
This is WIZnet Ethernet Interface using Hardware TCP/IP chip, W5500, W5200 and W5100. One of them can be selected by enabling it in wiznet.h.
Fork of WIZnet_Library by
W5500.cpp
00001 /* Copyright (C) 2012 mbed.org, MIT License 00002 * 00003 * Permission is hereby granted, free of charge, to any person obtaining a copy of this software 00004 * and associated documentation files (the "Software"), to deal in the Software without restriction, 00005 * including without limitation the rights to use, copy, modify, merge, publish, distribute, 00006 * sublicense, and/or sell copies of the Software, and to permit persons to whom the Software is 00007 * furnished to do so, subject to the following conditions: 00008 * 00009 * The above copyright notice and this permission notice shall be included in all copies or 00010 * substantial portions of the Software. 00011 * 00012 * THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR IMPLIED, INCLUDING 00013 * BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY, FITNESS FOR A PARTICULAR PURPOSE AND 00014 * NONINFRINGEMENT. IN NO EVENT SHALL THE AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, 00015 * DAMAGES OR OTHER LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM, 00016 * OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE SOFTWARE. 00017 */ 00018 00019 #include "mbed.h" 00020 #include "mbed_debug.h" 00021 #include "wiznet.h" 00022 #include "DNSClient.h" 00023 00024 #ifdef USE_W5500 00025 //Debug is disabled by default 00026 #if 0 00027 #define DBG(...) do{debug("%p %d %s ", this,__LINE__,__PRETTY_FUNCTION__); debug(__VA_ARGS__); } while(0); 00028 //#define DBG(x, ...) debug("[W5500:DBG]"x"\r\n", ##__VA_ARGS__); 00029 #define WARN(x, ...) debug("[W5500:WARN]"x"\r\n", ##__VA_ARGS__); 00030 #define ERR(x, ...) debug("[W5500:ERR]"x"\r\n", ##__VA_ARGS__); 00031 #else 00032 #define DBG(x, ...) 00033 #define WARN(x, ...) 00034 #define ERR(x, ...) 00035 #endif 00036 00037 #if 1 00038 #define INFO(x, ...) debug("[W5500:INFO]"x"\r\n", ##__VA_ARGS__); 00039 #else 00040 #define INFO(x, ...) 00041 #endif 00042 00043 #define DBG_SPI 0 00044 00045 WIZnet_Chip* WIZnet_Chip::inst; 00046 00047 WIZnet_Chip::WIZnet_Chip(PinName mosi, PinName miso, PinName sclk, PinName _cs, PinName _reset): 00048 cs(_cs), reset_pin(_reset) 00049 { 00050 spi = new SPI(mosi, miso, sclk); 00051 cs = 1; 00052 reset_pin = 1; 00053 inst = this; 00054 } 00055 00056 WIZnet_Chip::WIZnet_Chip(SPI* spi, PinName _cs, PinName _reset): 00057 cs(_cs), reset_pin(_reset) 00058 { 00059 this->spi = spi; 00060 cs = 1; 00061 reset_pin = 1; 00062 inst = this; 00063 } 00064 00065 // Set the IP 00066 bool WIZnet_Chip::setip() 00067 { 00068 reg_wr<uint32_t>(SIPR, ip); 00069 reg_wr<uint32_t>(GAR, gateway); 00070 reg_wr<uint32_t>(SUBR, netmask); 00071 return true; 00072 } 00073 00074 bool WIZnet_Chip::setProtocol(int socket, Protocol p) 00075 { 00076 if (socket < 0) { 00077 return false; 00078 } 00079 sreg<uint8_t>(socket, Sn_MR, p); 00080 return true; 00081 } 00082 00083 bool WIZnet_Chip::connect(int socket, const char * host, int port, int timeout_ms) 00084 { 00085 if (socket < 0) { 00086 return false; 00087 } 00088 sreg<uint8_t>(socket, Sn_MR, TCP); 00089 scmd(socket, OPEN); 00090 sreg_ip(socket, Sn_DIPR, host); 00091 sreg<uint16_t>(socket, Sn_DPORT, port); 00092 sreg<uint16_t>(socket, Sn_PORT, new_port()); 00093 scmd(socket, CONNECT); 00094 Timer t; 00095 t.reset(); 00096 t.start(); 00097 while(!is_connected(socket)) { 00098 if (t.read_ms() > timeout_ms) { 00099 return false; 00100 } 00101 } 00102 return true; 00103 } 00104 00105 bool WIZnet_Chip::gethostbyname(const char* host, uint32_t* ip) 00106 { 00107 uint32_t addr = str_to_ip(host); 00108 char buf[17]; 00109 snprintf(buf, sizeof(buf), "%d.%d.%d.%d", (addr>>24)&0xff, (addr>>16)&0xff, (addr>>8)&0xff, addr&0xff); 00110 if (strcmp(buf, host) == 0) { 00111 *ip = addr; 00112 return true; 00113 } 00114 DNSClient client; 00115 if(client.lookup(host)) { 00116 *ip = client.ip; 00117 return true; 00118 } 00119 return false; 00120 } 00121 00122 bool WIZnet_Chip::disconnect() 00123 { 00124 return true; 00125 } 00126 00127 bool WIZnet_Chip::is_connected(int socket) 00128 { 00129 if (sreg<uint8_t>(socket, Sn_SR) == SOCK_ESTABLISHED) { 00130 return true; 00131 } 00132 return false; 00133 } 00134 00135 // Reset the chip & set the buffer 00136 void WIZnet_Chip::reset() 00137 { 00138 reset_pin = 1; 00139 reset_pin = 0; 00140 wait_us(500); // 500us (w5500) 00141 reset_pin = 1; 00142 wait_ms(400); // 400ms (w5500) 00143 00144 #if defined(USE_WIZ550IO_MAC) 00145 reg_rd_mac(SHAR, mac); // read the MAC address inside the module 00146 #endif 00147 00148 reg_wr_mac(SHAR, mac); 00149 00150 // set RX and TX buffer size 00151 for (int socket = 0; socket < MAX_SOCK_NUM; socket++) { 00152 sreg<uint8_t>(socket, Sn_RXBUF_SIZE, 2); 00153 sreg<uint8_t>(socket, Sn_TXBUF_SIZE, 2); 00154 } 00155 } 00156 00157 00158 bool WIZnet_Chip::close(int socket) 00159 { 00160 if (socket < 0) { 00161 return false; 00162 } 00163 // if not connected, return 00164 if (sreg<uint8_t>(socket, Sn_SR) == SOCK_CLOSED) { 00165 return true; 00166 } 00167 if (sreg<uint8_t>(socket, Sn_MR) == TCP) { 00168 scmd(socket, DISCON); 00169 } 00170 scmd(socket, CLOSE); 00171 sreg<uint8_t>(socket, Sn_IR, 0xff); 00172 return true; 00173 } 00174 00175 int WIZnet_Chip::wait_readable(int socket, int wait_time_ms, int req_size) 00176 { 00177 if (socket < 0) { 00178 return -1; 00179 } 00180 Timer t; 00181 t.reset(); 00182 t.start(); 00183 while(1) { 00184 int size = sreg<uint16_t>(socket, Sn_RX_RSR); 00185 if (size > req_size) { 00186 return size; 00187 } 00188 if (wait_time_ms != (-1) && t.read_ms() > wait_time_ms) { 00189 break; 00190 } 00191 } 00192 return -1; 00193 } 00194 00195 int WIZnet_Chip::wait_writeable(int socket, int wait_time_ms, int req_size) 00196 { 00197 if (socket < 0) { 00198 return -1; 00199 } 00200 Timer t; 00201 t.reset(); 00202 t.start(); 00203 while(1) { 00204 int size = sreg<uint16_t>(socket, Sn_TX_FSR); 00205 if (size > req_size) { 00206 return size; 00207 } 00208 if (wait_time_ms != (-1) && t.read_ms() > wait_time_ms) { 00209 break; 00210 } 00211 } 00212 return -1; 00213 } 00214 00215 int WIZnet_Chip::send(int socket, const char * str, int len) 00216 { 00217 if (socket < 0) { 00218 return -1; 00219 } 00220 uint16_t ptr = sreg<uint16_t>(socket, Sn_TX_WR); 00221 uint8_t cntl_byte = (0x14 + (socket << 5)); 00222 spi_write(ptr, cntl_byte, (uint8_t*)str, len); 00223 sreg<uint16_t>(socket, Sn_TX_WR, ptr + len); 00224 scmd(socket, SEND); 00225 00226 uint8_t tmp_Sn_IR; 00227 // while ((sreg<uint8_t>(socket, Sn_IR) & INT_SEND_OK) != INT_SEND_OK) { 00228 while (( (tmp_Sn_IR = sreg<uint8_t>(socket, Sn_IR)) & INT_SEND_OK) != INT_SEND_OK) { 00229 /* 00230 if (sreg<uint8_t>(socket, Sn_SR) == CLOSED) { 00231 close(socket); 00232 return 0; 00233 } 00234 */ 00235 // @Jul.10, 2014 fix contant name, and udp sendto function. 00236 switch (sreg<uint8_t>(socket, Sn_SR)) { 00237 case SOCK_CLOSED : 00238 close(socket); 00239 return 0; 00240 //break; 00241 case SOCK_UDP : 00242 // ARP timeout is possible. 00243 if ((tmp_Sn_IR & INT_TIMEOUT) == INT_TIMEOUT) { 00244 sreg<uint8_t>(socket, Sn_IR, INT_TIMEOUT); 00245 return 0; 00246 } 00247 break; 00248 default : 00249 break; 00250 } 00251 } 00252 sreg<uint8_t>(socket, Sn_IR, INT_SEND_OK); 00253 00254 return len; 00255 } 00256 00257 int WIZnet_Chip::recv(int socket, char* buf, int len) 00258 { 00259 if (socket < 0) { 00260 return -1; 00261 } 00262 uint16_t ptr = sreg<uint16_t>(socket, Sn_RX_RD); 00263 uint8_t cntl_byte = (0x18 + (socket << 5)); 00264 spi_read(ptr, cntl_byte, (uint8_t*)buf, len); 00265 sreg<uint16_t>(socket, Sn_RX_RD, ptr + len); 00266 scmd(socket, RECV); 00267 return len; 00268 } 00269 00270 int WIZnet_Chip::new_socket() 00271 { 00272 for(int s = 0; s < MAX_SOCK_NUM; s++) { 00273 if (sreg<uint8_t>(s, Sn_SR) == SOCK_CLOSED) { 00274 return s; 00275 } 00276 } 00277 return -1; 00278 } 00279 00280 uint16_t WIZnet_Chip::new_port() 00281 { 00282 uint16_t port = rand(); 00283 port |= 49152; 00284 return port; 00285 } 00286 00287 void WIZnet_Chip::scmd(int socket, Command cmd) 00288 { 00289 sreg<uint8_t>(socket, Sn_CR, cmd); 00290 while(sreg<uint8_t>(socket, Sn_CR)); 00291 } 00292 00293 void WIZnet_Chip::spi_write(uint16_t addr, uint8_t cb, const uint8_t *buf, uint16_t len) 00294 { 00295 cs = 0; 00296 spi->write(addr >> 8); 00297 spi->write(addr & 0xff); 00298 spi->write(cb); 00299 for(int i = 0; i < len; i++) { 00300 spi->write(buf[i]); 00301 } 00302 cs = 1; 00303 00304 #if DBG_SPI 00305 debug("[SPI]W %04x(%02x %d)", addr, cb, len); 00306 for(int i = 0; i < len; i++) { 00307 debug(" %02x", buf[i]); 00308 if (i > 16) { 00309 debug(" ..."); 00310 break; 00311 } 00312 } 00313 debug("\r\n"); 00314 #endif 00315 } 00316 00317 void WIZnet_Chip::spi_read(uint16_t addr, uint8_t cb, uint8_t *buf, uint16_t len) 00318 { 00319 cs = 0; 00320 spi->write(addr >> 8); 00321 spi->write(addr & 0xff); 00322 spi->write(cb); 00323 for(int i = 0; i < len; i++) { 00324 buf[i] = spi->write(0); 00325 } 00326 cs = 1; 00327 00328 #if DBG_SPI 00329 debug("[SPI]R %04x(%02x %d)", addr, cb, len); 00330 for(int i = 0; i < len; i++) { 00331 debug(" %02x", buf[i]); 00332 if (i > 16) { 00333 debug(" ..."); 00334 break; 00335 } 00336 } 00337 debug("\r\n"); 00338 if ((addr&0xf0ff)==0x4026 || (addr&0xf0ff)==0x4003) { 00339 wait_ms(200); 00340 } 00341 #endif 00342 } 00343 00344 uint32_t str_to_ip(const char* str) 00345 { 00346 uint32_t ip = 0; 00347 char* p = (char*)str; 00348 for(int i = 0; i < 4; i++) { 00349 ip |= atoi(p); 00350 p = strchr(p, '.'); 00351 if (p == NULL) { 00352 break; 00353 } 00354 ip <<= 8; 00355 p++; 00356 } 00357 return ip; 00358 } 00359 00360 void printfBytes(char* str, uint8_t* buf, int len) 00361 { 00362 printf("%s %d:", str, len); 00363 for(int i = 0; i < len; i++) { 00364 printf(" %02x", buf[i]); 00365 } 00366 printf("\n"); 00367 } 00368 00369 void printHex(uint8_t* buf, int len) 00370 { 00371 for(int i = 0; i < len; i++) { 00372 if ((i%16) == 0) { 00373 printf("%p", buf+i); 00374 } 00375 printf(" %02x", buf[i]); 00376 if ((i%16) == 15) { 00377 printf("\n"); 00378 } 00379 } 00380 printf("\n"); 00381 } 00382 00383 void debug_hex(uint8_t* buf, int len) 00384 { 00385 for(int i = 0; i < len; i++) { 00386 if ((i%16) == 0) { 00387 debug("%p", buf+i); 00388 } 00389 debug(" %02x", buf[i]); 00390 if ((i%16) == 15) { 00391 debug("\n"); 00392 } 00393 } 00394 debug("\n"); 00395 } 00396 00397 #endif
Generated on Tue Jul 12 2022 19:24:11 by
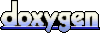