Reaction Wheel Actuated Satellite Dynamics Test Platform
Embed:
(wiki syntax)
Show/hide line numbers
UM6_usart.h
00001 /* ______________________________________________________________________________________ 00002 File: UM6_usart.h 00003 Author: CH Robotics, adapted for mbed by lhiggs 00004 Version: 1.0 00005 00006 Description: Function declarations for USART communucation 00007 -------------------------------------------------------------------------------------- */ 00008 00009 #ifndef _CHR_USART_H 00010 #define _CHR_USART_H 00011 00012 #define MAX_PACKET_DATA 40 00013 00014 // Definitions of states for USART receiver state machine (for receiving packets) 00015 #define USART_STATE_WAIT 1 00016 #define USART_STATE_TYPE 2 00017 #define USART_STATE_ADDRESS 3 00018 #define USART_STATE_DATA 4 00019 #define USART_STATE_CHECKSUM 5 00020 00021 // Flags for interpreting the packet type byte in communication packets 00022 #define PACKET_HAS_DATA (1 << 7) 00023 #define PACKET_IS_BATCH (1 << 6) 00024 #define PACKET_BATCH_LENGTH_MASK ( 0x0F ) 00025 00026 #define PACKET_BATCH_LENGTH_OFFSET 2 00027 00028 #define BATCH_SIZE_2 2 00029 #define BATCH_SIZE_3 3 00030 00031 #define PACKET_NO_DATA 0 00032 #define PACKET_COMMAND_FAILED (1 << 0) 00033 00034 00035 // Define flags for identifying the type of packet address received 00036 #define ADDRESS_TYPE_CONFIG 0 00037 #define ADDRESS_TYPE_DATA 1 00038 #define ADDRESS_TYPE_COMMAND 2 00039 00040 00041 extern uint8_t gUSART_State; 00042 00043 // Structure for storing TX and RX packet data 00044 typedef struct _USARTPacket 00045 { 00046 uint8_t PT; // Packet type 00047 uint8_t address; // Packet address 00048 uint16_t checksum; // Checksum 00049 00050 // Data included for convenience, but that isn't stored in the packet itself 00051 uint8_t data_length; // Number of bytes in data section 00052 uint8_t address_type; // Specified the address type (DATA, CONFIG, OR COMMAND) 00053 00054 uint8_t packet_data[MAX_PACKET_DATA]; 00055 00056 } USARTPacket; 00057 00058 uint16_t ComputeChecksum( USARTPacket* new_packet ); 00059 00060 #endif
Generated on Fri Jul 15 2022 05:24:10 by
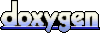