Red Light Work
Fork of realtimeMMLib by
Embed:
(wiki syntax)
Show/hide line numbers
mmdevice.cpp
00001 #include "mmdevice.h" 00002 #include <utility> // std::pair, std::make_pair 00003 #include <string> // std::string 00004 #include <iostream> // std::cout 00005 00006 00007 int mmdevice::getdata() 00008 { 00009 std::pair <std::string,double> product1; // default constructor 00010 std::pair <std::string,double> product2 ("tomatoes",2.30); // value init 00011 std::pair <std::string,double> product3 (product2); // copy constructor 00012 00013 product1 = std::make_pair(std::string("lightbulbs"),0.99); // using make_pair (move) 00014 00015 product2.first = "shoes"; // the type of first is string 00016 product2.second = 39.90; // the type of second is double 00017 00018 std::cout << "The price of " << product1.first << " is $" << product1.second << '\n'; 00019 std::cout << "The price of " << product2.first << " is $" << product2.second << '\n'; 00020 std::cout << "The price of " << product3.first << " is $" << product3.second << '\n'; 00021 return 1; 00022 }
Generated on Tue Jul 26 2022 06:36:17 by
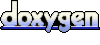