SerialFlow allows to send and receive packaged arrays of integer values via serial port.
Embed:
(wiki syntax)
Show/hide line numbers
SerialFlow.h
00001 /* mbed Serial Flow Library 00002 * Copyright (c) 2012 Oleg Evsegneev 00003 * Released under the MIT License: http://mbed.org/license/mit 00004 */ 00005 00006 #ifndef MBED_SERIALFLOW_H 00007 #define MBED_SERIALFLOW_H 00008 00009 // optional use of MODSERIAL 00010 #define USE_MODSERIAL 00011 00012 #include "mbed.h" 00013 00014 #ifdef USE_MODSERIAL 00015 #include "MODSERIAL.h" 00016 #endif 00017 00018 #define MAX_PACKET_SIZE 10 00019 #define RX_BUFFER_SIZE 32 00020 #define TX_BUFFER_SIZE 32 00021 00022 class SerialFlow { 00023 00024 public: 00025 /** Data format */ 00026 enum DataFormat { 00027 SIMPLE /**< Simple one byte (default) */ 00028 , COMPLEX /**< Packet of many short values */ 00029 }; 00030 00031 SerialFlow(PinName tx, PinName rx); 00032 00033 /** Set portal baud rate 00034 * 00035 * @param baud_rate Port baud rate. 00036 */ 00037 void baud(int baud_rate); 00038 00039 /** Set data packet format 00040 * 00041 * @param p_format Format type constant. 00042 * @param v_length Length of value in bytes. 00043 * @param p_size Number of values. 00044 */ 00045 void setPacketFormat(DataFormat p_format, char v_length, char p_size); 00046 00047 /** Set value to data packet 00048 * 00049 * @param value Value. 00050 */ 00051 void setPacketValue(short value); 00052 00053 /** Send packet to serial port 00054 */ 00055 bool sendPacket(); 00056 00057 /** Receive packet from serial port 00058 */ 00059 bool receivePacket(); 00060 00061 /** Get received packet 00062 * @param idx Index of value from packet. 00063 */ 00064 short getPacket( char idx ); 00065 00066 protected: 00067 #ifdef USE_MODSERIAL 00068 MODSERIAL _serial; 00069 #else 00070 Serial _serial; 00071 #endif 00072 00073 char _p_format; 00074 char _p_size; 00075 char _v_length; 00076 00077 short _vs[MAX_PACKET_SIZE]; 00078 char _vs_idx; 00079 00080 short _vr[MAX_PACKET_SIZE]; 00081 char _vr_val[2]; 00082 char _vr_idx; 00083 char _cr_idx; 00084 bool _escape; 00085 bool _collecting; 00086 }; 00087 00088 #endif
Generated on Fri Jul 15 2022 03:16:50 by
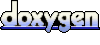