This is a simple library for the STMPE610 touchscreen controller used by the Adafruit 2.8" TFT LCD shield
SPI_STMPE610.h
00001 //******************************************************************************************** 00002 // * 00003 // This software is distributed as an example, "AS IS", in the hope that it could * 00004 // be useful, WITHOUT ANY WARRANTY of any kind, express or implied, included, but * 00005 // not limited, to the warranties of merchantability, fitness for a particular * 00006 // purpose, and non infringiment. In no event shall the authors be liable for any * 00007 // claim, damages or other liability, arising from, or in connection with this software. * 00008 // * 00009 //********************************************************************************************/ 00010 00011 00012 00013 #ifndef SPI_STMPE610_H 00014 #define SPI_STMPE610_H 00015 00016 #include "mbed.h" 00017 00018 00019 class SPI_STMPE610 00020 { 00021 00022 public: 00023 00024 SPI_STMPE610(PinName mosi, PinName miso, PinName sclk, PinName cs); 00025 00026 bool GetPoint(uint16_t *X, uint16_t *Y); 00027 00028 00029 private: 00030 00031 SPI m_spi; 00032 DigitalOut m_cs; 00033 00034 bool GetRawPoint(uint16_t *X, uint16_t *Y); 00035 uint8_t Read8(uint8_t Addr); 00036 uint16_t Read16(uint8_t Addr); 00037 void Write8(uint8_t Addr, uint8_t Data); 00038 00039 }; 00040 00041 #endif /* SPI_STMPE610_H */
Generated on Sun Jul 31 2022 19:14:05 by
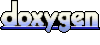