Hal Drivers for L4
Dependents: BSP OneHopeOnePrayer FINAL_AUDIO_RECORD AudioDemo
Fork of STM32L4xx_HAL_Driver by
stm32l4xx_hal_lcd.c
00001 /** 00002 ****************************************************************************** 00003 * @file stm32l4xx_hal_lcd.c 00004 * @author MCD Application Team 00005 * @version V1.1.0 00006 * @date 16-September-2015 00007 * @brief LCD Controller HAL module driver. 00008 * This file provides firmware functions to manage the following 00009 * functionalities of the LCD Controller (LCD) peripheral: 00010 * + Initialization/de-initialization methods 00011 * + I/O operation methods 00012 * + Peripheral State methods 00013 * 00014 @verbatim 00015 ============================================================================== 00016 ##### How to use this driver ##### 00017 ============================================================================== 00018 [..] The LCD HAL driver can be used as follows: 00019 00020 (#) Declare a LCD_HandleTypeDef handle structure. 00021 00022 -@- The frequency generator allows you to achieve various LCD frame rates 00023 starting from an LCD input clock frequency (LCDCLK) which can vary 00024 from 32 kHz up to 1 MHz. 00025 00026 (#) Initialize the LCD low level resources by implementing the HAL_LCD_MspInit() API: 00027 00028 (++) Enable the LCDCLK (same as RTCCLK): to configure the RTCCLK/LCDCLK, proceed as follows: 00029 (+++) Use RCC function HAL_RCCEx_PeriphCLKConfig in indicating RCC_PERIPHCLK_LCD and 00030 selected clock source (HSE, LSI or LSE) 00031 00032 (++) LCD pins configuration: 00033 (+++) Enable the clock for the LCD GPIOs. 00034 (+++) Configure these LCD pins as alternate function no-pull. 00035 (++) Enable the LCD interface clock. 00036 00037 00038 (#) Program the Prescaler, Divider, Blink mode, Blink Frequency Duty, Bias, 00039 Voltage Source, Dead Time, Pulse On Duration, Contrast, High drive and Multiplexer 00040 Segment in the Init structure of the LCD handle. 00041 00042 (#) Initialize the LCD registers by calling the HAL_LCD_Init() API. 00043 00044 -@- The HAL_LCD_Init() API configures also the low level Hardware GPIO, CLOCK, ...etc) 00045 by calling the customized HAL_LCD_MspInit() API. 00046 -@- After calling the HAL_LCD_Init() the LCD RAM memory is cleared 00047 00048 (#) Optionally you can update the LCD configuration using these macros: 00049 (++) LCD High Drive using the __HAL_LCD_HIGHDRIVER_ENABLE() and __HAL_LCD_HIGHDRIVER_DISABLE() macros 00050 (++) Voltage output buffer using __HAL_LCD_VOLTAGE_BUFFER_ENABLE() and __HAL_LCD_VOLTAGE_BUFFER_DISABLE() macros 00051 (++) LCD Pulse ON Duration using the __HAL_LCD_PULSEONDURATION_CONFIG() macro 00052 (++) LCD Dead Time using the __HAL_LCD_DEADTIME_CONFIG() macro 00053 (++) The LCD Blink mode and frequency using the __HAL_LCD_BLINK_CONFIG() macro 00054 (++) The LCD Contrast using the __HAL_LCD_CONTRAST_CONFIG() macro 00055 00056 (#) Write to the LCD RAM memory using the HAL_LCD_Write() API, this API can be called 00057 more time to update the different LCD RAM registers before calling 00058 HAL_LCD_UpdateDisplayRequest() API. 00059 00060 (#) The HAL_LCD_Clear() API can be used to clear the LCD RAM memory. 00061 00062 (#) When LCD RAM memory is updated enable the update display request using 00063 the HAL_LCD_UpdateDisplayRequest() API. 00064 00065 [..] LCD and low power modes: 00066 (#) The LCD remain active during Sleep, Low Power run, Low Power Sleep and 00067 STOP modes. 00068 00069 @endverbatim 00070 ****************************************************************************** 00071 * @attention 00072 * 00073 * <h2><center>© COPYRIGHT(c) 2015 STMicroelectronics</center></h2> 00074 * 00075 * Redistribution and use in source and binary forms, with or without modification, 00076 * are permitted provided that the following conditions are met: 00077 * 1. Redistributions of source code must retain the above copyright notice, 00078 * this list of conditions and the following disclaimer. 00079 * 2. Redistributions in binary form must reproduce the above copyright notice, 00080 * this list of conditions and the following disclaimer in the documentation 00081 * and/or other materials provided with the distribution. 00082 * 3. Neither the name of STMicroelectronics nor the names of its contributors 00083 * may be used to endorse or promote products derived from this software 00084 * without specific prior written permission. 00085 * 00086 * THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" 00087 * AND ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE 00088 * IMPLIED WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE 00089 * DISCLAIMED. IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE 00090 * FOR ANY DIRECT, INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL 00091 * DAMAGES (INCLUDING, BUT NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR 00092 * SERVICES; LOSS OF USE, DATA, OR PROFITS; OR BUSINESS INTERRUPTION) HOWEVER 00093 * CAUSED AND ON ANY THEORY OF LIABILITY, WHETHER IN CONTRACT, STRICT LIABILITY, 00094 * OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) ARISING IN ANY WAY OUT OF THE USE 00095 * OF THIS SOFTWARE, EVEN IF ADVISED OF THE POSSIBILITY OF SUCH DAMAGE. 00096 * 00097 ****************************************************************************** 00098 */ 00099 00100 /* Includes ------------------------------------------------------------------*/ 00101 #include "stm32l4xx_hal.h" 00102 00103 #if defined(STM32L476xx) || defined(STM32L486xx) 00104 00105 /** @addtogroup STM32L4xx_HAL_Driver 00106 * @{ 00107 */ 00108 00109 #ifdef HAL_LCD_MODULE_ENABLED 00110 00111 /** @defgroup LCD LCD 00112 * @brief LCD HAL module driver 00113 * @{ 00114 */ 00115 00116 /* Private typedef -----------------------------------------------------------*/ 00117 /* Private define ------------------------------------------------------------*/ 00118 /** @defgroup LCD_Private_Defines LCD Private Defines 00119 * @{ 00120 */ 00121 00122 #define LCD_TIMEOUT_VALUE 1000 00123 00124 /** 00125 * @} 00126 */ 00127 00128 /* Private macro -------------------------------------------------------------*/ 00129 /* Private variables ---------------------------------------------------------*/ 00130 /* Private function prototypes -----------------------------------------------*/ 00131 /* Exported functions --------------------------------------------------------*/ 00132 00133 /** @defgroup LCD_Exported_Functions LCD Exported Functions 00134 * @{ 00135 */ 00136 00137 /** @defgroup LCD_Exported_Functions_Group1 Initialization/de-initialization methods 00138 * @brief Initialization and Configuration functions 00139 * 00140 @verbatim 00141 =============================================================================== 00142 ##### Initialization and Configuration functions ##### 00143 =============================================================================== 00144 [..] 00145 00146 @endverbatim 00147 * @{ 00148 */ 00149 00150 /** 00151 * @brief Initialize the LCD peripheral according to the specified parameters 00152 * in the LCD_InitStruct and initialize the associated handle. 00153 * @note This function can be used only when the LCD is disabled. 00154 * @param hlcd: LCD handle 00155 * @retval None 00156 */ 00157 HAL_StatusTypeDef HAL_LCD_Init(LCD_HandleTypeDef *hlcd) 00158 { 00159 uint32_t tickstart = 0x00; 00160 uint32_t counter = 0; 00161 00162 /* Check the LCD handle allocation */ 00163 if(hlcd == NULL) 00164 { 00165 return HAL_ERROR; 00166 } 00167 00168 /* Check function parameters */ 00169 assert_param(IS_LCD_ALL_INSTANCE(hlcd->Instance)); 00170 assert_param(IS_LCD_PRESCALER(hlcd->Init.Prescaler)); 00171 assert_param(IS_LCD_DIVIDER(hlcd->Init.Divider)); 00172 assert_param(IS_LCD_DUTY(hlcd->Init.Duty)); 00173 assert_param(IS_LCD_BIAS(hlcd->Init.Bias)); 00174 assert_param(IS_LCD_VOLTAGE_SOURCE(hlcd->Init.VoltageSource)); 00175 assert_param(IS_LCD_PULSE_ON_DURATION(hlcd->Init.PulseOnDuration)); 00176 assert_param(IS_LCD_HIGH_DRIVE(hlcd->Init.HighDrive)); 00177 assert_param(IS_LCD_DEAD_TIME(hlcd->Init.DeadTime)); 00178 assert_param(IS_LCD_CONTRAST(hlcd->Init.Contrast)); 00179 assert_param(IS_LCD_BLINK_FREQUENCY(hlcd->Init.BlinkFrequency)); 00180 assert_param(IS_LCD_BLINK_MODE(hlcd->Init.BlinkMode)); 00181 assert_param(IS_LCD_MUX_SEGMENT(hlcd->Init.MuxSegment)); 00182 00183 if(hlcd->State == HAL_LCD_STATE_RESET) 00184 { 00185 /* Allocate lock resource and initialize it */ 00186 hlcd->Lock = HAL_UNLOCKED; 00187 00188 /* Initialize the low level hardware (MSP) */ 00189 HAL_LCD_MspInit(hlcd); 00190 } 00191 00192 hlcd->State = HAL_LCD_STATE_BUSY; 00193 00194 /* Disable the peripheral */ 00195 __HAL_LCD_DISABLE(hlcd); 00196 00197 /* Clear the LCD_RAM registers and enable the display request by setting the UDR bit 00198 in the LCD_SR register */ 00199 for(counter = LCD_RAM_REGISTER0; counter <= LCD_RAM_REGISTER15; counter++) 00200 { 00201 hlcd->Instance->RAM[counter] = 0; 00202 } 00203 /* Enable the display request */ 00204 hlcd->Instance->SR |= LCD_SR_UDR; 00205 00206 /* Configure the LCD Prescaler, Divider, Blink mode and Blink Frequency: 00207 Set PS[3:0] bits according to hlcd->Init.Prescaler value 00208 Set DIV[3:0] bits according to hlcd->Init.Divider value 00209 Set BLINK[1:0] bits according to hlcd->Init.BlinkMode value 00210 Set BLINKF[2:0] bits according to hlcd->Init.BlinkFrequency value 00211 Set DEAD[2:0] bits according to hlcd->Init.DeadTime value 00212 Set PON[2:0] bits according to hlcd->Init.PulseOnDuration value 00213 Set CC[2:0] bits according to hlcd->Init.Contrast value 00214 Set HD bit according to hlcd->Init.HighDrive value */ 00215 MODIFY_REG(hlcd->Instance->FCR, \ 00216 (LCD_FCR_PS | LCD_FCR_DIV | LCD_FCR_BLINK| LCD_FCR_BLINKF | \ 00217 LCD_FCR_DEAD | LCD_FCR_PON | LCD_FCR_CC | LCD_FCR_HD), \ 00218 (hlcd->Init.Prescaler | hlcd->Init.Divider | hlcd->Init.BlinkMode | hlcd->Init.BlinkFrequency | \ 00219 hlcd->Init.DeadTime | hlcd->Init.PulseOnDuration | hlcd->Init.Contrast | hlcd->Init.HighDrive)); 00220 00221 /* Wait until LCD Frame Control Register Synchronization flag (FCRSF) is set in the LCD_SR register 00222 This bit is set by hardware each time the LCD_FCR register is updated in the LCDCLK 00223 domain. It is cleared by hardware when writing to the LCD_FCR register.*/ 00224 LCD_WaitForSynchro(hlcd); 00225 00226 /* Configure the LCD Duty, Bias, Voltage Source, Dead Time, Pulse On Duration and Contrast: 00227 Set DUTY[2:0] bits according to hlcd->Init.Duty value 00228 Set BIAS[1:0] bits according to hlcd->Init.Bias value 00229 Set VSEL bit according to hlcd->Init.VoltageSource value 00230 Set MUX_SEG bit according to hlcd->Init.MuxSegment value */ 00231 MODIFY_REG(hlcd->Instance->CR, \ 00232 (LCD_CR_DUTY | LCD_CR_BIAS | LCD_CR_VSEL | LCD_CR_MUX_SEG), \ 00233 (hlcd->Init.Duty | hlcd->Init.Bias | hlcd->Init.VoltageSource | hlcd->Init.MuxSegment)); 00234 00235 /* Enable the peripheral */ 00236 __HAL_LCD_ENABLE(hlcd); 00237 00238 /* Get timeout */ 00239 tickstart = HAL_GetTick(); 00240 00241 /* Wait Until the LCD is enabled */ 00242 while(__HAL_LCD_GET_FLAG(hlcd, LCD_FLAG_ENS) == RESET) 00243 { 00244 if((HAL_GetTick() - tickstart ) > LCD_TIMEOUT_VALUE) 00245 { 00246 hlcd->ErrorCode = HAL_LCD_ERROR_ENS; 00247 return HAL_TIMEOUT; 00248 } 00249 } 00250 00251 /* Get timeout */ 00252 tickstart = HAL_GetTick(); 00253 00254 /*!< Wait Until the LCD Booster is ready */ 00255 while(__HAL_LCD_GET_FLAG(hlcd, LCD_FLAG_RDY) == RESET) 00256 { 00257 if((HAL_GetTick() - tickstart ) > LCD_TIMEOUT_VALUE) 00258 { 00259 hlcd->ErrorCode = HAL_LCD_ERROR_RDY; 00260 return HAL_TIMEOUT; 00261 } 00262 } 00263 00264 /* Initialize the LCD state */ 00265 hlcd->ErrorCode = HAL_LCD_ERROR_NONE; 00266 hlcd->State= HAL_LCD_STATE_READY; 00267 00268 return HAL_OK; 00269 } 00270 00271 /** 00272 * @brief DeInitialize the LCD peripheral. 00273 * @param hlcd: LCD handle 00274 * @retval HAL status 00275 */ 00276 HAL_StatusTypeDef HAL_LCD_DeInit(LCD_HandleTypeDef *hlcd) 00277 { 00278 /* Check the LCD handle allocation */ 00279 if(hlcd == NULL) 00280 { 00281 return HAL_ERROR; 00282 } 00283 00284 /* Check the parameters */ 00285 assert_param(IS_LCD_ALL_INSTANCE(hlcd->Instance)); 00286 00287 hlcd->State = HAL_LCD_STATE_BUSY; 00288 00289 /* DeInit the low level hardware */ 00290 HAL_LCD_MspDeInit(hlcd); 00291 00292 hlcd->ErrorCode = HAL_LCD_ERROR_NONE; 00293 hlcd->State = HAL_LCD_STATE_RESET; 00294 00295 /* Release Lock */ 00296 __HAL_UNLOCK(hlcd); 00297 00298 return HAL_OK; 00299 } 00300 00301 /** 00302 * @brief DeInitialize the LCD MSP. 00303 * @param hlcd: LCD handle 00304 * @retval None 00305 */ 00306 __weak void HAL_LCD_MspDeInit(LCD_HandleTypeDef *hlcd) 00307 { 00308 /* NOTE: This function should not be modified, when the callback is needed, 00309 the HAL_LCD_MspDeInit it to be implemented in the user file 00310 */ 00311 } 00312 00313 /** 00314 * @brief Initialize the LCD MSP. 00315 * @param hlcd: LCD handle 00316 * @retval None 00317 */ 00318 __weak void HAL_LCD_MspInit(LCD_HandleTypeDef *hlcd) 00319 { 00320 /* NOTE: This function should not be modified, when the callback is needed, 00321 the HAL_LCD_MspInit is to be implemented in the user file 00322 */ 00323 } 00324 00325 /** 00326 * @} 00327 */ 00328 00329 /** @defgroup LCD_Exported_Functions_Group2 IO operation methods 00330 * @brief LCD RAM functions 00331 * 00332 @verbatim 00333 =============================================================================== 00334 ##### IO operation functions ##### 00335 =============================================================================== 00336 [..] Using its double buffer memory the LCD controller ensures the coherency of the 00337 displayed information without having to use interrupts to control LCD_RAM 00338 modification. 00339 The application software can access the first buffer level (LCD_RAM) through 00340 the APB interface. Once it has modified the LCD_RAM using the HAL_LCD_Write() API, 00341 it sets the UDR flag in the LCD_SR register using the HAL_LCD_UpdateDisplayRequest() API. 00342 This UDR flag (update display request) requests the updated information to be 00343 moved into the second buffer level (LCD_DISPLAY). 00344 This operation is done synchronously with the frame (at the beginning of the 00345 next frame), until the update is completed, the LCD_RAM is write protected and 00346 the UDR flag stays high. 00347 Once the update is completed another flag (UDD - Update Display Done) is set and 00348 generates an interrupt if the UDDIE bit in the LCD_FCR register is set. 00349 The time it takes to update LCD_DISPLAY is, in the worst case, one odd and one 00350 even frame. 00351 The update will not occur (UDR = 1 and UDD = 0) until the display is 00352 enabled (LCDEN = 1). 00353 00354 @endverbatim 00355 * @{ 00356 */ 00357 00358 /** 00359 * @brief Write a word in the specific LCD RAM. 00360 * @param hlcd: LCD handle 00361 * @param RAMRegisterIndex: specifies the LCD RAM Register. 00362 * This parameter can be one of the following values: 00363 * @arg LCD_RAM_REGISTER0: LCD RAM Register 0 00364 * @arg LCD_RAM_REGISTER1: LCD RAM Register 1 00365 * @arg LCD_RAM_REGISTER2: LCD RAM Register 2 00366 * @arg LCD_RAM_REGISTER3: LCD RAM Register 3 00367 * @arg LCD_RAM_REGISTER4: LCD RAM Register 4 00368 * @arg LCD_RAM_REGISTER5: LCD RAM Register 5 00369 * @arg LCD_RAM_REGISTER6: LCD RAM Register 6 00370 * @arg LCD_RAM_REGISTER7: LCD RAM Register 7 00371 * @arg LCD_RAM_REGISTER8: LCD RAM Register 8 00372 * @arg LCD_RAM_REGISTER9: LCD RAM Register 9 00373 * @arg LCD_RAM_REGISTER10: LCD RAM Register 10 00374 * @arg LCD_RAM_REGISTER11: LCD RAM Register 11 00375 * @arg LCD_RAM_REGISTER12: LCD RAM Register 12 00376 * @arg LCD_RAM_REGISTER13: LCD RAM Register 13 00377 * @arg LCD_RAM_REGISTER14: LCD RAM Register 14 00378 * @arg LCD_RAM_REGISTER15: LCD RAM Register 15 00379 * @param RAMRegisterMask: specifies the LCD RAM Register Data Mask. 00380 * @param Data: specifies LCD Data Value to be written. 00381 * @retval None 00382 */ 00383 HAL_StatusTypeDef HAL_LCD_Write(LCD_HandleTypeDef *hlcd, uint32_t RAMRegisterIndex, uint32_t RAMRegisterMask, uint32_t Data) 00384 { 00385 uint32_t tickstart = 0x00; 00386 00387 if((hlcd->State == HAL_LCD_STATE_READY) || (hlcd->State == HAL_LCD_STATE_BUSY)) 00388 { 00389 /* Check the parameters */ 00390 assert_param(IS_LCD_RAM_REGISTER(RAMRegisterIndex)); 00391 00392 if(hlcd->State == HAL_LCD_STATE_READY) 00393 { 00394 /* Process Locked */ 00395 __HAL_LOCK(hlcd); 00396 hlcd->State = HAL_LCD_STATE_BUSY; 00397 00398 /* Get timeout */ 00399 tickstart = HAL_GetTick(); 00400 00401 /*!< Wait Until the LCD is ready */ 00402 while(__HAL_LCD_GET_FLAG(hlcd, LCD_FLAG_UDR) != RESET) 00403 { 00404 if((HAL_GetTick() - tickstart ) > LCD_TIMEOUT_VALUE) 00405 { 00406 hlcd->ErrorCode = HAL_LCD_ERROR_UDR; 00407 00408 /* Process Unlocked */ 00409 __HAL_UNLOCK(hlcd); 00410 00411 return HAL_TIMEOUT; 00412 } 00413 } 00414 } 00415 00416 /* Copy the new Data bytes to LCD RAM register */ 00417 MODIFY_REG(hlcd->Instance->RAM[RAMRegisterIndex], ~(RAMRegisterMask), Data); 00418 00419 return HAL_OK; 00420 } 00421 else 00422 { 00423 return HAL_ERROR; 00424 } 00425 } 00426 00427 /** 00428 * @brief Clear the LCD RAM registers. 00429 * @param hlcd: LCD handle 00430 * @retval None 00431 */ 00432 HAL_StatusTypeDef HAL_LCD_Clear(LCD_HandleTypeDef *hlcd) 00433 { 00434 uint32_t tickstart = 0x00; 00435 uint32_t counter = 0; 00436 00437 if((hlcd->State == HAL_LCD_STATE_READY) || (hlcd->State == HAL_LCD_STATE_BUSY)) 00438 { 00439 /* Process Locked */ 00440 __HAL_LOCK(hlcd); 00441 00442 hlcd->State = HAL_LCD_STATE_BUSY; 00443 00444 /* Get timeout */ 00445 tickstart = HAL_GetTick(); 00446 00447 /*!< Wait Until the LCD is ready */ 00448 while(__HAL_LCD_GET_FLAG(hlcd, LCD_FLAG_UDR) != RESET) 00449 { 00450 if((HAL_GetTick() - tickstart ) > LCD_TIMEOUT_VALUE) 00451 { 00452 hlcd->ErrorCode = HAL_LCD_ERROR_UDR; 00453 00454 /* Process Unlocked */ 00455 __HAL_UNLOCK(hlcd); 00456 00457 return HAL_TIMEOUT; 00458 } 00459 } 00460 /* Clear the LCD_RAM registers */ 00461 for(counter = LCD_RAM_REGISTER0; counter <= LCD_RAM_REGISTER15; counter++) 00462 { 00463 hlcd->Instance->RAM[counter] = 0; 00464 } 00465 00466 /* Update the LCD display */ 00467 HAL_LCD_UpdateDisplayRequest(hlcd); 00468 00469 return HAL_OK; 00470 } 00471 else 00472 { 00473 return HAL_ERROR; 00474 } 00475 } 00476 00477 /** 00478 * @brief Enable the Update Display Request. 00479 * @param hlcd: LCD handle 00480 * @note Each time software modifies the LCD_RAM it must set the UDR bit to 00481 * transfer the updated data to the second level buffer. 00482 * The UDR bit stays set until the end of the update and during this 00483 * time the LCD_RAM is write protected. 00484 * @note When the display is disabled, the update is performed for all 00485 * LCD_DISPLAY locations. 00486 * When the display is enabled, the update is performed only for locations 00487 * for which commons are active (depending on DUTY). For example if 00488 * DUTY = 1/2, only the LCD_DISPLAY of COM0 and COM1 will be updated. 00489 * @retval None 00490 */ 00491 HAL_StatusTypeDef HAL_LCD_UpdateDisplayRequest(LCD_HandleTypeDef *hlcd) 00492 { 00493 uint32_t tickstart = 0x00; 00494 00495 /* Clear the Update Display Done flag before starting the update display request */ 00496 __HAL_LCD_CLEAR_FLAG(hlcd, LCD_FLAG_UDD); 00497 00498 /* Enable the display request */ 00499 hlcd->Instance->SR |= LCD_SR_UDR; 00500 00501 /* Get timeout */ 00502 tickstart = HAL_GetTick(); 00503 00504 /*!< Wait Until the LCD display is done */ 00505 while(__HAL_LCD_GET_FLAG(hlcd, LCD_FLAG_UDD) == RESET) 00506 { 00507 if((HAL_GetTick() - tickstart ) > LCD_TIMEOUT_VALUE) 00508 { 00509 hlcd->ErrorCode = HAL_LCD_ERROR_UDD; 00510 00511 /* Process Unlocked */ 00512 __HAL_UNLOCK(hlcd); 00513 00514 return HAL_TIMEOUT; 00515 } 00516 } 00517 00518 hlcd->State = HAL_LCD_STATE_READY; 00519 00520 /* Process Unlocked */ 00521 __HAL_UNLOCK(hlcd); 00522 00523 return HAL_OK; 00524 } 00525 00526 /** 00527 * @} 00528 */ 00529 00530 /** @defgroup LCD_Exported_Functions_Group3 Peripheral State methods 00531 * @brief LCD State functions 00532 * 00533 @verbatim 00534 =============================================================================== 00535 ##### Peripheral State functions ##### 00536 =============================================================================== 00537 [..] 00538 This subsection provides a set of functions allowing to control the LCD: 00539 (+) HAL_LCD_GetState() API can be helpful to check in run-time the state of the LCD peripheral State. 00540 (+) HAL_LCD_GetError() API to return the LCD error code. 00541 @endverbatim 00542 * @{ 00543 */ 00544 00545 /** 00546 * @brief Return the LCD handle state. 00547 * @param hlcd: LCD handle 00548 * @retval HAL state 00549 */ 00550 HAL_LCD_StateTypeDef HAL_LCD_GetState(LCD_HandleTypeDef *hlcd) 00551 { 00552 /* Return LCD handle state */ 00553 return hlcd->State; 00554 } 00555 00556 /** 00557 * @brief Return the LCD error code. 00558 * @param hlcd: LCD handle 00559 * @retval LCD Error Code 00560 */ 00561 uint32_t HAL_LCD_GetError(LCD_HandleTypeDef *hlcd) 00562 { 00563 return hlcd->ErrorCode; 00564 } 00565 00566 /** 00567 * @} 00568 */ 00569 00570 /** 00571 * @} 00572 */ 00573 00574 /** @defgroup LCD_Private_Functions LCD Private Functions 00575 * @{ 00576 */ 00577 00578 /** 00579 * @brief Wait until the LCD FCR register is synchronized in the LCDCLK domain. 00580 * This function must be called after any write operation to LCD_FCR register. 00581 * @retval None 00582 */ 00583 HAL_StatusTypeDef LCD_WaitForSynchro(LCD_HandleTypeDef *hlcd) 00584 { 00585 uint32_t tickstart = 0x00; 00586 00587 /* Get timeout */ 00588 tickstart = HAL_GetTick(); 00589 00590 /* Loop until FCRSF flag is set */ 00591 while(__HAL_LCD_GET_FLAG(hlcd, LCD_FLAG_FCRSF) == RESET) 00592 { 00593 if((HAL_GetTick() - tickstart ) > LCD_TIMEOUT_VALUE) 00594 { 00595 hlcd->ErrorCode = HAL_LCD_ERROR_FCRSF; 00596 return HAL_TIMEOUT; 00597 } 00598 } 00599 00600 return HAL_OK; 00601 } 00602 00603 /** 00604 * @} 00605 */ 00606 00607 /** 00608 * @} 00609 */ 00610 00611 #endif /* STM32L476xx || STM32L486xx */ 00612 00613 #endif /* HAL_LCD_MODULE_ENABLED */ 00614 00615 /** 00616 * @} 00617 */ 00618 00619 /************************ (C) COPYRIGHT STMicroelectronics *****END OF FILE****/ 00620 00621
Generated on Tue Jul 12 2022 11:35:13 by
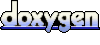