
Lalala
Embed:
(wiki syntax)
Show/hide line numbers
main.cpp
00001 #include "mbed.h" 00002 #include "BtnEventM0.h" 00003 00004 Serial pc(USBTX, USBRX); 00005 // LSB MSB 00006 BusOut lb(/*P1_13,P1_12,P1_7,*/P1_6,P1_4,P1_3,P1_1,P1_0,LED4,LED3,LED2,LED1); 00007 00008 BtnEventM0 sw4(P1_16), sw3(P0_23), sw1(P0_10), sw2(P0_15); 00009 00010 // Zustandsangebe 00011 BusOut stLED(P1_13,P1_12,P1_7); 00012 00013 00014 const int st_run = 1; 00015 const int st_editH = 2; 00016 const int st_editM = 3; 00017 const int st_editalarmH = 4; 00018 const int st_editalarmM = 5; 00019 00020 int blinkIdx = 0; 00021 int hh=0, mm=0; 00022 int alarmhh=0, alarmmm=0; 00023 bool alarm = false; 00024 00025 00026 class Timmer 00027 { 00028 public: 00029 void Init(); 00030 void Run(); 00031 void EditH(); 00032 void EditM(); 00033 void EditalarmH(); 00034 void EditalarmM(); 00035 public: 00036 int state; 00037 private: 00038 Timer t1; 00039 }; 00040 00041 Timmer tim1; 00042 00043 int main() 00044 { 00045 pc.baud(500000); 00046 sw4.Init(); // Switch state 00047 sw3.Init(); // Count UP 00048 sw1.Init(); // Count DOWN 00049 sw2.Init(); // Set Alarm 00050 tim1.Init(); 00051 00052 while(1) { 00053 if(tim1.state == st_run) { 00054 tim1.Run(); 00055 blinkIdx = 0; 00056 } 00057 if(tim1.state == st_editH) { 00058 tim1.EditH(); 00059 blinkIdx = 3; 00060 } 00061 if(tim1.state == st_editM) { 00062 tim1.EditM(); 00063 blinkIdx = -1; 00064 } 00065 if(tim1.state == st_editalarmH) { 00066 tim1.EditalarmH(); 00067 blinkIdx = 3; 00068 } 00069 if(tim1.state == st_editalarmM) { 00070 tim1.EditalarmM(); 00071 blinkIdx = -1; 00072 } 00073 } 00074 } 00075 00076 void Timmer::Init() 00077 { 00078 state= st_run; 00079 t1.start(); 00080 } 00081 00082 void Timmer::Run() 00083 { 00084 // 1..in die erste Zeile schreiben 00085 pc.printf("1 Clock running\n"); 00086 pc.printf("3 %d\n", blinkIdx); 00087 stLED = 1; 00088 00089 while(1) { 00090 if(sw4.CheckFlag()) { 00091 state = st_editH; 00092 return; 00093 } 00094 if(sw2.CheckFlag()) { 00095 state = st_editalarmH; 00096 alarm = true; 00097 return; 00098 } 00099 00100 if(t1.read_ms() > 50) { // Ausgabe mit 20Hz,aber dafür hate 1h 60min!!! 00101 t1.reset(); 00102 mm++; 00103 if(mm > 59) { 00104 mm = 0; 00105 hh++; 00106 } 00107 if(hh > 23) { 00108 hh = 0; 00109 } 00110 00111 // Alram 00112 if(alarm == true) { 00113 if(hh == alarmhh && mm == alarmmm) { 00114 pc.printf("1 !!!ALARM!!!\n"); 00115 alarmhh=0; 00116 alarmmm=0; 00117 alarm = false; 00118 } 00119 } 00120 pc.printf("2 %02d:%02d\n",hh,mm); 00121 } 00122 } 00123 } 00124 00125 /*********** SET TIME **************/ 00126 00127 void Timmer::EditH() 00128 { 00129 pc.printf("1 Edit hh\n"); 00130 pc.printf("2 %02d:%02d\n", hh, mm); 00131 pc.printf("3 %d\n", blinkIdx); 00132 stLED = 2; 00133 00134 while(1) { 00135 if(sw4.CheckFlag()) { 00136 state = st_editM; 00137 return; 00138 } 00139 00140 // Count UP 00141 if(sw3.CheckFlag()) { 00142 // Aktion für EinfachClick z.B. cnt++ ausführen 00143 hh++; 00144 if(hh > 23) 00145 hh = 0; 00146 pc.printf("2 %02d:%02d\n", hh, mm); 00147 00148 wait_ms(300); 00149 if(sw3.CheckButton()) 00150 while(sw3.CheckButton()) { 00151 // ContinousPress erkannt 00152 hh++; 00153 if(hh > 23) 00154 hh = 0; 00155 pc.printf("2 %02d:%02d\n", hh, mm); 00156 wait_ms(100); 00157 } 00158 } 00159 00160 // Count DOWN 00161 if(sw1.CheckFlag()) { 00162 // Aktion für EinfachClick z.B. cnt++ ausführen 00163 hh--; 00164 if(hh < 0) 00165 hh = 23; 00166 pc.printf("2 %02d:%02d\n", hh, mm); 00167 00168 wait_ms(300); 00169 if(sw1.CheckButton()) 00170 while(sw1.CheckButton()) { 00171 // ContinousPress erkannt 00172 hh--; 00173 if(hh < 0) 00174 hh = 23; 00175 pc.printf("2 %02d:%02d\n", hh, mm); 00176 wait_ms(100); 00177 } 00178 } 00179 } 00180 } 00181 00182 void Timmer::EditM() 00183 { 00184 pc.printf("1 Edit mm\n"); 00185 pc.printf("2 %02d:%02d\n", hh, mm); 00186 pc.printf("3 %d\n", blinkIdx); 00187 stLED = 3; 00188 00189 while(1) { 00190 if(sw4.CheckFlag()) { 00191 state = st_run; 00192 return; 00193 } 00194 00195 // Count UP 00196 if(sw3.CheckFlag()) { 00197 // Aktion für EinfachClick z.B. cnt++ ausführen 00198 mm++; 00199 if(mm > 59 ) 00200 mm = 0; 00201 pc.printf("2 %02d:%02d\n", hh, mm); 00202 00203 wait_ms(300); 00204 if(sw3.CheckButton()) 00205 while(sw3.CheckButton()) { 00206 // ContinousPress erkannt 00207 mm++; 00208 if(mm > 59) 00209 mm = 0; 00210 pc.printf("2 %02d:%02d\n", hh, mm); 00211 wait_ms(100); 00212 } 00213 } 00214 00215 // Count DOWN 00216 if(sw1.CheckFlag()) { 00217 // Aktion für EinfachClick z.B. cnt++ ausführen 00218 mm--; 00219 if(mm < 0) 00220 mm = 59; 00221 pc.printf("2 %02d:%02d\n", hh, mm); 00222 00223 wait_ms(300); 00224 if(sw1.CheckButton()) 00225 while(sw1.CheckButton()) { 00226 // ContinousPress erkannt 00227 mm--; 00228 if(mm < 0) 00229 mm = 59; 00230 pc.printf("2 %02d:%02d\n", hh, mm); 00231 wait_ms(100); 00232 } 00233 } 00234 } 00235 } 00236 00237 /*********** SET ALARM **************/ 00238 00239 void Timmer::EditalarmH() 00240 { 00241 pc.printf("1 Edit alarm hh\n"); 00242 pc.printf("2 %02d:%02d\n", alarmhh, alarmmm); 00243 pc.printf("3 %d\n", blinkIdx); 00244 stLED = 4; 00245 00246 while(1) { 00247 if(sw2.CheckFlag()) { 00248 state = st_editalarmM; 00249 return; 00250 } 00251 00252 // Count UP 00253 if(sw3.CheckFlag()) { 00254 // Aktion für EinfachClick z.B. cnt++ ausführen 00255 alarmhh++; 00256 if(alarmhh > 23) 00257 alarmhh = 0; 00258 pc.printf("2 %02d:%02d\n", alarmhh, alarmmm); 00259 00260 wait_ms(300); 00261 if(sw3.CheckButton()) 00262 while(sw3.CheckButton()) { 00263 // ContinousPress erkannt 00264 alarmhh++; 00265 if(alarmhh > 23) 00266 alarmhh = 0; 00267 pc.printf("2 %02d:%02d\n", alarmhh, alarmmm); 00268 wait_ms(100); 00269 } 00270 } 00271 00272 // Count DOWN 00273 if(sw1.CheckFlag()) { 00274 // Aktion für EinfachClick z.B. cnt++ ausführen 00275 alarmhh--; 00276 if(alarmhh < 0) 00277 alarmhh = 23; 00278 pc.printf("2 %02d:%02d\n", alarmhh, alarmmm); 00279 00280 wait_ms(300); 00281 if(sw1.CheckButton()) 00282 while(sw1.CheckButton()) { 00283 // ContinousPress erkannt 00284 alarmhh--; 00285 if(alarmhh < 0) 00286 alarmhh = 23; 00287 pc.printf("2 %02d:%02d\n", alarmhh, alarmmm); 00288 wait_ms(100); 00289 } 00290 } 00291 } 00292 } 00293 00294 void Timmer::EditalarmM() 00295 { 00296 pc.printf("1 Edit alarm mm\n"); 00297 pc.printf("2 %02d:%02d\n", alarmhh, alarmmm); 00298 pc.printf("3 %d\n", blinkIdx); 00299 stLED = 5; 00300 00301 while(1) { 00302 if(sw2.CheckFlag()) { 00303 state = st_run; 00304 return; 00305 } 00306 00307 // Count UP 00308 if(sw3.CheckFlag()) { 00309 // Aktion für EinfachClick z.B. cnt++ ausführen 00310 alarmmm++; 00311 if(alarmmm > 59 ) 00312 alarmmm = 0; 00313 pc.printf("2 %02d:%02d\n", alarmhh, alarmmm); 00314 00315 wait_ms(300); 00316 if(sw3.CheckButton()) 00317 while(sw3.CheckButton()) { 00318 // ContinousPress erkannt 00319 alarmmm++; 00320 if(alarmmm > 59) 00321 alarmmm = 0; 00322 pc.printf("2 %02d:%02d\n", alarmhh, alarmmm); 00323 wait_ms(100); 00324 } 00325 } 00326 00327 // Count DOWN 00328 if(sw1.CheckFlag()) { 00329 // Aktion für EinfachClick z.B. cnt++ ausführen 00330 alarmmm--; 00331 if(alarmmm < 0) 00332 alarmmm = 59; 00333 pc.printf("2 %02d:%02d\n", alarmhh, alarmmm); 00334 00335 wait_ms(300); 00336 if(sw1.CheckButton()) 00337 while(sw1.CheckButton()) { 00338 // ContinousPress erkannt 00339 alarmmm--; 00340 if(alarmmm < 0) 00341 alarmmm = 59; 00342 pc.printf("2 %02d:%02d\n", alarmhh, alarmmm); 00343 wait_ms(100); 00344 } 00345 } 00346 } 00347 }
Generated on Sat Jul 23 2022 21:20:55 by
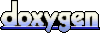