Hal Drivers for L4
Dependents: BSP OneHopeOnePrayer FINAL_AUDIO_RECORD AudioDemo
Fork of STM32L4xx_HAL_Driver by
stm32l4xx_hal_i2c.c File Reference
I2C HAL module driver. This file provides firmware functions to manage the following functionalities of the Inter Integrated Circuit (I2C) peripheral: + Initialization and de-initialization functions + IO operation functions + Peripheral State and Errors functions. More...
Go to the source code of this file.
Functions | |
static void | I2C_DMAMasterTransmitCplt (DMA_HandleTypeDef *hdma) |
DMA I2C master transmit process complete callback. | |
static void | I2C_DMAMasterReceiveCplt (DMA_HandleTypeDef *hdma) |
DMA I2C master receive process complete callback. | |
static void | I2C_DMASlaveTransmitCplt (DMA_HandleTypeDef *hdma) |
DMA I2C slave transmit process complete callback. | |
static void | I2C_DMASlaveReceiveCplt (DMA_HandleTypeDef *hdma) |
DMA I2C slave receive process complete callback. | |
static void | I2C_DMAMemTransmitCplt (DMA_HandleTypeDef *hdma) |
DMA I2C Memory Write process complete callback. | |
static void | I2C_DMAMemReceiveCplt (DMA_HandleTypeDef *hdma) |
DMA I2C Memory Read process complete callback. | |
static void | I2C_DMAError (DMA_HandleTypeDef *hdma) |
DMA I2C communication error callback. | |
static HAL_StatusTypeDef | I2C_RequestMemoryWrite (I2C_HandleTypeDef *hi2c, uint16_t DevAddress, uint16_t MemAddress, uint16_t MemAddSize, uint32_t Timeout) |
Master sends target device address followed by internal memory address for write request. | |
static HAL_StatusTypeDef | I2C_RequestMemoryRead (I2C_HandleTypeDef *hi2c, uint16_t DevAddress, uint16_t MemAddress, uint16_t MemAddSize, uint32_t Timeout) |
Master sends target device address followed by internal memory address for read request. | |
static HAL_StatusTypeDef | I2C_WaitOnFlagUntilTimeout (I2C_HandleTypeDef *hi2c, uint32_t Flag, FlagStatus Status, uint32_t Timeout) |
This function handles I2C Communication Timeout. | |
static HAL_StatusTypeDef | I2C_WaitOnTXISFlagUntilTimeout (I2C_HandleTypeDef *hi2c, uint32_t Timeout) |
This function handles I2C Communication Timeout for specific usage of TXIS flag. | |
static HAL_StatusTypeDef | I2C_WaitOnRXNEFlagUntilTimeout (I2C_HandleTypeDef *hi2c, uint32_t Timeout) |
This function handles I2C Communication Timeout for specific usage of RXNE flag. | |
static HAL_StatusTypeDef | I2C_WaitOnSTOPFlagUntilTimeout (I2C_HandleTypeDef *hi2c, uint32_t Timeout) |
This function handles I2C Communication Timeout for specific usage of STOP flag. | |
static HAL_StatusTypeDef | I2C_IsAcknowledgeFailed (I2C_HandleTypeDef *hi2c, uint32_t Timeout) |
This function handles Acknowledge failed detection during an I2C Communication. | |
static HAL_StatusTypeDef | I2C_MasterTransmit_ISR (I2C_HandleTypeDef *hi2c) |
Handle Interrupt Flags Master Transmit Mode. | |
static HAL_StatusTypeDef | I2C_MasterReceive_ISR (I2C_HandleTypeDef *hi2c) |
Handle Interrupt Flags Master Receive Mode. | |
static HAL_StatusTypeDef | I2C_SlaveTransmit_ISR (I2C_HandleTypeDef *hi2c) |
Handle Interrupt Flags Slave Transmit Mode. | |
static HAL_StatusTypeDef | I2C_SlaveReceive_ISR (I2C_HandleTypeDef *hi2c) |
Handle Interrupt Flags Slave Receive Mode. | |
static void | I2C_TransferConfig (I2C_HandleTypeDef *hi2c, uint16_t DevAddress, uint8_t Size, uint32_t Mode, uint32_t Request) |
Handles I2Cx communication when starting transfer or during transfer (TC or TCR flag are set). | |
HAL_StatusTypeDef | HAL_I2C_Init (I2C_HandleTypeDef *hi2c) |
Initializes the I2C according to the specified parameters in the I2C_InitTypeDef and initialize the associated handle. | |
HAL_StatusTypeDef | HAL_I2C_DeInit (I2C_HandleTypeDef *hi2c) |
DeInitialize the I2C peripheral. | |
__weak void | HAL_I2C_MspInit (I2C_HandleTypeDef *hi2c) |
Initialize the I2C MSP. | |
__weak void | HAL_I2C_MspDeInit (I2C_HandleTypeDef *hi2c) |
DeInitialize the I2C MSP. | |
HAL_StatusTypeDef | HAL_I2C_Master_Transmit (I2C_HandleTypeDef *hi2c, uint16_t DevAddress, uint8_t *pData, uint16_t Size, uint32_t Timeout) |
Transmits in master mode an amount of data in blocking mode. | |
HAL_StatusTypeDef | HAL_I2C_Master_Receive (I2C_HandleTypeDef *hi2c, uint16_t DevAddress, uint8_t *pData, uint16_t Size, uint32_t Timeout) |
Receives in master mode an amount of data in blocking mode. | |
HAL_StatusTypeDef | HAL_I2C_Slave_Transmit (I2C_HandleTypeDef *hi2c, uint8_t *pData, uint16_t Size, uint32_t Timeout) |
Transmits in slave mode an amount of data in blocking mode. | |
HAL_StatusTypeDef | HAL_I2C_Slave_Receive (I2C_HandleTypeDef *hi2c, uint8_t *pData, uint16_t Size, uint32_t Timeout) |
Receive in slave mode an amount of data in blocking mode. | |
HAL_StatusTypeDef | HAL_I2C_Master_Transmit_IT (I2C_HandleTypeDef *hi2c, uint16_t DevAddress, uint8_t *pData, uint16_t Size) |
Transmit in master mode an amount of data in non-blocking mode with Interrupt. | |
HAL_StatusTypeDef | HAL_I2C_Master_Receive_IT (I2C_HandleTypeDef *hi2c, uint16_t DevAddress, uint8_t *pData, uint16_t Size) |
Receive in master mode an amount of data in non-blocking mode with Interrupt. | |
HAL_StatusTypeDef | HAL_I2C_Slave_Transmit_IT (I2C_HandleTypeDef *hi2c, uint8_t *pData, uint16_t Size) |
Transmit in slave mode an amount of data in non-blocking mode with Interrupt. | |
HAL_StatusTypeDef | HAL_I2C_Slave_Receive_IT (I2C_HandleTypeDef *hi2c, uint8_t *pData, uint16_t Size) |
Receive in slave mode an amount of data in non-blocking mode with Interrupt. | |
HAL_StatusTypeDef | HAL_I2C_Master_Transmit_DMA (I2C_HandleTypeDef *hi2c, uint16_t DevAddress, uint8_t *pData, uint16_t Size) |
Transmit in master mode an amount of data in non-blocking mode with DMA. | |
HAL_StatusTypeDef | HAL_I2C_Master_Receive_DMA (I2C_HandleTypeDef *hi2c, uint16_t DevAddress, uint8_t *pData, uint16_t Size) |
Receive in master mode an amount of data in non-blocking mode with DMA. | |
HAL_StatusTypeDef | HAL_I2C_Slave_Transmit_DMA (I2C_HandleTypeDef *hi2c, uint8_t *pData, uint16_t Size) |
Transmit in slave mode an amount of data in non-blocking mode with DMA. | |
HAL_StatusTypeDef | HAL_I2C_Slave_Receive_DMA (I2C_HandleTypeDef *hi2c, uint8_t *pData, uint16_t Size) |
Receive in slave mode an amount of data in non-blocking mode with DMA. | |
HAL_StatusTypeDef | HAL_I2C_Mem_Write (I2C_HandleTypeDef *hi2c, uint16_t DevAddress, uint16_t MemAddress, uint16_t MemAddSize, uint8_t *pData, uint16_t Size, uint32_t Timeout) |
Write an amount of data in blocking mode to a specific memory address. | |
HAL_StatusTypeDef | HAL_I2C_Mem_Read (I2C_HandleTypeDef *hi2c, uint16_t DevAddress, uint16_t MemAddress, uint16_t MemAddSize, uint8_t *pData, uint16_t Size, uint32_t Timeout) |
Read an amount of data in blocking mode from a specific memory address. | |
HAL_StatusTypeDef | HAL_I2C_Mem_Write_IT (I2C_HandleTypeDef *hi2c, uint16_t DevAddress, uint16_t MemAddress, uint16_t MemAddSize, uint8_t *pData, uint16_t Size) |
Write an amount of data in non-blocking mode with Interrupt to a specific memory address. | |
HAL_StatusTypeDef | HAL_I2C_Mem_Read_IT (I2C_HandleTypeDef *hi2c, uint16_t DevAddress, uint16_t MemAddress, uint16_t MemAddSize, uint8_t *pData, uint16_t Size) |
Read an amount of data in non-blocking mode with Interrupt from a specific memory address. | |
HAL_StatusTypeDef | HAL_I2C_Mem_Write_DMA (I2C_HandleTypeDef *hi2c, uint16_t DevAddress, uint16_t MemAddress, uint16_t MemAddSize, uint8_t *pData, uint16_t Size) |
Write an amount of data in non-blocking mode with DMA to a specific memory address. | |
HAL_StatusTypeDef | HAL_I2C_Mem_Read_DMA (I2C_HandleTypeDef *hi2c, uint16_t DevAddress, uint16_t MemAddress, uint16_t MemAddSize, uint8_t *pData, uint16_t Size) |
Reads an amount of data in non-blocking mode with DMA from a specific memory address. | |
HAL_StatusTypeDef | HAL_I2C_IsDeviceReady (I2C_HandleTypeDef *hi2c, uint16_t DevAddress, uint32_t Trials, uint32_t Timeout) |
Checks if target device is ready for communication. | |
void | HAL_I2C_EV_IRQHandler (I2C_HandleTypeDef *hi2c) |
This function handles I2C event interrupt request. | |
void | HAL_I2C_ER_IRQHandler (I2C_HandleTypeDef *hi2c) |
This function handles I2C error interrupt request. | |
__weak void | HAL_I2C_MasterTxCpltCallback (I2C_HandleTypeDef *hi2c) |
Master Tx Transfer completed callbacks. | |
__weak void | HAL_I2C_MasterRxCpltCallback (I2C_HandleTypeDef *hi2c) |
Master Rx Transfer completed callbacks. | |
__weak void | HAL_I2C_SlaveTxCpltCallback (I2C_HandleTypeDef *hi2c) |
Slave Tx Transfer completed callbacks. | |
__weak void | HAL_I2C_SlaveRxCpltCallback (I2C_HandleTypeDef *hi2c) |
Slave Rx Transfer completed callbacks. | |
__weak void | HAL_I2C_MemTxCpltCallback (I2C_HandleTypeDef *hi2c) |
Memory Tx Transfer completed callbacks. | |
__weak void | HAL_I2C_MemRxCpltCallback (I2C_HandleTypeDef *hi2c) |
Memory Rx Transfer completed callbacks. | |
__weak void | HAL_I2C_ErrorCallback (I2C_HandleTypeDef *hi2c) |
I2C error callbacks. | |
HAL_I2C_StateTypeDef | HAL_I2C_GetState (I2C_HandleTypeDef *hi2c) |
Return the I2C handle state. | |
uint32_t | HAL_I2C_GetError (I2C_HandleTypeDef *hi2c) |
Return the I2C error code. |
Detailed Description
I2C HAL module driver. This file provides firmware functions to manage the following functionalities of the Inter Integrated Circuit (I2C) peripheral: + Initialization and de-initialization functions + IO operation functions + Peripheral State and Errors functions.
- Version:
- V1.1.0
- Date:
- 16-September-2015
============================================================================== ##### How to use this driver ##### ============================================================================== [..] The I2C HAL driver can be used as follows: (#) Declare a I2C_HandleTypeDef handle structure, for example: I2C_HandleTypeDef hi2c; (#)Initialize the I2C low level resources by implementing the HAL_I2C_MspInit() API: (##) Enable the I2Cx interface clock (##) I2C pins configuration (+++) Enable the clock for the I2C GPIOs (+++) Configure I2C pins as alternate function open-drain (##) NVIC configuration if you need to use interrupt process (+++) Configure the I2Cx interrupt priority (+++) Enable the NVIC I2C IRQ Channel (##) DMA Configuration if you need to use DMA process (+++) Declare a DMA_HandleTypeDef handle structure for the transmit or receive channel (+++) Enable the DMAx interface clock using (+++) Configure the DMA handle parameters (+++) Configure the DMA Tx or Rx channel (+++) Associate the initialized DMA handle to the hi2c DMA Tx or Rx handle (+++) Configure the priority and enable the NVIC for the transfer complete interrupt on the DMA Tx or Rx channel (#) Configure the Communication Clock Timing, Own Address1, Master Addressing Mode, Dual Addressing mode, Own Address2, Own Address2 Mask, General call and Nostretch mode in the hi2c Init structure. (#) Initialize the I2C registers by calling the HAL_I2C_Init(), configures also the low level Hardware (GPIO, CLOCK, NVIC...etc) by calling the customized HAL_I2C_MspInit(&hi2c) API. (#) To check if target device is ready for communication, use the function HAL_I2C_IsDeviceReady() (#) For I2C IO and IO MEM operations, three operation modes are available within this driver : *** Polling mode IO operation *** ================================= [..] (+) Transmit in master mode an amount of data in blocking mode using HAL_I2C_Master_Transmit() (+) Receive in master mode an amount of data in blocking mode using HAL_I2C_Master_Receive() (+) Transmit in slave mode an amount of data in blocking mode using HAL_I2C_Slave_Transmit() (+) Receive in slave mode an amount of data in blocking mode using HAL_I2C_Slave_Receive() *** Polling mode IO MEM operation *** ===================================== [..] (+) Write an amount of data in blocking mode to a specific memory address using HAL_I2C_Mem_Write() (+) Read an amount of data in blocking mode from a specific memory address using HAL_I2C_Mem_Read() *** Interrupt mode IO operation *** =================================== [..] (+) Transmit in master mode an amount of data in non-blocking mode using HAL_I2C_Master_Transmit_IT() (+) At transmission end of transfer HAL_I2C_MasterTxCpltCallback() is executed and user can add his own code by customization of function pointer HAL_I2C_MasterTxCpltCallback() (+) Receive in master mode an amount of data in non-blocking mode using HAL_I2C_Master_Receive_IT() (+) At reception end of transfer HAL_I2C_MasterRxCpltCallback() is executed and user can add his own code by customization of function pointer HAL_I2C_MasterRxCpltCallback() (+) Transmit in slave mode an amount of data in non-blocking mode using HAL_I2C_Slave_Transmit_IT() (+) At transmission end of transfer HAL_I2C_SlaveTxCpltCallback() is executed and user can add his own code by customization of function pointer HAL_I2C_SlaveTxCpltCallback() (+) Receive in slave mode an amount of data in non-blocking mode using HAL_I2C_Slave_Receive_IT() (+) At reception end of transfer HAL_I2C_SlaveRxCpltCallback() is executed and user can add his own code by customization of function pointer HAL_I2C_SlaveRxCpltCallback() (+) In case of transfer Error, HAL_I2C_ErrorCallback() function is executed and user can add his own code by customization of function pointer HAL_I2C_ErrorCallback() *** Interrupt mode IO MEM operation *** ======================================= [..] (+) Write an amount of data in non-blocking mode with Interrupt to a specific memory address using HAL_I2C_Mem_Write_IT() (+) At MEM end of write transfer HAL_I2C_MemTxCpltCallback() is executed and user can add his own code by customization of function pointer HAL_I2C_MemTxCpltCallback() (+) Read an amount of data in non-blocking mode with Interrupt from a specific memory address using HAL_I2C_Mem_Read_IT() (+) At MEM end of read transfer HAL_I2C_MemRxCpltCallback() is executed and user can add his own code by customization of function pointer HAL_I2C_MemRxCpltCallback() (+) In case of transfer Error, HAL_I2C_ErrorCallback() function is executed and user can add his own code by customization of function pointer HAL_I2C_ErrorCallback() *** DMA mode IO operation *** ============================== [..] (+) Transmit in master mode an amount of data in non-blocking mode (DMA) using HAL_I2C_Master_Transmit_DMA() (+) At transmission end of transfer HAL_I2C_MasterTxCpltCallback() is executed and user can add his own code by customization of function pointer HAL_I2C_MasterTxCpltCallback() (+) Receive in master mode an amount of data in non-blocking mode (DMA) using HAL_I2C_Master_Receive_DMA() (+) At reception end of transfer HAL_I2C_MasterRxCpltCallback() is executed and user can add his own code by customization of function pointer HAL_I2C_MasterRxCpltCallback() (+) Transmit in slave mode an amount of data in non-blocking mode (DMA) using HAL_I2C_Slave_Transmit_DMA() (+) At transmission end of transfer HAL_I2C_SlaveTxCpltCallback() is executed and user can add his own code by customization of function pointer HAL_I2C_SlaveTxCpltCallback() (+) Receive in slave mode an amount of data in non-blocking mode (DMA) using HAL_I2C_Slave_Receive_DMA() (+) At reception end of transfer HAL_I2C_SlaveRxCpltCallback() is executed and user can add his own code by customization of function pointer HAL_I2C_SlaveRxCpltCallback() (+) In case of transfer Error, HAL_I2C_ErrorCallback() function is executed and user can add his own code by customization of function pointer HAL_I2C_ErrorCallback() *** DMA mode IO MEM operation *** ================================= [..] (+) Write an amount of data in non-blocking mode with DMA to a specific memory address using HAL_I2C_Mem_Write_DMA() (+) At MEM end of write transfer HAL_I2C_MemTxCpltCallback() is executed and user can add his own code by customization of function pointer HAL_I2C_MemTxCpltCallback() (+) Read an amount of data in non-blocking mode with DMA from a specific memory address using HAL_I2C_Mem_Read_DMA() (+) At MEM end of read transfer HAL_I2C_MemRxCpltCallback() is executed and user can add his own code by customization of function pointer HAL_I2C_MemRxCpltCallback() (+) In case of transfer Error, HAL_I2C_ErrorCallback() function is executed and user can add his own code by customization of function pointer HAL_I2C_ErrorCallback() *** I2C HAL driver macros list *** ================================== [..] Below the list of most used macros in I2C HAL driver. (+) __HAL_I2C_ENABLE: Enable the I2C peripheral (+) __HAL_I2C_DISABLE: Disable the I2C peripheral (+) __HAL_I2C_GET_FLAG : Checks whether the specified I2C flag is set or not (+) __HAL_I2C_CLEAR_FLAG : Clear the specified I2C pending flag (+) __HAL_I2C_ENABLE_IT: Enable the specified I2C interrupt (+) __HAL_I2C_DISABLE_IT: Disable the specified I2C interrupt [..] (@) You can refer to the I2C HAL driver header file for more useful macros
- Attention:
© COPYRIGHT(c) 2015 STMicroelectronics
Redistribution and use in source and binary forms, with or without modification, are permitted provided that the following conditions are met: 1. Redistributions of source code must retain the above copyright notice, this list of conditions and the following disclaimer. 2. Redistributions in binary form must reproduce the above copyright notice, this list of conditions and the following disclaimer in the documentation and/or other materials provided with the distribution. 3. Neither the name of STMicroelectronics nor the names of its contributors may be used to endorse or promote products derived from this software without specific prior written permission.
THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE POSSIBILITY OF SUCH DAMAGE.
Definition in file stm32l4xx_hal_i2c.c.
Generated on Tue Jul 12 2022 11:35:17 by
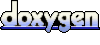