An I2C Scanner, to test that your I2C can be detected. Works with the NRF51 Development Kit.
Dependencies: mbed-src-nrf51822
main.cpp
00001 #include "mbed.h" 00002 #include "wire.h" 00003 00004 #define SCL 7 00005 #define SDA 30 00006 00007 TwoWire Wire = TwoWire(NRF_TWI1); 00008 00009 int main(void) 00010 { 00011 Wire.begin(SCL, SDA, TWI_FREQUENCY_100K); 00012 printf("IIC Demo Start \r\n"); 00013 00014 while(1) 00015 { 00016 int nDevices = 0; 00017 for(int address = 1; address < 127; address++ ) 00018 { 00019 int error = -1; 00020 Wire.beginTransmission(address << 1); 00021 Wire.write(0x00); 00022 error = Wire.endTransmission(); 00023 00024 if (error == 0) 00025 { 00026 printf("I2C device found at address %d 0x%X %d \n\r",address, address, error); //Returns 7-bit address 00027 nDevices++; 00028 } 00029 } 00030 if (nDevices == 0) 00031 { 00032 printf("No I2C devices found\n\r"); 00033 } 00034 else{} 00035 printf("\ndone\n\r"); 00036 00037 wait(2); // wait 2 seconds for next scan 00038 00039 } 00040 } 00041
Generated on Wed Jul 13 2022 05:37:38 by
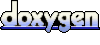