Hal Drivers for L4
Dependents: BSP OneHopeOnePrayer FINAL_AUDIO_RECORD AudioDemo
Fork of STM32L4xx_HAL_Driver by
stm32l4xx_hal_i2c.h
00001 /** 00002 ****************************************************************************** 00003 * @file stm32l4xx_hal_i2c.h 00004 * @author MCD Application Team 00005 * @version V1.1.0 00006 * @date 16-September-2015 00007 * @brief Header file of I2C HAL module. 00008 ****************************************************************************** 00009 * @attention 00010 * 00011 * <h2><center>© COPYRIGHT(c) 2015 STMicroelectronics</center></h2> 00012 * 00013 * Redistribution and use in source and binary forms, with or without modification, 00014 * are permitted provided that the following conditions are met: 00015 * 1. Redistributions of source code must retain the above copyright notice, 00016 * this list of conditions and the following disclaimer. 00017 * 2. Redistributions in binary form must reproduce the above copyright notice, 00018 * this list of conditions and the following disclaimer in the documentation 00019 * and/or other materials provided with the distribution. 00020 * 3. Neither the name of STMicroelectronics nor the names of its contributors 00021 * may be used to endorse or promote products derived from this software 00022 * without specific prior written permission. 00023 * 00024 * THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" 00025 * AND ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE 00026 * IMPLIED WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE 00027 * DISCLAIMED. IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE 00028 * FOR ANY DIRECT, INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL 00029 * DAMAGES (INCLUDING, BUT NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR 00030 * SERVICES; LOSS OF USE, DATA, OR PROFITS; OR BUSINESS INTERRUPTION) HOWEVER 00031 * CAUSED AND ON ANY THEORY OF LIABILITY, WHETHER IN CONTRACT, STRICT LIABILITY, 00032 * OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) ARISING IN ANY WAY OUT OF THE USE 00033 * OF THIS SOFTWARE, EVEN IF ADVISED OF THE POSSIBILITY OF SUCH DAMAGE. 00034 * 00035 ****************************************************************************** 00036 */ 00037 00038 /* Define to prevent recursive inclusion -------------------------------------*/ 00039 #ifndef __STM32L4xx_HAL_I2C_H 00040 #define __STM32L4xx_HAL_I2C_H 00041 00042 #ifdef __cplusplus 00043 extern "C" { 00044 #endif 00045 00046 /* Includes ------------------------------------------------------------------*/ 00047 #include "stm32l4xx_hal_def.h" 00048 00049 /** @addtogroup STM32L4xx_HAL_Driver 00050 * @{ 00051 */ 00052 00053 /** @addtogroup I2C 00054 * @{ 00055 */ 00056 00057 /* Exported types ------------------------------------------------------------*/ 00058 /** @defgroup I2C_Exported_Types I2C Exported Types 00059 * @{ 00060 */ 00061 00062 /** @defgroup I2C_Configuration_Structure_definition I2C Configuration Structure definition 00063 * @brief I2C Configuration Structure definition 00064 * @{ 00065 */ 00066 typedef struct 00067 { 00068 uint32_t Timing; /*!< Specifies the I2C_TIMINGR_register value. 00069 This parameter calculated by referring to I2C initialization 00070 section in Reference manual */ 00071 00072 uint32_t OwnAddress1; /*!< Specifies the first device own address. 00073 This parameter can be a 7-bit or 10-bit address. */ 00074 00075 uint32_t AddressingMode; /*!< Specifies if 7-bit or 10-bit addressing mode is selected. 00076 This parameter can be a value of @ref I2C_addressing_mode */ 00077 00078 uint32_t DualAddressMode; /*!< Specifies if dual addressing mode is selected. 00079 This parameter can be a value of @ref I2C_dual_addressing_mode */ 00080 00081 uint32_t OwnAddress2; /*!< Specifies the second device own address if dual addressing mode is selected 00082 This parameter can be a 7-bit address. */ 00083 00084 uint32_t OwnAddress2Masks; /*!< Specifies the acknowledge mask address second device own address if dual addressing mode is selected 00085 This parameter can be a value of @ref I2C_own_address2_masks */ 00086 00087 uint32_t GeneralCallMode; /*!< Specifies if general call mode is selected. 00088 This parameter can be a value of @ref I2C_general_call_addressing_mode */ 00089 00090 uint32_t NoStretchMode; /*!< Specifies if nostretch mode is selected. 00091 This parameter can be a value of @ref I2C_nostretch_mode */ 00092 00093 }I2C_InitTypeDef; 00094 00095 /** 00096 * @} 00097 */ 00098 00099 /** @defgroup HAL_state_structure_definition HAL state structure definition 00100 * @brief HAL State structure definition 00101 * @{ 00102 */ 00103 00104 typedef enum 00105 { 00106 HAL_I2C_STATE_RESET = 0x00, /*!< I2C not yet initialized or disabled */ 00107 HAL_I2C_STATE_READY = 0x01, /*!< I2C initialized and ready for use */ 00108 HAL_I2C_STATE_BUSY = 0x02, /*!< I2C internal process is ongoing */ 00109 HAL_I2C_STATE_MASTER_BUSY_TX = 0x12, /*!< Master Data Transmission process is ongoing */ 00110 HAL_I2C_STATE_MASTER_BUSY_RX = 0x22, /*!< Master Data Reception process is ongoing */ 00111 HAL_I2C_STATE_SLAVE_BUSY_TX = 0x32, /*!< Slave Data Transmission process is ongoing */ 00112 HAL_I2C_STATE_SLAVE_BUSY_RX = 0x42, /*!< Slave Data Reception process is ongoing */ 00113 HAL_I2C_STATE_MEM_BUSY_TX = 0x52, /*!< Memory Data Transmission process is ongoing */ 00114 HAL_I2C_STATE_MEM_BUSY_RX = 0x62, /*!< Memory Data Reception process is ongoing */ 00115 HAL_I2C_STATE_TIMEOUT = 0x03, /*!< Timeout state */ 00116 HAL_I2C_STATE_ERROR = 0x04 /*!< Reception process is ongoing */ 00117 }HAL_I2C_StateTypeDef; 00118 00119 /** 00120 * @} 00121 */ 00122 00123 /** @defgroup I2C_Error_Code_definition I2C Error Code definition 00124 * @brief I2C Error Code definition 00125 * @{ 00126 */ 00127 #define HAL_I2C_ERROR_NONE ((uint32_t)0x00000000) /*!< No error */ 00128 #define HAL_I2C_ERROR_BERR ((uint32_t)0x00000001) /*!< BERR error */ 00129 #define HAL_I2C_ERROR_ARLO ((uint32_t)0x00000002) /*!< ARLO error */ 00130 #define HAL_I2C_ERROR_AF ((uint32_t)0x00000004) /*!< ACKF error */ 00131 #define HAL_I2C_ERROR_OVR ((uint32_t)0x00000008) /*!< OVR error */ 00132 #define HAL_I2C_ERROR_DMA ((uint32_t)0x00000010) /*!< DMA transfer error */ 00133 #define HAL_I2C_ERROR_TIMEOUT ((uint32_t)0x00000020) /*!< Timeout error */ 00134 #define HAL_I2C_ERROR_SIZE ((uint32_t)0x00000040) /*!< Size Management error */ 00135 /** 00136 * @} 00137 */ 00138 00139 /** @defgroup I2C_handle_Structure_definition I2C handle Structure definition 00140 * @brief I2C handle Structure definition 00141 * @{ 00142 */ 00143 typedef struct 00144 { 00145 I2C_TypeDef *Instance; /*!< I2C registers base address */ 00146 00147 I2C_InitTypeDef Init; /*!< I2C communication parameters */ 00148 00149 uint8_t *pBuffPtr; /*!< Pointer to I2C transfer buffer */ 00150 00151 uint16_t XferSize; /*!< I2C transfer size */ 00152 00153 __IO uint16_t XferCount; /*!< I2C transfer counter */ 00154 00155 DMA_HandleTypeDef *hdmatx; /*!< I2C Tx DMA handle parameters */ 00156 00157 DMA_HandleTypeDef *hdmarx; /*!< I2C Rx DMA handle parameters */ 00158 00159 HAL_LockTypeDef Lock; /*!< I2C locking object */ 00160 00161 __IO HAL_I2C_StateTypeDef State; /*!< I2C communication state */ 00162 00163 __IO uint32_t ErrorCode; /* I2C Error code */ 00164 00165 }I2C_HandleTypeDef; 00166 /** 00167 * @} 00168 */ 00169 00170 /** 00171 * @} 00172 */ 00173 /* Exported constants --------------------------------------------------------*/ 00174 00175 /** @defgroup I2C_Exported_Constants I2C Exported Constants 00176 * @{ 00177 */ 00178 00179 /** @defgroup I2C_addressing_mode I2C addressing mode 00180 * @{ 00181 */ 00182 #define I2C_ADDRESSINGMODE_7BIT ((uint32_t)0x00000001) 00183 #define I2C_ADDRESSINGMODE_10BIT ((uint32_t)0x00000002) 00184 /** 00185 * @} 00186 */ 00187 00188 /** @defgroup I2C_dual_addressing_mode I2C dual addressing mode 00189 * @{ 00190 */ 00191 #define I2C_DUALADDRESS_DISABLE ((uint32_t)0x00000000) 00192 #define I2C_DUALADDRESS_ENABLE I2C_OAR2_OA2EN 00193 /** 00194 * @} 00195 */ 00196 00197 /** @defgroup I2C_own_address2_masks I2C own address2 masks 00198 * @{ 00199 */ 00200 #define I2C_OA2_NOMASK ((uint8_t)0x00) 00201 #define I2C_OA2_MASK01 ((uint8_t)0x01) 00202 #define I2C_OA2_MASK02 ((uint8_t)0x02) 00203 #define I2C_OA2_MASK03 ((uint8_t)0x03) 00204 #define I2C_OA2_MASK04 ((uint8_t)0x04) 00205 #define I2C_OA2_MASK05 ((uint8_t)0x05) 00206 #define I2C_OA2_MASK06 ((uint8_t)0x06) 00207 #define I2C_OA2_MASK07 ((uint8_t)0x07) 00208 /** 00209 * @} 00210 */ 00211 00212 /** @defgroup I2C_general_call_addressing_mode I2C general call addressing mode 00213 * @{ 00214 */ 00215 #define I2C_GENERALCALL_DISABLE ((uint32_t)0x00000000) 00216 #define I2C_GENERALCALL_ENABLE I2C_CR1_GCEN 00217 /** 00218 * @} 00219 */ 00220 00221 /** @defgroup I2C_nostretch_mode I2C nostretch mode 00222 * @{ 00223 */ 00224 #define I2C_NOSTRETCH_DISABLE ((uint32_t)0x00000000) 00225 #define I2C_NOSTRETCH_ENABLE I2C_CR1_NOSTRETCH 00226 /** 00227 * @} 00228 */ 00229 00230 /** @defgroup I2C_Memory_Address_Size I2C Memory Address Size 00231 * @{ 00232 */ 00233 #define I2C_MEMADD_SIZE_8BIT ((uint32_t)0x00000001) 00234 #define I2C_MEMADD_SIZE_16BIT ((uint32_t)0x00000002) 00235 /** 00236 * @} 00237 */ 00238 00239 /** @defgroup I2C_ReloadEndMode_definition I2C ReloadEndMode definition 00240 * @{ 00241 */ 00242 #define I2C_RELOAD_MODE I2C_CR2_RELOAD 00243 #define I2C_AUTOEND_MODE I2C_CR2_AUTOEND 00244 #define I2C_SOFTEND_MODE ((uint32_t)0x00000000) 00245 /** 00246 * @} 00247 */ 00248 00249 /** @defgroup I2C_StartStopMode_definition I2C StartStopMode definition 00250 * @{ 00251 */ 00252 #define I2C_NO_STARTSTOP ((uint32_t)0x00000000) 00253 #define I2C_GENERATE_STOP I2C_CR2_STOP 00254 #define I2C_GENERATE_START_READ (uint32_t)(I2C_CR2_START | I2C_CR2_RD_WRN) 00255 #define I2C_GENERATE_START_WRITE I2C_CR2_START 00256 /** 00257 * @} 00258 */ 00259 00260 /** @defgroup I2C_Interrupt_configuration_definition I2C Interrupt configuration definition 00261 * @brief I2C Interrupt definition 00262 * Elements values convention: 0xXXXXXXXX 00263 * - XXXXXXXX : Interrupt control mask 00264 * @{ 00265 */ 00266 #define I2C_IT_ERRI I2C_CR1_ERRIE 00267 #define I2C_IT_TCI I2C_CR1_TCIE 00268 #define I2C_IT_STOPI I2C_CR1_STOPIE 00269 #define I2C_IT_NACKI I2C_CR1_NACKIE 00270 #define I2C_IT_ADDRI I2C_CR1_ADDRIE 00271 #define I2C_IT_RXI I2C_CR1_RXIE 00272 #define I2C_IT_TXI I2C_CR1_TXIE 00273 00274 /** 00275 * @} 00276 */ 00277 00278 00279 /** @defgroup I2C_Flag_definition I2C Flag definition 00280 * @{ 00281 */ 00282 #define I2C_FLAG_TXE I2C_ISR_TXE 00283 #define I2C_FLAG_TXIS I2C_ISR_TXIS 00284 #define I2C_FLAG_RXNE I2C_ISR_RXNE 00285 #define I2C_FLAG_ADDR I2C_ISR_ADDR 00286 #define I2C_FLAG_AF I2C_ISR_NACKF 00287 #define I2C_FLAG_STOPF I2C_ISR_STOPF 00288 #define I2C_FLAG_TC I2C_ISR_TC 00289 #define I2C_FLAG_TCR I2C_ISR_TCR 00290 #define I2C_FLAG_BERR I2C_ISR_BERR 00291 #define I2C_FLAG_ARLO I2C_ISR_ARLO 00292 #define I2C_FLAG_OVR I2C_ISR_OVR 00293 #define I2C_FLAG_PECERR I2C_ISR_PECERR 00294 #define I2C_FLAG_TIMEOUT I2C_ISR_TIMEOUT 00295 #define I2C_FLAG_ALERT I2C_ISR_ALERT 00296 #define I2C_FLAG_BUSY I2C_ISR_BUSY 00297 #define I2C_FLAG_DIR I2C_ISR_DIR 00298 /** 00299 * @} 00300 */ 00301 00302 /** 00303 * @} 00304 */ 00305 00306 /* Exported macros -----------------------------------------------------------*/ 00307 00308 /** @defgroup I2C_Exported_Macros I2C Exported Macros 00309 * @{ 00310 */ 00311 00312 /** @brief Reset I2C handle state. 00313 * @param __HANDLE__: specifies the I2C Handle. 00314 * @retval None 00315 */ 00316 #define __HAL_I2C_RESET_HANDLE_STATE(__HANDLE__) ((__HANDLE__)->State = HAL_I2C_STATE_RESET) 00317 00318 /** @brief Enable the specified I2C interrupt. 00319 * @param __HANDLE__: specifies the I2C Handle. 00320 * @param __INTERRUPT__: specifies the interrupt source to enable. 00321 * This parameter can be one of the following values: 00322 * @arg I2C_IT_ERRI: Errors interrupt enable 00323 * @arg I2C_IT_TCI: Transfer complete interrupt enable 00324 * @arg I2C_IT_STOPI: STOP detection interrupt enable 00325 * @arg I2C_IT_NACKI: NACK received interrupt enable 00326 * @arg I2C_IT_ADDRI: Address match interrupt enable 00327 * @arg I2C_IT_RXI: RX interrupt enable 00328 * @arg I2C_IT_TXI: TX interrupt enable 00329 * 00330 * @retval None 00331 */ 00332 00333 #define __HAL_I2C_ENABLE_IT(__HANDLE__, __INTERRUPT__) ((__HANDLE__)->Instance->CR1 |= (__INTERRUPT__)) 00334 00335 /** @brief Disable the specified I2C interrupt. 00336 * @param __HANDLE__: specifies the I2C Handle. 00337 * @param __INTERRUPT__: specifies the interrupt source to disable. 00338 * This parameter can be one of the following values: 00339 * @arg I2C_IT_ERRI: Errors interrupt enable 00340 * @arg I2C_IT_TCI: Transfer complete interrupt enable 00341 * @arg I2C_IT_STOPI: STOP detection interrupt enable 00342 * @arg I2C_IT_NACKI: NACK received interrupt enable 00343 * @arg I2C_IT_ADDRI: Address match interrupt enable 00344 * @arg I2C_IT_RXI: RX interrupt enable 00345 * @arg I2C_IT_TXI: TX interrupt enable 00346 * 00347 * @retval None 00348 */ 00349 #define __HAL_I2C_DISABLE_IT(__HANDLE__, __INTERRUPT__) ((__HANDLE__)->Instance->CR1 &= (~(__INTERRUPT__))) 00350 00351 /** @brief Check whether the specified I2C interrupt source is enabled or not. 00352 * @param __HANDLE__: specifies the I2C Handle. 00353 * @param __INTERRUPT__: specifies the I2C interrupt source to check. 00354 * This parameter can be one of the following values: 00355 * @arg I2C_IT_ERRI: Errors interrupt enable 00356 * @arg I2C_IT_TCI: Transfer complete interrupt enable 00357 * @arg I2C_IT_STOPI: STOP detection interrupt enable 00358 * @arg I2C_IT_NACKI: NACK received interrupt enable 00359 * @arg I2C_IT_ADDRI: Address match interrupt enable 00360 * @arg I2C_IT_RXI: RX interrupt enable 00361 * @arg I2C_IT_TXI: TX interrupt enable 00362 * 00363 * @retval The new state of __INTERRUPT__ (TRUE or FALSE). 00364 */ 00365 #define __HAL_I2C_GET_IT_SOURCE(__HANDLE__, __INTERRUPT__) ((((__HANDLE__)->Instance->CR1 & (__INTERRUPT__)) == (__INTERRUPT__)) ? SET : RESET) 00366 00367 /** @brief Check whether the specified I2C flag is set or not. 00368 * @param __HANDLE__: specifies the I2C Handle. 00369 * @param __FLAG__: specifies the flag to check. 00370 * This parameter can be one of the following values: 00371 * @arg I2C_FLAG_TXE: Transmit data register empty 00372 * @arg I2C_FLAG_TXIS: Transmit interrupt status 00373 * @arg I2C_FLAG_RXNE: Receive data register not empty 00374 * @arg I2C_FLAG_ADDR: Address matched (slave mode) 00375 * @arg I2C_FLAG_AF: Acknowledge failure received flag 00376 * @arg I2C_FLAG_STOPF: STOP detection flag 00377 * @arg I2C_FLAG_TC: Transfer complete (master mode) 00378 * @arg I2C_FLAG_TCR: Transfer complete reload 00379 * @arg I2C_FLAG_BERR: Bus error 00380 * @arg I2C_FLAG_ARLO: Arbitration lost 00381 * @arg I2C_FLAG_OVR: Overrun/Underrun 00382 * @arg I2C_FLAG_PECERR: PEC error in reception 00383 * @arg I2C_FLAG_TIMEOUT: Timeout or Tlow detection flag 00384 * @arg I2C_FLAG_ALERT: SMBus alert 00385 * @arg I2C_FLAG_BUSY: Bus busy 00386 * @arg I2C_FLAG_DIR: Transfer direction (slave mode) 00387 * 00388 * @retval The new state of __FLAG__ (TRUE or FALSE). 00389 */ 00390 #define I2C_FLAG_MASK ((uint32_t)0x0001FFFF) 00391 #define __HAL_I2C_GET_FLAG(__HANDLE__, __FLAG__) (((((__HANDLE__)->Instance->ISR) & ((__FLAG__) & I2C_FLAG_MASK)) == ((__FLAG__) & I2C_FLAG_MASK))) 00392 00393 /** @brief Clear the I2C pending flags which are cleared by writing 1 in a specific bit. 00394 * @param __HANDLE__: specifies the I2C Handle. 00395 * @param __FLAG__: specifies the flag to clear. 00396 * This parameter can be any combination of the following values: 00397 * @arg I2C_FLAG_ADDR: Address matched (slave mode) 00398 * @arg I2C_FLAG_AF: Acknowledge failure received flag 00399 * @arg I2C_FLAG_STOPF: STOP detection flag 00400 * @arg I2C_FLAG_BERR: Bus error 00401 * @arg I2C_FLAG_ARLO: Arbitration lost 00402 * @arg I2C_FLAG_OVR: Overrun/Underrun 00403 * @arg I2C_FLAG_PECERR: PEC error in reception 00404 * @arg I2C_FLAG_TIMEOUT: Timeout or Tlow detection flag 00405 * @arg I2C_FLAG_ALERT: SMBus alert 00406 * 00407 * @retval None 00408 */ 00409 #define __HAL_I2C_CLEAR_FLAG(__HANDLE__, __FLAG__) ((__HANDLE__)->Instance->ICR = ((__FLAG__) & I2C_FLAG_MASK)) 00410 00411 /** @brief Enable the specified I2C peripheral. 00412 * @param __HANDLE__: specifies the I2C Handle. 00413 * @retval None 00414 */ 00415 #define __HAL_I2C_ENABLE(__HANDLE__) (SET_BIT((__HANDLE__)->Instance->CR1, I2C_CR1_PE)) 00416 00417 /** @brief Disable the specified I2C peripheral. 00418 * @param __HANDLE__: specifies the I2C Handle. 00419 * @retval None 00420 */ 00421 #define __HAL_I2C_DISABLE(__HANDLE__) (CLEAR_BIT((__HANDLE__)->Instance->CR1, I2C_CR1_PE)) 00422 00423 /** 00424 * @} 00425 */ 00426 00427 /* Include I2C HAL Extended module */ 00428 #include "stm32l4xx_hal_i2c_ex.h" 00429 00430 /* Exported functions --------------------------------------------------------*/ 00431 /** @addtogroup I2C_Exported_Functions 00432 * @{ 00433 */ 00434 00435 /** @addtogroup I2C_Exported_Functions_Group1 Initialization and de-initialization functions 00436 * @{ 00437 */ 00438 /* Initialization and de-initialization functions******************************/ 00439 HAL_StatusTypeDef HAL_I2C_Init(I2C_HandleTypeDef *hi2c); 00440 HAL_StatusTypeDef HAL_I2C_DeInit (I2C_HandleTypeDef *hi2c); 00441 void HAL_I2C_MspInit(I2C_HandleTypeDef *hi2c); 00442 void HAL_I2C_MspDeInit(I2C_HandleTypeDef *hi2c); 00443 /** 00444 * @} 00445 */ 00446 00447 /** @addtogroup I2C_Exported_Functions_Group2 Input and Output operation functions 00448 * @{ 00449 */ 00450 /* IO operation functions ****************************************************/ 00451 /******* Blocking mode: Polling */ 00452 HAL_StatusTypeDef HAL_I2C_Master_Transmit(I2C_HandleTypeDef *hi2c, uint16_t DevAddress, uint8_t *pData, uint16_t Size, uint32_t Timeout); 00453 HAL_StatusTypeDef HAL_I2C_Master_Receive(I2C_HandleTypeDef *hi2c, uint16_t DevAddress, uint8_t *pData, uint16_t Size, uint32_t Timeout); 00454 HAL_StatusTypeDef HAL_I2C_Slave_Transmit(I2C_HandleTypeDef *hi2c, uint8_t *pData, uint16_t Size, uint32_t Timeout); 00455 HAL_StatusTypeDef HAL_I2C_Slave_Receive(I2C_HandleTypeDef *hi2c, uint8_t *pData, uint16_t Size, uint32_t Timeout); 00456 HAL_StatusTypeDef HAL_I2C_Mem_Write(I2C_HandleTypeDef *hi2c, uint16_t DevAddress, uint16_t MemAddress, uint16_t MemAddSize, uint8_t *pData, uint16_t Size, uint32_t Timeout); 00457 HAL_StatusTypeDef HAL_I2C_Mem_Read(I2C_HandleTypeDef *hi2c, uint16_t DevAddress, uint16_t MemAddress, uint16_t MemAddSize, uint8_t *pData, uint16_t Size, uint32_t Timeout); 00458 HAL_StatusTypeDef HAL_I2C_IsDeviceReady(I2C_HandleTypeDef *hi2c, uint16_t DevAddress, uint32_t Trials, uint32_t Timeout); 00459 00460 /******* Non-Blocking mode: Interrupt */ 00461 HAL_StatusTypeDef HAL_I2C_Master_Transmit_IT(I2C_HandleTypeDef *hi2c, uint16_t DevAddress, uint8_t *pData, uint16_t Size); 00462 HAL_StatusTypeDef HAL_I2C_Master_Receive_IT(I2C_HandleTypeDef *hi2c, uint16_t DevAddress, uint8_t *pData, uint16_t Size); 00463 HAL_StatusTypeDef HAL_I2C_Slave_Transmit_IT(I2C_HandleTypeDef *hi2c, uint8_t *pData, uint16_t Size); 00464 HAL_StatusTypeDef HAL_I2C_Slave_Receive_IT(I2C_HandleTypeDef *hi2c, uint8_t *pData, uint16_t Size); 00465 HAL_StatusTypeDef HAL_I2C_Mem_Write_IT(I2C_HandleTypeDef *hi2c, uint16_t DevAddress, uint16_t MemAddress, uint16_t MemAddSize, uint8_t *pData, uint16_t Size); 00466 HAL_StatusTypeDef HAL_I2C_Mem_Read_IT(I2C_HandleTypeDef *hi2c, uint16_t DevAddress, uint16_t MemAddress, uint16_t MemAddSize, uint8_t *pData, uint16_t Size); 00467 00468 /******* Non-Blocking mode: DMA */ 00469 HAL_StatusTypeDef HAL_I2C_Master_Transmit_DMA(I2C_HandleTypeDef *hi2c, uint16_t DevAddress, uint8_t *pData, uint16_t Size); 00470 HAL_StatusTypeDef HAL_I2C_Master_Receive_DMA(I2C_HandleTypeDef *hi2c, uint16_t DevAddress, uint8_t *pData, uint16_t Size); 00471 HAL_StatusTypeDef HAL_I2C_Slave_Transmit_DMA(I2C_HandleTypeDef *hi2c, uint8_t *pData, uint16_t Size); 00472 HAL_StatusTypeDef HAL_I2C_Slave_Receive_DMA(I2C_HandleTypeDef *hi2c, uint8_t *pData, uint16_t Size); 00473 HAL_StatusTypeDef HAL_I2C_Mem_Write_DMA(I2C_HandleTypeDef *hi2c, uint16_t DevAddress, uint16_t MemAddress, uint16_t MemAddSize, uint8_t *pData, uint16_t Size); 00474 HAL_StatusTypeDef HAL_I2C_Mem_Read_DMA(I2C_HandleTypeDef *hi2c, uint16_t DevAddress, uint16_t MemAddress, uint16_t MemAddSize, uint8_t *pData, uint16_t Size); 00475 /** 00476 * @} 00477 */ 00478 00479 /** @addtogroup I2C_IRQ_Handler_and_Callbacks IRQ Handler and Callbacks 00480 * @{ 00481 */ 00482 /******* I2C IRQHandler and Callbacks used in non blocking modes (Interrupt and DMA) */ 00483 void HAL_I2C_EV_IRQHandler(I2C_HandleTypeDef *hi2c); 00484 void HAL_I2C_ER_IRQHandler(I2C_HandleTypeDef *hi2c); 00485 void HAL_I2C_MasterTxCpltCallback(I2C_HandleTypeDef *hi2c); 00486 void HAL_I2C_MasterRxCpltCallback(I2C_HandleTypeDef *hi2c); 00487 void HAL_I2C_SlaveTxCpltCallback(I2C_HandleTypeDef *hi2c); 00488 void HAL_I2C_SlaveRxCpltCallback(I2C_HandleTypeDef *hi2c); 00489 void HAL_I2C_MemTxCpltCallback(I2C_HandleTypeDef *hi2c); 00490 void HAL_I2C_MemRxCpltCallback(I2C_HandleTypeDef *hi2c); 00491 void HAL_I2C_ErrorCallback(I2C_HandleTypeDef *hi2c); 00492 /** 00493 * @} 00494 */ 00495 00496 /** @addtogroup I2C_Exported_Functions_Group3 Peripheral State and Errors functions 00497 * @{ 00498 */ 00499 /* Peripheral State and Errors functions *************************************/ 00500 HAL_I2C_StateTypeDef HAL_I2C_GetState(I2C_HandleTypeDef *hi2c); 00501 uint32_t HAL_I2C_GetError(I2C_HandleTypeDef *hi2c); 00502 00503 /** 00504 * @} 00505 */ 00506 00507 /** 00508 * @} 00509 */ 00510 00511 /* Private constants ---------------------------------------------------------*/ 00512 /** @defgroup I2C_Private_Constants I2C Private Constants 00513 * @{ 00514 */ 00515 00516 /** 00517 * @} 00518 */ 00519 00520 /* Private macros ------------------------------------------------------------*/ 00521 /** @defgroup I2C_Private_Macro I2C Private Macros 00522 * @{ 00523 */ 00524 00525 #define IS_I2C_ADDRESSING_MODE(MODE) (((MODE) == I2C_ADDRESSINGMODE_7BIT) || \ 00526 ((MODE) == I2C_ADDRESSINGMODE_10BIT)) 00527 00528 #define IS_I2C_DUAL_ADDRESS(ADDRESS) (((ADDRESS) == I2C_DUALADDRESS_DISABLE) || \ 00529 ((ADDRESS) == I2C_DUALADDRESS_ENABLE)) 00530 00531 #define IS_I2C_OWN_ADDRESS2_MASK(MASK) (((MASK) == I2C_OA2_NOMASK) || \ 00532 ((MASK) == I2C_OA2_MASK01) || \ 00533 ((MASK) == I2C_OA2_MASK02) || \ 00534 ((MASK) == I2C_OA2_MASK03) || \ 00535 ((MASK) == I2C_OA2_MASK04) || \ 00536 ((MASK) == I2C_OA2_MASK05) || \ 00537 ((MASK) == I2C_OA2_MASK06) || \ 00538 ((MASK) == I2C_OA2_MASK07)) 00539 00540 #define IS_I2C_GENERAL_CALL(CALL) (((CALL) == I2C_GENERALCALL_DISABLE) || \ 00541 ((CALL) == I2C_GENERALCALL_ENABLE)) 00542 00543 #define IS_I2C_NO_STRETCH(STRETCH) (((STRETCH) == I2C_NOSTRETCH_DISABLE) || \ 00544 ((STRETCH) == I2C_NOSTRETCH_ENABLE)) 00545 00546 #define IS_I2C_MEMADD_SIZE(SIZE) (((SIZE) == I2C_MEMADD_SIZE_8BIT) || \ 00547 ((SIZE) == I2C_MEMADD_SIZE_16BIT)) 00548 00549 00550 #define IS_TRANSFER_MODE(MODE) (((MODE) == I2C_RELOAD_MODE) || \ 00551 ((MODE) == I2C_AUTOEND_MODE) || \ 00552 ((MODE) == I2C_SOFTEND_MODE)) 00553 00554 #define IS_TRANSFER_REQUEST(REQUEST) (((REQUEST) == I2C_GENERATE_STOP) || \ 00555 ((REQUEST) == I2C_GENERATE_START_READ) || \ 00556 ((REQUEST) == I2C_GENERATE_START_WRITE) || \ 00557 ((REQUEST) == I2C_NO_STARTSTOP)) 00558 00559 00560 #define I2C_RESET_CR2(__HANDLE__) ((__HANDLE__)->Instance->CR2 &= (uint32_t)~((uint32_t)(I2C_CR2_SADD | I2C_CR2_HEAD10R | I2C_CR2_NBYTES | I2C_CR2_RELOAD | I2C_CR2_RD_WRN))) 00561 00562 #define IS_I2C_OWN_ADDRESS1(ADDRESS1) ((ADDRESS1) <= (uint32_t)0x000003FF) 00563 #define IS_I2C_OWN_ADDRESS2(ADDRESS2) ((ADDRESS2) <= (uint16_t)0x00FF) 00564 00565 #define I2C_MEM_ADD_MSB(__ADDRESS__) ((uint8_t)((uint16_t)(((uint16_t)((__ADDRESS__) & (uint16_t)(0xFF00))) >> 8))) 00566 #define I2C_MEM_ADD_LSB(__ADDRESS__) ((uint8_t)((uint16_t)((__ADDRESS__) & (uint16_t)(0x00FF)))) 00567 00568 #define I2C_GENERATE_START(__ADDMODE__,__ADDRESS__) (((__ADDMODE__) == I2C_ADDRESSINGMODE_7BIT) ? (uint32_t)((((uint32_t)(__ADDRESS__) & (I2C_CR2_SADD)) | (I2C_CR2_START) | (I2C_CR2_AUTOEND)) & (~I2C_CR2_RD_WRN)) : \ 00569 (uint32_t)((((uint32_t)(__ADDRESS__) & (I2C_CR2_SADD)) | (I2C_CR2_ADD10) | (I2C_CR2_START)) & (~I2C_CR2_RD_WRN))) 00570 /** 00571 * @} 00572 */ 00573 00574 /* Private Functions ---------------------------------------------------------*/ 00575 /** @defgroup I2C_Private_Functions I2C Private Functions 00576 * @{ 00577 */ 00578 /* Private functions are defined in stm32l4xx_hal_i2c.c file */ 00579 /** 00580 * @} 00581 */ 00582 00583 /** 00584 * @} 00585 */ 00586 00587 /** 00588 * @} 00589 */ 00590 00591 #ifdef __cplusplus 00592 } 00593 #endif 00594 00595 00596 #endif /* __STM32L4xx_HAL_I2C_H */ 00597 00598 /************************ (C) COPYRIGHT STMicroelectronics *****END OF FILE****/ 00599
Generated on Tue Jul 12 2022 11:35:12 by
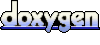