Hal Drivers for L4
Dependents: BSP OneHopeOnePrayer FINAL_AUDIO_RECORD AudioDemo
Fork of STM32L4xx_HAL_Driver by
stm32l4xx_hal_dma.h
00001 /** 00002 ****************************************************************************** 00003 * @file stm32l4xx_hal_dma.h 00004 * @author MCD Application Team 00005 * @version V1.1.0 00006 * @date 16-September-2015 00007 * @brief Header file of DMA HAL module. 00008 ****************************************************************************** 00009 * @attention 00010 * 00011 * <h2><center>© COPYRIGHT(c) 2015 STMicroelectronics</center></h2> 00012 * 00013 * Redistribution and use in source and binary forms, with or without modification, 00014 * are permitted provided that the following conditions are met: 00015 * 1. Redistributions of source code must retain the above copyright notice, 00016 * this list of conditions and the following disclaimer. 00017 * 2. Redistributions in binary form must reproduce the above copyright notice, 00018 * this list of conditions and the following disclaimer in the documentation 00019 * and/or other materials provided with the distribution. 00020 * 3. Neither the name of STMicroelectronics nor the names of its contributors 00021 * may be used to endorse or promote products derived from this software 00022 * without specific prior written permission. 00023 * 00024 * THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" 00025 * AND ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE 00026 * IMPLIED WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE 00027 * DISCLAIMED. IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE 00028 * FOR ANY DIRECT, INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL 00029 * DAMAGES (INCLUDING, BUT NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR 00030 * SERVICES; LOSS OF USE, DATA, OR PROFITS; OR BUSINESS INTERRUPTION) HOWEVER 00031 * CAUSED AND ON ANY THEORY OF LIABILITY, WHETHER IN CONTRACT, STRICT LIABILITY, 00032 * OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) ARISING IN ANY WAY OUT OF THE USE 00033 * OF THIS SOFTWARE, EVEN IF ADVISED OF THE POSSIBILITY OF SUCH DAMAGE. 00034 * 00035 ****************************************************************************** 00036 */ 00037 00038 /* Define to prevent recursive inclusion -------------------------------------*/ 00039 #ifndef __STM32L4xx_HAL_DMA_H 00040 #define __STM32L4xx_HAL_DMA_H 00041 00042 #ifdef __cplusplus 00043 extern "C" { 00044 #endif 00045 00046 /* Includes ------------------------------------------------------------------*/ 00047 #include "stm32l4xx_hal_def.h" 00048 00049 /** @addtogroup STM32L4xx_HAL_Driver 00050 * @{ 00051 */ 00052 00053 /** @addtogroup DMA 00054 * @{ 00055 */ 00056 00057 /* Exported types ------------------------------------------------------------*/ 00058 /** @defgroup DMA_Exported_Types DMA Exported Types 00059 * @{ 00060 */ 00061 00062 /** 00063 * @brief DMA Configuration Structure definition 00064 */ 00065 typedef struct 00066 { 00067 uint32_t Request; /*!< Specifies the request selected for the specified channel. 00068 This parameter can be a value of @ref DMA_request */ 00069 00070 uint32_t Direction; /*!< Specifies if the data will be transferred from memory to peripheral, 00071 from memory to memory or from peripheral to memory. 00072 This parameter can be a value of @ref DMA_Data_transfer_direction */ 00073 00074 uint32_t PeriphInc; /*!< Specifies whether the Peripheral address register should be incremented or not. 00075 This parameter can be a value of @ref DMA_Peripheral_incremented_mode */ 00076 00077 uint32_t MemInc; /*!< Specifies whether the memory address register should be incremented or not. 00078 This parameter can be a value of @ref DMA_Memory_incremented_mode */ 00079 00080 uint32_t PeriphDataAlignment; /*!< Specifies the Peripheral data width. 00081 This parameter can be a value of @ref DMA_Peripheral_data_size */ 00082 00083 uint32_t MemDataAlignment; /*!< Specifies the Memory data width. 00084 This parameter can be a value of @ref DMA_Memory_data_size */ 00085 00086 uint32_t Mode; /*!< Specifies the operation mode of the DMAy Channelx. 00087 This parameter can be a value of @ref DMA_mode 00088 @note The circular buffer mode cannot be used if the memory-to-memory 00089 data transfer is configured on the selected Channel */ 00090 00091 uint32_t Priority; /*!< Specifies the software priority for the DMAy Channelx. 00092 This parameter can be a value of @ref DMA_Priority_level */ 00093 } DMA_InitTypeDef; 00094 00095 /** 00096 * @brief DMA Configuration enumeration values definition 00097 */ 00098 typedef enum 00099 { 00100 DMA_MODE = 0, /*!< Control related DMA mode Parameter in DMA_InitTypeDef */ 00101 DMA_PRIORITY = 1 /*!< Control related priority level Parameter in DMA_InitTypeDef */ 00102 00103 } DMA_ControlTypeDef; 00104 00105 /** 00106 * @brief HAL DMA State structures definition 00107 */ 00108 typedef enum 00109 { 00110 HAL_DMA_STATE_RESET = 0x00, /*!< DMA not yet initialized or disabled */ 00111 HAL_DMA_STATE_READY = 0x01, /*!< DMA process success and ready for use */ 00112 HAL_DMA_STATE_READY_HALF = 0x11, /*!< DMA Half process success */ 00113 HAL_DMA_STATE_BUSY = 0x02, /*!< DMA process is ongoing */ 00114 HAL_DMA_STATE_TIMEOUT = 0x03, /*!< DMA timeout state */ 00115 HAL_DMA_STATE_ERROR = 0x04 /*!< DMA error state */ 00116 }HAL_DMA_StateTypeDef; 00117 00118 /** 00119 * @brief HAL DMA Error Code structure definition 00120 */ 00121 typedef enum 00122 { 00123 HAL_DMA_FULL_TRANSFER = 0x00, /*!< Full transfer */ 00124 HAL_DMA_HALF_TRANSFER = 0x01 /*!< Half Transfer */ 00125 }HAL_DMA_LevelCompleteTypeDef; 00126 00127 00128 /** 00129 * @brief DMA handle Structure definition 00130 */ 00131 typedef struct __DMA_HandleTypeDef 00132 { 00133 DMA_Channel_TypeDef *Instance; /*!< Register base address */ 00134 00135 DMA_InitTypeDef Init; /*!< DMA communication parameters */ 00136 00137 HAL_LockTypeDef Lock; /*!< DMA locking object */ 00138 00139 __IO HAL_DMA_StateTypeDef State; /*!< DMA transfer state */ 00140 00141 void *Parent; /*!< Parent object state */ 00142 00143 void (* XferCpltCallback)(struct __DMA_HandleTypeDef * hdma); /*!< DMA transfer complete callback */ 00144 00145 void (* XferHalfCpltCallback)(struct __DMA_HandleTypeDef * hdma); /*!< DMA Half transfer complete callback */ 00146 00147 void (* XferErrorCallback)(struct __DMA_HandleTypeDef * hdma); /*!< DMA transfer error callback */ 00148 00149 __IO uint32_t ErrorCode; /*!< DMA Error code */ 00150 }DMA_HandleTypeDef; 00151 00152 /** 00153 * @} 00154 */ 00155 00156 /* Exported constants --------------------------------------------------------*/ 00157 00158 /** @defgroup DMA_Exported_Constants DMA Exported Constants 00159 * @{ 00160 */ 00161 00162 /** @defgroup DMA_Error_Code DMA Error Code 00163 * @{ 00164 */ 00165 #define HAL_DMA_ERROR_NONE ((uint32_t)0x00000000) /*!< No error */ 00166 #define HAL_DMA_ERROR_TE ((uint32_t)0x00000001) /*!< Transfer error */ 00167 #define HAL_DMA_ERROR_TIMEOUT ((uint32_t)0x00000020) /*!< Timeout error */ 00168 /** 00169 * @} 00170 */ 00171 00172 /** @defgroup DMA_request DMA request 00173 * @{ 00174 */ 00175 #define DMA_REQUEST_0 ((uint32_t)0x00000000) 00176 #define DMA_REQUEST_1 ((uint32_t)0x00000001) 00177 #define DMA_REQUEST_2 ((uint32_t)0x00000002) 00178 #define DMA_REQUEST_3 ((uint32_t)0x00000003) 00179 #define DMA_REQUEST_4 ((uint32_t)0x00000004) 00180 #define DMA_REQUEST_5 ((uint32_t)0x00000005) 00181 #define DMA_REQUEST_6 ((uint32_t)0x00000006) 00182 #define DMA_REQUEST_7 ((uint32_t)0x00000007) 00183 /** 00184 * @} 00185 */ 00186 00187 /** @defgroup DMA_Data_transfer_direction DMA Data transfer direction 00188 * @{ 00189 */ 00190 #define DMA_PERIPH_TO_MEMORY ((uint32_t)0x00000000) /*!< Peripheral to memory direction */ 00191 #define DMA_MEMORY_TO_PERIPH ((uint32_t)DMA_CCR_DIR) /*!< Memory to peripheral direction */ 00192 #define DMA_MEMORY_TO_MEMORY ((uint32_t)DMA_CCR_MEM2MEM) /*!< Memory to memory direction */ 00193 /** 00194 * @} 00195 */ 00196 00197 /** @defgroup DMA_Peripheral_incremented_mode DMA Peripheral incremented mode 00198 * @{ 00199 */ 00200 #define DMA_PINC_ENABLE ((uint32_t)DMA_CCR_PINC) /*!< Peripheral increment mode Enable */ 00201 #define DMA_PINC_DISABLE ((uint32_t)0x00000000) /*!< Peripheral increment mode Disable */ 00202 /** 00203 * @} 00204 */ 00205 00206 /** @defgroup DMA_Memory_incremented_mode DMA Memory incremented mode 00207 * @{ 00208 */ 00209 #define DMA_MINC_ENABLE ((uint32_t)DMA_CCR_MINC) /*!< Memory increment mode Enable */ 00210 #define DMA_MINC_DISABLE ((uint32_t)0x00000000) /*!< Memory increment mode Disable */ 00211 /** 00212 * @} 00213 */ 00214 00215 /** @defgroup DMA_Peripheral_data_size DMA Peripheral data size 00216 * @{ 00217 */ 00218 #define DMA_PDATAALIGN_BYTE ((uint32_t)0x00000000) /*!< Peripheral data alignment : Byte */ 00219 #define DMA_PDATAALIGN_HALFWORD ((uint32_t)DMA_CCR_PSIZE_0) /*!< Peripheral data alignment : HalfWord */ 00220 #define DMA_PDATAALIGN_WORD ((uint32_t)DMA_CCR_PSIZE_1) /*!< Peripheral data alignment : Word */ 00221 /** 00222 * @} 00223 */ 00224 00225 /** @defgroup DMA_Memory_data_size DMA Memory data size 00226 * @{ 00227 */ 00228 #define DMA_MDATAALIGN_BYTE ((uint32_t)0x00000000) /*!< Memory data alignment : Byte */ 00229 #define DMA_MDATAALIGN_HALFWORD ((uint32_t)DMA_CCR_MSIZE_0) /*!< Memory data alignment : HalfWord */ 00230 #define DMA_MDATAALIGN_WORD ((uint32_t)DMA_CCR_MSIZE_1) /*!< Memory data alignment : Word */ 00231 /** 00232 * @} 00233 */ 00234 00235 /** @defgroup DMA_mode DMA mode 00236 * @{ 00237 */ 00238 #define DMA_NORMAL ((uint32_t)0x00000000) /*!< Normal mode */ 00239 #define DMA_CIRCULAR ((uint32_t)DMA_CCR_CIRC) /*!< Circular mode */ 00240 /** 00241 * @} 00242 */ 00243 00244 /** @defgroup DMA_Priority_level DMA Priority level 00245 * @{ 00246 */ 00247 #define DMA_PRIORITY_LOW ((uint32_t)0x00000000) /*!< Priority level : Low */ 00248 #define DMA_PRIORITY_MEDIUM ((uint32_t)DMA_CCR_PL_0) /*!< Priority level : Medium */ 00249 #define DMA_PRIORITY_HIGH ((uint32_t)DMA_CCR_PL_1) /*!< Priority level : High */ 00250 #define DMA_PRIORITY_VERY_HIGH ((uint32_t)DMA_CCR_PL) /*!< Priority level : Very_High */ 00251 /** 00252 * @} 00253 */ 00254 00255 00256 /** @defgroup DMA_interrupt_enable_definitions DMA interrupt enable definitions 00257 * @{ 00258 */ 00259 #define DMA_IT_TC ((uint32_t)DMA_CCR_TCIE) 00260 #define DMA_IT_HT ((uint32_t)DMA_CCR_HTIE) 00261 #define DMA_IT_TE ((uint32_t)DMA_CCR_TEIE) 00262 /** 00263 * @} 00264 */ 00265 00266 /** @defgroup DMA_flag_definitions DMA flag definitions 00267 * @{ 00268 */ 00269 #define DMA_FLAG_GL1 ((uint32_t)0x00000001) 00270 #define DMA_FLAG_TC1 ((uint32_t)0x00000002) 00271 #define DMA_FLAG_HT1 ((uint32_t)0x00000004) 00272 #define DMA_FLAG_TE1 ((uint32_t)0x00000008) 00273 #define DMA_FLAG_GL2 ((uint32_t)0x00000010) 00274 #define DMA_FLAG_TC2 ((uint32_t)0x00000020) 00275 #define DMA_FLAG_HT2 ((uint32_t)0x00000040) 00276 #define DMA_FLAG_TE2 ((uint32_t)0x00000080) 00277 #define DMA_FLAG_GL3 ((uint32_t)0x00000100) 00278 #define DMA_FLAG_TC3 ((uint32_t)0x00000200) 00279 #define DMA_FLAG_HT3 ((uint32_t)0x00000400) 00280 #define DMA_FLAG_TE3 ((uint32_t)0x00000800) 00281 #define DMA_FLAG_GL4 ((uint32_t)0x00001000) 00282 #define DMA_FLAG_TC4 ((uint32_t)0x00002000) 00283 #define DMA_FLAG_HT4 ((uint32_t)0x00004000) 00284 #define DMA_FLAG_TE4 ((uint32_t)0x00008000) 00285 #define DMA_FLAG_GL5 ((uint32_t)0x00010000) 00286 #define DMA_FLAG_TC5 ((uint32_t)0x00020000) 00287 #define DMA_FLAG_HT5 ((uint32_t)0x00040000) 00288 #define DMA_FLAG_TE5 ((uint32_t)0x00080000) 00289 #define DMA_FLAG_GL6 ((uint32_t)0x00100000) 00290 #define DMA_FLAG_TC6 ((uint32_t)0x00200000) 00291 #define DMA_FLAG_HT6 ((uint32_t)0x00400000) 00292 #define DMA_FLAG_TE6 ((uint32_t)0x00800000) 00293 #define DMA_FLAG_GL7 ((uint32_t)0x01000000) 00294 #define DMA_FLAG_TC7 ((uint32_t)0x02000000) 00295 #define DMA_FLAG_HT7 ((uint32_t)0x04000000) 00296 #define DMA_FLAG_TE7 ((uint32_t)0x08000000) 00297 /** 00298 * @} 00299 */ 00300 00301 /** 00302 * @} 00303 */ 00304 00305 /* Exported macros -----------------------------------------------------------*/ 00306 /** @defgroup DMA_Exported_Macros DMA Exported Macros 00307 * @{ 00308 */ 00309 00310 /** @brief Reset DMA handle state. 00311 * @param __HANDLE__: DMA handle 00312 * @retval None 00313 */ 00314 #define __HAL_DMA_RESET_HANDLE_STATE(__HANDLE__) ((__HANDLE__)->State = HAL_DMA_STATE_RESET) 00315 00316 /** 00317 * @brief Enable the specified DMA Channel. 00318 * @param __HANDLE__: DMA handle 00319 * @retval None 00320 */ 00321 #define __HAL_DMA_ENABLE(__HANDLE__) ((__HANDLE__)->Instance->CCR |= DMA_CCR_EN) 00322 00323 /** 00324 * @brief Disable the specified DMA Channel. 00325 * @param __HANDLE__: DMA handle 00326 * @retval None 00327 */ 00328 #define __HAL_DMA_DISABLE(__HANDLE__) ((__HANDLE__)->Instance->CCR &= ~DMA_CCR_EN) 00329 00330 00331 /* Interrupt & Flag management */ 00332 00333 /** 00334 * @brief Return the current DMA Channel transfer complete flag. 00335 * @param __HANDLE__: DMA handle 00336 * @retval The specified transfer complete flag index. 00337 */ 00338 00339 #define __HAL_DMA_GET_TC_FLAG_INDEX(__HANDLE__) \ 00340 (((uint32_t)((__HANDLE__)->Instance) == ((uint32_t)DMA1_Channel1))? DMA_FLAG_TC1 :\ 00341 ((uint32_t)((__HANDLE__)->Instance) == ((uint32_t)DMA2_Channel1))? DMA_FLAG_TC1 :\ 00342 ((uint32_t)((__HANDLE__)->Instance) == ((uint32_t)DMA1_Channel2))? DMA_FLAG_TC2 :\ 00343 ((uint32_t)((__HANDLE__)->Instance) == ((uint32_t)DMA2_Channel2))? DMA_FLAG_TC2 :\ 00344 ((uint32_t)((__HANDLE__)->Instance) == ((uint32_t)DMA1_Channel3))? DMA_FLAG_TC3 :\ 00345 ((uint32_t)((__HANDLE__)->Instance) == ((uint32_t)DMA2_Channel3))? DMA_FLAG_TC3 :\ 00346 ((uint32_t)((__HANDLE__)->Instance) == ((uint32_t)DMA1_Channel4))? DMA_FLAG_TC4 :\ 00347 ((uint32_t)((__HANDLE__)->Instance) == ((uint32_t)DMA2_Channel4))? DMA_FLAG_TC4 :\ 00348 ((uint32_t)((__HANDLE__)->Instance) == ((uint32_t)DMA1_Channel5))? DMA_FLAG_TC5 :\ 00349 ((uint32_t)((__HANDLE__)->Instance) == ((uint32_t)DMA2_Channel5))? DMA_FLAG_TC5 :\ 00350 ((uint32_t)((__HANDLE__)->Instance) == ((uint32_t)DMA1_Channel6))? DMA_FLAG_TC6 :\ 00351 ((uint32_t)((__HANDLE__)->Instance) == ((uint32_t)DMA2_Channel6))? DMA_FLAG_TC6 :\ 00352 DMA_FLAG_TC7) 00353 00354 /** 00355 * @brief Return the current DMA Channel half transfer complete flag. 00356 * @param __HANDLE__: DMA handle 00357 * @retval The specified half transfer complete flag index. 00358 */ 00359 #define __HAL_DMA_GET_HT_FLAG_INDEX(__HANDLE__)\ 00360 (((uint32_t)((__HANDLE__)->Instance) == ((uint32_t)DMA1_Channel1))? DMA_FLAG_HT1 :\ 00361 ((uint32_t)((__HANDLE__)->Instance) == ((uint32_t)DMA2_Channel1))? DMA_FLAG_HT1 :\ 00362 ((uint32_t)((__HANDLE__)->Instance) == ((uint32_t)DMA1_Channel2))? DMA_FLAG_HT2 :\ 00363 ((uint32_t)((__HANDLE__)->Instance) == ((uint32_t)DMA2_Channel2))? DMA_FLAG_HT2 :\ 00364 ((uint32_t)((__HANDLE__)->Instance) == ((uint32_t)DMA1_Channel3))? DMA_FLAG_HT3 :\ 00365 ((uint32_t)((__HANDLE__)->Instance) == ((uint32_t)DMA2_Channel3))? DMA_FLAG_HT3 :\ 00366 ((uint32_t)((__HANDLE__)->Instance) == ((uint32_t)DMA1_Channel4))? DMA_FLAG_HT4 :\ 00367 ((uint32_t)((__HANDLE__)->Instance) == ((uint32_t)DMA2_Channel4))? DMA_FLAG_HT4 :\ 00368 ((uint32_t)((__HANDLE__)->Instance) == ((uint32_t)DMA1_Channel5))? DMA_FLAG_HT5 :\ 00369 ((uint32_t)((__HANDLE__)->Instance) == ((uint32_t)DMA2_Channel5))? DMA_FLAG_HT5 :\ 00370 ((uint32_t)((__HANDLE__)->Instance) == ((uint32_t)DMA1_Channel6))? DMA_FLAG_HT6 :\ 00371 ((uint32_t)((__HANDLE__)->Instance) == ((uint32_t)DMA2_Channel6))? DMA_FLAG_HT6 :\ 00372 DMA_FLAG_HT7) 00373 00374 /** 00375 * @brief Return the current DMA Channel transfer error flag. 00376 * @param __HANDLE__: DMA handle 00377 * @retval The specified transfer error flag index. 00378 */ 00379 #define __HAL_DMA_GET_TE_FLAG_INDEX(__HANDLE__)\ 00380 (((uint32_t)((__HANDLE__)->Instance) == ((uint32_t)DMA1_Channel1))? DMA_FLAG_TE1 :\ 00381 ((uint32_t)((__HANDLE__)->Instance) == ((uint32_t)DMA2_Channel1))? DMA_FLAG_TE1 :\ 00382 ((uint32_t)((__HANDLE__)->Instance) == ((uint32_t)DMA1_Channel2))? DMA_FLAG_TE2 :\ 00383 ((uint32_t)((__HANDLE__)->Instance) == ((uint32_t)DMA2_Channel2))? DMA_FLAG_TE2 :\ 00384 ((uint32_t)((__HANDLE__)->Instance) == ((uint32_t)DMA1_Channel3))? DMA_FLAG_TE3 :\ 00385 ((uint32_t)((__HANDLE__)->Instance) == ((uint32_t)DMA2_Channel3))? DMA_FLAG_TE3 :\ 00386 ((uint32_t)((__HANDLE__)->Instance) == ((uint32_t)DMA1_Channel4))? DMA_FLAG_TE4 :\ 00387 ((uint32_t)((__HANDLE__)->Instance) == ((uint32_t)DMA2_Channel4))? DMA_FLAG_TE4 :\ 00388 ((uint32_t)((__HANDLE__)->Instance) == ((uint32_t)DMA1_Channel5))? DMA_FLAG_TE5 :\ 00389 ((uint32_t)((__HANDLE__)->Instance) == ((uint32_t)DMA2_Channel5))? DMA_FLAG_TE5 :\ 00390 ((uint32_t)((__HANDLE__)->Instance) == ((uint32_t)DMA1_Channel6))? DMA_FLAG_TE6 :\ 00391 ((uint32_t)((__HANDLE__)->Instance) == ((uint32_t)DMA2_Channel6))? DMA_FLAG_TE6 :\ 00392 DMA_FLAG_TE7) 00393 00394 /** 00395 * @brief Return the current DMA Channel Global interrupt flag. 00396 * @param __HANDLE__: DMA handle 00397 * @retval The specified transfer error flag index. 00398 */ 00399 #define __HAL_DMA_GET_GI_FLAG_INDEX(__HANDLE__)\ 00400 (((uint32_t)((__HANDLE__)->Instance) == ((uint32_t)DMA1_Channel1))? DMA_ISR_GIF1 :\ 00401 ((uint32_t)((__HANDLE__)->Instance) == ((uint32_t)DMA2_Channel1))? DMA_ISR_GIF1 :\ 00402 ((uint32_t)((__HANDLE__)->Instance) == ((uint32_t)DMA1_Channel2))? DMA_ISR_GIF2 :\ 00403 ((uint32_t)((__HANDLE__)->Instance) == ((uint32_t)DMA2_Channel2))? DMA_ISR_GIF2 :\ 00404 ((uint32_t)((__HANDLE__)->Instance) == ((uint32_t)DMA1_Channel3))? DMA_ISR_GIF3 :\ 00405 ((uint32_t)((__HANDLE__)->Instance) == ((uint32_t)DMA2_Channel3))? DMA_ISR_GIF3 :\ 00406 ((uint32_t)((__HANDLE__)->Instance) == ((uint32_t)DMA1_Channel4))? DMA_ISR_GIF4 :\ 00407 ((uint32_t)((__HANDLE__)->Instance) == ((uint32_t)DMA2_Channel4))? DMA_ISR_GIF4 :\ 00408 ((uint32_t)((__HANDLE__)->Instance) == ((uint32_t)DMA1_Channel5))? DMA_ISR_GIF5 :\ 00409 ((uint32_t)((__HANDLE__)->Instance) == ((uint32_t)DMA2_Channel5))? DMA_ISR_GIF5 :\ 00410 ((uint32_t)((__HANDLE__)->Instance) == ((uint32_t)DMA1_Channel6))? DMA_ISR_GIF6 :\ 00411 ((uint32_t)((__HANDLE__)->Instance) == ((uint32_t)DMA2_Channel6))? DMA_ISR_GIF6 :\ 00412 DMA_ISR_GIF7) 00413 00414 /** 00415 * @brief Get the DMA Channel pending flags. 00416 * @param __HANDLE__: DMA handle 00417 * @param __FLAG__: Get the specified flag. 00418 * This parameter can be any combination of the following values: 00419 * @arg DMA_FLAG_TCIFx: Transfer complete flag 00420 * @arg DMA_FLAG_HTIFx: Half transfer complete flag 00421 * @arg DMA_FLAG_TEIFx: Transfer error flag 00422 * @arg DMA_ISR_GIFx: Global interrupt flag 00423 * Where x can be 0_4, 1_5, 2_6 or 3_7 to select the DMA Channel flag. 00424 * @retval The state of FLAG (SET or RESET). 00425 */ 00426 #define __HAL_DMA_GET_FLAG(__HANDLE__, __FLAG__) (((uint32_t)((__HANDLE__)->Instance) > ((uint32_t)DMA1_Channel7))? \ 00427 (DMA2->ISR & (__FLAG__)) : (DMA1->ISR & (__FLAG__))) 00428 00429 /** 00430 * @brief Clear the DMA Channel pending flags. 00431 * @param __HANDLE__: DMA handle 00432 * @param __FLAG__: specifies the flag to clear. 00433 * This parameter can be any combination of the following values: 00434 * @arg DMA_FLAG_TCIFx: Transfer complete flag 00435 * @arg DMA_FLAG_HTIFx: Half transfer complete flag 00436 * @arg DMA_FLAG_TEIFx: Transfer error flag 00437 * @arg DMA_ISR_GIFx: Global interrupt flag 00438 * Where x can be 0_4, 1_5, 2_6 or 3_7 to select the DMA Channel flag. 00439 * @retval None 00440 */ 00441 #define __HAL_DMA_CLEAR_FLAG(__HANDLE__, __FLAG__) (((uint32_t)((__HANDLE__)->Instance) > ((uint32_t)DMA1_Channel7))? \ 00442 (DMA2->IFCR |= (__FLAG__)) : (DMA1->IFCR |= (__FLAG__))) 00443 00444 /** 00445 * @brief Enable the specified DMA Channel interrupts. 00446 * @param __HANDLE__: DMA handle 00447 * @param __INTERRUPT__: specifies the DMA interrupt sources to be enabled or disabled. 00448 * This parameter can be any combination of the following values: 00449 * @arg DMA_IT_TC: Transfer complete interrupt mask 00450 * @arg DMA_IT_HT: Half transfer complete interrupt mask 00451 * @arg DMA_IT_TE: Transfer error interrupt mask 00452 * @retval None 00453 */ 00454 #define __HAL_DMA_ENABLE_IT(__HANDLE__, __INTERRUPT__) ((__HANDLE__)->Instance->CCR |= (__INTERRUPT__)) 00455 00456 /** 00457 * @brief Disable the specified DMA Channel interrupts. 00458 * @param __HANDLE__: DMA handle 00459 * @param __INTERRUPT__: specifies the DMA interrupt sources to be enabled or disabled. 00460 * This parameter can be any combination of the following values: 00461 * @arg DMA_IT_TC: Transfer complete interrupt mask 00462 * @arg DMA_IT_HT: Half transfer complete interrupt mask 00463 * @arg DMA_IT_TE: Transfer error interrupt mask 00464 * @retval None 00465 */ 00466 #define __HAL_DMA_DISABLE_IT(__HANDLE__, __INTERRUPT__) ((__HANDLE__)->Instance->CCR &= ~(__INTERRUPT__)) 00467 00468 /** 00469 * @brief Check whether the specified DMA Channel interrupt is enabled or not. 00470 * @param __HANDLE__: DMA handle 00471 * @param __INTERRUPT__: specifies the DMA interrupt source to check. 00472 * This parameter can be one of the following values: 00473 * @arg DMA_IT_TC: Transfer complete interrupt mask 00474 * @arg DMA_IT_HT: Half transfer complete interrupt mask 00475 * @arg DMA_IT_TE: Transfer error interrupt mask 00476 * @retval The state of DMA_IT (SET or RESET). 00477 */ 00478 #define __HAL_DMA_GET_IT_SOURCE(__HANDLE__, __INTERRUPT__) (((__HANDLE__)->Instance->CCR & (__INTERRUPT__))) 00479 00480 /** 00481 * @} 00482 */ 00483 00484 /* Exported functions --------------------------------------------------------*/ 00485 00486 /** @addtogroup DMA_Exported_Functions 00487 * @{ 00488 */ 00489 00490 /** @addtogroup DMA_Exported_Functions_Group1 00491 * @{ 00492 */ 00493 /* Initialization and de-initialization functions *****************************/ 00494 HAL_StatusTypeDef HAL_DMA_Init(DMA_HandleTypeDef *hdma); 00495 HAL_StatusTypeDef HAL_DMA_DeInit (DMA_HandleTypeDef *hdma); 00496 /** 00497 * @} 00498 */ 00499 00500 /** @addtogroup DMA_Exported_Functions_Group2 00501 * @{ 00502 */ 00503 /* IO operation functions *****************************************************/ 00504 HAL_StatusTypeDef HAL_DMA_Start (DMA_HandleTypeDef *hdma, uint32_t SrcAddress, uint32_t DstAddress, uint32_t DataLength); 00505 HAL_StatusTypeDef HAL_DMA_Start_IT(DMA_HandleTypeDef *hdma, uint32_t SrcAddress, uint32_t DstAddress, uint32_t DataLength); 00506 HAL_StatusTypeDef HAL_DMA_Abort(DMA_HandleTypeDef *hdma); 00507 HAL_StatusTypeDef HAL_DMA_PollForTransfer(DMA_HandleTypeDef *hdma, uint32_t CompleteLevel, uint32_t Timeout); 00508 void HAL_DMA_IRQHandler(DMA_HandleTypeDef *hdma); 00509 /** 00510 * @} 00511 */ 00512 00513 /** @addtogroup DMA_Exported_Functions_Group3 00514 * @{ 00515 */ 00516 /* Peripheral State and Error functions ***************************************/ 00517 HAL_DMA_StateTypeDef HAL_DMA_GetState(DMA_HandleTypeDef *hdma); 00518 uint32_t HAL_DMA_GetError(DMA_HandleTypeDef *hdma); 00519 /** 00520 * @} 00521 */ 00522 00523 /** 00524 * @} 00525 */ 00526 00527 /* Private macros ------------------------------------------------------------*/ 00528 /** @defgroup DMA_Private_Macros DMA Private Macros 00529 * @{ 00530 */ 00531 00532 #define IS_DMA_DIRECTION(DIRECTION) (((DIRECTION) == DMA_PERIPH_TO_MEMORY ) || \ 00533 ((DIRECTION) == DMA_MEMORY_TO_PERIPH) || \ 00534 ((DIRECTION) == DMA_MEMORY_TO_MEMORY)) 00535 00536 #define IS_DMA_BUFFER_SIZE(SIZE) (((SIZE) >= 0x1) && ((SIZE) < 0x10000)) 00537 00538 #define IS_DMA_PERIPHERAL_INC_STATE(STATE) (((STATE) == DMA_PINC_ENABLE) || \ 00539 ((STATE) == DMA_PINC_DISABLE)) 00540 00541 #define IS_DMA_MEMORY_INC_STATE(STATE) (((STATE) == DMA_MINC_ENABLE) || \ 00542 ((STATE) == DMA_MINC_DISABLE)) 00543 00544 #define IS_DMA_ALL_REQUEST(REQUEST) (((REQUEST) == DMA_REQUEST_0) || \ 00545 ((REQUEST) == DMA_REQUEST_1) || \ 00546 ((REQUEST) == DMA_REQUEST_2) || \ 00547 ((REQUEST) == DMA_REQUEST_3) || \ 00548 ((REQUEST) == DMA_REQUEST_4) || \ 00549 ((REQUEST) == DMA_REQUEST_5) || \ 00550 ((REQUEST) == DMA_REQUEST_6) || \ 00551 ((REQUEST) == DMA_REQUEST_7)) 00552 00553 #define IS_DMA_PERIPHERAL_DATA_SIZE(SIZE) (((SIZE) == DMA_PDATAALIGN_BYTE) || \ 00554 ((SIZE) == DMA_PDATAALIGN_HALFWORD) || \ 00555 ((SIZE) == DMA_PDATAALIGN_WORD)) 00556 00557 #define IS_DMA_MEMORY_DATA_SIZE(SIZE) (((SIZE) == DMA_MDATAALIGN_BYTE) || \ 00558 ((SIZE) == DMA_MDATAALIGN_HALFWORD) || \ 00559 ((SIZE) == DMA_MDATAALIGN_WORD )) 00560 00561 #define IS_DMA_MODE(MODE) (((MODE) == DMA_NORMAL ) || \ 00562 ((MODE) == DMA_CIRCULAR)) 00563 00564 #define IS_DMA_PRIORITY(PRIORITY) (((PRIORITY) == DMA_PRIORITY_LOW ) || \ 00565 ((PRIORITY) == DMA_PRIORITY_MEDIUM) || \ 00566 ((PRIORITY) == DMA_PRIORITY_HIGH) || \ 00567 ((PRIORITY) == DMA_PRIORITY_VERY_HIGH)) 00568 00569 /** 00570 * @} 00571 */ 00572 00573 /* Private functions ---------------------------------------------------------*/ 00574 00575 /** 00576 * @} 00577 */ 00578 00579 /** 00580 * @} 00581 */ 00582 00583 #ifdef __cplusplus 00584 } 00585 #endif 00586 00587 #endif /* __STM32L4xx_HAL_DMA_H */ 00588 00589 /************************ (C) COPYRIGHT STMicroelectronics *****END OF FILE****/ 00590
Generated on Tue Jul 12 2022 11:35:09 by
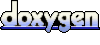