
Driver for Bosch Sensortec BME280 combined humidity and pressure sensor
Embed:
(wiki syntax)
Show/hide line numbers
bme280.h
00001 /* 00002 bme280.h - driver for Bosch Sensortec BME280 combined humidity and pressure sensor. 00003 00004 Copyright (c) 2015 Elektor 00005 00006 26/11/2015 - CPV, Initial release. 00007 00008 This library is free software; you can redistribute it and/or 00009 modify it under the terms of the GNU Lesser General Public 00010 License as published by the Free Software Foundation; either 00011 version 2.1 of the License, or (at your option) any later version. 00012 00013 This library is distributed in the hope that it will be useful, 00014 but WITHOUT ANY WARRANTY; without even the implied warranty of 00015 MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the GNU 00016 Lesser General Public License for more details. 00017 00018 You should have received a copy of the GNU Lesser General 00019 Public License along with this library; if not, write to the 00020 Free Software Foundation, Inc., 59 Temple Place, Suite 330, 00021 Boston, MA 02111-1307 USA 00022 00023 */ 00024 00025 #ifndef __BME280_H__ 00026 #define __BME280_H__ 00027 00028 #include <stdint.h> 00029 00030 00031 #define BME280_ALLOW_FLOAT (1) 00032 00033 #if BME280_ALLOW_FLOAT!=0 00034 typedef double temperature_t; 00035 typedef double pressure_t; 00036 typedef double humidity_t; 00037 #else 00038 typedef int32_t temperature_t; 00039 typedef uint32_t pressure_t; 00040 typedef uint32_t humidity_t; 00041 #endif /* BME280_ALLOW_FLOAT */ 00042 00043 00044 // Two possible addresses, depending on level on SDO pin. 00045 #define BME280_I2C_ADDRESS1 (0x76) 00046 #define BME280_I2C_ADDRESS2 (0x77) 00047 00048 // Calibration registers. 00049 #define BME280_CAL_T1 (0x88) 00050 #define BME280_CAL_T2 (0x8a) 00051 #define BME280_CAL_T3 (0x8c) 00052 #define BME280_CAL_P1 (0x8e) 00053 #define BME280_CAL_P2 (0x90) 00054 #define BME280_CAL_P3 (0x92) 00055 #define BME280_CAL_P4 (0x94) 00056 #define BME280_CAL_P5 (0x96) 00057 #define BME280_CAL_P6 (0x98) 00058 #define BME280_CAL_P7 (0x9a) 00059 #define BME280_CAL_P8 (0x9c) 00060 #define BME280_CAL_P9 (0x9e) 00061 #define BME280_CAL_H1 (0xa1) /* 8 bits */ 00062 #define BME280_CAL_H2 (0xe1) 00063 #define BME280_CAL_H3 (0xe3) /* 8 bits */ 00064 #define BME280_CAL_H4 (0xe4) /* 12 bits, combined with H45 */ 00065 #define BME280_CAL_H45 (0xe5) /* 12 bits, combined with H5 */ 00066 #define BME280_CAL_H5 (0xe6) /* 8 bits */ 00067 #define BME280_CAL_H6 (0xe7) /* 8 bits */ 00068 00069 // Control registers. 00070 #define BME280_ID_REGISTER (0xd0) /* 8 bits */ 00071 #define BME280_RESET_REGISTER (0xe0) /* 8 bits */ 00072 #define BME280_CTRL_HUM_REGISTER (0xf2) /* 8 bits */ 00073 #define BME280_STATUS_REGISTER (0xf3) /* 8 bits */ 00074 #define BME280_CTRL_MEAS_REGISTER (0xf4) /* 8 bits */ 00075 #define BME280_CONFIG_REGISTER (0xf5) /* 8 bits */ 00076 00077 // Measurement registers. 00078 #define BME280_PRESSURE (0xf7) /* 20 bits */ 00079 #define BME280_PRESSURE_MSB (0xf7) /* 8 bits */ 00080 #define BME280_PRESSURE_LSB (0xf8) /* 8 bits */ 00081 #define BME280_PRESSURE_XLSB (0xf9) /* 8 bits */ 00082 #define BME280_TEMPERATURE (0xfa) /* 20 bits */ 00083 #define BME280_TEMPERATURE_MSB (0xfa) /* 8 bits */ 00084 #define BME280_TEMPERATURE_LSB (0xfb) /* 8 bits */ 00085 #define BME280_TEMPERATURE_XLSB (0xfc) /* 8 bits */ 00086 #define BME280_HUMIDITY (0xfd) /* 16 bits */ 00087 #define BME280_HUMIDITY_MSB (0xfd) /* 8 bits */ 00088 #define BME280_HUMIDITY_LSB (0xfe) /* 8 bits */ 00089 00090 // It is recommended to read all the measurements in one go. 00091 #define BME280_MEASUREMENT_REGISTER (BME280_PRESSURE) 00092 #define BME280_MEASUREMENT_SIZE (8) 00093 00094 // Values for osrs_p & osrs_t fields of CTRL_MEAS register. 00095 #define BME280_SKIP (0) 00096 #define BME280_OVERSAMPLING_1X (1) 00097 #define BME280_OVERSAMPLING_2X (2) 00098 #define BME280_OVERSAMPLING_4X (3) 00099 #define BME280_OVERSAMPLING_8X (4) 00100 #define BME280_OVERSAMPLING_16X (5) 00101 00102 // Values for mode field of CTRL_MEAS register. 00103 #define BME280_MODE_SLEEP (0) 00104 #define BME280_MODE_FORCED (1) 00105 #define BME280_MODE_NORMAL (3) 00106 00107 // Value for RESET register. 00108 #define BME280_RESET (0xb6) 00109 00110 // Value of ID register. 00111 #define BME280_ID (0x60) 00112 00113 // Values for t_sb field of CONFIG register 00114 #define BME280_STANDBY_500_US (0) 00115 #define BME280_STANDBY_10_MS (6) 00116 #define BME280_STANDBY_20_MS (7) 00117 #define BME280_STANDBY_63_MS (1) 00118 #define BME280_STANDBY_125_MS (2) 00119 #define BME280_STANDBY_250_MS (3) 00120 #define BME280_STANDBY_500_MS (4) 00121 #define BME280_STANDBY_1000_MS (5) 00122 00123 // Values for filter field of CONFIG register 00124 #define BME280_FILTER_OFF (0) 00125 #define BME280_FILTER_COEFF_2 (1) 00126 #define BME280_FILTER_COEFF_4 (2) 00127 #define BME280_FILTER_COEFF_8 (3) 00128 #define BME280_FILTER_COEFF_16 (4) 00129 00130 00131 // I2C write function must support repeated start to avoid interruption of transactions. 00132 // User-provided function to write data_size bytes from buffer p_data to I2C device at bus address i2c_address. 00133 // Provide empty function if not used. 00134 extern void i2cWrite(uint8_t i2c_address, uint8_t *p_data, uint8_t data_size, uint8_t repeated_start); 00135 // User-provided function to read data_size bytes from I2C device at bus address i2c_address to buffer p_data. 00136 // Provide empty function if not used. 00137 extern void i2cRead(uint8_t i2c_address, uint8_t *p_data, uint8_t data_size); 00138 00139 // SPI functions must activate (make low) BME280 CSB pin before doing a transfer 00140 // and deactivate it (make high) when done. 00141 // User-provided function to write data_size bytes from buffer p_data to SPI device. 00142 // Provide empty function if not used. 00143 extern void spiWrite(uint8_t *p_data, uint8_t data_size); 00144 // User-provided function to read data_size bytes from SPI device to buffer p_data. 00145 // Provide empty function if not used. 00146 extern void spiRead(uint8_t *p_data, uint8_t data_size); 00147 00148 00149 class BME280 00150 { 00151 public: 00152 BME280(void); 00153 uint8_t begin(uint8_t i2cAddress=0); // I2C address not set means SPI. 00154 00155 void readCalibrationData(void); 00156 uint8_t readId(void); 00157 uint8_t readFrom(uint8_t reg, uint8_t data_size, uint8_t *p_data); 00158 00159 void read(void); 00160 00161 // If using floating points, values are scaled, if not then 00162 // temperature is 100*T, pressure is Pa en humidity is 1024*%RH 00163 temperature_t temperature(void) { return _temperature; } // [degrees Celsius] or 100*[degrees Celsius] 00164 pressure_t pressure(void) { return _pressure; } // [Pa] 00165 humidity_t humidity(void) { return _humidity; } // [%RH] or 1024*[%RH] 00166 00167 void writeControlRegisters(uint8_t osrs_t, uint8_t osrs_p, uint8_t osrs_h, uint8_t mode); 00168 void writeConfigRegister(uint8_t t_sb, uint8_t filter, uint8_t spi); 00169 00170 void reset(void); 00171 00172 private: 00173 uint8_t _i2c_address; 00174 00175 void busWrite(uint8_t *p_data, uint8_t data_size, uint8_t repeated_start); 00176 void busRead(uint8_t *p_data, uint8_t data_size); 00177 00178 // Calibration data. 00179 uint16_t _dig_T1; 00180 int16_t _dig_T2; 00181 int16_t _dig_T3; 00182 uint16_t _dig_P1; 00183 int16_t _dig_P2; 00184 int16_t _dig_P3; 00185 int16_t _dig_P4; 00186 int16_t _dig_P5; 00187 int16_t _dig_P6; 00188 int16_t _dig_P7; 00189 int16_t _dig_P8; 00190 int16_t _dig_P9; 00191 uint8_t _dig_H1; 00192 int16_t _dig_H2; 00193 uint8_t _dig_H3; 00194 int16_t _dig_H4; 00195 int16_t _dig_H5; 00196 int8_t _dig_H6; 00197 void clearCalibrationData(void); 00198 00199 uint8_t readUint8(uint8_t reg); 00200 uint16_t readUint16(uint8_t reg); 00201 00202 int32_t assembleRawValue(uint8_t *p_data, uint8_t has_xlsb); 00203 00204 int32_t _t_fine; 00205 00206 temperature_t compensateTemperature(int32_t adc_T); 00207 pressure_t compensatePressure(int32_t adc_P); 00208 humidity_t compensateHumidity(int32_t adc_H); 00209 00210 temperature_t _temperature; 00211 pressure_t _pressure; 00212 humidity_t _humidity; 00213 }; 00214 00215 00216 #endif /* __BME280_H__*/
Generated on Tue Jul 12 2022 18:38:32 by
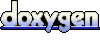