Hal Drivers for L4
Dependents: BSP OneHopeOnePrayer FINAL_AUDIO_RECORD AudioDemo
Fork of STM32L4xx_HAL_Driver by
stm32l4xx_hal_pwr.c
00001 /** 00002 ****************************************************************************** 00003 * @file stm32l4xx_hal_pwr.c 00004 * @author MCD Application Team 00005 * @version V1.1.0 00006 * @date 16-September-2015 00007 * @brief PWR HAL module driver. 00008 * This file provides firmware functions to manage the following 00009 * functionalities of the Power Controller (PWR) peripheral: 00010 * + Initialization/de-initialization functions 00011 * + Peripheral Control functions 00012 * 00013 ****************************************************************************** 00014 * @attention 00015 * 00016 * <h2><center>© COPYRIGHT(c) 2015 STMicroelectronics</center></h2> 00017 * 00018 * Redistribution and use in source and binary forms, with or without modification, 00019 * are permitted provided that the following conditions are met: 00020 * 1. Redistributions of source code must retain the above copyright notice, 00021 * this list of conditions and the following disclaimer. 00022 * 2. Redistributions in binary form must reproduce the above copyright notice, 00023 * this list of conditions and the following disclaimer in the documentation 00024 * and/or other materials provided with the distribution. 00025 * 3. Neither the name of STMicroelectronics nor the names of its contributors 00026 * may be used to endorse or promote products derived from this software 00027 * without specific prior written permission. 00028 * 00029 * THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" 00030 * AND ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE 00031 * IMPLIED WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE 00032 * DISCLAIMED. IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE 00033 * FOR ANY DIRECT, INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL 00034 * DAMAGES (INCLUDING, BUT NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR 00035 * SERVICES; LOSS OF USE, DATA, OR PROFITS; OR BUSINESS INTERRUPTION) HOWEVER 00036 * CAUSED AND ON ANY THEORY OF LIABILITY, WHETHER IN CONTRACT, STRICT LIABILITY, 00037 * OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) ARISING IN ANY WAY OUT OF THE USE 00038 * OF THIS SOFTWARE, EVEN IF ADVISED OF THE POSSIBILITY OF SUCH DAMAGE. 00039 * 00040 ****************************************************************************** 00041 */ 00042 00043 /* Includes ------------------------------------------------------------------*/ 00044 #include "stm32l4xx_hal.h" 00045 00046 /** @addtogroup STM32L4xx_HAL_Driver 00047 * @{ 00048 */ 00049 00050 /** @defgroup PWR PWR 00051 * @brief PWR HAL module driver 00052 * @{ 00053 */ 00054 00055 #ifdef HAL_PWR_MODULE_ENABLED 00056 00057 /* Private typedef -----------------------------------------------------------*/ 00058 /* Private define ------------------------------------------------------------*/ 00059 00060 /** @defgroup PWR_Private_Defines PWR Private Defines 00061 * @{ 00062 */ 00063 00064 /** @defgroup PWR_PVD_Mode_Mask PWR PVD Mode Mask 00065 * @{ 00066 */ 00067 #define PVD_MODE_IT ((uint32_t)0x00010000) /*!< Mask for interruption yielded by PVD threshold crossing */ 00068 #define PVD_MODE_EVT ((uint32_t)0x00020000) /*!< Mask for event yielded by PVD threshold crossing */ 00069 #define PVD_RISING_EDGE ((uint32_t)0x00000001) /*!< Mask for rising edge set as PVD trigger */ 00070 #define PVD_FALLING_EDGE ((uint32_t)0x00000002) /*!< Mask for falling edge set as PVD trigger */ 00071 /** 00072 * @} 00073 */ 00074 00075 /** 00076 * @} 00077 */ 00078 00079 /* Private macro -------------------------------------------------------------*/ 00080 /* Private variables ---------------------------------------------------------*/ 00081 /* Private function prototypes -----------------------------------------------*/ 00082 /* Exported functions --------------------------------------------------------*/ 00083 00084 /** @defgroup PWR_Exported_Functions PWR Exported Functions 00085 * @{ 00086 */ 00087 00088 /** @defgroup PWR_Exported_Functions_Group1 Initialization and de-initialization functions 00089 * @brief Initialization and de-initialization functions 00090 * 00091 @verbatim 00092 =============================================================================== 00093 ##### Initialization and de-initialization functions ##### 00094 =============================================================================== 00095 [..] 00096 00097 @endverbatim 00098 * @{ 00099 */ 00100 00101 /** 00102 * @brief Deinitialize the HAL PWR peripheral registers to their default reset values. 00103 * @retval None 00104 */ 00105 void HAL_PWR_DeInit(void) 00106 { 00107 __HAL_RCC_PWR_FORCE_RESET(); 00108 __HAL_RCC_PWR_RELEASE_RESET(); 00109 } 00110 00111 /** 00112 * @brief Enable access to the backup domain 00113 * (RTC registers, RTC backup data registers). 00114 * @note After reset, the backup domain is protected against 00115 * possible unwanted write accesses. 00116 * @note RTCSEL that sets the RTC clock source selection is in the RTC back-up domain. 00117 * In order to set or modify the RTC clock, the backup domain access must be 00118 * disabled. 00119 * @note LSEON bit that switches on and off the LSE crystal belongs as well to the 00120 * back-up domain. 00121 * @retval None 00122 */ 00123 void HAL_PWR_EnableBkUpAccess(void) 00124 { 00125 SET_BIT(PWR->CR1, PWR_CR1_DBP); 00126 } 00127 00128 /** 00129 * @brief Disable access to the backup domain 00130 * (RTC registers, RTC backup data registers). 00131 * @retval None 00132 */ 00133 void HAL_PWR_DisableBkUpAccess(void) 00134 { 00135 CLEAR_BIT(PWR->CR1, PWR_CR1_DBP); 00136 } 00137 00138 00139 00140 00141 /** 00142 * @} 00143 */ 00144 00145 00146 00147 /** @defgroup PWR_Exported_Functions_Group2 Peripheral Control functions 00148 * @brief Low Power modes configuration functions 00149 * 00150 @verbatim 00151 00152 =============================================================================== 00153 ##### Peripheral Control functions ##### 00154 =============================================================================== 00155 00156 [..] 00157 *** PVD configuration *** 00158 ========================= 00159 [..] 00160 (+) The PVD is used to monitor the VDD power supply by comparing it to a 00161 threshold selected by the PVD Level (PLS[2:0] bits in PWR_CR2 register). 00162 00163 (+) PVDO flag is available to indicate if VDD/VDDA is higher or lower 00164 than the PVD threshold. This event is internally connected to the EXTI 00165 line16 and can generate an interrupt if enabled. This is done through 00166 __HAL_PVD_EXTI_ENABLE_IT() macro. 00167 (+) The PVD is stopped in Standby mode. 00168 00169 00170 *** WakeUp pin configuration *** 00171 ================================ 00172 [..] 00173 (+) WakeUp pins are used to wakeup the system from Standby mode or Shutdown mode. 00174 The polarity of these pins can be set to configure event detection on high 00175 level (rising edge) or low level (falling edge). 00176 00177 00178 00179 *** Low Power modes configuration *** 00180 ===================================== 00181 [..] 00182 The devices feature 8 low-power modes: 00183 (+) Low-power Run mode: core and peripherals are running, regulator in low power mode. 00184 (+) Sleep mode: Cortex-M4 core stopped, peripherals kept running, regulator in normal mode. 00185 (+) Low-power Sleep mode: Cortex-M4 core stopped, peripherals kept running, regulator in low power mode. 00186 (+) Stop 1 mode: all clocks are stopped except LSI and LSE, regulator in normal or low power mode. 00187 (+) Stop 2 mode: all clocks are stopped except LSI and LSE, regulator in low power mode, reduced set of waking up IPs compared to Stop 1 mode. 00188 (+) Standby mode with SRAM2: all clocks are stopped except LSI and LSE, SRAM2 content preserved, regulator in low power mode. 00189 (+) Standby mode without SRAM2: all clocks are stopped except LSI and LSE, regulator off. 00190 (+) Shutdown mode: all clocks are stopped except LSE, regulator off. 00191 00192 00193 *** Low-power run mode *** 00194 ========================== 00195 [..] 00196 (+) Entry: (from main run mode) 00197 (++) set LPR bit with HAL_PWREx_EnableLowPowerRunMode() API after having decreased the system clock below 2 MHz. 00198 00199 (+) Exit: 00200 (++) clear LPR bit then wait for REGLP bit to be reset with HAL_PWREx_DisableLowPowerRunMode() API. Only 00201 then can the system clock frequency be increased above 2 MHz. 00202 00203 00204 *** Sleep mode / Low-power sleep mode *** 00205 ========================================= 00206 [..] 00207 (+) Entry: 00208 The Sleep mode / Low-power Sleep mode is entered thru HAL_PWR_EnterSLEEPMode() API 00209 in specifying whether or not the regulator is forced to low-power mode and if exit is interrupt or event-triggered. 00210 (++) PWR_MAINREGULATOR_ON: Sleep mode (regulator in main mode). 00211 (++) PWR_LOWPOWERREGULATOR_ON: Low-power sleep (regulator in low power mode). 00212 In the latter case, the system clock frequency must have been decreased below 2 MHz beforehand. 00213 (++) PWR_SLEEPENTRY_WFI: enter SLEEP mode with WFI instruction 00214 (++) PWR_SLEEPENTRY_WFE: enter SLEEP mode with WFE instruction 00215 00216 (+) WFI Exit: 00217 (++) Any peripheral interrupt acknowledged by the nested vectored interrupt 00218 controller (NVIC) or any wake-up event. 00219 00220 (+) WFE Exit: 00221 (++) Any wake-up event such as an EXTI line configured in event mode. 00222 00223 [..] When exiting the Low-power sleep mode by issuing an interrupt or a wakeup event, 00224 the MCU is in Low-power Run mode. 00225 00226 *** Stop 1 and Stop 2 modes *** 00227 =============================== 00228 [..] 00229 (+) Entry: 00230 The Stop 1 or Stop 2 modes are entered thru the following API's: 00231 (++) HAL_PWR_EnterSTOPMode() [for legacy porting reasons] or HAL_PWREx_EnterSTOP1Mode() for mode 1 00232 (++) HAL_PWREx_EnterSTOP2Mode for mode 2. 00233 (+) Regulator setting (applicable to Stop 1 mode only): 00234 (++) PWR_MAINREGULATOR_ON 00235 (++) PWR_LOWPOWERREGULATOR_ON 00236 (+) Exit (interrupt or event-triggered, specified when entering STOP mode): 00237 (++) PWR_STOPENTRY_WFI: enter Stop mode with WFI instruction 00238 (++) PWR_STOPENTRY_WFE: enter Stop mode with WFE instruction 00239 00240 (+) WFI Exit: 00241 (++) Any EXTI Line (Internal or External) configured in Interrupt mode. 00242 (++) Some specific communication peripherals (USART, LPUART, I2C) interrupts 00243 when programmed in wakeup mode. 00244 (+) WFE Exit: 00245 (++) Any EXTI Line (Internal or External) configured in Event mode. 00246 00247 [..] 00248 When exiting Stop 1 mode, the MCU is either in Run mode or in Low-power Run mode 00249 depending on the LPR bit setting. 00250 When exiting Stop 2 mode, the MCU is in Run mode. 00251 00252 *** Standby mode *** 00253 ==================== 00254 [..] 00255 The Standby mode offers two options: 00256 (+) option a) all clocks off except LSI and LSE, RRS bit set (keeps voltage regulator in low power mode). 00257 SRAM and registers contents are lost except for the SRAM2 content, the RTC registers, RTC backup registers 00258 and Standby circuitry. 00259 (+) option b) all clocks off except LSI and LSE, RRS bit cleared (voltage regulator then disabled). 00260 SRAM and register contents are lost except for the RTC registers, RTC backup registers 00261 and Standby circuitry. 00262 00263 (++) Entry: 00264 (+++) The Standby mode is entered thru HAL_PWR_EnterSTANDBYMode() API. 00265 SRAM1 and register contents are lost except for registers in the Backup domain and 00266 Standby circuitry. SRAM2 content can be preserved if the bit RRS is set in PWR_CR3 register. 00267 To enable this feature, the user can resort to HAL_PWREx_EnableSRAM2ContentRetention() API 00268 to set RRS bit. 00269 00270 (++) Exit: 00271 (+++) WKUP pin rising edge, RTC alarm or wakeup, tamper event, time-stamp event, 00272 external reset in NRST pin, IWDG reset. 00273 00274 [..] After waking up from Standby mode, program execution restarts in the same way as after a Reset. 00275 00276 00277 *** Shutdown mode *** 00278 ====================== 00279 [..] 00280 In Shutdown mode, 00281 voltage regulator is disabled, all clocks are off except LSE, RRS bit is cleared. 00282 SRAM and registers contents are lost except for backup domain registers. 00283 00284 (+) Entry: 00285 The Shutdown mode is entered thru HAL_PWREx_EnterSHUTDOWNMode() API. 00286 00287 (+) Exit: 00288 (++) WKUP pin rising edge, RTC alarm or wakeup, tamper event, time-stamp event, 00289 external reset in NRST pin. 00290 00291 [..] After waking up from Shutdown mode, program execution restarts in the same way as after a Reset. 00292 00293 00294 *** Auto-wakeup (AWU) from low-power mode *** 00295 ============================================= 00296 [..] 00297 The MCU can be woken up from low-power mode by an RTC Alarm event, an RTC 00298 Wakeup event, a tamper event or a time-stamp event, without depending on 00299 an external interrupt (Auto-wakeup mode). 00300 00301 (+) RTC auto-wakeup (AWU) from the Stop, Standby and Shutdown modes 00302 00303 00304 (++) To wake up from the Stop mode with an RTC alarm event, it is necessary to 00305 configure the RTC to generate the RTC alarm using the HAL_RTC_SetAlarm_IT() function. 00306 00307 (++) To wake up from the Stop mode with an RTC Tamper or time stamp event, it 00308 is necessary to configure the RTC to detect the tamper or time stamp event using the 00309 HAL_RTCEx_SetTimeStamp_IT() or HAL_RTCEx_SetTamper_IT() functions. 00310 00311 (++) To wake up from the Stop mode with an RTC WakeUp event, it is necessary to 00312 configure the RTC to generate the RTC WakeUp event using the HAL_RTCEx_SetWakeUpTimer_IT() function. 00313 00314 @endverbatim 00315 * @{ 00316 */ 00317 00318 00319 00320 /** 00321 * @brief Configure the voltage threshold detected by the Power Voltage Detector (PVD). 00322 * @param sConfigPVD: pointer to a PWR_PVDTypeDef structure that contains the PVD 00323 * configuration information. 00324 * @note Refer to the electrical characteristics of your device datasheet for 00325 * more details about the voltage thresholds corresponding to each 00326 * detection level. 00327 * @retval None 00328 */ 00329 HAL_StatusTypeDef HAL_PWR_ConfigPVD(PWR_PVDTypeDef *sConfigPVD) 00330 { 00331 /* Check the parameters */ 00332 assert_param(IS_PWR_PVD_LEVEL(sConfigPVD->PVDLevel)); 00333 assert_param(IS_PWR_PVD_MODE(sConfigPVD->Mode)); 00334 00335 /* Set PLS bits according to PVDLevel value */ 00336 MODIFY_REG(PWR->CR2, PWR_CR2_PLS, sConfigPVD->PVDLevel); 00337 00338 /* Clear any previous config. Keep it clear if no event or IT mode is selected */ 00339 __HAL_PWR_PVD_EXTI_DISABLE_EVENT(); 00340 __HAL_PWR_PVD_EXTI_DISABLE_IT(); 00341 __HAL_PWR_PVD_EXTI_DISABLE_FALLING_EDGE(); 00342 __HAL_PWR_PVD_EXTI_DISABLE_RISING_EDGE(); 00343 00344 /* Configure interrupt mode */ 00345 if((sConfigPVD->Mode & PVD_MODE_IT) == PVD_MODE_IT) 00346 { 00347 __HAL_PWR_PVD_EXTI_ENABLE_IT(); 00348 } 00349 00350 /* Configure event mode */ 00351 if((sConfigPVD->Mode & PVD_MODE_EVT) == PVD_MODE_EVT) 00352 { 00353 __HAL_PWR_PVD_EXTI_ENABLE_EVENT(); 00354 } 00355 00356 /* Configure the edge */ 00357 if((sConfigPVD->Mode & PVD_RISING_EDGE) == PVD_RISING_EDGE) 00358 { 00359 __HAL_PWR_PVD_EXTI_ENABLE_RISING_EDGE(); 00360 } 00361 00362 if((sConfigPVD->Mode & PVD_FALLING_EDGE) == PVD_FALLING_EDGE) 00363 { 00364 __HAL_PWR_PVD_EXTI_ENABLE_FALLING_EDGE(); 00365 } 00366 00367 return HAL_OK; 00368 } 00369 00370 00371 /** 00372 * @brief Enable the Power Voltage Detector (PVD). 00373 * @retval None 00374 */ 00375 void HAL_PWR_EnablePVD(void) 00376 { 00377 SET_BIT(PWR->CR2, PWR_CR2_PVDE); 00378 } 00379 00380 /** 00381 * @brief Disable the Power Voltage Detector (PVD). 00382 * @retval None 00383 */ 00384 void HAL_PWR_DisablePVD(void) 00385 { 00386 CLEAR_BIT(PWR->CR2, PWR_CR2_PVDE); 00387 } 00388 00389 00390 00391 00392 /** 00393 * @brief Enable the WakeUp PINx functionality. 00394 * @param WakeUpPinPolarity: Specifies which Wake-Up pin to enable. 00395 * This parameter can be one of the following legacy values which set the default polarity 00396 * i.e. detection on high level (rising edge): 00397 * @arg PWR_WAKEUP_PIN1, PWR_WAKEUP_PIN2, PWR_WAKEUP_PIN3, PWR_WAKEUP_PIN4, PWR_WAKEUP_PIN5 00398 * 00399 * or one of the following value where the user can explicitly specify the enabled pin and 00400 * the chosen polarity: 00401 * @arg PWR_WAKEUP_PIN1_HIGH or PWR_WAKEUP_PIN1_LOW 00402 * @arg PWR_WAKEUP_PIN2_HIGH or PWR_WAKEUP_PIN2_LOW 00403 * @arg PWR_WAKEUP_PIN3_HIGH or PWR_WAKEUP_PIN3_LOW 00404 * @arg PWR_WAKEUP_PIN4_HIGH or PWR_WAKEUP_PIN4_LOW 00405 * @arg PWR_WAKEUP_PIN5_HIGH or PWR_WAKEUP_PIN5_LOW 00406 * @note PWR_WAKEUP_PINx and PWR_WAKEUP_PINx_HIGH are equivalent. 00407 * @retval None 00408 */ 00409 void HAL_PWR_EnableWakeUpPin(uint32_t WakeUpPinPolarity) 00410 { 00411 assert_param(IS_PWR_WAKEUP_PIN(WakeUpPinPolarity)); 00412 00413 /* Specifies the Wake-Up pin polarity for the event detection 00414 (rising or falling edge) */ 00415 MODIFY_REG(PWR->CR4, (PWR_CR3_EWUP & WakeUpPinPolarity), (WakeUpPinPolarity >> PWR_WUP_POLARITY_SHIFT)); 00416 00417 /* Enable wake-up pin */ 00418 SET_BIT(PWR->CR3, (PWR_CR3_EWUP & WakeUpPinPolarity)); 00419 00420 00421 } 00422 00423 /** 00424 * @brief Disable the WakeUp PINx functionality. 00425 * @param WakeUpPinx: Specifies the Power Wake-Up pin to disable. 00426 * This parameter can be one of the following values: 00427 * @arg PWR_WAKEUP_PIN1, PWR_WAKEUP_PIN2, PWR_WAKEUP_PIN3, PWR_WAKEUP_PIN4, PWR_WAKEUP_PIN5 00428 * @retval None 00429 */ 00430 void HAL_PWR_DisableWakeUpPin(uint32_t WakeUpPinx) 00431 { 00432 assert_param(IS_PWR_WAKEUP_PIN(WakeUpPinx)); 00433 00434 CLEAR_BIT(PWR->CR3, WakeUpPinx); 00435 } 00436 00437 00438 /** 00439 * @brief Enter Sleep or Low-power Sleep mode. 00440 * @note In Sleep/Low-power Sleep mode, all I/O pins keep the same state as in Run mode. 00441 * @param Regulator: Specifies the regulator state in Sleep/Low-power Sleep mode. 00442 * This parameter can be one of the following values: 00443 * @arg PWR_MAINREGULATOR_ON: Sleep mode (regulator in main mode) 00444 * @arg PWR_LOWPOWERREGULATOR_ON: Low-power Sleep mode (regulator in low-power mode) 00445 * @note Low-power Sleep mode is entered from Low-power Run mode. Therefore, if not yet 00446 * in Low-power Run mode before calling HAL_PWR_EnterSLEEPMode() with Regulator set 00447 * to PWR_LOWPOWERREGULATOR_ON, the user can optionally configure the 00448 * Flash in power-down monde in setting the SLEEP_PD bit in FLASH_ACR register. 00449 * Additionally, the clock frequency must be reduced below 2 MHz. 00450 * Setting SLEEP_PD in FLASH_ACR then appropriately reducing the clock frequency must 00451 * be done before calling HAL_PWR_EnterSLEEPMode() API. 00452 * @note When exiting Low-power Sleep mode, the MCU is in Low-power Run mode. To move in 00453 * Run mode, the user must resort to HAL_PWREx_DisableLowPowerRunMode() API. 00454 * @param SLEEPEntry: Specifies if Sleep mode is entered with WFI or WFE instruction. 00455 * This parameter can be one of the following values: 00456 * @arg PWR_SLEEPENTRY_WFI: enter Sleep or Low-power Sleep mode with WFI instruction 00457 * @arg PWR_SLEEPENTRY_WFE: enter Sleep or Low-power Sleep mode with WFE instruction 00458 * @note When WFI entry is used, tick interrupt have to be disabled if not desired as 00459 * the interrupt wake up source. 00460 * @retval None 00461 */ 00462 void HAL_PWR_EnterSLEEPMode(uint32_t Regulator, uint8_t SLEEPEntry) 00463 { 00464 /* Check the parameters */ 00465 assert_param(IS_PWR_REGULATOR(Regulator)); 00466 assert_param(IS_PWR_SLEEP_ENTRY(SLEEPEntry)); 00467 00468 /* Set Regulator parameter */ 00469 if (Regulator == PWR_MAINREGULATOR_ON) 00470 { 00471 /* If in low-power run mode at this point, exit it */ 00472 if (HAL_IS_BIT_SET(PWR->SR2, PWR_SR2_REGLPF)) 00473 { 00474 HAL_PWREx_DisableLowPowerRunMode(); 00475 } 00476 /* Regulator now in main mode. */ 00477 } 00478 else 00479 { 00480 /* If in run mode, first move to low-power run mode. 00481 The system clock frequency must be below 2 MHz at this point. */ 00482 if (HAL_IS_BIT_SET(PWR->SR2, PWR_SR2_REGLPF) == RESET) 00483 { 00484 HAL_PWREx_EnableLowPowerRunMode(); 00485 } 00486 } 00487 00488 /* Clear SLEEPDEEP bit of Cortex System Control Register */ 00489 CLEAR_BIT(SCB->SCR, ((uint32_t)SCB_SCR_SLEEPDEEP_Msk)); 00490 00491 /* Select SLEEP mode entry -------------------------------------------------*/ 00492 if(SLEEPEntry == PWR_SLEEPENTRY_WFI) 00493 { 00494 /* Request Wait For Interrupt */ 00495 __WFI(); 00496 } 00497 else 00498 { 00499 /* Request Wait For Event */ 00500 __SEV(); 00501 __WFE(); 00502 __WFE(); 00503 } 00504 00505 } 00506 00507 00508 /** 00509 * @brief Enter Stop 1 mode 00510 * @note This API is named HAL_PWR_EnterSTOPMode to ensure compatibility with legacy code running 00511 * on devices where only "Stop mode" is mentioned. On STM32L4, Stop 1 mode and Stop modes 00512 * are equivalent. 00513 * @note In Stop 1 mode, all I/O pins keep the same state as in Run mode. 00514 * @note All clocks in the VCORE domain are stopped; the PLL, the MSI, 00515 * the HSI and the HSE oscillators are disabled. Some peripherals with the wakeup capability 00516 * (I2Cx, USARTx and LPUART) can switch on the HSI to receive a frame, and switch off the HSI 00517 * after receiving the frame if it is not a wakeup frame. In this case, the HSI clock is propagated 00518 * only to the peripheral requesting it. 00519 * SRAM1, SRAM2 and register contents are preserved. 00520 * The BOR is available. 00521 * The voltage regulator can be configured either in normal or low-power mode. 00522 * @note When exiting Stop 1 mode by issuing an interrupt or a wakeup event, 00523 * the HSI RC oscillator is selected as system clock if STOPWUCK bit in RCC_CFGR register 00524 * is set; the MSI oscillator is selected if STOPWUCK is cleared. 00525 * @note When the voltage regulator operates in low power mode, an additional 00526 * startup delay is incurred when waking up from Stop 1 mode. 00527 * By keeping the internal regulator ON during Stop 1 mode, the consumption 00528 * is higher although the startup time is reduced. 00529 * @param Regulator: Specifies the regulator state in Stop 1 mode. 00530 * This parameter can be one of the following values: 00531 * @arg PWR_MAINREGULATOR_ON: Stop 1 mode with regulator ON 00532 * @arg PWR_LOWPOWERREGULATOR_ON: Stop 1 mode with low power regulator ON 00533 * @param STOPEntry: Specifies if Stop 1 mode in entered with WFI or WFE instruction. 00534 * This parameter can be one of the following values: 00535 * @arg PWR_STOPENTRY_WFI:Enter Stop 1 mode with WFI instruction 00536 * @arg PWR_STOPENTRY_WFE: Enter Stop 1 mode with WFE instruction 00537 * @retval None 00538 */ 00539 void HAL_PWR_EnterSTOPMode(uint32_t Regulator, uint8_t STOPEntry) 00540 { 00541 HAL_PWREx_EnterSTOP1Mode(Regulator, STOPEntry); 00542 } 00543 00544 /** 00545 * @brief Enter Standby mode. 00546 * @note In Standby mode, the PLL, the HSI, the MSI and the HSE oscillators are switched 00547 * off. The voltage regulator is disabled, except when SRAM2 content is preserved 00548 * in which case the regulator is in low-power mode. 00549 * SRAM1 and register contents are lost except for registers in the Backup domain and 00550 * Standby circuitry. SRAM2 content can be preserved if the bit RRS is set in PWR_CR3 register. 00551 * To enable this feature, the user can resort to HAL_PWREx_EnableSRAM2ContentRetention() API 00552 * to set RRS bit. 00553 * The BOR is available. 00554 * @note The I/Os can be configured either with a pull-up or pull-down or can be kept in analog state. 00555 * HAL_PWREx_EnableGPIOPullUp() and HAL_PWREx_EnableGPIOPullDown() respectively enable Pull Up and 00556 * Pull Down state, HAL_PWREx_DisableGPIOPullUp() and HAL_PWREx_DisableGPIOPullDown() disable the 00557 * same. 00558 * These states are effective in Standby mode only if APC bit is set through 00559 * HAL_PWREx_EnablePullUpPullDownConfig() API. 00560 * @retval None 00561 */ 00562 void HAL_PWR_EnterSTANDBYMode(void) 00563 { 00564 /* Set Stand-by mode */ 00565 MODIFY_REG(PWR->CR1, PWR_CR1_LPMS, PWR_CR1_LPMS_STANDBY); 00566 00567 /* Set SLEEPDEEP bit of Cortex System Control Register */ 00568 SET_BIT(SCB->SCR, ((uint32_t)SCB_SCR_SLEEPDEEP_Msk)); 00569 00570 /* This option is used to ensure that store operations are completed */ 00571 #if defined ( __CC_ARM) 00572 __force_stores(); 00573 #endif 00574 /* Request Wait For Interrupt */ 00575 __WFI(); 00576 } 00577 00578 00579 00580 /** 00581 * @brief Indicate Sleep-On-Exit when returning from Handler mode to Thread mode. 00582 * @note Set SLEEPONEXIT bit of SCR register. When this bit is set, the processor 00583 * re-enters SLEEP mode when an interruption handling is over. 00584 * Setting this bit is useful when the processor is expected to run only on 00585 * interruptions handling. 00586 * @retval None 00587 */ 00588 void HAL_PWR_EnableSleepOnExit(void) 00589 { 00590 /* Set SLEEPONEXIT bit of Cortex System Control Register */ 00591 SET_BIT(SCB->SCR, ((uint32_t)SCB_SCR_SLEEPONEXIT_Msk)); 00592 } 00593 00594 00595 /** 00596 * @brief Disable Sleep-On-Exit feature when returning from Handler mode to Thread mode. 00597 * @note Clear SLEEPONEXIT bit of SCR register. When this bit is set, the processor 00598 * re-enters SLEEP mode when an interruption handling is over. 00599 * @retval None 00600 */ 00601 void HAL_PWR_DisableSleepOnExit(void) 00602 { 00603 /* Clear SLEEPONEXIT bit of Cortex System Control Register */ 00604 CLEAR_BIT(SCB->SCR, ((uint32_t)SCB_SCR_SLEEPONEXIT_Msk)); 00605 } 00606 00607 00608 00609 /** 00610 * @brief Enable CORTEX M4 SEVONPEND bit. 00611 * @note Set SEVONPEND bit of SCR register. When this bit is set, this causes 00612 * WFE to wake up when an interrupt moves from inactive to pended. 00613 * @retval None 00614 */ 00615 void HAL_PWR_EnableSEVOnPend(void) 00616 { 00617 /* Set SEVONPEND bit of Cortex System Control Register */ 00618 SET_BIT(SCB->SCR, ((uint32_t)SCB_SCR_SEVONPEND_Msk)); 00619 } 00620 00621 00622 /** 00623 * @brief Disable CORTEX M4 SEVONPEND bit. 00624 * @note Clear SEVONPEND bit of SCR register. When this bit is set, this causes 00625 * WFE to wake up when an interrupt moves from inactive to pended. 00626 * @retval None 00627 */ 00628 void HAL_PWR_DisableSEVOnPend(void) 00629 { 00630 /* Clear SEVONPEND bit of Cortex System Control Register */ 00631 CLEAR_BIT(SCB->SCR, ((uint32_t)SCB_SCR_SEVONPEND_Msk)); 00632 } 00633 00634 00635 00636 00637 00638 /** 00639 * @brief PWR PVD interrupt callback 00640 * @retval None 00641 */ 00642 __weak void HAL_PWR_PVDCallback(void) 00643 { 00644 /* NOTE : This function should not be modified; when the callback is needed, 00645 the HAL_PWR_PVDCallback can be implemented in the user file 00646 */ 00647 } 00648 00649 /** 00650 * @} 00651 */ 00652 00653 /** 00654 * @} 00655 */ 00656 00657 #endif /* HAL_PWR_MODULE_ENABLED */ 00658 /** 00659 * @} 00660 */ 00661 00662 /** 00663 * @} 00664 */ 00665 00666 /************************ (C) COPYRIGHT STMicroelectronics *****END OF FILE****/ 00667
Generated on Tue Jul 12 2022 11:35:14 by
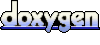