Source lists for AD converter is explained on "Interface" No.10, CQ publishing Co.,Ltd, 2014. Source lists for DA converter is explained on "Interface" No.11, CQ publishing Co.,Ltd, 2014. 本ライブラリのADコンバータ用のソースリストについては,CQ出版社のインターフェース 2014年10月号に載っています. DAコンバータ用のソースリストについての説明は,CQ出版社のインターフェース 2014年11月号に載っています.
Dependents: FFT_Sampling FIR_LPF_Direct FIR_LPF_Symmetry IIR_LPF ... more
Fork of SignalProcessingIO by
MCP4922Single.cpp
00001 //------------------------------------------------------ 00002 // Class for 1 DAC in MCP4922 00003 // 00004 // Copyright (c) 2014 MIKAMI, Naoki, 2014/06/18 00005 //------------------------------------------------------ 00006 00007 #include "MCP4922Single.hpp" 00008 00009 namespace Mikami 00010 { 00011 Dac::Dac(DAC dac, PinName mosi, PinName sclk, 00012 PinName cs, PinName ldac, int hz) 00013 : wcr_(dac | 0x3000), mySpi_(mosi, NC, sclk), 00014 myCs_(cs, 1), myLd_(ldac, 0) 00015 { 00016 // Set SPI format and bus frequency 00017 mySpi_.format(16, 0); 00018 mySpi_.frequency(hz); 00019 00020 // Set DAC to 0 00021 WriteDac(0); 00022 } 00023 00024 void Dac::Write(float value) 00025 { 00026 if (value < -1.0f) value = -1.0f; 00027 if (value > 1.0f) value = 1.0f; 00028 00029 WriteDac((uint16_t)((value + 1.0f)*2047)); 00030 } 00031 00032 void Dac::Write(uint16_t value) 00033 { 00034 WriteDac((value > 4095) ? 4095 : value); 00035 } 00036 00037 void Dac::Ldac() 00038 { 00039 myLd_.write(0); 00040 wait_us(1); 00041 myLd_.write(1); 00042 } 00043 00044 void Dac::WriteDac(uint16_t value) 00045 { 00046 myCs_.write(0); // cs <= L 00047 mySpi_.write(value | wcr_); 00048 myCs_.write(1); // cs <= H 00049 } 00050 }
Generated on Tue Jul 12 2022 16:21:17 by
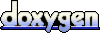