A library for the Avago ADJD-S311-CR999 Color Light Sensor
Dependents: ADJD-S311_HelloWorld
ADJDs311.h
00001 #ifndef ADJDs311_h 00002 #define ADJDs311_h 00003 00004 00005 //Includes 00006 00007 #include "mbed.h" 00008 00009 // ADJD-S311's I2C address, don't change 00010 #define WRITE_ADDRESS 0xE8 // Address for write 00011 #define READ_ADDRESS 0xE9 // Address for read 00012 00013 00014 // ADJD-S311's register list 00015 #define CTRL 0x00 00016 #define CONFIG 0x01 00017 #define CAP_RED 0x06 00018 #define CAP_GREEN 0x07 00019 #define CAP_BLUE 0x08 00020 #define CAP_CLEAR 0x09 00021 #define INT_RED_LO 0xA 00022 #define INT_RED_HI 0xB 00023 #define INT_GREEN_LO 0xC 00024 #define INT_GREEN_HI 0xD 00025 #define INT_BLUE_LO 0xE 00026 #define INT_BLUE_HI 0xF 00027 #define INT_CLEAR_LO 0x10 00028 #define INT_CLEAR_HI 0x11 00029 #define DATA_RED_LO 0x40 00030 #define DATA_RED_HI 0x41 00031 #define DATA_GREEN_LO 0x42 00032 #define DATA_GREEN_HI 0x43 00033 #define DATA_BLUE_LO 0x44 00034 #define DATA_BLUE_HI 0x45 00035 #define DATA_CLEAR_LO 0x46 00036 #define DATA_CLEAR_HI 0x47 00037 #define OFFSET_RED 0x48 00038 #define OFFSET_GREEN 0x49 00039 #define OFFSET_BLUE 0x4A 00040 #define OFFSET_CLEAR 0x4B 00041 00042 /** 00043 * A structure contain info about the red, green, blue and clear channel 00044 */ 00045 struct RGBC{ 00046 int red; 00047 int blue; 00048 int green; 00049 int clear; 00050 }; 00051 00052 /** 00053 * A ADJD-S311 color sensor class 00054 */ 00055 class ADJDs311{ 00056 public: 00057 00058 /** 00059 * Create a color sensor interface 00060 * 00061 * @param sda Pin connected to sda of color sensor 00062 * @param scl Pin connected to scl of color sensor 00063 * @param led Pin connected to on board led of the sensor 00064 */ 00065 ADJDs311(PinName sda, PinName scl, PinName led); 00066 00067 00068 /** 00069 * Calibrate the capacitance and integration time slot so that the current 00070 * readings are as close to 1000 as possible and the difference between RGB 00071 * readings are as small as possible 00072 */ 00073 void calibrate(); 00074 00075 /** 00076 * Turn the on board LED on/off. 00077 * 00078 * @param ledOn Whether to turn the LED on. 00079 */ 00080 void ledMode(bool ledOn); 00081 00082 /** 00083 * Get the current offset stored in offset registers 00084 * 00085 * @return Current offset stored in offset registers 00086 */ 00087 RGBC getOffset(); 00088 00089 /** 00090 * Use the current light condition to set the offset 00091 * 00092 * @param useOffset Wether to use the offset 00093 * @return The offset set 00094 */ 00095 RGBC setOffset(bool useOffset = true); 00096 00097 00098 /** 00099 * Use the offset registers to automatically subtract offset from the readings 00100 * 00101 * @param useOffset Wether to use the offset 00102 */ 00103 void offsetMode(bool useOffset); 00104 00105 /** 00106 * Read in the color value from the sensor 00107 * 00108 * @return Structure containing the value of red, green, blue and clear 00109 */ 00110 RGBC read(); 00111 00112 /** 00113 * Get the gain of number of capacitor for each channel, in the range of 0 to 00114 * 15. Less capacitor will give higher sensitivity. 00115 * 00116 * @return Structure containing the gain of number of capacitor for each 00117 * channel. 00118 */ 00119 RGBC getColorCap(); 00120 00121 /** 00122 * Get the gain of number of integration time slot, in the range of 0 to 4095. 00123 * More integration time slot will give higher sensitivity. 00124 * 00125 * @return Structure containing the gain of number of integration time 00126 * slot for each channel. 00127 */ 00128 RGBC getColorInt(); 00129 00130 /** 00131 * Set the gain of number of capacitor for each channel, in the range of 0 to 00132 * 15. Less capacitor will give higher sensitivity. 00133 * 00134 * @param red gain value for red 00135 * @param green gain value for green 00136 * @param blue gain value for blue 00137 * @param clear gain value for clear 00138 */ 00139 void setColorCap(int red, int green, int blue, int clear); 00140 00141 /** 00142 * Set the gain of number of integration time slot, in the range of 0 to 4095. 00143 * More integration time slot will give higher sensitivity. 00144 * 00145 * @param red gain value for red 00146 * @param green gain value for green 00147 * @param blue gain value for blue 00148 * @param clear gain value for clear 00149 */ 00150 void setColorInt(int red, int green, int blue, int clear); 00151 00152 private: 00153 00154 // fields 00155 I2C _i2c; 00156 DigitalOut _led; 00157 00158 RGBC colorCap; 00159 RGBC colorInt; 00160 RGBC colorOffset; 00161 00162 // private memeber functions 00163 00164 /** Write a byte of data to ADJD-S311 register 00165 * 00166 * @param data byte data to write to the register 00167 * @param regAddr address of the register to write 00168 */ 00169 void writeRegister(char data, char regAddr); 00170 00171 /** Read a byte of data from ADJD-S311 register 00172 * 00173 * @param regAddr address of the register to write 00174 * @retrun byte data read from the register 00175 */ 00176 char readRegister(char regAddr); 00177 00178 /** Read 2 bytes of data from ADJD-S311 registers and return as an integer 00179 * 00180 * @param loRegAddr low register address 00181 * @return value from registers as an int 00182 */ 00183 int readInt(char loRegAddr); 00184 00185 /** Write an integer data of 2 bytes to ADJD-S311 registers 00186 * 00187 * @param loRegAddr low register address 00188 * @param data integer value to write 00189 */ 00190 void writeInt(int data, char loRegAddr); 00191 00192 /** 00193 * Tell the color sensor to perform measurement and store data to the color and 00194 * clear registers. Must be called before reading 00195 * color values 00196 */ 00197 void performMeasurement(); 00198 00199 /** 00200 * Calibrate the clear integration time slot so that current reading of the 00201 * clear channel will be as close to 1000 as possible 00202 */ 00203 void calibrateClearInt(); 00204 00205 /** 00206 * Calibrate the color integration time slots so that the max current reading 00207 * of the RGB channels will be as close to 1000 as possible 00208 */ 00209 void calibrateColorInt(); 00210 00211 /** 00212 * Calibrate the color capacitors so the difference of reading of different 00213 * channels are as small as possible 00214 */ 00215 void calibrateCapacitors(); 00216 }; 00217 00218 #endif
Generated on Thu Jul 14 2022 17:07:11 by
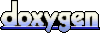