
This library allows any user to use their Mbed project with a transient energy source( e.g. windturbine, solar power). You can do this by simply import the "hibernus.h" header file, and call the "Hibernus()" method at the beginning of you main program. In case of a power loss the state of your programm will be saved to the nonvolatile memory and it will be resumed from the same point as soon as there is enough power for the board to run properly.
Dependencies: FreescaleIAP mbed
config.h
00001 /** Hibernus Library 00002 * University of Southampton 2017 00003 * 00004 * Open-source liberary that enable any of your Mbed project work with transient enegy sources. 00005 * In order to use this library include the "hibernus.h" header file, and use the "Hibernus()" method at the beginning of you main funtion. 00006 * For more details and example see the "main.cpp" exampe file, and the attached documnetation 00007 * 00008 * 00009 * Released under the MIT License: http://mbed.org/license/mit 00010 */ 00011 #ifndef CONFIG_H_ 00012 #define CONFIG_H_ 00013 #include "mbed.h" 00014 #include "FreescaleIAP.h" 00015 00016 #define HasInternalComparator 1 //Set on 1 if there is an internal comparator 0 otherwise 00017 #define SaveFlagsInFlash 1 //set on 1 if there is enough Flash memory available for the flags to have their own flash sector, 0 otherwise 00018 //if there is not enough flash memory, the flags will be saved in RAM memory 00019 00020 // RAM addresses split in 1 KB sectors 00021 #define RAM_1_Address 0x1FFFFC00 00022 #define FLASH_Start 0x00000000 00023 #define RAM_Size 4096 00024 #define flash_Size flash_size() //32K flash 00025 #define sector_Size SECTOR_SIZE //4K sector size 00026 #define Flash_Flags_Sector_Start (flash_end -1*sector_Size) 00027 00028 #define No_Of_4B_Peripheral_Reg 19 00029 #define No_Of_1B_Peripheral_Reg 8 00030 00031 00032 const unsigned int REG_Addresses_4B[83] = // Constant peripheral register addresses which are 4 bytes in size 00033 { 00034 (unsigned int)&FPTA->PDOR,(unsigned int)&FPTA->PDDR,(unsigned int)&FPTB->PDOR,(unsigned int)&FPTB->PDDR, 00035 //0x4000F000, 0x4000F014, 0x4000F040, 0x4000F054, // GPIO controller aliased to ox400FF000 00036 (unsigned int)&SIM->SOPT1,(unsigned int)&SIM->SOPT1CFG,(unsigned int)&SIM->SOPT2,(unsigned int)&SIM->SOPT4, 00037 (unsigned int)&SIM->SOPT5,(unsigned int)&SIM->SOPT7,(unsigned int)&SIM->SCGC4,(unsigned int)&SIM->SCGC5, 00038 (unsigned int)&SIM->SCGC6,(unsigned int)&SIM->SCGC7,(unsigned int)&SIM->CLKDIV1,(unsigned int)&SIM->FCFG1, 00039 (unsigned int)&SIM->COPC, 00040 //0x40047000, 0x40047004, 0x40048004, 0x4004800C, 0x40048010, 0x40048018, 0x40048034, 0x40048038, 0x4004803C, 0x40048040, 00041 //0x40048044, 0x4004804C, 0x40048100, // SIM 00042 /*(unsigned int)&PORTA->PCR[0],(unsigned int)&PORTA->PCR[1],(unsigned int)&PORTA->PCR[2],(unsigned int)&PORTA->PCR[3], 00043 (unsigned int)&PORTA->PCR[4],(unsigned int)&PORTA->PCR[5],(unsigned int)&PORTA->PCR[6],(unsigned int)&PORTA->PCR[7], 00044 (unsigned int)&PORTA->PCR[8],(unsigned int)&PORTA->PCR[9],(unsigned int)&PORTA->PCR[10],(unsigned int)&PORTA->PCR[11], 00045 (unsigned int)&PORTA->PCR[12],(unsigned int)&PORTA->PCR[13],(unsigned int)&PORTA->PCR[14],(unsigned int)&PORTA->PCR[15], 00046 (unsigned int)&PORTA->PCR[16],(unsigned int)&PORTA->PCR[17],(unsigned int)&PORTA->PCR[18],(unsigned int)&PORTA->PCR[19], 00047 (unsigned int)&PORTA->PCR[20],(unsigned int)&PORTA->PCR[21],(unsigned int)&PORTA->PCR[22],(unsigned int)&PORTA->PCR[23], 00048 (unsigned int)&PORTA->PCR[24],(unsigned int)&PORTA->PCR[25],(unsigned int)&PORTA->PCR[26],(unsigned int)&PORTA->PCR[27], 00049 (unsigned int)&PORTA->PCR[28],(unsigned int)&PORTA->PCR[29],(unsigned int)&PORTA->PCR[30],(unsigned int)&PORTA->PCR[31], 00050 //0x40049000, 0x40049004, 0x40049008, 0x4004900C, 0x40049010, 0x40049014, 0x40049018, 0x4004901C, 0x40049020, 0x40049024, 00051 //0x40049028, 0x4004902C, 0x40049030, 0x40049034, 0x40049038, 0x4004903C, 0x40049040, 0x40049044, 0x40049048, 0x4004904C, 00052 //0x40049050, 0x40049054, 0x40049058, 0x4004905C, 0x40049060, 0x40049064, 0x40049068, 0x4004906C, 0x40049070, 0x40049074, 00053 //0x40049078, 0x4004907C, // Port A multiplexing control 00054 (unsigned int)&PORTB->PCR[0],(unsigned int)&PORTB->PCR[1],(unsigned int)&PORTB->PCR[2],(unsigned int)&PORTB->PCR[3], 00055 (unsigned int)&PORTB->PCR[4],(unsigned int)&PORTB->PCR[5],(unsigned int)&PORTB->PCR[6],(unsigned int)&PORTB->PCR[7], 00056 (unsigned int)&PORTB->PCR[8],(unsigned int)&PORTB->PCR[9],(unsigned int)&PORTB->PCR[10],(unsigned int)&PORTB->PCR[11], 00057 (unsigned int)&PORTB->PCR[12],(unsigned int)&PORTB->PCR[13],(unsigned int)&PORTB->PCR[14],(unsigned int)&PORTB->PCR[15], 00058 (unsigned int)&PORTB->PCR[16],(unsigned int)&PORTB->PCR[17],(unsigned int)&PORTB->PCR[18],(unsigned int)&PORTB->PCR[19], 00059 (unsigned int)&PORTB->PCR[20],(unsigned int)&PORTB->PCR[21],(unsigned int)&PORTB->PCR[22],(unsigned int)&PORTB->PCR[23], 00060 (unsigned int)&PORTB->PCR[24],(unsigned int)&PORTB->PCR[25],(unsigned int)&PORTB->PCR[26],(unsigned int)&PORTB->PCR[27], 00061 (unsigned int)&PORTB->PCR[28],(unsigned int)&PORTB->PCR[29],(unsigned int)&PORTB->PCR[30],(unsigned int)&PORTB->PCR[31], 00062 //0x4004A000, 0x4004A004, 0x4004A008, 0x4004A00C, 0x4004A010, 0x4004A014, 0x4004A018, 0x4004A01C, 0x4004A020, 0x4004A024, 00063 //0x4004A028, 0x4004A02C, 0x4004A030, 0x4004A034, 0x4004A038, 0x4004A03C, 0x4004A040, 0x4004A044, 0x4004A048, 0x4004A04C, 00064 //0x4004A050, 0x4004A054, 0x4004A058, 0x4004A05C, 0x4004A060, 0x4004A064, 0x4004A068, 0x4004A06C, 0x4004A070, 0x4004A074, 00065 //0x4004A078, 0x4004A07C, // Port B multiplexing control*/ 00066 (unsigned int)&MCM->PLACR,(unsigned int)&MCM->CPO 00067 //0xF000300C, 0xF0003040 // MCM 00068 }; 00069 00070 const unsigned int REG_Addresses_1B[30] = // Constant peripheral register addresses which are 1 byte in size 00071 { 00072 (unsigned int)&MCG->C1,(unsigned int)&MCG->C2,(unsigned int)&MCG->C3,(unsigned int)&MCG->C4, 00073 (unsigned int)&MCG->C6,(unsigned int)&MCG->SC,(unsigned int)&MCG->ATCVH,(unsigned int)&MCG->ATCVL, 00074 //0x40064000, 0x40064001, 0x40064002, 0x40064003, 0x40064005, 0x40064008, 0x4006400A, 0x4006400B, // MCG 00075 //(unsigned int)&OSC0->CR, 00076 //0x40065000, // Oscillator 00077 //(unsigned int)&UART0->BDH,(unsigned int)&UART0->BDL,(unsigned int)&UART0->C1,(unsigned int)&UART0->C2, 00078 //(unsigned int)&UART0->S1,(unsigned int)&UART0->S2,(unsigned int)&UART0->C3,(unsigned int)&UART0->D, 00079 //(unsigned int)&UART0->MA1,(unsigned int)&UART0->MA2,(unsigned int)&UART0->C4,(unsigned int)&UART0->C5, 00080 //0x4006A000, 0x4006A001, 0x4006A002, 0x4006A003, 0x4006A004, 0x4006A005, 0x4006A006, 0x4006A007, 0x4006A008, 0x4006A009, 00081 //0x4006A00A, 0x4006A00B, // UART0 00082 //(unsigned int)&PMC->LVDSC1,(unsigned int)&PMC->LVDSC2,(unsigned int)&PMC->REGSC, 00083 //0x4007D000, 0x4007D001, 0x4007D002, // PMC 00084 //(unsigned int)&SMC->PMPROT,(unsigned int)&SMC->PMCTRL,(unsigned int)&SMC->STOPCTRL,(unsigned int)&SMC->PMSTAT, 00085 //0x4007E000, 0x4007E001, 0x4007E002, 0x4007E003, // SMC 00086 //(unsigned int)&RCM->RPFC,(unsigned int)&RCM->RPFW 00087 //0x4007F004, 0x4007F005 // RCM 00088 }; 00089 00090 00091 extern "C" void FLEX_INT0_IRQHandler(void); 00092 extern "C" void FLEX_INT1_IRQHandler(void); 00093 00094 #if HasInternalComparator == 1 00095 void configure_VH_comparator_interrupt(void); 00096 #else 00097 void configure_VH_gpio_interrupt(void); 00098 void configure_VR_gpio_interrupt(void); 00099 #endif 00100 00101 void Comparator_Setup(void); 00102 00103 void erase_flags_memory(void); 00104 void restore_flags(void); 00105 void Enter_LLS(void); 00106 void setFlag(volatile unsigned int*); 00107 bool isFlagSet(volatile unsigned int*); 00108 void copyRamToFlash(void); 00109 00110 #endif 00111
Generated on Thu Jul 28 2022 06:18:24 by
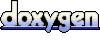