
Example program using the ESPAT library. Made for BULME Bertl 2017.
Embed:
(wiki syntax)
Show/hide line numbers
main.cpp
00001 #include "mbed.h" 00002 #include "ESPAT.h" 00003 00004 /* 00005 Example program using the ESPAT library. 00006 Made for BULME Bertl 2017 (LPC11U68) 00007 Opens an Access Point called ESP with the password 12345678 00008 # Features: 00009 - Led D10 turns on when ready (wifi connected + server listening) 00010 - Navigate to the device's ip in your browser and use the buttons to control Led D11 00011 (C)2019 Elias Nestl 00012 */ 00013 00014 ESPAT esp(P1_0, P0_20, 115200); // Library initialisieren 00015 00016 DigitalOut Led0 (P1_18); // D10 00017 DigitalOut Led1 (P2_16); // D11 00018 00019 string htmlPage = "<html>" 00020 "<head><title>Led toggler</title></head>" 00021 "<body>" 00022 "<button onclick=\"fetch('./ledOn')\">Led ON</button>" 00023 "<button onclick=\"fetch('./ledOff')\">Led OFF</button>" 00024 "</body></html>"; // Website code 00025 00026 void handleRequest(int linkId, string path) { // Bei HTTP Request 00027 if (path == "/") { // Bei direkter IP 00028 esp.httpReply(linkId, "200 OK", htmlPage); // Website senden 00029 } else if (path == "/ledOn") { // Bei Pfad ledOn/ledOff, Led ein bzw ausschalten 00030 Led1 = 0; 00031 esp.httpReply(linkId, "200 OK", "success"); 00032 } else if (path == "/ledOff") { 00033 Led1 = 1; 00034 esp.httpReply(linkId, "200 OK", "success"); 00035 } else { // Bei unbekannter Seite 404 antworten 00036 esp.httpReply(linkId, "404 Not Found", "404 Not found!"); 00037 } 00038 } 00039 00040 int main() { 00041 Led0 = Led1 = 1; // Leds aus 00042 esp.resetEsp(); // ESP Reset 00043 esp.initWifiAP("ESP", "12345678"); // ESP Access Point Initialiseren 00044 Led0 = 0; // LED ein um zu zeigen, dass Bertl aktiv ist 00045 esp.initServer(handleRequest); // Request Handler initialisieren 00046 }
Generated on Tue Jul 19 2022 19:16:03 by
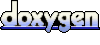