Initial for Condor Simulator
Dependents: USBJoystick_2 USBJoystick_NEW
Fork of USBJoystick by
USBJoystick.cpp
00001 /* mbed USBJoystick Library 00002 * Copyright (c) 2012, v01: Initial version, WH, 00003 * Modified USBMouse code ARM Limited. 00004 * (c) 2010-2011 mbed.org, MIT License 00005 * 2016, v02: Updated USBDevice Lib, Added waitForConnect, Updated 32 bits button 00006 * 00007 * Permission is hereby granted, free of charge, to any person obtaining a copy 00008 * of this software and associated documentation files (the "Software"), to deal 00009 * in the Software without restriction, inclumosig without limitation the rights 00010 * to use, copy, modify, merge, publish, distribute, sublicense, and/or sell 00011 * copies of the Software, and to permit persons to whom the Software is 00012 * furnished to do so, subject to the following conditions: 00013 * 00014 * The above copyright notice and this permission notice shall be included in 00015 * all copies or substantial portions of the Software. 00016 * 00017 * THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR 00018 * IMPLIED, INCLUmosiG BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY, 00019 * FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE 00020 * AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER 00021 * LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM, 00022 * OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN 00023 * THE SOFTWARE. 00024 */ 00025 00026 00027 #include "stdint.h" 00028 #include "USBJoystick.h" 00029 00030 bool USBJoystick::update(int16_t x, int16_t y, int16_t b, int16_t f, int16_t r, int16_t t, uint8_t hat, uint32_t buttons) 00031 { 00032 00033 _x = x; 00034 _y = y; 00035 _b = b; 00036 _f = f; 00037 _r = r; 00038 _t = t; 00039 _hat = hat; 00040 _buttons = buttons; 00041 00042 return update(); 00043 } 00044 00045 bool USBJoystick::update() 00046 { 00047 uint8_t pos = 0; 00048 HID_REPORT report; 00049 00050 // Fill the report according to the Joystick Descriptor 00051 report.data[pos] = (_x >> 0) & 0xff; 00052 00053 report.data[++pos] = (_x >> 8) & 0xff; 00054 report.data[++pos] = _y & 0xff; 00055 report.data[++pos] = (_y >> 8) & 0xff; 00056 report.data[++pos] = _b & 0xff; 00057 report.data[++pos] = (_b >> 8) & 0xff; 00058 report.data[++pos] = _f & 0xff; 00059 report.data[++pos] = (_f >> 8) & 0xff; 00060 report.data[++pos] = _r & 0xff; 00061 report.data[++pos] = (_r >> 8) & 0xff; 00062 report.data[++pos] = _t & 0xff; 00063 report.data[++pos] = (_t >> 8) & 0xff; 00064 00065 #if (HAT4 == 1) 00066 //Use 4 bit padding for hat4 or hat8 00067 report.data[++pos] = (_hat & 0x0f) ; 00068 #endif 00069 00070 #if (HAT8 == 1) 00071 //Use 4 bit padding for hat4 or hat8 00072 report.data[++pos] = (_hat & 0x0f) ; 00073 #endif 00074 00075 #if (BUTTONS4 == 1) 00076 //Use 4 bit padding for buttons 00077 report.data[++pos] = (_buttons & 0x0f) ; 00078 report.length = ++pos; 00079 #endif 00080 00081 #if (BUTTONS8 == 1) 00082 //Use 8 bits for buttons 00083 report.data[++pos] = (_buttons & 0xff) ; 00084 report.length = ++pos; 00085 #endif 00086 00087 #if (BUTTONS32 == 1) 00088 //No bit padding for 32 buttons 00089 report.data[++pos] = (_buttons >> 0) & 0xff; 00090 report.data[++pos] = (_buttons >> 8) & 0xff; 00091 report.data[++pos] = (_buttons >> 16) & 0xff; 00092 report.data[++pos] = (_buttons >> 24) & 0xff; 00093 report.length = ++pos; 00094 #endif 00095 00096 return send(&report); 00097 } 00098 00099 bool USBJoystick::move(int16_t x, int16_t y) 00100 { 00101 _x = x; 00102 _y = y; 00103 return update(); 00104 } 00105 00106 bool USBJoystick::diveBreak(int16_t b) 00107 { 00108 _b = b; 00109 return update(); 00110 } 00111 00112 bool USBJoystick::flaps(int16_t f) 00113 { 00114 _f = f; 00115 return update(); 00116 } 00117 00118 bool USBJoystick::rudder(int16_t r) 00119 { 00120 _r = r; 00121 return update(); 00122 } 00123 00124 bool USBJoystick::throttle(int16_t t) 00125 { 00126 _t = t; 00127 return update(); 00128 } 00129 00130 bool USBJoystick::hat(uint8_t hat) 00131 { 00132 _hat = hat; 00133 return update(); 00134 } 00135 00136 bool USBJoystick::buttons(uint32_t buttons) 00137 { 00138 _buttons = buttons; 00139 return update(); 00140 } 00141 00142 00143 00144 void USBJoystick::_init() 00145 { 00146 _x = 32767; // X-Axis 00147 _y = 32767; // Y-Axis 00148 _b = 32767; // Breaks 00149 _f = 32767; // Wing Flaps 00150 _r = 32767; // Rudder 00151 _t = 32767; // Throttle 00152 _hat = 0x00; // POV Hat-Switches 00153 _buttons = 0x00000000; // Buttons 00154 } 00155 00156 00157 uint8_t * USBJoystick::reportDesc() 00158 { 00159 static uint8_t reportDescriptor[] = { 00160 00161 USAGE_PAGE(1), 0x01, // Generic Desktop 00162 USAGE(1), 0x04, // Usage (Joystick) 00163 COLLECTION(1), 0x01, // Application 00164 00165 //******* Joystick Axis and Sliders ******* 00166 USAGE_PAGE(1), 0x01, // Generic Desktop 00167 USAGE(1), 0x01, // Usage (Pointer) - (Joystick = 0x04) 00168 COLLECTION(1), 0x00, // Physical Usage in main.cpp 00169 USAGE(1), 0x30, // X X 00170 USAGE(1), 0x31, // Y Y 00171 USAGE(1), 0x32, // Z Breaks 00172 USAGE(1), 0x33, // Rx Flaps 00173 // USAGE(1), 0x34, // Ry 00174 // USAGE(1), 0x35, // Rz (Rudder on Usage Page 0x02) 00175 // USAGE(1), 0x36 // Slider (Throttle on Usage Page 0x02) 00176 LOGICAL_MINIMUM(2), 0xff, 0x08, // war 0, 0x00, 0x80 00177 LOGICAL_MAXIMUM(2), 0x00, 0x08, // war 65536, 0xff 0x7f 00178 REPORT_SIZE(1), 0x10, 00179 REPORT_COUNT(1), 0x04, 00180 INPUT(1), 0x02, // Data, Variable, Absolute 00181 END_COLLECTION(0), 00182 00183 USAGE_PAGE(1), 0x02, // Simulation Controls 00184 USAGE(1), 0xBA, // Usage (Rudder) 00185 USAGE(1), 0xBB, // Usage (Throttle) 00186 LOGICAL_MINIMUM(2), 0xff, 0x08, // 0 00187 LOGICAL_MAXIMUM(2), 0x00, 0x08, // 65536 00188 REPORT_SIZE(1), 0x10, 00189 REPORT_COUNT(1), 0x02, 00190 INPUT(1), 0x02, // Data, Variable, Absolute 00191 00192 //******* Hat Switches ******* 00193 USAGE_PAGE(1), 0x01, // Generic Desktop 00194 USAGE(1), 0x39, // Usage (Hat switch) 00195 #if (HAT4 == 1 && HAT4_8 == 0) 00196 // 4 Position Hat Switch 00197 LOGICAL_MINIMUM(1), 0x00, // 0 00198 LOGICAL_MAXIMUM(1), 0x03, // 3 00199 PHYSICAL_MINIMUM(1), 0x00, // Physical_Minimum (0) 00200 PHYSICAL_MAXIMUM(2), 0x0E, 0x01, // Physical_Maximum (270) 00201 //UNIT_EXPONENT(1), 0x00, // Unit Exponent (0) 00202 UNIT(1), 0x14, // Unit (Degrees) 00203 #endif 00204 #if (HAT8 == 1) || (HAT4 == 1 && HAT4_8 == 1) 00205 // 8 Position Hat Switch 00206 LOGICAL_MINIMUM(1), 0x00, // 0 00207 LOGICAL_MAXIMUM(1), 0x07, // 7 00208 PHYSICAL_MINIMUM(1), 0x00, // Physical_Minimum (0) 00209 PHYSICAL_MAXIMUM(2), 0x3B, 0x01, // Physical_Maximum (315) 00210 //UNIT_EXPONENT(1), 0x00, // Unit Exponent (0) 00211 UNIT(1), 0x14, // Unit (Degrees) 00212 #endif 00213 REPORT_SIZE(1), 0x04, 00214 REPORT_COUNT(1), 0x01, 00215 INPUT(1), 0x42, // Data, Variable, Absolute, Null State 00216 // Padding 4 bits 00217 REPORT_SIZE(1), 0x01, 00218 REPORT_COUNT(1), 0x04, 00219 INPUT(1), 0x01, // Constant 00220 00221 //******* Buttons ******* 00222 USAGE_PAGE(1), 0x09, // Buttons 00223 #if (BUTTONS4 == 1) 00224 // 4 Buttons 00225 USAGE_MINIMUM(1), 0x01, // 1 00226 USAGE_MAXIMUM(1), 0x04, // 4 00227 LOGICAL_MINIMUM(1), 0x00, // 0 00228 LOGICAL_MAXIMUM(1), 0x01, // 1 00229 REPORT_SIZE(1), 0x01, 00230 REPORT_COUNT(1), 0x04, 00231 //UNIT_EXPONENT(1), 0x00, // Unit_Exponent (0) 00232 UNIT(1), 0x00, // Unit (None) 00233 INPUT(1), 0x02, // Data, Variable, Absolute 00234 // Padding 4 bits 00235 REPORT_SIZE(1), 0x01, 00236 REPORT_COUNT(1), 0x04, 00237 INPUT(1), 0x01, // Constant 00238 #endif 00239 #if (BUTTONS8 == 1) 00240 // 8 Buttons 00241 USAGE_MINIMUM(1), 0x01, // 1 00242 USAGE_MAXIMUM(1), 0x08, // 8 00243 LOGICAL_MINIMUM(1), 0x00, // 0 00244 LOGICAL_MAXIMUM(1), 0x01, // 1 00245 REPORT_SIZE(1), 0x01, 00246 REPORT_COUNT(1), 0x08, 00247 //UNIT_EXPONENT(1), 0x00, // Unit_Exponent (0) 00248 UNIT(1), 0x00, // Unit (None) 00249 INPUT(1), 0x02, // Data, Variable, Absolute 00250 #endif 00251 #if (BUTTONS32 == 1) 00252 // 32 Buttons 00253 USAGE_MINIMUM(1), 0x01, // 1 00254 USAGE_MAXIMUM(1), 0x20, // 32 00255 LOGICAL_MINIMUM(1), 0x00, // 0 00256 LOGICAL_MAXIMUM(1), 0x01, // 1 00257 REPORT_SIZE(1), 0x01, 00258 REPORT_COUNT(1), 0x20, 00259 //UNIT_EXPONENT(1), 0x00, // Unit_Exponent (0) 00260 UNIT(1), 0x00, // Unit (None) 00261 INPUT(1), 0x02, // Data, Variable, Absolute 00262 #endif 00263 00264 END_COLLECTION(0) 00265 }; 00266 00267 reportLength = sizeof(reportDescriptor); 00268 return reportDescriptor; 00269 } 00270
Generated on Sat Jul 16 2022 21:30:57 by
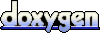