
Server for window shades - using Soffy DCT-30 motors - more details here http://robdobson.com/2013/10/moving-my-window-shades-control-to-mbed/
Dependencies: EthernetInterface RdWebServer mbed-rtos mbed
main.cpp
00001 /* main.cpp 00002 Rob Dobson 2013 00003 More details at http://robdobson.com/2013/10/moving-my-window-shades-control-to-mbed/ 00004 */ 00005 00006 #include "mbed.h" 00007 #include "EthernetInterface.h" 00008 #include <stdio.h> 00009 #include <string.h> 00010 #include "RdWebServer.h" 00011 #include "WindowShade.h" 00012 00013 #define PORT 80 00014 00015 Serial pc(USBTX, USBRX); 00016 00017 RdWebServer webServer; 00018 00019 EthernetInterface eth; 00020 00021 DigitalOut led1(LED1); //server listning status 00022 DigitalOut led2(LED2); //socket connecting status 00023 00024 Ticker ledTick; 00025 00026 const int TICK_MS = 250; 00027 const int MAX_WINDOW_SHADES = 5; 00028 int numWindowShades = 0; 00029 WindowShade* pWindowShades[MAX_WINDOW_SHADES]; 00030 char* indexHtmName = ""; 00031 00032 void ledTickfunc() 00033 { 00034 if(webServer.isListening()) 00035 { 00036 led1 = !led1; 00037 } 00038 else 00039 { 00040 led1 = false; 00041 } 00042 for (int shadeIdx = 0; shadeIdx < numWindowShades; shadeIdx++) 00043 pWindowShades[shadeIdx]->CheckTimeouts(TICK_MS); 00044 } 00045 00046 void handleCmd_blindControl(char* cmdStr, char* argStr) 00047 { 00048 printf("BLINDS COMMAND %s %s\n\r", cmdStr, argStr); 00049 if (argStr == NULL) 00050 return; 00051 char* pch = strtok (argStr,"/"); 00052 if (pch == NULL) 00053 return; 00054 int blindNum = pch[0] - '0'; 00055 if (blindNum < 1 || blindNum > MAX_WINDOW_SHADES) 00056 return; 00057 int blindIdx = blindNum-1; 00058 char* pDirn = strtok (NULL,"/"); 00059 if (pDirn == NULL) 00060 return; 00061 char* pDuration = strtok (NULL,"/"); 00062 if (pDuration == NULL) 00063 return; 00064 pWindowShades[blindIdx]->DoCommand(pDirn, pDuration); 00065 } 00066 00067 void setShadesConfig() 00068 { 00069 printf("Rob Blinds Server - Configured for "); 00070 // Check for a config file on the local file system 00071 LocalFileSystem local("local"); 00072 FILE* fp = fopen("office.cnf", "r"); 00073 if (fp != NULL) 00074 { 00075 fclose(fp); 00076 indexHtmName = "ixoffice.htm"; 00077 pWindowShades[0] = new WindowShade("Left", p15, p16, p17); 00078 pWindowShades[1] = new WindowShade("Mid-left", p18, p19, p20); 00079 pWindowShades[2] = new WindowShade("Mid-right", p21, p22, p23); 00080 pWindowShades[3] = new WindowShade("Right", p24, p25, p26); 00081 pWindowShades[4] = new WindowShade("Rob's", p27, p28, p29); 00082 numWindowShades = 5; 00083 printf("Office Blinds\n\r"); 00084 } 00085 else 00086 { 00087 indexHtmName = "ixgames.htm"; 00088 pWindowShades[0] = new WindowShade("Blind1", p21, p22, p23); 00089 pWindowShades[1] = new WindowShade("Blind2", p24, p25, p26); 00090 numWindowShades = 2; 00091 printf("Playroom Blinds\n\r"); 00092 } 00093 } 00094 00095 int main (void) 00096 { 00097 pc.baud(115200); 00098 00099 setShadesConfig(); 00100 00101 ledTick.attach(&ledTickfunc,TICK_MS / 1000.0); 00102 00103 // setup ethernet interface 00104 eth.init(); //Use DHCP 00105 eth.connect(); 00106 printf("IP Address is %s\n\r", eth.getIPAddress()); 00107 00108 webServer.addCommand("", RdWebServerCmdDef::CMD_LOCALFILE, NULL, indexHtmName, true); 00109 webServer.addCommand("gear-gr.png", RdWebServerCmdDef::CMD_LOCALFILE, NULL, NULL, true); 00110 webServer.addCommand("blind", RdWebServerCmdDef::CMD_CALLBACK, &handleCmd_blindControl); 00111 webServer.init(PORT, &led2); 00112 webServer.run(); 00113 }
Generated on Tue Jul 12 2022 21:48:28 by
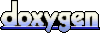