
This library allows any user to use their Mbed project with a transient energy source( e.g. windturbine, solar power). You can do this by simply import the "hibernus.h" header file, and call the "Hibernus()" method at the beginning of you main program. In case of a power loss the state of your programm will be saved to the nonvolatile memory and it will be resumed from the same point as soon as there is enough power for the board to run properly.
Dependencies: FreescaleIAP mbed
hibernus.h
00001 /** Hibernus Library 00002 * University of Southampton 2017 00003 * 00004 * Open-source liberary that enable any of your Mbed project work with transient enegy sources. 00005 * In order to use this library include the "hibernus.h" header file, and use the "Hibernus()" method at the beginning of you main funtion. 00006 * For more details and example see the "main.cpp" exampe file, and the attached documnetation 00007 * 00008 * 00009 * Released under the MIT License: http://mbed.org/license/mit 00010 */ 00011 #ifndef HIBERNUS_H_ 00012 #define HIBERNUS_H_ 00013 00014 #define Fixed_Add_Vars_Offset 0x40 //the Offset added to the first address of RAM, in order to compute the address of first fixed Add variable 00015 #define Fixed_Add_Vars_Size 0x2C //size of the all fixed address variables (0x28 = 44(dec) = 4Bytes*(4 flags+3CoreReg+4LoopVar)) 00016 #define flash_end (FLASH_Start + flash_Size) 00017 #define ramToFlash_sectors_number (RAM_Size / sector_Size) //how many flash sectors do we have to erase, before saving the RAM content to flash 00018 #define flash_ramSection_start (flash_end - RAM_Size) 00019 00020 // Declare register integers for the SP, LR, and PC 00021 register volatile unsigned int _SP __asm("r13"); 00022 register volatile unsigned int _LR __asm("r14"); 00023 register volatile unsigned int _PC __asm("r15"); 00024 00025 // Value written to non-volatile flags to indicate that they are set or not 00026 #define Flag_set 0xFFFF0000 00027 #define Flag_erase 0xFFFFFFFF 00028 00029 // Declare external assembly language function (in a *.s file) 00030 extern "C" void asm_restore(void); 00031 extern "C" volatile unsigned int* getFlag_1(void); 00032 extern "C" volatile unsigned int* getFlag_2(void); 00033 extern "C" volatile unsigned int* getFlag_3(void); 00034 extern "C" volatile unsigned int* getFlag_4(void); 00035 extern "C" volatile unsigned int* getCore_SP(void); 00036 extern "C" volatile unsigned int* getCore_LR(void); 00037 extern "C" volatile unsigned int* getCore_PC(void); 00038 00039 void Hibernus(void); 00040 void Save_RAM_Regs(void); 00041 void Restore_Regs(void); 00042 void restore(void); 00043 void hibernate(void); 00044 00045 #endif
Generated on Thu Jul 28 2022 06:18:24 by
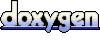