my customized lib
Embed:
(wiki syntax)
Show/hide line numbers
easy-connect.h
00001 #ifndef __MAGIC_CONNECT_H__ 00002 #define __MAGIC_CONNECT_H__ 00003 00004 #include "mbed.h" 00005 00006 Serial output(USBTX, USBRX); 00007 00008 #define ETHERNET 1 00009 #define WIFI_ESP8266 2 00010 #define MESH_LOWPAN_ND 3 00011 #define MESH_THREAD 4 00012 00013 #if MBED_CONF_APP_NETWORK_INTERFACE == WIFI_ESP8266 00014 #include "ESP8266Interface.h" 00015 00016 #ifdef MBED_CONF_APP_ESP8266_DEBUG 00017 ESP8266Interface esp(MBED_CONF_APP_ESP8266_TX, MBED_CONF_APP_ESP8266_RX, MBED_CONF_APP_ESP8266_DEBUG); 00018 #else 00019 ESP8266Interface esp(MBED_CONF_APP_ESP8266_TX, MBED_CONF_APP_ESP8266_RX); 00020 #endif 00021 00022 #elif MBED_CONF_APP_NETWORK_INTERFACE == ETHERNET 00023 #include "EthernetInterface.h" 00024 EthernetInterface eth; 00025 #elif MBED_CONF_APP_NETWORK_INTERFACE == MESH_LOWPAN_ND 00026 #define MESH 00027 #include "NanostackInterface.h" 00028 LoWPANNDInterface mesh; 00029 #elif MBED_CONF_APP_NETWORK_INTERFACE == MESH_THREAD 00030 #define MESH 00031 #include "NanostackInterface.h" 00032 ThreadInterface mesh; 00033 #else 00034 #error "No connectivity method chosen. Please add 'config.network-interfaces.value' to your mbed_app.json (see README.md for more information)." 00035 #endif 00036 00037 #if defined(MESH) 00038 #if MBED_CONF_APP_MESH_RADIO_TYPE == ATMEL 00039 #include "NanostackRfPhyAtmel.h" 00040 NanostackRfPhyAtmel rf_phy(ATMEL_SPI_MOSI, ATMEL_SPI_MISO, ATMEL_SPI_SCLK, ATMEL_SPI_CS, 00041 ATMEL_SPI_RST, ATMEL_SPI_SLP, ATMEL_SPI_IRQ, ATMEL_I2C_SDA, ATMEL_I2C_SCL); 00042 #elif MBED_CONF_APP_MESH_RADIO_TYPE == MCR20 00043 #include "NanostackRfPhyMcr20a.h" 00044 NanostackRfPhyMcr20a rf_phy(MCR20A_SPI_MOSI, MCR20A_SPI_MISO, MCR20A_SPI_SCLK, MCR20A_SPI_CS, MCR20A_SPI_RST, MCR20A_SPI_IRQ); 00045 #endif //MBED_CONF_APP_RADIO_TYPE 00046 #endif //MESH 00047 00048 #ifndef MESH 00049 // This is address to mbed Device Connector 00050 #define MBED_SERVER_ADDRESS "coap://api.connector.mbed.com:5684" 00051 #else 00052 // This is address to mbed Device Connector 00053 #define MBED_SERVER_ADDRESS "coaps://[2607:f0d0:2601:52::20]:5684" 00054 #endif 00055 00056 NetworkInterface* easy_connect(bool log_messages = false) { 00057 NetworkInterface* network_interface = 0; 00058 int connect_success = -1; 00059 #if MBED_CONF_APP_NETWORK_INTERFACE == WIFI_ESP8266 00060 if (log_messages) { 00061 output.printf("[EasyConnect] Using WiFi (ESP8266) \r\n"); 00062 output.printf("[EasyConnect] Connecting to WiFi..\r\n"); 00063 } 00064 connect_success = esp.connect(MBED_CONF_APP_ESP8266_SSID, MBED_CONF_APP_ESP8266_PASSWORD); 00065 network_interface = &esp; 00066 #elif MBED_CONF_APP_NETWORK_INTERFACE == ETHERNET 00067 if (log_messages) { 00068 output.printf("[EasyConnect] Using Ethernet\r\n"); 00069 } 00070 connect_success = eth.connect(); 00071 network_interface = ð 00072 #endif 00073 #ifdef MESH 00074 if (log_messages) { 00075 output.printf("[EasyConnect] Using Mesh\r\n"); 00076 output.printf("[EasyConnect] Connecting to Mesh..\r\n"); 00077 } 00078 connect_success = mesh.connect(); 00079 network_interface = &mesh; 00080 #endif 00081 if(connect_success == 0) { 00082 if (log_messages) { 00083 output.printf("[EasyConnect] Connected to Network successfully\r\n"); 00084 } 00085 } else { 00086 if (log_messages) { 00087 output.printf("[EasyConnect] Connection to Network Failed %d!\r\n", connect_success); 00088 } 00089 return NULL; 00090 } 00091 if (log_messages) { 00092 const char *ip_addr = network_interface->get_ip_address(); 00093 if (ip_addr) { 00094 output.printf("[EasyConnect] IP address %s\r\n", ip_addr); 00095 } else { 00096 output.printf("[EasyConnect] No IP address\r\n"); 00097 } 00098 } 00099 return network_interface; 00100 } 00101 00102 #endif // __MAGIC_CONNECT_H__
Generated on Tue Jul 12 2022 19:14:50 by
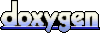