MSIRANGE_4 /*!< MSI = 1 MHz */ 00320 #define RCC_MSIRANGE_5 RCC_CR_MSIRANGE_5 /*!< MSI = 2 MHz */ 00321 #define RCC_MSIRANGE_6 RCC_CR_MSIRANGE_6 /*!< MSI = 4 MHz */ 00322 #define RCC_MSIRANGE_7 RCC_CR_MSIRANGE_7 /*!< MSI = 8 MHz */ 00323 #define RCC_MSIRANGE_8 RCC_CR_MSIRANGE_8 /*!< MSI = 16 MHz */ 00324 #define RCC_MSIRANGE_9 RCC_CR_MSIRANGE_9 /*!< MSI = 24 MHz */ 00325 #define RCC_MSIRANGE_10 RCC_CR_MSIRANGE_10 /*!< MSI = 32 MHz */ 00326 #define RCC_MSIRANGE_11 RCC_CR_MSIRANGE_11 /*!< MSI = 48 MHz */ 00327 /** 00328 * @} 00329 */ 00330 00331 /** @defgroup RCC_System_Clock_Type System Clock Type 00332 * @{ 00333 */ 00334 #define RCC_CLOCKTYPE_SYSCLK ((uint32_t)0x00000001) /*!< SYSCLK to configure */ 00335 #define RCC_CLOCKTYPE_HCLK ((uint32_t)0x00000002) /*!< HCLK to configure */ 00336 #define RCC_CLOCKTYPE_PCLK1 ((uint32_t)0x00000004) /*!< PCLK1 to configure */ 00337 #define RCC_CLOCKTYPE_PCLK2 ((uint32_t)0x00000008) /*!< PCLK2 to configure */ 00338 /** 00339 * @} 00340 */ 00341 00342 /** @defgroup RCC_System_Clock_Source System Clock Source 00343 * @{ 00344 */ 00345 #define RCC_SYSCLKSOURCE_MSI RCC_CFGR_SW_MSI /*!< MSI selection as system clock */ 00346 #define RCC_SYSCLKSOURCE_HSI RCC_CFGR_SW_HSI /*!< HSI selection as system clock */ 00347 #define RCC_SYSCLKSOURCE_HSE RCC_CFGR_SW_HSE /*!< HSE selection as system clock */ 00348 #define RCC_SYSCLKSOURCE_PLLCLK RCC_CFGR_SW_PLL /*!< PLL selection as system clock */ 00349 /** 00350 * @} 00351 */ 00352 00353 /** @defgroup RCC_System_Clock_Source_Status System Clock Source Status 00354 * @{ 00355 */ 00356 #define RCC_SYSCLKSOURCE_STATUS_MSI RCC_CFGR_SWS_MSI /*!< MSI used as system clock */ 00357 #define RCC_SYSCLKSOURCE_STATUS_HSI RCC_CFGR_SWS_HSI /*!< HSI used as system clock */ 00358 #define RCC_SYSCLKSOURCE_STATUS_HSE RCC_CFGR_SWS_HSE /*!< HSE used as system clock */ 00359 #define RCC_SYSCLKSOURCE_STATUS_PLLCLK RCC_CFGR_SWS_PLL /*!< PLL used as system clock */ 00360 /** 00361 * @} 00362 */ 00363 00364 /** @defgroup RCC_AHB_Clock_Source AHB Clock Source 00365 * @{ 00366 */ 00367 #define RCC_SYSCLK_DIV1 RCC_CFGR_HPRE_DIV1 /*!< SYSCLK not divided */ 00368 #define RCC_SYSCLK_DIV2 RCC_CFGR_HPRE_DIV2 /*!< SYSCLK divided by 2 */ 00369 #define RCC_SYSCLK_DIV4 RCC_CFGR_HPRE_DIV4 /*!< SYSCLK divided by 4 */ 00370 #define RCC_SYSCLK_DIV8 RCC_CFGR_HPRE_DIV8 /*!< SYSCLK divided by 8 */ 00371 #define RCC_SYSCLK_DIV16 RCC_CFGR_HPRE_DIV16 /*!< SYSCLK divided by 16 */ 00372 #define RCC_SYSCLK_DIV64 RCC_CFGR_HPRE_DIV64 /*!< SYSCLK divided by 64 */ 00373 #define RCC_SYSCLK_DIV128 RCC_CFGR_HPRE_DIV128 /*!< SYSCLK divided by 128 */ 00374 #define RCC_SYSCLK_DIV256 RCC_CFGR_HPRE_DIV256 /*!< SYSCLK divided by 256 */ 00375 #define RCC_SYSCLK_DIV512 RCC_CFGR_HPRE_DIV512 /*!< SYSCLK divided by 512 */ 00376 /** 00377 * @} 00378 */ 00379 00380 /** @defgroup RCC_APB1_APB2_Clock_Source APB1 APB2 Clock Source 00381 * @{ 00382 */ 00383 #define RCC_HCLK_DIV1 RCC_CFGR_PPRE1_DIV1 /*!< HCLK not divided */ 00384 #define RCC_HCLK_DIV2 RCC_CFGR_PPRE1_DIV2 /*!< HCLK divided by 2 */ 00385 #define RCC_HCLK_DIV4 RCC_CFGR_PPRE1_DIV4 /*!< HCLK divided by 4 */ 00386 #define RCC_HCLK_DIV8 RCC_CFGR_PPRE1_DIV8 /*!< HCLK divided by 8 */ 00387 #define RCC_HCLK_DIV16 RCC_CFGR_PPRE1_DIV16 /*!< HCLK divided by 16 */ 00388 /** 00389 * @} 00390 */ 00391 00392 /** @defgroup RCC_RTC_Clock_Source RTC Clock Source 00393 * @{ 00394 */ 00395 #define RCC_RTCCLKSOURCE_LSE RCC_BDCR_RTCSEL_0 /*!< LSE oscillator clock used as RTC clock */ 00396 #define RCC_RTCCLKSOURCE_LSI RCC_BDCR_RTCSEL_1 /*!< LSI oscillator clock used as RTC clock */ 00397 #define RCC_RTCCLKSOURCE_HSE_DIV32 RCC_BDCR_RTCSEL /*!< HSE oscillator clock divided by 32 used as RTC clock */ 00398 /** 00399 * @} 00400 */ 00401 00402 /** @defgroup RCC_MCO_Index MCO Index 00403 * @{ 00404 */ 00405 #define RCC_MCO1 ((uint32_t)0x00000000) 00406 #define RCC_MCO RCC_MCO1 /*!< MCO1 to be compliant with other families with 2 MCOs*/ 00407 /** 00408 * @} 00409 */ 00410 00411 /** @defgroup RCC_MCO1_Clock_Source MCO1 Clock Source 00412 * @{ 00413 */ 00414 #define RCC_MCO1SOURCE_NOCLOCK ((uint32_t)0x00000000) /*!< MCO1 output disabled, no clock on MCO1 */ 00415 #define RCC_MCO1SOURCE_SYSCLK RCC_CFGR_MCOSEL_0 /*!< SYSCLK selection as MCO1 source */ 00416 #define RCC_MCO1SOURCE_MSI RCC_CFGR_MCOSEL_1 /*!< MSI selection as MCO1 source */ 00417 #define RCC_MCO1SOURCE_HSI (RCC_CFGR_MCOSEL_0| RCC_CFGR_MCOSEL_1) /*!< HSI selection as MCO1 source */ 00418 #define RCC_MCO1SOURCE_HSE RCC_CFGR_MCOSEL_2 /*!< HSE selection as MCO1 source */ 00419 #define RCC_MCO1SOURCE_PLLCLK (RCC_CFGR_MCOSEL_0|RCC_CFGR_MCOSEL_2) /*!< PLLCLK selection as MCO1 source */ 00420 #define RCC_MCO1SOURCE_LSI (RCC_CFGR_MCOSEL_1|RCC_CFGR_MCOSEL_2) /*!< LSI selection as MCO1 source */ 00421 #define RCC_MCO1SOURCE_LSE (RCC_CFGR_MCOSEL_0|RCC_CFGR_MCOSEL_1|RCC_CFGR_MCOSEL_2) /*!< LSE selection as MCO1 source */ 00422 /** 00423 * @} 00424 */ 00425 00426 /** @defgroup RCC_MCOx_Clock_Prescaler MCO1 Clock Prescaler 00427 * @{ 00428 */ 00429 #define RCC_MCODIV_1 RCC_CFGR_MCO_PRE_1 /*!< MCO not divided */ 00430 #define RCC_MCODIV_2 RCC_CFGR_MCO_PRE_2 /*!< MCO divided by 2 */ 00431 #define RCC_MCODIV_4 RCC_CFGR_MCO_PRE_4 /*!< MCO divided by 4 */ 00432 #define RCC_MCODIV_8 RCC_CFGR_MCO_PRE_8 /*!< MCO divided by 8 */ 00433 #define RCC_MCODIV_16 RCC_CFGR_MCO_PRE_16 /*!< MCO divided by 16 */ 00434 /** 00435 * @} 00436 */ 00437 00438 /** @defgroup RCC_Interrupt Interrupts 00439 * @{ 00440 */ 00441 #define RCC_IT_LSIRDY RCC_CIFR_LSIRDYF /*!< LSI Ready Interrupt flag */ 00442 #define RCC_IT_LSERDY RCC_CIFR_LSERDYF /*!< LSE Ready Interrupt flag */ 00443 #define RCC_IT_MSIRDY RCC_CIFR_MSIRDYF /*!< MSI Ready Interrupt flag */ 00444 #define RCC_IT_HSIRDY RCC_CIFR_HSIRDYF /*!< HSI16 Ready Interrupt flag */ 00445 #define RCC_IT_HSERDY RCC_CIFR_HSERDYF /*!< HSE Ready Interrupt flag */ 00446 #define RCC_IT_PLLRDY RCC_CIFR_PLLRDYF /*!< PLL Ready Interrupt flag */ 00447 #define RCC_IT_PLLSAI1RDY RCC_CIFR_PLLSAI1RDYF /*!< PLLSAI1 Ready Interrupt flag */ 00448 #define RCC_IT_PLLSAI2RDY RCC_CIFR_PLLSAI2RDYF /*!< PLLSAI2 Ready Interrupt flag */ 00449 #define RCC_IT_CSS RCC_CIFR_CSSF /*!< Clock Security System Interrupt flag */ 00450 #define RCC_IT_LSECSS RCC_CIFR_LSECSSF /*!< LSE Clock Security System Interrupt flag */ 00451 /** 00452 * @} 00453 */ 00454 00455 /** @defgroup RCC_Flag Flags 00456 * Elements values convention: XXXYYYYYb 00457 * - YYYYY : Flag position in the register 00458 * - XXX : Register index 00459 * - 001: CR register 00460 * - 010: BDCR register 00461 * - 011: CSR register 00462 * @{ 00463 */ 00464 /* Flags in the CR register */ 00465 #define RCC_FLAG_MSIRDY ((uint32_t)((CR_REG_INDEX << 5) | POSITION_VAL(RCC_CR_MSIRDY))) /*!< MSI Ready flag */ 00466 #define RCC_FLAG_HSIRDY ((uint32_t)((CR_REG_INDEX << 5) | POSITION_VAL(RCC_CR_HSIRDY))) /*!< HSI Ready flag */ 00467 #define RCC_FLAG_HSERDY ((uint32_t)((CR_REG_INDEX << 5) | POSITION_VAL(RCC_CR_HSERDY))) /*!< HSE Ready flag */ 00468 #define RCC_FLAG_PLLRDY ((uint32_t)((CR_REG_INDEX << 5) | POSITION_VAL(RCC_CR_PLLRDY))) /*!< PLL Ready flag */ 00469 #define RCC_FLAG_PLLSAI1RDY ((uint32_t)((CR_REG_INDEX << 5) | POSITION_VAL(RCC_CR_PLLSAI1RDY))) /*!< PLLSAI1 Ready flag */ 00470 #define RCC_FLAG_PLLSAI2RDY ((uint32_t)((CR_REG_INDEX << 5) | POSITION_VAL(RCC_CR_PLLSAI2RDY))) /*!< PLLSAI2 Ready flag */ 00471 00472 /* Flags in the BDCR register */ 00473 #define RCC_FLAG_LSERDY ((uint32_t)((BDCR_REG_INDEX << 5) | POSITION_VAL(RCC_BDCR_LSERDY))) /*!< LSE Ready flag */ 00474 #define RCC_FLAG_LSECSSD ((uint32_t)((BDCR_REG_INDEX << 5) | POSITION_VAL(RCC_BDCR_LSECSSD))) /*!< LSE Clock Security System Interrupt flag */ 00475 00476 /* Flags in the CSR register */ 00477 #define RCC_FLAG_LSIRDY ((uint32_t)((CSR_REG_INDEX << 5) | POSITION_VAL(RCC_CSR_LSIRDY))) /*!< LSI Ready flag */ 00478 #define RCC_FLAG_RMVF ((uint32_t)((CSR_REG_INDEX << 5) | POSITION_VAL(RCC_CSR_RMVF))) /*!< Remove reset flag */ 00479 #define RCC_FLAG_FWRST ((uint32_t)((CSR_REG_INDEX << 5) | POSITION_VAL(RCC_CSR_FWRSTF))) /*!< Firewall reset flag */ 00480 #define RCC_FLAG_OBLRST ((uint32_t)((CSR_REG_INDEX << 5) | POSITION_VAL(RCC_CSR_OBLRSTF))) /*!< Option Byte Loader reset flag */ 00481 #define RCC_FLAG_PINRST ((uint32_t)((CSR_REG_INDEX << 5) | POSITION_VAL(RCC_CSR_PINRSTF))) /*!< PIN reset flag */ 00482 #define RCC_FLAG_BORRST ((uint32_t)((CSR_REG_INDEX << 5) | POSITION_VAL(RCC_CSR_BORRSTF))) /*!< BOR reset flag */ 00483 #define RCC_FLAG_SFTRST ((uint32_t)((CSR_REG_INDEX << 5) | POSITION_VAL(RCC_CSR_SFTRSTF))) /*!< Software Reset flag */ 00484 #define RCC_FLAG_IWDGRST ((uint32_t)((CSR_REG_INDEX << 5) | POSITION_VAL(RCC_CSR_IWDGRSTF))) /*!< Independent Watchdog reset flag */ 00485 #define RCC_FLAG_WWDGRST ((uint32_t)((CSR_REG_INDEX << 5) | POSITION_VAL(RCC_CSR_WWDGRSTF))) /*!< Window watchdog reset flag */ 00486 #define RCC_FLAG_LPWRRST ((uint32_t)((CSR_REG_INDEX << 5) | POSITION_VAL(RCC_CSR_LPWRRSTF))) /*!< Low-Power reset flag */ 00487 00488 /** 00489 * @} 00490 */ 00491 00492 /** @defgroup RCC_LSEDrive_Config LSE Drive Config 00493 * @{ 00494 */ 00495 #define RCC_LSEDRIVE_LOW ((uint32_t)0x00000000) /*!< LSE low drive capability */ 00496 #define RCC_LSEDRIVE_MEDIUMLOW RCC_BDCR_LSEDRV_0 /*!< LSE medium low drive capability */ 00497 #define RCC_LSEDRIVE_MEDIUMHIGH RCC_BDCR_LSEDRV_1 /*!< LSE medium high drive capability */ 00498 #define RCC_LSEDRIVE_HIGH RCC_BDCR_LSEDRV /*!< LSE high drive capability */ 00499 /** 00500 * @} 00501 */ 00502 00503 /** @defgroup RCC_Stop_WakeUpClock Wake-Up from STOP Clock 00504 * @{ 00505 */ 00506 #define RCC_STOP_WAKEUPCLOCK_MSI ((uint32_t)0x00000000) /*!< MSI selection after wake-up from STOP */ 00507 #define RCC_STOP_WAKEUPCLOCK_HSI RCC_CFGR_STOPWUCK /*!< HSI selection after wake-up from STOP */ 00508 /** 00509 * @} 00510 */ 00511 00512 /** 00513 * @} 00514 */ 00515 00516 /* Exported macros -----------------------------------------------------------*/ 00517 00518 /** @defgroup RCC_Exported_Macros RCC Exported Macros 00519 * @{ 00520 */ 00521 00522 /** @defgroup RCC_AHB1_Peripheral_Clock_Enable_Disable AHB1 Peripheral Clock Enable Disable 00523 * @brief Enable or disable the AHB1 peripheral clock. 00524 * @note After reset, the peripheral clock (used for registers read/write access) 00525 * is disabled and the application software has to enable this clock before 00526 * using it. 00527 * @{ 00528 */ 00529 00530 #define __HAL_RCC_DMA1_CLK_ENABLE() do { \ 00531 __IO uint32_t tmpreg; \ 00532 SET_BIT(RCC->AHB1ENR, RCC_AHB1ENR_DMA1EN); \ 00533 /* Delay after an RCC peripheral clock enabling */ \ 00534 tmpreg = READ_BIT(RCC->AHB1ENR, RCC_AHB1ENR_DMA1EN); \ 00535 UNUSED(tmpreg); \ 00536 } while(0) 00537 00538 #define __HAL_RCC_DMA2_CLK_ENABLE() do { \ 00539 __IO uint32_t tmpreg; \ 00540 SET_BIT(RCC->AHB1ENR, RCC_AHB1ENR_DMA2EN); \ 00541 /* Delay after an RCC peripheral clock enabling */ \ 00542 tmpreg = READ_BIT(RCC->AHB1ENR, RCC_AHB1ENR_DMA2EN); \ 00543 UNUSED(tmpreg); \ 00544 } while(0) 00545 00546 #define __HAL_RCC_FLASH_CLK_ENABLE() do { \ 00547 __IO uint32_t tmpreg; \ 00548 SET_BIT(RCC->AHB1ENR, RCC_AHB1ENR_FLASHEN); \ 00549 /* Delay after an RCC peripheral clock enabling */ \ 00550 tmpreg = READ_BIT(RCC->AHB1ENR, RCC_AHB1ENR_FLASHEN); \ 00551 UNUSED(tmpreg); \ 00552 } while(0) 00553 00554 #define __HAL_RCC_CRC_CLK_ENABLE() do { \ 00555 __IO uint32_t tmpreg; \ 00556 SET_BIT(RCC->AHB1ENR, RCC_AHB1ENR_CRCEN); \ 00557 /* Delay after an RCC peripheral clock enabling */ \ 00558 tmpreg = READ_BIT(RCC->AHB1ENR, RCC_AHB1ENR_CRCEN); \ 00559 UNUSED(tmpreg); \ 00560 } while(0) 00561 00562 #define __HAL_RCC_TSC_CLK_ENABLE() do { \ 00563 __IO uint32_t tmpreg; \ 00564 SET_BIT(RCC->AHB1ENR, RCC_AHB1ENR_TSCEN); \ 00565 /* Delay after an RCC peripheral clock enabling */ \ 00566 tmpreg = READ_BIT(RCC->AHB1ENR, RCC_AHB1ENR_TSCEN); \ 00567 UNUSED(tmpreg); \ 00568 } while(0) 00569 00570 #define __HAL_RCC_DMA1_CLK_DISABLE() CLEAR_BIT(RCC->AHB1ENR, RCC_AHB1ENR_DMA1EN) 00571 00572 #define __HAL_RCC_DMA2_CLK_DISABLE() CLEAR_BIT(RCC->AHB1ENR, RCC_AHB1ENR_DMA2EN) 00573 00574 #define __HAL_RCC_FLASH_CLK_DISABLE() CLEAR_BIT(RCC->AHB1ENR, RCC_AHB1ENR_FLASHEN) 00575 00576 #define __HAL_RCC_CRC_CLK_DISABLE() CLEAR_BIT(RCC->AHB1ENR, RCC_AHB1ENR_CRCEN) 00577 00578 #define __HAL_RCC_TSC_CLK_DISABLE() CLEAR_BIT(RCC->AHB1ENR, RCC_AHB1ENR_TSCEN) 00579 00580 /** 00581 * @} 00582 */ 00583 00584 /** @defgroup RCC_AHB2_Peripheral_Clock_Enable_Disable AHB2 Peripheral Clock Enable Disable 00585 * @brief Enable or disable the AHB2 peripheral clock. 00586 * @note After reset, the peripheral clock (used for registers read/write access) 00587 * is disabled and the application software has to enable this clock before 00588 * using it. 00589 * @{ 00590 */ 00591 00592 #define __HAL_RCC_GPIOA_CLK_ENABLE() do { \ 00593 __IO uint32_t tmpreg; \ 00594 SET_BIT(RCC->AHB2ENR, RCC_AHB2ENR_GPIOAEN); \ 00595 /* Delay after an RCC peripheral clock enabling */ \ 00596 tmpreg = READ_BIT(RCC->AHB2ENR, RCC_AHB2ENR_GPIOAEN); \ 00597 UNUSED(tmpreg); \ 00598 } while(0) 00599 00600 #define __HAL_RCC_GPIOB_CLK_ENABLE() do { \ 00601 __IO uint32_t tmpreg; \ 00602 SET_BIT(RCC->AHB2ENR, RCC_AHB2ENR_GPIOBEN); \ 00603 /* Delay after an RCC peripheral clock enabling */ \ 00604 tmpreg = READ_BIT(RCC->AHB2ENR, RCC_AHB2ENR_GPIOBEN); \ 00605 UNUSED(tmpreg); \ 00606 } while(0) 00607 00608 #define __HAL_RCC_GPIOC_CLK_ENABLE() do { \ 00609 __IO uint32_t tmpreg; \ 00610 SET_BIT(RCC->AHB2ENR, RCC_AHB2ENR_GPIOCEN); \ 00611 /* Delay after an RCC peripheral clock enabling */ \ 00612 tmpreg = READ_BIT(RCC->AHB2ENR, RCC_AHB2ENR_GPIOCEN); \ 00613 UNUSED(tmpreg); \ 00614 } while(0) 00615 00616 #define __HAL_RCC_GPIOD_CLK_ENABLE() do { \ 00617 __IO uint32_t tmpreg; \ 00618 SET_BIT(RCC->AHB2ENR, RCC_AHB2ENR_GPIODEN); \ 00619 /* Delay after an RCC peripheral clock enabling */ \ 00620 tmpreg = READ_BIT(RCC->AHB2ENR, RCC_AHB2ENR_GPIODEN); \ 00621 UNUSED(tmpreg); \ 00622 } while(0) 00623 00624 #define __HAL_RCC_GPIOE_CLK_ENABLE() do { \ 00625 __IO uint32_t tmpreg; \ 00626 SET_BIT(RCC->AHB2ENR, RCC_AHB2ENR_GPIOEEN); \ 00627 /* Delay after an RCC peripheral clock enabling */ \ 00628 tmpreg = READ_BIT(RCC->AHB2ENR, RCC_AHB2ENR_GPIOEEN); \ 00629 UNUSED(tmpreg); \ 00630 } while(0) 00631 00632 #define __HAL_RCC_GPIOF_CLK_ENABLE() do { \ 00633 __IO uint32_t tmpreg; \ 00634 SET_BIT(RCC->AHB2ENR, RCC_AHB2ENR_GPIOFEN); \ 00635 /* Delay after an RCC peripheral clock enabling */ \ 00636 tmpreg = READ_BIT(RCC->AHB2ENR, RCC_AHB2ENR_GPIOFEN); \ 00637 UNUSED(tmpreg); \ 00638 } while(0) 00639 00640 #define __HAL_RCC_GPIOG_CLK_ENABLE() do { \ 00641 __IO uint32_t tmpreg; \ 00642 SET_BIT(RCC->AHB2ENR, RCC_AHB2ENR_GPIOGEN); \ 00643 /* Delay after an RCC peripheral clock enabling */ \ 00644 tmpreg = READ_BIT(RCC->AHB2ENR, RCC_AHB2ENR_GPIOGEN); \ 00645 UNUSED(tmpreg); \ 00646 } while(0) 00647 00648 #define __HAL_RCC_GPIOH_CLK_ENABLE() do { \ 00649 __IO uint32_t tmpreg; \ 00650 SET_BIT(RCC->AHB2ENR, RCC_AHB2ENR_GPIOHEN); \ 00651 /* Delay after an RCC peripheral clock enabling */ \ 00652 tmpreg = READ_BIT(RCC->AHB2ENR, RCC_AHB2ENR_GPIOHEN); \ 00653 UNUSED(tmpreg); \ 00654 } while(0) 00655 00656 #if defined(STM32L475xx) || defined(STM32L476xx) || defined(STM32L485xx) || defined(STM32L486xx) 00657 00658 #define __HAL_RCC_USB_OTG_FS_CLK_ENABLE() do { \ 00659 __IO uint32_t tmpreg; \ 00660 SET_BIT(RCC->AHB2ENR, RCC_AHB2ENR_OTGFSEN); \ 00661 /* Delay after an RCC peripheral clock enabling */ \ 00662 tmpreg = READ_BIT(RCC->AHB2ENR, RCC_AHB2ENR_OTGFSEN); \ 00663 UNUSED(tmpreg); \ 00664 } while(0) 00665 00666 #endif /* STM32L475xx || STM32L476xx || STM32L485xx || STM32L486xx */ 00667 00668 #define __HAL_RCC_ADC_CLK_ENABLE() do { \ 00669 __IO uint32_t tmpreg; \ 00670 SET_BIT(RCC->AHB2ENR, RCC_AHB2ENR_ADCEN); \ 00671 /* Delay after an RCC peripheral clock enabling */ \ 00672 tmpreg = READ_BIT(RCC->AHB2ENR, RCC_AHB2ENR_ADCEN); \ 00673 UNUSED(tmpreg); \ 00674 } while(0) 00675 00676 #if defined(STM32L485xx) || defined(STM32L486xx) 00677 00678 #define __HAL_RCC_AES_CLK_ENABLE() do { \ 00679 __IO uint32_t tmpreg; \ 00680 SET_BIT(RCC->AHB2ENR, RCC_AHB2ENR_AESEN); \ 00681 /* Delay after an RCC peripheral clock enabling */ \ 00682 tmpreg = READ_BIT(RCC->AHB2ENR, RCC_AHB2ENR_AESEN); \ 00683 UNUSED(tmpreg); \ 00684 } while(0) 00685 00686 #endif /* STM32L485xx || STM32L486xx */ 00687 00688 #define __HAL_RCC_RNG_CLK_ENABLE() do { \ 00689 __IO uint32_t tmpreg; \ 00690 SET_BIT(RCC->AHB2ENR, RCC_AHB2ENR_RNGEN); \ 00691 /* Delay after an RCC peripheral clock enabling */ \ 00692 tmpreg = READ_BIT(RCC->AHB2ENR, RCC_AHB2ENR_RNGEN); \ 00693 UNUSED(tmpreg); \ 00694 } while(0) 00695 00696 #define __HAL_RCC_GPIOA_CLK_DISABLE() CLEAR_BIT(RCC->AHB2ENR, RCC_AHB2ENR_GPIOAEN) 00697 00698 #define __HAL_RCC_GPIOB_CLK_DISABLE() CLEAR_BIT(RCC->AHB2ENR, RCC_AHB2ENR_GPIOBEN) 00699 00700 #define __HAL_RCC_GPIOC_CLK_DISABLE() CLEAR_BIT(RCC->AHB2ENR, RCC_AHB2ENR_GPIOCEN) 00701 00702 #define __HAL_RCC_GPIOD_CLK_DISABLE() CLEAR_BIT(RCC->AHB2ENR, RCC_AHB2ENR_GPIODEN) 00703 00704 #define __HAL_RCC_GPIOE_CLK_DISABLE() CLEAR_BIT(RCC->AHB2ENR, RCC_AHB2ENR_GPIOEEN) 00705 00706 #define __HAL_RCC_GPIOF_CLK_DISABLE() CLEAR_BIT(RCC->AHB2ENR, RCC_AHB2ENR_GPIOFEN) 00707 00708 #define __HAL_RCC_GPIOG_CLK_DISABLE() CLEAR_BIT(RCC->AHB2ENR, RCC_AHB2ENR_GPIOGEN) 00709 00710 #define __HAL_RCC_GPIOH_CLK_DISABLE() CLEAR_BIT(RCC->AHB2ENR, RCC_AHB2ENR_GPIOHEN) 00711 00712 #if defined(STM32L475xx) || defined(STM32L476xx) || defined(STM32L485xx) || defined(STM32L486xx) 00713 00714 #define __HAL_RCC_USB_OTG_FS_CLK_DISABLE() CLEAR_BIT(RCC->AHB2ENR, RCC_AHB2ENR_OTGFSEN); 00715 00716 #endif /* STM32L475xx || STM32L476xx || STM32L485xx || STM32L486xx */ 00717 00718 #define __HAL_RCC_ADC_CLK_DISABLE() CLEAR_BIT(RCC->AHB2ENR, RCC_AHB2ENR_ADCEN) 00719 00720 #if defined(STM32L485xx) || defined(STM32L486xx) 00721 00722 #define __HAL_RCC_AES_CLK_DISABLE() CLEAR_BIT(RCC->AHB2ENR, RCC_AHB2ENR_AESEN); 00723 00724 #endif /* STM32L485xx || STM32L486xx */ 00725 00726 #define __HAL_RCC_RNG_CLK_DISABLE() CLEAR_BIT(RCC->AHB2ENR, RCC_AHB2ENR_RNGEN) 00727 00728 /** 00729 * @} 00730 */ 00731 00732 /** @defgroup RCC_AHB3_Clock_Enable_Disable AHB3 Peripheral Clock Enable Disable 00733 * @brief Enable or disable the AHB3 peripheral clock. 00734 * @note After reset, the peripheral clock (used for registers read/write access) 00735 * is disabled and the application software has to enable this clock before 00736 * using it. 00737 * @{ 00738 */ 00739 00740 #define __HAL_RCC_FMC_CLK_ENABLE() do { \ 00741 __IO uint32_t tmpreg; \ 00742 SET_BIT(RCC->AHB3ENR, RCC_AHB3ENR_FMCEN); \ 00743 /* Delay after an RCC peripheral clock enabling */ \ 00744 tmpreg = READ_BIT(RCC->AHB3ENR, RCC_AHB3ENR_FMCEN); \ 00745 UNUSED(tmpreg); \ 00746 } while(0) 00747 00748 #define __HAL_RCC_QSPI_CLK_ENABLE() do { \ 00749 __IO uint32_t tmpreg; \ 00750 SET_BIT(RCC->AHB3ENR, RCC_AHB3ENR_QSPIEN); \ 00751 /* Delay after an RCC peripheral clock enabling */ \ 00752 tmpreg = READ_BIT(RCC->AHB3ENR, RCC_AHB3ENR_QSPIEN); \ 00753 UNUSED(tmpreg); \ 00754 } while(0) 00755 00756 #define __HAL_RCC_FMC_CLK_DISABLE() CLEAR_BIT(RCC->AHB3ENR, RCC_AHB3ENR_FMCEN) 00757 00758 #define __HAL_RCC_QSPI_CLK_DISABLE() CLEAR_BIT(RCC->AHB3ENR, RCC_AHB3ENR_QSPIEN) 00759 00760 /** 00761 * @} 00762 */ 00763 00764 /** @defgroup RCC_APB1_Clock_Enable_Disable APB1 Peripheral Clock Enable Disable 00765 * @brief Enable or disable the APB1 peripheral clock. 00766 * @note After reset, the peripheral clock (used for registers read/write access) 00767 * is disabled and the application software has to enable this clock before 00768 * using it. 00769 * @{ 00770 */ 00771 00772 #define __HAL_RCC_TIM2_CLK_ENABLE() do { \ 00773 __IO uint32_t tmpreg; \ 00774 SET_BIT(RCC->APB1ENR1, RCC_APB1ENR1_TIM2EN); \ 00775 /* Delay after an RCC peripheral clock enabling */ \ 00776 tmpreg = READ_BIT(RCC->APB1ENR1, RCC_APB1ENR1_TIM2EN); \ 00777 UNUSED(tmpreg); \ 00778 } while(0) 00779 00780 #define __HAL_RCC_TIM3_CLK_ENABLE() do { \ 00781 __IO uint32_t tmpreg; \ 00782 SET_BIT(RCC->APB1ENR1, RCC_APB1ENR1_TIM3EN); \ 00783 /* Delay after an RCC peripheral clock enabling */ \ 00784 tmpreg = READ_BIT(RCC->APB1ENR1, RCC_APB1ENR1_TIM3EN); \ 00785 UNUSED(tmpreg); \ 00786 } while(0) 00787 00788 #define __HAL_RCC_TIM4_CLK_ENABLE() do { \ 00789 __IO uint32_t tmpreg; \ 00790 SET_BIT(RCC->APB1ENR1, RCC_APB1ENR1_TIM4EN); \ 00791 /* Delay after an RCC peripheral clock enabling */ \ 00792 tmpreg = READ_BIT(RCC->APB1ENR1, RCC_APB1ENR1_TIM4EN); \ 00793 UNUSED(tmpreg); \ 00794 } while(0) 00795 00796 #define __HAL_RCC_TIM5_CLK_ENABLE() do { \ 00797 __IO uint32_t tmpreg; \ 00798 SET_BIT(RCC->APB1ENR1, RCC_APB1ENR1_TIM5EN); \ 00799 /* Delay after an RCC peripheral clock enabling */ \ 00800 tmpreg = READ_BIT(RCC->APB1ENR1, RCC_APB1ENR1_TIM5EN); \ 00801 UNUSED(tmpreg); \ 00802 } while(0) 00803 00804 #define __HAL_RCC_TIM6_CLK_ENABLE() do { \ 00805 __IO uint32_t tmpreg; \ 00806 SET_BIT(RCC->APB1ENR1, RCC_APB1ENR1_TIM6EN); \ 00807 /* Delay after an RCC peripheral clock enabling */ \ 00808 tmpreg = READ_BIT(RCC->APB1ENR1, RCC_APB1ENR1_TIM6EN); \ 00809 UNUSED(tmpreg); \ 00810 } while(0) 00811 00812 #define __HAL_RCC_TIM7_CLK_ENABLE() do { \ 00813 __IO uint32_t tmpreg; \ 00814 SET_BIT(RCC->APB1ENR1, RCC_APB1ENR1_TIM7EN); \ 00815 /* Delay after an RCC peripheral clock enabling */ \ 00816 tmpreg = READ_BIT(RCC->APB1ENR1, RCC_APB1ENR1_TIM7EN); \ 00817 UNUSED(tmpreg); \ 00818 } while(0) 00819 00820 #if defined(STM32L476xx) || defined(STM32L486xx) 00821 #define __HAL_RCC_LCD_CLK_ENABLE() do { \ 00822 __IO uint32_t tmpreg; \ 00823 SET_BIT(RCC->APB1ENR1, RCC_APB1ENR1_LCDEN); \ 00824 /* Delay after an RCC peripheral clock enabling */ \ 00825 tmpreg = READ_BIT(RCC->APB1ENR1, RCC_APB1ENR1_LCDEN); \ 00826 UNUSED(tmpreg); \ 00827 } while(0) 00828 #endif /* STM32L476xx || STM32L486xx */ 00829 00830 #define __HAL_RCC_WWDG_CLK_ENABLE() do { \ 00831 __IO uint32_t tmpreg; \ 00832 SET_BIT(RCC->APB1ENR1, RCC_APB1ENR1_WWDGEN); \ 00833 /* Delay after an RCC peripheral clock enabling */ \ 00834 tmpreg = READ_BIT(RCC->APB1ENR1, RCC_APB1ENR1_WWDGEN); \ 00835 UNUSED(tmpreg); \ 00836 } while(0) 00837 00838 #define __HAL_RCC_SPI2_CLK_ENABLE() do { \ 00839 __IO uint32_t tmpreg; \ 00840 SET_BIT(RCC->APB1ENR1, RCC_APB1ENR1_SPI2EN); \ 00841 /* Delay after an RCC peripheral clock enabling */ \ 00842 tmpreg = READ_BIT(RCC->APB1ENR1, RCC_APB1ENR1_SPI2EN); \ 00843 UNUSED(tmpreg); \ 00844 } while(0) 00845 00846 #define __HAL_RCC_SPI3_CLK_ENABLE() do { \ 00847 __IO uint32_t tmpreg; \ 00848 SET_BIT(RCC->APB1ENR1, RCC_APB1ENR1_SPI3EN); \ 00849 /* Delay after an RCC peripheral clock enabling */ \ 00850 tmpreg = READ_BIT(RCC->APB1ENR1, RCC_APB1ENR1_SPI3EN); \ 00851 UNUSED(tmpreg); \ 00852 } while(0) 00853 00854 #define __HAL_RCC_USART2_CLK_ENABLE() do { \ 00855 __IO uint32_t tmpreg; \ 00856 SET_BIT(RCC->APB1ENR1, RCC_APB1ENR1_USART2EN); \ 00857 /* Delay after an RCC peripheral clock enabling */ \ 00858 tmpreg = READ_BIT(RCC->APB1ENR1, RCC_APB1ENR1_USART2EN); \ 00859 UNUSED(tmpreg); \ 00860 } while(0) 00861 00862 #define __HAL_RCC_USART3_CLK_ENABLE() do { \ 00863 __IO uint32_t tmpreg; \ 00864 SET_BIT(RCC->APB1ENR1, RCC_APB1ENR1_USART3EN); \ 00865 /* Delay after an RCC peripheral clock enabling */ \ 00866 tmpreg = READ_BIT(RCC->APB1ENR1, RCC_APB1ENR1_USART3EN); \ 00867 UNUSED(tmpreg); \ 00868 } while(0) 00869 00870 #define __HAL_RCC_UART4_CLK_ENABLE() do { \ 00871 __IO uint32_t tmpreg; \ 00872 SET_BIT(RCC->APB1ENR1, RCC_APB1ENR1_UART4EN); \ 00873 /* Delay after an RCC peripheral clock enabling */ \ 00874 tmpreg = READ_BIT(RCC->APB1ENR1, RCC_APB1ENR1_UART4EN); \ 00875 UNUSED(tmpreg); \ 00876 } while(0) 00877 00878 #define __HAL_RCC_UART5_CLK_ENABLE() do { \ 00879 __IO uint32_t tmpreg; \ 00880 SET_BIT(RCC->APB1ENR1, RCC_APB1ENR1_UART5EN); \ 00881 /* Delay after an RCC peripheral clock enabling */ \ 00882 tmpreg = READ_BIT(RCC->APB1ENR1, RCC_APB1ENR1_UART5EN); \ 00883 UNUSED(tmpreg); \ 00884 } while(0) 00885 00886 #define __HAL_RCC_I2C1_CLK_ENABLE() do { \ 00887 __IO uint32_t tmpreg; \ 00888 SET_BIT(RCC->APB1ENR1, RCC_APB1ENR1_I2C1EN); \ 00889 /* Delay after an RCC peripheral clock enabling */ \ 00890 tmpreg = READ_BIT(RCC->APB1ENR1, RCC_APB1ENR1_I2C1EN); \ 00891 UNUSED(tmpreg); \ 00892 } while(0) 00893 00894 #define __HAL_RCC_I2C2_CLK_ENABLE() do { \ 00895 __IO uint32_t tmpreg; \ 00896 SET_BIT(RCC->APB1ENR1, RCC_APB1ENR1_I2C2EN); \ 00897 /* Delay after an RCC peripheral clock enabling */ \ 00898 tmpreg = READ_BIT(RCC->APB1ENR1, RCC_APB1ENR1_I2C2EN); \ 00899 UNUSED(tmpreg); \ 00900 } while(0) 00901 00902 #define __HAL_RCC_I2C3_CLK_ENABLE() do { \ 00903 __IO uint32_t tmpreg; \ 00904 SET_BIT(RCC->APB1ENR1, RCC_APB1ENR1_I2C3EN); \ 00905 /* Delay after an RCC peripheral clock enabling */ \ 00906 tmpreg = READ_BIT(RCC->APB1ENR1, RCC_APB1ENR1_I2C3EN); \ 00907 UNUSED(tmpreg); \ 00908 } while(0) 00909 00910 #define __HAL_RCC_CAN1_CLK_ENABLE() do { \ 00911 __IO uint32_t tmpreg; \ 00912 SET_BIT(RCC->APB1ENR1, RCC_APB1ENR1_CAN1EN); \ 00913 /* Delay after an RCC peripheral clock enabling */ \ 00914 tmpreg = READ_BIT(RCC->APB1ENR1, RCC_APB1ENR1_CAN1EN); \ 00915 UNUSED(tmpreg); \ 00916 } while(0) 00917 00918 #define __HAL_RCC_PWR_CLK_ENABLE() do { \ 00919 __IO uint32_t tmpreg; \ 00920 SET_BIT(RCC->APB1ENR1, RCC_APB1ENR1_PWREN); \ 00921 /* Delay after an RCC peripheral clock enabling */ \ 00922 tmpreg = READ_BIT(RCC->APB1ENR1, RCC_APB1ENR1_PWREN); \ 00923 UNUSED(tmpreg); \ 00924 } while(0) 00925 00926 #define __HAL_RCC_DAC1_CLK_ENABLE() do { \ 00927 __IO uint32_t tmpreg; \ 00928 SET_BIT(RCC->APB1ENR1, RCC_APB1ENR1_DAC1EN); \ 00929 /* Delay after an RCC peripheral clock enabling */ \ 00930 tmpreg = READ_BIT(RCC->APB1ENR1, RCC_APB1ENR1_DAC1EN); \ 00931 UNUSED(tmpreg); \ 00932 } while(0) 00933 00934 #define __HAL_RCC_OPAMP_CLK_ENABLE() do { \ 00935 __IO uint32_t tmpreg; \ 00936 SET_BIT(RCC->APB1ENR1, RCC_APB1ENR1_OPAMPEN); \ 00937 /* Delay after an RCC peripheral clock enabling */ \ 00938 tmpreg = READ_BIT(RCC->APB1ENR1, RCC_APB1ENR1_OPAMPEN); \ 00939 UNUSED(tmpreg); \ 00940 } while(0) 00941 00942 #define __HAL_RCC_LPTIM1_CLK_ENABLE() do { \ 00943 __IO uint32_t tmpreg; \ 00944 SET_BIT(RCC->APB1ENR1, RCC_APB1ENR1_LPTIM1EN); \ 00945 /* Delay after an RCC peripheral clock enabling */ \ 00946 tmpreg = READ_BIT(RCC->APB1ENR1, RCC_APB1ENR1_LPTIM1EN); \ 00947 UNUSED(tmpreg); \ 00948 } while(0) 00949 00950 #define __HAL_RCC_LPUART1_CLK_ENABLE() do { \ 00951 __IO uint32_t tmpreg; \ 00952 SET_BIT(RCC->APB1ENR2, RCC_APB1ENR2_LPUART1EN); \ 00953 /* Delay after an RCC peripheral clock enabling */ \ 00954 tmpreg = READ_BIT(RCC->APB1ENR2, RCC_APB1ENR2_LPUART1EN); \ 00955 UNUSED(tmpreg); \ 00956 } while(0) 00957 00958 #define __HAL_RCC_SWPMI1_CLK_ENABLE() do { \ 00959 __IO uint32_t tmpreg; \ 00960 SET_BIT(RCC->APB1ENR2, RCC_APB1ENR2_SWPMI1EN); \ 00961 /* Delay after an RCC peripheral clock enabling */ \ 00962 tmpreg = READ_BIT(RCC->APB1ENR2, RCC_APB1ENR2_SWPMI1EN); \ 00963 UNUSED(tmpreg); \ 00964 } while(0) 00965 00966 #define __HAL_RCC_LPTIM2_CLK_ENABLE() do { \ 00967 __IO uint32_t tmpreg; \ 00968 SET_BIT(RCC->APB1ENR2, RCC_APB1ENR2_LPTIM2EN); \ 00969 /* Delay after an RCC peripheral clock enabling */ \ 00970 tmpreg = READ_BIT(RCC->APB1ENR2, RCC_APB1ENR2_LPTIM2EN); \ 00971 UNUSED(tmpreg); \ 00972 } while(0) 00973 00974 #define __HAL_RCC_TIM2_CLK_DISABLE() CLEAR_BIT(RCC->APB1ENR1, RCC_APB1ENR1_TIM2EN) 00975 00976 #define __HAL_RCC_TIM3_CLK_DISABLE() CLEAR_BIT(RCC->APB1ENR1, RCC_APB1ENR1_TIM3EN) 00977 00978 #define __HAL_RCC_TIM4_CLK_DISABLE() CLEAR_BIT(RCC->APB1ENR1, RCC_APB1ENR1_TIM4EN) 00979 00980 #define __HAL_RCC_TIM5_CLK_DISABLE() CLEAR_BIT(RCC->APB1ENR1, RCC_APB1ENR1_TIM5EN) 00981 00982 #define __HAL_RCC_TIM6_CLK_DISABLE() CLEAR_BIT(RCC->APB1ENR1, RCC_APB1ENR1_TIM6EN) 00983 00984 #define __HAL_RCC_TIM7_CLK_DISABLE() CLEAR_BIT(RCC->APB1ENR1, RCC_APB1ENR1_TIM7EN) 00985 00986 #if defined(STM32L476xx) || defined(STM32L486xx) 00987 #define __HAL_RCC_LCD_CLK_DISABLE() CLEAR_BIT(RCC->APB1ENR1, RCC_APB1ENR1_LCDEN); 00988 #endif /* STM32L476xx || STM32L486xx */ 00989 00990 #define __HAL_RCC_WWDG_CLK_DISABLE() CLEAR_BIT(RCC->APB1ENR1, RCC_APB1ENR1_WWDGEN) 00991 00992 #define __HAL_RCC_SPI2_CLK_DISABLE() CLEAR_BIT(RCC->APB1ENR1, RCC_APB1ENR1_SPI2EN) 00993 00994 #define __HAL_RCC_SPI3_CLK_DISABLE() CLEAR_BIT(RCC->APB1ENR1, RCC_APB1ENR1_SPI3EN) 00995 00996 #define __HAL_RCC_USART2_CLK_DISABLE() CLEAR_BIT(RCC->APB1ENR1, RCC_APB1ENR1_USART2EN) 00997 00998 #define __HAL_RCC_USART3_CLK_DISABLE() CLEAR_BIT(RCC->APB1ENR1, RCC_APB1ENR1_USART3EN) 00999 01000 #define __HAL_RCC_UART4_CLK_DISABLE() CLEAR_BIT(RCC->APB1ENR1, RCC_APB1ENR1_UART4EN) 01001 01002 #define __HAL_RCC_UART5_CLK_DISABLE() CLEAR_BIT(RCC->APB1ENR1, RCC_APB1ENR1_UART5EN) 01003 01004 #define __HAL_RCC_I2C1_CLK_DISABLE() CLEAR_BIT(RCC->APB1ENR1, RCC_APB1ENR1_I2C1EN) 01005 01006 #define __HAL_RCC_I2C2_CLK_DISABLE() CLEAR_BIT(RCC->APB1ENR1, RCC_APB1ENR1_I2C2EN) 01007 01008 #define __HAL_RCC_I2C3_CLK_DISABLE() CLEAR_BIT(RCC->APB1ENR1, RCC_APB1ENR1_I2C3EN) 01009 01010 #define __HAL_RCC_CAN1_CLK_DISABLE() CLEAR_BIT(RCC->APB1ENR1, RCC_APB1ENR1_CAN1EN) 01011 01012 #define __HAL_RCC_PWR_CLK_DISABLE() CLEAR_BIT(RCC->APB1ENR1, RCC_APB1ENR1_PWREN) 01013 01014 #define __HAL_RCC_DAC1_CLK_DISABLE() CLEAR_BIT(RCC->APB1ENR1, RCC_APB1ENR1_DAC1EN) 01015 01016 #define __HAL_RCC_OPAMP_CLK_DISABLE() CLEAR_BIT(RCC->APB1ENR1, RCC_APB1ENR1_OPAMPEN) 01017 01018 #define __HAL_RCC_LPTIM1_CLK_DISABLE() CLEAR_BIT(RCC->APB1ENR1, RCC_APB1ENR1_LPTIM1EN) 01019 01020 #define __HAL_RCC_LPUART1_CLK_DISABLE() CLEAR_BIT(RCC->APB1ENR2, RCC_APB1ENR2_LPUART1EN) 01021 01022 #define __HAL_RCC_SWPMI1_CLK_DISABLE() CLEAR_BIT(RCC->APB1ENR2, RCC_APB1ENR2_SWPMI1EN) 01023 01024 #define __HAL_RCC_LPTIM2_CLK_DISABLE() CLEAR_BIT(RCC->APB1ENR2, RCC_APB1ENR2_LPTIM2EN) 01025 01026 /** 01027 * @} 01028 */ 01029 01030 /** @defgroup RCC_APB2_Clock_Enable_Disable APB2 Peripheral Clock Enable Disable 01031 * @brief Enable or disable the APB2 peripheral clock. 01032 * @note After reset, the peripheral clock (used for registers read/write access) 01033 * is disabled and the application software has to enable this clock before 01034 * using it. 01035 * @{ 01036 */ 01037 01038 #define __HAL_RCC_SYSCFG_CLK_ENABLE() do { \ 01039 __IO uint32_t tmpreg; \ 01040 SET_BIT(RCC->APB2ENR, RCC_APB2ENR_SYSCFGEN); \ 01041 /* Delay after an RCC peripheral clock enabling */ \ 01042 tmpreg = READ_BIT(RCC->APB2ENR, RCC_APB2ENR_SYSCFGEN); \ 01043 UNUSED(tmpreg); \ 01044 } while(0) 01045 01046 #define __HAL_RCC_FIREWALL_CLK_ENABLE() do { \ 01047 __IO uint32_t tmpreg; \ 01048 SET_BIT(RCC->APB2ENR, RCC_APB2ENR_FWEN); \ 01049 /* Delay after an RCC peripheral clock enabling */ \ 01050 tmpreg = READ_BIT(RCC->APB2ENR, RCC_APB2ENR_FWEN); \ 01051 UNUSED(tmpreg); \ 01052 } while(0) 01053 01054 #define __HAL_RCC_SDMMC1_CLK_ENABLE() do { \ 01055 __IO uint32_t tmpreg; \ 01056 SET_BIT(RCC->APB2ENR, RCC_APB2ENR_SDMMC1EN); \ 01057 /* Delay after an RCC peripheral clock enabling */ \ 01058 tmpreg = READ_BIT(RCC->APB2ENR, RCC_APB2ENR_SDMMC1EN); \ 01059 UNUSED(tmpreg); \ 01060 } while(0) 01061 01062 #define __HAL_RCC_TIM1_CLK_ENABLE() do { \ 01063 __IO uint32_t tmpreg; \ 01064 SET_BIT(RCC->APB2ENR, RCC_APB2ENR_TIM1EN); \ 01065 /* Delay after an RCC peripheral clock enabling */ \ 01066 tmpreg = READ_BIT(RCC->APB2ENR, RCC_APB2ENR_TIM1EN); \ 01067 UNUSED(tmpreg); \ 01068 } while(0) 01069 01070 #define __HAL_RCC_SPI1_CLK_ENABLE() do { \ 01071 __IO uint32_t tmpreg; \ 01072 SET_BIT(RCC->APB2ENR, RCC_APB2ENR_SPI1EN); \ 01073 /* Delay after an RCC peripheral clock enabling */ \ 01074 tmpreg = READ_BIT(RCC->APB2ENR, RCC_APB2ENR_SPI1EN); \ 01075 UNUSED(tmpreg); \ 01076 } while(0) 01077 01078 #define __HAL_RCC_TIM8_CLK_ENABLE() do { \ 01079 __IO uint32_t tmpreg; \ 01080 SET_BIT(RCC->APB2ENR, RCC_APB2ENR_TIM8EN); \ 01081 /* Delay after an RCC peripheral clock enabling */ \ 01082 tmpreg = READ_BIT(RCC->APB2ENR, RCC_APB2ENR_TIM8EN); \ 01083 UNUSED(tmpreg); \ 01084 } while(0) 01085 01086 #define __HAL_RCC_USART1_CLK_ENABLE() do { \ 01087 __IO uint32_t tmpreg; \ 01088 SET_BIT(RCC->APB2ENR, RCC_APB2ENR_USART1EN); \ 01089 /* Delay after an RCC peripheral clock enabling */ \ 01090 tmpreg = READ_BIT(RCC->APB2ENR, RCC_APB2ENR_USART1EN); \ 01091 UNUSED(tmpreg); \ 01092 } while(0) 01093 01094 01095 #define __HAL_RCC_TIM15_CLK_ENABLE() do { \ 01096 __IO uint32_t tmpreg; \ 01097 SET_BIT(RCC->APB2ENR, RCC_APB2ENR_TIM15EN); \ 01098 /* Delay after an RCC peripheral clock enabling */ \ 01099 tmpreg = READ_BIT(RCC->APB2ENR, RCC_APB2ENR_TIM15EN); \ 01100 UNUSED(tmpreg); \ 01101 } while(0) 01102 01103 #define __HAL_RCC_TIM16_CLK_ENABLE() do { \ 01104 __IO uint32_t tmpreg; \ 01105 SET_BIT(RCC->APB2ENR, RCC_APB2ENR_TIM16EN); \ 01106 /* Delay after an RCC peripheral clock enabling */ \ 01107 tmpreg = READ_BIT(RCC->APB2ENR, RCC_APB2ENR_TIM16EN); \ 01108 UNUSED(tmpreg); \ 01109 } while(0) 01110 01111 #define __HAL_RCC_TIM17_CLK_ENABLE() do { \ 01112 __IO uint32_t tmpreg; \ 01113 SET_BIT(RCC->APB2ENR, RCC_APB2ENR_TIM17EN); \ 01114 /* Delay after an RCC peripheral clock enabling */ \ 01115 tmpreg = READ_BIT(RCC->APB2ENR, RCC_APB2ENR_TIM17EN); \ 01116 UNUSED(tmpreg); \ 01117 } while(0) 01118 01119 #define __HAL_RCC_SAI1_CLK_ENABLE() do { \ 01120 __IO uint32_t tmpreg; \ 01121 SET_BIT(RCC->APB2ENR, RCC_APB2ENR_SAI1EN); \ 01122 /* Delay after an RCC peripheral clock enabling */ \ 01123 tmpreg = READ_BIT(RCC->APB2ENR, RCC_APB2ENR_SAI1EN); \ 01124 UNUSED(tmpreg); \ 01125 } while(0) 01126 01127 #define __HAL_RCC_SAI2_CLK_ENABLE() do { \ 01128 __IO uint32_t tmpreg; \ 01129 SET_BIT(RCC->APB2ENR, RCC_APB2ENR_SAI2EN); \ 01130 /* Delay after an RCC peripheral clock enabling */ \ 01131 tmpreg = READ_BIT(RCC->APB2ENR, RCC_APB2ENR_SAI2EN); \ 01132 UNUSED(tmpreg); \ 01133 } while(0) 01134 01135 #define __HAL_RCC_DFSDM_CLK_ENABLE() do { \ 01136 __IO uint32_t tmpreg; \ 01137 SET_BIT(RCC->APB2ENR, RCC_APB2ENR_DFSDMEN); \ 01138 /* Delay after an RCC peripheral clock enabling */ \ 01139 tmpreg = READ_BIT(RCC->APB2ENR, RCC_APB2ENR_DFSDMEN); \ 01140 UNUSED(tmpreg); \ 01141 } while(0) 01142 01143 #define __HAL_RCC_SYSCFG_CLK_DISABLE() CLEAR_BIT(RCC->APB2ENR, RCC_APB2ENR_SYSCFGEN) 01144 01145 #define __HAL_RCC_SDMMC1_CLK_DISABLE() CLEAR_BIT(RCC->APB2ENR, RCC_APB2ENR_SDMMC1EN) 01146 01147 #define __HAL_RCC_TIM1_CLK_DISABLE() CLEAR_BIT(RCC->APB2ENR, RCC_APB2ENR_TIM1EN) 01148 01149 #define __HAL_RCC_SPI1_CLK_DISABLE() CLEAR_BIT(RCC->APB2ENR, RCC_APB2ENR_SPI1EN) 01150 01151 #define __HAL_RCC_TIM8_CLK_DISABLE() CLEAR_BIT(RCC->APB2ENR, RCC_APB2ENR_TIM8EN) 01152 01153 #define __HAL_RCC_USART1_CLK_DISABLE() CLEAR_BIT(RCC->APB2ENR, RCC_APB2ENR_USART1EN) 01154 01155 #define __HAL_RCC_TIM15_CLK_DISABLE() CLEAR_BIT(RCC->APB2ENR, RCC_APB2ENR_TIM15EN) 01156 01157 #define __HAL_RCC_TIM16_CLK_DISABLE() CLEAR_BIT(RCC->APB2ENR, RCC_APB2ENR_TIM16EN) 01158 01159 #define __HAL_RCC_TIM17_CLK_DISABLE() CLEAR_BIT(RCC->APB2ENR, RCC_APB2ENR_TIM17EN) 01160 01161 #define __HAL_RCC_SAI1_CLK_DISABLE() CLEAR_BIT(RCC->APB2ENR, RCC_APB2ENR_SAI1EN) 01162 01163 #define __HAL_RCC_SAI2_CLK_DISABLE() CLEAR_BIT(RCC->APB2ENR, RCC_APB2ENR_SAI2EN) 01164 01165 #define __HAL_RCC_DFSDM_CLK_DISABLE() CLEAR_BIT(RCC->APB2ENR, RCC_APB2ENR_DFSDMEN) 01166 01167 /** 01168 * @} 01169 */ 01170 01171 /** @defgroup RCC_AHB1_Peripheral_Clock_Enable_Disable_Status AHB1 Peripheral Clock Enabled or Disabled Status 01172 * @brief Check whether the AHB1 peripheral clock is enabled or not. 01173 * @note After reset, the peripheral clock (used for registers read/write access) 01174 * is disabled and the application software has to enable this clock before 01175 * using it. 01176 * @{ 01177 */ 01178 01179 #define __HAL_RCC_DMA1_IS_CLK_ENABLED() (READ_BIT(RCC->AHB1ENR, RCC_AHB1ENR_DMA1EN) != RESET) 01180 01181 #define __HAL_RCC_DMA2_IS_CLK_ENABLED() (READ_BIT(RCC->AHB1ENR, RCC_AHB1ENR_DMA2EN) != RESET) 01182 01183 #define __HAL_RCC_FLASH_IS_CLK_ENABLED() (READ_BIT(RCC->AHB1ENR, RCC_AHB1ENR_FLASHEN) != RESET) 01184 01185 #define __HAL_RCC_CRC_IS_CLK_ENABLED() (READ_BIT(RCC->AHB1ENR, RCC_AHB1ENR_CRCEN) != RESET) 01186 01187 #define __HAL_RCC_TSC_IS_CLK_ENABLED() (READ_BIT(RCC->AHB1ENR, RCC_AHB1ENR_TSCEN) != RESET) 01188 01189 #define __HAL_RCC_DMA1_IS_CLK_DISABLED() (READ_BIT(RCC->AHB1ENR, RCC_AHB1ENR_DMA1EN) == RESET) 01190 01191 #define __HAL_RCC_DMA2_IS_CLK_DISABLED() (READ_BIT(RCC->AHB1ENR, RCC_AHB1ENR_DMA2EN) == RESET) 01192 01193 #define __HAL_RCC_FLASH_IS_CLK_DISABLED() (READ_BIT(RCC->AHB1ENR, RCC_AHB1ENR_FLASHEN) == RESET) 01194 01195 #define __HAL_RCC_CRC_IS_CLK_DISABLED() (READ_BIT(RCC->AHB1ENR, RCC_AHB1ENR_CRCEN) == RESET) 01196 01197 #define __HAL_RCC_TSC_IS_CLK_DISABLED() (READ_BIT(RCC->AHB1ENR, RCC_AHB1ENR_TSCEN) == RESET) 01198 01199 /** 01200 * @} 01201 */ 01202 01203 /** @defgroup RCC_AHB2_Clock_Enable_Disable_Status AHB2 Peripheral Clock Enabled or Disabled Status 01204 * @brief Check whether the AHB2 peripheral clock is enabled or not. 01205 * @note After reset, the peripheral clock (used for registers read/write access) 01206 * is disabled and the application software has to enable this clock before 01207 * using it. 01208 * @{ 01209 */ 01210 01211 #define __HAL_RCC_GPIOA_IS_CLK_ENABLED() (READ_BIT(RCC->AHB2ENR, RCC_AHB2ENR_GPIOAEN) != RESET) 01212 01213 #define __HAL_RCC_GPIOB_IS_CLK_ENABLED() (READ_BIT(RCC->AHB2ENR, RCC_AHB2ENR_GPIOCEN) != RESET) 01214 01215 #define __HAL_RCC_GPIOC_IS_CLK_ENABLED() (READ_BIT(RCC->AHB2ENR, RCC_AHB2ENR_GPIOCEN) != RESET) 01216 01217 #define __HAL_RCC_GPIOD_IS_CLK_ENABLED() (READ_BIT(RCC->AHB2ENR, RCC_AHB2ENR_GPIODEN) != RESET) 01218 01219 #define __HAL_RCC_GPIOE_IS_CLK_ENABLED() (READ_BIT(RCC->AHB2ENR, RCC_AHB2ENR_GPIOEEN) != RESET) 01220 01221 #define __HAL_RCC_GPIOF_IS_CLK_ENABLED() (READ_BIT(RCC->AHB2ENR, RCC_AHB2ENR_GPIOFEN) != RESET) 01222 01223 #define __HAL_RCC_GPIOG_IS_CLK_ENABLED() (READ_BIT(RCC->AHB2ENR, RCC_AHB2ENR_GPIOGEN) != RESET) 01224 01225 #define __HAL_RCC_GPIOH_IS_CLK_ENABLED() (READ_BIT(RCC->AHB2ENR, RCC_AHB2ENR_GPIOHEN) != RESET) 01226 01227 #if defined(STM32L475xx) || defined(STM32L476xx) || defined(STM32L485xx) || defined(STM32L486xx) 01228 #define __HAL_RCC_USB_OTG_FS_IS_CLK_ENABLED() (READ_BIT(RCC->AHB2ENR, RCC_AHB2ENR_OTGFSEN) != RESET) 01229 #endif /* STM32L475xx || STM32L476xx || STM32L485xx || STM32L486xx */ 01230 01231 #define __HAL_RCC_ADC_IS_CLK_ENABLED() (READ_BIT(RCC->AHB2ENR, RCC_AHB2ENR_ADCEN) != RESET) 01232 01233 #if defined(STM32L485xx) || defined(STM32L486xx) 01234 #define __HAL_RCC_AES_IS_CLK_ENABLED() (READ_BIT(RCC->AHB2ENR, RCC_AHB2ENR_AESEN) != RESET) 01235 #endif /* STM32L485xx || STM32L486xx */ 01236 01237 #define __HAL_RCC_RNG_IS_CLK_ENABLED() (READ_BIT(RCC->AHB2ENR, RCC_AHB2ENR_RNGEN) != RESET) 01238 01239 #define __HAL_RCC_GPIOA_IS_CLK_DISABLED() (READ_BIT(RCC->AHB2ENR, RCC_AHB2ENR_GPIOAEN) == RESET) 01240 01241 #define __HAL_RCC_GPIOB_IS_CLK_DISABLED() (READ_BIT(RCC->AHB2ENR, RCC_AHB2ENR_GPIOBEN) == RESET) 01242 01243 #define __HAL_RCC_GPIOC_IS_CLK_DISABLED() (READ_BIT(RCC->AHB2ENR, RCC_AHB2ENR_GPIOCEN) == RESET) 01244 01245 #define __HAL_RCC_GPIOD_IS_CLK_DISABLED() (READ_BIT(RCC->AHB2ENR, RCC_AHB2ENR_GPIODEN) == RESET) 01246 01247 #define __HAL_RCC_GPIOE_IS_CLK_DISABLED() (READ_BIT(RCC->AHB2ENR, RCC_AHB2ENR_GPIOEEN) == RESET) 01248 01249 #define __HAL_RCC_GPIOF_IS_CLK_DISABLED() (READ_BIT(RCC->AHB2ENR, RCC_AHB2ENR_GPIOFEN) == RESET) 01250 01251 #define __HAL_RCC_GPIOG_IS_CLK_DISABLED() (READ_BIT(RCC->AHB2ENR, RCC_AHB2ENR_GPIOGEN) == RESET) 01252 01253 #define __HAL_RCC_GPIOH_IS_CLK_DISABLED() (READ_BIT(RCC->AHB2ENR, RCC_AHB2ENR_GPIOHEN) == RESET) 01254 01255 #if defined(STM32L475xx) || defined(STM32L476xx) || defined(STM32L485xx) || defined(STM32L486xx) 01256 #define __HAL_RCC_USB_OTG_FS_IS_CLK_DISABLED() (READ_BIT(RCC->AHB2ENR, RCC_AHB2ENR_OTGFSEN) == RESET) 01257 #endif /* STM32L475xx || STM32L476xx || STM32L485xx || STM32L486xx */ 01258 01259 #define __HAL_RCC_ADC_IS_CLK_DISABLED() (READ_BIT(RCC->AHB2ENR, RCC_AHB2ENR_ADCEN) == RESET) 01260 01261 #if defined(STM32L485xx) || defined(STM32L486xx) 01262 #define __HAL_RCC_AES_IS_CLK_DISABLED() (READ_BIT(RCC->AHB2ENR, RCC_AHB2ENR_AESEN) == RESET) 01263 #endif /* STM32L485xx || STM32L486xx */ 01264 01265 #define __HAL_RCC_RNG_IS_CLK_DISABLED() (READ_BIT(RCC->AHB2ENR, RCC_AHB2ENR_RNGEN) == RESET) 01266 01267 /** 01268 * @} 01269 */ 01270 01271 /** @defgroup RCC_AHB3_Clock_Enable_Disable_Status AHB3 Peripheral Clock Enabled or Disabled Status 01272 * @brief Check whether the AHB3 peripheral clock is enabled or not. 01273 * @note After reset, the peripheral clock (used for registers read/write access) 01274 * is disabled and the application software has to enable this clock before 01275 * using it. 01276 * @{ 01277 */ 01278 01279 #define __HAL_RCC_FMC_IS_CLK_ENABLED() (READ_BIT(RCC->AHB3ENR, RCC_AHB3ENR_FMCEN) != RESET) 01280 01281 #define __HAL_RCC_QSPI_IS_CLK_ENABLED() (READ_BIT(RCC->AHB3ENR, RCC_AHB3ENR_QSPIEN) != RESET) 01282 01283 #define __HAL_RCC_FMC_IS_CLK_DISABLED() (READ_BIT(RCC->AHB3ENR, RCC_AHB3ENR_FMCEN) == RESET) 01284 01285 #define __HAL_RCC_QSPI_IS_CLK_DISABLED() (READ_BIT(RCC->AHB3ENR, RCC_AHB3ENR_QSPIEN) == RESET) 01286 01287 /** 01288 * @} 01289 */ 01290 01291 /** @defgroup RCC_APB1_Clock_Enable_Disable_Status APB1 Peripheral Clock Enabled or Disabled Status 01292 * @brief Check whether the APB1 peripheral clock is enabled or not. 01293 * @note After reset, the peripheral clock (used for registers read/write access) 01294 * is disabled and the application software has to enable this clock before 01295 * using it. 01296 * @{ 01297 */ 01298 01299 #define __HAL_RCC_TIM2_IS_CLK_ENABLED() (READ_BIT(RCC->APB1ENR1, RCC_APB1ENR1_TIM2EN) != RESET) 01300 01301 #define __HAL_RCC_TIM3_IS_CLK_ENABLED() (READ_BIT(RCC->APB1ENR1, RCC_APB1ENR1_TIM3EN) != RESET) 01302 01303 #define __HAL_RCC_TIM4_IS_CLK_ENABLED() (READ_BIT(RCC->APB1ENR1, RCC_APB1ENR1_TIM4EN) != RESET) 01304 01305 #define __HAL_RCC_TIM5_IS_CLK_ENABLED() (READ_BIT(RCC->APB1ENR1, RCC_APB1ENR1_TIM5EN) != RESET) 01306 01307 #define __HAL_RCC_TIM6_IS_CLK_ENABLED() (READ_BIT(RCC->APB1ENR1, RCC_APB1ENR1_TIM6EN) != RESET) 01308 01309 #define __HAL_RCC_TIM7_IS_CLK_ENABLED() (READ_BIT(RCC->APB1ENR1, RCC_APB1ENR1_TIM7EN) != RESET) 01310 01311 #if defined(STM32L476xx) || defined(STM32L486xx) 01312 #define __HAL_RCC_LCD_IS_CLK_ENABLED() (READ_BIT(RCC->APB1ENR1, RCC_APB1ENR1_LCDEN) != RESET) 01313 #endif /* STM32L476xx || STM32L486xx */ 01314 01315 #define __HAL_RCC_WWDG_IS_CLK_ENABLED() (READ_BIT(RCC->APB1ENR1, RCC_APB1ENR1_WWDGEN) != RESET) 01316 01317 #define __HAL_RCC_SPI2_IS_CLK_ENABLED() (READ_BIT(RCC->APB1ENR1, RCC_APB1ENR1_SPI2EN) != RESET) 01318 01319 #define __HAL_RCC_SPI3_IS_CLK_ENABLED() (READ_BIT(RCC->APB1ENR1, RCC_APB1ENR1_SPI3EN) != RESET) 01320 01321 #define __HAL_RCC_USART2_IS_CLK_ENABLED() (READ_BIT(RCC->APB1ENR1, RCC_APB1ENR1_USART2EN) != RESET) 01322 01323 #define __HAL_RCC_USART3_IS_CLK_ENABLED() (READ_BIT(RCC->APB1ENR1, RCC_APB1ENR1_USART3EN) != RESET) 01324 01325 #define __HAL_RCC_UART4_IS_CLK_ENABLED() (READ_BIT(RCC->APB1ENR1, RCC_APB1ENR1_UART4EN) != RESET) 01326 01327 #define __HAL_RCC_UART5_IS_CLK_ENABLED() (READ_BIT(RCC->APB1ENR1, RCC_APB1ENR1_UART5EN) != RESET) 01328 01329 #define __HAL_RCC_I2C1_IS_CLK_ENABLED() (READ_BIT(RCC->APB1ENR1, RCC_APB1ENR1_I2C1EN) != RESET) 01330 01331 #define __HAL_RCC_I2C2_IS_CLK_ENABLED() (READ_BIT(RCC->APB1ENR1, RCC_APB1ENR1_I2C2EN) != RESET) 01332 01333 #define __HAL_RCC_I2C3_IS_CLK_ENABLED() (READ_BIT(RCC->APB1ENR1, RCC_APB1ENR1_I2C3EN) != RESET) 01334 01335 #define __HAL_RCC_CAN1_IS_CLK_ENABLED() (READ_BIT(RCC->APB1ENR1, RCC_APB1ENR1_CAN1EN) != RESET) 01336 01337 #define __HAL_RCC_PWR_IS_CLK_ENABLED() (READ_BIT(RCC->APB1ENR1, RCC_APB1ENR1_PWREN) != RESET) 01338 01339 #define __HAL_RCC_DAC1_IS_CLK_ENABLED() (READ_BIT(RCC->APB1ENR1, RCC_APB1ENR1_DAC1EN) != RESET) 01340 01341 #define __HAL_RCC_OPAMP_IS_CLK_ENABLED() (READ_BIT(RCC->APB1ENR1, RCC_APB1ENR1_OPAMPEN) != RESET) 01342 01343 #define __HAL_RCC_LPTIM1_IS_CLK_ENABLED() (READ_BIT(RCC->APB1ENR1, RCC_APB1ENR1_LPTIM1EN) != RESET) 01344 01345 #define __HAL_RCC_LPUART1_IS_CLK_ENABLED() (READ_BIT(RCC->APB1ENR2, RCC_APB1ENR2_LPUART1EN) != RESET) 01346 01347 #define __HAL_RCC_SWPMI1_IS_CLK_ENABLED() (READ_BIT(RCC->APB1ENR2, RCC_APB1ENR2_SWPMI1EN) != RESET) 01348 01349 #define __HAL_RCC_LPTIM2_IS_CLK_ENABLED() (READ_BIT(RCC->APB1ENR2, RCC_APB1ENR2_LPTIM2EN) != RESET) 01350 01351 #define __HAL_RCC_TIM2_IS_CLK_DISABLED() (READ_BIT(RCC->APB1ENR1, RCC_APB1ENR1_TIM2EN) == RESET) 01352 01353 #define __HAL_RCC_TIM3_IS_CLK_DISABLED() (READ_BIT(RCC->APB1ENR1, RCC_APB1ENR1_TIM3EN) == RESET) 01354 01355 #define __HAL_RCC_TIM4_IS_CLK_DISABLED() (READ_BIT(RCC->APB1ENR1, RCC_APB1ENR1_TIM4EN) == RESET) 01356 01357 #define __HAL_RCC_TIM5_IS_CLK_DISABLED() (READ_BIT(RCC->APB1ENR1, RCC_APB1ENR1_TIM5EN) == RESET) 01358 01359 #define __HAL_RCC_TIM6_IS_CLK_DISABLED() (READ_BIT(RCC->APB1ENR1, RCC_APB1ENR1_TIM6EN) == RESET) 01360 01361 #define __HAL_RCC_TIM7_IS_CLK_DISABLED() (READ_BIT(RCC->APB1ENR1, RCC_APB1ENR1_TIM7EN) == RESET) 01362 01363 #if defined(STM32L476xx) || defined(STM32L486xx) 01364 #define __HAL_RCC_LCD_IS_CLK_DISABLED() (READ_BIT(RCC->APB1ENR1, RCC_APB1ENR1_LCDEN) == RESET) 01365 #endif /* STM32L476xx || STM32L486xx */ 01366 01367 #define __HAL_RCC_WWDG_IS_CLK_DISABLED() (READ_BIT(RCC->APB1ENR1, RCC_APB1ENR1_WWDGEN) == RESET) 01368 01369 #define __HAL_RCC_SPI2_IS_CLK_DISABLED() (READ_BIT(RCC->APB1ENR1, RCC_APB1ENR1_SPI2EN) == RESET) 01370 01371 #define __HAL_RCC_SPI3_IS_CLK_DISABLED() (READ_BIT(RCC->APB1ENR1, RCC_APB1ENR1_SPI3EN) == RESET) 01372 01373 #define __HAL_RCC_USART2_IS_CLK_DISABLED() (READ_BIT(RCC->APB1ENR1, RCC_APB1ENR1_USART2EN) == RESET) 01374 01375 #define __HAL_RCC_USART3_IS_CLK_DISABLED() (READ_BIT(RCC->APB1ENR1, RCC_APB1ENR1_USART3EN) == RESET) 01376 01377 #define __HAL_RCC_UART4_IS_CLK_DISABLED() (READ_BIT(RCC->APB1ENR1, RCC_APB1ENR1_UART4EN) == RESET) 01378 01379 #define __HAL_RCC_UART5_IS_CLK_DISABLED() (READ_BIT(RCC->APB1ENR1, RCC_APB1ENR1_UART5EN) == RESET) 01380 01381 #define __HAL_RCC_I2C1_IS_CLK_DISABLED() (READ_BIT(RCC->APB1ENR1, RCC_APB1ENR1_I2C1EN) == RESET) 01382 01383 #define __HAL_RCC_I2C2_IS_CLK_DISABLED() (READ_BIT(RCC->APB1ENR1, RCC_APB1ENR1_I2C2EN) == RESET) 01384 01385 #define __HAL_RCC_I2C3_IS_CLK_DISABLED() (READ_BIT(RCC->APB1ENR1, RCC_APB1ENR1_I2C3EN) == RESET) 01386 01387 #define __HAL_RCC_CAN1_IS_CLK_DISABLED() (READ_BIT(RCC->APB1ENR1, RCC_APB1ENR1_CAN1EN) == RESET) 01388 01389 #define __HAL_RCC_PWR_IS_CLK_DISABLED() (READ_BIT(RCC->APB1ENR1, RCC_APB1ENR1_PWREN) == RESET) 01390 01391 #define __HAL_RCC_DAC1_IS_CLK_DISABLED() (READ_BIT(RCC->APB1ENR1, RCC_APB1ENR1_DAC1EN) == RESET) 01392 01393 #define __HAL_RCC_OPAMP_IS_CLK_DISABLED() (READ_BIT(RCC->APB1ENR1, RCC_APB1ENR1_OPAMPEN) == RESET) 01394 01395 #define __HAL_RCC_LPTIM1_IS_CLK_DISABLED() (READ_BIT(RCC->APB1ENR1, RCC_APB1ENR1_LPTIM1EN) == RESET) 01396 01397 #define __HAL_RCC_LPUART1_IS_CLK_DISABLED() (READ_BIT(RCC->APB1ENR2, RCC_APB1ENR2_LPUART1EN) == RESET) 01398 01399 #define __HAL_RCC_SWPMI1_IS_CLK_DISABLED() (READ_BIT(RCC->APB1ENR2, RCC_APB1ENR2_SWPMI1EN) == RESET) 01400 01401 #define __HAL_RCC_LPTIM2_IS_CLK_DISABLED() (READ_BIT(RCC->APB1ENR2, RCC_APB1ENR2_LPTIM2EN) == RESET) 01402 01403 /** 01404 * @} 01405 */ 01406 01407 /** @defgroup RCC_APB2_Clock_Enable_Disable_Status APB2 Peripheral Clock Enabled or Disabled Status 01408 * @brief Check whether the APB2 peripheral clock is enabled or not. 01409 * @note After reset, the peripheral clock (used for registers read/write access) 01410 * is disabled and the application software has to enable this clock before 01411 * using it. 01412 * @{ 01413 */ 01414 01415 #define __HAL_RCC_SYSCFG_IS_CLK_ENABLED() (READ_BIT(RCC->APB2ENR, RCC_APB2ENR_SYSCFGEN) != RESET) 01416 01417 #define __HAL_RCC_FIREWALL_IS_CLK_ENABLED() (READ_BIT(RCC->APB2ENR, RCC_APB2ENR_FWEN) != RESET) 01418 01419 #define __HAL_RCC_SDMMC1_IS_CLK_ENABLED() (READ_BIT(RCC->APB2ENR, RCC_APB2ENR_SDMMC1EN) != RESET) 01420 01421 #define __HAL_RCC_TIM1_IS_CLK_ENABLED() (READ_BIT(RCC->APB2ENR, RCC_APB2ENR_TIM1EN) != RESET) 01422 01423 #define __HAL_RCC_SPI1_IS_CLK_ENABLED() (READ_BIT(RCC->APB2ENR, RCC_APB2ENR_SPI1EN) != RESET) 01424 01425 #define __HAL_RCC_TIM8_IS_CLK_ENABLED() (READ_BIT(RCC->APB2ENR, RCC_APB2ENR_TIM8EN) != RESET) 01426 01427 #define __HAL_RCC_USART1_IS_CLK_ENABLED() (READ_BIT(RCC->APB2ENR, RCC_APB2ENR_USART1EN) != RESET) 01428 01429 #define __HAL_RCC_TIM15_IS_CLK_ENABLED() (READ_BIT(RCC->APB2ENR, RCC_APB2ENR_TIM15EN) != RESET) 01430 01431 #define __HAL_RCC_TIM16_IS_CLK_ENABLED() (READ_BIT(RCC->APB2ENR, RCC_APB2ENR_TIM16EN) != RESET) 01432 01433 #define __HAL_RCC_TIM17_IS_CLK_ENABLED() (READ_BIT(RCC->APB2ENR, RCC_APB2ENR_TIM17EN) != RESET) 01434 01435 #define __HAL_RCC_SAI1_IS_CLK_ENABLED() (READ_BIT(RCC->APB2ENR, RCC_APB2ENR_SAI1EN) != RESET) 01436 01437 #define __HAL_RCC_SAI2_IS_CLK_ENABLED() (READ_BIT(RCC->APB2ENR, RCC_APB2ENR_SAI2EN) != RESET) 01438 01439 #define __HAL_RCC_DFSDM_IS_CLK_ENABLED() (READ_BIT(RCC->APB2ENR, RCC_APB2ENR_DFSDMEN) != RESET) 01440 01441 #define __HAL_RCC_SYSCFG_IS_CLK_DISABLED() (READ_BIT(RCC->APB2ENR, RCC_APB2ENR_SYSCFGEN) == RESET) 01442 01443 #define __HAL_RCC_SDMMC1_IS_CLK_DISABLED() (READ_BIT(RCC->APB2ENR, RCC_APB2ENR_SDMMC1EN) == RESET) 01444 01445 #define __HAL_RCC_TIM1_IS_CLK_DISABLED() (READ_BIT(RCC->APB2ENR, RCC_APB2ENR_TIM1EN) == RESET) 01446 01447 #define __HAL_RCC_SPI1_IS_CLK_DISABLED() (READ_BIT(RCC->APB2ENR, RCC_APB2ENR_SPI1EN) == RESET) 01448 01449 #define __HAL_RCC_TIM8_IS_CLK_DISABLED() (READ_BIT(RCC->APB2ENR, RCC_APB2ENR_TIM8EN) == RESET) 01450 01451 #define __HAL_RCC_USART1_IS_CLK_DISABLED() (READ_BIT(RCC->APB2ENR, RCC_APB2ENR_USART1EN) == RESET) 01452 01453 #define __HAL_RCC_TIM15_IS_CLK_DISABLED() (READ_BIT(RCC->APB2ENR, RCC_APB2ENR_TIM15EN) == RESET) 01454 01455 #define __HAL_RCC_TIM16_IS_CLK_DISABLED() (READ_BIT(RCC->APB2ENR, RCC_APB2ENR_TIM16EN) == RESET) 01456 01457 #define __HAL_RCC_TIM17_IS_CLK_DISABLED() (READ_BIT(RCC->APB2ENR, RCC_APB2ENR_TIM17EN) == RESET) 01458 01459 #define __HAL_RCC_SAI1_IS_CLK_DISABLED() (READ_BIT(RCC->APB2ENR, RCC_APB2ENR_SAI1EN) == RESET) 01460 01461 #define __HAL_RCC_SAI2_IS_CLK_DISABLED() (READ_BIT(RCC->APB2ENR, RCC_APB2ENR_SAI2EN) == RESET) 01462 01463 #define __HAL_RCC_DFSDM_IS_CLK_DISABLED() (READ_BIT(RCC->APB2ENR, RCC_APB2ENR_DFSDMEN) == RESET) 01464 01465 /** 01466 * @} 01467 */ 01468 01469 /** @defgroup RCC_AHB1_Force_Release_Reset AHB1 Peripheral Force Release Reset 01470 * @brief Force or release AHB1 peripheral reset. 01471 * @{ 01472 */ 01473 #define __HAL_RCC_AHB1_FORCE_RESET() WRITE_REG(RCC->AHB1RSTR, 0xFFFFFFFF) 01474 01475 #define __HAL_RCC_DMA1_FORCE_RESET() SET_BIT(RCC->AHB1RSTR, RCC_AHB1RSTR_DMA1RST) 01476 01477 #define __HAL_RCC_DMA2_FORCE_RESET() SET_BIT(RCC->AHB1RSTR, RCC_AHB1RSTR_DMA2RST) 01478 01479 #define __HAL_RCC_FLASH_FORCE_RESET() SET_BIT(RCC->AHB1RSTR, RCC_AHB1RSTR_FLASHRST) 01480 01481 #define __HAL_RCC_CRC_FORCE_RESET() SET_BIT(RCC->AHB1RSTR, RCC_AHB1RSTR_CRCRST) 01482 01483 #define __HAL_RCC_TSC_FORCE_RESET() SET_BIT(RCC->AHB1RSTR, RCC_AHB1RSTR_TSCRST) 01484 01485 #define __HAL_RCC_AHB1_RELEASE_RESET() WRITE_REG(RCC->AHB1RSTR, 0x00000000) 01486 01487 #define __HAL_RCC_DMA1_RELEASE_RESET() CLEAR_BIT(RCC->AHB1RSTR, RCC_AHB1RSTR_DMA1RST) 01488 01489 #define __HAL_RCC_DMA2_RELEASE_RESET() CLEAR_BIT(RCC->AHB1RSTR, RCC_AHB1RSTR_DMA2RST) 01490 01491 #define __HAL_RCC_FLASH_RELEASE_RESET() CLEAR_BIT(RCC->AHB1RSTR, RCC_AHB1RSTR_FLASHRST) 01492 01493 #define __HAL_RCC_CRC_RELEASE_RESET() CLEAR_BIT(RCC->AHB1RSTR, RCC_AHB1RSTR_CRCRST) 01494 01495 #define __HAL_RCC_TSC_RELEASE_RESET() CLEAR_BIT(RCC->AHB1RSTR, RCC_AHB1RSTR_TSCRST) 01496 01497 /** 01498 * @} 01499 */ 01500 01501 /** @defgroup RCC_AHB2_Force_Release_Reset AHB2 Peripheral Force Release Reset 01502 * @brief Force or release AHB2 peripheral reset. 01503 * @{ 01504 */ 01505 #define __HAL_RCC_AHB2_FORCE_RESET() WRITE_REG(RCC->AHB2RSTR, 0xFFFFFFFF) 01506 01507 #define __HAL_RCC_GPIOA_FORCE_RESET() SET_BIT(RCC->AHB2RSTR, RCC_AHB2RSTR_GPIOARST) 01508 01509 #define __HAL_RCC_GPIOB_FORCE_RESET() SET_BIT(RCC->AHB2RSTR, RCC_AHB2RSTR_GPIOBRST) 01510 01511 #define __HAL_RCC_GPIOC_FORCE_RESET() SET_BIT(RCC->AHB2RSTR, RCC_AHB2RSTR_GPIOCRST) 01512 01513 #define __HAL_RCC_GPIOD_FORCE_RESET() SET_BIT(RCC->AHB2RSTR, RCC_AHB2RSTR_GPIODRST) 01514 01515 #define __HAL_RCC_GPIOE_FORCE_RESET() SET_BIT(RCC->AHB2RSTR, RCC_AHB2RSTR_GPIOERST) 01516 01517 #define __HAL_RCC_GPIOF_FORCE_RESET() SET_BIT(RCC->AHB2RSTR, RCC_AHB2RSTR_GPIOFRST) 01518 01519 #define __HAL_RCC_GPIOG_FORCE_RESET() SET_BIT(RCC->AHB2RSTR, RCC_AHB2RSTR_GPIOGRST) 01520 01521 #define __HAL_RCC_GPIOH_FORCE_RESET() SET_BIT(RCC->AHB2RSTR, RCC_AHB2RSTR_GPIOHRST) 01522 01523 #if defined(STM32L475xx) || defined(STM32L476xx) || defined(STM32L485xx) || defined(STM32L486xx) 01524 #define __HAL_RCC_USB_OTG_FS_FORCE_RESET() SET_BIT(RCC->AHB2RSTR, RCC_AHB2RSTR_OTGFSRST) 01525 #endif /* STM32L475xx || STM32L476xx || STM32L485xx || STM32L486xx */ 01526 01527 #define __HAL_RCC_ADC_FORCE_RESET() SET_BIT(RCC->AHB2RSTR, RCC_AHB2RSTR_ADCRST) 01528 01529 #if defined(STM32L485xx) || defined(STM32L486xx) 01530 #define __HAL_RCC_AES_FORCE_RESET() SET_BIT(RCC->AHB2RSTR, RCC_AHB2RSTR_AESRST) 01531 #endif /* STM32L485xx || STM32L486xx */ 01532 01533 #define __HAL_RCC_RNG_FORCE_RESET() SET_BIT(RCC->AHB2RSTR, RCC_AHB2RSTR_RNGRST) 01534 01535 #define __HAL_RCC_AHB2_RELEASE_RESET() WRITE_REG(RCC->AHB2RSTR, 0x00000000) 01536 01537 #define __HAL_RCC_GPIOA_RELEASE_RESET() CLEAR_BIT(RCC->AHB2RSTR, RCC_AHB2RSTR_GPIOARST) 01538 01539 #define __HAL_RCC_GPIOB_RELEASE_RESET() CLEAR_BIT(RCC->AHB2RSTR, RCC_AHB2RSTR_GPIOBRST) 01540 01541 #define __HAL_RCC_GPIOC_RELEASE_RESET() CLEAR_BIT(RCC->AHB2RSTR, RCC_AHB2RSTR_GPIOCRST) 01542 01543 #define __HAL_RCC_GPIOD_RELEASE_RESET() CLEAR_BIT(RCC->AHB2RSTR, RCC_AHB2RSTR_GPIODRST) 01544 01545 #define __HAL_RCC_GPIOE_RELEASE_RESET() CLEAR_BIT(RCC->AHB2RSTR, RCC_AHB2RSTR_GPIOERST) 01546 01547 #define __HAL_RCC_GPIOF_RELEASE_RESET() CLEAR_BIT(RCC->AHB2RSTR, RCC_AHB2RSTR_GPIOFRST) 01548 01549 #define __HAL_RCC_GPIOG_RELEASE_RESET() CLEAR_BIT(RCC->AHB2RSTR, RCC_AHB2RSTR_GPIOGRST) 01550 01551 #define __HAL_RCC_GPIOH_RELEASE_RESET() CLEAR_BIT(RCC->AHB2RSTR, RCC_AHB2RSTR_GPIOHRST) 01552 01553 #if defined(STM32L475xx) || defined(STM32L476xx) || defined(STM32L485xx) || defined(STM32L486xx) 01554 #define __HAL_RCC_USB_OTG_FS_RELEASE_RESET() CLEAR_BIT(RCC->AHB2RSTR, RCC_AHB2RSTR_OTGFSRST) 01555 #endif /* STM32L475xx || STM32L476xx || STM32L485xx || STM32L486xx */ 01556 01557 #define __HAL_RCC_ADC_RELEASE_RESET() CLEAR_BIT(RCC->AHB2RSTR, RCC_AHB2RSTR_ADCRST) 01558 01559 #if defined(STM32L485xx) || defined(STM32L486xx) 01560 #define __HAL_RCC_AES_RELEASE_RESET() CLEAR_BIT(RCC->AHB2RSTR, RCC_AHB2RSTR_AESRST) 01561 #endif /* STM32L485xx || STM32L486xx */ 01562 01563 #define __HAL_RCC_RNG_RELEASE_RESET() CLEAR_BIT(RCC->AHB2RSTR, RCC_AHB2RSTR_RNGRST) 01564 01565 /** 01566 * @} 01567 */ 01568 01569 /** @defgroup RCC_AHB3_Force_Release_Reset AHB3 Peripheral Force Release Reset 01570 * @brief Force or release AHB3 peripheral reset. 01571 * @{ 01572 */ 01573 #define __HAL_RCC_AHB3_FORCE_RESET() WRITE_REG(RCC->AHB3RSTR, 0xFFFFFFFF) 01574 01575 #define __HAL_RCC_FMC_FORCE_RESET() SET_BIT(RCC->AHB3RSTR, RCC_AHB3RSTR_FMCRST) 01576 01577 #define __HAL_RCC_QSPI_FORCE_RESET() SET_BIT(RCC->AHB3RSTR, RCC_AHB3RSTR_QSPIRST) 01578 01579 #define __HAL_RCC_AHB3_RELEASE_RESET() WRITE_REG(RCC->AHB3RSTR, 0x00000000) 01580 01581 #define __HAL_RCC_FMC_RELEASE_RESET() CLEAR_BIT(RCC->AHB3RSTR, RCC_AHB3RSTR_FMCRST) 01582 01583 #define __HAL_RCC_QSPI_RELEASE_RESET() CLEAR_BIT(RCC->AHB3RSTR, RCC_AHB3RSTR_QSPIRST) 01584 01585 /** 01586 * @} 01587 */ 01588 01589 /** @defgroup RCC_APB1_Force_Release_Reset APB1 Peripheral Force Release Reset 01590 * @brief Force or release APB1 peripheral reset. 01591 * @{ 01592 */ 01593 #define __HAL_RCC_APB1_FORCE_RESET() WRITE_REG(RCC->APB1RSTR1, 0xFFFFFFFF) 01594 01595 #define __HAL_RCC_TIM2_FORCE_RESET() SET_BIT(RCC->APB1RSTR1, RCC_APB1RSTR1_TIM2RST) 01596 01597 #define __HAL_RCC_TIM3_FORCE_RESET() SET_BIT(RCC->APB1RSTR1, RCC_APB1RSTR1_TIM3RST) 01598 01599 #define __HAL_RCC_TIM4_FORCE_RESET() SET_BIT(RCC->APB1RSTR1, RCC_APB1RSTR1_TIM4RST) 01600 01601 #define __HAL_RCC_TIM5_FORCE_RESET() SET_BIT(RCC->APB1RSTR1, RCC_APB1RSTR1_TIM5RST) 01602 01603 #define __HAL_RCC_TIM6_FORCE_RESET() SET_BIT(RCC->APB1RSTR1, RCC_APB1RSTR1_TIM6RST) 01604 01605 #define __HAL_RCC_TIM7_FORCE_RESET() SET_BIT(RCC->APB1RSTR1, RCC_APB1RSTR1_TIM7RST) 01606 01607 #if defined(STM32L476xx) || defined(STM32L486xx) 01608 #define __HAL_RCC_LCD_FORCE_RESET() SET_BIT(RCC->APB1RSTR1, RCC_APB1RSTR1_LCDRST) 01609 #endif /* STM32L476xx || STM32L486xx */ 01610 01611 #define __HAL_RCC_SPI2_FORCE_RESET() SET_BIT(RCC->APB1RSTR1, RCC_APB1RSTR1_SPI2RST) 01612 01613 #define __HAL_RCC_SPI3_FORCE_RESET() SET_BIT(RCC->APB1RSTR1, RCC_APB1RSTR1_SPI3RST) 01614 01615 #define __HAL_RCC_USART2_FORCE_RESET() SET_BIT(RCC->APB1RSTR1, RCC_APB1RSTR1_USART2RST) 01616 01617 #define __HAL_RCC_USART3_FORCE_RESET() SET_BIT(RCC->APB1RSTR1, RCC_APB1RSTR1_USART3RST) 01618 01619 #define __HAL_RCC_UART4_FORCE_RESET() SET_BIT(RCC->APB1RSTR1, RCC_APB1RSTR1_UART4RST) 01620 01621 #define __HAL_RCC_UART5_FORCE_RESET() SET_BIT(RCC->APB1RSTR1, RCC_APB1RSTR1_UART5RST) 01622 01623 #define __HAL_RCC_I2C1_FORCE_RESET() SET_BIT(RCC->APB1RSTR1, RCC_APB1RSTR1_I2C1RST) 01624 01625 #define __HAL_RCC_I2C2_FORCE_RESET() SET_BIT(RCC->APB1RSTR1, RCC_APB1RSTR1_I2C2RST) 01626 01627 #define __HAL_RCC_I2C3_FORCE_RESET() SET_BIT(RCC->APB1RSTR1, RCC_APB1RSTR1_I2C3RST) 01628 01629 #define __HAL_RCC_CAN1_FORCE_RESET() SET_BIT(RCC->APB1RSTR1, RCC_APB1RSTR1_CAN1RST) 01630 01631 #define __HAL_RCC_PWR_FORCE_RESET() SET_BIT(RCC->APB1RSTR1, RCC_APB1RSTR1_PWRRST) 01632 01633 #define __HAL_RCC_DAC1_FORCE_RESET() SET_BIT(RCC->APB1RSTR1, RCC_APB1RSTR1_DAC1RST) 01634 01635 #define __HAL_RCC_OPAMP_FORCE_RESET() SET_BIT(RCC->APB1RSTR1, RCC_APB1RSTR1_OPAMPRST) 01636 01637 #define __HAL_RCC_LPTIM1_FORCE_RESET() SET_BIT(RCC->APB1RSTR1, RCC_APB1RSTR1_LPTIM1RST) 01638 01639 #define __HAL_RCC_LPUART1_FORCE_RESET() SET_BIT(RCC->APB1RSTR2, RCC_APB1RSTR2_LPUART1RST) 01640 01641 #define __HAL_RCC_SWPMI1_FORCE_RESET() SET_BIT(RCC->APB1RSTR2, RCC_APB1RSTR2_SWPMI1RST) 01642 01643 #define __HAL_RCC_LPTIM2_FORCE_RESET() SET_BIT(RCC->APB1RSTR2, RCC_APB1RSTR2_LPTIM2RST) 01644 01645 #define __HAL_RCC_APB1_RELEASE_RESET() WRITE_REG(RCC->APB1RSTR1, 0x00000000) 01646 01647 #define __HAL_RCC_TIM2_RELEASE_RESET() CLEAR_BIT(RCC->APB1RSTR1, RCC_APB1RSTR1_TIM2RST) 01648 01649 #define __HAL_RCC_TIM3_RELEASE_RESET() CLEAR_BIT(RCC->APB1RSTR1, RCC_APB1RSTR1_TIM3RST) 01650 01651 #define __HAL_RCC_TIM4_RELEASE_RESET() CLEAR_BIT(RCC->APB1RSTR1, RCC_APB1RSTR1_TIM4RST) 01652 01653 #define __HAL_RCC_TIM5_RELEASE_RESET() CLEAR_BIT(RCC->APB1RSTR1, RCC_APB1RSTR1_TIM5RST) 01654 01655 #define __HAL_RCC_TIM6_RELEASE_RESET() CLEAR_BIT(RCC->APB1RSTR1, RCC_APB1RSTR1_TIM6RST) 01656 01657 #define __HAL_RCC_TIM7_RELEASE_RESET() CLEAR_BIT(RCC->APB1RSTR1, RCC_APB1RSTR1_TIM7RST) 01658 01659 #if defined(STM32L476xx) || defined(STM32L486xx) 01660 #define __HAL_RCC_LCD_RELEASE_RESET() CLEAR_BIT(RCC->APB1RSTR1, RCC_APB1RSTR1_LCDRST) 01661 #endif /* STM32L476xx || STM32L486xx */ 01662 01663 #define __HAL_RCC_SPI2_RELEASE_RESET() CLEAR_BIT(RCC->APB1RSTR1, RCC_APB1RSTR1_SPI2RST) 01664 01665 #define __HAL_RCC_SPI3_RELEASE_RESET() CLEAR_BIT(RCC->APB1RSTR1, RCC_APB1RSTR1_SPI3RST) 01666 01667 #define __HAL_RCC_USART2_RELEASE_RESET() CLEAR_BIT(RCC->APB1RSTR1, RCC_APB1RSTR1_USART2RST) 01668 01669 #define __HAL_RCC_USART3_RELEASE_RESET() CLEAR_BIT(RCC->APB1RSTR1, RCC_APB1RSTR1_USART3RST) 01670 01671 #define __HAL_RCC_UART4_RELEASE_RESET() CLEAR_BIT(RCC->APB1RSTR1, RCC_APB1RSTR1_UART4RST) 01672 01673 #define __HAL_RCC_UART5_RELEASE_RESET() CLEAR_BIT(RCC->APB1RSTR1, RCC_APB1RSTR1_UART5RST) 01674 01675 #define __HAL_RCC_I2C1_RELEASE_RESET() CLEAR_BIT(RCC->APB1RSTR1, RCC_APB1RSTR1_I2C1RST) 01676 01677 #define __HAL_RCC_I2C2_RELEASE_RESET() CLEAR_BIT(RCC->APB1RSTR1, RCC_APB1RSTR1_I2C2RST) 01678 01679 #define __HAL_RCC_I2C3_RELEASE_RESET() CLEAR_BIT(RCC->APB1RSTR1, RCC_APB1RSTR1_I2C3RST) 01680 01681 #define __HAL_RCC_CAN1_RELEASE_RESET() CLEAR_BIT(RCC->APB1RSTR1, RCC_APB1RSTR1_CAN1RST) 01682 01683 #define __HAL_RCC_PWR_RELEASE_RESET() CLEAR_BIT(RCC->APB1RSTR1, RCC_APB1RSTR1_PWRRST) 01684 01685 #define __HAL_RCC_DAC1_RELEASE_RESET() CLEAR_BIT(RCC->APB1RSTR1, RCC_APB1RSTR1_DAC1RST) 01686 01687 #define __HAL_RCC_OPAMP_RELEASE_RESET() CLEAR_BIT(RCC->APB1RSTR1, RCC_APB1RSTR1_OPAMPRST) 01688 01689 #define __HAL_RCC_LPTIM1_RELEASE_RESET() CLEAR_BIT(RCC->APB1RSTR1, RCC_APB1RSTR1_LPTIM1RST) 01690 01691 #define __HAL_RCC_LPUART1_RELEASE_RESET() CLEAR_BIT(RCC->APB1RSTR2, RCC_APB1RSTR2_LPUART1RST) 01692 01693 #define __HAL_RCC_SWPMI1_RELEASE_RESET() CLEAR_BIT(RCC->APB1RSTR2, RCC_APB1RSTR2_SWPMI1RST) 01694 01695 #define __HAL_RCC_LPTIM2_RELEASE_RESET() CLEAR_BIT(RCC->APB1RSTR2, RCC_APB1RSTR2_LPTIM2RST) 01696 01697 /** 01698 * @} 01699 */ 01700 01701 /** @defgroup RCC_APB2_Force_Release_Reset APB2 Peripheral Force Release Reset 01702 * @brief Force or release APB2 peripheral reset. 01703 * @{ 01704 */ 01705 #define __HAL_RCC_APB2_FORCE_RESET() WRITE_REG(RCC->APB2RSTR, 0xFFFFFFFF) 01706 01707 #define __HAL_RCC_SYSCFG_FORCE_RESET() SET_BIT(RCC->APB2RSTR, RCC_APB2RSTR_SYSCFGRST) 01708 01709 #define __HAL_RCC_SDMMC1_FORCE_RESET() SET_BIT(RCC->APB2RSTR, RCC_APB2RSTR_SDMMC1RST) 01710 01711 #define __HAL_RCC_TIM1_FORCE_RESET() SET_BIT(RCC->APB2RSTR, RCC_APB2RSTR_TIM1RST) 01712 01713 #define __HAL_RCC_SPI1_FORCE_RESET() SET_BIT(RCC->APB2RSTR, RCC_APB2RSTR_SPI1RST) 01714 01715 #define __HAL_RCC_TIM8_FORCE_RESET() SET_BIT(RCC->APB2RSTR, RCC_APB2RSTR_TIM8RST) 01716 01717 #define __HAL_RCC_USART1_FORCE_RESET() SET_BIT(RCC->APB2RSTR, RCC_APB2RSTR_USART1RST) 01718 01719 #define __HAL_RCC_TIM15_FORCE_RESET() SET_BIT(RCC->APB2RSTR, RCC_APB2RSTR_TIM15RST) 01720 01721 #define __HAL_RCC_TIM16_FORCE_RESET() SET_BIT(RCC->APB2RSTR, RCC_APB2RSTR_TIM16RST) 01722 01723 #define __HAL_RCC_TIM17_FORCE_RESET() SET_BIT(RCC->APB2RSTR, RCC_APB2RSTR_TIM17RST) 01724 01725 #define __HAL_RCC_SAI1_FORCE_RESET() SET_BIT(RCC->APB2RSTR, RCC_APB2RSTR_SAI1RST) 01726 01727 #define __HAL_RCC_SAI2_FORCE_RESET() SET_BIT(RCC->APB2RSTR, RCC_APB2RSTR_SAI2RST) 01728 01729 #define __HAL_RCC_DFSDM_FORCE_RESET() SET_BIT(RCC->APB2RSTR, RCC_APB2RSTR_DFSDMRST) 01730 01731 #define __HAL_RCC_APB2_RELEASE_RESET() WRITE_REG(RCC->APB2RSTR, 0x00000000) 01732 01733 #define __HAL_RCC_SYSCFG_RELEASE_RESET() CLEAR_BIT(RCC->APB2RSTR, RCC_APB2RSTR_SYSCFGRST) 01734 01735 #define __HAL_RCC_SDMMC1_RELEASE_RESET() CLEAR_BIT(RCC->APB2RSTR, RCC_APB2RSTR_SDMMC1RST) 01736 01737 #define __HAL_RCC_TIM1_RELEASE_RESET() CLEAR_BIT(RCC->APB2RSTR, RCC_APB2RSTR_TIM1RST) 01738 01739 #define __HAL_RCC_SPI1_RELEASE_RESET() CLEAR_BIT(RCC->APB2RSTR, RCC_APB2RSTR_SPI1RST) 01740 01741 #define __HAL_RCC_TIM8_RELEASE_RESET() CLEAR_BIT(RCC->APB2RSTR, RCC_APB2RSTR_TIM8RST) 01742 01743 #define __HAL_RCC_USART1_RELEASE_RESET() CLEAR_BIT(RCC->APB2RSTR, RCC_APB2RSTR_USART1RST) 01744 01745 #define __HAL_RCC_TIM15_RELEASE_RESET() CLEAR_BIT(RCC->APB2RSTR, RCC_APB2RSTR_TIM15RST) 01746 01747 #define __HAL_RCC_TIM16_RELEASE_RESET() CLEAR_BIT(RCC->APB2RSTR, RCC_APB2RSTR_TIM16RST) 01748 01749 #define __HAL_RCC_TIM17_RELEASE_RESET() CLEAR_BIT(RCC->APB2RSTR, RCC_APB2RSTR_TIM17RST) 01750 01751 #define __HAL_RCC_SAI1_RELEASE_RESET() CLEAR_BIT(RCC->APB2RSTR, RCC_APB2RSTR_SAI1RST) 01752 01753 #define __HAL_RCC_SAI2_RELEASE_RESET() CLEAR_BIT(RCC->APB2RSTR, RCC_APB2RSTR_SAI2RST) 01754 01755 #define __HAL_RCC_DFSDM_RELEASE_RESET() CLEAR_BIT(RCC->APB2RSTR, RCC_APB2RSTR_DFSDMRST) 01756 01757 /** 01758 * @} 01759 */ 01760 01761 /** @defgroup RCC_AHB1_Clock_Sleep_Enable_Disable AHB1 Peripheral Clock Sleep Enable Disable 01762 * @brief Enable or disable the AHB1 peripheral clock during Low Power (Sleep) mode. 01763 * @note Peripheral clock gating in SLEEP mode can be used to further reduce 01764 * power consumption. 01765 * @note After wakeup from SLEEP mode, the peripheral clock is enabled again. 01766 * @note By default, all peripheral clocks are enabled during SLEEP mode. 01767 * @{ 01768 */ 01769 01770 #define __HAL_RCC_DMA1_CLK_SLEEP_ENABLE() SET_BIT(RCC->AHB1SMENR, RCC_AHB1SMENR_DMA1SMEN) 01771 01772 #define __HAL_RCC_DMA2_CLK_SLEEP_ENABLE() SET_BIT(RCC->AHB1SMENR, RCC_AHB1SMENR_DMA2SMEN) 01773 01774 #define __HAL_RCC_FLASH_CLK_SLEEP_ENABLE() SET_BIT(RCC->AHB1SMENR, RCC_AHB1SMENR_FLASHSMEN) 01775 01776 #define __HAL_RCC_SRAM1_CLK_SLEEP_ENABLE() SET_BIT(RCC->AHB1SMENR, RCC_AHB1SMENR_SRAM1SMEN) 01777 01778 #define __HAL_RCC_CRC_CLK_SLEEP_ENABLE() SET_BIT(RCC->AHB1SMENR, RCC_AHB1SMENR_CRCSMEN) 01779 01780 #define __HAL_RCC_TSC_CLK_SLEEP_ENABLE() SET_BIT(RCC->AHB1SMENR, RCC_AHB1SMENR_TSCSMEN) 01781 01782 #define __HAL_RCC_DMA1_CLK_SLEEP_DISABLE() CLEAR_BIT(RCC->AHB1SMENR, RCC_AHB1SMENR_DMA1SMEN) 01783 01784 #define __HAL_RCC_DMA2_CLK_SLEEP_DISABLE() CLEAR_BIT(RCC->AHB1SMENR, RCC_AHB1SMENR_DMA2SMEN) 01785 01786 #define __HAL_RCC_FLASH_CLK_SLEEP_DISABLE() CLEAR_BIT(RCC->AHB1SMENR, RCC_AHB1SMENR_FLASHSMEN) 01787 01788 #define __HAL_RCC_SRAM1_CLK_SLEEP_DISABLE() CLEAR_BIT(RCC->AHB1SMENR, RCC_AHB1SMENR_SRAM1SMEN) 01789 01790 #define __HAL_RCC_CRC_CLK_SLEEP_DISABLE() CLEAR_BIT(RCC->AHB1SMENR, RCC_AHB1SMENR_CRCSMEN) 01791 01792 #define __HAL_RCC_TSC_CLK_SLEEP_DISABLE() CLEAR_BIT(RCC->AHB1SMENR, RCC_AHB1SMENR_TSCSMEN) 01793 01794 /** 01795 * @} 01796 */ 01797 01798 /** @defgroup RCC_AHB2_Clock_Sleep_Enable_Disable AHB2 Peripheral Clock Sleep Enable Disable 01799 * @brief Enable or disable the AHB2 peripheral clock during Low Power (Sleep) mode. 01800 * @note Peripheral clock gating in SLEEP mode can be used to further reduce 01801 * power consumption. 01802 * @note After wakeup from SLEEP mode, the peripheral clock is enabled again. 01803 * @note By default, all peripheral clocks are enabled during SLEEP mode. 01804 * @{ 01805 */ 01806 01807 #define __HAL_RCC_GPIOA_CLK_SLEEP_ENABLE() SET_BIT(RCC->AHB2SMENR, RCC_AHB2SMENR_GPIOASMEN) 01808 01809 #define __HAL_RCC_GPIOB_CLK_SLEEP_ENABLE() SET_BIT(RCC->AHB2SMENR, RCC_AHB2SMENR_GPIOBSMEN) 01810 01811 #define __HAL_RCC_GPIOC_CLK_SLEEP_ENABLE() SET_BIT(RCC->AHB2SMENR, RCC_AHB2SMENR_GPIOCSMEN) 01812 01813 #define __HAL_RCC_GPIOD_CLK_SLEEP_ENABLE() SET_BIT(RCC->AHB2SMENR, RCC_AHB2SMENR_GPIODSMEN) 01814 01815 #define __HAL_RCC_GPIOE_CLK_SLEEP_ENABLE() SET_BIT(RCC->AHB2SMENR, RCC_AHB2SMENR_GPIOESMEN) 01816 01817 #define __HAL_RCC_GPIOF_CLK_SLEEP_ENABLE() SET_BIT(RCC->AHB2SMENR, RCC_AHB2SMENR_GPIOFSMEN) 01818 01819 #define __HAL_RCC_GPIOG_CLK_SLEEP_ENABLE() SET_BIT(RCC->AHB2SMENR, RCC_AHB2SMENR_GPIOGSMEN) 01820 01821 #define __HAL_RCC_GPIOH_CLK_SLEEP_ENABLE() SET_BIT(RCC->AHB2SMENR, RCC_AHB2SMENR_GPIOHSMEN) 01822 01823 #define __HAL_RCC_SRAM2_CLK_SLEEP_ENABLE() SET_BIT(RCC->AHB2SMENR, RCC_AHB2SMENR_SRAM2SMEN) 01824 01825 #if defined(STM32L475xx) || defined(STM32L476xx) || defined(STM32L485xx) || defined(STM32L486xx) 01826 #define __HAL_RCC_USB_OTG_FS_CLK_SLEEP_ENABLE() SET_BIT(RCC->AHB2SMENR, RCC_AHB2SMENR_OTGFSSMEN) 01827 #endif /* STM32L475xx || STM32L476xx || STM32L485xx || STM32L486xx */ 01828 01829 #define __HAL_RCC_ADC_CLK_SLEEP_ENABLE() SET_BIT(RCC->AHB2SMENR, RCC_AHB2SMENR_ADCSMEN) 01830 01831 #if defined(STM32L485xx) || defined(STM32L486xx) 01832 #define __HAL_RCC_AES_CLK_SLEEP_ENABLE() SET_BIT(RCC->AHB2SMENR, RCC_AHB2SMENR_AESSMEN) 01833 #endif /* STM32L485xx || STM32L486xx */ 01834 01835 #define __HAL_RCC_RNG_CLK_SLEEP_ENABLE() SET_BIT(RCC->AHB2SMENR, RCC_AHB2SMENR_RNGSMEN) 01836 01837 #define __HAL_RCC_GPIOA_CLK_SLEEP_DISABLE() CLEAR_BIT(RCC->AHB2SMENR, RCC_AHB2SMENR_GPIOASMEN) 01838 01839 #define __HAL_RCC_GPIOB_CLK_SLEEP_DISABLE() CLEAR_BIT(RCC->AHB2SMENR, RCC_AHB2SMENR_GPIOBSMEN) 01840 01841 #define __HAL_RCC_GPIOC_CLK_SLEEP_DISABLE() CLEAR_BIT(RCC->AHB2SMENR, RCC_AHB2SMENR_GPIOCSMEN) 01842 01843 #define __HAL_RCC_GPIOD_CLK_SLEEP_DISABLE() CLEAR_BIT(RCC->AHB2SMENR, RCC_AHB2SMENR_GPIODSMEN) 01844 01845 #define __HAL_RCC_GPIOE_CLK_SLEEP_DISABLE() CLEAR_BIT(RCC->AHB2SMENR, RCC_AHB2SMENR_GPIOESMEN) 01846 01847 #define __HAL_RCC_GPIOF_CLK_SLEEP_DISABLE() CLEAR_BIT(RCC->AHB2SMENR, RCC_AHB2SMENR_GPIOFSMEN) 01848 01849 #define __HAL_RCC_GPIOG_CLK_SLEEP_DISABLE() CLEAR_BIT(RCC->AHB2SMENR, RCC_AHB2SMENR_GPIOGSMEN) 01850 01851 #define __HAL_RCC_GPIOH_CLK_SLEEP_DISABLE() CLEAR_BIT(RCC->AHB2SMENR, RCC_AHB2SMENR_GPIOHSMEN) 01852 01853 #define __HAL_RCC_SRAM2_CLK_SLEEP_DISABLE() CLEAR_BIT(RCC->AHB2SMENR, RCC_AHB2SMENR_SRAM2SMEN) 01854 01855 #if defined(STM32L475xx) || defined(STM32L476xx) || defined(STM32L485xx) || defined(STM32L486xx) 01856 #define __HAL_RCC_USB_OTG_FS_CLK_SLEEP_DISABLE() CLEAR_BIT(RCC->AHB2SMENR, RCC_AHB2SMENR_OTGFSSMEN) 01857 #endif /* STM32L475xx || STM32L476xx || STM32L485xx || STM32L486xx */ 01858 01859 #define __HAL_RCC_ADC_CLK_SLEEP_DISABLE() CLEAR_BIT(RCC->AHB2SMENR, RCC_AHB2SMENR_ADCSMEN) 01860 01861 #if defined(STM32L485xx) || defined(STM32L486xx) 01862 #define __HAL_RCC_AES_CLK_SLEEP_DISABLE() CLEAR_BIT(RCC->AHB2SMENR, RCC_AHB2SMENR_AESSMEN) 01863 #endif /* STM32L485xx || STM32L486xx */ 01864 01865 #define __HAL_RCC_RNG_CLK_SLEEP_DISABLE() CLEAR_BIT(RCC->AHB2SMENR, RCC_AHB2SMENR_RNGSMEN) 01866 01867 /** 01868 * @} 01869 */ 01870 01871 /** @defgroup RCC_AHB3_Clock_Sleep_Enable_Disable AHB3 Peripheral Clock Sleep Enable Disable 01872 * @brief Enable or disable the AHB3 peripheral clock during Low Power (Sleep) mode. 01873 * @note Peripheral clock gating in SLEEP mode can be used to further reduce 01874 * power consumption. 01875 * @note After wakeup from SLEEP mode, the peripheral clock is enabled again. 01876 * @note By default, all peripheral clocks are enabled during SLEEP mode. 01877 * @{ 01878 */ 01879 01880 #define __HAL_RCC_QSPI_CLK_SLEEP_ENABLE() SET_BIT(RCC->AHB3SMENR, RCC_AHB3SMENR_QSPISMEN) 01881 01882 #define __HAL_RCC_FMC_CLK_SLEEP_ENABLE() SET_BIT(RCC->AHB3SMENR, RCC_AHB3SMENR_FMCSMEN) 01883 01884 #define __HAL_RCC_QSPI_CLK_SLEEP_DISABLE() CLEAR_BIT(RCC->AHB3SMENR, RCC_AHB3SMENR_QSPISMEN) 01885 01886 #define __HAL_RCC_FMC_CLK_SLEEP_DISABLE() CLEAR_BIT(RCC->AHB3SMENR, RCC_AHB3SMENR_FMCSMEN) 01887 01888 /** 01889 * @} 01890 */ 01891 01892 /** @defgroup RCC_APB1_Clock_Sleep_Enable_Disable APB1 Peripheral Clock Sleep Enable Disable 01893 * @brief Enable or disable the APB1 peripheral clock during Low Power (Sleep) mode. 01894 * @note Peripheral clock gating in SLEEP mode can be used to further reduce 01895 * power consumption. 01896 * @note After wakeup from SLEEP mode, the peripheral clock is enabled again. 01897 * @note By default, all peripheral clocks are enabled during SLEEP mode. 01898 * @{ 01899 */ 01900 01901 #define __HAL_RCC_TIM2_CLK_SLEEP_ENABLE() SET_BIT(RCC->APB1SMENR1, RCC_APB1SMENR1_TIM2SMEN) 01902 01903 #define __HAL_RCC_TIM3_CLK_SLEEP_ENABLE() SET_BIT(RCC->APB1SMENR1, RCC_APB1SMENR1_TIM3SMEN) 01904 01905 #define __HAL_RCC_TIM4_CLK_SLEEP_ENABLE() SET_BIT(RCC->APB1SMENR1, RCC_APB1SMENR1_TIM4SMEN) 01906 01907 #define __HAL_RCC_TIM5_CLK_SLEEP_ENABLE() SET_BIT(RCC->APB1SMENR1, RCC_APB1SMENR1_TIM5SMEN) 01908 01909 #define __HAL_RCC_TIM6_CLK_SLEEP_ENABLE() SET_BIT(RCC->APB1SMENR1, RCC_APB1SMENR1_TIM6SMEN) 01910 01911 #define __HAL_RCC_TIM7_CLK_SLEEP_ENABLE() SET_BIT(RCC->APB1SMENR1, RCC_APB1SMENR1_TIM7SMEN) 01912 01913 #if defined(STM32L476xx) || defined(STM32L486xx) 01914 #define __HAL_RCC_LCD_CLK_SLEEP_ENABLE() SET_BIT(RCC->APB1SMENR1, RCC_APB1SMENR1_LCDSMEN) 01915 #endif /* STM32L476xx || STM32L486xx */ 01916 01917 #define __HAL_RCC_WWDG_CLK_SLEEP_ENABLE() SET_BIT(RCC->APB1SMENR1, RCC_APB1SMENR1_WWDGSMEN) 01918 01919 #define __HAL_RCC_SPI2_CLK_SLEEP_ENABLE() SET_BIT(RCC->APB1SMENR1, RCC_APB1SMENR1_SPI2SMEN) 01920 01921 #define __HAL_RCC_SPI3_CLK_SLEEP_ENABLE() SET_BIT(RCC->APB1SMENR1, RCC_APB1SMENR1_SPI3SMEN) 01922 01923 #define __HAL_RCC_USART2_CLK_SLEEP_ENABLE() SET_BIT(RCC->APB1SMENR1, RCC_APB1SMENR1_USART2SMEN) 01924 01925 #define __HAL_RCC_USART3_CLK_SLEEP_ENABLE() SET_BIT(RCC->APB1SMENR1, RCC_APB1SMENR1_USART3SMEN) 01926 01927 #define __HAL_RCC_UART4_CLK_SLEEP_ENABLE() SET_BIT(RCC->APB1SMENR1, RCC_APB1SMENR1_UART4SMEN) 01928 01929 #define __HAL_RCC_UART5_CLK_SLEEP_ENABLE() SET_BIT(RCC->APB1SMENR1, RCC_APB1SMENR1_UART5SMEN) 01930 01931 #define __HAL_RCC_I2C1_CLK_SLEEP_ENABLE() SET_BIT(RCC->APB1SMENR1, RCC_APB1SMENR1_I2C1SMEN) 01932 01933 #define __HAL_RCC_I2C2_CLK_SLEEP_ENABLE() SET_BIT(RCC->APB1SMENR1, RCC_APB1SMENR1_I2C2SMEN) 01934 01935 #define __HAL_RCC_I2C3_CLK_SLEEP_ENABLE() SET_BIT(RCC->APB1SMENR1, RCC_APB1SMENR1_I2C3SMEN) 01936 01937 #define __HAL_RCC_CAN1_CLK_SLEEP_ENABLE() SET_BIT(RCC->APB1SMENR1, RCC_APB1SMENR1_CAN1SMEN) 01938 01939 #define __HAL_RCC_PWR_CLK_SLEEP_ENABLE() SET_BIT(RCC->APB1SMENR1, RCC_APB1SMENR1_PWRSMEN) 01940 01941 #define __HAL_RCC_DAC1_CLK_SLEEP_ENABLE() SET_BIT(RCC->APB1SMENR1, RCC_APB1SMENR1_DAC1SMEN) 01942 01943 #define __HAL_RCC_OPAMP_CLK_SLEEP_ENABLE() SET_BIT(RCC->APB1SMENR1, RCC_APB1SMENR1_OPAMPSMEN) 01944 01945 #define __HAL_RCC_LPTIM1_CLK_SLEEP_ENABLE() SET_BIT(RCC->APB1SMENR1, RCC_APB1SMENR1_LPTIM1SMEN) 01946 01947 #define __HAL_RCC_LPUART1_CLK_SLEEP_ENABLE() SET_BIT(RCC->APB1SMENR2, RCC_APB1SMENR2_LPUART1SMEN) 01948 01949 #define __HAL_RCC_SWPMI1_CLK_SLEEP_ENABLE() SET_BIT(RCC->APB1SMENR2, RCC_APB1SMENR2_SWPMI1SMEN) 01950 01951 #define __HAL_RCC_LPTIM2_CLK_SLEEP_ENABLE() SET_BIT(RCC->APB1SMENR2, RCC_APB1SMENR2_LPTIM2SMEN) 01952 01953 #define __HAL_RCC_TIM2_CLK_SLEEP_DISABLE() CLEAR_BIT(RCC->APB1SMENR1, RCC_APB1SMENR1_TIM2SMEN) 01954 01955 #define __HAL_RCC_TIM3_CLK_SLEEP_DISABLE() CLEAR_BIT(RCC->APB1SMENR1, RCC_APB1SMENR1_TIM3SMEN) 01956 01957 #define __HAL_RCC_TIM4_CLK_SLEEP_DISABLE() CLEAR_BIT(RCC->APB1SMENR1, RCC_APB1SMENR1_TIM4SMEN) 01958 01959 #define __HAL_RCC_TIM5_CLK_SLEEP_DISABLE() CLEAR_BIT(RCC->APB1SMENR1, RCC_APB1SMENR1_TIM5SMEN) 01960 01961 #define __HAL_RCC_TIM6_CLK_SLEEP_DISABLE() CLEAR_BIT(RCC->APB1SMENR1, RCC_APB1SMENR1_TIM6SMEN) 01962 01963 #define __HAL_RCC_TIM7_CLK_SLEEP_DISABLE() CLEAR_BIT(RCC->APB1SMENR1, RCC_APB1SMENR1_TIM7SMEN) 01964 01965 #if defined(STM32L476xx) || defined(STM32L486xx) 01966 #define __HAL_RCC_LCD_CLK_SLEEP_DISABLE() CLEAR_BIT(RCC->APB1SMENR1, RCC_APB1SMENR1_LCDSMEN) 01967 #endif /* STM32L476xx || STM32L486xx */ 01968 01969 #define __HAL_RCC_WWDG_CLK_SLEEP_DISABLE() CLEAR_BIT(RCC->APB1SMENR1, RCC_APB1SMENR1_WWDGSMEN) 01970 01971 #define __HAL_RCC_SPI2_CLK_SLEEP_DISABLE() CLEAR_BIT(RCC->APB1SMENR1, RCC_APB1SMENR1_SPI2SMEN) 01972 01973 #define __HAL_RCC_SPI3_CLK_SLEEP_DISABLE() CLEAR_BIT(RCC->APB1SMENR1, RCC_APB1SMENR1_SPI3SMEN) 01974 01975 #define __HAL_RCC_USART2_CLK_SLEEP_DISABLE() CLEAR_BIT(RCC->APB1SMENR1, RCC_APB1SMENR1_USART2SMEN) 01976 01977 #define __HAL_RCC_USART3_CLK_SLEEP_DISABLE() CLEAR_BIT(RCC->APB1SMENR1, RCC_APB1SMENR1_USART3SMEN) 01978 01979 #define __HAL_RCC_UART4_CLK_SLEEP_DISABLE() CLEAR_BIT(RCC->APB1SMENR1, RCC_APB1SMENR1_UART4SMEN) 01980 01981 #define __HAL_RCC_UART5_CLK_SLEEP_DISABLE() CLEAR_BIT(RCC->APB1SMENR1, RCC_APB1SMENR1_UART5SMEN) 01982 01983 #define __HAL_RCC_I2C1_CLK_SLEEP_DISABLE() CLEAR_BIT(RCC->APB1SMENR1, RCC_APB1SMENR1_I2C1SMEN) 01984 01985 #define __HAL_RCC_I2C2_CLK_SLEEP_DISABLE() CLEAR_BIT(RCC->APB1SMENR1, RCC_APB1SMENR1_I2C2SMEN) 01986 01987 #define __HAL_RCC_I2C3_CLK_SLEEP_DISABLE() CLEAR_BIT(RCC->APB1SMENR1, RCC_APB1SMENR1_I2C3SMEN) 01988 01989 #define __HAL_RCC_CAN1_CLK_SLEEP_DISABLE() CLEAR_BIT(RCC->APB1SMENR1, RCC_APB1SMENR1_CAN1SMEN) 01990 01991 #define __HAL_RCC_PWR_CLK_SLEEP_DISABLE() CLEAR_BIT(RCC->APB1SMENR1, RCC_APB1SMENR1_PWRSMEN) 01992 01993 #define __HAL_RCC_DAC1_CLK_SLEEP_DISABLE() CLEAR_BIT(RCC->APB1SMENR1, RCC_APB1SMENR1_DAC1SMEN) 01994 01995 #define __HAL_RCC_OPAMP_CLK_SLEEP_DISABLE() CLEAR_BIT(RCC->APB1SMENR1, RCC_APB1SMENR1_OPAMPSMEN) 01996 01997 #define __HAL_RCC_LPTIM1_CLK_SLEEP_DISABLE() CLEAR_BIT(RCC->APB1SMENR1, RCC_APB1SMENR1_LPTIM1SMEN) 01998 01999 #define __HAL_RCC_LPUART1_CLK_SLEEP_DISABLE() CLEAR_BIT(RCC->APB1SMENR2, RCC_APB1SMENR2_LPUART1SMEN) 02000 02001 #define __HAL_RCC_SWPMI1_CLK_SLEEP_DISABLE() CLEAR_BIT(RCC->APB1SMENR2, RCC_APB1SMENR2_SWPMI1SMEN) 02002 02003 #define __HAL_RCC_LPTIM2_CLK_SLEEP_DISABLE() CLEAR_BIT(RCC->APB1SMENR2, RCC_APB1SMENR2_LPTIM2SMEN) 02004 02005 /** 02006 * @} 02007 */ 02008 02009 /** @defgroup RCC_APB2_Clock_Sleep_Enable_Disable APB2 Peripheral Clock Sleep Enable Disable 02010 * @brief Enable or disable the APB2 peripheral clock during Low Power (Sleep) mode. 02011 * @note Peripheral clock gating in SLEEP mode can be used to further reduce 02012 * power consumption. 02013 * @note After wakeup from SLEEP mode, the peripheral clock is enabled again. 02014 * @note By default, all peripheral clocks are enabled during SLEEP mode. 02015 * @{ 02016 */ 02017 02018 #define __HAL_RCC_SYSCFG_CLK_SLEEP_ENABLE() SET_BIT(RCC->APB2SMENR, RCC_APB2SMENR_SYSCFGSMEN) 02019 02020 #define __HAL_RCC_SDMMC1_CLK_SLEEP_ENABLE() SET_BIT(RCC->APB2SMENR, RCC_APB2SMENR_SDMMC1SMEN) 02021 02022 #define __HAL_RCC_TIM1_CLK_SLEEP_ENABLE() SET_BIT(RCC->APB2SMENR, RCC_APB2SMENR_TIM1SMEN) 02023 02024 #define __HAL_RCC_SPI1_CLK_SLEEP_ENABLE() SET_BIT(RCC->APB2SMENR, RCC_APB2SMENR_SPI1SMEN) 02025 02026 #define __HAL_RCC_TIM8_CLK_SLEEP_ENABLE() SET_BIT(RCC->APB2SMENR, RCC_APB2SMENR_TIM8SMEN) 02027 02028 #define __HAL_RCC_USART1_CLK_SLEEP_ENABLE() SET_BIT(RCC->APB2SMENR, RCC_APB2SMENR_USART1SMEN) 02029 02030 #define __HAL_RCC_TIM15_CLK_SLEEP_ENABLE() SET_BIT(RCC->APB2SMENR, RCC_APB2SMENR_TIM15SMEN) 02031 02032 #define __HAL_RCC_TIM16_CLK_SLEEP_ENABLE() SET_BIT(RCC->APB2SMENR, RCC_APB2SMENR_TIM16SMEN) 02033 02034 #define __HAL_RCC_TIM17_CLK_SLEEP_ENABLE() SET_BIT(RCC->APB2SMENR, RCC_APB2SMENR_TIM17SMEN) 02035 02036 #define __HAL_RCC_SAI1_CLK_SLEEP_ENABLE() SET_BIT(RCC->APB2SMENR, RCC_APB2SMENR_SAI1SMEN) 02037 02038 #define __HAL_RCC_SAI2_CLK_SLEEP_ENABLE() SET_BIT(RCC->APB2SMENR, RCC_APB2SMENR_SAI2SMEN) 02039 02040 #define __HAL_RCC_DFSDM_CLK_SLEEP_ENABLE() SET_BIT(RCC->APB2SMENR, RCC_APB2SMENR_DFSDMSMEN) 02041 02042 #define __HAL_RCC_SYSCFG_CLK_SLEEP_DISABLE() CLEAR_BIT(RCC->APB2SMENR, RCC_APB2SMENR_SYSCFGSMEN) 02043 02044 #define __HAL_RCC_SDMMC1_CLK_SLEEP_DISABLE() CLEAR_BIT(RCC->APB2SMENR, RCC_APB2SMENR_SDMMC1SMEN) 02045 02046 #define __HAL_RCC_TIM1_CLK_SLEEP_DISABLE() CLEAR_BIT(RCC->APB2SMENR, RCC_APB2SMENR_TIM1SMEN) 02047 02048 #define __HAL_RCC_SPI1_CLK_SLEEP_DISABLE() CLEAR_BIT(RCC->APB2SMENR, RCC_APB2SMENR_SPI1SMEN) 02049 02050 #define __HAL_RCC_TIM8_CLK_SLEEP_DISABLE() CLEAR_BIT(RCC->APB2SMENR, RCC_APB2SMENR_TIM8SMEN) 02051 02052 #define __HAL_RCC_USART1_CLK_SLEEP_DISABLE() CLEAR_BIT(RCC->APB2SMENR, RCC_APB2SMENR_USART1SMEN) 02053 02054 #define __HAL_RCC_TIM15_CLK_SLEEP_DISABLE() CLEAR_BIT(RCC->APB2SMENR, RCC_APB2SMENR_TIM15SMEN) 02055 02056 #define __HAL_RCC_TIM16_CLK_SLEEP_DISABLE() CLEAR_BIT(RCC->APB2SMENR, RCC_APB2SMENR_TIM16SMEN) 02057 02058 #define __HAL_RCC_TIM17_CLK_SLEEP_DISABLE() CLEAR_BIT(RCC->APB2SMENR, RCC_APB2SMENR_TIM17SMEN) 02059 02060 #define __HAL_RCC_SAI1_CLK_SLEEP_DISABLE() CLEAR_BIT(RCC->APB2SMENR, RCC_APB2SMENR_SAI1SMEN) 02061 02062 #define __HAL_RCC_SAI2_CLK_SLEEP_DISABLE() CLEAR_BIT(RCC->APB2SMENR, RCC_APB2SMENR_SAI2SMEN) 02063 02064 #define __HAL_RCC_DFSDM_CLK_SLEEP_DISABLE() CLEAR_BIT(RCC->APB2SMENR, RCC_APB2SMENR_DFSDMSMEN) 02065 02066 /** 02067 * @} 02068 */ 02069 02070 /** @defgroup RCC_AHB1_Clock_Sleep_Enable_Disable_Status AHB1 Peripheral Clock Sleep Enabled or Disabled Status 02071 * @brief Check whether the AHB1 peripheral clock during Low Power (Sleep) mode is enabled or not. 02072 * @note Peripheral clock gating in SLEEP mode can be used to further reduce 02073 * power consumption. 02074 * @note After wakeup from SLEEP mode, the peripheral clock is enabled again. 02075 * @note By default, all peripheral clocks are enabled during SLEEP mode. 02076 * @{ 02077 */ 02078 02079 #define __HAL_RCC_DMA1_IS_CLK_SLEEP_ENABLED() (READ_BIT(RCC->AHB1SMENR, RCC_AHB1SMENR_DMA1SMEN) != RESET) 02080 02081 #define __HAL_RCC_DMA2_IS_CLK_SLEEP_ENABLED() (READ_BIT(RCC->AHB1SMENR, RCC_AHB1SMENR_DMA2SMEN) != RESET) 02082 02083 #define __HAL_RCC_FLASH_IS_CLK_SLEEP_ENABLED() (READ_BIT(RCC->AHB1SMENR, RCC_AHB1SMENR_FLASHSMEN) != RESET) 02084 02085 #define __HAL_RCC_SRAM1_IS_CLK_SLEEP_ENABLED() (READ_BIT(RCC->AHB1SMENR, RCC_AHB1SMENR_SRAM1SMEN) != RESET) 02086 02087 #define __HAL_RCC_CRC_IS_CLK_SLEEP_ENABLED() (READ_BIT(RCC->AHB1SMENR, RCC_AHB1SMENR_CRCSMEN) != RESET) 02088 02089 #define __HAL_RCC_TSC_IS_CLK_SLEEP_ENABLED() (READ_BIT(RCC->AHB1SMENR, RCC_AHB1SMENR_TSCSMEN) != RESET) 02090 02091 #define __HAL_RCC_DMA1_IS_CLK_SLEEP_DISABLED() (READ_BIT(RCC->AHB1SMENR, RCC_AHB1SMENR_DMA1SMEN) == RESET) 02092 02093 #define __HAL_RCC_DMA2_IS_CLK_SLEEP_DISABLED() (READ_BIT(RCC->AHB1SMENR, RCC_AHB1SMENR_DMA2SMEN) == RESET) 02094 02095 #define __HAL_RCC_FLASH_IS_CLK_SLEEP_DISABLED() (READ_BIT(RCC->AHB1SMENR, RCC_AHB1SMENR_FLASHSMEN) == RESET) 02096 02097 #define __HAL_RCC_SRAM1_IS_CLK_SLEEP_DISABLED() (READ_BIT(RCC->AHB1SMENR, RCC_AHB1SMENR_SRAM1SMEN) == RESET) 02098 02099 #define __HAL_RCC_CRC_IS_CLK_SLEEP_DISABLED() (READ_BIT(RCC->AHB1SMENR, RCC_AHB1SMENR_CRCSMEN) == RESET) 02100 02101 #define __HAL_RCC_TSC_IS_CLK_SLEEP_DISABLED() (READ_BIT(RCC->AHB1SMENR, RCC_AHB1SMENR_TSCSMEN) == RESET) 02102 02103 /** 02104 * @} 02105 */ 02106 02107 /** @defgroup RCC_AHB2_Clock_Sleep_Enable_Disable_Status AHB2 Peripheral Clock Sleep Enabled or Disabled Status 02108 * @brief Check whether the AHB2 peripheral clock during Low Power (Sleep) mode is enabled or not. 02109 * @note Peripheral clock gating in SLEEP mode can be used to further reduce 02110 * power consumption. 02111 * @note After wakeup from SLEEP mode, the peripheral clock is enabled again. 02112 * @note By default, all peripheral clocks are enabled during SLEEP mode. 02113 * @{ 02114 */ 02115 02116 #define __HAL_RCC_GPIOA_IS_CLK_SLEEP_ENABLED() (READ_BIT(RCC->AHB2SMENR, RCC_AHB2SMENR_GPIOASMEN) != RESET) 02117 02118 #define __HAL_RCC_GPIOB_IS_CLK_SLEEP_ENABLED() (READ_BIT(RCC->AHB2SMENR, RCC_AHB2SMENR_GPIOBSMEN) != RESET) 02119 02120 #define __HAL_RCC_GPIOC_IS_CLK_SLEEP_ENABLED() (READ_BIT(RCC->AHB2SMENR, RCC_AHB2SMENR_GPIOCSMEN) != RESET) 02121 02122 #define __HAL_RCC_GPIOD_IS_CLK_SLEEP_ENABLED() (READ_BIT(RCC->AHB2SMENR, RCC_AHB2SMENR_GPIODSMEN) != RESET) 02123 02124 #define __HAL_RCC_GPIOE_IS_CLK_SLEEP_ENABLED() (READ_BIT(RCC->AHB2SMENR, RCC_AHB2SMENR_GPIOESMEN) != RESET) 02125 02126 #define __HAL_RCC_GPIOF_IS_CLK_SLEEP_ENABLED() (READ_BIT(RCC->AHB2SMENR, RCC_AHB2SMENR_GPIOFSMEN) != RESET) 02127 02128 #define __HAL_RCC_GPIOG_IS_CLK_SLEEP_ENABLED() (READ_BIT(RCC->AHB2SMENR, RCC_AHB2SMENR_GPIOGSMEN) != RESET) 02129 02130 #define __HAL_RCC_GPIOH_IS_CLK_SLEEP_ENABLED() (READ_BIT(RCC->AHB2SMENR, RCC_AHB2SMENR_GPIOHSMEN) != RESET) 02131 02132 #define __HAL_RCC_SRAM2_IS_CLK_SLEEP_ENABLED() (READ_BIT(RCC->AHB2SMENR, RCC_AHB2SMENR_SRAM2SMEN) != RESET) 02133 02134 #if defined(STM32L475xx) || defined(STM32L476xx) || defined(STM32L485xx) || defined(STM32L486xx) 02135 #define __HAL_RCC_USB_OTG_FS_IS_CLK_SLEEP_ENABLED() (READ_BIT(RCC->AHB2SMENR, RCC_AHB2SMENR_OTGFSSMEN) != RESET) 02136 #endif /* STM32L475xx || STM32L476xx || STM32L485xx || STM32L486xx */ 02137 02138 #define __HAL_RCC_ADC_IS_CLK_SLEEP_ENABLED() (READ_BIT(RCC->AHB2SMENR, RCC_AHB2SMENR_ADCSMEN) != RESET) 02139 02140 #if defined(STM32L485xx) || defined(STM32L486xx) 02141 #define __HAL_RCC_AES_IS_CLK_SLEEP_ENABLED() (READ_BIT(RCC->AHB2SMENR, RCC_AHB2SMENR_AESSMEN) != RESET) 02142 #endif /* STM32L485xx || STM32L486xx */ 02143 02144 #define __HAL_RCC_RNG_IS_CLK_SLEEP_ENABLED() (READ_BIT(RCC->AHB2SMENR, RCC_AHB2SMENR_RNGSMEN) != RESET) 02145 02146 #define __HAL_RCC_GPIOA_IS_CLK_SLEEP_DISABLED() (READ_BIT(RCC->AHB2SMENR, RCC_AHB2SMENR_GPIOASMEN) == RESET) 02147 02148 #define __HAL_RCC_GPIOB_IS_CLK_SLEEP_DISABLED() (READ_BIT(RCC->AHB2SMENR, RCC_AHB2SMENR_GPIOBSMEN) == RESET) 02149 02150 #define __HAL_RCC_GPIOC_IS_CLK_SLEEP_DISABLED() (READ_BIT(RCC->AHB2SMENR, RCC_AHB2SMENR_GPIOCSMEN) == RESET) 02151 02152 #define __HAL_RCC_GPIOD_IS_CLK_SLEEP_DISABLED() (READ_BIT(RCC->AHB2SMENR, RCC_AHB2SMENR_GPIODSMEN) == RESET) 02153 02154 #define __HAL_RCC_GPIOE_IS_CLK_SLEEP_DISABLED() (READ_BIT(RCC->AHB2SMENR, RCC_AHB2SMENR_GPIOESMEN) == RESET) 02155 02156 #define __HAL_RCC_GPIOF_IS_CLK_SLEEP_DISABLED() (READ_BIT(RCC->AHB2SMENR, RCC_AHB2SMENR_GPIOFSMEN) == RESET) 02157 02158 #define __HAL_RCC_GPIOG_IS_CLK_SLEEP_DISABLED() (READ_BIT(RCC->AHB2SMENR, RCC_AHB2SMENR_GPIOGSMEN) == RESET) 02159 02160 #define __HAL_RCC_GPIOH_IS_CLK_SLEEP_DISABLED() (READ_BIT(RCC->AHB2SMENR, RCC_AHB2SMENR_GPIOHSMEN) == RESET) 02161 02162 #define __HAL_RCC_SRAM2_IS_CLK_SLEEP_DISABLED() (READ_BIT(RCC->AHB2SMENR, RCC_AHB2SMENR_SRAM2SMEN) == RESET) 02163 02164 #if defined(STM32L475xx) || defined(STM32L476xx) || defined(STM32L485xx) || defined(STM32L486xx) 02165 #define __HAL_RCC_USB_OTG_FS_IS_CLK_SLEEP_DISABLED() (READ_BIT(RCC->AHB2SMENR, RCC_AHB2SMENR_OTGFSSMEN) == RESET) 02166 #endif /* STM32L475xx || STM32L476xx || STM32L485xx || STM32L486xx */ 02167 02168 #define __HAL_RCC_ADC_IS_CLK_SLEEP_DISABLED() (READ_BIT(RCC->AHB2SMENR, RCC_AHB2SMENR_ADCSMEN) == RESET) 02169 02170 #if defined(STM32L485xx) || defined(STM32L486xx) 02171 #define __HAL_RCC_AES_IS_CLK_SLEEP_DISABLED() (READ_BIT(RCC->AHB2SMENR, RCC_AHB2SMENR_AESSMEN) == RESET) 02172 #endif /* STM32L485xx || STM32L486xx */ 02173 02174 #define __HAL_RCC_RNG_IS_CLK_SLEEP_DISABLED() (READ_BIT(RCC->AHB2SMENR, RCC_AHB2SMENR_RNGSMEN) == RESET) 02175 02176 /** 02177 * @} 02178 */ 02179 02180 /** @defgroup RCC_AHB3_Clock_Sleep_Enable_Disable_Status AHB3 Peripheral Clock Sleep Enabled or Disabled Status 02181 * @brief Check whether the AHB3 peripheral clock during Low Power (Sleep) mode is enabled or not. 02182 * @note Peripheral clock gating in SLEEP mode can be used to further reduce 02183 * power consumption. 02184 * @note After wakeup from SLEEP mode, the peripheral clock is enabled again. 02185 * @note By default, all peripheral clocks are enabled during SLEEP mode. 02186 * @{ 02187 */ 02188 02189 #define __HAL_RCC_QSPI_IS_CLK_SLEEP_ENABLED() (READ_BIT(RCC->AHB3SMENR, RCC_AHB3SMENR_QSPISMEN) != RESET) 02190 02191 #define __HAL_RCC_FMC_IS_CLK_SLEEP_ENABLED() (READ_BIT(RCC->AHB3SMENR, RCC_AHB3SMENR_FMCSMEN) != RESET) 02192 02193 #define __HAL_RCC_QSPI_IS_CLK_SLEEP_DISABLED() (READ_BIT(RCC->AHB3SMENR, RCC_AHB3SMENR_QSPISMEN) == RESET) 02194 02195 #define __HAL_RCC_FMC_IS_CLK_SLEEP_DISABLED() (READ_BIT(RCC->AHB3SMENR, RCC_AHB3SMENR_FMCSMEN) == RESET) 02196 02197 /** 02198 * @} 02199 */ 02200 02201 /** @defgroup RCC_APB1_Clock_Sleep_Enable_Disable_Status APB1 Peripheral Clock Sleep Enabled or Disabled Status 02202 * @brief Check whether the APB1 peripheral clock during Low Power (Sleep) mode is enabled or not. 02203 * @note Peripheral clock gating in SLEEP mode can be used to further reduce 02204 * power consumption. 02205 * @note After wakeup from SLEEP mode, the peripheral clock is enabled again. 02206 * @note By default, all peripheral clocks are enabled during SLEEP mode. 02207 * @{ 02208 */ 02209 02210 #define __HAL_RCC_TIM2_IS_CLK_SLEEP_ENABLED() (READ_BIT(RCC->APB1SMENR1, RCC_APB1SMENR1_TIM2SMEN) != RESET) 02211 02212 #define __HAL_RCC_TIM3_IS_CLK_SLEEP_ENABLED() (READ_BIT(RCC->APB1SMENR1, RCC_APB1SMENR1_TIM3SMEN) != RESET) 02213 02214 #define __HAL_RCC_TIM4_IS_CLK_SLEEP_ENABLED() (READ_BIT(RCC->APB1SMENR1, RCC_APB1SMENR1_TIM4SMEN) != RESET) 02215 02216 #define __HAL_RCC_TIM5_IS_CLK_SLEEP_ENABLED() (READ_BIT(RCC->APB1SMENR1, RCC_APB1SMENR1_TIM5SMEN) != RESET) 02217 02218 #define __HAL_RCC_TIM6_IS_CLK_SLEEP_ENABLED() (READ_BIT(RCC->APB1SMENR1, RCC_APB1SMENR1_TIM6SMEN) != RESET) 02219 02220 #define __HAL_RCC_TIM7_IS_CLK_SLEEP_ENABLED() (READ_BIT(RCC->APB1SMENR1, RCC_APB1SMENR1_TIM7SMEN) != RESET) 02221 02222 #if defined(STM32L476xx) || defined(STM32L486xx) 02223 #define __HAL_RCC_LCD_IS_CLK_SLEEP_ENABLED() (READ_BIT(RCC->APB1SMENR1, RCC_APB1SMENR1_LCDSMEN) != RESET) 02224 #endif /* STM32L476xx || STM32L486xx */ 02225 02226 #define __HAL_RCC_WWDG_IS_CLK_SLEEP_ENABLED() (READ_BIT(RCC->APB1SMENR1, RCC_APB1SMENR1_WWDGSMEN) != RESET) 02227 02228 #define __HAL_RCC_SPI2_IS_CLK_SLEEP_ENABLED() (READ_BIT(RCC->APB1SMENR1, RCC_APB1SMENR1_SPI2SMEN) != RESET) 02229 02230 #define __HAL_RCC_SPI3_IS_CLK_SLEEP_ENABLED() (READ_BIT(RCC->APB1SMENR1, RCC_APB1SMENR1_SPI3SMEN) != RESET) 02231 02232 #define __HAL_RCC_USART2_IS_CLK_SLEEP_ENABLED() (READ_BIT(RCC->APB1SMENR1, RCC_APB1SMENR1_USART2SMEN) != RESET) 02233 02234 #define __HAL_RCC_USART3_IS_CLK_SLEEP_ENABLED() (READ_BIT(RCC->APB1SMENR1, RCC_APB1SMENR1_USART3SMEN) != RESET) 02235 02236 #define __HAL_RCC_UART4_IS_CLK_SLEEP_ENABLED() (READ_BIT(RCC->APB1SMENR1, RCC_APB1SMENR1_UART4SMEN) != RESET) 02237 02238 #define __HAL_RCC_UART5_IS_CLK_SLEEP_ENABLED() (READ_BIT(RCC->APB1SMENR1, RCC_APB1SMENR1_UART5SMEN) != RESET) 02239 02240 #define __HAL_RCC_I2C1_IS_CLK_SLEEP_ENABLED() (READ_BIT(RCC->APB1SMENR1, RCC_APB1SMENR1_I2C1SMEN) != RESET) 02241 02242 #define __HAL_RCC_I2C2_IS_CLK_SLEEP_ENABLED() (READ_BIT(RCC->APB1SMENR1, RCC_APB1SMENR1_I2C2SMEN) != RESET) 02243 02244 #define __HAL_RCC_I2C3_IS_CLK_SLEEP_ENABLED() (READ_BIT(RCC->APB1SMENR1, RCC_APB1SMENR1_I2C3SMEN) != RESET) 02245 02246 #define __HAL_RCC_CAN1_IS_CLK_SLEEP_ENABLED() (READ_BIT(RCC->APB1SMENR1, RCC_APB1SMENR1_CAN1SMEN) != RESET) 02247 02248 #define __HAL_RCC_PWR_IS_CLK_SLEEP_ENABLED() (READ_BIT(RCC->APB1SMENR1, RCC_APB1SMENR1_PWRSMEN) != RESET) 02249 02250 #define __HAL_RCC_DAC1_IS_CLK_SLEEP_ENABLED() (READ_BIT(RCC->APB1SMENR1, RCC_APB1SMENR1_DAC1SMEN) != RESET) 02251 02252 #define __HAL_RCC_OPAMP_IS_CLK_SLEEP_ENABLED() (READ_BIT(RCC->APB1SMENR1, RCC_APB1SMENR1_OPAMPSMEN) != RESET) 02253 02254 #define __HAL_RCC_LPTIM1_IS_CLK_SLEEP_ENABLED() (READ_BIT(RCC->APB1SMENR1, RCC_APB1SMENR1_LPTIM1SMEN) != RESET) 02255 02256 #define __HAL_RCC_LPUART1_IS_CLK_SLEEP_ENABLED() (READ_BIT(RCC->APB1SMENR2, RCC_APB1SMENR2_LPUART1SMEN) != RESET) 02257 02258 #define __HAL_RCC_SWPMI1_IS_CLK_SLEEP_ENABLED() (READ_BIT(RCC->APB1SMENR2, RCC_APB1SMENR2_SWPMI1SMEN) != RESET) 02259 02260 #define __HAL_RCC_LPTIM2_IS_CLK_SLEEP_ENABLED() (READ_BIT(RCC->APB1SMENR2, RCC_APB1SMENR2_LPTIM2SMEN) != RESET) 02261 02262 #define __HAL_RCC_TIM2_IS_CLK_SLEEP_DISABLED() (READ_BIT(RCC->APB1SMENR1, RCC_APB1SMENR1_TIM2SMEN) == RESET) 02263 02264 #define __HAL_RCC_TIM3_IS_CLK_SLEEP_DISABLED() (READ_BIT(RCC->APB1SMENR1, RCC_APB1SMENR1_TIM3SMEN) == RESET) 02265 02266 #define __HAL_RCC_TIM4_IS_CLK_SLEEP_DISABLED() (READ_BIT(RCC->APB1SMENR1, RCC_APB1SMENR1_TIM4SMEN) == RESET) 02267 02268 #define __HAL_RCC_TIM5_IS_CLK_SLEEP_DISABLED() (READ_BIT(RCC->APB1SMENR1, RCC_APB1SMENR1_TIM5SMEN) == RESET) 02269 02270 #define __HAL_RCC_TIM6_IS_CLK_SLEEP_DISABLED() (READ_BIT(RCC->APB1SMENR1, RCC_APB1SMENR1_TIM6SMEN) == RESET) 02271 02272 #define __HAL_RCC_TIM7_IS_CLK_SLEEP_DISABLED() (READ_BIT(RCC->APB1SMENR1, RCC_APB1SMENR1_TIM7SMEN) == RESET) 02273 02274 #if defined(STM32L476xx) || defined(STM32L486xx) 02275 #define __HAL_RCC_LCD_IS_CLK_SLEEP_DISABLED() (READ_BIT(RCC->APB1SMENR1, RCC_APB1SMENR1_LCDSMEN) == RESET) 02276 #endif /* STM32L476xx || STM32L486xx */ 02277 02278 #define __HAL_RCC_WWDG_IS_CLK_SLEEP_DISABLED() (READ_BIT(RCC->APB1SMENR1, RCC_APB1SMENR1_WWDGSMEN) == RESET) 02279 02280 #define __HAL_RCC_SPI2_IS_CLK_SLEEP_DISABLED() (READ_BIT(RCC->APB1SMENR1, RCC_APB1SMENR1_SPI2SMEN) == RESET) 02281 02282 #define __HAL_RCC_SPI3_IS_CLK_SLEEP_DISABLED() (READ_BIT(RCC->APB1SMENR1, RCC_APB1SMENR1_SPI3SMEN) == RESET) 02283 02284 #define __HAL_RCC_USART2_IS_CLK_SLEEP_DISABLED() (READ_BIT(RCC->APB1SMENR1, RCC_APB1SMENR1_USART2SMEN) == RESET) 02285 02286 #define __HAL_RCC_USART3_IS_CLK_SLEEP_DISABLED() (READ_BIT(RCC->APB1SMENR1, RCC_APB1SMENR1_USART3SMEN) == RESET) 02287 02288 #define __HAL_RCC_UART4_IS_CLK_SLEEP_DISABLED() (READ_BIT(RCC->APB1SMENR1, RCC_APB1SMENR1_UART4SMEN) == RESET) 02289 02290 #define __HAL_RCC_UART5_IS_CLK_SLEEP_DISABLED() (READ_BIT(RCC->APB1SMENR1, RCC_APB1SMENR1_UART5SMEN) == RESET) 02291 02292 #define __HAL_RCC_I2C1_IS_CLK_SLEEP_DISABLED() (READ_BIT(RCC->APB1SMENR1, RCC_APB1SMENR1_I2C1SMEN) == RESET) 02293 02294 #define __HAL_RCC_I2C2_IS_CLK_SLEEP_DISABLED() (READ_BIT(RCC->APB1SMENR1, RCC_APB1SMENR1_I2C2SMEN) == RESET) 02295 02296 #define __HAL_RCC_I2C3_IS_CLK_SLEEP_DISABLED() (READ_BIT(RCC->APB1SMENR1, RCC_APB1SMENR1_I2C3SMEN) == RESET) 02297 02298 #define __HAL_RCC_CAN1_IS_CLK_SLEEP_DISABLED() (READ_BIT(RCC->APB1SMENR1, RCC_APB1SMENR1_CAN1SMEN) == RESET) 02299 02300 #define __HAL_RCC_PWR_IS_CLK_SLEEP_DISABLED() (READ_BIT(RCC->APB1SMENR1, RCC_APB1SMENR1_PWRSMEN) == RESET) 02301 02302 #define __HAL_RCC_DAC1_IS_CLK_SLEEP_DISABLED() (READ_BIT(RCC->APB1SMENR1, RCC_APB1SMENR1_DAC1SMEN) == RESET) 02303 02304 #define __HAL_RCC_OPAMP_IS_CLK_SLEEP_DISABLED() (READ_BIT(RCC->APB1SMENR1, RCC_APB1SMENR1_OPAMPSMEN) == RESET) 02305 02306 #define __HAL_RCC_LPTIM1_IS_CLK_SLEEP_DISABLED() (READ_BIT(RCC->APB1SMENR1, RCC_APB1SMENR1_LPTIM1SMEN) == RESET) 02307 02308 #define __HAL_RCC_LPUART1_IS_CLK_SLEEP_DISABLED() (READ_BIT(RCC->APB1SMENR2, RCC_APB1SMENR2_LPUART1SMEN) == RESET) 02309 02310 #define __HAL_RCC_SWPMI1_IS_CLK_SLEEP_DISABLED() (READ_BIT(RCC->APB1SMENR2, RCC_APB1SMENR2_SWPMI1SMEN) == RESET) 02311 02312 #define __HAL_RCC_LPTIM2_IS_CLK_SLEEP_DISABLED() (READ_BIT(RCC->APB1SMENR2, RCC_APB1SMENR2_LPTIM2SMEN) == RESET) 02313 02314 /** 02315 * @} 02316 */ 02317 02318 /** @defgroup RCC_APB2_Clock_Sleep_Enable_Disable_Status APB2 Peripheral Clock Sleep Enabled or Disabled Status 02319 * @brief Check whether the APB2 peripheral clock during Low Power (Sleep) mode is enabled or not. 02320 * @note Peripheral clock gating in SLEEP mode can be used to further reduce 02321 * power consumption. 02322 * @note After wakeup from SLEEP mode, the peripheral clock is enabled again. 02323 * @note By default, all peripheral clocks are enabled during SLEEP mode. 02324 * @{ 02325 */ 02326 02327 #define __HAL_RCC_SYSCFG_IS_CLK_SLEEP_ENABLED() (READ_BIT(RCC->APB2SMENR, RCC_APB2SMENR_SYSCFGSMEN) != RESET) 02328 02329 #define __HAL_RCC_SDMMC1_IS_CLK_SLEEP_ENABLED() (READ_BIT(RCC->APB2SMENR, RCC_APB2SMENR_SDMMC1SMEN) != RESET) 02330 02331 #define __HAL_RCC_TIM1_IS_CLK_SLEEP_ENABLED() (READ_BIT(RCC->APB2SMENR, RCC_APB2SMENR_TIM1SMEN) != RESET) 02332 02333 #define __HAL_RCC_SPI1_IS_CLK_SLEEP_ENABLED() (READ_BIT(RCC->APB2SMENR, RCC_APB2SMENR_SPI1SMEN) != RESET) 02334 02335 #define __HAL_RCC_TIM8_IS_CLK_SLEEP_ENABLED() (READ_BIT(RCC->APB2SMENR, RCC_APB2SMENR_TIM8SMEN) != RESET) 02336 02337 #define __HAL_RCC_USART1_IS_CLK_SLEEP_ENABLED() (READ_BIT(RCC->APB2SMENR, RCC_APB2SMENR_USART1SMEN) != RESET) 02338 02339 #define __HAL_RCC_TIM15_IS_CLK_SLEEP_ENABLED() (READ_BIT(RCC->APB2SMENR, RCC_APB2SMENR_TIM15SMEN) != RESET) 02340 02341 #define __HAL_RCC_TIM16_IS_CLK_SLEEP_ENABLED() (READ_BIT(RCC->APB2SMENR, RCC_APB2SMENR_TIM16SMEN) != RESET) 02342 02343 #define __HAL_RCC_TIM17_IS_CLK_SLEEP_ENABLED() (READ_BIT(RCC->APB2SMENR, RCC_APB2SMENR_TIM17SMEN) != RESET) 02344 02345 #define __HAL_RCC_SAI1_IS_CLK_SLEEP_ENABLED() (READ_BIT(RCC->APB2SMENR, RCC_APB2SMENR_SAI1SMEN) != RESET) 02346 02347 #define __HAL_RCC_SAI2_IS_CLK_SLEEP_ENABLED() (READ_BIT(RCC->APB2SMENR, RCC_APB2SMENR_SAI2SMEN) != RESET) 02348 02349 #define __HAL_RCC_DFSDM_IS_CLK_SLEEP_ENABLED() (READ_BIT(RCC->APB2SMENR, RCC_APB2SMENR_DFSDMSMEN) != RESET) 02350 02351 #define __HAL_RCC_SYSCFG_IS_CLK_SLEEP_DISABLED() (READ_BIT(RCC->APB2SMENR, RCC_APB2SMENR_SYSCFGSMEN) == RESET) 02352 02353 #define __HAL_RCC_SDMMC1_IS_CLK_SLEEP_DISABLED() (READ_BIT(RCC->APB2SMENR, RCC_APB2SMENR_SDMMC1SMEN) == RESET) 02354 02355 #define __HAL_RCC_TIM1_IS_CLK_SLEEP_DISABLED() (READ_BIT(RCC->APB2SMENR, RCC_APB2SMENR_TIM1SMEN) == RESET) 02356 02357 #define __HAL_RCC_SPI1_IS_CLK_SLEEP_DISABLED() (READ_BIT(RCC->APB2SMENR, RCC_APB2SMENR_SPI1SMEN) == RESET) 02358 02359 #define __HAL_RCC_TIM8_IS_CLK_SLEEP_DISABLED() (READ_BIT(RCC->APB2SMENR, RCC_APB2SMENR_TIM8SMEN) == RESET) 02360 02361 #define __HAL_RCC_USART1_IS_CLK_SLEEP_DISABLED() (READ_BIT(RCC->APB2SMENR, RCC_APB2SMENR_USART1SMEN) == RESET) 02362 02363 #define __HAL_RCC_TIM15_IS_CLK_SLEEP_DISABLED() (READ_BIT(RCC->APB2SMENR, RCC_APB2SMENR_TIM15SMEN) == RESET) 02364 02365 #define __HAL_RCC_TIM16_IS_CLK_SLEEP_DISABLED() (READ_BIT(RCC->APB2SMENR, RCC_APB2SMENR_TIM16SMEN) == RESET) 02366 02367 #define __HAL_RCC_TIM17_IS_CLK_SLEEP_DISABLED() (READ_BIT(RCC->APB2SMENR, RCC_APB2SMENR_TIM17SMEN) == RESET) 02368 02369 #define __HAL_RCC_SAI1_IS_CLK_SLEEP_DISABLED() (READ_BIT(RCC->APB2SMENR, RCC_APB2SMENR_SAI1SMEN) == RESET) 02370 02371 #define __HAL_RCC_SAI2_IS_CLK_SLEEP_DISABLED() (READ_BIT(RCC->APB2SMENR, RCC_APB2SMENR_SAI2SMEN) == RESET) 02372 02373 #define __HAL_RCC_DFSDM_IS_CLK_SLEEP_DISABLED() (READ_BIT(RCC->APB2SMENR, RCC_APB2SMENR_DFSDMSMEN) == RESET) 02374 02375 /** 02376 * @} 02377 */ 02378 02379 /** @defgroup RCC_Backup_Domain_Reset RCC Backup Domain Reset 02380 * @{ 02381 */ 02382 02383 /** @brief Macros to force or release the Backup domain reset. 02384 * @note This function resets the RTC peripheral (including the backup registers) 02385 * and the RTC clock source selection in RCC_CSR register. 02386 * @note The BKPSRAM is not affected by this reset. 02387 * @retval None 02388 */ 02389 #define __HAL_RCC_BACKUPRESET_FORCE() SET_BIT(RCC->BDCR, RCC_BDCR_BDRST) 02390 02391 #define __HAL_RCC_BACKUPRESET_RELEASE() CLEAR_BIT(RCC->BDCR, RCC_BDCR_BDRST) 02392 02393 /** 02394 * @} 02395 */ 02396 02397 /** @defgroup RCC_RTC_Clock_Configuration RCC RTC Clock Configuration 02398 * @{ 02399 */ 02400 02401 /** @brief Macros to enable or disable the RTC clock. 02402 * @note As the RTC is in the Backup domain and write access is denied to 02403 * this domain after reset, you have to enable write access using 02404 * HAL_PWR_EnableBkUpAccess() function before to configure the RTC 02405 * (to be done once after reset). 02406 * @note These macros must be used after the RTC clock source was selected. 02407 * @retval None 02408 */ 02409 #define __HAL_RCC_RTC_ENABLE() SET_BIT(RCC->BDCR, RCC_BDCR_RTCEN) 02410 02411 #define __HAL_RCC_RTC_DISABLE() CLEAR_BIT(RCC->BDCR, RCC_BDCR_RTCEN) 02412 02413 /** 02414 * @} 02415 */ 02416 02417 /** @brief Macros to enable or disable the Internal High Speed 16MHz oscillator (HSI). 02418 * @note The HSI is stopped by hardware when entering STOP and STANDBY modes. 02419 * It is used (enabled by hardware) as system clock source after startup 02420 * from Reset, wakeup from STOP and STANDBY mode, or in case of failure 02421 * of the HSE used directly or indirectly as system clock (if the Clock 02422 * Security System CSS is enabled). 02423 * @note HSI can not be stopped if it is used as system clock source. In this case, 02424 * you have to select another source of the system clock then stop the HSI. 02425 * @note After enabling the HSI, the application software should wait on HSIRDY 02426 * flag to be set indicating that HSI clock is stable and can be used as 02427 * system clock source. 02428 * This parameter can be: ENABLE or DISABLE. 02429 * @note When the HSI is stopped, HSIRDY flag goes low after 6 HSI oscillator 02430 * clock cycles. 02431 * @retval None 02432 */ 02433 #define __HAL_RCC_HSI_ENABLE() SET_BIT(RCC->CR, RCC_CR_HSION) 02434 02435 #define __HAL_RCC_HSI_DISABLE() CLEAR_BIT(RCC->CR, RCC_CR_HSION) 02436 02437 /** @brief Macro to adjust the Internal High Speed 16MHz oscillator (HSI) calibration value. 02438 * @note The calibration is used to compensate for the variations in voltage 02439 * and temperature that influence the frequency of the internal HSI RC. 02440 * @param __HSICALIBRATIONVALUE__: specifies the calibration trimming value 02441 * (default is RCC_HSICALIBRATION_DEFAULT). 02442 * This parameter must be a number between 0 and 31. 02443 * @retval None 02444 */ 02445 #define __HAL_RCC_HSI_CALIBRATIONVALUE_ADJUST(__HSICALIBRATIONVALUE__) \ 02446 MODIFY_REG(RCC->ICSCR, RCC_ICSCR_HSITRIM, (uint32_t)(__HSICALIBRATIONVALUE__) << POSITION_VAL(RCC_ICSCR_HSITRIM)) 02447 02448 /** 02449 * @brief Macros to enable or disable the wakeup the Internal High Speed oscillator (HSI) 02450 * in parallel to the Internal Multi Speed oscillator (MSI) used at system wakeup. 02451 * @note The enable of this function has not effect on the HSION bit. 02452 * This parameter can be: ENABLE or DISABLE. 02453 * @retval None 02454 */ 02455 #define __HAL_RCC_HSIAUTOMATIC_START_ENABLE() SET_BIT(RCC->CR, RCC_CR_HSIASFS) 02456 02457 #define __HAL_RCC_HSIAUTOMATIC_START_DISABLE() CLEAR_BIT(RCC->CR, RCC_CR_HSIASFS) 02458 02459 /** 02460 * @brief Macros to enable or disable the force of the Internal High Speed oscillator (HSI) 02461 * in STOP mode to be quickly available as kernel clock for USARTs and I2Cs. 02462 * @note Keeping the HSI ON in STOP mode allows to avoid slowing down the communication 02463 * speed because of the HSI startup time. 02464 * @note The enable of this function has not effect on the HSION bit. 02465 * This parameter can be: ENABLE or DISABLE. 02466 * @retval None 02467 */ 02468 #define __HAL_RCC_HSISTOP_ENABLE() SET_BIT(RCC->CR, RCC_CR_HSIKERON) 02469 02470 #define __HAL_RCC_HSISTOP_DISABLE() CLEAR_BIT(RCC->CR, RCC_CR_HSIKERON) 02471 02472 /** 02473 * @brief Macros to enable or disable the Internal Multi Speed oscillator (MSI). 02474 * @note The MSI is stopped by hardware when entering STOP and STANDBY modes. 02475 * It is used (enabled by hardware) as system clock source after 02476 * startup from Reset, wakeup from STOP and STANDBY mode, or in case 02477 * of failure of the HSE used directly or indirectly as system clock 02478 * (if the Clock Security System CSS is enabled). 02479 * @note MSI can not be stopped if it is used as system clock source. 02480 * In this case, you have to select another source of the system 02481 * clock then stop the MSI. 02482 * @note After enabling the MSI, the application software should wait on 02483 * MSIRDY flag to be set indicating that MSI clock is stable and can 02484 * be used as system clock source. 02485 * @note When the MSI is stopped, MSIRDY flag goes low after 6 MSI oscillator 02486 * clock cycles. 02487 * @retval None 02488 */ 02489 #define __HAL_RCC_MSI_ENABLE() SET_BIT(RCC->CR, RCC_CR_MSION) 02490 02491 #define __HAL_RCC_MSI_DISABLE() CLEAR_BIT(RCC->CR, RCC_CR_MSION) 02492 02493 /** @brief Macro Adjusts the Internal Multi Speed oscillator (MSI) calibration value. 02494 * @note The calibration is used to compensate for the variations in voltage 02495 * and temperature that influence the frequency of the internal MSI RC. 02496 * Refer to the Application Note AN3300 for more details on how to 02497 * calibrate the MSI. 02498 * @param __MSICALIBRATIONVALUE__: specifies the calibration trimming value 02499 * (default is RCC_MSICALIBRATION_DEFAULT). 02500 * This parameter must be a number between 0 and 255. 02501 * @retval None 02502 */ 02503 #define __HAL_RCC_MSI_CALIBRATIONVALUE_ADJUST(__MSICALIBRATIONVALUE__) \ 02504 MODIFY_REG(RCC->ICSCR, RCC_ICSCR_MSITRIM, (uint32_t)(__MSICALIBRATIONVALUE__) << 8) 02505 02506 /** 02507 * @brief Macro configures the Internal Multi Speed oscillator (MSI) clock range in run mode 02508 * @note After restart from Reset , the MSI clock is around 4 MHz. 02509 * After stop the startup clock can be MSI (at any of its possible 02510 * frequencies, the one that was used before entering stop mode) or HSI. 02511 * After Standby its frequency can be selected between 4 possible values 02512 * (1, 2, 4 or 8 MHz). 02513 * @note MSIRANGE can be modified when MSI is OFF (MSION=0) or when MSI is ready 02514 * (MSIRDY=1). 02515 * @note The MSI clock range after reset can be modified on the fly. 02516 * @param __MSIRANGEVALUE__: specifies the MSI clock range. 02517 * This parameter must be one of the following values: 02518 * @arg RCC_MSIRANGE_0: MSI clock is around 100 KHz 02519 * @arg RCC_MSIRANGE_1: MSI clock is around 200 KHz 02520 * @arg RCC_MSIRANGE_2: MSI clock is around 400 KHz 02521 * @arg RCC_MSIRANGE_3: MSI clock is around 800 KHz 02522 * @arg RCC_MSIRANGE_4: MSI clock is around 1 MHz 02523 * @arg RCC_MSIRANGE_5: MSI clock is around 2MHz 02524 * @arg RCC_MSIRANGE_6: MSI clock is around 4MHz (default after Reset) 02525 * @arg RCC_MSIRANGE_7: MSI clock is around 8 MHz 02526 * @arg RCC_MSIRANGE_8: MSI clock is around 16 MHz 02527 * @arg RCC_MSIRANGE_9: MSI clock is around 24 MHz 02528 * @arg RCC_MSIRANGE_10: MSI clock is around 32 MHz 02529 * @arg RCC_MSIRANGE_11: MSI clock is around 48 MHz 02530 * @retval None 02531 */ 02532 #define __HAL_RCC_MSI_RANGE_CONFIG(__MSIRANGEVALUE__) \ 02533 do { \ 02534 SET_BIT(RCC->CR, RCC_CR_MSIRGSEL); \ 02535 MODIFY_REG(RCC->CR, RCC_CR_MSIRANGE, (__MSIRANGEVALUE__)); \ 02536 } while(0) 02537 02538 /** 02539 * @brief Macro configures the Internal Multi Speed oscillator (MSI) clock range after Standby mode 02540 * After Standby its frequency can be selected between 4 possible values (1, 2, 4 or 8 MHz). 02541 * @param __MSIRANGEVALUE__: specifies the MSI clock range. 02542 * This parameter must be one of the following values: 02543 * @arg RCC_MSIRANGE_4: MSI clock is around 1 MHz 02544 * @arg RCC_MSIRANGE_5: MSI clock is around 2MHz 02545 * @arg RCC_MSIRANGE_6: MSI clock is around 4MHz (default after Reset) 02546 * @arg RCC_MSIRANGE_7: MSI clock is around 8 MHz 02547 * @retval None 02548 */ 02549 #define __HAL_RCC_MSI_STANDBY_RANGE_CONFIG(__MSIRANGEVALUE__) \ 02550 MODIFY_REG(RCC->CSR, RCC_CSR_MSISRANGE, (__MSIRANGEVALUE__) << 4U) 02551 02552 /** @brief Macro to get the Internal Multi Speed oscillator (MSI) clock range in run mode 02553 * @retval MSI clock range. 02554 * This parameter must be one of the following values: 02555 * @arg RCC_MSIRANGE_0: MSI clock is around 100 KHz 02556 * @arg RCC_MSIRANGE_1: MSI clock is around 200 KHz 02557 * @arg RCC_MSIRANGE_2: MSI clock is around 400 KHz 02558 * @arg RCC_MSIRANGE_3: MSI clock is around 800 KHz 02559 * @arg RCC_MSIRANGE_4: MSI clock is around 1 MHz 02560 * @arg RCC_MSIRANGE_5: MSI clock is around 2MHz 02561 * @arg RCC_MSIRANGE_6: MSI clock is around 4MHz (default after Reset) 02562 * @arg RCC_MSIRANGE_7: MSI clock is around 8 MHz 02563 * @arg RCC_MSIRANGE_8: MSI clock is around 16 MHz 02564 * @arg RCC_MSIRANGE_9: MSI clock is around 24 MHz 02565 * @arg RCC_MSIRANGE_10: MSI clock is around 32 MHz 02566 * @arg RCC_MSIRANGE_11: MSI clock is around 48 MHz 02567 */ 02568 #define __HAL_RCC_GET_MSI_RANGE() \ 02569 ((READ_BIT(RCC->CR, RCC_CR_MSIRGSEL) != RESET) ? \ 02570 (uint32_t)(READ_BIT(RCC->CR, RCC_CR_MSIRANGE)) : \ 02571 (uint32_t)(READ_BIT(RCC->CSR, RCC_CSR_MSISRANGE) >> 4)) 02572 02573 /** @brief Macros to enable or disable the Internal Low Speed oscillator (LSI). 02574 * @note After enabling the LSI, the application software should wait on 02575 * LSIRDY flag to be set indicating that LSI clock is stable and can 02576 * be used to clock the IWDG and/or the RTC. 02577 * @note LSI can not be disabled if the IWDG is running. 02578 * @note When the LSI is stopped, LSIRDY flag goes low after 6 LSI oscillator 02579 * clock cycles. 02580 * @retval None 02581 */ 02582 #define __HAL_RCC_LSI_ENABLE() SET_BIT(RCC->CSR, RCC_CSR_LSION) 02583 02584 #define __HAL_RCC_LSI_DISABLE() CLEAR_BIT(RCC->CSR, RCC_CSR_LSION) 02585 02586 /** 02587 * @brief Macro to configure the External High Speed oscillator (HSE). 02588 * @note Transition HSE Bypass to HSE On and HSE On to HSE Bypass are not 02589 * supported by this macro. User should request a transition to HSE Off 02590 * first and then HSE On or HSE Bypass. 02591 * @note After enabling the HSE (RCC_HSE_ON or RCC_HSE_Bypass), the application 02592 * software should wait on HSERDY flag to be set indicating that HSE clock 02593 * is stable and can be used to clock the PLL and/or system clock. 02594 * @note HSE state can not be changed if it is used directly or through the 02595 * PLL as system clock. In this case, you have to select another source 02596 * of the system clock then change the HSE state (ex. disable it). 02597 * @note The HSE is stopped by hardware when entering STOP and STANDBY modes. 02598 * @note This function reset the CSSON bit, so if the clock security system(CSS) 02599 * was previously enabled you have to enable it again after calling this 02600 * function. 02601 * @param __STATE__: specifies the new state of the HSE. 02602 * This parameter can be one of the following values: 02603 * @arg RCC_HSE_OFF: turn OFF the HSE oscillator, HSERDY flag goes low after 02604 * 6 HSE oscillator clock cycles. 02605 * @arg RCC_HSE_ON: turn ON the HSE oscillator. 02606 * @arg RCC_HSE_BYPASS: HSE oscillator bypassed with external clock. 02607 * @retval None 02608 */ 02609 #define __HAL_RCC_HSE_CONFIG(__STATE__) \ 02610 do { \ 02611 if((__STATE__) == RCC_HSE_ON) \ 02612 { \ 02613 SET_BIT(RCC->CR, RCC_CR_HSEON); \ 02614 } \ 02615 else if((__STATE__) == RCC_HSE_BYPASS) \ 02616 { \ 02617 CLEAR_BIT(RCC->CR, RCC_CR_HSEON); \ 02618 SET_BIT(RCC->CR, RCC_CR_HSEBYP); \ 02619 SET_BIT(RCC->CR, RCC_CR_HSEON); \ 02620 } \ 02621 else \ 02622 { \ 02623 CLEAR_BIT(RCC->CR, RCC_CR_HSEON); \ 02624 CLEAR_BIT(RCC->CR, RCC_CR_HSEBYP); \ 02625 } \ 02626 } while(0) 02627 02628 /** 02629 * @brief Macro to configure the External Low Speed oscillator (LSE). 02630 * @note Transitions LSE Bypass to LSE On and LSE On to LSE Bypass are not 02631 * supported by this macro. User should request a transition to LSE Off 02632 * first and then LSE On or LSE Bypass. 02633 * @note As the LSE is in the Backup domain and write access is denied to 02634 * this domain after reset, you have to enable write access using 02635 * HAL_PWR_EnableBkUpAccess() function before to configure the LSE 02636 * (to be done once after reset). 02637 * @note After enabling the LSE (RCC_LSE_ON or RCC_LSE_BYPASS), the application 02638 * software should wait on LSERDY flag to be set indicating that LSE clock 02639 * is stable and can be used to clock the RTC. 02640 * @param __STATE__: specifies the new state of the LSE. 02641 * This parameter can be one of the following values: 02642 * @arg RCC_LSE_OFF: turn OFF the LSE oscillator, LSERDY flag goes low after 02643 * 6 LSE oscillator clock cycles. 02644 * @arg RCC_LSE_ON: turn ON the LSE oscillator. 02645 * @arg RCC_LSE_BYPASS: LSE oscillator bypassed with external clock. 02646 * @retval None 02647 */ 02648 #define __HAL_RCC_LSE_CONFIG(__STATE__) \ 02649 do { \ 02650 if((__STATE__) == RCC_LSE_ON) \ 02651 { \ 02652 SET_BIT(RCC->BDCR, RCC_BDCR_LSEON); \ 02653 } \ 02654 else if((__STATE__) == RCC_LSE_OFF) \ 02655 { \ 02656 CLEAR_BIT(RCC->BDCR, RCC_BDCR_LSEON); \ 02657 CLEAR_BIT(RCC->BDCR, RCC_BDCR_LSEBYP); \ 02658 } \ 02659 else if((__STATE__) == RCC_LSE_BYPASS) \ 02660 { \ 02661 CLEAR_BIT(RCC->BDCR, RCC_BDCR_LSEON); \ 02662 SET_BIT(RCC->BDCR, RCC_BDCR_LSEBYP); \ 02663 SET_BIT(RCC->BDCR, RCC_BDCR_LSEON); \ 02664 } \ 02665 else \ 02666 { \ 02667 CLEAR_BIT(RCC->BDCR, RCC_BDCR_LSEON); \ 02668 CLEAR_BIT(RCC->BDCR, RCC_BDCR_LSEBYP); \ 02669 } \ 02670 } while(0) 02671 02672 /** @brief Macros to configure the RTC clock (RTCCLK). 02673 * @note As the RTC clock configuration bits are in the Backup domain and write 02674 * access is denied to this domain after reset, you have to enable write 02675 * access using the Power Backup Access macro before to configure 02676 * the RTC clock source (to be done once after reset). 02677 * @note Once the RTC clock is configured it cannot be changed unless the 02678 * Backup domain is reset using __HAL_RCC_BACKUPRESET_FORCE() macro, or by 02679 * a Power On Reset (POR). 02680 * 02681 * @param __RTC_CLKSOURCE__: specifies the RTC clock source. 02682 * This parameter can be one of the following values: 02683 * @arg RCC_RTCCLKSOURCE_LSE: LSE selected as RTC clock. 02684 * @arg RCC_RTCCLKSOURCE_LSI: LSI selected as RTC clock. 02685 * @arg RCC_RTCCLKSOURCE_HSE_DIV32: HSE clock divided by 32 selected 02686 * 02687 * @note If the LSE or LSI is used as RTC clock source, the RTC continues to 02688 * work in STOP and STANDBY modes, and can be used as wakeup source. 02689 * However, when the HSE clock is used as RTC clock source, the RTC 02690 * cannot be used in STOP and STANDBY modes. 02691 * @note The maximum input clock frequency for RTC is 1MHz (when using HSE as 02692 * RTC clock source). 02693 * @retval None 02694 */ 02695 #define __HAL_RCC_RTC_CONFIG(__RTC_CLKSOURCE__) \ 02696 MODIFY_REG( RCC->BDCR, RCC_BDCR_RTCSEL, (__RTC_CLKSOURCE__)) 02697 02698 02699 /** @brief Macro to get the RTC clock source. 02700 * @retval The returned value can be one of the following: 02701 * @arg RCC_RTCCLKSOURCE_LSE: LSE selected as RTC clock. 02702 * @arg RCC_RTCCLKSOURCE_LSI: LSI selected as RTC clock. 02703 * @arg RCC_RTCCLKSOURCE_HSE_DIV32: HSE clock divided by 32 selected 02704 */ 02705 #define __HAL_RCC_GET_RTC_SOURCE() ((uint32_t)(READ_BIT(RCC->BDCR, RCC_BDCR_RTCSEL))) 02706 02707 /** @brief Macros to enable or disable the main PLL. 02708 * @note After enabling the main PLL, the application software should wait on 02709 * PLLRDY flag to be set indicating that PLL clock is stable and can 02710 * be used as system clock source. 02711 * @note The main PLL can not be disabled if it is used as system clock source 02712 * @note The main PLL is disabled by hardware when entering STOP and STANDBY modes. 02713 * @retval None 02714 */ 02715 #define __HAL_RCC_PLL_ENABLE() SET_BIT(RCC->CR, RCC_CR_PLLON) 02716 02717 #define __HAL_RCC_PLL_DISABLE() CLEAR_BIT(RCC->CR, RCC_CR_PLLON) 02718 02719 /** @brief Macro to configure the PLL clock source. 02720 * @note This function must be used only when the main PLL is disabled. 02721 * @param __PLLSOURCE__: specifies the PLL entry clock source. 02722 * This parameter can be one of the following values: 02723 * @arg RCC_PLLSOURCE_NONE: No clock selected as PLL clock entry 02724 * @arg RCC_PLLSOURCE_MSI: MSI oscillator clock selected as PLL clock entry 02725 * @arg RCC_PLLSOURCE_HSI: HSI oscillator clock selected as PLL clock entry 02726 * @arg RCC_PLLSOURCE_HSE: HSE oscillator clock selected as PLL clock entry 02727 * @note This clock source is common for the main PLL and audio PLL (PLLSAI1 and PLLSAI2). 02728 * @retval None 02729 * 02730 */ 02731 #define __HAL_RCC_PLL_PLLSOURCE_CONFIG(__PLLSOURCE__) \ 02732 MODIFY_REG(RCC->PLLCFGR, RCC_PLLCFGR_PLLSRC, (__PLLSOURCE__)) 02733 02734 /** @brief Macro to configure the PLL multiplication factor. 02735 * @note This function must be used only when the main PLL is disabled. 02736 * @param __PLLM__: specifies the division factor for PLL VCO input clock 02737 * This parameter must be a number between Min_Data = 1 and Max_Data = 8. 02738 * @note You have to set the PLLM parameter correctly to ensure that the VCO input 02739 * frequency ranges from 4 to 16 MHz. It is recommended to select a frequency 02740 * of 16 MHz to limit PLL jitter. 02741 * @retval None 02742 * 02743 */ 02744 #define __HAL_RCC_PLL_PLLM_CONFIG(__PLLM__) \ 02745 MODIFY_REG(RCC->PLLCFGR, RCC_PLLCFGR_PLLM, ((__PLLM__) - 1) << 4U) 02746 02747 /** 02748 * @brief Macro to configure the main PLL clock source, multiplication and division factors. 02749 * @note This function must be used only when the main PLL is disabled. 02750 * 02751 * @param __PLLSOURCE__: specifies the PLL entry clock source. 02752 * This parameter can be one of the following values: 02753 * @arg RCC_PLLSOURCE_NONE: No clock selected as PLL clock entry 02754 * @arg RCC_PLLSOURCE_MSI: MSI oscillator clock selected as PLL clock entry 02755 * @arg RCC_PLLSOURCE_HSI: HSI oscillator clock selected as PLL clock entry 02756 * @arg RCC_PLLSOURCE_HSE: HSE oscillator clock selected as PLL clock entry 02757 * @note This clock source is common for the main PLL and audio PLL (PLLSAI1 and PLLSAI2). 02758 * 02759 * @param __PLLM__: specifies the division factor for PLL VCO input clock. 02760 * This parameter must be a number between 1 and 8. 02761 * @note You have to set the PLLM parameter correctly to ensure that the VCO input 02762 * frequency ranges from 4 to 16 MHz. It is recommended to select a frequency 02763 * of 16 MHz to limit PLL jitter. 02764 * 02765 * @param __PLLN__: specifies the multiplication factor for PLL VCO output clock. 02766 * This parameter must be a number between 8 and 86. 02767 * @note You have to set the PLLN parameter correctly to ensure that the VCO 02768 * output frequency is between 64 and 344 MHz. 02769 * 02770 * @param __PLLP__: specifies the division factor for SAI clock. 02771 * This parameter must be a number in the range (7 or 17). 02772 * 02773 * @param __PLLQ__: specifies the division factor for OTG FS, SDMMC1 and RNG clocks. 02774 * This parameter must be in the range (2, 4, 6 or 8). 02775 * @note If the USB OTG FS is used in your application, you have to set the 02776 * PLLQ parameter correctly to have 48 MHz clock for the USB. However, 02777 * the SDMMC1 and RNG need a frequency lower than or equal to 48 MHz to work 02778 * correctly. 02779 * @param __PLLR__: specifies the division factor for the main system clock. 02780 * @note You have to set the PLLR parameter correctly to not exceed 80MHZ. 02781 * This parameter must be in the range (2, 4, 6 or 8). 02782 * @retval None 02783 */ 02784 #define __HAL_RCC_PLL_CONFIG(__PLLSOURCE__, __PLLM__, __PLLN__, __PLLP__, __PLLQ__,__PLLR__ ) \ 02785 (RCC->PLLCFGR = (uint32_t)(((__PLLM__) - 1) << 4U) | (uint32_t)((__PLLN__) << 8U) | (uint32_t)(((__PLLP__) >> 4U ) << 17U) | \ 02786 (uint32_t)(__PLLSOURCE__) | (uint32_t)((((__PLLQ__) >> 1U) - 1) << 21U) | (uint32_t)((((__PLLR__) >> 1U) - 1) << 25U)) 02787 02788 02789 /** @brief Macro to get the oscillator used as PLL clock source. 02790 * @retval The oscillator used as PLL clock source. The returned value can be one 02791 * of the following: 02792 * - RCC_PLLSOURCE_NONE: No oscillator is used as PLL clock source. 02793 * - RCC_PLLSOURCE_MSI: MSI oscillator is used as PLL clock source. 02794 * - RCC_PLLSOURCE_HSI: HSI oscillator is used as PLL clock source. 02795 * - RCC_PLLSOURCE_HSE: HSE oscillator is used as PLL clock source. 02796 */ 02797 #define __HAL_RCC_GET_PLL_OSCSOURCE() ((uint32_t)(RCC->PLLCFGR & RCC_PLLCFGR_PLLSRC)) 02798 02799 /** 02800 * @brief Enable or disable each clock output (RCC_PLL_SYSCLK, RCC_PLL_48M1CLK, RCC_PLL_SAI3CLK) 02801 * @note Enabling/disabling clock outputs RCC_PLL_SAI3CLK and RCC_PLL_48M1CLK can be done at anytime 02802 * without the need to stop the PLL in order to save power. But RCC_PLL_SYSCLK cannot 02803 * be stopped if used as System Clock. 02804 * @param __PLLCLOCKOUT__: specifies the PLL clock to be output. 02805 * This parameter can be one or a combination of the following values: 02806 * @arg RCC_PLL_SAI3CLK: This clock is used to generate an accurate clock to achieve 02807 * high-quality audio performance on SAI interface in case. 02808 * @arg RCC_PLL_48M1CLK: This Clock is used to generate the clock for the USB OTG FS (48 MHz), 02809 * the random analog generator (<=48 MHz) and the SDMMC1 (<= 48 MHz). 02810 * @arg RCC_PLL_SYSCLK: This Clock is used to generate the high speed system clock (up to 80MHz) 02811 * @retval None 02812 */ 02813 #define __HAL_RCC_PLLCLKOUT_ENABLE(__PLLCLOCKOUT__) SET_BIT(RCC->PLLCFGR, (__PLLCLOCKOUT__)) 02814 02815 #define __HAL_RCC_PLLCLKOUT_DISABLE(__PLLCLOCKOUT__) CLEAR_BIT(RCC->PLLCFGR, (__PLLCLOCKOUT__)) 02816 02817 /** 02818 * @brief Get clock output enable status (RCC_PLL_SYSCLK, RCC_PLL_48M1CLK, RCC_PLL_SAI3CLK) 02819 * @param __PLLCLOCKOUT__: specifies the output PLL clock to be checked. 02820 * This parameter can be one of the following values: 02821 * @arg RCC_PLL_SAI3CLK: This clock is used to generate an accurate clock to achieve 02822 * high-quality audio performance on SAI interface in case. 02823 * @arg RCC_PLL_48M1CLK: This Clock is used to generate the clock for the USB OTG FS (48 MHz), 02824 * the random analog generator (<=48 MHz) and the SDMMC1 (<= 48 MHz). 02825 * @arg RCC_PLL_SYSCLK: This Clock is used to generate the high speed system clock (up to 80MHz) 02826 * @retval SET / RESET 02827 */ 02828 #define __HAL_RCC_GET_PLLCLKOUT_CONFIG(__PLLCLOCKOUT__) READ_BIT(RCC->PLLCFGR, (__PLLCLOCKOUT__)) 02829 02830 /** 02831 * @brief Macro to configure the system clock source. 02832 * @param __SYSCLKSOURCE__: specifies the system clock source. 02833 * This parameter can be one of the following values: 02834 * - RCC_SYSCLKSOURCE_MSI: MSI oscillator is used as system clock source. 02835 * - RCC_SYSCLKSOURCE_HSI: HSI oscillator is used as system clock source. 02836 * - RCC_SYSCLKSOURCE_HSE: HSE oscillator is used as system clock source. 02837 * - RCC_SYSCLKSOURCE_PLLCLK: PLL output is used as system clock source. 02838 * @retval None 02839 */ 02840 #define __HAL_RCC_SYSCLK_CONFIG(__SYSCLKSOURCE__) \ 02841 MODIFY_REG(RCC->CFGR, RCC_CFGR_SW, (__SYSCLKSOURCE__)) 02842 02843 /** @brief Macro to get the clock source used as system clock. 02844 * @retval The clock source used as system clock. The returned value can be one 02845 * of the following: 02846 * - RCC_SYSCLKSOURCE_STATUS_MSI: MSI used as system clock. 02847 * - RCC_SYSCLKSOURCE_STATUS_HSI: HSI used as system clock. 02848 * - RCC_SYSCLKSOURCE_STATUS_HSE: HSE used as system clock. 02849 * - RCC_SYSCLKSOURCE_STATUS_PLLCLK: PLL used as system clock. 02850 */ 02851 #define __HAL_RCC_GET_SYSCLK_SOURCE() ((uint32_t)(RCC->CFGR & RCC_CFGR_SWS)) 02852 02853 /** 02854 * @brief Macro to configure the External Low Speed oscillator (LSE) drive capability. 02855 * @note As the LSE is in the Backup domain and write access is denied to 02856 * this domain after reset, you have to enable write access using 02857 * HAL_PWR_EnableBkUpAccess() function before to configure the LSE 02858 * (to be done once after reset). 02859 * @param __LSEDRIVE__: specifies the new state of the LSE drive capability. 02860 * This parameter can be one of the following values: 02861 * @arg RCC_LSEDRIVE_LOW: LSE oscillator low drive capability. 02862 * @arg RCC_LSEDRIVE_MEDIUMLOW: LSE oscillator medium low drive capability. 02863 * @arg RCC_LSEDRIVE_MEDIUMHIGH: LSE oscillator medium high drive capability. 02864 * @arg RCC_LSEDRIVE_HIGH: LSE oscillator high drive capability. 02865 * @retval None 02866 */ 02867 #define __HAL_RCC_LSEDRIVE_CONFIG(__LSEDRIVE__) \ 02868 MODIFY_REG(RCC->BDCR, RCC_BDCR_LSEDRV, (uint32_t)(__LSEDRIVE__)) 02869 02870 /** 02871 * @brief Macro to configure the wake up from stop clock. 02872 * @param __STOPWUCLK__: specifies the clock source used after wake up from stop. 02873 * This parameter can be one of the following values: 02874 * @arg RCC_STOP_WAKEUPCLOCK_MSI: MSI selected as system clock source 02875 * @arg RCC_STOP_WAKEUPCLOCK_HSI: HSI selected as system clock source 02876 * @retval None 02877 */ 02878 #define __HAL_RCC_WAKEUPSTOP_CLK_CONFIG(__STOPWUCLK__) \ 02879 MODIFY_REG(RCC->CFGR, RCC_CFGR_STOPWUCK, (__STOPWUCLK__)) 02880 02881 02882 /** @brief Macro to configure the MCO clock. 02883 * @param __MCOCLKSOURCE__ specifies the MCO clock source. 02884 * This parameter can be one of the following values: 02885 * @arg RCC_MCO1SOURCE_NOCLOCK: MCO output disabled 02886 * @arg RCC_MCO1SOURCE_SYSCLK: system clock selected as MCO source 02887 * @arg RCC_MCO1SOURCE_MSI: MSI clock selected as MCO source 02888 * @arg RCC_MCO1SOURCE_HSI: HSI clock selected as MCO source 02889 * @arg RCC_MCO1SOURCE_HSE: HSE clock selected as MCO sourcee 02890 * @arg RCC_MCO1SOURCE_PLLCLK: main PLL clock selected as MCO source 02891 * @arg RCC_MCO1SOURCE_LSI: LSI clock selected as MCO source 02892 * @arg RCC_MCO1SOURCE_LSE: LSE clock selected as MCO source 02893 * @arg RCC_MCO1SOURCE_HSI48: HSI48 clock selected as MCO source for devices with HSI48 02894 * @param __MCODIV__ specifies the MCO clock prescaler. 02895 * This parameter can be one of the following values: 02896 * @arg RCC_MCODIV_1 MCO clock source is divided by 1 02897 * @arg RCC_MCODIV_2 MCO clock source is divided by 2 02898 * @arg RCC_MCODIV_4 MCO clock source is divided by 4 02899 * @arg RCC_MCODIV_8 MCO clock source is divided by 8 02900 * @arg RCC_MCODIV_16 MCO clock source is divided by 16 02901 */ 02902 #define __HAL_RCC_MCO1_CONFIG(__MCOCLKSOURCE__, __MCODIV__) \ 02903 MODIFY_REG(RCC->CFGR, (RCC_CFGR_MCOSEL | RCC_CFGR_MCO_PRE), ((__MCOCLKSOURCE__) | (__MCODIV__))) 02904 02905 /** @defgroup RCC_Flags_Interrupts_Management Flags Interrupts Management 02906 * @brief macros to manage the specified RCC Flags and interrupts. 02907 * @{ 02908 */ 02909 02910 /** @brief Enable RCC interrupt (Perform Byte access to RCC_CIR[14:8] bits to enable 02911 * the selected interrupts). 02912 * @param __INTERRUPT__: specifies the RCC interrupt sources to be enabled. 02913 * This parameter can be any combination of the following values: 02914 * @arg RCC_IT_LSIRDY: LSI ready interrupt 02915 * @arg RCC_IT_LSERDY: LSE ready interrupt 02916 * @arg RCC_IT_MSIRDY: HSI ready interrupt 02917 * @arg RCC_IT_HSIRDY: HSI ready interrupt 02918 * @arg RCC_IT_HSERDY: HSE ready interrupt 02919 * @arg RCC_IT_PLLRDY: main PLL ready interrupt 02920 * @arg RCC_IT_PLLSAI1RDY: PLLSAI1 ready interrupt 02921 * @arg RCC_IT_PLLSAI2RDY: PLLSAI2 ready interrupt 02922 * @arg RCC_IT_LSECSS: Clock security system interrupt 02923 * @retval None 02924 */ 02925 #define __HAL_RCC_ENABLE_IT(__INTERRUPT__) SET_BIT(RCC->CIER, (__INTERRUPT__)) 02926 02927 /** @brief Disable RCC interrupt (Perform Byte access to RCC_CIR[14:8] bits to disable 02928 * the selected interrupts). 02929 * @param __INTERRUPT__: specifies the RCC interrupt sources to be disabled. 02930 * This parameter can be any combination of the following values: 02931 * @arg RCC_IT_LSIRDY: LSI ready interrupt 02932 * @arg RCC_IT_LSERDY: LSE ready interrupt 02933 * @arg RCC_IT_MSIRDY: HSI ready interrupt 02934 * @arg RCC_IT_HSIRDY: HSI ready interrupt 02935 * @arg RCC_IT_HSERDY: HSE ready interrupt 02936 * @arg RCC_IT_PLLRDY: main PLL ready interrupt 02937 * @arg RCC_IT_PLLSAI1RDY: PLLSAI1 ready interrupt 02938 * @arg RCC_IT_PLLSAI2RDY: PLLSAI2 ready interrupt 02939 * @arg RCC_IT_LSECSS: Clock security system interrupt 02940 * @retval None 02941 */ 02942 #define __HAL_RCC_DISABLE_IT(__INTERRUPT__) CLEAR_BIT(RCC->CIER, (__INTERRUPT__)) 02943 02944 /** @brief Clear the RCC's interrupt pending bits (Perform Byte access to RCC_CIR[23:16] 02945 * bits to clear the selected interrupt pending bits. 02946 * @param __INTERRUPT__: specifies the interrupt pending bit to clear. 02947 * This parameter can be any combination of the following values: 02948 * @arg RCC_IT_LSIRDY: LSI ready interrupt 02949 * @arg RCC_IT_LSERDY: LSE ready interrupt 02950 * @arg RCC_IT_MSIRDY: MSI ready interrupt 02951 * @arg RCC_IT_HSIRDY: HSI ready interrupt 02952 * @arg RCC_IT_HSERDY: HSE ready interrupt 02953 * @arg RCC_IT_PLLRDY: main PLL ready interrupt 02954 * @arg RCC_IT_PLLSAI1RDY: PLLSAI1 ready interrupt 02955 * @arg RCC_IT_PLLSAI2RDY: PLLSAI2 ready interrupt 02956 * @arg RCC_IT_HSECSS: HSE Clock Security interrupt 02957 * @arg RCC_IT_LSECSS: Clock security system interrupt 02958 * @retval None 02959 */ 02960 #define __HAL_RCC_CLEAR_IT(__INTERRUPT__) (RCC->CICR = (__INTERRUPT__)) 02961 02962 /** @brief Check whether the RCC interrupt has occurred or not. 02963 * @param __INTERRUPT__: specifies the RCC interrupt source to check. 02964 * This parameter can be one of the following values: 02965 * @arg RCC_IT_LSIRDY: LSI ready interrupt 02966 * @arg RCC_IT_LSERDY: LSE ready interrupt 02967 * @arg RCC_IT_MSIRDY: MSI ready interrupt 02968 * @arg RCC_IT_HSIRDY: HSI ready interrupt 02969 * @arg RCC_IT_HSERDY: HSE ready interrupt 02970 * @arg RCC_IT_PLLRDY: main PLL ready interrupt 02971 * @arg RCC_IT_PLLSAI1RDY: PLLSAI1 ready interrupt 02972 * @arg RCC_IT_PLLSAI2RDY: PLLSAI2 ready interrupt 02973 * @arg RCC_IT_HSECSS: HSE Clock Security interrupt 02974 * @arg RCC_IT_LSECSS: Clock security system interrupt 02975 * @retval The new state of __INTERRUPT__ (TRUE or FALSE). 02976 */ 02977 #define __HAL_RCC_GET_IT(__INTERRUPT__) ((RCC->CIFR & (__INTERRUPT__)) == (__INTERRUPT__)) 02978 02979 /** @brief Set RMVF bit to clear the reset flags. 02980 * The reset flags are: RCC_FLAG_FWRRST, RCC_FLAG_OBLRST, RCC_FLAG_PINRST, RCC_FLAG_BORRST, 02981 * RCC_FLAG_SFTRST, RCC_FLAG_IWDGRST, RCC_FLAG_WWDGRST and RCC_FLAG_LPWRRST. 02982 * @retval None 02983 */ 02984 #define __HAL_RCC_CLEAR_RESET_FLAGS() (RCC->CSR |= RCC_CSR_RMVF) 02985 02986 /** @brief Check whether the selected RCC flag is set or not. 02987 * @param __FLAG__: specifies the flag to check. 02988 * This parameter can be one of the following values: 02989 * @arg RCC_FLAG_MSIRDY: MSI oscillator clock ready 02990 * @arg RCC_FLAG_HSIRDY: HSI oscillator clock ready 02991 * @arg RCC_FLAG_HSERDY: HSE oscillator clock ready 02992 * @arg RCC_FLAG_PLLRDY: main PLL clock ready 02993 * @arg RCC_FLAG_PLLSAI2RDY: PLLSAI2 clock ready 02994 * @arg RCC_FLAG_PLLSAI1RDY: PLLSAI1 clock ready 02995 * @arg RCC_FLAG_LSERDY: LSE oscillator clock ready 02996 * @arg RCC_FLAG_LSECSSD: Clock security system failure on LSE oscillator detection 02997 * @arg RCC_FLAG_LSIRDY: LSI oscillator clock ready 02998 * @arg RCC_FLAG_BORRST: BOR reset 02999 * @arg RCC_FLAG_OBLRST: OBLRST reset 03000 * @arg RCC_FLAG_PINRST: Pin reset 03001 * @arg RCC_FLAG_FWRST: FIREWALL reset 03002 * @arg RCC_FLAG_RMVF: Remove reset Flag 03003 * @arg RCC_FLAG_SFTRST: Software reset 03004 * @arg RCC_FLAG_IWDGRST: Independent Watchdog reset 03005 * @arg RCC_FLAG_WWDGRST: Window Watchdog reset 03006 * @arg RCC_FLAG_LPWRRST: Low Power reset 03007 * @retval The new state of __FLAG__ (TRUE or FALSE). 03008 */ 03009 #define __HAL_RCC_GET_FLAG(__FLAG__) (((((((__FLAG__) >> 5U) == 1U) ? RCC->CR : \ 03010 ((((__FLAG__) >> 5U) == 2U) ? RCC->BDCR : \ 03011 ((((__FLAG__) >> 5U) == 3U) ? RCC->CSR : RCC->CIFR))) & \ 03012 ((uint32_t)1 << ((__FLAG__) & RCC_FLAG_MASK))) != 0) \ 03013 ? 1 : 0) 03014 03015 /** 03016 * @} 03017 */ 03018 03019 /** 03020 * @} 03021 */ 03022 03023 /* Private constants ---------------------------------------------------------*/ 03024 /** @defgroup RCC_Private_Constants RCC Private Constants 03025 * @{ 03026 */ 03027 /* Defines used for Flags */ 03028 #define CR_REG_INDEX ((uint32_t)1) 03029 #define BDCR_REG_INDEX ((uint32_t)2) 03030 #define CSR_REG_INDEX ((uint32_t)3) 03031 03032 #define RCC_FLAG_MASK ((uint32_t)0x1F) 03033 /** 03034 * @} 03035 */ 03036 03037 /* Private macros ------------------------------------------------------------*/ 03038 /** @addtogroup RCC_Private_Macros 03039 * @{ 03040 */ 03041 03042 #define IS_RCC_OSCILLATORTYPE(__OSCILLATOR__) (((__OSCILLATOR__) == RCC_OSCILLATORTYPE_NONE) || \ 03043 (((__OSCILLATOR__) & RCC_OSCILLATORTYPE_HSE) == RCC_OSCILLATORTYPE_HSE) || \ 03044 (((__OSCILLATOR__) & RCC_OSCILLATORTYPE_HSI) == RCC_OSCILLATORTYPE_HSI) || \ 03045 (((__OSCILLATOR__) & RCC_OSCILLATORTYPE_MSI) == RCC_OSCILLATORTYPE_MSI) || \ 03046 (((__OSCILLATOR__) & RCC_OSCILLATORTYPE_LSI) == RCC_OSCILLATORTYPE_LSI) || \ 03047 (((__OSCILLATOR__) & RCC_OSCILLATORTYPE_LSE) == RCC_OSCILLATORTYPE_LSE)) 03048 03049 #define IS_RCC_HSE(__HSE__) (((__HSE__) == RCC_HSE_OFF) || ((__HSE__) == RCC_HSE_ON) || \ 03050 ((__HSE__) == RCC_HSE_BYPASS)) 03051 03052 #define IS_RCC_LSE(__LSE__) (((__LSE__) == RCC_LSE_OFF) || ((__LSE__) == RCC_LSE_ON) || \ 03053 ((__LSE__) == RCC_LSE_BYPASS)) 03054 03055 #define IS_RCC_HSI(__HSI__) (((__HSI__) == RCC_HSI_OFF) || ((__HSI__) == RCC_HSI_ON)) 03056 03057 #define IS_RCC_HSI_CALIBRATION_VALUE(__VALUE__) ((__VALUE__) <= (uint32_t)31) 03058 03059 #define IS_RCC_LSI(__LSI__) (((__LSI__) == RCC_LSI_OFF) || ((__LSI__) == RCC_LSI_ON)) 03060 03061 #define IS_RCC_MSI(__MSI__) (((__MSI__) == RCC_MSI_OFF) || ((__MSI__) == RCC_MSI_ON)) 03062 03063 #define IS_RCC_MSICALIBRATION_VALUE(__VALUE__) ((__VALUE__) <= (uint32_t)255) 03064 03065 #define IS_RCC_PLL(__PLL__) (((__PLL__) == RCC_PLL_NONE) ||((__PLL__) == RCC_PLL_OFF) || \ 03066 ((__PLL__) == RCC_PLL_ON)) 03067 03068 #define IS_RCC_PLLSOURCE(__SOURCE__) (((__SOURCE__) == RCC_PLLSOURCE_NONE) || \ 03069 ((__SOURCE__) == RCC_PLLSOURCE_MSI) || \ 03070 ((__SOURCE__) == RCC_PLLSOURCE_HSI) || \ 03071 ((__SOURCE__) == RCC_PLLSOURCE_HSE)) 03072 03073 #define IS_RCC_PLLM_VALUE(__VALUE__) ((__VALUE__) <= 8) 03074 03075 #define IS_RCC_PLLN_VALUE(__VALUE__) ((8 <= (__VALUE__)) && ((__VALUE__) <= 86)) 03076 03077 #define IS_RCC_PLLP_VALUE(__VALUE__) (((__VALUE__) == 7) || ((__VALUE__) == 17)) 03078 03079 #define IS_RCC_PLLQ_VALUE(__VALUE__) (((__VALUE__) == 2 ) || ((__VALUE__) == 4) || \ 03080 ((__VALUE__) == 6) || ((__VALUE__) == 8)) 03081 03082 #define IS_RCC_PLLR_VALUE(__VALUE__) (((__VALUE__) == 2 ) || ((__VALUE__) == 4) || \ 03083 ((__VALUE__) == 6) || ((__VALUE__) == 8)) 03084 03085 #define IS_RCC_PLLSAI1CLOCKOUT_VALUE(__VALUE__) (((((__VALUE__) & RCC_PLLSAI1_SAI1CLK) == RCC_PLLSAI1_SAI1CLK) || \ 03086 (((__VALUE__) & RCC_PLLSAI1_48M2CLK) == RCC_PLLSAI1_48M2CLK) || \ 03087 (((__VALUE__) & RCC_PLLSAI1_ADC1CLK) == RCC_PLLSAI1_ADC1CLK)) && \ 03088 (((__VALUE__) & ~(RCC_PLLSAI1_SAI1CLK|RCC_PLLSAI1_48M2CLK|RCC_PLLSAI1_ADC1CLK)) == 0)) 03089 03090 #define IS_RCC_PLLSAI2CLOCKOUT_VALUE(__VALUE__) (((((__VALUE__) & RCC_PLLSAI2_SAI2CLK) == RCC_PLLSAI2_SAI2CLK ) || \ 03091 (((__VALUE__) & RCC_PLLSAI2_ADC2CLK) == RCC_PLLSAI2_ADC2CLK)) && \ 03092 (((__VALUE__) & ~(RCC_PLLSAI2_SAI2CLK|RCC_PLLSAI2_ADC2CLK)) == 0)) 03093 03094 #define IS_RCC_MSI_CLOCK_RANGE(__RANGE__) (((__RANGE__) == RCC_MSIRANGE_0) || \ 03095 ((__RANGE__) == RCC_MSIRANGE_1) || \ 03096 ((__RANGE__) == RCC_MSIRANGE_2) || \ 03097 ((__RANGE__) == RCC_MSIRANGE_3) || \ 03098 ((__RANGE__) == RCC_MSIRANGE_4) || \ 03099 ((__RANGE__) == RCC_MSIRANGE_5) || \ 03100 ((__RANGE__) == RCC_MSIRANGE_6) || \ 03101 ((__RANGE__) == RCC_MSIRANGE_7) || \ 03102 ((__RANGE__) == RCC_MSIRANGE_8) || \ 03103 ((__RANGE__) == RCC_MSIRANGE_9) || \ 03104 ((__RANGE__) == RCC_MSIRANGE_10) || \ 03105 ((__RANGE__) == RCC_MSIRANGE_11)) 03106 03107 #define IS_RCC_MSI_STANDBY_CLOCK_RANGE(__RANGE__) (((__RANGE__) == RCC_MSIRANGE_4) || \ 03108 ((__RANGE__) == RCC_MSIRANGE_5) || \ 03109 ((__RANGE__) == RCC_MSIRANGE_6) || \ 03110 ((__RANGE__) == RCC_MSIRANGE_7)) 03111 03112 #define IS_RCC_CLOCKTYPE(__CLK__) ((1 <= (__CLK__)) && ((__CLK__) <= 15)) 03113 03114 #define IS_RCC_SYSCLKSOURCE(__SOURCE__) (((__SOURCE__) == RCC_SYSCLKSOURCE_MSI) || \ 03115 ((__SOURCE__) == RCC_SYSCLKSOURCE_HSI) || \ 03116 ((__SOURCE__) == RCC_SYSCLKSOURCE_HSE) || \ 03117 ((__SOURCE__) == RCC_SYSCLKSOURCE_PLLCLK)) 03118 03119 #define IS_RCC_HCLK(__HCLK__) (((__HCLK__) == RCC_SYSCLK_DIV1) || ((__HCLK__) == RCC_SYSCLK_DIV2) || \ 03120 ((__HCLK__) == RCC_SYSCLK_DIV4) || ((__HCLK__) == RCC_SYSCLK_DIV8) || \ 03121 ((__HCLK__) == RCC_SYSCLK_DIV16) || ((__HCLK__) == RCC_SYSCLK_DIV64) || \ 03122 ((__HCLK__) == RCC_SYSCLK_DIV128) || ((__HCLK__) == RCC_SYSCLK_DIV256) || \ 03123 ((__HCLK__) == RCC_SYSCLK_DIV512)) 03124 03125 #define IS_RCC_PCLK(__PCLK__) (((__PCLK__) == RCC_HCLK_DIV1) || ((__PCLK__) == RCC_HCLK_DIV2) || \ 03126 ((__PCLK__) == RCC_HCLK_DIV4) || ((__PCLK__) == RCC_HCLK_DIV8) || \ 03127 ((__PCLK__) == RCC_HCLK_DIV16)) 03128 03129 #define IS_RCC_RTCCLKSOURCE(__SOURCE__) (((__SOURCE__) == RCC_RTCCLKSOURCE_LSE) || \ 03130 ((__SOURCE__) == RCC_RTCCLKSOURCE_LSI) || \ 03131 ((__SOURCE__) == RCC_RTCCLKSOURCE_HSE_DIV32)) 03132 03133 #define IS_RCC_MCO(__MCOX__) ((__MCOX__) == RCC_MCO1) 03134 03135 #define IS_RCC_MCO1SOURCE(__SOURCE__) (((__SOURCE__) == RCC_MCO1SOURCE_NOCLOCK) || \ 03136 ((__SOURCE__) == RCC_MCO1SOURCE_SYSCLK) || \ 03137 ((__SOURCE__) == RCC_MCO1SOURCE_MSI) || \ 03138 ((__SOURCE__) == RCC_MCO1SOURCE_HSI) || \ 03139 ((__SOURCE__) == RCC_MCO1SOURCE_HSE) || \ 03140 ((__SOURCE__) == RCC_MCO1SOURCE_PLLCLK) || \ 03141 ((__SOURCE__) == RCC_MCO1SOURCE_LSI) || \ 03142 ((__SOURCE__) == RCC_MCO1SOURCE_LSE)) 03143 03144 #define IS_RCC_MCODIV(__DIV__) (((__DIV__) == RCC_MCODIV_1) || ((__DIV__) == RCC_MCODIV_2) || \ 03145 ((__DIV__) == RCC_MCODIV_4) || ((__DIV__) == RCC_MCODIV_8) || \ 03146 ((__DIV__) == RCC_MCODIV_16)) 03147 03148 #define IS_RCC_LSE_DRIVE(__DRIVE__) (((__DRIVE__) == RCC_LSEDRIVE_LOW) || \ 03149 ((__DRIVE__) == RCC_LSEDRIVE_MEDIUMLOW) || \ 03150 ((__DRIVE__) == RCC_LSEDRIVE_MEDIUMHIGH) || \ 03151 ((__DRIVE__) == RCC_LSEDRIVE_HIGH)) 03152 03153 #define IS_RCC_STOP_WAKEUPCLOCK(__SOURCE__) (((__SOURCE__) == RCC_STOP_WAKEUPCLOCK_MSI) || \ 03154 ((__SOURCE__) == RCC_STOP_WAKEUPCLOCK_HSI)) 03155 /** 03156 * @} 03157 */ 03158 03159 /* Include RCC HAL Extended module */ 03160 #include "stm32l4xx_hal_rcc_ex.h" 03161 03162 /* Exported functions --------------------------------------------------------*/ 03163 /** @addtogroup RCC_Exported_Functions 03164 * @{ 03165 */ 03166 03167 03168 /** @addtogroup RCC_Exported_Functions_Group1 03169 * @{ 03170 */ 03171 03172 /* Initialization and de-initialization functions ******************************/ 03173 void HAL_RCC_DeInit(void); 03174 HAL_StatusTypeDef HAL_RCC_OscConfig(RCC_OscInitTypeDef *RCC_OscInitStruct); 03175 HAL_StatusTypeDef HAL_RCC_ClockConfig(RCC_ClkInitTypeDef *RCC_ClkInitStruct, uint32_t FLatency); 03176 03177 /** 03178 * @} 03179 */ 03180 03181 /** @addtogroup RCC_Exported_Functions_Group2 03182 * @{ 03183 */ 03184 03185 /* Peripheral Control functions ************************************************/ 03186 void HAL_RCC_MCOConfig(uint32_t RCC_MCOx, uint32_t RCC_MCOSource, uint32_t RCC_MCODiv); 03187 void HAL_RCC_EnableCSS(void); 03188 uint32_t HAL_RCC_GetSysClockFreq(void); 03189 uint32_t HAL_RCC_GetHCLKFreq(void); 03190 uint32_t HAL_RCC_GetPCLK1Freq(void); 03191 uint32_t HAL_RCC_GetPCLK2Freq(void); 03192 void HAL_RCC_GetOscConfig(RCC_OscInitTypeDef *RCC_OscInitStruct); 03193 void HAL_RCC_GetClockConfig(RCC_ClkInitTypeDef *RCC_ClkInitStruct, uint32_t *pFLatency); 03194 /* CSS NMI IRQ handler */ 03195 void HAL_RCC_NMI_IRQHandler(void); 03196 /* User Callbacks in non blocking mode (IT mode) */ 03197 void HAL_RCC_CSSCallback(void); 03198 03199 /** 03200 * @} 03201 */ 03202 03203 /** 03204 * @} 03205 */ 03206 03207 /** 03208 * @} 03209 */ 03210 03211 /** 03212 * @} 03213 */ 03214 03215 #ifdef __cplusplus 03216 } 03217 #endif 03218 03219 #endif /* __STM32L4xx_HAL_RCC_H */ 03220 03221 /************************ (C) COPYRIGHT STMicroelectronics *****END OF FILE****/ 03222
Generated on Tue Jul 12 2022 11:35:14 by
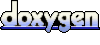