Hal Drivers for L4
Dependents: BSP OneHopeOnePrayer FINAL_AUDIO_RECORD AudioDemo
Fork of STM32L4xx_HAL_Driver by
stm32l4xx_hal_lcd.h
00001 /** 00002 ****************************************************************************** 00003 * @file stm32l4xx_hal_lcd.h 00004 * @author MCD Application Team 00005 * @version V1.1.0 00006 * @date 16-September-2015 00007 * @brief Header file of LCD Controller HAL module. 00008 ****************************************************************************** 00009 * @attention 00010 * 00011 * <h2><center>© COPYRIGHT(c) 2015 STMicroelectronics</center></h2> 00012 * 00013 * Redistribution and use in source and binary forms, with or without modification, 00014 * are permitted provided that the following conditions are met: 00015 * 1. Redistributions of source code must retain the above copyright notice, 00016 * this list of conditions and the following disclaimer. 00017 * 2. Redistributions in binary form must reproduce the above copyright notice, 00018 * this list of conditions and the following disclaimer in the documentation 00019 * and/or other materials provided with the distribution. 00020 * 3. Neither the name of STMicroelectronics nor the names of its contributors 00021 * may be used to endorse or promote products derived from this software 00022 * without specific prior written permission. 00023 * 00024 * THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" 00025 * AND ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE 00026 * IMPLIED WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE 00027 * DISCLAIMED. IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE 00028 * FOR ANY DIRECT, INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL 00029 * DAMAGES (INCLUDING, BUT NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR 00030 * SERVICES; LOSS OF USE, DATA, OR PROFITS; OR BUSINESS INTERRUPTION) HOWEVER 00031 * CAUSED AND ON ANY THEORY OF LIABILITY, WHETHER IN CONTRACT, STRICT LIABILITY, 00032 * OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) ARISING IN ANY WAY OUT OF THE USE 00033 * OF THIS SOFTWARE, EVEN IF ADVISED OF THE POSSIBILITY OF SUCH DAMAGE. 00034 * 00035 ****************************************************************************** 00036 */ 00037 00038 /* Define to prevent recursive inclusion -------------------------------------*/ 00039 #ifndef __STM32L4xx_HAL_LCD_H 00040 #define __STM32L4xx_HAL_LCD_H 00041 00042 #ifdef __cplusplus 00043 extern "C" { 00044 #endif 00045 00046 #if defined(STM32L476xx) || defined(STM32L486xx) 00047 00048 /* Includes ------------------------------------------------------------------*/ 00049 #include "stm32l4xx_hal_def.h" 00050 00051 /** @addtogroup STM32L4xx_HAL_Driver 00052 * @{ 00053 */ 00054 00055 /** @addtogroup LCD 00056 * @{ 00057 */ 00058 00059 /* Exported types ------------------------------------------------------------*/ 00060 /** @defgroup LCD_Exported_Types LCD Exported Types 00061 * @{ 00062 */ 00063 00064 /** 00065 * @brief LCD Init structure definition 00066 */ 00067 00068 typedef struct 00069 { 00070 uint32_t Prescaler; /*!< Configures the LCD Prescaler. 00071 This parameter can be one value of @ref LCD_Prescaler */ 00072 uint32_t Divider; /*!< Configures the LCD Divider. 00073 This parameter can be one value of @ref LCD_Divider */ 00074 uint32_t Duty; /*!< Configures the LCD Duty. 00075 This parameter can be one value of @ref LCD_Duty */ 00076 uint32_t Bias; /*!< Configures the LCD Bias. 00077 This parameter can be one value of @ref LCD_Bias */ 00078 uint32_t VoltageSource; /*!< Selects the LCD Voltage source. 00079 This parameter can be one value of @ref LCD_Voltage_Source */ 00080 uint32_t Contrast; /*!< Configures the LCD Contrast. 00081 This parameter can be one value of @ref LCD_Contrast */ 00082 uint32_t DeadTime; /*!< Configures the LCD Dead Time. 00083 This parameter can be one value of @ref LCD_DeadTime */ 00084 uint32_t PulseOnDuration; /*!< Configures the LCD Pulse On Duration. 00085 This parameter can be one value of @ref LCD_PulseOnDuration */ 00086 uint32_t HighDrive; /*!< Enable or disable the low resistance divider. 00087 This parameter can be one value of @ref LCD_HighDrive */ 00088 uint32_t BlinkMode; /*!< Configures the LCD Blink Mode. 00089 This parameter can be one value of @ref LCD_BlinkMode */ 00090 uint32_t BlinkFrequency; /*!< Configures the LCD Blink frequency. 00091 This parameter can be one value of @ref LCD_BlinkFrequency */ 00092 uint32_t MuxSegment; /*!< Enable or disable mux segment. 00093 This parameter can be one value of @ref LCD_MuxSegment */ 00094 } LCD_InitTypeDef; 00095 00096 /** 00097 * @brief HAL LCD State structures definition 00098 */ 00099 typedef enum 00100 { 00101 HAL_LCD_STATE_RESET = 0x00, /*!< Peripheral is not yet Initialized */ 00102 HAL_LCD_STATE_READY = 0x01, /*!< Peripheral Initialized and ready for use */ 00103 HAL_LCD_STATE_BUSY = 0x02, /*!< an internal process is ongoing */ 00104 HAL_LCD_STATE_TIMEOUT = 0x03, /*!< Timeout state */ 00105 HAL_LCD_STATE_ERROR = 0x04 /*!< Error */ 00106 } HAL_LCD_StateTypeDef; 00107 00108 /** 00109 * @brief UART handle Structure definition 00110 */ 00111 typedef struct 00112 { 00113 LCD_TypeDef *Instance; /* LCD registers base address */ 00114 00115 LCD_InitTypeDef Init; /* LCD communication parameters */ 00116 00117 HAL_LockTypeDef Lock; /* Locking object */ 00118 00119 __IO HAL_LCD_StateTypeDef State; /* LCD communication state */ 00120 00121 __IO uint32_t ErrorCode; /* LCD Error code */ 00122 00123 }LCD_HandleTypeDef; 00124 /** 00125 * @} 00126 */ 00127 00128 /* Exported constants --------------------------------------------------------*/ 00129 /** @defgroup LCD_Exported_Constants LCD Exported Constants 00130 * @{ 00131 */ 00132 00133 /** @defgroup LCD_ErrorCode LCD Error Code 00134 * @{ 00135 */ 00136 #define HAL_LCD_ERROR_NONE ((uint32_t)0x00) /*!< No error */ 00137 #define HAL_LCD_ERROR_FCRSF ((uint32_t)0x01) /*!< Synchro flag timeout error */ 00138 #define HAL_LCD_ERROR_UDR ((uint32_t)0x02) /*!< Update display request flag timeout error */ 00139 #define HAL_LCD_ERROR_UDD ((uint32_t)0x04) /*!< Update display done flag timeout error */ 00140 #define HAL_LCD_ERROR_ENS ((uint32_t)0x08) /*!< LCD enabled status flag timeout error */ 00141 #define HAL_LCD_ERROR_RDY ((uint32_t)0x10) /*!< LCD Booster ready timeout error */ 00142 /** 00143 * @} 00144 */ 00145 00146 /** @defgroup LCD_Prescaler LCD Prescaler 00147 * @{ 00148 */ 00149 #define LCD_PRESCALER_1 ((uint32_t)0x00000000) /*!< CLKPS = LCDCLK */ 00150 #define LCD_PRESCALER_2 ((uint32_t)0x00400000) /*!< CLKPS = LCDCLK/2 */ 00151 #define LCD_PRESCALER_4 ((uint32_t)0x00800000) /*!< CLKPS = LCDCLK/4 */ 00152 #define LCD_PRESCALER_8 ((uint32_t)0x00C00000) /*!< CLKPS = LCDCLK/8 */ 00153 #define LCD_PRESCALER_16 ((uint32_t)0x01000000) /*!< CLKPS = LCDCLK/16 */ 00154 #define LCD_PRESCALER_32 ((uint32_t)0x01400000) /*!< CLKPS = LCDCLK/32 */ 00155 #define LCD_PRESCALER_64 ((uint32_t)0x01800000) /*!< CLKPS = LCDCLK/64 */ 00156 #define LCD_PRESCALER_128 ((uint32_t)0x01C00000) /*!< CLKPS = LCDCLK/128 */ 00157 #define LCD_PRESCALER_256 ((uint32_t)0x02000000) /*!< CLKPS = LCDCLK/256 */ 00158 #define LCD_PRESCALER_512 ((uint32_t)0x02400000) /*!< CLKPS = LCDCLK/512 */ 00159 #define LCD_PRESCALER_1024 ((uint32_t)0x02800000) /*!< CLKPS = LCDCLK/1024 */ 00160 #define LCD_PRESCALER_2048 ((uint32_t)0x02C00000) /*!< CLKPS = LCDCLK/2048 */ 00161 #define LCD_PRESCALER_4096 ((uint32_t)0x03000000) /*!< CLKPS = LCDCLK/4096 */ 00162 #define LCD_PRESCALER_8192 ((uint32_t)0x03400000) /*!< CLKPS = LCDCLK/8192 */ 00163 #define LCD_PRESCALER_16384 ((uint32_t)0x03800000) /*!< CLKPS = LCDCLK/16384 */ 00164 #define LCD_PRESCALER_32768 ((uint32_t)0x03C00000) /*!< CLKPS = LCDCLK/32768 */ 00165 /** 00166 * @} 00167 */ 00168 00169 /** @defgroup LCD_Divider LCD Divider 00170 * @{ 00171 */ 00172 #define LCD_DIVIDER_16 ((uint32_t)0x00000000) /*!< LCD frequency = CLKPS/16 */ 00173 #define LCD_DIVIDER_17 ((uint32_t)0x00040000) /*!< LCD frequency = CLKPS/17 */ 00174 #define LCD_DIVIDER_18 ((uint32_t)0x00080000) /*!< LCD frequency = CLKPS/18 */ 00175 #define LCD_DIVIDER_19 ((uint32_t)0x000C0000) /*!< LCD frequency = CLKPS/19 */ 00176 #define LCD_DIVIDER_20 ((uint32_t)0x00100000) /*!< LCD frequency = CLKPS/20 */ 00177 #define LCD_DIVIDER_21 ((uint32_t)0x00140000) /*!< LCD frequency = CLKPS/21 */ 00178 #define LCD_DIVIDER_22 ((uint32_t)0x00180000) /*!< LCD frequency = CLKPS/22 */ 00179 #define LCD_DIVIDER_23 ((uint32_t)0x001C0000) /*!< LCD frequency = CLKPS/23 */ 00180 #define LCD_DIVIDER_24 ((uint32_t)0x00200000) /*!< LCD frequency = CLKPS/24 */ 00181 #define LCD_DIVIDER_25 ((uint32_t)0x00240000) /*!< LCD frequency = CLKPS/25 */ 00182 #define LCD_DIVIDER_26 ((uint32_t)0x00280000) /*!< LCD frequency = CLKPS/26 */ 00183 #define LCD_DIVIDER_27 ((uint32_t)0x002C0000) /*!< LCD frequency = CLKPS/27 */ 00184 #define LCD_DIVIDER_28 ((uint32_t)0x00300000) /*!< LCD frequency = CLKPS/28 */ 00185 #define LCD_DIVIDER_29 ((uint32_t)0x00340000) /*!< LCD frequency = CLKPS/29 */ 00186 #define LCD_DIVIDER_30 ((uint32_t)0x00380000) /*!< LCD frequency = CLKPS/30 */ 00187 #define LCD_DIVIDER_31 ((uint32_t)0x003C0000) /*!< LCD frequency = CLKPS/31 */ 00188 /** 00189 * @} 00190 */ 00191 00192 00193 /** @defgroup LCD_Duty LCD Duty 00194 * @{ 00195 */ 00196 #define LCD_DUTY_STATIC ((uint32_t)0x00000000) /*!< Static duty */ 00197 #define LCD_DUTY_1_2 (LCD_CR_DUTY_0) /*!< 1/2 duty */ 00198 #define LCD_DUTY_1_3 (LCD_CR_DUTY_1) /*!< 1/3 duty */ 00199 #define LCD_DUTY_1_4 ((LCD_CR_DUTY_1 | LCD_CR_DUTY_0)) /*!< 1/4 duty */ 00200 #define LCD_DUTY_1_8 (LCD_CR_DUTY_2) /*!< 1/8 duty */ 00201 /** 00202 * @} 00203 */ 00204 00205 00206 /** @defgroup LCD_Bias LCD Bias 00207 * @{ 00208 */ 00209 #define LCD_BIAS_1_4 ((uint32_t)0x00000000) /*!< 1/4 Bias */ 00210 #define LCD_BIAS_1_2 LCD_CR_BIAS_0 /*!< 1/2 Bias */ 00211 #define LCD_BIAS_1_3 LCD_CR_BIAS_1 /*!< 1/3 Bias */ 00212 /** 00213 * @} 00214 */ 00215 00216 /** @defgroup LCD_Voltage_Source LCD Voltage Source 00217 * @{ 00218 */ 00219 #define LCD_VOLTAGESOURCE_INTERNAL ((uint32_t)0x00000000) /*!< Internal voltage source for the LCD */ 00220 #define LCD_VOLTAGESOURCE_EXTERNAL LCD_CR_VSEL /*!< External voltage source for the LCD */ 00221 /** 00222 * @} 00223 */ 00224 00225 /** @defgroup LCD_Interrupts LCD Interrupts 00226 * @{ 00227 */ 00228 #define LCD_IT_SOF LCD_FCR_SOFIE 00229 #define LCD_IT_UDD LCD_FCR_UDDIE 00230 /** 00231 * @} 00232 */ 00233 00234 /** @defgroup LCD_PulseOnDuration LCD Pulse On Duration 00235 * @{ 00236 */ 00237 #define LCD_PULSEONDURATION_0 ((uint32_t)0x00000000) /*!< Pulse ON duration = 0 pulse */ 00238 #define LCD_PULSEONDURATION_1 (LCD_FCR_PON_0) /*!< Pulse ON duration = 1/CK_PS */ 00239 #define LCD_PULSEONDURATION_2 (LCD_FCR_PON_1) /*!< Pulse ON duration = 2/CK_PS */ 00240 #define LCD_PULSEONDURATION_3 (LCD_FCR_PON_1 | LCD_FCR_PON_0) /*!< Pulse ON duration = 3/CK_PS */ 00241 #define LCD_PULSEONDURATION_4 (LCD_FCR_PON_2) /*!< Pulse ON duration = 4/CK_PS */ 00242 #define LCD_PULSEONDURATION_5 (LCD_FCR_PON_2 | LCD_FCR_PON_0) /*!< Pulse ON duration = 5/CK_PS */ 00243 #define LCD_PULSEONDURATION_6 (LCD_FCR_PON_2 | LCD_FCR_PON_1) /*!< Pulse ON duration = 6/CK_PS */ 00244 #define LCD_PULSEONDURATION_7 (LCD_FCR_PON) /*!< Pulse ON duration = 7/CK_PS */ 00245 /** 00246 * @} 00247 */ 00248 00249 00250 /** @defgroup LCD_DeadTime LCD Dead Time 00251 * @{ 00252 */ 00253 #define LCD_DEADTIME_0 ((uint32_t)0x00000000) /*!< No dead Time */ 00254 #define LCD_DEADTIME_1 (LCD_FCR_DEAD_0) /*!< One Phase between different couple of Frame */ 00255 #define LCD_DEADTIME_2 (LCD_FCR_DEAD_1) /*!< Two Phase between different couple of Frame */ 00256 #define LCD_DEADTIME_3 (LCD_FCR_DEAD_1 | LCD_FCR_DEAD_0) /*!< Three Phase between different couple of Frame */ 00257 #define LCD_DEADTIME_4 (LCD_FCR_DEAD_2) /*!< Four Phase between different couple of Frame */ 00258 #define LCD_DEADTIME_5 (LCD_FCR_DEAD_2 | LCD_FCR_DEAD_0) /*!< Five Phase between different couple of Frame */ 00259 #define LCD_DEADTIME_6 (LCD_FCR_DEAD_2 | LCD_FCR_DEAD_1) /*!< Six Phase between different couple of Frame */ 00260 #define LCD_DEADTIME_7 (LCD_FCR_DEAD) /*!< Seven Phase between different couple of Frame */ 00261 /** 00262 * @} 00263 */ 00264 00265 /** @defgroup LCD_BlinkMode LCD Blink Mode 00266 * @{ 00267 */ 00268 #define LCD_BLINKMODE_OFF ((uint32_t)0x00000000) /*!< Blink disabled */ 00269 #define LCD_BLINKMODE_SEG0_COM0 (LCD_FCR_BLINK_0) /*!< Blink enabled on SEG[0], COM[0] (1 pixel) */ 00270 #define LCD_BLINKMODE_SEG0_ALLCOM (LCD_FCR_BLINK_1) /*!< Blink enabled on SEG[0], all COM (up to 00271 8 pixels according to the programmed duty) */ 00272 #define LCD_BLINKMODE_ALLSEG_ALLCOM (LCD_FCR_BLINK) /*!< Blink enabled on all SEG and all COM (all pixels) */ 00273 /** 00274 * @} 00275 */ 00276 00277 /** @defgroup LCD_BlinkFrequency LCD Blink Frequency 00278 * @{ 00279 */ 00280 #define LCD_BLINKFREQUENCY_DIV8 ((uint32_t)0x00000000) /*!< The Blink frequency = fLCD/8 */ 00281 #define LCD_BLINKFREQUENCY_DIV16 (LCD_FCR_BLINKF_0) /*!< The Blink frequency = fLCD/16 */ 00282 #define LCD_BLINKFREQUENCY_DIV32 (LCD_FCR_BLINKF_1) /*!< The Blink frequency = fLCD/32 */ 00283 #define LCD_BLINKFREQUENCY_DIV64 (LCD_FCR_BLINKF_1 | LCD_FCR_BLINKF_0) /*!< The Blink frequency = fLCD/64 */ 00284 #define LCD_BLINKFREQUENCY_DIV128 (LCD_FCR_BLINKF_2) /*!< The Blink frequency = fLCD/128 */ 00285 #define LCD_BLINKFREQUENCY_DIV256 (LCD_FCR_BLINKF_2 |LCD_FCR_BLINKF_0) /*!< The Blink frequency = fLCD/256 */ 00286 #define LCD_BLINKFREQUENCY_DIV512 (LCD_FCR_BLINKF_2 |LCD_FCR_BLINKF_1) /*!< The Blink frequency = fLCD/512 */ 00287 #define LCD_BLINKFREQUENCY_DIV1024 (LCD_FCR_BLINKF) /*!< The Blink frequency = fLCD/1024 */ 00288 /** 00289 * @} 00290 */ 00291 00292 /** @defgroup LCD_Contrast LCD Contrast 00293 * @{ 00294 */ 00295 #define LCD_CONTRASTLEVEL_0 ((uint32_t)0x00000000) /*!< Maximum Voltage = 2.60V */ 00296 #define LCD_CONTRASTLEVEL_1 (LCD_FCR_CC_0) /*!< Maximum Voltage = 2.73V */ 00297 #define LCD_CONTRASTLEVEL_2 (LCD_FCR_CC_1) /*!< Maximum Voltage = 2.86V */ 00298 #define LCD_CONTRASTLEVEL_3 (LCD_FCR_CC_1 | LCD_FCR_CC_0) /*!< Maximum Voltage = 2.99V */ 00299 #define LCD_CONTRASTLEVEL_4 (LCD_FCR_CC_2) /*!< Maximum Voltage = 3.12V */ 00300 #define LCD_CONTRASTLEVEL_5 (LCD_FCR_CC_2 | LCD_FCR_CC_0) /*!< Maximum Voltage = 3.26V */ 00301 #define LCD_CONTRASTLEVEL_6 (LCD_FCR_CC_2 | LCD_FCR_CC_1) /*!< Maximum Voltage = 3.40V */ 00302 #define LCD_CONTRASTLEVEL_7 (LCD_FCR_CC) /*!< Maximum Voltage = 3.55V */ 00303 /** 00304 * @} 00305 */ 00306 00307 /** @defgroup LCD_RAMRegister LCD RAMRegister 00308 * @{ 00309 */ 00310 #define LCD_RAM_REGISTER0 ((uint32_t)0x00000000) /*!< LCD RAM Register 0 */ 00311 #define LCD_RAM_REGISTER1 ((uint32_t)0x00000001) /*!< LCD RAM Register 1 */ 00312 #define LCD_RAM_REGISTER2 ((uint32_t)0x00000002) /*!< LCD RAM Register 2 */ 00313 #define LCD_RAM_REGISTER3 ((uint32_t)0x00000003) /*!< LCD RAM Register 3 */ 00314 #define LCD_RAM_REGISTER4 ((uint32_t)0x00000004) /*!< LCD RAM Register 4 */ 00315 #define LCD_RAM_REGISTER5 ((uint32_t)0x00000005) /*!< LCD RAM Register 5 */ 00316 #define LCD_RAM_REGISTER6 ((uint32_t)0x00000006) /*!< LCD RAM Register 6 */ 00317 #define LCD_RAM_REGISTER7 ((uint32_t)0x00000007) /*!< LCD RAM Register 7 */ 00318 #define LCD_RAM_REGISTER8 ((uint32_t)0x00000008) /*!< LCD RAM Register 8 */ 00319 #define LCD_RAM_REGISTER9 ((uint32_t)0x00000009) /*!< LCD RAM Register 9 */ 00320 #define LCD_RAM_REGISTER10 ((uint32_t)0x0000000A) /*!< LCD RAM Register 10 */ 00321 #define LCD_RAM_REGISTER11 ((uint32_t)0x0000000B) /*!< LCD RAM Register 11 */ 00322 #define LCD_RAM_REGISTER12 ((uint32_t)0x0000000C) /*!< LCD RAM Register 12 */ 00323 #define LCD_RAM_REGISTER13 ((uint32_t)0x0000000D) /*!< LCD RAM Register 13 */ 00324 #define LCD_RAM_REGISTER14 ((uint32_t)0x0000000E) /*!< LCD RAM Register 14 */ 00325 #define LCD_RAM_REGISTER15 ((uint32_t)0x0000000F) /*!< LCD RAM Register 15 */ 00326 /** 00327 * @} 00328 */ 00329 00330 /** @defgroup LCD_HighDrive LCD High Drive 00331 * @{ 00332 */ 00333 00334 #define LCD_HIGHDRIVE_DISABLE ((uint32_t)0x00000000) /*!< High drive disabled */ 00335 #define LCD_HIGHDRIVE_ENABLE (LCD_FCR_HD) /*!< High drive enabled */ 00336 /** 00337 * @} 00338 */ 00339 00340 /** @defgroup LCD_MuxSegment LCD Mux Segment 00341 * @{ 00342 */ 00343 00344 #define LCD_MUXSEGMENT_DISABLE ((uint32_t)0x00000000) /*!< SEG pin multiplexing disabled */ 00345 #define LCD_MUXSEGMENT_ENABLE (LCD_CR_MUX_SEG) /*!< SEG[31:28] are multiplexed with SEG[43:40] */ 00346 /** 00347 * @} 00348 */ 00349 00350 /** @defgroup LCD_Flag_Definition LCD Flags Definition 00351 * @{ 00352 */ 00353 #define LCD_FLAG_ENS LCD_SR_ENS /*!< LCD enabled status */ 00354 #define LCD_FLAG_SOF LCD_SR_SOF /*!< Start of frame flag */ 00355 #define LCD_FLAG_UDR LCD_SR_UDR /*!< Update display request */ 00356 #define LCD_FLAG_UDD LCD_SR_UDD /*!< Update display done */ 00357 #define LCD_FLAG_RDY LCD_SR_RDY /*!< Ready flag */ 00358 #define LCD_FLAG_FCRSF LCD_SR_FCRSR /*!< LCD Frame Control Register Synchronization flag */ 00359 /** 00360 * @} 00361 */ 00362 00363 /** 00364 * @} 00365 */ 00366 00367 /* Exported macros -----------------------------------------------------------*/ 00368 /** @defgroup LCD_Exported_Macros LCD Exported Macros 00369 * @{ 00370 */ 00371 00372 /** @brief Reset LCD handle state. 00373 * @param __HANDLE__: specifies the LCD Handle. 00374 * @retval None 00375 */ 00376 #define __HAL_LCD_RESET_HANDLE_STATE(__HANDLE__) ((__HANDLE__)->State = HAL_LCD_STATE_RESET) 00377 00378 /** @brief Enable the LCD peripheral. 00379 * @param __HANDLE__: specifies the LCD Handle. 00380 * @retval None 00381 */ 00382 #define __HAL_LCD_ENABLE(__HANDLE__) SET_BIT((__HANDLE__)->Instance->CR, LCD_CR_LCDEN) 00383 00384 /** @brief Disable the LCD peripheral. 00385 * @param __HANDLE__: specifies the LCD Handle. 00386 * @retval None 00387 */ 00388 #define __HAL_LCD_DISABLE(__HANDLE__) CLEAR_BIT((__HANDLE__)->Instance->CR, LCD_CR_LCDEN) 00389 00390 /** @brief Enable the low resistance divider. 00391 * @param __HANDLE__: specifies the LCD Handle. 00392 * @note Displays with high internal resistance may need a longer drive time to 00393 * achieve satisfactory contrast. This function is useful in this case if 00394 * some additional power consumption can be tolerated. 00395 * @note When this mode is enabled, the PulseOn Duration (PON) have to be 00396 * programmed to 1/CK_PS (LCD_PULSEONDURATION_1). 00397 * @retval None 00398 */ 00399 #define __HAL_LCD_HIGHDRIVER_ENABLE(__HANDLE__) \ 00400 do { \ 00401 SET_BIT((__HANDLE__)->Instance->FCR, LCD_FCR_HD); \ 00402 LCD_WaitForSynchro(__HANDLE__); \ 00403 } while(0) 00404 00405 /** @brief Disable the low resistance divider. 00406 * @param __HANDLE__: specifies the LCD Handle. 00407 * @retval None 00408 */ 00409 #define __HAL_LCD_HIGHDRIVER_DISABLE(__HANDLE__) \ 00410 do { \ 00411 CLEAR_BIT((__HANDLE__)->Instance->FCR, LCD_FCR_HD); \ 00412 LCD_WaitForSynchro(__HANDLE__); \ 00413 } while(0) 00414 00415 /** @brief Enable the voltage output buffer for higher driving capability. 00416 * @param __HANDLE__: specifies the LCD Handle. 00417 * @retval None 00418 */ 00419 #define __HAL_LCD_VOLTAGE_BUFFER_ENABLE(__HANDLE__) SET_BIT((__HANDLE__)->Instance->CR, LCD_CR_BUFEN) 00420 00421 /** @brief Disable the voltage output buffer for higher driving capability. 00422 * @param __HANDLE__: specifies the LCD Handle. 00423 * @retval None 00424 */ 00425 #define __HAL_LCD_VOLTAGE_BUFFER_DISABLE(__HANDLE__) CLEAR_BIT((__HANDLE__)->Instance->CR, LCD_CR_BUFEN) 00426 00427 /** 00428 * @brief Configure the LCD pulse on duration. 00429 * @param __HANDLE__: specifies the LCD Handle. 00430 * @param __DURATION__: specifies the LCD pulse on duration in terms of 00431 * CK_PS (prescaled LCD clock period) pulses. 00432 * This parameter can be one of the following values: 00433 * @arg LCD_PULSEONDURATION_0: 0 pulse 00434 * @arg LCD_PULSEONDURATION_1: Pulse ON duration = 1/CK_PS 00435 * @arg LCD_PULSEONDURATION_2: Pulse ON duration = 2/CK_PS 00436 * @arg LCD_PULSEONDURATION_3: Pulse ON duration = 3/CK_PS 00437 * @arg LCD_PULSEONDURATION_4: Pulse ON duration = 4/CK_PS 00438 * @arg LCD_PULSEONDURATION_5: Pulse ON duration = 5/CK_PS 00439 * @arg LCD_PULSEONDURATION_6: Pulse ON duration = 6/CK_PS 00440 * @arg LCD_PULSEONDURATION_7: Pulse ON duration = 7/CK_PS 00441 * @retval None 00442 */ 00443 #define __HAL_LCD_PULSEONDURATION_CONFIG(__HANDLE__, __DURATION__) \ 00444 do { \ 00445 MODIFY_REG((__HANDLE__)->Instance->FCR, LCD_FCR_PON, (__DURATION__)); \ 00446 LCD_WaitForSynchro(__HANDLE__); \ 00447 } while(0) 00448 00449 /** 00450 * @brief Configure the LCD dead time. 00451 * @param __HANDLE__: specifies the LCD Handle. 00452 * @param __DEADTIME__: specifies the LCD dead time. 00453 * This parameter can be one of the following values: 00454 * @arg LCD_DEADTIME_0: No dead Time 00455 * @arg LCD_DEADTIME_1: One Phase between different couple of Frame 00456 * @arg LCD_DEADTIME_2: Two Phase between different couple of Frame 00457 * @arg LCD_DEADTIME_3: Three Phase between different couple of Frame 00458 * @arg LCD_DEADTIME_4: Four Phase between different couple of Frame 00459 * @arg LCD_DEADTIME_5: Five Phase between different couple of Frame 00460 * @arg LCD_DEADTIME_6: Six Phase between different couple of Frame 00461 * @arg LCD_DEADTIME_7: Seven Phase between different couple of Frame 00462 * @retval None 00463 */ 00464 #define __HAL_LCD_DEADTIME_CONFIG(__HANDLE__, __DEADTIME__) \ 00465 do { \ 00466 MODIFY_REG((__HANDLE__)->Instance->FCR, LCD_FCR_DEAD, (__DEADTIME__)); \ 00467 LCD_WaitForSynchro(__HANDLE__); \ 00468 } while(0) 00469 00470 /** 00471 * @brief Configure the LCD contrast. 00472 * @param __HANDLE__: specifies the LCD Handle. 00473 * @param __CONTRAST__: specifies the LCD Contrast. 00474 * This parameter can be one of the following values: 00475 * @arg LCD_CONTRASTLEVEL_0: Maximum Voltage = 2.60V 00476 * @arg LCD_CONTRASTLEVEL_1: Maximum Voltage = 2.73V 00477 * @arg LCD_CONTRASTLEVEL_2: Maximum Voltage = 2.86V 00478 * @arg LCD_CONTRASTLEVEL_3: Maximum Voltage = 2.99V 00479 * @arg LCD_CONTRASTLEVEL_4: Maximum Voltage = 3.12V 00480 * @arg LCD_CONTRASTLEVEL_5: Maximum Voltage = 3.25V 00481 * @arg LCD_CONTRASTLEVEL_6: Maximum Voltage = 3.38V 00482 * @arg LCD_CONTRASTLEVEL_7: Maximum Voltage = 3.51V 00483 * @retval None 00484 */ 00485 #define __HAL_LCD_CONTRAST_CONFIG(__HANDLE__, __CONTRAST__) \ 00486 do { \ 00487 MODIFY_REG((__HANDLE__)->Instance->FCR, LCD_FCR_CC, (__CONTRAST__)); \ 00488 LCD_WaitForSynchro(__HANDLE__); \ 00489 } while(0) 00490 00491 /** 00492 * @brief Configure the LCD Blink mode and Blink frequency. 00493 * @param __HANDLE__: specifies the LCD Handle. 00494 * @param __BLINKMODE__: specifies the LCD blink mode. 00495 * This parameter can be one of the following values: 00496 * @arg LCD_BLINKMODE_OFF: Blink disabled 00497 * @arg LCD_BLINKMODE_SEG0_COM0: Blink enabled on SEG[0], COM[0] (1 pixel) 00498 * @arg LCD_BLINKMODE_SEG0_ALLCOM: Blink enabled on SEG[0], all COM (up to 8 00499 * pixels according to the programmed duty) 00500 * @arg LCD_BLINKMODE_ALLSEG_ALLCOM: Blink enabled on all SEG and all COM 00501 * (all pixels) 00502 * @param __BLINKFREQUENCY__: specifies the LCD blink frequency. 00503 * @arg LCD_BLINKFREQUENCY_DIV8: The Blink frequency = fLcd/8 00504 * @arg LCD_BLINKFREQUENCY_DIV16: The Blink frequency = fLcd/16 00505 * @arg LCD_BLINKFREQUENCY_DIV32: The Blink frequency = fLcd/32 00506 * @arg LCD_BLINKFREQUENCY_DIV64: The Blink frequency = fLcd/64 00507 * @arg LCD_BLINKFREQUENCY_DIV128: The Blink frequency = fLcd/128 00508 * @arg LCD_BLINKFREQUENCY_DIV256: The Blink frequency = fLcd/256 00509 * @arg LCD_BLINKFREQUENCY_DIV512: The Blink frequency = fLcd/512 00510 * @arg LCD_BLINKFREQUENCY_DIV1024: The Blink frequency = fLcd/1024 00511 * @retval None 00512 */ 00513 #define __HAL_LCD_BLINK_CONFIG(__HANDLE__, __BLINKMODE__, __BLINKFREQUENCY__) \ 00514 do { \ 00515 MODIFY_REG((__HANDLE__)->Instance->FCR, (LCD_FCR_BLINKF | LCD_FCR_BLINK), ((__BLINKMODE__) | (__BLINKFREQUENCY__))); \ 00516 LCD_WaitForSynchro(__HANDLE__); \ 00517 } while(0) 00518 00519 /** @brief Enable the specified LCD interrupt. 00520 * @param __HANDLE__: specifies the LCD Handle. 00521 * @param __INTERRUPT__: specifies the LCD interrupt source to be enabled. 00522 * This parameter can be one of the following values: 00523 * @arg LCD_IT_SOF: Start of Frame Interrupt 00524 * @arg LCD_IT_UDD: Update Display Done Interrupt 00525 * @retval None 00526 */ 00527 #define __HAL_LCD_ENABLE_IT(__HANDLE__, __INTERRUPT__) \ 00528 do { \ 00529 SET_BIT((__HANDLE__)->Instance->FCR, (__INTERRUPT__)); \ 00530 LCD_WaitForSynchro(__HANDLE__); \ 00531 } while(0) 00532 00533 /** @brief Disable the specified LCD interrupt. 00534 * @param __HANDLE__: specifies the LCD Handle. 00535 * @param __INTERRUPT__: specifies the LCD interrupt source to be disabled. 00536 * This parameter can be one of the following values: 00537 * @arg LCD_IT_SOF: Start of Frame Interrupt 00538 * @arg LCD_IT_UDD: Update Display Done Interrupt 00539 * @retval None 00540 */ 00541 #define __HAL_LCD_DISABLE_IT(__HANDLE__, __INTERRUPT__) \ 00542 do { \ 00543 CLEAR_BIT((__HANDLE__)->Instance->FCR, (__INTERRUPT__)); \ 00544 LCD_WaitForSynchro(__HANDLE__); \ 00545 } while(0) 00546 00547 /** @brief Check whether the specified LCD interrupt source is enabled or not. 00548 * @param __HANDLE__: specifies the LCD Handle. 00549 * @param __IT__: specifies the LCD interrupt source to check. 00550 * This parameter can be one of the following values: 00551 * @arg LCD_IT_SOF: Start of Frame Interrupt 00552 * @arg LCD_IT_UDD: Update Display Done Interrupt. 00553 * @note If the device is in STOP mode (PCLK not provided) UDD will not 00554 * generate an interrupt even if UDDIE = 1. 00555 * If the display is not enabled the UDD interrupt will never occur. 00556 * @retval The state of __IT__ (TRUE or FALSE). 00557 */ 00558 #define __HAL_LCD_GET_IT_SOURCE(__HANDLE__, __IT__) (((__HANDLE__)->Instance->FCR) & (__IT__)) 00559 00560 /** @brief Check whether the specified LCD flag is set or not. 00561 * @param __HANDLE__: specifies the LCD Handle. 00562 * @param __FLAG__: specifies the flag to check. 00563 * This parameter can be one of the following values: 00564 * @arg LCD_FLAG_ENS: LCD Enabled flag. It indicates the LCD controller status. 00565 * @note The ENS bit is set immediately when the LCDEN bit in the LCD_CR 00566 * goes from 0 to 1. On deactivation it reflects the real status of 00567 * LCD so it becomes 0 at the end of the last displayed frame. 00568 * @arg LCD_FLAG_SOF: Start of Frame flag. This flag is set by hardware at 00569 * the beginning of a new frame, at the same time as the display data is 00570 * updated. 00571 * @arg LCD_FLAG_UDR: Update Display Request flag. 00572 * @arg LCD_FLAG_UDD: Update Display Done flag. 00573 * @arg LCD_FLAG_RDY: Step_up converter Ready flag. It indicates the status 00574 * of the step-up converter. 00575 * @arg LCD_FLAG_FCRSF: LCD Frame Control Register Synchronization Flag. 00576 * This flag is set by hardware each time the LCD_FCR register is updated 00577 * in the LCDCLK domain. 00578 * @retval The new state of __FLAG__ (TRUE or FALSE). 00579 */ 00580 #define __HAL_LCD_GET_FLAG(__HANDLE__, __FLAG__) (((__HANDLE__)->Instance->SR & (__FLAG__)) == (__FLAG__)) 00581 00582 /** @brief Clear the specified LCD pending flag. 00583 * @param __HANDLE__: specifies the LCD Handle. 00584 * @param __FLAG__: specifies the flag to clear. 00585 * This parameter can be any combination of the following values: 00586 * @arg LCD_FLAG_SOF: Start of Frame Interrupt 00587 * @arg LCD_FLAG_UDD: Update Display Done Interrupt 00588 * @retval None 00589 */ 00590 #define __HAL_LCD_CLEAR_FLAG(__HANDLE__, __FLAG__) WRITE_REG((__HANDLE__)->Instance->CLR, (__FLAG__)) 00591 00592 /** 00593 * @} 00594 */ 00595 00596 /* Exported functions ------------------------------------------------------- */ 00597 /** @addtogroup LCD_Exported_Functions 00598 * @{ 00599 */ 00600 00601 /* Initialization/de-initialization methods **********************************/ 00602 /** @addtogroup LCD_Exported_Functions_Group1 00603 * @{ 00604 */ 00605 HAL_StatusTypeDef HAL_LCD_DeInit(LCD_HandleTypeDef *hlcd); 00606 HAL_StatusTypeDef HAL_LCD_Init(LCD_HandleTypeDef *hlcd); 00607 void HAL_LCD_MspInit(LCD_HandleTypeDef *hlcd); 00608 void HAL_LCD_MspDeInit(LCD_HandleTypeDef *hlcd); 00609 /** 00610 * @} 00611 */ 00612 00613 /* IO operation methods *******************************************************/ 00614 /** @addtogroup LCD_Exported_Functions_Group2 00615 * @{ 00616 */ 00617 HAL_StatusTypeDef HAL_LCD_Write(LCD_HandleTypeDef *hlcd, uint32_t RAMRegisterIndex, uint32_t RAMRegisterMask, uint32_t Data); 00618 HAL_StatusTypeDef HAL_LCD_Clear(LCD_HandleTypeDef *hlcd); 00619 HAL_StatusTypeDef HAL_LCD_UpdateDisplayRequest(LCD_HandleTypeDef *hlcd); 00620 /** 00621 * @} 00622 */ 00623 00624 /* Peripheral State methods **************************************************/ 00625 /** @addtogroup LCD_Exported_Functions_Group3 00626 * @{ 00627 */ 00628 HAL_LCD_StateTypeDef HAL_LCD_GetState(LCD_HandleTypeDef *hlcd); 00629 uint32_t HAL_LCD_GetError(LCD_HandleTypeDef *hlcd); 00630 /** 00631 * @} 00632 */ 00633 00634 /** 00635 * @} 00636 */ 00637 00638 /* Private types -------------------------------------------------------------*/ 00639 /* Private variables ---------------------------------------------------------*/ 00640 /* Private constants ---------------------------------------------------------*/ 00641 /* Private macros ------------------------------------------------------------*/ 00642 /** @defgroup LCD_Private_Macros LCD Private Macros 00643 * @{ 00644 */ 00645 00646 #define IS_LCD_PRESCALER(__PRESCALER__) (((__PRESCALER__) == LCD_PRESCALER_1) || \ 00647 ((__PRESCALER__) == LCD_PRESCALER_2) || \ 00648 ((__PRESCALER__) == LCD_PRESCALER_4) || \ 00649 ((__PRESCALER__) == LCD_PRESCALER_8) || \ 00650 ((__PRESCALER__) == LCD_PRESCALER_16) || \ 00651 ((__PRESCALER__) == LCD_PRESCALER_32) || \ 00652 ((__PRESCALER__) == LCD_PRESCALER_64) || \ 00653 ((__PRESCALER__) == LCD_PRESCALER_128) || \ 00654 ((__PRESCALER__) == LCD_PRESCALER_256) || \ 00655 ((__PRESCALER__) == LCD_PRESCALER_512) || \ 00656 ((__PRESCALER__) == LCD_PRESCALER_1024) || \ 00657 ((__PRESCALER__) == LCD_PRESCALER_2048) || \ 00658 ((__PRESCALER__) == LCD_PRESCALER_4096) || \ 00659 ((__PRESCALER__) == LCD_PRESCALER_8192) || \ 00660 ((__PRESCALER__) == LCD_PRESCALER_16384) || \ 00661 ((__PRESCALER__) == LCD_PRESCALER_32768)) 00662 00663 #define IS_LCD_DIVIDER(__DIVIDER__) (((__DIVIDER__) == LCD_DIVIDER_16) || \ 00664 ((__DIVIDER__) == LCD_DIVIDER_17) || \ 00665 ((__DIVIDER__) == LCD_DIVIDER_18) || \ 00666 ((__DIVIDER__) == LCD_DIVIDER_19) || \ 00667 ((__DIVIDER__) == LCD_DIVIDER_20) || \ 00668 ((__DIVIDER__) == LCD_DIVIDER_21) || \ 00669 ((__DIVIDER__) == LCD_DIVIDER_22) || \ 00670 ((__DIVIDER__) == LCD_DIVIDER_23) || \ 00671 ((__DIVIDER__) == LCD_DIVIDER_24) || \ 00672 ((__DIVIDER__) == LCD_DIVIDER_25) || \ 00673 ((__DIVIDER__) == LCD_DIVIDER_26) || \ 00674 ((__DIVIDER__) == LCD_DIVIDER_27) || \ 00675 ((__DIVIDER__) == LCD_DIVIDER_28) || \ 00676 ((__DIVIDER__) == LCD_DIVIDER_29) || \ 00677 ((__DIVIDER__) == LCD_DIVIDER_30) || \ 00678 ((__DIVIDER__) == LCD_DIVIDER_31)) 00679 00680 #define IS_LCD_DUTY(__DUTY__) (((__DUTY__) == LCD_DUTY_STATIC) || \ 00681 ((__DUTY__) == LCD_DUTY_1_2) || \ 00682 ((__DUTY__) == LCD_DUTY_1_3) || \ 00683 ((__DUTY__) == LCD_DUTY_1_4) || \ 00684 ((__DUTY__) == LCD_DUTY_1_8)) 00685 00686 #define IS_LCD_BIAS(__BIAS__) (((__BIAS__) == LCD_BIAS_1_4) || \ 00687 ((__BIAS__) == LCD_BIAS_1_2) || \ 00688 ((__BIAS__) == LCD_BIAS_1_3)) 00689 00690 #define IS_LCD_VOLTAGE_SOURCE(SOURCE) (((SOURCE) == LCD_VOLTAGESOURCE_INTERNAL) || \ 00691 ((SOURCE) == LCD_VOLTAGESOURCE_EXTERNAL)) 00692 00693 00694 #define IS_LCD_PULSE_ON_DURATION(__DURATION__) (((__DURATION__) == LCD_PULSEONDURATION_0) || \ 00695 ((__DURATION__) == LCD_PULSEONDURATION_1) || \ 00696 ((__DURATION__) == LCD_PULSEONDURATION_2) || \ 00697 ((__DURATION__) == LCD_PULSEONDURATION_3) || \ 00698 ((__DURATION__) == LCD_PULSEONDURATION_4) || \ 00699 ((__DURATION__) == LCD_PULSEONDURATION_5) || \ 00700 ((__DURATION__) == LCD_PULSEONDURATION_6) || \ 00701 ((__DURATION__) == LCD_PULSEONDURATION_7)) 00702 00703 #define IS_LCD_DEAD_TIME(__TIME__) (((__TIME__) == LCD_DEADTIME_0) || \ 00704 ((__TIME__) == LCD_DEADTIME_1) || \ 00705 ((__TIME__) == LCD_DEADTIME_2) || \ 00706 ((__TIME__) == LCD_DEADTIME_3) || \ 00707 ((__TIME__) == LCD_DEADTIME_4) || \ 00708 ((__TIME__) == LCD_DEADTIME_5) || \ 00709 ((__TIME__) == LCD_DEADTIME_6) || \ 00710 ((__TIME__) == LCD_DEADTIME_7)) 00711 00712 #define IS_LCD_BLINK_MODE(__MODE__) (((__MODE__) == LCD_BLINKMODE_OFF) || \ 00713 ((__MODE__) == LCD_BLINKMODE_SEG0_COM0) || \ 00714 ((__MODE__) == LCD_BLINKMODE_SEG0_ALLCOM) || \ 00715 ((__MODE__) == LCD_BLINKMODE_ALLSEG_ALLCOM)) 00716 00717 #define IS_LCD_BLINK_FREQUENCY(__FREQUENCY__) (((__FREQUENCY__) == LCD_BLINKFREQUENCY_DIV8) || \ 00718 ((__FREQUENCY__) == LCD_BLINKFREQUENCY_DIV16) || \ 00719 ((__FREQUENCY__) == LCD_BLINKFREQUENCY_DIV32) || \ 00720 ((__FREQUENCY__) == LCD_BLINKFREQUENCY_DIV64) || \ 00721 ((__FREQUENCY__) == LCD_BLINKFREQUENCY_DIV128) || \ 00722 ((__FREQUENCY__) == LCD_BLINKFREQUENCY_DIV256) || \ 00723 ((__FREQUENCY__) == LCD_BLINKFREQUENCY_DIV512) || \ 00724 ((__FREQUENCY__) == LCD_BLINKFREQUENCY_DIV1024)) 00725 00726 #define IS_LCD_CONTRAST(__CONTRAST__) (((__CONTRAST__) == LCD_CONTRASTLEVEL_0) || \ 00727 ((__CONTRAST__) == LCD_CONTRASTLEVEL_1) || \ 00728 ((__CONTRAST__) == LCD_CONTRASTLEVEL_2) || \ 00729 ((__CONTRAST__) == LCD_CONTRASTLEVEL_3) || \ 00730 ((__CONTRAST__) == LCD_CONTRASTLEVEL_4) || \ 00731 ((__CONTRAST__) == LCD_CONTRASTLEVEL_5) || \ 00732 ((__CONTRAST__) == LCD_CONTRASTLEVEL_6) || \ 00733 ((__CONTRAST__) == LCD_CONTRASTLEVEL_7)) 00734 00735 #define IS_LCD_RAM_REGISTER(__REGISTER__) (((__REGISTER__) == LCD_RAM_REGISTER0) || \ 00736 ((__REGISTER__) == LCD_RAM_REGISTER1) || \ 00737 ((__REGISTER__) == LCD_RAM_REGISTER2) || \ 00738 ((__REGISTER__) == LCD_RAM_REGISTER3) || \ 00739 ((__REGISTER__) == LCD_RAM_REGISTER4) || \ 00740 ((__REGISTER__) == LCD_RAM_REGISTER5) || \ 00741 ((__REGISTER__) == LCD_RAM_REGISTER6) || \ 00742 ((__REGISTER__) == LCD_RAM_REGISTER7) || \ 00743 ((__REGISTER__) == LCD_RAM_REGISTER8) || \ 00744 ((__REGISTER__) == LCD_RAM_REGISTER9) || \ 00745 ((__REGISTER__) == LCD_RAM_REGISTER10) || \ 00746 ((__REGISTER__) == LCD_RAM_REGISTER11) || \ 00747 ((__REGISTER__) == LCD_RAM_REGISTER12) || \ 00748 ((__REGISTER__) == LCD_RAM_REGISTER13) || \ 00749 ((__REGISTER__) == LCD_RAM_REGISTER14) || \ 00750 ((__REGISTER__) == LCD_RAM_REGISTER15)) 00751 00752 #define IS_LCD_HIGH_DRIVE(__VALUE__) (((__VALUE__) == LCD_HIGHDRIVE_DISABLE) || \ 00753 ((__VALUE__) == LCD_HIGHDRIVE_ENABLE)) 00754 00755 #define IS_LCD_MUX_SEGMENT(__VALUE__) (((__VALUE__) == LCD_MUXSEGMENT_ENABLE) || \ 00756 ((__VALUE__) == LCD_MUXSEGMENT_DISABLE)) 00757 00758 /** 00759 * @} 00760 */ 00761 00762 /* Private functions ---------------------------------------------------------*/ 00763 /** @addtogroup LCD_Private_Functions 00764 * @{ 00765 */ 00766 00767 HAL_StatusTypeDef LCD_WaitForSynchro(LCD_HandleTypeDef *hlcd); 00768 00769 /** 00770 * @} 00771 */ 00772 00773 /** 00774 * @} 00775 */ 00776 00777 /** 00778 * @} 00779 */ 00780 00781 #endif /* STM32L476xx || STM32L486xx */ 00782 00783 #ifdef __cplusplus 00784 } 00785 #endif 00786 00787 #endif /* __STM32L4xx_HAL_LCD_H */ 00788 00789 /******************* (C) COPYRIGHT 2015 STMicroelectronics *****END OF FILE****/ 00790
Generated on Tue Jul 12 2022 11:35:13 by
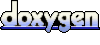