
The experiment using this program is introduced on "Interface" No.2, CQ publishing Co.,Ltd, 2015. 本プログラムを使った実験は,CQ出版社のインターフェース 2015年2月号で紹介しています.
Dependencies: DSProcessingIO mbed
Biquad.hpp
00001 //-------------------------------------------------------------- 00002 // Biquad filter for IIR filter of cascade structure 00003 // Copyright (c) 2014 MIKAMI, Naoki, 2014/07/15 00004 //-------------------------------------------------------------- 00005 00006 #ifndef IIR_BIQUAD_HPP 00007 #define IIR_BIQUAD_HPP 00008 00009 #include "mbed.h" 00010 00011 namespace Mikami 00012 { 00013 // 2nd order IIR filter 00014 class Biquad 00015 { 00016 public: 00017 struct Coefs { float a1, a2, b1, b2; }; 00018 00019 Biquad() {} // Default constructore 00020 00021 Biquad(const Coefs ck) 00022 : a1_(ck.a1), a2_(ck.a2), b1_(ck.b1), b2_(ck.b2) 00023 { Clear(); } 00024 00025 float Execute(float xn) 00026 { 00027 float un = xn + a1_*un1_ + a2_*un2_; 00028 float yn = un + b1_*un1_ + b2_*un2_; 00029 00030 un2_ = un1_; 00031 un1_ = un; 00032 00033 return yn; 00034 } 00035 00036 void Clear() { un1_ = un2_ = 0; } 00037 00038 private: 00039 float a1_, a2_, b1_, b2_; 00040 float un1_, un2_; 00041 00042 Biquad(const Biquad&); 00043 }; 00044 } 00045 #endif // IIR_BIQUAD_HPP
Generated on Wed Jul 13 2022 09:59:17 by
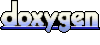