
Server for window shades - using Soffy DCT-30 motors - more details here http://robdobson.com/2013/10/moving-my-window-shades-control-to-mbed/
Dependencies: EthernetInterface RdWebServer mbed-rtos mbed
WindowShade.cpp
00001 /* WindowShade.cpp 00002 Rob Dobson 2013 00003 More details at http://robdobson.com/2013/10/moving-my-window-shades-control-to-mbed/ 00004 */ 00005 00006 #include "WindowShade.h" 00007 00008 WindowShade::WindowShade(char* name, PinName up, PinName stop, PinName down) 00009 { 00010 strncpy(_shadeName, name, MAX_SHADE_NAME_LEN); 00011 _shadeName[MAX_SHADE_NAME_LEN-1] = '\0'; 00012 _pUpPin = new DigitalOut(up); 00013 _pStopPin = new DigitalOut(stop); 00014 _pDownPin = new DigitalOut(down); 00015 _msTimeout = 0; 00016 _tickCount = 0; 00017 00018 } 00019 00020 void WindowShade::ClearOutputs() 00021 { 00022 // Clear any existing command 00023 _msTimeout = 0; 00024 _tickCount = 0; 00025 *_pUpPin = false; 00026 *_pStopPin = false; 00027 *_pDownPin = false; 00028 } 00029 00030 void WindowShade::SetTimedOutput(DigitalOut* pPin, bool state, long msDuration, bool bClearExisting) 00031 { 00032 // printf("Setting %s %d %ld %d\n\r", _shadeName, state, msDuration, bClearExisting); 00033 00034 if (bClearExisting) 00035 ClearOutputs(); 00036 *pPin = state; 00037 if (msDuration != 0) 00038 { 00039 _msTimeout = msDuration; 00040 _tickCount = 0; 00041 } 00042 } 00043 00044 void WindowShade::CheckTimeouts(int tickMs) 00045 { 00046 // Couldn't get Timer to work so doing with a tick-count 00047 if (_msTimeout != 0) 00048 { 00049 _tickCount++; 00050 if ((_tickCount-1)*tickMs > _msTimeout) 00051 { 00052 ClearOutputs(); 00053 _msTimeout = 0; 00054 } 00055 } 00056 } 00057 00058 void WindowShade::DoCommand(char* pDirn, char* pDuration) 00059 { 00060 // printf("DoCommand %s %s %s\n\r", _shadeName, pDirn, pDuration); 00061 00062 // Duration and state 00063 int state = false; 00064 int msDuration = 0; 00065 if (strcmp(pDuration, "on") == 0) 00066 { 00067 state = true; 00068 msDuration = MAX_SHADE_ON_MILLSECS; 00069 } 00070 else if (strcmp(pDuration, "off") == 0) 00071 { 00072 state = false; 00073 msDuration = 0; 00074 } 00075 else if (strcmp(pDuration, "pulse") == 0) 00076 { 00077 state = true; 00078 msDuration = PULSE_ON_MILLISECS; 00079 } 00080 00081 // Direction & config 00082 if (strcmp(pDirn, "up") == 0) 00083 { 00084 SetTimedOutput(_pUpPin, state, msDuration, true); 00085 } 00086 else if (strcmp(pDirn, "stop") == 0) 00087 { 00088 SetTimedOutput(_pStopPin, state, msDuration, true); 00089 } 00090 else if (strcmp(pDirn, "down") == 0) 00091 { 00092 SetTimedOutput(_pDownPin, state, msDuration, true); 00093 } 00094 else if (strcmp(pDirn, "setuplimit") == 0) 00095 { 00096 SetTimedOutput(_pStopPin, state, 0, true); 00097 SetTimedOutput(_pDownPin, state, msDuration, false); 00098 } 00099 else if (strcmp(pDirn, "setdownlimit") == 0) 00100 { 00101 SetTimedOutput(_pStopPin, state, 0, true); 00102 SetTimedOutput(_pUpPin, state, msDuration, false); 00103 } 00104 else if (strcmp(pDirn, "resetmemory") == 0) 00105 { 00106 SetTimedOutput(_pStopPin, state, 0, true); 00107 SetTimedOutput(_pDownPin, state, 0, false); 00108 SetTimedOutput(_pUpPin, state, msDuration, false); 00109 } 00110 00111 }
Generated on Tue Jul 12 2022 21:48:28 by
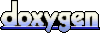