SerialFlow allows to send and receive packaged arrays of integer values via serial port.
Embed:
(wiki syntax)
Show/hide line numbers
SerialFlow.cpp
00001 /* mbed Serial Flow Library 00002 * Copyright (c) 2012 Oleg Evsegneev 00003 * Released under the MIT License: http://mbed.org/license/mit 00004 */ 00005 00006 #include "SerialFlow.h" 00007 #include "mbed.h" 00008 00009 #ifdef USE_MODSERIAL 00010 SerialFlow::SerialFlow(PinName tx, PinName rx): _serial(tx, rx, TX_BUFFER_SIZE, RX_BUFFER_SIZE) { 00011 #else 00012 SerialFlow::SerialFlow(PinName tx, PinName rx): _serial(tx, rx) { 00013 #endif 00014 _escape = 0; 00015 _collecting = 0; 00016 } 00017 00018 void SerialFlow::baud(int baud_rate) { 00019 _serial.baud(baud_rate); 00020 } 00021 00022 void SerialFlow::setPacketFormat(DataFormat p_format, char v_length, char p_size) { 00023 _p_format = p_format; 00024 _v_length = v_length; 00025 _p_size = p_size; 00026 _vs_idx = 0; 00027 _vr_idx = 0; 00028 } 00029 00030 void SerialFlow::setPacketValue(short value) { 00031 if( _vs_idx < _p_size ){ 00032 _vs[_vs_idx++] = value; 00033 } 00034 } 00035 00036 bool SerialFlow::sendPacket() { 00037 char v; 00038 if( !_serial.writeable() ){ 00039 _vs_idx = 0; 00040 return 0; 00041 } 00042 00043 _serial.putc( 0x12 ); 00044 for( char i=0; i<_vs_idx; i++ ){ 00045 // low byte 00046 v = _vs[i] & 0xFF; 00047 if( v==0x12 || v==0x13 || v==0x7D || v==0x10 ) 00048 _serial.putc( 0x7D ); 00049 _serial.putc( v ); 00050 00051 // high byte 00052 v = (_vs[i]>>8) & 0xFF; 00053 if( v==0x12 || v==0x13 || v==0x7D || v==0x10 ) 00054 _serial.putc( 0x7D ); 00055 _serial.putc( v ); 00056 00057 // separate values 00058 if( i<_vs_idx-1 ) 00059 _serial.putc(0x10); 00060 } 00061 00062 _serial.putc( 0x13 ); 00063 _vs_idx = 0; 00064 return 1; 00065 } 00066 00067 bool SerialFlow::receivePacket() { 00068 char c; 00069 while( _serial.readable() ){ 00070 c = _serial.getc(); 00071 if( _collecting ) 00072 if( _escape ){ 00073 _vr_val[_cr_idx++] = c; 00074 _escape = 0; 00075 } 00076 // escape 00077 else if( c == 0x7D ){ 00078 _escape = 1; 00079 } 00080 // value separator 00081 else if( c == 0x10 ){ 00082 _vr[_vr_idx++] = _vr_val[0] | (_vr_val[1] << 8); 00083 _cr_idx = 0; 00084 } 00085 // end 00086 else if( c == 0x13 ){ 00087 _vr[_vr_idx++] = _vr_val[0] | (_vr_val[1] << 8); 00088 _collecting = 0; 00089 return 1; 00090 } 00091 else{ 00092 _vr_val[_cr_idx++] = c; 00093 } 00094 // begin 00095 else if( c == 0x12 ){ 00096 _collecting = 1; 00097 _cr_idx = 0; 00098 _vr_idx = 0; 00099 } 00100 } 00101 return 0; 00102 } 00103 00104 short SerialFlow::getPacket( char idx ) { 00105 return _vr[idx]; 00106 }
Generated on Fri Jul 15 2022 03:16:50 by
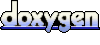