Hal Drivers for L4
Dependents: BSP OneHopeOnePrayer FINAL_AUDIO_RECORD AudioDemo
Fork of STM32L4xx_HAL_Driver by
stm32l4xx_hal_dfsdm.c
00001 /** 00002 ****************************************************************************** 00003 * @file stm32l4xx_hal_dfsdm.c 00004 * @author MCD Application Team 00005 * @version V1.1.0 00006 * @date 16-September-2015 00007 * @brief This file provides firmware functions to manage the following 00008 * functionalities of the Digital Filter for Sigma-Delta Modulators 00009 * (DFSDM) peripherals: 00010 * + Initialization and configuration of channels and filters 00011 * + Regular channels configuration 00012 * + Injected channels configuration 00013 * + Regular/Injected Channels DMA Configuration 00014 * + Interrupts and flags management 00015 * + Analog watchdog feature 00016 * + Short-circuit detector feature 00017 * + Extremes detector feature 00018 * + Clock absence detector feature 00019 * + Break generation on analog watchdog or short-circuit event 00020 * 00021 @verbatim 00022 ============================================================================== 00023 ##### How to use this driver ##### 00024 ============================================================================== 00025 [..] 00026 *** Channel initialization *** 00027 ============================== 00028 [..] 00029 (#) User has first to initialize channels (before filters initialization). 00030 (#) As prerequisite, fill in the HAL_DFSDM_ChannelMspInit() : 00031 (++) Enable DFSDM clock interface with __HAL_RCC_DFSDM_CLK_ENABLE(). 00032 (++) Enable the clocks for the DFSDM GPIOS with __HAL_RCC_GPIOx_CLK_ENABLE(). 00033 (++) Configure these DFSDM pins in alternate mode using HAL_GPIO_Init(). 00034 (++) If interrupt mode is used, enable and configure DFSDM1 global 00035 interrupt with HAL_NVIC_SetPriority() and HAL_NVIC_EnableIRQ(). 00036 (#) Configure the output clock, input, serial interface, analog watchdog, 00037 offset and data right bit shift parameters for this channel using the 00038 HAL_DFSDM_ChannelInit() function. 00039 00040 *** Channel clock absence detector *** 00041 ====================================== 00042 [..] 00043 (#) Start clock absence detector using HAL_DFSDM_ChannelCkabStart() or 00044 HAL_DFSDM_ChannelCkabStart_IT(). 00045 (#) In polling mode, use HAL_DFSDM_ChannelPollForCkab() to detect the clock 00046 absence. 00047 (#) In interrupt mode, HAL_DFSDM_ChannelCkabCallback() will be called if 00048 clock absence is detected. 00049 (#) Stop clock absence detector using HAL_DFSDM_ChannelCkabStop() or 00050 HAL_DFSDM_ChannelCkabStop_IT(). 00051 (#) Please note that the same mode (polling or interrupt) has to be used 00052 for all channels because the channels are sharing the same interrupt. 00053 (#) Please note also that in interrupt mode, if clock absence detector is 00054 stopped for one channel, interrupt will be disabled for all channels. 00055 00056 *** Channel short circuit detector *** 00057 ====================================== 00058 [..] 00059 (#) Start short circuit detector using HAL_DFSDM_ChannelScdStart() or 00060 or HAL_DFSDM_ChannelScdStart_IT(). 00061 (#) In polling mode, use HAL_DFSDM_ChannelPollForScd() to detect short 00062 circuit. 00063 (#) In interrupt mode, HAL_DFSDM_ChannelScdCallback() will be called if 00064 short circuit is detected. 00065 (#) Stop short circuit detector using HAL_DFSDM_ChannelScdStop() or 00066 or HAL_DFSDM_ChannelScdStop_IT(). 00067 (#) Please note that the same mode (polling or interrupt) has to be used 00068 for all channels because the channels are sharing the same interrupt. 00069 (#) Please note also that in interrupt mode, if short circuit detector is 00070 stopped for one channel, interrupt will be disabled for all channels. 00071 00072 *** Channel analog watchdog value *** 00073 ===================================== 00074 [..] 00075 (#) Get analog watchdog filter value of a channel using 00076 HAL_DFSDM_ChannelGetAwdValue(). 00077 00078 *** Channel offset value *** 00079 ===================================== 00080 [..] 00081 (#) Modify offset value of a channel using HAL_DFSDM_ChannelModifyOffset(). 00082 00083 *** Filter initialization *** 00084 ============================= 00085 [..] 00086 (#) After channel initialization, user has to init filters. 00087 (#) As prerequisite, fill in the HAL_DFSDM_FilterMspInit() : 00088 (++) If interrupt mode is used , enable and configure DFSDMx global 00089 interrupt with HAL_NVIC_SetPriority() and HAL_NVIC_EnableIRQ(). 00090 Please note that DFSDM0 global interrupt could be already 00091 enabled if interrupt is used for channel. 00092 (++) If DMA mode is used, configure DMA with HAL_DMA_Init() and link it 00093 with DFSDMx filter handle using __HAL_LINKDMA(). 00094 (#) Configure the regular conversion, injected conversion and filter 00095 parameters for this filter using the HAL_DFSDM_FilterInit() function. 00096 00097 *** Filter regular channel conversion *** 00098 ========================================= 00099 [..] 00100 (#) Select regular channel and enable/disable continuous mode using 00101 HAL_DFSDM_FilterConfigRegChannel(). 00102 (#) Start regular conversion using HAL_DFSDM_FilterRegularStart(), 00103 HAL_DFSDM_FilterRegularStart_IT(), HAL_DFSDM_FilterRegularStart_DMA() or 00104 HAL_DFSDM_FilterRegularMsbStart_DMA(). 00105 (#) In polling mode, use HAL_DFSDM_FilterPollForRegConversion() to detect 00106 the end of regular conversion. 00107 (#) In interrupt mode, HAL_DFSDM_FilterRegConvCpltCallback() will be called 00108 at the end of regular conversion. 00109 (#) Get value of regular conversion and corresponding channel using 00110 HAL_DFSDM_FilterGetRegularValue(). 00111 (#) In DMA mode, HAL_DFSDM_FilterRegConvHalfCpltCallback() and 00112 HAL_DFSDM_FilterRegConvCpltCallback() will be called respectively at the 00113 half transfer and at the transfer complete. Please note that 00114 HAL_DFSDM_FilterRegConvHalfCpltCallback() will be called only in DMA 00115 circular mode. 00116 (#) Stop regular conversion using HAL_DFSDM_FilterRegularStop(), 00117 HAL_DFSDM_FilterRegularStop_IT() or HAL_DFSDM_FilterRegularStop_DMA(). 00118 00119 *** Filter injected channels conversion *** 00120 =========================================== 00121 [..] 00122 (#) Select injected channels using HAL_DFSDM_FilterConfigInjChannel(). 00123 (#) Start injected conversion using HAL_DFSDM_FilterInjectedStart(), 00124 HAL_DFSDM_FilterInjectedStart_IT(), HAL_DFSDM_FilterInjectedStart_DMA() or 00125 HAL_DFSDM_FilterInjectedMsbStart_DMA(). 00126 (#) In polling mode, use HAL_DFSDM_FilterPollForInjConversion() to detect 00127 the end of injected conversion. 00128 (#) In interrupt mode, HAL_DFSDM_FilterInjConvCpltCallback() will be called 00129 at the end of injected conversion. 00130 (#) Get value of injected conversion and corresponding channel using 00131 HAL_DFSDM_FilterGetInjectedValue(). 00132 (#) In DMA mode, HAL_DFSDM_FilterInjConvHalfCpltCallback() and 00133 HAL_DFSDM_FilterInjConvCpltCallback() will be called respectively at the 00134 half transfer and at the transfer complete. Please note that 00135 HAL_DFSDM_FilterInjConvCpltCallback() will be called only in DMA 00136 circular mode. 00137 (#) Stop injected conversion using HAL_DFSDM_FilterInjectedStop(), 00138 HAL_DFSDM_FilterInjectedStop_IT() or HAL_DFSDM_FilterInjectedStop_DMA(). 00139 00140 *** Filter analog watchdog *** 00141 ============================== 00142 [..] 00143 (#) Start filter analog watchdog using HAL_DFSDM_FilterAwdStart_IT(). 00144 (#) HAL_DFSDM_FilterAwdCallback() will be called if analog watchdog occurs. 00145 (#) Stop filter analog watchdog using HAL_DFSDM_FilterAwdStop_IT(). 00146 00147 *** Filter extreme detector *** 00148 =============================== 00149 [..] 00150 (#) Start filter extreme detector using HAL_DFSDM_FilterExdStart(). 00151 (#) Get extreme detector maximum value using HAL_DFSDM_FilterGetExdMaxValue(). 00152 (#) Get extreme detector minimum value using HAL_DFSDM_FilterGetExdMinValue(). 00153 (#) Start filter extreme detector using HAL_DFSDM_FilterExdStop(). 00154 00155 *** Filter conversion time *** 00156 ============================== 00157 [..] 00158 (#) Get conversion time value using HAL_DFSDM_FilterGetConvTimeValue(). 00159 00160 @endverbatim 00161 ****************************************************************************** 00162 * @attention 00163 * 00164 * <h2><center>© COPYRIGHT(c) 2015 STMicroelectronics</center></h2> 00165 * 00166 * Redistribution and use in source and binary forms, with or without modification, 00167 * are permitted provided that the following conditions are met: 00168 * 1. Redistributions of source code must retain the above copyright notice, 00169 * this list of conditions and the following disclaimer. 00170 * 2. Redistributions in binary form must reproduce the above copyright notice, 00171 * this list of conditions and the following disclaimer in the documentation 00172 * and/or other materials provided with the distribution. 00173 * 3. Neither the name of STMicroelectronics nor the names of its contributors 00174 * may be used to endorse or promote products derived from this software 00175 * without specific prior written permission. 00176 * 00177 * THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" 00178 * AND ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE 00179 * IMPLIED WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE 00180 * DISCLAIMED. IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE 00181 * FOR ANY DIRECT, INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL 00182 * DAMAGES (INCLUDING, BUT NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR 00183 * SERVICES; LOSS OF USE, DATA, OR PROFITS; OR BUSINESS INTERRUPTION) HOWEVER 00184 * CAUSED AND ON ANY THEORY OF LIABILITY, WHETHER IN CONTRACT, STRICT LIABILITY, 00185 * OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) ARISING IN ANY WAY OUT OF THE USE 00186 * OF THIS SOFTWARE, EVEN IF ADVISED OF THE POSSIBILITY OF SUCH DAMAGE. 00187 * 00188 ****************************************************************************** 00189 */ 00190 00191 /* Includes ------------------------------------------------------------------*/ 00192 #include "stm32l4xx_hal.h" 00193 00194 /** @addtogroup STM32L4xx_HAL_Driver 00195 * @{ 00196 */ 00197 #ifdef HAL_DFSDM_MODULE_ENABLED 00198 /** @defgroup DFSDM DFSDM 00199 * @brief DFSDM HAL driver module 00200 * @{ 00201 */ 00202 00203 /* Private typedef -----------------------------------------------------------*/ 00204 /* Private define ------------------------------------------------------------*/ 00205 /** @defgroup DFSDM_Private_Define DFSDM Private Define 00206 * @{ 00207 */ 00208 #define DFSDM_CHCFGR1_CLK_DIV_OFFSET POSITION_VAL(DFSDM_CHCFGR1_CKOUTDIV) 00209 #define DFSDM_AWSCDR_BKSCD_OFFSET POSITION_VAL(DFSDM_AWSCDR_BKSCD) 00210 #define DFSDM_AWSCDR_FOSR_OFFSET POSITION_VAL(DFSDM_AWSCDR_AWFOSR) 00211 #define DFSDM_CHCFGR2_OFFSET_OFFSET POSITION_VAL(DFSDM_CHCFGR2_OFFSET) 00212 #define DFSDM_CHCFGR2_DTRBS_OFFSET POSITION_VAL(DFSDM_CHCFGR2_DTRBS) 00213 #define DFSDM_FCR_FOSR_OFFSET POSITION_VAL(DFSDM_FCR_FOSR) 00214 #define DFSDM_CR1_MSB_RCH_OFFSET 8 00215 #define DFSDM_CR2_EXCH_OFFSET POSITION_VAL(DFSDM_CR2_EXCH) 00216 #define DFSDM_CR2_AWDCH_OFFSET POSITION_VAL(DFSDM_CR2_AWDCH) 00217 #define DFSDM_ISR_CKABF_OFFSET POSITION_VAL(DFSDM_ISR_CKABF) 00218 #define DFSDM_ISR_SCDF_OFFSET POSITION_VAL(DFSDM_ISR_SCDF) 00219 #define DFSDM_ICR_CLRCKABF_OFFSET POSITION_VAL(DFSDM_ICR_CLRCKABF) 00220 #define DFSDM_ICR_CLRSCDF_OFFSET POSITION_VAL(DFSDM_ICR_CLRSCSDF) 00221 #define DFSDM_RDATAR_DATA_OFFSET POSITION_VAL(DFSDM_RDATAR_RDATA) 00222 #define DFSDM_JDATAR_DATA_OFFSET POSITION_VAL(DFSDM_JDATAR_JDATA) 00223 #define DFSDM_AWHTR_THRESHOLD_OFFSET POSITION_VAL(DFSDM_AWHTR_AWHT) 00224 #define DFSDM_AWLTR_THRESHOLD_OFFSET POSITION_VAL(DFSDM_AWLTR_AWLT) 00225 #define DFSDM_EXMAX_DATA_OFFSET POSITION_VAL(DFSDM_EXMAX_EXMAX) 00226 #define DFSDM_EXMIN_DATA_OFFSET POSITION_VAL(DFSDM_EXMIN_EXMIN) 00227 #define DFSDM_CNVTIMR_DATA_OFFSET POSITION_VAL(DFSDM_CNVTIMR_CNVCNT) 00228 #define DFSDM_AWSR_HIGH_OFFSET POSITION_VAL(DFSDM_AWSR_AWHTF) 00229 #define DFSDM_MSB_MASK 0xFFFF0000 00230 #define DFSDM_LSB_MASK 0x0000FFFF 00231 #define DFSDM_CKAB_TIMEOUT 5000 00232 #define DFSDM_CHANNEL_NUMBER 8 00233 /** 00234 * @} 00235 */ 00236 00237 /* Private macro -------------------------------------------------------------*/ 00238 /* Private variables ---------------------------------------------------------*/ 00239 /** @defgroup DFSDM_Private_Variables DFSDM Private Variables 00240 * @{ 00241 */ 00242 __IO uint32_t v_dfsdmChannelCounter = 0; 00243 DFSDM_Channel_HandleTypeDef* a_dfsdmChannelHandle[DFSDM_CHANNEL_NUMBER] = {(DFSDM_Channel_HandleTypeDef *) NULL}; 00244 /** 00245 * @} 00246 */ 00247 00248 /* Private function prototypes -----------------------------------------------*/ 00249 /** @defgroup DFSDM_Private_Functions DFSDM Private Functions 00250 * @{ 00251 */ 00252 static uint32_t DFSDM_GetInjChannelsNbr(uint32_t Channels); 00253 static uint32_t DFSDM_GetChannelFromInstance(DFSDM_Channel_TypeDef* Instance); 00254 static void DFSDM_RegConvStart(DFSDM_Filter_HandleTypeDef *hdfsdm_filter); 00255 static void DFSDM_RegConvStop(DFSDM_Filter_HandleTypeDef* hdfsdm_filter); 00256 static void DFSDM_InjConvStart(DFSDM_Filter_HandleTypeDef* hdfsdm_filter); 00257 static void DFSDM_InjConvStop(DFSDM_Filter_HandleTypeDef* hdfsdm_filter); 00258 static void DFSDM_DMARegularHalfConvCplt(DMA_HandleTypeDef *hdma); 00259 static void DFSDM_DMARegularConvCplt(DMA_HandleTypeDef *hdma); 00260 static void DFSDM_DMAInjectedHalfConvCplt(DMA_HandleTypeDef *hdma); 00261 static void DFSDM_DMAInjectedConvCplt(DMA_HandleTypeDef *hdma); 00262 static void DFSDM_DMAError(DMA_HandleTypeDef *hdma); 00263 /** 00264 * @} 00265 */ 00266 00267 /* Exported functions --------------------------------------------------------*/ 00268 /** @defgroup DFSDM_Exported_Functions DFSDM Exported Functions 00269 * @{ 00270 */ 00271 00272 /** @defgroup DFSDM_Exported_Functions_Group1_Channel Channel initialization and de-initialization functions 00273 * @brief Channel initialization and de-initialization functions 00274 * 00275 @verbatim 00276 ============================================================================== 00277 ##### Channel initialization and de-initialization functions ##### 00278 ============================================================================== 00279 [..] This section provides functions allowing to: 00280 (+) Initialize the DFSDM channel. 00281 (+) De-initialize the DFSDM channel. 00282 @endverbatim 00283 * @{ 00284 */ 00285 00286 /** 00287 * @brief Initialize the DFSDM channel according to the specified parameters 00288 * in the DFSDM_ChannelInitTypeDef structure and initialize the associated handle. 00289 * @param hdfsdm_channel : DFSDM channel handle. 00290 * @retval HAL status. 00291 */ 00292 HAL_StatusTypeDef HAL_DFSDM_ChannelInit(DFSDM_Channel_HandleTypeDef *hdfsdm_channel) 00293 { 00294 /* Check parameters */ 00295 assert_param(IS_DFSDM_CHANNEL_ALL_INSTANCE(hdfsdm_channel->Instance)); 00296 assert_param(IS_FUNCTIONAL_STATE(hdfsdm_channel->Init.OutputClock.Activation)); 00297 assert_param(IS_DFSDM_CHANNEL_INPUT(hdfsdm_channel->Init.Input.Multiplexer)); 00298 assert_param(IS_DFSDM_CHANNEL_DATA_PACKING(hdfsdm_channel->Init.Input.DataPacking)); 00299 assert_param(IS_DFSDM_CHANNEL_INPUT_PINS(hdfsdm_channel->Init.Input.Pins)); 00300 assert_param(IS_DFSDM_CHANNEL_SERIAL_INTERFACE_TYPE(hdfsdm_channel->Init.SerialInterface.Type)); 00301 assert_param(IS_DFSDM_CHANNEL_SPI_CLOCK(hdfsdm_channel->Init.SerialInterface.SpiClock)); 00302 assert_param(IS_DFSDM_CHANNEL_FILTER_ORDER(hdfsdm_channel->Init.Awd.FilterOrder)); 00303 assert_param(IS_DFSDM_CHANNEL_FILTER_OVS_RATIO(hdfsdm_channel->Init.Awd.Oversampling)); 00304 assert_param(IS_DFSDM_CHANNEL_OFFSET(hdfsdm_channel->Init.Offset)); 00305 assert_param(IS_DFSDM_CHANNEL_RIGHT_BIT_SHIFT(hdfsdm_channel->Init.RightBitShift)); 00306 00307 /* Check DFSDM Channel handle */ 00308 if(hdfsdm_channel == NULL) 00309 { 00310 return HAL_ERROR; 00311 } 00312 /* Check that channel has not been already initialized */ 00313 if(a_dfsdmChannelHandle[DFSDM_GetChannelFromInstance(hdfsdm_channel->Instance)] != NULL) 00314 { 00315 return HAL_ERROR; 00316 } 00317 00318 /* Call MSP init function */ 00319 HAL_DFSDM_ChannelMspInit(hdfsdm_channel); 00320 00321 /* Update the channel counter */ 00322 v_dfsdmChannelCounter++; 00323 00324 /* Configure output serial clock and enable global DFSDM interface only for first channel */ 00325 if(v_dfsdmChannelCounter == 1) 00326 { 00327 assert_param(IS_DFSDM_CHANNEL_OUTPUT_CLOCK(hdfsdm_channel->Init.OutputClock.Selection)); 00328 /* Set the output serial clock source */ 00329 DFSDM_Channel0->CHCFGR1 &= ~(DFSDM_CHCFGR1_CKOUTSRC); 00330 DFSDM_Channel0->CHCFGR1 |= hdfsdm_channel->Init.OutputClock.Selection; 00331 00332 /* Reset clock divider */ 00333 DFSDM_Channel0->CHCFGR1 &= ~(DFSDM_CHCFGR1_CKOUTDIV); 00334 if(hdfsdm_channel->Init.OutputClock.Activation == ENABLE) 00335 { 00336 assert_param(IS_DFSDM_CHANNEL_OUTPUT_CLOCK_DIVIDER(hdfsdm_channel->Init.OutputClock.Divider)); 00337 /* Set the output clock divider */ 00338 DFSDM_Channel0->CHCFGR1 |= (uint32_t) ((hdfsdm_channel->Init.OutputClock.Divider - 1) << 00339 DFSDM_CHCFGR1_CLK_DIV_OFFSET); 00340 } 00341 00342 /* enable the DFSDM global interface */ 00343 DFSDM_Channel0->CHCFGR1 |= DFSDM_CHCFGR1_DFSDMEN; 00344 } 00345 00346 /* Set channel input parameters */ 00347 hdfsdm_channel->Instance->CHCFGR1 &= ~(DFSDM_CHCFGR1_DATPACK | DFSDM_CHCFGR1_DATMPX | 00348 DFSDM_CHCFGR1_CHINSEL); 00349 hdfsdm_channel->Instance->CHCFGR1 |= (hdfsdm_channel->Init.Input.Multiplexer | 00350 hdfsdm_channel->Init.Input.DataPacking | 00351 hdfsdm_channel->Init.Input.Pins); 00352 00353 /* Set serial interface parameters */ 00354 hdfsdm_channel->Instance->CHCFGR1 &= ~(DFSDM_CHCFGR1_SITP | DFSDM_CHCFGR1_SPICKSEL); 00355 hdfsdm_channel->Instance->CHCFGR1 |= (hdfsdm_channel->Init.SerialInterface.Type | 00356 hdfsdm_channel->Init.SerialInterface.SpiClock); 00357 00358 /* Set analog watchdog parameters */ 00359 hdfsdm_channel->Instance->AWSCDR &= ~(DFSDM_AWSCDR_AWFORD | DFSDM_AWSCDR_AWFOSR); 00360 hdfsdm_channel->Instance->AWSCDR |= (hdfsdm_channel->Init.Awd.FilterOrder | 00361 ((hdfsdm_channel->Init.Awd.Oversampling - 1) << DFSDM_AWSCDR_FOSR_OFFSET)); 00362 00363 /* Set channel offset and right bit shift */ 00364 hdfsdm_channel->Instance->CHCFGR2 &= ~(DFSDM_CHCFGR2_OFFSET | DFSDM_CHCFGR2_DTRBS); 00365 hdfsdm_channel->Instance->CHCFGR2 |= ((hdfsdm_channel->Init.Offset << DFSDM_CHCFGR2_OFFSET_OFFSET) | 00366 (hdfsdm_channel->Init.RightBitShift << DFSDM_CHCFGR2_DTRBS_OFFSET)); 00367 00368 /* Enable DFSDM channel */ 00369 hdfsdm_channel->Instance->CHCFGR1 |= DFSDM_CHCFGR1_CHEN; 00370 00371 /* Set DFSDM Channel to ready state */ 00372 hdfsdm_channel->State = HAL_DFSDM_CHANNEL_STATE_READY; 00373 00374 /* Store channel handle in DFSDM channel handle table */ 00375 a_dfsdmChannelHandle[DFSDM_GetChannelFromInstance(hdfsdm_channel->Instance)] = hdfsdm_channel; 00376 00377 return HAL_OK; 00378 } 00379 00380 /** 00381 * @brief De-initialize the DFSDM channel. 00382 * @param hdfsdm_channel : DFSDM channel handle. 00383 * @retval HAL status. 00384 */ 00385 HAL_StatusTypeDef HAL_DFSDM_ChannelDeInit(DFSDM_Channel_HandleTypeDef *hdfsdm_channel) 00386 { 00387 /* Check parameters */ 00388 assert_param(IS_DFSDM_CHANNEL_ALL_INSTANCE(hdfsdm_channel->Instance)); 00389 00390 /* Check DFSDM Channel handle */ 00391 if(hdfsdm_channel == NULL) 00392 { 00393 return HAL_ERROR; 00394 } 00395 /* Check that channel has not been already deinitialized */ 00396 if(a_dfsdmChannelHandle[DFSDM_GetChannelFromInstance(hdfsdm_channel->Instance)] == NULL) 00397 { 00398 return HAL_ERROR; 00399 } 00400 00401 /* Disable the DFSDM channel */ 00402 hdfsdm_channel->Instance->CHCFGR1 &= ~(DFSDM_CHCFGR1_CHEN); 00403 00404 /* Update the channel counter */ 00405 v_dfsdmChannelCounter--; 00406 00407 /* Disable global DFSDM at deinit of last channel */ 00408 if(v_dfsdmChannelCounter == 0) 00409 { 00410 DFSDM_Channel0->CHCFGR1 &= ~(DFSDM_CHCFGR1_DFSDMEN); 00411 } 00412 00413 /* Call MSP deinit function */ 00414 HAL_DFSDM_ChannelMspDeInit(hdfsdm_channel); 00415 00416 /* Set DFSDM Channel in reset state */ 00417 hdfsdm_channel->State = HAL_DFSDM_CHANNEL_STATE_RESET; 00418 00419 /* Reset channel handle in DFSDM channel handle table */ 00420 a_dfsdmChannelHandle[DFSDM_GetChannelFromInstance(hdfsdm_channel->Instance)] = (DFSDM_Channel_HandleTypeDef *) NULL; 00421 00422 return HAL_OK; 00423 } 00424 00425 /** 00426 * @brief Initialize the DFSDM channel MSP. 00427 * @param hdfsdm_channel : DFSDM channel handle. 00428 * @retval None 00429 */ 00430 __weak void HAL_DFSDM_ChannelMspInit(DFSDM_Channel_HandleTypeDef *hdfsdm_channel) 00431 { 00432 /* NOTE : This function should not be modified, when the function is needed, 00433 the HAL_DFSDM_ChannelMspInit could be implemented in the user file. 00434 */ 00435 } 00436 00437 /** 00438 * @brief De-initialize the DFSDM channel MSP. 00439 * @param hdfsdm_channel : DFSDM channel handle. 00440 * @retval None 00441 */ 00442 __weak void HAL_DFSDM_ChannelMspDeInit(DFSDM_Channel_HandleTypeDef *hdfsdm_channel) 00443 { 00444 /* NOTE : This function should not be modified, when the function is needed, 00445 the HAL_DFSDM_ChannelMspDeInit could be implemented in the user file. 00446 */ 00447 } 00448 00449 /** 00450 * @} 00451 */ 00452 00453 /** @defgroup DFSDM_Exported_Functions_Group2_Channel Channel operation functions 00454 * @brief Channel operation functions 00455 * 00456 @verbatim 00457 ============================================================================== 00458 ##### Channel operation functions ##### 00459 ============================================================================== 00460 [..] This section provides functions allowing to: 00461 (+) Manage clock absence detector feature. 00462 (+) Manage short circuit detector feature. 00463 (+) Get analog watchdog value. 00464 (+) Modify offset value. 00465 @endverbatim 00466 * @{ 00467 */ 00468 00469 /** 00470 * @brief This function allows to start clock absence detection in polling mode. 00471 * @note Same mode has to be used for all channels. 00472 * @note If clock is not available on this channel during 5 seconds, 00473 * clock absence detection will not be activated and function 00474 * will return HAL_TIMEOUT error. 00475 * @param hdfsdm_channel : DFSDM channel handle. 00476 * @retval HAL status 00477 */ 00478 HAL_StatusTypeDef HAL_DFSDM_ChannelCkabStart(DFSDM_Channel_HandleTypeDef *hdfsdm_channel) 00479 { 00480 HAL_StatusTypeDef status = HAL_OK; 00481 uint32_t channel; 00482 uint32_t tickstart; 00483 00484 /* Check parameters */ 00485 assert_param(IS_DFSDM_CHANNEL_ALL_INSTANCE(hdfsdm_channel->Instance)); 00486 00487 /* Check DFSDM channel state */ 00488 if(hdfsdm_channel->State != HAL_DFSDM_CHANNEL_STATE_READY) 00489 { 00490 /* Return error status */ 00491 status = HAL_ERROR; 00492 } 00493 else 00494 { 00495 /* Get channel number from channel instance */ 00496 channel = DFSDM_GetChannelFromInstance(hdfsdm_channel->Instance); 00497 00498 /* Get timeout */ 00499 tickstart = HAL_GetTick(); 00500 00501 /* Clear clock absence flag */ 00502 while((((DFSDM_Filter0->ISR & DFSDM_ISR_CKABF) >> (DFSDM_ISR_CKABF_OFFSET + channel)) & 1) != 0) 00503 { 00504 DFSDM_Filter0->ICR = (1 << (DFSDM_ICR_CLRCKABF_OFFSET + channel)); 00505 00506 /* Check the Timeout */ 00507 if((HAL_GetTick()-tickstart) > DFSDM_CKAB_TIMEOUT) 00508 { 00509 /* Set timeout status */ 00510 status = HAL_TIMEOUT; 00511 break; 00512 } 00513 } 00514 00515 if(status == HAL_OK) 00516 { 00517 /* Start clock absence detection */ 00518 hdfsdm_channel->Instance->CHCFGR1 |= DFSDM_CHCFGR1_CKABEN; 00519 } 00520 } 00521 /* Return function status */ 00522 return status; 00523 } 00524 00525 /** 00526 * @brief This function allows to poll for the clock absence detection. 00527 * @param hdfsdm_channel : DFSDM channel handle. 00528 * @param Timeout : Timeout value in milliseconds. 00529 * @retval HAL status 00530 */ 00531 HAL_StatusTypeDef HAL_DFSDM_ChannelPollForCkab(DFSDM_Channel_HandleTypeDef *hdfsdm_channel, 00532 uint32_t Timeout) 00533 { 00534 uint32_t tickstart; 00535 uint32_t channel; 00536 00537 /* Check parameters */ 00538 assert_param(IS_DFSDM_CHANNEL_ALL_INSTANCE(hdfsdm_channel->Instance)); 00539 00540 /* Check DFSDM channel state */ 00541 if(hdfsdm_channel->State != HAL_DFSDM_CHANNEL_STATE_READY) 00542 { 00543 /* Return error status */ 00544 return HAL_ERROR; 00545 } 00546 else 00547 { 00548 /* Get channel number from channel instance */ 00549 channel = DFSDM_GetChannelFromInstance(hdfsdm_channel->Instance); 00550 00551 /* Get timeout */ 00552 tickstart = HAL_GetTick(); 00553 00554 /* Wait clock absence detection */ 00555 while((((DFSDM_Filter0->ISR & DFSDM_ISR_CKABF) >> (DFSDM_ISR_CKABF_OFFSET + channel)) & 1) == 0) 00556 { 00557 /* Check the Timeout */ 00558 if(Timeout != HAL_MAX_DELAY) 00559 { 00560 if((Timeout == 0) || ((HAL_GetTick()-tickstart) > Timeout)) 00561 { 00562 /* Return timeout status */ 00563 return HAL_TIMEOUT; 00564 } 00565 } 00566 } 00567 00568 /* Clear clock absence detection flag */ 00569 DFSDM_Filter0->ICR = (1 << (DFSDM_ICR_CLRCKABF_OFFSET + channel)); 00570 00571 /* Return function status */ 00572 return HAL_OK; 00573 } 00574 } 00575 00576 /** 00577 * @brief This function allows to stop clock absence detection in polling mode. 00578 * @param hdfsdm_channel : DFSDM channel handle. 00579 * @retval HAL status 00580 */ 00581 HAL_StatusTypeDef HAL_DFSDM_ChannelCkabStop(DFSDM_Channel_HandleTypeDef *hdfsdm_channel) 00582 { 00583 HAL_StatusTypeDef status = HAL_OK; 00584 uint32_t channel; 00585 00586 /* Check parameters */ 00587 assert_param(IS_DFSDM_CHANNEL_ALL_INSTANCE(hdfsdm_channel->Instance)); 00588 00589 /* Check DFSDM channel state */ 00590 if(hdfsdm_channel->State != HAL_DFSDM_CHANNEL_STATE_READY) 00591 { 00592 /* Return error status */ 00593 status = HAL_ERROR; 00594 } 00595 else 00596 { 00597 /* Stop clock absence detection */ 00598 hdfsdm_channel->Instance->CHCFGR1 &= ~(DFSDM_CHCFGR1_CKABEN); 00599 00600 /* Clear clock absence flag */ 00601 channel = DFSDM_GetChannelFromInstance(hdfsdm_channel->Instance); 00602 DFSDM_Filter0->ICR = (1 << (DFSDM_ICR_CLRCKABF_OFFSET + channel)); 00603 } 00604 /* Return function status */ 00605 return status; 00606 } 00607 00608 /** 00609 * @brief This function allows to start clock absence detection in interrupt mode. 00610 * @note Same mode has to be used for all channels. 00611 * @note If clock is not available on this channel during 5 seconds, 00612 * clock absence detection will not be activated and function 00613 * will return HAL_TIMEOUT error. 00614 * @param hdfsdm_channel : DFSDM channel handle. 00615 * @retval HAL status 00616 */ 00617 HAL_StatusTypeDef HAL_DFSDM_ChannelCkabStart_IT(DFSDM_Channel_HandleTypeDef *hdfsdm_channel) 00618 { 00619 HAL_StatusTypeDef status = HAL_OK; 00620 uint32_t channel; 00621 uint32_t tickstart; 00622 00623 /* Check parameters */ 00624 assert_param(IS_DFSDM_CHANNEL_ALL_INSTANCE(hdfsdm_channel->Instance)); 00625 00626 /* Check DFSDM channel state */ 00627 if(hdfsdm_channel->State != HAL_DFSDM_CHANNEL_STATE_READY) 00628 { 00629 /* Return error status */ 00630 status = HAL_ERROR; 00631 } 00632 else 00633 { 00634 /* Get channel number from channel instance */ 00635 channel = DFSDM_GetChannelFromInstance(hdfsdm_channel->Instance); 00636 00637 /* Get timeout */ 00638 tickstart = HAL_GetTick(); 00639 00640 /* Clear clock absence flag */ 00641 while((((DFSDM_Filter0->ISR & DFSDM_ISR_CKABF) >> (DFSDM_ISR_CKABF_OFFSET + channel)) & 1) != 0) 00642 { 00643 DFSDM_Filter0->ICR = (1 << (DFSDM_ICR_CLRCKABF_OFFSET + channel)); 00644 00645 /* Check the Timeout */ 00646 if((HAL_GetTick()-tickstart) > DFSDM_CKAB_TIMEOUT) 00647 { 00648 /* Set timeout status */ 00649 status = HAL_TIMEOUT; 00650 break; 00651 } 00652 } 00653 00654 if(status == HAL_OK) 00655 { 00656 /* Activate clock absence detection interrupt */ 00657 DFSDM_Filter0->CR2 |= DFSDM_CR2_CKABIE; 00658 00659 /* Start clock absence detection */ 00660 hdfsdm_channel->Instance->CHCFGR1 |= DFSDM_CHCFGR1_CKABEN; 00661 } 00662 } 00663 /* Return function status */ 00664 return status; 00665 } 00666 00667 /** 00668 * @brief Clock absence detection callback. 00669 * @param hdfsdm_channel : DFSDM channel handle. 00670 * @retval None 00671 */ 00672 __weak void HAL_DFSDM_ChannelCkabCallback(DFSDM_Channel_HandleTypeDef *hdfsdm_channel) 00673 { 00674 /* NOTE : This function should not be modified, when the callback is needed, 00675 the HAL_DFSDM_ChannelCkabCallback could be implemented in the user file 00676 */ 00677 } 00678 00679 /** 00680 * @brief This function allows to stop clock absence detection in interrupt mode. 00681 * @note Interrupt will be disabled for all channels 00682 * @param hdfsdm_channel : DFSDM channel handle. 00683 * @retval HAL status 00684 */ 00685 HAL_StatusTypeDef HAL_DFSDM_ChannelCkabStop_IT(DFSDM_Channel_HandleTypeDef *hdfsdm_channel) 00686 { 00687 HAL_StatusTypeDef status = HAL_OK; 00688 uint32_t channel; 00689 00690 /* Check parameters */ 00691 assert_param(IS_DFSDM_CHANNEL_ALL_INSTANCE(hdfsdm_channel->Instance)); 00692 00693 /* Check DFSDM channel state */ 00694 if(hdfsdm_channel->State != HAL_DFSDM_CHANNEL_STATE_READY) 00695 { 00696 /* Return error status */ 00697 status = HAL_ERROR; 00698 } 00699 else 00700 { 00701 /* Stop clock absence detection */ 00702 hdfsdm_channel->Instance->CHCFGR1 &= ~(DFSDM_CHCFGR1_CKABEN); 00703 00704 /* Clear clock absence flag */ 00705 channel = DFSDM_GetChannelFromInstance(hdfsdm_channel->Instance); 00706 DFSDM_Filter0->ICR = (1 << (DFSDM_ICR_CLRCKABF_OFFSET + channel)); 00707 00708 /* Disable clock absence detection interrupt */ 00709 DFSDM_Filter0->CR2 &= ~(DFSDM_CR2_CKABIE); 00710 } 00711 /* Return function status */ 00712 return status; 00713 } 00714 00715 /** 00716 * @brief This function allows to start short circuit detection in polling mode. 00717 * @note Same mode has to be used for all channels 00718 * @param hdfsdm_channel : DFSDM channel handle. 00719 * @param Threshold : Short circuit detector threshold. 00720 * This parameter must be a number between Min_Data = 0 and Max_Data = 255. 00721 * @param BreakSignal : Break signals assigned to short circuit event. 00722 * This parameter can be a values combination of @ref DFSDM_BreakSignals. 00723 * @retval HAL status 00724 */ 00725 HAL_StatusTypeDef HAL_DFSDM_ChannelScdStart(DFSDM_Channel_HandleTypeDef *hdfsdm_channel, 00726 uint32_t Threshold, 00727 uint32_t BreakSignal) 00728 { 00729 HAL_StatusTypeDef status = HAL_OK; 00730 00731 /* Check parameters */ 00732 assert_param(IS_DFSDM_CHANNEL_ALL_INSTANCE(hdfsdm_channel->Instance)); 00733 assert_param(IS_DFSDM_CHANNEL_SCD_THRESHOLD(Threshold)); 00734 assert_param(IS_DFSDM_BREAK_SIGNALS(BreakSignal)); 00735 00736 /* Check DFSDM channel state */ 00737 if(hdfsdm_channel->State != HAL_DFSDM_CHANNEL_STATE_READY) 00738 { 00739 /* Return error status */ 00740 status = HAL_ERROR; 00741 } 00742 else 00743 { 00744 /* Configure threshold and break signals */ 00745 hdfsdm_channel->Instance->AWSCDR &= ~(DFSDM_AWSCDR_BKSCD | DFSDM_AWSCDR_SCDT); 00746 hdfsdm_channel->Instance->AWSCDR |= ((BreakSignal << DFSDM_AWSCDR_BKSCD_OFFSET) | \ 00747 Threshold); 00748 00749 /* Start short circuit detection */ 00750 hdfsdm_channel->Instance->CHCFGR1 |= DFSDM_CHCFGR1_SCDEN; 00751 } 00752 /* Return function status */ 00753 return status; 00754 } 00755 00756 /** 00757 * @brief This function allows to poll for the short circuit detection. 00758 * @param hdfsdm_channel : DFSDM channel handle. 00759 * @param Timeout : Timeout value in milliseconds. 00760 * @retval HAL status 00761 */ 00762 HAL_StatusTypeDef HAL_DFSDM_ChannelPollForScd(DFSDM_Channel_HandleTypeDef *hdfsdm_channel, 00763 uint32_t Timeout) 00764 { 00765 uint32_t tickstart; 00766 uint32_t channel; 00767 00768 /* Check parameters */ 00769 assert_param(IS_DFSDM_CHANNEL_ALL_INSTANCE(hdfsdm_channel->Instance)); 00770 00771 /* Check DFSDM channel state */ 00772 if(hdfsdm_channel->State != HAL_DFSDM_CHANNEL_STATE_READY) 00773 { 00774 /* Return error status */ 00775 return HAL_ERROR; 00776 } 00777 else 00778 { 00779 /* Get channel number from channel instance */ 00780 channel = DFSDM_GetChannelFromInstance(hdfsdm_channel->Instance); 00781 00782 /* Get timeout */ 00783 tickstart = HAL_GetTick(); 00784 00785 /* Wait short circuit detection */ 00786 while(((DFSDM_Filter0->ISR & DFSDM_ISR_SCDF) >> (DFSDM_ISR_SCDF_OFFSET + channel)) == 0) 00787 { 00788 /* Check the Timeout */ 00789 if(Timeout != HAL_MAX_DELAY) 00790 { 00791 if((Timeout == 0) || ((HAL_GetTick()-tickstart) > Timeout)) 00792 { 00793 /* Return timeout status */ 00794 return HAL_TIMEOUT; 00795 } 00796 } 00797 } 00798 00799 /* Clear short circuit detection flag */ 00800 DFSDM_Filter0->ICR = (1 << (DFSDM_ICR_CLRSCDF_OFFSET + channel)); 00801 00802 /* Return function status */ 00803 return HAL_OK; 00804 } 00805 } 00806 00807 /** 00808 * @brief This function allows to stop short circuit detection in polling mode. 00809 * @param hdfsdm_channel : DFSDM channel handle. 00810 * @retval HAL status 00811 */ 00812 HAL_StatusTypeDef HAL_DFSDM_ChannelScdStop(DFSDM_Channel_HandleTypeDef *hdfsdm_channel) 00813 { 00814 HAL_StatusTypeDef status = HAL_OK; 00815 uint32_t channel; 00816 00817 /* Check parameters */ 00818 assert_param(IS_DFSDM_CHANNEL_ALL_INSTANCE(hdfsdm_channel->Instance)); 00819 00820 /* Check DFSDM channel state */ 00821 if(hdfsdm_channel->State != HAL_DFSDM_CHANNEL_STATE_READY) 00822 { 00823 /* Return error status */ 00824 status = HAL_ERROR; 00825 } 00826 else 00827 { 00828 /* Stop short circuit detection */ 00829 hdfsdm_channel->Instance->CHCFGR1 &= ~(DFSDM_CHCFGR1_SCDEN); 00830 00831 /* Clear short circuit detection flag */ 00832 channel = DFSDM_GetChannelFromInstance(hdfsdm_channel->Instance); 00833 DFSDM_Filter0->ICR = (1 << (DFSDM_ICR_CLRSCDF_OFFSET + channel)); 00834 } 00835 /* Return function status */ 00836 return status; 00837 } 00838 00839 /** 00840 * @brief This function allows to start short circuit detection in interrupt mode. 00841 * @note Same mode has to be used for all channels 00842 * @param hdfsdm_channel : DFSDM channel handle. 00843 * @param Threshold : Short circuit detector threshold. 00844 * This parameter must be a number between Min_Data = 0 and Max_Data = 255. 00845 * @param BreakSignal : Break signals assigned to short circuit event. 00846 * This parameter can be a values combination of @ref DFSDM_BreakSignals. 00847 * @retval HAL status 00848 */ 00849 HAL_StatusTypeDef HAL_DFSDM_ChannelScdStart_IT(DFSDM_Channel_HandleTypeDef *hdfsdm_channel, 00850 uint32_t Threshold, 00851 uint32_t BreakSignal) 00852 { 00853 HAL_StatusTypeDef status = HAL_OK; 00854 00855 /* Check parameters */ 00856 assert_param(IS_DFSDM_CHANNEL_ALL_INSTANCE(hdfsdm_channel->Instance)); 00857 assert_param(IS_DFSDM_CHANNEL_SCD_THRESHOLD(Threshold)); 00858 assert_param(IS_DFSDM_BREAK_SIGNALS(BreakSignal)); 00859 00860 /* Check DFSDM channel state */ 00861 if(hdfsdm_channel->State != HAL_DFSDM_CHANNEL_STATE_READY) 00862 { 00863 /* Return error status */ 00864 status = HAL_ERROR; 00865 } 00866 else 00867 { 00868 /* Activate short circuit detection interrupt */ 00869 DFSDM_Filter0->CR2 |= DFSDM_CR2_SCDIE; 00870 00871 /* Configure threshold and break signals */ 00872 hdfsdm_channel->Instance->AWSCDR &= ~(DFSDM_AWSCDR_BKSCD | DFSDM_AWSCDR_SCDT); 00873 hdfsdm_channel->Instance->AWSCDR |= ((BreakSignal << DFSDM_AWSCDR_BKSCD_OFFSET) | \ 00874 Threshold); 00875 00876 /* Start short circuit detection */ 00877 hdfsdm_channel->Instance->CHCFGR1 |= DFSDM_CHCFGR1_SCDEN; 00878 } 00879 /* Return function status */ 00880 return status; 00881 } 00882 00883 /** 00884 * @brief Short circuit detection callback. 00885 * @param hdfsdm_channel : DFSDM channel handle. 00886 * @retval None 00887 */ 00888 __weak void HAL_DFSDM_ChannelScdCallback(DFSDM_Channel_HandleTypeDef *hdfsdm_channel) 00889 { 00890 /* NOTE : This function should not be modified, when the callback is needed, 00891 the HAL_DFSDM_ChannelScdCallback could be implemented in the user file 00892 */ 00893 } 00894 00895 /** 00896 * @brief This function allows to stop short circuit detection in interrupt mode. 00897 * @note Interrupt will be disabled for all channels 00898 * @param hdfsdm_channel : DFSDM channel handle. 00899 * @retval HAL status 00900 */ 00901 HAL_StatusTypeDef HAL_DFSDM_ChannelScdStop_IT(DFSDM_Channel_HandleTypeDef *hdfsdm_channel) 00902 { 00903 HAL_StatusTypeDef status = HAL_OK; 00904 uint32_t channel; 00905 00906 /* Check parameters */ 00907 assert_param(IS_DFSDM_CHANNEL_ALL_INSTANCE(hdfsdm_channel->Instance)); 00908 00909 /* Check DFSDM channel state */ 00910 if(hdfsdm_channel->State != HAL_DFSDM_CHANNEL_STATE_READY) 00911 { 00912 /* Return error status */ 00913 status = HAL_ERROR; 00914 } 00915 else 00916 { 00917 /* Stop short circuit detection */ 00918 hdfsdm_channel->Instance->CHCFGR1 &= ~(DFSDM_CHCFGR1_SCDEN); 00919 00920 /* Clear short circuit detection flag */ 00921 channel = DFSDM_GetChannelFromInstance(hdfsdm_channel->Instance); 00922 DFSDM_Filter0->ICR = (1 << (DFSDM_ICR_CLRSCDF_OFFSET + channel)); 00923 00924 /* Disable short circuit detection interrupt */ 00925 DFSDM_Filter0->CR2 &= ~(DFSDM_CR2_SCDIE); 00926 } 00927 /* Return function status */ 00928 return status; 00929 } 00930 00931 /** 00932 * @brief This function allows to get channel analog watchdog value. 00933 * @param hdfsdm_channel : DFSDM channel handle. 00934 * @retval Channel analog watchdog value. 00935 */ 00936 int16_t HAL_DFSDM_ChannelGetAwdValue(DFSDM_Channel_HandleTypeDef *hdfsdm_channel) 00937 { 00938 return (int16_t) hdfsdm_channel->Instance->CHWDATAR; 00939 } 00940 00941 /** 00942 * @brief This function allows to modify channel offset value. 00943 * @param hdfsdm_channel : DFSDM channel handle. 00944 * @param Offset : DFSDM channel offset. 00945 * This parameter must be a number between Min_Data = -8388608 and Max_Data = 8388607. 00946 * @retval HAL status. 00947 */ 00948 HAL_StatusTypeDef HAL_DFSDM_ChannelModifyOffset(DFSDM_Channel_HandleTypeDef *hdfsdm_channel, 00949 int32_t Offset) 00950 { 00951 HAL_StatusTypeDef status = HAL_OK; 00952 00953 /* Check parameters */ 00954 assert_param(IS_DFSDM_CHANNEL_ALL_INSTANCE(hdfsdm_channel->Instance)); 00955 assert_param(IS_DFSDM_CHANNEL_OFFSET(Offset)); 00956 00957 /* Check DFSDM channel state */ 00958 if(hdfsdm_channel->State != HAL_DFSDM_CHANNEL_STATE_READY) 00959 { 00960 /* Return error status */ 00961 status = HAL_ERROR; 00962 } 00963 else 00964 { 00965 /* Modify channel offset */ 00966 hdfsdm_channel->Instance->CHCFGR2 &= ~(DFSDM_CHCFGR2_OFFSET); 00967 hdfsdm_channel->Instance->CHCFGR2 |= (Offset << DFSDM_CHCFGR2_OFFSET_OFFSET); 00968 } 00969 /* Return function status */ 00970 return status; 00971 } 00972 00973 /** 00974 * @} 00975 */ 00976 00977 /** @defgroup DFSDM_Exported_Functions_Group3_Channel Channel state function 00978 * @brief Channel state function 00979 * 00980 @verbatim 00981 ============================================================================== 00982 ##### Channel state function ##### 00983 ============================================================================== 00984 [..] This section provides function allowing to: 00985 (+) Get channel handle state. 00986 @endverbatim 00987 * @{ 00988 */ 00989 00990 /** 00991 * @brief This function allows to get the current DFSDM channel handle state. 00992 * @param hdfsdm_channel : DFSDM channel handle. 00993 * @retval DFSDM channel state. 00994 */ 00995 HAL_DFSDM_Channel_StateTypeDef HAL_DFSDM_ChannelGetState(DFSDM_Channel_HandleTypeDef *hdfsdm_channel) 00996 { 00997 /* Return DFSDM channel handle state */ 00998 return hdfsdm_channel->State; 00999 } 01000 01001 /** 01002 * @} 01003 */ 01004 01005 /** @defgroup DFSDM_Exported_Functions_Group1_Filter Filter initialization and de-initialization functions 01006 * @brief Filter initialization and de-initialization functions 01007 * 01008 @verbatim 01009 ============================================================================== 01010 ##### Filter initialization and de-initialization functions ##### 01011 ============================================================================== 01012 [..] This section provides functions allowing to: 01013 (+) Initialize the DFSDM filter. 01014 (+) De-initialize the DFSDM filter. 01015 @endverbatim 01016 * @{ 01017 */ 01018 01019 /** 01020 * @brief Initialize the DFSDM filter according to the specified parameters 01021 * in the DFSDM_FilterInitTypeDef structure and initialize the associated handle. 01022 * @param hdfsdm_filter : DFSDM filter handle. 01023 * @retval HAL status. 01024 */ 01025 HAL_StatusTypeDef HAL_DFSDM_FilterInit(DFSDM_Filter_HandleTypeDef *hdfsdm_filter) 01026 { 01027 /* Check parameters */ 01028 assert_param(IS_DFSDM_FILTER_ALL_INSTANCE(hdfsdm_filter->Instance)); 01029 assert_param(IS_DFSDM_FILTER_REG_TRIGGER(hdfsdm_filter->Init.RegularParam.Trigger)); 01030 assert_param(IS_FUNCTIONAL_STATE(hdfsdm_filter->Init.RegularParam.FastMode)); 01031 assert_param(IS_FUNCTIONAL_STATE(hdfsdm_filter->Init.RegularParam.DmaMode)); 01032 assert_param(IS_DFSDM_FILTER_INJ_TRIGGER(hdfsdm_filter->Init.InjectedParam.Trigger)); 01033 assert_param(IS_FUNCTIONAL_STATE(hdfsdm_filter->Init.InjectedParam.ScanMode)); 01034 assert_param(IS_FUNCTIONAL_STATE(hdfsdm_filter->Init.InjectedParam.DmaMode)); 01035 assert_param(IS_DFSDM_FILTER_SINC_ORDER(hdfsdm_filter->Init.FilterParam.SincOrder)); 01036 assert_param(IS_DFSDM_FILTER_OVS_RATIO(hdfsdm_filter->Init.FilterParam.Oversampling)); 01037 assert_param(IS_DFSDM_FILTER_INTEGRATOR_OVS_RATIO(hdfsdm_filter->Init.FilterParam.IntOversampling)); 01038 01039 /* Check DFSDM Channel handle */ 01040 if(hdfsdm_filter == NULL) 01041 { 01042 return HAL_ERROR; 01043 } 01044 01045 /* Check parameters compatibility */ 01046 if((hdfsdm_filter->Instance == DFSDM_Filter0) && 01047 ((hdfsdm_filter->Init.RegularParam.Trigger == DFSDM_FILTER_SYNC_TRIGGER) || 01048 (hdfsdm_filter->Init.InjectedParam.Trigger == DFSDM_FILTER_SYNC_TRIGGER))) 01049 { 01050 return HAL_ERROR; 01051 } 01052 01053 /* Initialize DFSDM filter variables with default values */ 01054 hdfsdm_filter->RegularContMode = DFSDM_CONTINUOUS_CONV_OFF; 01055 hdfsdm_filter->InjectedChannelsNbr = 1; 01056 hdfsdm_filter->InjConvRemaining = 1; 01057 hdfsdm_filter->ErrorCode = DFSDM_FILTER_ERROR_NONE; 01058 01059 /* Call MSP init function */ 01060 HAL_DFSDM_FilterMspInit(hdfsdm_filter); 01061 01062 /* Set regular parameters */ 01063 hdfsdm_filter->Instance->CR1 &= ~(DFSDM_CR1_RSYNC); 01064 if(hdfsdm_filter->Init.RegularParam.FastMode == ENABLE) 01065 { 01066 hdfsdm_filter->Instance->CR1 |= DFSDM_CR1_FAST; 01067 } 01068 else 01069 { 01070 hdfsdm_filter->Instance->CR1 &= ~(DFSDM_CR1_FAST); 01071 } 01072 01073 if(hdfsdm_filter->Init.RegularParam.DmaMode == ENABLE) 01074 { 01075 hdfsdm_filter->Instance->CR1 |= DFSDM_CR1_RDMAEN; 01076 } 01077 else 01078 { 01079 hdfsdm_filter->Instance->CR1 &= ~(DFSDM_CR1_RDMAEN); 01080 } 01081 01082 /* Set injected parameters */ 01083 hdfsdm_filter->Instance->CR1 &= ~(DFSDM_CR1_JSYNC | DFSDM_CR1_JEXTEN | DFSDM_CR1_JEXTSEL); 01084 if(hdfsdm_filter->Init.InjectedParam.Trigger == DFSDM_FILTER_EXT_TRIGGER) 01085 { 01086 assert_param(IS_DFSDM_FILTER_EXT_TRIG(hdfsdm_filter->Init.InjectedParam.ExtTrigger)); 01087 assert_param(IS_DFSDM_FILTER_EXT_TRIG_EDGE(hdfsdm_filter->Init.InjectedParam.ExtTriggerEdge)); 01088 hdfsdm_filter->Instance->CR1 |= (hdfsdm_filter->Init.InjectedParam.ExtTrigger); 01089 } 01090 01091 if(hdfsdm_filter->Init.InjectedParam.ScanMode == ENABLE) 01092 { 01093 hdfsdm_filter->Instance->CR1 |= DFSDM_CR1_JSCAN; 01094 } 01095 else 01096 { 01097 hdfsdm_filter->Instance->CR1 &= ~(DFSDM_CR1_JSCAN); 01098 } 01099 01100 if(hdfsdm_filter->Init.InjectedParam.DmaMode == ENABLE) 01101 { 01102 hdfsdm_filter->Instance->CR1 |= DFSDM_CR1_JDMAEN; 01103 } 01104 else 01105 { 01106 hdfsdm_filter->Instance->CR1 &= ~(DFSDM_CR1_JDMAEN); 01107 } 01108 01109 /* Set filter parameters */ 01110 hdfsdm_filter->Instance->FCR &= ~(DFSDM_FCR_FORD | DFSDM_FCR_FOSR | DFSDM_FCR_IOSR); 01111 hdfsdm_filter->Instance->FCR |= (hdfsdm_filter->Init.FilterParam.SincOrder | 01112 ((hdfsdm_filter->Init.FilterParam.Oversampling - 1) << DFSDM_FCR_FOSR_OFFSET) | 01113 (hdfsdm_filter->Init.FilterParam.IntOversampling - 1)); 01114 01115 /* Store regular and injected triggers and injected scan mode*/ 01116 hdfsdm_filter->RegularTrigger = hdfsdm_filter->Init.RegularParam.Trigger; 01117 hdfsdm_filter->InjectedTrigger = hdfsdm_filter->Init.InjectedParam.Trigger; 01118 hdfsdm_filter->ExtTriggerEdge = hdfsdm_filter->Init.InjectedParam.ExtTriggerEdge; 01119 hdfsdm_filter->InjectedScanMode = hdfsdm_filter->Init.InjectedParam.ScanMode; 01120 01121 /* Enable DFSDM filter */ 01122 hdfsdm_filter->Instance->CR1 |= DFSDM_CR1_DFEN; 01123 01124 /* Set DFSDM filter to ready state */ 01125 hdfsdm_filter->State = HAL_DFSDM_FILTER_STATE_READY; 01126 01127 return HAL_OK; 01128 } 01129 01130 /** 01131 * @brief De-initializes the DFSDM filter. 01132 * @param hdfsdm_filter : DFSDM filter handle. 01133 * @retval HAL status. 01134 */ 01135 HAL_StatusTypeDef HAL_DFSDM_FilterDeInit(DFSDM_Filter_HandleTypeDef *hdfsdm_filter) 01136 { 01137 /* Check parameters */ 01138 assert_param(IS_DFSDM_FILTER_ALL_INSTANCE(hdfsdm_filter->Instance)); 01139 01140 /* Check DFSDM filter handle */ 01141 if(hdfsdm_filter == NULL) 01142 { 01143 return HAL_ERROR; 01144 } 01145 01146 /* Disable the DFSDM filter */ 01147 hdfsdm_filter->Instance->CR1 &= ~(DFSDM_CR1_DFEN); 01148 01149 /* Call MSP deinit function */ 01150 HAL_DFSDM_FilterMspDeInit(hdfsdm_filter); 01151 01152 /* Set DFSDM filter in reset state */ 01153 hdfsdm_filter->State = HAL_DFSDM_FILTER_STATE_RESET; 01154 01155 return HAL_OK; 01156 } 01157 01158 /** 01159 * @brief Initializes the DFSDM filter MSP. 01160 * @param hdfsdm_filter : DFSDM filter handle. 01161 * @retval None 01162 */ 01163 __weak void HAL_DFSDM_FilterMspInit(DFSDM_Filter_HandleTypeDef *hdfsdm_filter) 01164 { 01165 /* NOTE : This function should not be modified, when the function is needed, 01166 the HAL_DFSDM_FilterMspInit could be implemented in the user file. 01167 */ 01168 } 01169 01170 /** 01171 * @brief De-initializes the DFSDM filter MSP. 01172 * @param hdfsdm_filter : DFSDM filter handle. 01173 * @retval None 01174 */ 01175 __weak void HAL_DFSDM_FilterMspDeInit(DFSDM_Filter_HandleTypeDef *hdfsdm_filter) 01176 { 01177 /* NOTE : This function should not be modified, when the function is needed, 01178 the HAL_DFSDM_FilterMspDeInit could be implemented in the user file. 01179 */ 01180 } 01181 01182 /** 01183 * @} 01184 */ 01185 01186 /** @defgroup DFSDM_Exported_Functions_Group2_Filter Filter control functions 01187 * @brief Filter control functions 01188 * 01189 @verbatim 01190 ============================================================================== 01191 ##### Filter control functions ##### 01192 ============================================================================== 01193 [..] This section provides functions allowing to: 01194 (+) Select channel and enable/disable continuous mode for regular conversion. 01195 (+) Select channels for injected conversion. 01196 @endverbatim 01197 * @{ 01198 */ 01199 01200 /** 01201 * @brief This function allows to select channel and to enable/disable 01202 * continuous mode for regular conversion. 01203 * @param hdfsdm_filter : DFSDM filter handle. 01204 * @param Channel : Channel for regular conversion. 01205 * This parameter can be a value of @ref DFSDM_Channel_Selection. 01206 * @param ContinuousMode : Enable/disable continuous mode for regular conversion. 01207 * This parameter can be a value of @ref DFSDM_ContinuousMode. 01208 * @retval HAL status 01209 */ 01210 HAL_StatusTypeDef HAL_DFSDM_FilterConfigRegChannel(DFSDM_Filter_HandleTypeDef *hdfsdm_filter, 01211 uint32_t Channel, 01212 uint32_t ContinuousMode) 01213 { 01214 HAL_StatusTypeDef status = HAL_OK; 01215 01216 /* Check parameters */ 01217 assert_param(IS_DFSDM_FILTER_ALL_INSTANCE(hdfsdm_filter->Instance)); 01218 assert_param(IS_DFSDM_REGULAR_CHANNEL(Channel)); 01219 assert_param(IS_DFSDM_CONTINUOUS_MODE(ContinuousMode)); 01220 01221 /* Check DFSDM filter state */ 01222 if((hdfsdm_filter->State != HAL_DFSDM_FILTER_STATE_RESET) && 01223 (hdfsdm_filter->State != HAL_DFSDM_FILTER_STATE_ERROR)) 01224 { 01225 /* Configure channel and continuous mode for regular conversion */ 01226 hdfsdm_filter->Instance->CR1 &= ~(DFSDM_CR1_RCH | DFSDM_CR1_RCONT); 01227 if(ContinuousMode == DFSDM_CONTINUOUS_CONV_ON) 01228 { 01229 hdfsdm_filter->Instance->CR1 |= (uint32_t) (((Channel & DFSDM_MSB_MASK) << DFSDM_CR1_MSB_RCH_OFFSET) | 01230 DFSDM_CR1_RCONT); 01231 } 01232 else 01233 { 01234 hdfsdm_filter->Instance->CR1 |= (uint32_t) ((Channel & DFSDM_MSB_MASK) << DFSDM_CR1_MSB_RCH_OFFSET); 01235 } 01236 /* Store continuous mode information */ 01237 hdfsdm_filter->RegularContMode = ContinuousMode; 01238 } 01239 else 01240 { 01241 status = HAL_ERROR; 01242 } 01243 01244 /* Return function status */ 01245 return status; 01246 } 01247 01248 /** 01249 * @brief This function allows to select channels for injected conversion. 01250 * @param hdfsdm_filter : DFSDM filter handle. 01251 * @param Channel : Channels for injected conversion. 01252 * This parameter can be a values combination of @ref DFSDM_Channel_Selection. 01253 * @retval HAL status 01254 */ 01255 HAL_StatusTypeDef HAL_DFSDM_FilterConfigInjChannel(DFSDM_Filter_HandleTypeDef *hdfsdm_filter, 01256 uint32_t Channel) 01257 { 01258 HAL_StatusTypeDef status = HAL_OK; 01259 01260 /* Check parameters */ 01261 assert_param(IS_DFSDM_FILTER_ALL_INSTANCE(hdfsdm_filter->Instance)); 01262 assert_param(IS_DFSDM_INJECTED_CHANNEL(Channel)); 01263 01264 /* Check DFSDM filter state */ 01265 if((hdfsdm_filter->State != HAL_DFSDM_FILTER_STATE_RESET) && 01266 (hdfsdm_filter->State != HAL_DFSDM_FILTER_STATE_ERROR)) 01267 { 01268 /* Configure channel for injected conversion */ 01269 hdfsdm_filter->Instance->JCHGR = (uint32_t) (Channel & DFSDM_LSB_MASK); 01270 /* Store number of injected channels */ 01271 hdfsdm_filter->InjectedChannelsNbr = DFSDM_GetInjChannelsNbr(Channel); 01272 /* Update number of injected channels remaining */ 01273 hdfsdm_filter->InjConvRemaining = (hdfsdm_filter->InjectedScanMode == ENABLE) ? \ 01274 hdfsdm_filter->InjectedChannelsNbr : 1; 01275 } 01276 else 01277 { 01278 status = HAL_ERROR; 01279 } 01280 /* Return function status */ 01281 return status; 01282 } 01283 01284 /** 01285 * @} 01286 */ 01287 01288 /** @defgroup DFSDM_Exported_Functions_Group3_Filter Filter operation functions 01289 * @brief Filter operation functions 01290 * 01291 @verbatim 01292 ============================================================================== 01293 ##### Filter operation functions ##### 01294 ============================================================================== 01295 [..] This section provides functions allowing to: 01296 (+) Start conversion of regular/injected channel. 01297 (+) Poll for the end of regular/injected conversion. 01298 (+) Stop conversion of regular/injected channel. 01299 (+) Start conversion of regular/injected channel and enable interrupt. 01300 (+) Call the callback functions at the end of regular/injected conversions. 01301 (+) Stop conversion of regular/injected channel and disable interrupt. 01302 (+) Start conversion of regular/injected channel and enable DMA transfer. 01303 (+) Stop conversion of regular/injected channel and disable DMA transfer. 01304 (+) Start analog watchdog and enable interrupt. 01305 (+) Call the callback function when analog watchdog occurs. 01306 (+) Stop analog watchdog and disable interrupt. 01307 (+) Start extreme detector. 01308 (+) Stop extreme detector. 01309 (+) Get result of regular channel conversion. 01310 (+) Get result of injected channel conversion. 01311 (+) Get extreme detector maximum and minimum values. 01312 (+) Get conversion time. 01313 (+) Handle DFSDM interrupt request. 01314 @endverbatim 01315 * @{ 01316 */ 01317 01318 /** 01319 * @brief This function allows to start regular conversion in polling mode. 01320 * @note This function should be called only when DFSDM filter instance is 01321 * in idle state or if injected conversion is ongoing. 01322 * @param hdfsdm_filter : DFSDM filter handle. 01323 * @retval HAL status 01324 */ 01325 HAL_StatusTypeDef HAL_DFSDM_FilterRegularStart(DFSDM_Filter_HandleTypeDef *hdfsdm_filter) 01326 { 01327 HAL_StatusTypeDef status = HAL_OK; 01328 01329 /* Check parameters */ 01330 assert_param(IS_DFSDM_FILTER_ALL_INSTANCE(hdfsdm_filter->Instance)); 01331 01332 /* Check DFSDM filter state */ 01333 if((hdfsdm_filter->State == HAL_DFSDM_FILTER_STATE_READY) || \ 01334 (hdfsdm_filter->State == HAL_DFSDM_FILTER_STATE_INJ)) 01335 { 01336 /* Start regular conversion */ 01337 DFSDM_RegConvStart(hdfsdm_filter); 01338 } 01339 else 01340 { 01341 status = HAL_ERROR; 01342 } 01343 /* Return function status */ 01344 return status; 01345 } 01346 01347 /** 01348 * @brief This function allows to poll for the end of regular conversion. 01349 * @note This function should be called only if regular conversion is ongoing. 01350 * @param hdfsdm_filter : DFSDM filter handle. 01351 * @param Timeout : Timeout value in milliseconds. 01352 * @retval HAL status 01353 */ 01354 HAL_StatusTypeDef HAL_DFSDM_FilterPollForRegConversion(DFSDM_Filter_HandleTypeDef *hdfsdm_filter, 01355 uint32_t Timeout) 01356 { 01357 uint32_t tickstart; 01358 01359 /* Check parameters */ 01360 assert_param(IS_DFSDM_FILTER_ALL_INSTANCE(hdfsdm_filter->Instance)); 01361 01362 /* Check DFSDM filter state */ 01363 if((hdfsdm_filter->State != HAL_DFSDM_FILTER_STATE_REG) && \ 01364 (hdfsdm_filter->State != HAL_DFSDM_FILTER_STATE_REG_INJ)) 01365 { 01366 /* Return error status */ 01367 return HAL_ERROR; 01368 } 01369 else 01370 { 01371 /* Get timeout */ 01372 tickstart = HAL_GetTick(); 01373 01374 /* Wait end of regular conversion */ 01375 while((hdfsdm_filter->Instance->ISR & DFSDM_ISR_REOCF) != DFSDM_ISR_REOCF) 01376 { 01377 /* Check the Timeout */ 01378 if(Timeout != HAL_MAX_DELAY) 01379 { 01380 if((Timeout == 0) || ((HAL_GetTick()-tickstart) > Timeout)) 01381 { 01382 /* Return timeout status */ 01383 return HAL_TIMEOUT; 01384 } 01385 } 01386 } 01387 /* Check if overrun occurs */ 01388 if((hdfsdm_filter->Instance->ISR & DFSDM_ISR_ROVRF) == DFSDM_ISR_ROVRF) 01389 { 01390 /* Update error code and call error callback */ 01391 hdfsdm_filter->ErrorCode = DFSDM_FILTER_ERROR_REGULAR_OVERRUN; 01392 HAL_DFSDM_FilterErrorCallback(hdfsdm_filter); 01393 01394 /* Clear regular overrun flag */ 01395 hdfsdm_filter->Instance->ICR = DFSDM_ICR_CLRROVRF; 01396 } 01397 /* Update DFSDM filter state only if not continuous conversion and SW trigger */ 01398 if((hdfsdm_filter->RegularContMode == DFSDM_CONTINUOUS_CONV_OFF) && \ 01399 (hdfsdm_filter->RegularTrigger == DFSDM_FILTER_SW_TRIGGER)) 01400 { 01401 hdfsdm_filter->State = (hdfsdm_filter->State == HAL_DFSDM_FILTER_STATE_REG) ? \ 01402 HAL_DFSDM_FILTER_STATE_READY : HAL_DFSDM_FILTER_STATE_INJ; 01403 } 01404 /* Return function status */ 01405 return HAL_OK; 01406 } 01407 } 01408 01409 /** 01410 * @brief This function allows to stop regular conversion in polling mode. 01411 * @note This function should be called only if regular conversion is ongoing. 01412 * @param hdfsdm_filter : DFSDM filter handle. 01413 * @retval HAL status 01414 */ 01415 HAL_StatusTypeDef HAL_DFSDM_FilterRegularStop(DFSDM_Filter_HandleTypeDef *hdfsdm_filter) 01416 { 01417 HAL_StatusTypeDef status = HAL_OK; 01418 01419 /* Check parameters */ 01420 assert_param(IS_DFSDM_FILTER_ALL_INSTANCE(hdfsdm_filter->Instance)); 01421 01422 /* Check DFSDM filter state */ 01423 if((hdfsdm_filter->State != HAL_DFSDM_FILTER_STATE_REG) && \ 01424 (hdfsdm_filter->State != HAL_DFSDM_FILTER_STATE_REG_INJ)) 01425 { 01426 /* Return error status */ 01427 status = HAL_ERROR; 01428 } 01429 else 01430 { 01431 /* Stop regular conversion */ 01432 DFSDM_RegConvStop(hdfsdm_filter); 01433 } 01434 /* Return function status */ 01435 return status; 01436 } 01437 01438 /** 01439 * @brief This function allows to start regular conversion in interrupt mode. 01440 * @note This function should be called only when DFSDM filter instance is 01441 * in idle state or if injected conversion is ongoing. 01442 * @param hdfsdm_filter : DFSDM filter handle. 01443 * @retval HAL status 01444 */ 01445 HAL_StatusTypeDef HAL_DFSDM_FilterRegularStart_IT(DFSDM_Filter_HandleTypeDef *hdfsdm_filter) 01446 { 01447 HAL_StatusTypeDef status = HAL_OK; 01448 01449 /* Check parameters */ 01450 assert_param(IS_DFSDM_FILTER_ALL_INSTANCE(hdfsdm_filter->Instance)); 01451 01452 /* Check DFSDM filter state */ 01453 if((hdfsdm_filter->State == HAL_DFSDM_FILTER_STATE_READY) || \ 01454 (hdfsdm_filter->State == HAL_DFSDM_FILTER_STATE_INJ)) 01455 { 01456 /* Enable interrupts for regular conversions */ 01457 hdfsdm_filter->Instance->CR2 |= (DFSDM_CR2_REOCIE | DFSDM_CR2_ROVRIE); 01458 01459 /* Start regular conversion */ 01460 DFSDM_RegConvStart(hdfsdm_filter); 01461 } 01462 else 01463 { 01464 status = HAL_ERROR; 01465 } 01466 /* Return function status */ 01467 return status; 01468 } 01469 01470 /** 01471 * @brief This function allows to stop regular conversion in interrupt mode. 01472 * @note This function should be called only if regular conversion is ongoing. 01473 * @param hdfsdm_filter : DFSDM filter handle. 01474 * @retval HAL status 01475 */ 01476 HAL_StatusTypeDef HAL_DFSDM_FilterRegularStop_IT(DFSDM_Filter_HandleTypeDef *hdfsdm_filter) 01477 { 01478 HAL_StatusTypeDef status = HAL_OK; 01479 01480 /* Check parameters */ 01481 assert_param(IS_DFSDM_FILTER_ALL_INSTANCE(hdfsdm_filter->Instance)); 01482 01483 /* Check DFSDM filter state */ 01484 if((hdfsdm_filter->State != HAL_DFSDM_FILTER_STATE_REG) && \ 01485 (hdfsdm_filter->State != HAL_DFSDM_FILTER_STATE_REG_INJ)) 01486 { 01487 /* Return error status */ 01488 status = HAL_ERROR; 01489 } 01490 else 01491 { 01492 /* Disable interrupts for regular conversions */ 01493 hdfsdm_filter->Instance->CR2 &= ~(DFSDM_CR2_REOCIE | DFSDM_CR2_ROVRIE); 01494 01495 /* Stop regular conversion */ 01496 DFSDM_RegConvStop(hdfsdm_filter); 01497 } 01498 /* Return function status */ 01499 return status; 01500 } 01501 01502 /** 01503 * @brief This function allows to start regular conversion in DMA mode. 01504 * @note This function should be called only when DFSDM filter instance is 01505 * in idle state or if injected conversion is ongoing. 01506 * Please note that data on buffer will contain signed regular conversion 01507 * value on 24 most significant bits and corresponding channel on 3 least 01508 * significant bits. 01509 * @param hdfsdm_filter : DFSDM filter handle. 01510 * @param pData : The destination buffer address. 01511 * @param Length : The length of data to be transferred from DFSDM filter to memory. 01512 * @retval HAL status 01513 */ 01514 HAL_StatusTypeDef HAL_DFSDM_FilterRegularStart_DMA(DFSDM_Filter_HandleTypeDef *hdfsdm_filter, 01515 int32_t *pData, 01516 uint32_t Length) 01517 { 01518 HAL_StatusTypeDef status = HAL_OK; 01519 01520 /* Check parameters */ 01521 assert_param(IS_DFSDM_FILTER_ALL_INSTANCE(hdfsdm_filter->Instance)); 01522 01523 /* Check destination address and length */ 01524 if((pData == NULL) || (Length == 0)) 01525 { 01526 status = HAL_ERROR; 01527 } 01528 /* Check that DMA is enabled for regular conversion */ 01529 else if((hdfsdm_filter->Instance->CR1 & DFSDM_CR1_RDMAEN) != DFSDM_CR1_RDMAEN) 01530 { 01531 status = HAL_ERROR; 01532 } 01533 /* Check parameters compatibility */ 01534 else if((hdfsdm_filter->RegularTrigger == DFSDM_FILTER_SW_TRIGGER) && \ 01535 (hdfsdm_filter->RegularContMode == DFSDM_CONTINUOUS_CONV_OFF) && \ 01536 (hdfsdm_filter->hdmaReg->Init.Mode == DMA_NORMAL) && \ 01537 (Length != 1)) 01538 { 01539 status = HAL_ERROR; 01540 } 01541 else if((hdfsdm_filter->RegularTrigger == DFSDM_FILTER_SW_TRIGGER) && \ 01542 (hdfsdm_filter->RegularContMode == DFSDM_CONTINUOUS_CONV_OFF) && \ 01543 (hdfsdm_filter->hdmaReg->Init.Mode == DMA_CIRCULAR)) 01544 { 01545 status = HAL_ERROR; 01546 } 01547 /* Check DFSDM filter state */ 01548 else if((hdfsdm_filter->State == HAL_DFSDM_FILTER_STATE_READY) || \ 01549 (hdfsdm_filter->State == HAL_DFSDM_FILTER_STATE_INJ)) 01550 { 01551 /* Set callbacks on DMA handler */ 01552 hdfsdm_filter->hdmaReg->XferCpltCallback = DFSDM_DMARegularConvCplt; 01553 hdfsdm_filter->hdmaReg->XferErrorCallback = DFSDM_DMAError; 01554 hdfsdm_filter->hdmaReg->XferHalfCpltCallback = (hdfsdm_filter->hdmaReg->Init.Mode == DMA_CIRCULAR) ?\ 01555 DFSDM_DMARegularHalfConvCplt : NULL; 01556 01557 /* Start DMA in interrupt mode */ 01558 if(HAL_DMA_Start_IT(hdfsdm_filter->hdmaReg, (uint32_t)&hdfsdm_filter->Instance->RDATAR, \ 01559 (uint32_t) pData, Length) != HAL_OK) 01560 { 01561 /* Set DFSDM filter in error state */ 01562 hdfsdm_filter->State = HAL_DFSDM_FILTER_STATE_ERROR; 01563 status = HAL_ERROR; 01564 } 01565 else 01566 { 01567 /* Start regular conversion */ 01568 DFSDM_RegConvStart(hdfsdm_filter); 01569 } 01570 } 01571 else 01572 { 01573 status = HAL_ERROR; 01574 } 01575 /* Return function status */ 01576 return status; 01577 } 01578 01579 /** 01580 * @brief This function allows to start regular conversion in DMA mode and to get 01581 * only the 16 most significant bits of conversion. 01582 * @note This function should be called only when DFSDM filter instance is 01583 * in idle state or if injected conversion is ongoing. 01584 * Please note that data on buffer will contain signed 16 most significant 01585 * bits of regular conversion. 01586 * @param hdfsdm_filter : DFSDM filter handle. 01587 * @param pData : The destination buffer address. 01588 * @param Length : The length of data to be transferred from DFSDM filter to memory. 01589 * @retval HAL status 01590 */ 01591 HAL_StatusTypeDef HAL_DFSDM_FilterRegularMsbStart_DMA(DFSDM_Filter_HandleTypeDef *hdfsdm_filter, 01592 int16_t *pData, 01593 uint32_t Length) 01594 { 01595 HAL_StatusTypeDef status = HAL_OK; 01596 01597 /* Check parameters */ 01598 assert_param(IS_DFSDM_FILTER_ALL_INSTANCE(hdfsdm_filter->Instance)); 01599 01600 /* Check destination address and length */ 01601 if((pData == NULL) || (Length == 0)) 01602 { 01603 status = HAL_ERROR; 01604 } 01605 /* Check that DMA is enabled for regular conversion */ 01606 else if((hdfsdm_filter->Instance->CR1 & DFSDM_CR1_RDMAEN) != DFSDM_CR1_RDMAEN) 01607 { 01608 status = HAL_ERROR; 01609 } 01610 /* Check parameters compatibility */ 01611 else if((hdfsdm_filter->RegularTrigger == DFSDM_FILTER_SW_TRIGGER) && \ 01612 (hdfsdm_filter->RegularContMode == DFSDM_CONTINUOUS_CONV_OFF) && \ 01613 (hdfsdm_filter->hdmaReg->Init.Mode == DMA_NORMAL) && \ 01614 (Length != 1)) 01615 { 01616 status = HAL_ERROR; 01617 } 01618 else if((hdfsdm_filter->RegularTrigger == DFSDM_FILTER_SW_TRIGGER) && \ 01619 (hdfsdm_filter->RegularContMode == DFSDM_CONTINUOUS_CONV_OFF) && \ 01620 (hdfsdm_filter->hdmaReg->Init.Mode == DMA_CIRCULAR)) 01621 { 01622 status = HAL_ERROR; 01623 } 01624 /* Check DFSDM filter state */ 01625 else if((hdfsdm_filter->State == HAL_DFSDM_FILTER_STATE_READY) || \ 01626 (hdfsdm_filter->State == HAL_DFSDM_FILTER_STATE_INJ)) 01627 { 01628 /* Set callbacks on DMA handler */ 01629 hdfsdm_filter->hdmaReg->XferCpltCallback = DFSDM_DMARegularConvCplt; 01630 hdfsdm_filter->hdmaReg->XferErrorCallback = DFSDM_DMAError; 01631 hdfsdm_filter->hdmaReg->XferHalfCpltCallback = (hdfsdm_filter->hdmaReg->Init.Mode == DMA_CIRCULAR) ?\ 01632 DFSDM_DMARegularHalfConvCplt : NULL; 01633 01634 /* Start DMA in interrupt mode */ 01635 if(HAL_DMA_Start_IT(hdfsdm_filter->hdmaReg, (uint32_t)(&hdfsdm_filter->Instance->RDATAR) + 2, \ 01636 (uint32_t) pData, Length) != HAL_OK) 01637 { 01638 /* Set DFSDM filter in error state */ 01639 hdfsdm_filter->State = HAL_DFSDM_FILTER_STATE_ERROR; 01640 status = HAL_ERROR; 01641 } 01642 else 01643 { 01644 /* Start regular conversion */ 01645 DFSDM_RegConvStart(hdfsdm_filter); 01646 } 01647 } 01648 else 01649 { 01650 status = HAL_ERROR; 01651 } 01652 /* Return function status */ 01653 return status; 01654 } 01655 01656 /** 01657 * @brief This function allows to stop regular conversion in DMA mode. 01658 * @note This function should be called only if regular conversion is ongoing. 01659 * @param hdfsdm_filter : DFSDM filter handle. 01660 * @retval HAL status 01661 */ 01662 HAL_StatusTypeDef HAL_DFSDM_FilterRegularStop_DMA(DFSDM_Filter_HandleTypeDef *hdfsdm_filter) 01663 { 01664 HAL_StatusTypeDef status = HAL_OK; 01665 01666 /* Check parameters */ 01667 assert_param(IS_DFSDM_FILTER_ALL_INSTANCE(hdfsdm_filter->Instance)); 01668 01669 /* Check DFSDM filter state */ 01670 if((hdfsdm_filter->State != HAL_DFSDM_FILTER_STATE_REG) && \ 01671 (hdfsdm_filter->State != HAL_DFSDM_FILTER_STATE_REG_INJ)) 01672 { 01673 /* Return error status */ 01674 status = HAL_ERROR; 01675 } 01676 else 01677 { 01678 /* Stop current DMA transfer */ 01679 if(HAL_DMA_Abort(hdfsdm_filter->hdmaReg) != HAL_OK) 01680 { 01681 /* Set DFSDM filter in error state */ 01682 hdfsdm_filter->State = HAL_DFSDM_FILTER_STATE_ERROR; 01683 status = HAL_ERROR; 01684 } 01685 else 01686 { 01687 /* Stop regular conversion */ 01688 DFSDM_RegConvStop(hdfsdm_filter); 01689 } 01690 } 01691 /* Return function status */ 01692 return status; 01693 } 01694 01695 /** 01696 * @brief This function allows to get regular conversion value. 01697 * @param hdfsdm_filter : DFSDM filter handle. 01698 * @param Channel : Corresponding channel of regular conversion. 01699 * @retval Regular conversion value 01700 */ 01701 int32_t HAL_DFSDM_FilterGetRegularValue(DFSDM_Filter_HandleTypeDef *hdfsdm_filter, 01702 uint32_t *Channel) 01703 { 01704 uint32_t reg = 0; 01705 int32_t value = 0; 01706 01707 /* Check parameters */ 01708 assert_param(IS_DFSDM_FILTER_ALL_INSTANCE(hdfsdm_filter->Instance)); 01709 assert_param(Channel != NULL); 01710 01711 /* Get value of data register for regular channel */ 01712 reg = hdfsdm_filter->Instance->RDATAR; 01713 01714 /* Extract channel and regular conversion value */ 01715 *Channel = (reg & DFSDM_RDATAR_RDATACH); 01716 value = ((int32_t)(reg & DFSDM_RDATAR_RDATA) >> DFSDM_RDATAR_DATA_OFFSET); 01717 01718 /* return regular conversion value */ 01719 return value; 01720 } 01721 01722 /** 01723 * @brief This function allows to start injected conversion in polling mode. 01724 * @note This function should be called only when DFSDM filter instance is 01725 * in idle state or if regular conversion is ongoing. 01726 * @param hdfsdm_filter : DFSDM filter handle. 01727 * @retval HAL status 01728 */ 01729 HAL_StatusTypeDef HAL_DFSDM_FilterInjectedStart(DFSDM_Filter_HandleTypeDef *hdfsdm_filter) 01730 { 01731 HAL_StatusTypeDef status = HAL_OK; 01732 01733 /* Check parameters */ 01734 assert_param(IS_DFSDM_FILTER_ALL_INSTANCE(hdfsdm_filter->Instance)); 01735 01736 /* Check DFSDM filter state */ 01737 if((hdfsdm_filter->State == HAL_DFSDM_FILTER_STATE_READY) || \ 01738 (hdfsdm_filter->State == HAL_DFSDM_FILTER_STATE_REG)) 01739 { 01740 /* Start injected conversion */ 01741 DFSDM_InjConvStart(hdfsdm_filter); 01742 } 01743 else 01744 { 01745 status = HAL_ERROR; 01746 } 01747 /* Return function status */ 01748 return status; 01749 } 01750 01751 /** 01752 * @brief This function allows to poll for the end of injected conversion. 01753 * @note This function should be called only if injected conversion is ongoing. 01754 * @param hdfsdm_filter : DFSDM filter handle. 01755 * @param Timeout : Timeout value in milliseconds. 01756 * @retval HAL status 01757 */ 01758 HAL_StatusTypeDef HAL_DFSDM_FilterPollForInjConversion(DFSDM_Filter_HandleTypeDef *hdfsdm_filter, 01759 uint32_t Timeout) 01760 { 01761 uint32_t tickstart; 01762 01763 /* Check parameters */ 01764 assert_param(IS_DFSDM_FILTER_ALL_INSTANCE(hdfsdm_filter->Instance)); 01765 01766 /* Check DFSDM filter state */ 01767 if((hdfsdm_filter->State != HAL_DFSDM_FILTER_STATE_INJ) && \ 01768 (hdfsdm_filter->State != HAL_DFSDM_FILTER_STATE_REG_INJ)) 01769 { 01770 /* Return error status */ 01771 return HAL_ERROR; 01772 } 01773 else 01774 { 01775 /* Get timeout */ 01776 tickstart = HAL_GetTick(); 01777 01778 /* Wait end of injected conversions */ 01779 while((hdfsdm_filter->Instance->ISR & DFSDM_ISR_JEOCF) != DFSDM_ISR_JEOCF) 01780 { 01781 /* Check the Timeout */ 01782 if(Timeout != HAL_MAX_DELAY) 01783 { 01784 if((Timeout == 0) || ((HAL_GetTick()-tickstart) > Timeout)) 01785 { 01786 /* Return timeout status */ 01787 return HAL_TIMEOUT; 01788 } 01789 } 01790 } 01791 /* Check if overrun occurs */ 01792 if((hdfsdm_filter->Instance->ISR & DFSDM_ISR_JOVRF) == DFSDM_ISR_JOVRF) 01793 { 01794 /* Update error code and call error callback */ 01795 hdfsdm_filter->ErrorCode = DFSDM_FILTER_ERROR_INJECTED_OVERRUN; 01796 HAL_DFSDM_FilterErrorCallback(hdfsdm_filter); 01797 01798 /* Clear injected overrun flag */ 01799 hdfsdm_filter->Instance->ICR = DFSDM_ICR_CLRJOVRF; 01800 } 01801 01802 /* Update remaining injected conversions */ 01803 hdfsdm_filter->InjConvRemaining--; 01804 if(hdfsdm_filter->InjConvRemaining == 0) 01805 { 01806 /* Update DFSDM filter state only if trigger is software */ 01807 if(hdfsdm_filter->InjectedTrigger == DFSDM_FILTER_SW_TRIGGER) 01808 { 01809 hdfsdm_filter->State = (hdfsdm_filter->State == HAL_DFSDM_FILTER_STATE_INJ) ? \ 01810 HAL_DFSDM_FILTER_STATE_READY : HAL_DFSDM_FILTER_STATE_REG; 01811 } 01812 01813 /* end of injected sequence, reset the value */ 01814 hdfsdm_filter->InjConvRemaining = (hdfsdm_filter->InjectedScanMode == ENABLE) ? \ 01815 hdfsdm_filter->InjectedChannelsNbr : 1; 01816 } 01817 01818 /* Return function status */ 01819 return HAL_OK; 01820 } 01821 } 01822 01823 /** 01824 * @brief This function allows to stop injected conversion in polling mode. 01825 * @note This function should be called only if injected conversion is ongoing. 01826 * @param hdfsdm_filter : DFSDM filter handle. 01827 * @retval HAL status 01828 */ 01829 HAL_StatusTypeDef HAL_DFSDM_FilterInjectedStop(DFSDM_Filter_HandleTypeDef *hdfsdm_filter) 01830 { 01831 HAL_StatusTypeDef status = HAL_OK; 01832 01833 /* Check parameters */ 01834 assert_param(IS_DFSDM_FILTER_ALL_INSTANCE(hdfsdm_filter->Instance)); 01835 01836 /* Check DFSDM filter state */ 01837 if((hdfsdm_filter->State != HAL_DFSDM_FILTER_STATE_INJ) && \ 01838 (hdfsdm_filter->State != HAL_DFSDM_FILTER_STATE_REG_INJ)) 01839 { 01840 /* Return error status */ 01841 status = HAL_ERROR; 01842 } 01843 else 01844 { 01845 /* Stop injected conversion */ 01846 DFSDM_InjConvStop(hdfsdm_filter); 01847 } 01848 /* Return function status */ 01849 return status; 01850 } 01851 01852 /** 01853 * @brief This function allows to start injected conversion in interrupt mode. 01854 * @note This function should be called only when DFSDM filter instance is 01855 * in idle state or if regular conversion is ongoing. 01856 * @param hdfsdm_filter : DFSDM filter handle. 01857 * @retval HAL status 01858 */ 01859 HAL_StatusTypeDef HAL_DFSDM_FilterInjectedStart_IT(DFSDM_Filter_HandleTypeDef *hdfsdm_filter) 01860 { 01861 HAL_StatusTypeDef status = HAL_OK; 01862 01863 /* Check parameters */ 01864 assert_param(IS_DFSDM_FILTER_ALL_INSTANCE(hdfsdm_filter->Instance)); 01865 01866 /* Check DFSDM filter state */ 01867 if((hdfsdm_filter->State == HAL_DFSDM_FILTER_STATE_READY) || \ 01868 (hdfsdm_filter->State == HAL_DFSDM_FILTER_STATE_REG)) 01869 { 01870 /* Enable interrupts for injected conversions */ 01871 hdfsdm_filter->Instance->CR2 |= (DFSDM_CR2_JEOCIE | DFSDM_CR2_JOVRIE); 01872 01873 /* Start injected conversion */ 01874 DFSDM_InjConvStart(hdfsdm_filter); 01875 } 01876 else 01877 { 01878 status = HAL_ERROR; 01879 } 01880 /* Return function status */ 01881 return status; 01882 } 01883 01884 /** 01885 * @brief This function allows to stop injected conversion in interrupt mode. 01886 * @note This function should be called only if injected conversion is ongoing. 01887 * @param hdfsdm_filter : DFSDM filter handle. 01888 * @retval HAL status 01889 */ 01890 HAL_StatusTypeDef HAL_DFSDM_FilterInjectedStop_IT(DFSDM_Filter_HandleTypeDef *hdfsdm_filter) 01891 { 01892 HAL_StatusTypeDef status = HAL_OK; 01893 01894 /* Check parameters */ 01895 assert_param(IS_DFSDM_FILTER_ALL_INSTANCE(hdfsdm_filter->Instance)); 01896 01897 /* Check DFSDM filter state */ 01898 if((hdfsdm_filter->State != HAL_DFSDM_FILTER_STATE_INJ) && \ 01899 (hdfsdm_filter->State != HAL_DFSDM_FILTER_STATE_REG_INJ)) 01900 { 01901 /* Return error status */ 01902 status = HAL_ERROR; 01903 } 01904 else 01905 { 01906 /* Disable interrupts for injected conversions */ 01907 hdfsdm_filter->Instance->CR2 &= ~(DFSDM_CR2_JEOCIE | DFSDM_CR2_JOVRIE); 01908 01909 /* Stop injected conversion */ 01910 DFSDM_InjConvStop(hdfsdm_filter); 01911 } 01912 /* Return function status */ 01913 return status; 01914 } 01915 01916 /** 01917 * @brief This function allows to start injected conversion in DMA mode. 01918 * @note This function should be called only when DFSDM filter instance is 01919 * in idle state or if regular conversion is ongoing. 01920 * Please note that data on buffer will contain signed injected conversion 01921 * value on 24 most significant bits and corresponding channel on 3 least 01922 * significant bits. 01923 * @param hdfsdm_filter : DFSDM filter handle. 01924 * @param pData : The destination buffer address. 01925 * @param Length : The length of data to be transferred from DFSDM filter to memory. 01926 * @retval HAL status 01927 */ 01928 HAL_StatusTypeDef HAL_DFSDM_FilterInjectedStart_DMA(DFSDM_Filter_HandleTypeDef *hdfsdm_filter, 01929 int32_t *pData, 01930 uint32_t Length) 01931 { 01932 HAL_StatusTypeDef status = HAL_OK; 01933 01934 /* Check parameters */ 01935 assert_param(IS_DFSDM_FILTER_ALL_INSTANCE(hdfsdm_filter->Instance)); 01936 01937 /* Check destination address and length */ 01938 if((pData == NULL) || (Length == 0)) 01939 { 01940 status = HAL_ERROR; 01941 } 01942 /* Check that DMA is enabled for injected conversion */ 01943 else if((hdfsdm_filter->Instance->CR1 & DFSDM_CR1_JDMAEN) != DFSDM_CR1_JDMAEN) 01944 { 01945 status = HAL_ERROR; 01946 } 01947 /* Check parameters compatibility */ 01948 else if((hdfsdm_filter->InjectedTrigger == DFSDM_FILTER_SW_TRIGGER) && \ 01949 (hdfsdm_filter->hdmaInj->Init.Mode == DMA_NORMAL) && \ 01950 (Length > hdfsdm_filter->InjConvRemaining)) 01951 { 01952 status = HAL_ERROR; 01953 } 01954 else if((hdfsdm_filter->InjectedTrigger == DFSDM_FILTER_SW_TRIGGER) && \ 01955 (hdfsdm_filter->hdmaInj->Init.Mode == DMA_CIRCULAR)) 01956 { 01957 status = HAL_ERROR; 01958 } 01959 /* Check DFSDM filter state */ 01960 else if((hdfsdm_filter->State == HAL_DFSDM_FILTER_STATE_READY) || \ 01961 (hdfsdm_filter->State == HAL_DFSDM_FILTER_STATE_REG)) 01962 { 01963 /* Set callbacks on DMA handler */ 01964 hdfsdm_filter->hdmaInj->XferCpltCallback = DFSDM_DMAInjectedConvCplt; 01965 hdfsdm_filter->hdmaInj->XferErrorCallback = DFSDM_DMAError; 01966 hdfsdm_filter->hdmaInj->XferHalfCpltCallback = (hdfsdm_filter->hdmaInj->Init.Mode == DMA_CIRCULAR) ?\ 01967 DFSDM_DMAInjectedHalfConvCplt : NULL; 01968 01969 /* Start DMA in interrupt mode */ 01970 if(HAL_DMA_Start_IT(hdfsdm_filter->hdmaInj, (uint32_t)&hdfsdm_filter->Instance->JDATAR, \ 01971 (uint32_t) pData, Length) != HAL_OK) 01972 { 01973 /* Set DFSDM filter in error state */ 01974 hdfsdm_filter->State = HAL_DFSDM_FILTER_STATE_ERROR; 01975 status = HAL_ERROR; 01976 } 01977 else 01978 { 01979 /* Start injected conversion */ 01980 DFSDM_InjConvStart(hdfsdm_filter); 01981 } 01982 } 01983 else 01984 { 01985 status = HAL_ERROR; 01986 } 01987 /* Return function status */ 01988 return status; 01989 } 01990 01991 /** 01992 * @brief This function allows to start injected conversion in DMA mode and to get 01993 * only the 16 most significant bits of conversion. 01994 * @note This function should be called only when DFSDM filter instance is 01995 * in idle state or if regular conversion is ongoing. 01996 * Please note that data on buffer will contain signed 16 most significant 01997 * bits of injected conversion. 01998 * @param hdfsdm_filter : DFSDM filter handle. 01999 * @param pData : The destination buffer address. 02000 * @param Length : The length of data to be transferred from DFSDM filter to memory. 02001 * @retval HAL status 02002 */ 02003 HAL_StatusTypeDef HAL_DFSDM_FilterInjectedMsbStart_DMA(DFSDM_Filter_HandleTypeDef *hdfsdm_filter, 02004 int16_t *pData, 02005 uint32_t Length) 02006 { 02007 HAL_StatusTypeDef status = HAL_OK; 02008 02009 /* Check parameters */ 02010 assert_param(IS_DFSDM_FILTER_ALL_INSTANCE(hdfsdm_filter->Instance)); 02011 02012 /* Check destination address and length */ 02013 if((pData == NULL) || (Length == 0)) 02014 { 02015 status = HAL_ERROR; 02016 } 02017 /* Check that DMA is enabled for injected conversion */ 02018 else if((hdfsdm_filter->Instance->CR1 & DFSDM_CR1_JDMAEN) != DFSDM_CR1_JDMAEN) 02019 { 02020 status = HAL_ERROR; 02021 } 02022 /* Check parameters compatibility */ 02023 else if((hdfsdm_filter->InjectedTrigger == DFSDM_FILTER_SW_TRIGGER) && \ 02024 (hdfsdm_filter->hdmaInj->Init.Mode == DMA_NORMAL) && \ 02025 (Length > hdfsdm_filter->InjConvRemaining)) 02026 { 02027 status = HAL_ERROR; 02028 } 02029 else if((hdfsdm_filter->InjectedTrigger == DFSDM_FILTER_SW_TRIGGER) && \ 02030 (hdfsdm_filter->hdmaInj->Init.Mode == DMA_CIRCULAR)) 02031 { 02032 status = HAL_ERROR; 02033 } 02034 /* Check DFSDM filter state */ 02035 else if((hdfsdm_filter->State == HAL_DFSDM_FILTER_STATE_READY) || \ 02036 (hdfsdm_filter->State == HAL_DFSDM_FILTER_STATE_REG)) 02037 { 02038 /* Set callbacks on DMA handler */ 02039 hdfsdm_filter->hdmaInj->XferCpltCallback = DFSDM_DMAInjectedConvCplt; 02040 hdfsdm_filter->hdmaInj->XferErrorCallback = DFSDM_DMAError; 02041 hdfsdm_filter->hdmaInj->XferHalfCpltCallback = (hdfsdm_filter->hdmaInj->Init.Mode == DMA_CIRCULAR) ?\ 02042 DFSDM_DMAInjectedHalfConvCplt : NULL; 02043 02044 /* Start DMA in interrupt mode */ 02045 if(HAL_DMA_Start_IT(hdfsdm_filter->hdmaInj, (uint32_t)(&hdfsdm_filter->Instance->JDATAR) + 2, \ 02046 (uint32_t) pData, Length) != HAL_OK) 02047 { 02048 /* Set DFSDM filter in error state */ 02049 hdfsdm_filter->State = HAL_DFSDM_FILTER_STATE_ERROR; 02050 status = HAL_ERROR; 02051 } 02052 else 02053 { 02054 /* Start injected conversion */ 02055 DFSDM_InjConvStart(hdfsdm_filter); 02056 } 02057 } 02058 else 02059 { 02060 status = HAL_ERROR; 02061 } 02062 /* Return function status */ 02063 return status; 02064 } 02065 02066 /** 02067 * @brief This function allows to stop injected conversion in DMA mode. 02068 * @note This function should be called only if injected conversion is ongoing. 02069 * @param hdfsdm_filter : DFSDM filter handle. 02070 * @retval HAL status 02071 */ 02072 HAL_StatusTypeDef HAL_DFSDM_FilterInjectedStop_DMA(DFSDM_Filter_HandleTypeDef *hdfsdm_filter) 02073 { 02074 HAL_StatusTypeDef status = HAL_OK; 02075 02076 /* Check parameters */ 02077 assert_param(IS_DFSDM_FILTER_ALL_INSTANCE(hdfsdm_filter->Instance)); 02078 02079 /* Check DFSDM filter state */ 02080 if((hdfsdm_filter->State != HAL_DFSDM_FILTER_STATE_INJ) && \ 02081 (hdfsdm_filter->State != HAL_DFSDM_FILTER_STATE_REG_INJ)) 02082 { 02083 /* Return error status */ 02084 status = HAL_ERROR; 02085 } 02086 else 02087 { 02088 /* Stop current DMA transfer */ 02089 if(HAL_DMA_Abort(hdfsdm_filter->hdmaInj) != HAL_OK) 02090 { 02091 /* Set DFSDM filter in error state */ 02092 hdfsdm_filter->State = HAL_DFSDM_FILTER_STATE_ERROR; 02093 status = HAL_ERROR; 02094 } 02095 else 02096 { 02097 /* Stop regular conversion */ 02098 DFSDM_InjConvStop(hdfsdm_filter); 02099 } 02100 } 02101 /* Return function status */ 02102 return status; 02103 } 02104 02105 /** 02106 * @brief This function allows to get injected conversion value. 02107 * @param hdfsdm_filter : DFSDM filter handle. 02108 * @param Channel : Corresponding channel of injected conversion. 02109 * @retval Injected conversion value 02110 */ 02111 int32_t HAL_DFSDM_FilterGetInjectedValue(DFSDM_Filter_HandleTypeDef *hdfsdm_filter, 02112 uint32_t *Channel) 02113 { 02114 uint32_t reg = 0; 02115 int32_t value = 0; 02116 02117 /* Check parameters */ 02118 assert_param(IS_DFSDM_FILTER_ALL_INSTANCE(hdfsdm_filter->Instance)); 02119 assert_param(Channel != NULL); 02120 02121 /* Get value of data register for injected channel */ 02122 reg = hdfsdm_filter->Instance->JDATAR; 02123 02124 /* Extract channel and injected conversion value */ 02125 *Channel = (reg & DFSDM_JDATAR_JDATACH); 02126 value = ((int32_t)(reg & DFSDM_JDATAR_JDATA) >> DFSDM_JDATAR_DATA_OFFSET); 02127 02128 /* return regular conversion value */ 02129 return value; 02130 } 02131 02132 /** 02133 * @brief This function allows to start filter analog watchdog in interrupt mode. 02134 * @param hdfsdm_filter : DFSDM filter handle. 02135 * @param awdParam : DFSDM filter analog watchdog parameters. 02136 * @retval HAL status 02137 */ 02138 HAL_StatusTypeDef HAL_DFSDM_FilterAwdStart_IT(DFSDM_Filter_HandleTypeDef *hdfsdm_filter, 02139 DFSDM_Filter_AwdParamTypeDef *awdParam) 02140 { 02141 HAL_StatusTypeDef status = HAL_OK; 02142 02143 /* Check parameters */ 02144 assert_param(IS_DFSDM_FILTER_ALL_INSTANCE(hdfsdm_filter->Instance)); 02145 assert_param(IS_DFSDM_FILTER_AWD_DATA_SOURCE(awdParam->DataSource)); 02146 assert_param(IS_DFSDM_INJECTED_CHANNEL(awdParam->Channel)); 02147 assert_param(IS_DFSDM_FILTER_AWD_THRESHOLD(awdParam->HighThreshold)); 02148 assert_param(IS_DFSDM_FILTER_AWD_THRESHOLD(awdParam->LowThreshold)); 02149 assert_param(IS_DFSDM_BREAK_SIGNALS(awdParam->HighBreakSignal)); 02150 assert_param(IS_DFSDM_BREAK_SIGNALS(awdParam->LowBreakSignal)); 02151 02152 /* Check DFSDM filter state */ 02153 if((hdfsdm_filter->State == HAL_DFSDM_FILTER_STATE_RESET) || \ 02154 (hdfsdm_filter->State == HAL_DFSDM_FILTER_STATE_ERROR)) 02155 { 02156 /* Return error status */ 02157 status = HAL_ERROR; 02158 } 02159 else 02160 { 02161 /* Set analog watchdog data source */ 02162 hdfsdm_filter->Instance->CR1 &= ~(DFSDM_CR1_AWFSEL); 02163 hdfsdm_filter->Instance->CR1 |= awdParam->DataSource; 02164 02165 /* Set thresholds and break signals */ 02166 hdfsdm_filter->Instance->AWHTR &= ~(DFSDM_AWHTR_AWHT | DFSDM_AWHTR_BKAWH); 02167 hdfsdm_filter->Instance->AWHTR |= ((awdParam->HighThreshold << DFSDM_AWHTR_THRESHOLD_OFFSET) | \ 02168 awdParam->HighBreakSignal); 02169 hdfsdm_filter->Instance->AWLTR &= ~(DFSDM_AWLTR_AWLT | DFSDM_AWLTR_BKAWL); 02170 hdfsdm_filter->Instance->AWLTR |= ((awdParam->LowThreshold << DFSDM_AWLTR_THRESHOLD_OFFSET) | \ 02171 awdParam->LowBreakSignal); 02172 02173 /* Set channels and interrupt for analog watchdog */ 02174 hdfsdm_filter->Instance->CR2 &= ~(DFSDM_CR2_AWDCH); 02175 hdfsdm_filter->Instance->CR2 |= (((awdParam->Channel & DFSDM_LSB_MASK) << DFSDM_CR2_AWDCH_OFFSET) | \ 02176 DFSDM_CR2_AWDIE); 02177 } 02178 /* Return function status */ 02179 return status; 02180 } 02181 02182 /** 02183 * @brief This function allows to stop filter analog watchdog in interrupt mode. 02184 * @param hdfsdm_filter : DFSDM filter handle. 02185 * @retval HAL status 02186 */ 02187 HAL_StatusTypeDef HAL_DFSDM_FilterAwdStop_IT(DFSDM_Filter_HandleTypeDef *hdfsdm_filter) 02188 { 02189 HAL_StatusTypeDef status = HAL_OK; 02190 02191 /* Check parameters */ 02192 assert_param(IS_DFSDM_FILTER_ALL_INSTANCE(hdfsdm_filter->Instance)); 02193 02194 /* Check DFSDM filter state */ 02195 if((hdfsdm_filter->State == HAL_DFSDM_FILTER_STATE_RESET) || \ 02196 (hdfsdm_filter->State == HAL_DFSDM_FILTER_STATE_ERROR)) 02197 { 02198 /* Return error status */ 02199 status = HAL_ERROR; 02200 } 02201 else 02202 { 02203 /* Reset channels for analog watchdog and deactivate interrupt */ 02204 hdfsdm_filter->Instance->CR2 &= ~(DFSDM_CR2_AWDCH | DFSDM_CR2_AWDIE); 02205 02206 /* Clear all analog watchdog flags */ 02207 hdfsdm_filter->Instance->AWCFR = (DFSDM_AWCFR_CLRAWHTF | DFSDM_AWCFR_CLRAWLTF); 02208 02209 /* Reset thresholds and break signals */ 02210 hdfsdm_filter->Instance->AWHTR &= ~(DFSDM_AWHTR_AWHT | DFSDM_AWHTR_BKAWH); 02211 hdfsdm_filter->Instance->AWLTR &= ~(DFSDM_AWLTR_AWLT | DFSDM_AWLTR_BKAWL); 02212 02213 /* Reset analog watchdog data source */ 02214 hdfsdm_filter->Instance->CR1 &= ~(DFSDM_CR1_AWFSEL); 02215 } 02216 /* Return function status */ 02217 return status; 02218 } 02219 02220 /** 02221 * @brief This function allows to start extreme detector feature. 02222 * @param hdfsdm_filter : DFSDM filter handle. 02223 * @param Channel : Channels where extreme detector is enabled. 02224 * This parameter can be a values combination of @ref DFSDM_Channel_Selection. 02225 * @retval HAL status 02226 */ 02227 HAL_StatusTypeDef HAL_DFSDM_FilterExdStart(DFSDM_Filter_HandleTypeDef *hdfsdm_filter, 02228 uint32_t Channel) 02229 { 02230 HAL_StatusTypeDef status = HAL_OK; 02231 02232 /* Check parameters */ 02233 assert_param(IS_DFSDM_FILTER_ALL_INSTANCE(hdfsdm_filter->Instance)); 02234 assert_param(IS_DFSDM_INJECTED_CHANNEL(Channel)); 02235 02236 /* Check DFSDM filter state */ 02237 if((hdfsdm_filter->State == HAL_DFSDM_FILTER_STATE_RESET) || \ 02238 (hdfsdm_filter->State == HAL_DFSDM_FILTER_STATE_ERROR)) 02239 { 02240 /* Return error status */ 02241 status = HAL_ERROR; 02242 } 02243 else 02244 { 02245 /* Set channels for extreme detector */ 02246 hdfsdm_filter->Instance->CR2 &= ~(DFSDM_CR2_EXCH); 02247 hdfsdm_filter->Instance->CR2 |= ((Channel & DFSDM_LSB_MASK) << DFSDM_CR2_EXCH_OFFSET); 02248 } 02249 /* Return function status */ 02250 return status; 02251 } 02252 02253 /** 02254 * @brief This function allows to stop extreme detector feature. 02255 * @param hdfsdm_filter : DFSDM filter handle. 02256 * @retval HAL status 02257 */ 02258 HAL_StatusTypeDef HAL_DFSDM_FilterExdStop(DFSDM_Filter_HandleTypeDef *hdfsdm_filter) 02259 { 02260 HAL_StatusTypeDef status = HAL_OK; 02261 __IO uint32_t reg; 02262 02263 /* Check parameters */ 02264 assert_param(IS_DFSDM_FILTER_ALL_INSTANCE(hdfsdm_filter->Instance)); 02265 02266 /* Check DFSDM filter state */ 02267 if((hdfsdm_filter->State == HAL_DFSDM_FILTER_STATE_RESET) || \ 02268 (hdfsdm_filter->State == HAL_DFSDM_FILTER_STATE_ERROR)) 02269 { 02270 /* Return error status */ 02271 status = HAL_ERROR; 02272 } 02273 else 02274 { 02275 /* Reset channels for extreme detector */ 02276 hdfsdm_filter->Instance->CR2 &= ~(DFSDM_CR2_EXCH); 02277 02278 /* Clear extreme detector values */ 02279 reg = hdfsdm_filter->Instance->EXMAX; 02280 reg = hdfsdm_filter->Instance->EXMIN; 02281 UNUSED(reg); /* To avoid GCC warning */ 02282 } 02283 /* Return function status */ 02284 return status; 02285 } 02286 02287 /** 02288 * @brief This function allows to get extreme detector maximum value. 02289 * @param hdfsdm_filter : DFSDM filter handle. 02290 * @param Channel : Corresponding channel. 02291 * @retval Extreme detector maximum value 02292 * This value is between Min_Data = -8388608 and Max_Data = 8388607. 02293 */ 02294 int32_t HAL_DFSDM_FilterGetExdMaxValue(DFSDM_Filter_HandleTypeDef *hdfsdm_filter, 02295 uint32_t *Channel) 02296 { 02297 uint32_t reg = 0; 02298 int32_t value = 0; 02299 02300 /* Check parameters */ 02301 assert_param(IS_DFSDM_FILTER_ALL_INSTANCE(hdfsdm_filter->Instance)); 02302 assert_param(Channel != NULL); 02303 02304 /* Get value of extreme detector maximum register */ 02305 reg = hdfsdm_filter->Instance->EXMAX; 02306 02307 /* Extract channel and extreme detector maximum value */ 02308 *Channel = (reg & DFSDM_EXMAX_EXMAXCH); 02309 value = ((int32_t)(reg & DFSDM_EXMAX_EXMAX) >> DFSDM_EXMAX_DATA_OFFSET); 02310 02311 /* return extreme detector maximum value */ 02312 return value; 02313 } 02314 02315 /** 02316 * @brief This function allows to get extreme detector minimum value. 02317 * @param hdfsdm_filter : DFSDM filter handle. 02318 * @param Channel : Corresponding channel. 02319 * @retval Extreme detector minimum value 02320 * This value is between Min_Data = -8388608 and Max_Data = 8388607. 02321 */ 02322 int32_t HAL_DFSDM_FilterGetExdMinValue(DFSDM_Filter_HandleTypeDef *hdfsdm_filter, 02323 uint32_t *Channel) 02324 { 02325 uint32_t reg = 0; 02326 int32_t value = 0; 02327 02328 /* Check parameters */ 02329 assert_param(IS_DFSDM_FILTER_ALL_INSTANCE(hdfsdm_filter->Instance)); 02330 assert_param(Channel != NULL); 02331 02332 /* Get value of extreme detector minimum register */ 02333 reg = hdfsdm_filter->Instance->EXMIN; 02334 02335 /* Extract channel and extreme detector minimum value */ 02336 *Channel = (reg & DFSDM_EXMIN_EXMINCH); 02337 value = ((int32_t)(reg & DFSDM_EXMIN_EXMIN) >> DFSDM_EXMIN_DATA_OFFSET); 02338 02339 /* return extreme detector minimum value */ 02340 return value; 02341 } 02342 02343 /** 02344 * @brief This function allows to get conversion time value. 02345 * @param hdfsdm_filter : DFSDM filter handle. 02346 * @retval Conversion time value 02347 * @note To get time in second, this value has to be divided by DFSDM clock frequency. 02348 */ 02349 uint32_t HAL_DFSDM_FilterGetConvTimeValue(DFSDM_Filter_HandleTypeDef *hdfsdm_filter) 02350 { 02351 uint32_t reg = 0; 02352 uint32_t value = 0; 02353 02354 /* Check parameters */ 02355 assert_param(IS_DFSDM_FILTER_ALL_INSTANCE(hdfsdm_filter->Instance)); 02356 02357 /* Get value of conversion timer register */ 02358 reg = hdfsdm_filter->Instance->CNVTIMR; 02359 02360 /* Extract conversion time value */ 02361 value = ((reg & DFSDM_CNVTIMR_CNVCNT) >> DFSDM_CNVTIMR_DATA_OFFSET); 02362 02363 /* return extreme detector minimum value */ 02364 return value; 02365 } 02366 02367 /** 02368 * @brief This function handles the DFSDM interrupts. 02369 * @param hdfsdm_filter : DFSDM filter handle. 02370 * @retval None 02371 */ 02372 void HAL_DFSDM_IRQHandler(DFSDM_Filter_HandleTypeDef *hdfsdm_filter) 02373 { 02374 /* Check if overrun occurs during regular conversion */ 02375 if(((hdfsdm_filter->Instance->ISR & DFSDM_ISR_ROVRF) != 0) && \ 02376 ((hdfsdm_filter->Instance->CR2 & DFSDM_CR2_ROVRIE) != 0)) 02377 { 02378 /* Clear regular overrun flag */ 02379 hdfsdm_filter->Instance->ICR = DFSDM_ICR_CLRROVRF; 02380 02381 /* Update error code */ 02382 hdfsdm_filter->ErrorCode = DFSDM_FILTER_ERROR_REGULAR_OVERRUN; 02383 02384 /* Call error callback */ 02385 HAL_DFSDM_FilterErrorCallback(hdfsdm_filter); 02386 } 02387 /* Check if overrun occurs during injected conversion */ 02388 else if(((hdfsdm_filter->Instance->ISR & DFSDM_ISR_JOVRF) != 0) && \ 02389 ((hdfsdm_filter->Instance->CR2 & DFSDM_CR2_JOVRIE) != 0)) 02390 { 02391 /* Clear injected overrun flag */ 02392 hdfsdm_filter->Instance->ICR = DFSDM_ICR_CLRJOVRF; 02393 02394 /* Update error code */ 02395 hdfsdm_filter->ErrorCode = DFSDM_FILTER_ERROR_INJECTED_OVERRUN; 02396 02397 /* Call error callback */ 02398 HAL_DFSDM_FilterErrorCallback(hdfsdm_filter); 02399 } 02400 /* Check if end of regular conversion */ 02401 else if(((hdfsdm_filter->Instance->ISR & DFSDM_ISR_REOCF) != 0) && \ 02402 ((hdfsdm_filter->Instance->CR2 & DFSDM_CR2_REOCIE) != 0)) 02403 { 02404 /* Call regular conversion complete callback */ 02405 HAL_DFSDM_FilterRegConvCpltCallback(hdfsdm_filter); 02406 02407 /* End of conversion if mode is not continuous and software trigger */ 02408 if((hdfsdm_filter->RegularContMode == DFSDM_CONTINUOUS_CONV_OFF) && \ 02409 (hdfsdm_filter->RegularTrigger == DFSDM_FILTER_SW_TRIGGER)) 02410 { 02411 /* Disable interrupts for regular conversions */ 02412 hdfsdm_filter->Instance->CR2 &= ~(DFSDM_CR2_REOCIE); 02413 02414 /* Update DFSDM filter state */ 02415 hdfsdm_filter->State = (hdfsdm_filter->State == HAL_DFSDM_FILTER_STATE_REG) ? \ 02416 HAL_DFSDM_FILTER_STATE_READY : HAL_DFSDM_FILTER_STATE_INJ; 02417 } 02418 } 02419 /* Check if end of injected conversion */ 02420 else if(((hdfsdm_filter->Instance->ISR & DFSDM_ISR_JEOCF) != 0) && \ 02421 ((hdfsdm_filter->Instance->CR2 & DFSDM_CR2_JEOCIE) != 0)) 02422 { 02423 /* Call injected conversion complete callback */ 02424 HAL_DFSDM_FilterInjConvCpltCallback(hdfsdm_filter); 02425 02426 /* Update remaining injected conversions */ 02427 hdfsdm_filter->InjConvRemaining--; 02428 if(hdfsdm_filter->InjConvRemaining == 0) 02429 { 02430 /* End of conversion if trigger is software */ 02431 if(hdfsdm_filter->InjectedTrigger == DFSDM_FILTER_SW_TRIGGER) 02432 { 02433 /* Disable interrupts for injected conversions */ 02434 hdfsdm_filter->Instance->CR2 &= ~(DFSDM_CR2_JEOCIE); 02435 02436 /* Update DFSDM filter state */ 02437 hdfsdm_filter->State = (hdfsdm_filter->State == HAL_DFSDM_FILTER_STATE_INJ) ? \ 02438 HAL_DFSDM_FILTER_STATE_READY : HAL_DFSDM_FILTER_STATE_REG; 02439 } 02440 /* end of injected sequence, reset the value */ 02441 hdfsdm_filter->InjConvRemaining = (hdfsdm_filter->InjectedScanMode == ENABLE) ? \ 02442 hdfsdm_filter->InjectedChannelsNbr : 1; 02443 } 02444 } 02445 /* Check if analog watchdog occurs */ 02446 else if(((hdfsdm_filter->Instance->ISR & DFSDM_ISR_AWDF) != 0) && \ 02447 ((hdfsdm_filter->Instance->CR2 & DFSDM_CR2_AWDIE) != 0)) 02448 { 02449 uint32_t reg = 0; 02450 uint32_t threshold = 0; 02451 uint32_t channel = 0; 02452 02453 /* Get channel and threshold */ 02454 reg = hdfsdm_filter->Instance->AWSR; 02455 threshold = (reg & DFSDM_AWSR_AWLTF) ? DFSDM_AWD_LOW_THRESHOLD : DFSDM_AWD_HIGH_THRESHOLD; 02456 if(threshold == DFSDM_AWD_HIGH_THRESHOLD) 02457 { 02458 reg = reg >> DFSDM_AWSR_HIGH_OFFSET; 02459 } 02460 while((reg & 1) == 0) 02461 { 02462 channel++; 02463 reg = reg >> 1; 02464 } 02465 /* Clear analog watchdog flag */ 02466 hdfsdm_filter->Instance->AWCFR = (threshold == DFSDM_AWD_HIGH_THRESHOLD) ? \ 02467 (1 << (DFSDM_AWSR_HIGH_OFFSET + channel)) : \ 02468 (1 << channel); 02469 02470 /* Call analog watchdog callback */ 02471 HAL_DFSDM_FilterAwdCallback(hdfsdm_filter, channel, threshold); 02472 } 02473 /* Check if clock absence occurs */ 02474 else if((hdfsdm_filter->Instance == DFSDM_Filter0) && \ 02475 ((hdfsdm_filter->Instance->ISR & DFSDM_ISR_CKABF) != 0) && \ 02476 ((hdfsdm_filter->Instance->CR2 & DFSDM_CR2_CKABIE) != 0)) 02477 { 02478 uint32_t reg = 0; 02479 uint32_t channel = 0; 02480 02481 reg = ((hdfsdm_filter->Instance->ISR & DFSDM_ISR_CKABF) >> DFSDM_ISR_CKABF_OFFSET); 02482 02483 while(channel < DFSDM_CHANNEL_NUMBER) 02484 { 02485 /* Check if flag is set and corresponding channel is enabled */ 02486 if((reg & 1) && (a_dfsdmChannelHandle[channel] != NULL)) 02487 { 02488 /* Check clock absence has been enabled for this channel */ 02489 if((a_dfsdmChannelHandle[channel]->Instance->CHCFGR1 & DFSDM_CHCFGR1_CKABEN) != 0) 02490 { 02491 /* Clear clock absence flag */ 02492 hdfsdm_filter->Instance->ICR = (1 << (DFSDM_ICR_CLRCKABF_OFFSET + channel)); 02493 02494 /* Call clock absence callback */ 02495 HAL_DFSDM_ChannelCkabCallback(a_dfsdmChannelHandle[channel]); 02496 } 02497 } 02498 channel++; 02499 reg = reg >> 1; 02500 } 02501 } 02502 /* Check if short circuit detection occurs */ 02503 else if((hdfsdm_filter->Instance == DFSDM_Filter0) && \ 02504 ((hdfsdm_filter->Instance->ISR & DFSDM_ISR_SCDF) != 0) && \ 02505 ((hdfsdm_filter->Instance->CR2 & DFSDM_CR2_SCDIE) != 0)) 02506 { 02507 uint32_t reg = 0; 02508 uint32_t channel = 0; 02509 02510 /* Get channel */ 02511 reg = ((hdfsdm_filter->Instance->ISR & DFSDM_ISR_SCDF) >> DFSDM_ISR_SCDF_OFFSET); 02512 while((reg & 1) == 0) 02513 { 02514 channel++; 02515 reg = reg >> 1; 02516 } 02517 02518 /* Clear short circuit detection flag */ 02519 hdfsdm_filter->Instance->ICR = (1 << (DFSDM_ICR_CLRSCDF_OFFSET + channel)); 02520 02521 /* Call short circuit detection callback */ 02522 HAL_DFSDM_ChannelScdCallback(a_dfsdmChannelHandle[channel]); 02523 } 02524 } 02525 02526 /** 02527 * @brief Regular conversion complete callback. 02528 * @note In interrupt mode, user has to read conversion value in this function 02529 * using HAL_DFSDM_FilterGetRegularValue. 02530 * @param hdfsdm_filter : DFSDM filter handle. 02531 * @retval None 02532 */ 02533 __weak void HAL_DFSDM_FilterRegConvCpltCallback(DFSDM_Filter_HandleTypeDef *hdfsdm_filter) 02534 { 02535 /* NOTE : This function should not be modified, when the callback is needed, 02536 the HAL_DFSDM_FilterRegConvCpltCallback could be implemented in the user file. 02537 */ 02538 } 02539 02540 /** 02541 * @brief Half regular conversion complete callback. 02542 * @param hdfsdm_filter : DFSDM filter handle. 02543 * @retval None 02544 */ 02545 __weak void HAL_DFSDM_FilterRegConvHalfCpltCallback(DFSDM_Filter_HandleTypeDef *hdfsdm_filter) 02546 { 02547 /* NOTE : This function should not be modified, when the callback is needed, 02548 the HAL_DFSDM_FilterRegConvHalfCpltCallback could be implemented in the user file. 02549 */ 02550 } 02551 02552 /** 02553 * @brief Injected conversion complete callback. 02554 * @note In interrupt mode, user has to read conversion value in this function 02555 * using HAL_DFSDM_FilterGetInjectedValue. 02556 * @param hdfsdm_filter : DFSDM filter handle. 02557 * @retval None 02558 */ 02559 __weak void HAL_DFSDM_FilterInjConvCpltCallback(DFSDM_Filter_HandleTypeDef *hdfsdm_filter) 02560 { 02561 /* NOTE : This function should not be modified, when the callback is needed, 02562 the HAL_DFSDM_FilterInjConvCpltCallback could be implemented in the user file. 02563 */ 02564 } 02565 02566 /** 02567 * @brief Half injected conversion complete callback. 02568 * @param hdfsdm_filter : DFSDM filter handle. 02569 * @retval None 02570 */ 02571 __weak void HAL_DFSDM_FilterInjConvHalfCpltCallback(DFSDM_Filter_HandleTypeDef *hdfsdm_filter) 02572 { 02573 /* NOTE : This function should not be modified, when the callback is needed, 02574 the HAL_DFSDM_FilterInjConvHalfCpltCallback could be implemented in the user file. 02575 */ 02576 } 02577 02578 /** 02579 * @brief Filter analog watchdog callback. 02580 * @param hdfsdm_filter : DFSDM filter handle. 02581 * @param Channel : Corresponding channel. 02582 * @param Threshold : Low or high threshold has been reached. 02583 * @retval None 02584 */ 02585 __weak void HAL_DFSDM_FilterAwdCallback(DFSDM_Filter_HandleTypeDef *hdfsdm_filter, 02586 uint32_t Channel, uint32_t Threshold) 02587 { 02588 /* NOTE : This function should not be modified, when the callback is needed, 02589 the HAL_DFSDM_FilterAwdCallback could be implemented in the user file. 02590 */ 02591 } 02592 02593 /** 02594 * @brief Error callback. 02595 * @param hdfsdm_filter : DFSDM filter handle. 02596 * @retval None 02597 */ 02598 __weak void HAL_DFSDM_FilterErrorCallback(DFSDM_Filter_HandleTypeDef *hdfsdm_filter) 02599 { 02600 /* NOTE : This function should not be modified, when the callback is needed, 02601 the HAL_DFSDM_FilterErrorCallback could be implemented in the user file. 02602 */ 02603 } 02604 02605 /** 02606 * @} 02607 */ 02608 02609 /** @defgroup DFSDM_Exported_Functions_Group4_Filter Filter state functions 02610 * @brief Filter state functions 02611 * 02612 @verbatim 02613 ============================================================================== 02614 ##### Filter state functions ##### 02615 ============================================================================== 02616 [..] This section provides functions allowing to: 02617 (+) Get the DFSDM filter state. 02618 (+) Get the DFSDM filter error. 02619 @endverbatim 02620 * @{ 02621 */ 02622 02623 /** 02624 * @brief This function allows to get the current DFSDM filter handle state. 02625 * @param hdfsdm_filter : DFSDM filter handle. 02626 * @retval DFSDM filter state. 02627 */ 02628 HAL_DFSDM_Filter_StateTypeDef HAL_DFSDM_FilterGetState(DFSDM_Filter_HandleTypeDef *hdfsdm_filter) 02629 { 02630 /* Return DFSDM filter handle state */ 02631 return hdfsdm_filter->State; 02632 } 02633 02634 /** 02635 * @brief This function allows to get the current DFSDM filter error. 02636 * @param hdfsdm_filter : DFSDM filter handle. 02637 * @retval DFSDM filter error code. 02638 */ 02639 uint32_t HAL_DFSDM_FilterGetError(DFSDM_Filter_HandleTypeDef *hdfsdm_filter) 02640 { 02641 return hdfsdm_filter->ErrorCode; 02642 } 02643 02644 /** 02645 * @} 02646 */ 02647 02648 /** 02649 * @} 02650 */ 02651 /* End of exported functions -------------------------------------------------*/ 02652 02653 /* Private functions ---------------------------------------------------------*/ 02654 /** @addtogroup DFSDM_Private_Functions DFSDM Private Functions 02655 * @{ 02656 */ 02657 02658 /** 02659 * @brief DMA half transfer complete callback for regular conversion. 02660 * @param hdma : DMA handle. 02661 * @retval None 02662 */ 02663 static void DFSDM_DMARegularHalfConvCplt(DMA_HandleTypeDef *hdma) 02664 { 02665 /* Get DFSDM filter handle */ 02666 DFSDM_Filter_HandleTypeDef* hdfsdm_filter = (DFSDM_Filter_HandleTypeDef*) ((DMA_HandleTypeDef*)hdma)->Parent; 02667 02668 /* Call regular half conversion complete callback */ 02669 HAL_DFSDM_FilterRegConvHalfCpltCallback(hdfsdm_filter); 02670 } 02671 02672 /** 02673 * @brief DMA transfer complete callback for regular conversion. 02674 * @param hdma : DMA handle. 02675 * @retval None 02676 */ 02677 static void DFSDM_DMARegularConvCplt(DMA_HandleTypeDef *hdma) 02678 { 02679 /* Get DFSDM filter handle */ 02680 DFSDM_Filter_HandleTypeDef* hdfsdm_filter = (DFSDM_Filter_HandleTypeDef*) ((DMA_HandleTypeDef*)hdma)->Parent; 02681 02682 /* Call regular conversion complete callback */ 02683 HAL_DFSDM_FilterRegConvCpltCallback(hdfsdm_filter); 02684 } 02685 02686 /** 02687 * @brief DMA half transfer complete callback for injected conversion. 02688 * @param hdma : DMA handle. 02689 * @retval None 02690 */ 02691 static void DFSDM_DMAInjectedHalfConvCplt(DMA_HandleTypeDef *hdma) 02692 { 02693 /* Get DFSDM filter handle */ 02694 DFSDM_Filter_HandleTypeDef* hdfsdm_filter = (DFSDM_Filter_HandleTypeDef*) ((DMA_HandleTypeDef*)hdma)->Parent; 02695 02696 /* Call injected half conversion complete callback */ 02697 HAL_DFSDM_FilterInjConvHalfCpltCallback(hdfsdm_filter); 02698 } 02699 02700 /** 02701 * @brief DMA transfer complete callback for injected conversion. 02702 * @param hdma : DMA handle. 02703 * @retval None 02704 */ 02705 static void DFSDM_DMAInjectedConvCplt(DMA_HandleTypeDef *hdma) 02706 { 02707 /* Get DFSDM filter handle */ 02708 DFSDM_Filter_HandleTypeDef* hdfsdm_filter = (DFSDM_Filter_HandleTypeDef*) ((DMA_HandleTypeDef*)hdma)->Parent; 02709 02710 /* Call injected conversion complete callback */ 02711 HAL_DFSDM_FilterInjConvCpltCallback(hdfsdm_filter); 02712 } 02713 02714 /** 02715 * @brief DMA error callback. 02716 * @param hdma : DMA handle. 02717 * @retval None 02718 */ 02719 static void DFSDM_DMAError(DMA_HandleTypeDef *hdma) 02720 { 02721 /* Get DFSDM filter handle */ 02722 DFSDM_Filter_HandleTypeDef* hdfsdm_filter = (DFSDM_Filter_HandleTypeDef*) ((DMA_HandleTypeDef*)hdma)->Parent; 02723 02724 /* Update error code */ 02725 hdfsdm_filter->ErrorCode = DFSDM_FILTER_ERROR_DMA; 02726 02727 /* Call error callback */ 02728 HAL_DFSDM_FilterErrorCallback(hdfsdm_filter); 02729 } 02730 02731 /** 02732 * @brief This function allows to get the number of injected channels. 02733 * @param Channels : bitfield of injected channels. 02734 * @retval Number of injected channels. 02735 */ 02736 static uint32_t DFSDM_GetInjChannelsNbr(uint32_t Channels) 02737 { 02738 uint32_t nbChannels = 0; 02739 uint32_t tmp; 02740 02741 /* Get the number of channels from bitfield */ 02742 tmp = (uint32_t) (Channels & DFSDM_LSB_MASK); 02743 while(tmp != 0) 02744 { 02745 if(tmp & 1) 02746 { 02747 nbChannels++; 02748 } 02749 tmp = (uint32_t) (tmp >> 1); 02750 } 02751 return nbChannels; 02752 } 02753 02754 /** 02755 * @brief This function allows to get the channel number from channel instance. 02756 * @param Instance : DFSDM channel instance. 02757 * @retval Channel number. 02758 */ 02759 static uint32_t DFSDM_GetChannelFromInstance(DFSDM_Channel_TypeDef* Instance) 02760 { 02761 uint32_t channel = 0; 02762 02763 /* Get channel from instance */ 02764 if(Instance == DFSDM_Channel0) 02765 { 02766 channel = 0; 02767 } 02768 else if(Instance == DFSDM_Channel1) 02769 { 02770 channel = 1; 02771 } 02772 else if(Instance == DFSDM_Channel2) 02773 { 02774 channel = 2; 02775 } 02776 else if(Instance == DFSDM_Channel3) 02777 { 02778 channel = 3; 02779 } 02780 else if(Instance == DFSDM_Channel4) 02781 { 02782 channel = 4; 02783 } 02784 else if(Instance == DFSDM_Channel5) 02785 { 02786 channel = 5; 02787 } 02788 else if(Instance == DFSDM_Channel6) 02789 { 02790 channel = 6; 02791 } 02792 else if(Instance == DFSDM_Channel7) 02793 { 02794 channel = 7; 02795 } 02796 02797 return channel; 02798 } 02799 02800 /** 02801 * @brief This function allows to really start regular conversion. 02802 * @param hdfsdm_filter : DFSDM filter handle. 02803 * @retval None 02804 */ 02805 static void DFSDM_RegConvStart(DFSDM_Filter_HandleTypeDef* hdfsdm_filter) 02806 { 02807 /* Check regular trigger */ 02808 if(hdfsdm_filter->RegularTrigger == DFSDM_FILTER_SW_TRIGGER) 02809 { 02810 /* Software start of regular conversion */ 02811 hdfsdm_filter->Instance->CR1 |= DFSDM_CR1_RSWSTART; 02812 } 02813 else /* synchronous trigger */ 02814 { 02815 /* Disable DFSDM filter */ 02816 hdfsdm_filter->Instance->CR1 &= ~(DFSDM_CR1_DFEN); 02817 02818 /* Set RSYNC bit in DFSDM_CR1 register */ 02819 hdfsdm_filter->Instance->CR1 |= DFSDM_CR1_RSYNC; 02820 02821 /* Enable DFSDM filter */ 02822 hdfsdm_filter->Instance->CR1 |= DFSDM_CR1_DFEN; 02823 02824 /* If injected conversion was in progress, restart it */ 02825 if(hdfsdm_filter->State == HAL_DFSDM_FILTER_STATE_INJ) 02826 { 02827 if(hdfsdm_filter->InjectedTrigger == DFSDM_FILTER_SW_TRIGGER) 02828 { 02829 hdfsdm_filter->Instance->CR1 |= DFSDM_CR1_JSWSTART; 02830 } 02831 /* Update remaining injected conversions */ 02832 hdfsdm_filter->InjConvRemaining = (hdfsdm_filter->InjectedScanMode == ENABLE) ? \ 02833 hdfsdm_filter->InjectedChannelsNbr : 1; 02834 } 02835 } 02836 /* Update DFSDM filter state */ 02837 hdfsdm_filter->State = (hdfsdm_filter->State == HAL_DFSDM_FILTER_STATE_READY) ? \ 02838 HAL_DFSDM_FILTER_STATE_REG : HAL_DFSDM_FILTER_STATE_REG_INJ; 02839 } 02840 02841 /** 02842 * @brief This function allows to really stop regular conversion. 02843 * @param hdfsdm_filter : DFSDM filter handle. 02844 * @retval None 02845 */ 02846 static void DFSDM_RegConvStop(DFSDM_Filter_HandleTypeDef* hdfsdm_filter) 02847 { 02848 /* Disable DFSDM filter */ 02849 hdfsdm_filter->Instance->CR1 &= ~(DFSDM_CR1_DFEN); 02850 02851 /* If regular trigger was synchronous, reset RSYNC bit in DFSDM_CR1 register */ 02852 if(hdfsdm_filter->RegularTrigger == DFSDM_FILTER_SYNC_TRIGGER) 02853 { 02854 hdfsdm_filter->Instance->CR1 &= ~(DFSDM_CR1_RSYNC); 02855 } 02856 02857 /* Enable DFSDM filter */ 02858 hdfsdm_filter->Instance->CR1 |= DFSDM_CR1_DFEN; 02859 02860 /* If injected conversion was in progress, restart it */ 02861 if(hdfsdm_filter->State == HAL_DFSDM_FILTER_STATE_REG_INJ) 02862 { 02863 if(hdfsdm_filter->InjectedTrigger == DFSDM_FILTER_SW_TRIGGER) 02864 { 02865 hdfsdm_filter->Instance->CR1 |= DFSDM_CR1_JSWSTART; 02866 } 02867 /* Update remaining injected conversions */ 02868 hdfsdm_filter->InjConvRemaining = (hdfsdm_filter->InjectedScanMode == ENABLE) ? \ 02869 hdfsdm_filter->InjectedChannelsNbr : 1; 02870 } 02871 02872 /* Update DFSDM filter state */ 02873 hdfsdm_filter->State = (hdfsdm_filter->State == HAL_DFSDM_FILTER_STATE_REG) ? \ 02874 HAL_DFSDM_FILTER_STATE_READY : HAL_DFSDM_FILTER_STATE_INJ; 02875 } 02876 02877 /** 02878 * @brief This function allows to really start injected conversion. 02879 * @param hdfsdm_filter : DFSDM filter handle. 02880 * @retval None 02881 */ 02882 static void DFSDM_InjConvStart(DFSDM_Filter_HandleTypeDef* hdfsdm_filter) 02883 { 02884 /* Check injected trigger */ 02885 if(hdfsdm_filter->InjectedTrigger == DFSDM_FILTER_SW_TRIGGER) 02886 { 02887 /* Software start of injected conversion */ 02888 hdfsdm_filter->Instance->CR1 |= DFSDM_CR1_JSWSTART; 02889 } 02890 else /* external or synchronous trigger */ 02891 { 02892 /* Disable DFSDM filter */ 02893 hdfsdm_filter->Instance->CR1 &= ~(DFSDM_CR1_DFEN); 02894 02895 if(hdfsdm_filter->InjectedTrigger == DFSDM_FILTER_SYNC_TRIGGER) 02896 { 02897 /* Set JSYNC bit in DFSDM_CR1 register */ 02898 hdfsdm_filter->Instance->CR1 |= DFSDM_CR1_JSYNC; 02899 } 02900 else /* external trigger */ 02901 { 02902 /* Set JEXTEN[1:0] bits in DFSDM_CR1 register */ 02903 hdfsdm_filter->Instance->CR1 |= hdfsdm_filter->ExtTriggerEdge; 02904 } 02905 02906 /* Enable DFSDM filter */ 02907 hdfsdm_filter->Instance->CR1 |= DFSDM_CR1_DFEN; 02908 02909 /* If regular conversion was in progress, restart it */ 02910 if((hdfsdm_filter->State == HAL_DFSDM_FILTER_STATE_REG) && \ 02911 (hdfsdm_filter->RegularTrigger == DFSDM_FILTER_SW_TRIGGER)) 02912 { 02913 hdfsdm_filter->Instance->CR1 |= DFSDM_CR1_RSWSTART; 02914 } 02915 } 02916 /* Update DFSDM filter state */ 02917 hdfsdm_filter->State = (hdfsdm_filter->State == HAL_DFSDM_FILTER_STATE_READY) ? \ 02918 HAL_DFSDM_FILTER_STATE_INJ : HAL_DFSDM_FILTER_STATE_REG_INJ; 02919 } 02920 02921 /** 02922 * @brief This function allows to really stop injected conversion. 02923 * @param hdfsdm_filter : DFSDM filter handle. 02924 * @retval None 02925 */ 02926 static void DFSDM_InjConvStop(DFSDM_Filter_HandleTypeDef* hdfsdm_filter) 02927 { 02928 /* Disable DFSDM filter */ 02929 hdfsdm_filter->Instance->CR1 &= ~(DFSDM_CR1_DFEN); 02930 02931 /* If injected trigger was synchronous, reset JSYNC bit in DFSDM_CR1 register */ 02932 if(hdfsdm_filter->InjectedTrigger == DFSDM_FILTER_SYNC_TRIGGER) 02933 { 02934 hdfsdm_filter->Instance->CR1 &= ~(DFSDM_CR1_JSYNC); 02935 } 02936 else if(hdfsdm_filter->InjectedTrigger == DFSDM_FILTER_EXT_TRIGGER) 02937 { 02938 /* Reset JEXTEN[1:0] bits in DFSDM_CR1 register */ 02939 hdfsdm_filter->Instance->CR1 &= ~(DFSDM_CR1_JEXTEN); 02940 } 02941 02942 /* Enable DFSDM filter */ 02943 hdfsdm_filter->Instance->CR1 |= DFSDM_CR1_DFEN; 02944 02945 /* If regular conversion was in progress, restart it */ 02946 if((hdfsdm_filter->State == HAL_DFSDM_FILTER_STATE_REG_INJ) && \ 02947 (hdfsdm_filter->RegularTrigger == DFSDM_FILTER_SW_TRIGGER)) 02948 { 02949 hdfsdm_filter->Instance->CR1 |= DFSDM_CR1_RSWSTART; 02950 } 02951 02952 /* Update remaining injected conversions */ 02953 hdfsdm_filter->InjConvRemaining = (hdfsdm_filter->InjectedScanMode == ENABLE) ? \ 02954 hdfsdm_filter->InjectedChannelsNbr : 1; 02955 02956 /* Update DFSDM filter state */ 02957 hdfsdm_filter->State = (hdfsdm_filter->State == HAL_DFSDM_FILTER_STATE_INJ) ? \ 02958 HAL_DFSDM_FILTER_STATE_READY : HAL_DFSDM_FILTER_STATE_REG; 02959 } 02960 02961 /** 02962 * @} 02963 */ 02964 /* End of private functions --------------------------------------------------*/ 02965 02966 /** 02967 * @} 02968 */ 02969 #endif /* HAL_DFSDM_MODULE_ENABLED */ 02970 /** 02971 * @} 02972 */ 02973 02974 /************************ (C) COPYRIGHT STMicroelectronics *****END OF FILE****/ 02975
Generated on Tue Jul 12 2022 11:35:08 by
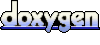