
This code will be implemented on the receiving end of the device, which contains a speaker, an Emic2 text-to-speech converter, as well as a vehicle module that can move or rotate upon request
Dependencies: mbed 4DGL-uLCD-SE emic2 PinDetect
main.cpp
00001 #include "mbed.h" 00002 #include "emic2.h" 00003 #include "uLCD_4DGL.h" 00004 #include<string> 00005 00006 using namespace std; 00007 //serial RX,TX pins to emic 00008 uLCD_4DGL uLCD(p28,p27,p5); // serial tx, serial rx, reset pin; 00009 #define length_sprite 20 00010 #define width_sprite 75 00011 #define width_sprite2 47 00012 #define length_sprite2 18 00013 00014 #define Q 0x808000 //OLIVE 00015 #define I 0x008000 //GREEN 00016 #define S 0xC0C0C0 //SILVER 00017 #define R 0xF1C40F //YELLOW 00018 #define O 0xF39C12 //ORANGE 00019 #define X 0xFFFFFF //WHITE 00020 #define B 0x0000FF //BLUE 00021 #define G 0xAAB7B8 //GREY 00022 #define D 0x797D7F //DARK GREY 00023 #define L 0x00FF00 //LIME 00024 #define P 0xFF00FF //K 00025 #define _ 0x000000 //BLACK 00026 #define r 0xFF0000 //RED 00027 00028 00029 class TMP36{ 00030 public: 00031 TMP36(PinName pin); 00032 TMP36(); 00033 float read(); 00034 private: 00035 //class sets up the AnalogIn pin 00036 AnalogIn _pin; 00037 }; 00038 TMP36::TMP36(PinName pin) : _pin(pin) {} //This is an initializer list 00039 float TMP36::read() 00040 { 00041 //convert sensor reading to temperature in degrees C 00042 return ((_pin.read()*3.3)-0.500)*100.0; 00043 //read() function returns a normalized value of the voltage from 0 to 1 as a float } 00044 //instantiate new class to set p15 to analog input //to read and convert TMP36 sensor's voltage output 00045 } 00046 TMP36 myTMP36(p20); // analog in pin for temp sensor 00047 00048 00049 // make 3 different sprites 00050 // decrease the sprite dimensions to increasing processing speed 00051 // for the glove sprite appearing in the beginning 00052 int Glove[length_sprite*width_sprite] = { 00053 _,_,_,_,R,_,_,_,_,_,_,_,_,_,_,_,_,_,_,_,_,_,R,_,S,S,S,S,S,S,_,O,_,_,_,_,_,r,r,r,r,r,_,D,D,D,D,D,D,_,I,I,_,_,_,_,_,_,_,I,I,_,R,R,R,R,R,R,_,_,_,_,_,_, 00054 _,_,_,_,_,R,_,_,_,_,_,_,_,_,_,_,_,_,_,_,_,R,_,_,S,_,_,_,_,_,_,O,_,_,_,_,_,r,_,_,_,_,_,D,_,_,_,_,D,_,I,_,I,_,_,_,_,_,I,_,I,_,R,_,_,_,_,_,_,_,_,_,_,_, 00055 _,_,_,_,_,_,R,_,_,_,_,_,R,R,_,_,_,_,_,_,R,_,_,_,S,_,_,_,_,_,_,O,_,_,_,_,_,r,_,_,_,_,_,D,_,_,_,_,D,_,I,_,_,I,_,_,_,I,_,_,I,_,R,_,_,_,_,_,_,_,_,_,_,_, 00056 _,_,_,_,_,_,_,R,_,_,_,R,_,_,R,_,_,_,_,R,_,_,_,_,S,S,S,S,_,_,_,O,_,_,_,_,_,r,_,_,_,_,_,D,_,_,_,_,D,_,I,_,_,_,I,_,I,_,_,_,I,_,R,R,R,R,_,_,_,_,_,_,_,_, 00057 _,_,_,_,_,_,_,_,R,_,R,_,_,_,_,R,_,_,R,_,_,_,_,_,S,_,_,_,_,_,_,O,_,_,_,_,_,r,_,_,_,_,_,D,_,_,_,_,D,_,I,_,_,_,_,I,_,_,_,_,I,_,R,_,_,_,_,_,_,_,_,_,_,_, 00058 _,_,_,_,_,_,_,_,_,R,_,_,_,_,_,_,R,R,_,_,_,_,_,_,S,_,_,_,_,_,_,O,_,_,_,_,_,r,_,_,_,_,_,D,_,_,_,_,D,_,I,_,_,_,_,_,_,_,_,_,I,_,R,_,_,_,_,_,_,_,_,_,_,_, 00059 _,_,_,_,_,_,_,_,_,_,_,_,_,_,_,_,_,_,_,_,_,_,_,_,S,S,S,S,S,S,_,O,O,O,O,O,_,r,r,r,r,r,_,D,D,D,D,D,D,_,I,_,_,_,_,_,_,_,_,_,I,_,R,R,R,R,R,R,_,_,_,_,_,_, 00060 _,_,_,_,_,_,_,_,_,_,_,_,_,_,_,_,_,_,_,_,_,_,_,_,_,_,_,_,_,_,_,_,_,_,_,_,_,_,_,_,_,_,_,_,_,_,_,_,_,_,_,_,_,_,_,_,_,_,_,_,_,_,_,_,_,_,_,_,_,_,_,_,_,_, 00061 _,_,_,_,_,_,_,_,_,_,_,_,_,_,_,_,_,_,_,_,_,_,_,_,_,_,_,_,_,_,_,_,_,_,_,_,_,_,_,_,_,_,_,_,_,_,_,_,_,_,_,_,_,_,_,_,_,_,_,_,_,_,_,_,_,_,_,_,_,_,_,_,_,_, 00062 _,_,_,_,_,_,_,_,_,_,_,_,_,_,_,_,_,_,_,_,_,_,_,_,_,_,_,_,_,_,_,_,_,_,_,_,_,_,_,_,_,_,_,_,_,_,_,_,_,_,_,_,_,_,_,_,_,_,_,_,_,_,_,_,_,_,_,_,_,_,_,_,_,_, 00063 _,_,_,_,_,_,_,_,_,_,_,_,_,_,_,_,_,_,_,_,_,G,G,G,G,G,G,G,G,G,_,_,B,B,B,B,B,B,B,_,_,_,_,_,_,_,_,_,_,_,_,_,_,_,_,_,_,_,_,_,_,_,_,_,_,_,_,_,_,_,_,_,_,_, 00064 _,_,_,_,_,_,_,_,_,_,_,_,_,_,_,_,_,_,_,_,_,_,_,_,_,G,_,_,_,_,_,_,B,_,_,_,_,_,B,_,_,_,_,_,_,_,_,_,_,_,_,_,_,_,_,_,_,_,_,_,_,_,_,_,_,_,_,_,_,_,_,_,_,_, 00065 _,_,_,_,_,_,_,_,_,_,_,_,_,_,_,_,_,_,_,_,_,_,_,_,_,G,_,_,_,_,_,_,B,_,_,_,_,_,B,_,_,_,_,_,_,_,_,_,_,_,_,_,_,_,_,_,_,_,_,_,_,_,_,_,_,_,_,_,_,_,_,_,_,_, 00066 _,_,_,_,_,_,_,_,_,_,_,_,_,_,_,_,_,_,_,_,_,_,_,_,_,G,_,_,_,_,_,_,B,_,_,_,_,_,B,_,_,_,_,_,_,_,_,_,_,_,_,_,_,_,_,_,_,_,_,_,_,_,_,_,_,_,_,_,_,_,_,_,_,_, 00067 _,_,_,_,_,_,_,_,_,_,_,_,_,_,_,_,_,_,_,_,_,_,_,_,_,G,_,_,_,_,_,_,B,_,_,_,_,_,B,_,_,_,_,_,_,_,_,_,_,_,_,_,_,_,_,_,_,_,_,_,_,_,_,_,_,_,_,_,_,_,_,_,_,_, 00068 _,_,_,_,_,_,_,_,_,_,_,_,_,_,_,_,_,_,_,_,_,_,_,_,_,G,_,_,_,_,_,_,B,_,_,_,_,_,B,_,_,_,_,_,_,_,_,_,_,_,_,_,_,_,_,_,_,_,_,_,_,_,_,_,_,_,_,_,_,_,_,_,_,_, 00069 _,_,_,_,_,_,_,_,_,_,_,_,_,_,_,_,_,_,_,_,_,_,_,_,_,G,_,_,_,_,_,_,B,_,_,_,_,_,B,_,_,_,_,_,_,_,_,_,_,_,_,_,_,_,_,_,_,_,_,_,_,_,_,_,_,_,_,_,_,_,_,_,_,_, 00070 _,_,_,_,_,_,_,_,_,_,_,_,_,_,_,_,_,_,_,_,_,_,_,_,_,G,_,_,_,_,_,_,B,_,_,_,_,_,B,_,_,_,_,_,_,_,_,_,_,_,_,_,_,_,_,_,_,_,_,_,_,_,_,_,_,_,_,_,_,_,_,_,_,_, 00071 _,_,_,_,_,_,_,_,_,_,_,_,_,_,_,_,_,_,_,_,_,_,_,_,_,G,_,_,_,_,_,_,B,B,B,B,B,B,B,_,_,_,_,_,_,_,_,_,_,_,_,_,_,_,_,_,_,_,_,_,_,_,_,_,_,_,_,_,_,_,_,_,_,_, 00072 _,_,_,_,_,_,_,_,_,_,_,_,_,_,_,_,_,_,_,_,_,_,_,_,_,_,_,_,_,_,_,_,_,_,_,_,_,_,_,_,_,_,_,_,_,_,_,_,_,_,_,_,_,_,_,_,_,_,_,_,_,_,_,_,_,_,_,_,_,_,_,_,_,_, 00073 }; 00074 00075 00076 int Fourty_One_Eighty[width_sprite2*length_sprite2]= { 00077 _,_,_,_,_,_,_,_,_,_,_,_,_,_,_,_,_,_,_,_,_,_,_,_,_,_,_,_,_,_,_,_,_,_,_,_,_,_,_,_,_,_,_,_,_,_,_, 00078 _,_,_,_,_,_,_,_,_,_,_,_,L,_,_,_,_,O,O,O,_,_,_,_,_,G,G,G,G,G,G,G,G,G,_,_,_,B,B,B,B,B,B,B,B,B,_, 00079 _,_,_,_,_,_,_,_,_,_,_,L,L,_,_,_,_,O,O,O,_,_,_,_,_,G,_,_,_,_,_,_,_,G,_,_,_,B,_,_,_,_,_,_,_,B,_, 00080 _,_,_,_,_,_,_,_,_,_,L,_,L,_,_,_,_,O,O,O,_,_,_,_,_,G,_,_,_,_,_,_,_,G,_,_,_,B,_,_,_,_,_,_,_,B,_, 00081 _,_,_,_,_,_,_,_,_,L,_,_,L,_,_,_,_,O,O,O,_,_,_,_,_,G,_,_,_,_,_,_,_,G,_,_,_,B,_,_,_,_,_,_,_,B,_, 00082 _,_,_,_,_,_,_,_,L,_,_,_,L,_,_,_,_,O,O,O,_,_,_,_,_,G,_,_,_,_,_,_,_,G,_,_,_,B,_,_,_,_,_,_,_,B,_, 00083 _,_,_,_,_,_,_,L,_,_,_,_,L,_,_,_,_,O,O,O,_,_,_,_,_,G,_,_,_,_,_,_,_,G,_,_,_,B,_,_,_,_,_,_,_,B,_, 00084 _,_,_,_,_,_,L,_,_,_,_,_,L,_,_,_,_,O,O,O,_,_,_,_,_,G,_,_,_,_,_,_,_,G,_,_,_,B,_,_,_,_,_,_,_,B,_, 00085 _,_,_,_,_,L,L,L,L,L,L,L,L,_,_,_,_,O,O,O,_,_,_,_,_,G,G,G,G,G,G,G,G,G,_,_,_,B,_,_,_,_,_,_,_,B,_, 00086 _,_,_,_,_,_,_,_,_,_,_,_,L,_,_,_,_,O,O,O,_,_,_,_,_,G,G,G,G,G,G,G,G,G,_,_,_,B,_,_,_,_,_,_,_,B,_, 00087 _,_,_,_,_,_,_,_,_,_,_,_,L,_,_,_,_,O,O,O,_,_,_,_,_,G,_,_,_,_,_,_,_,G,_,_,_,B,_,_,_,_,_,_,_,B,_, 00088 _,_,_,_,_,_,_,_,_,_,_,_,L,_,_,_,_,O,O,O,_,_,_,_,_,G,_,_,_,_,_,_,_,G,_,_,_,B,_,_,_,_,_,_,_,B,_, 00089 _,_,_,_,_,_,_,_,_,_,_,_,L,_,_,_,_,O,O,O,_,_,_,_,_,G,_,_,_,_,_,_,_,G,_,_,_,B,_,_,_,_,_,_,_,B,_, 00090 _,_,_,_,_,_,_,_,_,_,_,_,L,_,_,_,_,O,O,O,_,_,_,_,_,G,_,_,_,_,_,_,_,G,_,_,_,B,_,_,_,_,_,_,_,B,_, 00091 _,_,_,_,_,_,_,_,_,_,_,_,L,_,_,_,_,O,O,O,_,_,_,_,_,G,_,_,_,_,_,_,_,G,_,_,_,B,_,_,_,_,_,_,_,B,_, 00092 _,_,_,_,_,_,_,_,_,_,_,_,L,_,_,_,_,O,O,O,_,_,_,_,_,G,_,_,_,_,_,_,_,G,_,_,_,B,_,_,_,_,_,_,_,B,_, 00093 _,_,_,_,_,_,_,_,_,_,_,_,L,_,_,_,_,O,O,O,_,_,_,_,_,G,G,G,G,G,G,G,G,G,_,_,_,B,B,B,B,B,B,B,B,B,_, 00094 _,_,_,_,_,_,_,_,_,_,_,_,_,_,_,_,_,_,_,_,_,_,_,_,_,_,_,_,_,_,_,_,_,_,_,_,_,_,_,_,_,_,_,_,_,_,_, 00095 00096 }; 00097 00098 00099 Serial xbee1(p9,p10); 00100 DigitalOut rst1(p11); 00101 00102 DigitalOut myled1(LED1); 00103 DigitalOut myled2(LED2); 00104 DigitalOut myled3(LED3); 00105 DigitalOut myled4(LED4); 00106 //emic2 myTTS(p13, p14); 00107 AnalogIn a1(p15); 00108 AnalogIn a2(p16); 00109 AnalogIn a3(p17); 00110 AnalogIn a4(p18); 00111 AnalogIn a5(p19); 00112 Serial pc(USBTX, USBRX); 00113 float i1; 00114 float i2; 00115 float i3; 00116 float i4; 00117 float i5; 00118 float ref1; 00119 float ref2; 00120 float ref3; 00121 float ref4; 00122 float ref5; 00123 float avg1; 00124 float avg2; 00125 float avg3; 00126 float avg4; 00127 float avg5; 00128 float diff1, diff2, diff3, diff4, diff5; 00129 int counter; 00130 float sum; 00131 int main() { 00132 float tempC, tempF; 00133 int i = 0; 00134 uLCD.BLIT(30,30,width_sprite, length_sprite, Glove); 00135 wait(1); 00136 uLCD.cls(); 00137 00138 uLCD.BLIT(30,30, width_sprite2, length_sprite2, Fourty_One_Eighty); 00139 00140 wait(1.0); 00141 00142 00143 for (unsigned int j = 0; j <= 128; j+=7){ 00144 // DRAW ---- animation of walls 00145 uLCD.line(j,127,j+7,0,RED); // horizontal animation 00146 uLCD.line(j+1, 127, j+8, 0, RED); 00147 00148 uLCD.line(127 - j, 0, 127 - (j+7), 127, BLUE); 00149 uLCD.line(127-j-1,0,127 - (j+8), 127, BLUE); 00150 00151 // Erase 00152 wait(.1); 00153 uLCD.line(j,127,j+7,0,BLACK); 00154 uLCD.line(j+1, 127, j+8, 0, BLACK); 00155 uLCD.line(127 - j, 0, 127 - (j+7), 127, BLACK); // vertical animation 00156 uLCD.line(127-j-1,0,127 - (j+8), 127, BLACK); 00157 } 00158 00159 uLCD.cls(); 00160 00161 00162 00163 ref1 = 0; 00164 ref2 = 0; 00165 ref3 = 0; 00166 ref4 = 0; 00167 ref5 = 0; 00168 counter = 0; 00169 rst1 = 0; 00170 wait_ms(1); 00171 rst1 = 1; 00172 wait_ms(1); 00173 char o; 00174 while(1) { 00175 wait(0.5); 00176 i1 = a1; 00177 i2 = a2; 00178 i3 = a3; 00179 i4 = a4; 00180 i5 = a5; 00181 ref1 += i1; 00182 ref2 += i2; 00183 ref3 += i3; 00184 ref4 += i4; 00185 ref5 += i5; 00186 avg1 = ref1 / counter; 00187 avg2 = ref2 / counter; 00188 avg3 = ref3 / counter; 00189 avg4 = ref4 / counter; 00190 avg5 = ref5 / counter; 00191 o = 'I'; 00192 myled1 = 0; 00193 myled2 = 0; 00194 myled3 = 0; 00195 myled4 = 0; 00196 diff1 = i1 - avg1; 00197 diff2 = i2 - avg2; 00198 diff3 = i3 - avg3; 00199 diff4 = i4 - avg4; 00200 diff5 = i5 - avg5; 00201 if (diff1 > 0.01) { 00202 o = 'A'; 00203 ref1 -= i1; 00204 myled1 = 1; 00205 } else if (diff2 > 0.01) 00206 { 00207 o = 'B'; 00208 ref2 -= i2; 00209 myled2 = 1; 00210 } else if (diff3 > 0.01) 00211 { 00212 o = 'C'; 00213 ref3 -= i3; 00214 myled3 = 1; 00215 } else if (diff4 > 0.01) 00216 { 00217 o = 'D'; 00218 ref4 -= i4; 00219 myled4 = 1; 00220 } else if (diff5 > 0.01) 00221 { 00222 o = 'E'; 00223 ref5 -= i5; 00224 myled1 = 1; 00225 myled2 = 1; 00226 } else { 00227 counter++; 00228 } 00229 xbee1.putc(o); 00230 00231 pc.printf("%4.2f, %4.2f\r\n", avg4, diff4); 00232 uLCD.filled_circle(63,63,63, 0x0000ff); 00233 00234 uLCD.baudrate(3000000); 00235 //uLCD.set_font_size(3,3); 00236 /* 00237 uLCD.text_char('B', 9, 8, BLACK); 00238 uLCD.text_char('I',10, 8, BLACK); 00239 uLCD.text_char('G',11, 8, BLACK); 00240 uLCD.text_italic(ON); */ 00241 if (i == 0){ 00242 uLCD.locate(40,40); 00243 uLCD.text_italic(ON); 00244 uLCD.text_string("Welcome to 4180", 0, 5, FONT_7X8, WHITE); 00245 uLCD.text_italic(ON); 00246 wait(0.2); 00247 uLCD.cls(); 00248 uLCD.text_string("Final", 0, 9, FONT_7X8, WHITE); 00249 uLCD.text_string("Project", 0, 11, FONT_7X8, WHITE); 00250 00251 i++; uLCD.cls(); 00252 uLCD.filled_circle(63,63,63, 0x0000ff); 00253 wait(0.2); 00254 } 00255 00256 tempC = myTMP36.read(); //convert to degrees F 00257 tempF = (9.0*tempC)/5.0 + 32.0; //print current temp 00258 00259 uLCD.locate(0,0); 00260 uLCD.printf("%5.2f C %5.2f F \n\r", tempC, tempF); 00261 00262 00263 uLCD.filled_rectangle(40, 127, 50, 127 - 2*tempC , 0xFF0000); // MAX- Celcius 64 for celcius bar 00264 uLCD.filled_rectangle(60, 127, 70, 127 - tempF, 0xFFFF00); // MAX- farenheit yellow for farenheit bar 00265 wait(0.2); 00266 // uLCD.cls(); 00267 uLCD.filled_rectangle(0,0,127, 7, 0x000000); 00268 uLCD.filled_circle(63,63,63, 0x0000ff); 00269 uLCD.filled_rectangle(40, 127, 50, 127 - 2*tempC , 0x000000); // ERASE 00270 uLCD.filled_rectangle(60, 127, 70, 127 - tempF, 0x000000); // MAX- farenheit yellow for farenheit bar 00271 } 00272 }
Generated on Sat Jul 16 2022 05:58:17 by
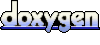