
mDot processor with ROHM sensor board on UDK2.
Dependencies: MbedJSONValue libmDot mbed-rtos mbed
Fork of VVV_MultiTech_Dragonfly_ATT_Dallas by
main.cpp
00001 /************************************************************************* 00002 * Originally this was a 00003 * Dragonfly Example program for 2015 AT&T Government Solutions Hackathon 00004 * 00005 * This is in process of being convertered to a mDot processor. mDot has a 00006 * limited set of IO that are available to the ROHM board. Most of the 00007 * Sensors will be used but the ones that can't have been commented out. 00008 * 00009 * The following hardware is required to successfully run this program: 00010 * - MultiTech UDK2 (4" square white PCB with Arduino headers, antenna 00011 * connector, micro USB ports, and 40-pin connector for Dragonfly) 00012 * - MultiTech mDot with a LoRa radio 00013 * - Seeed Studio Base Shield to elevate the ROHM board connectors away from mDOt 00014 * - MEMs Inertial and Environmental Nucleo Expansion board (LSM6DS0 00015 * 3-axis accelerometer + 3-axis gyroscope, LIS3MDL 3-axis 00016 * magnetometer, HTS221 humidity and temperature sensor and LPS25HB 00017 * pressure sensor) 00018 * 00019 * What this program does: 00020 * - reads data from all sensors on MEMs board on a periodic basis 00021 * - prints all sensor data to debug port on a periodic basis 00022 * - optionally sends LoRa sensor data when the timer expires 00023 * 00024 * This needs to be written yet. 00025 * - optionally sends sensor data to AT&T M2X cloud platform (user must 00026 * create own M2X account and configure a device) 00027 * - you need to set the "m2x_api_key" field and the "m2x_device_id" 00028 * field based on your M2X account for this to work 00029 * - you need to set the "do_cloud_post" flag to true for this to 00030 * work 00031 * 00032 * Setup: 00033 * - Seat the mDot on the UDK2 board 00034 * - Stack the Base Shield on the UDK2 Arduino headers 00035 * - Make sure the reference voltage selector switch (next to the A0 00036 * socket) is switched to 3.3V so you don't blow the mDot analog converter 00037 * accuracy will suffer as a result when compared to 5V. 00038 * - Stack the MEMs board on top of the Base Shield 00039 * - Plug in the power cable 00040 * - Plug a micro USB cable away from the multiple LED String 00041 * 00042 * Go have fun and make something cool! 00043 * 00044 ************************************************************************/ 00045 /* 00046 Sample Program Description: 00047 This Program will enable to Multi-Tech mDot platform to utilize ROHM's Multi-sensor Shield Board. 00048 This program will initialize most of the sensors on the shield and then read back the sensor data. 00049 Data will then be output to the UART Debug Terminal every 1 second. 00050 00051 Sample Program Author: 00052 ROHM USDC 00053 00054 Additional Resources: 00055 ROHM Sensor Shield GitHub Repository: https://github.com/ROHMUSDC/ROHM_SensorPlatform_Multi-Sensor-Shield 00056 */ 00057 00058 00059 #include "mbed.h" 00060 #include "MbedJSONValue.h" 00061 #include <string> 00062 00063 // added the following help files for a mDot not required for Dragonfly. 00064 #include "mDot.h" 00065 #include "MTSLog.h" 00066 #include <vector> 00067 #include <algorithm> 00068 #include "rtos.h" 00069 00070 00071 // Debug serial port 00072 static Serial debug(USBTX, USBRX); 00073 00074 // variables for sensor data 00075 float temp_celsius; 00076 float humidity_percent; 00077 float pressure_mbar; 00078 float moisture_percent; 00079 int32_t mag_mgauss[3]; 00080 int32_t acc_mg[3]; 00081 int32_t gyro_mdps[3]; 00082 00083 // misc variables 00084 static char wall_of_dash[] = "--------------------------------------------------"; 00085 static int thpm_interval_ms = 5000; 00086 static int motion_interval_ms = 5000; 00087 static int print_interval_ms = 5000; 00088 static int sms_interval_ms = 60000; 00089 int debug_baud = 115200; 00090 00091 00092 /**************************************************************************************************** 00093 00094 ****************************************************************************************************/ 00095 00096 //Macros for checking each of the different Sensor Devices 00097 #define AnalogTemp //BDE0600 00098 // #define AnalogUV //ML8511 // analog pin A4 on Arduino connector is not connected to the mDot on the UDK. 00099 #define HallSensor //BU52011 00100 #define RPR0521 //RPR0521 00101 #define KMX62 //KMX61, Accel/Mag 00102 #define COLOR //BH1745 00103 #define KX022 //KX022, Accel Only 00104 #define Pressure //BM1383 00105 00106 #define SMS //allow SMS messaging now sending LORA!!!! 00107 //#define Web //allow M2X communication 00108 00109 00110 //Define Pins for I2C Interface 00111 I2C i2c(I2C_SDA, I2C_SCL); 00112 bool RepStart = true; 00113 bool NoRepStart = false; 00114 00115 //Define Sensor Variables 00116 #ifdef AnalogTemp 00117 AnalogIn BDE0600_Temp(PC_1); //Mapped to A2 pin 15 on the mDot 00118 uint16_t BDE0600_Temp_value; 00119 float BDE0600_output; 00120 #endif 00121 00122 //#ifdef AnalogUV // analog pin A4 on Arduino connector is not connected to the mDot on the UDK. 00123 //AnalogIn ML8511_UV(PA_7); //Mapped to A4 not a pin routed on the UDK to the mDot 00124 //uint16_t ML8511_UV_value; 00125 //float ML8511_output; 00126 //#endif 00127 00128 #ifdef HallSensor 00129 DigitalIn Hall_GPIO0(PA_4); // assigned to D10 on Arduino, mapped to pin 17 on mDot 00130 DigitalIn Hall_GPIO1(PA_7); // assigned to D11 on Arduino, mapped to pin 11 on mDot 00131 int Hall_Return1; 00132 int Hall_Return0; 00133 int32_t Hall_Return[2]; 00134 #endif 00135 00136 #ifdef RPR0521 00137 int RPR0521_addr_w = 0x70; //7bit addr = 0x38, with write bit 0 00138 int RPR0521_addr_r = 0x71; //7bit addr = 0x38, with read bit 1 00139 char RPR0521_ModeControl[2] = {0x41, 0xE6}; 00140 char RPR0521_ALSPSControl[2] = {0x42, 0x03}; 00141 char RPR0521_Persist[2] = {0x43, 0x20}; 00142 char RPR0521_Addr_ReadData = 0x44; 00143 char RPR0521_Content_ReadData[6]; 00144 int RPR0521_PS_RAWOUT = 0; //this is an output 00145 float RPR0521_PS_OUT = 0; 00146 int RPR0521_ALS_D0_RAWOUT = 0; 00147 int RPR0521_ALS_D1_RAWOUT = 0; 00148 float RPR0521_ALS_DataRatio = 0; 00149 float RPR0521_ALS_OUT = 0; //this is an output 00150 float RPR0521_ALS[2]; // is this ok taking an int to the [0] value and float to [1]??????????? 00151 #endif 00152 00153 #ifdef KMX62 00154 int KMX62_addr_w = 0x1C; //7bit addr = 0x38, with write bit 0 00155 int KMX62_addr_r = 0x1D; //7bit addr = 0x38, with read bit 1 00156 char KMX62_CNTL2[2] = {0x3A, 0x5F}; 00157 char KMX62_Addr_Accel_ReadData = 0x0A; 00158 char KMX62_Content_Accel_ReadData[6]; 00159 char KMX62_Addr_Mag_ReadData = 0x10; 00160 char KMX62_Content_Mag_ReadData[6]; 00161 short int MEMS_Accel_Xout = 0; 00162 short int MEMS_Accel_Yout = 0; 00163 short int MEMS_Accel_Zout = 0; 00164 double MEMS_Accel_Conv_Xout = 0; 00165 double MEMS_Accel_Conv_Yout = 0; 00166 double MEMS_Accel_Conv_Zout = 0; 00167 00168 short int MEMS_Mag_Xout = 0; 00169 short int MEMS_Mag_Yout = 0; 00170 short int MEMS_Mag_Zout = 0; 00171 float MEMS_Mag_Conv_Xout = 0; 00172 float MEMS_Mag_Conv_Yout = 0; 00173 float MEMS_Mag_Conv_Zout = 0; 00174 00175 double MEMS_Accel[3]; 00176 float MEMS_Mag[3]; 00177 #endif 00178 00179 #ifdef COLOR 00180 int BH1745_addr_w = 0x72; //write 00181 int BH1745_addr_r = 0x73; //read 00182 char BH1745_persistence[2] = {0x61, 0x03}; 00183 char BH1745_mode1[2] = {0x41, 0x00}; 00184 char BH1745_mode2[2] = {0x42, 0x92}; 00185 char BH1745_mode3[2] = {0x43, 0x02}; 00186 char BH1745_Content_ReadData[6]; 00187 char BH1745_Addr_color_ReadData = 0x50; 00188 int BH1745_Red; 00189 int BH1745_Blue; 00190 int BH1745_Green; 00191 int32_t BH1745[3]; //Red, Blue Green matrix 00192 #endif 00193 00194 #ifdef KX022 00195 int KX022_addr_w = 0x3C; //write 00196 int KX022_addr_r = 0x3D; //read 00197 char KX022_Accel_CNTL1[2] = {0x18, 0x41}; 00198 char KX022_Accel_ODCNTL[2] = {0x1B, 0x02}; 00199 char KX022_Accel_CNTL3[2] = {0x1A, 0xD8}; 00200 char KX022_Accel_TILT_TIMER[2] = {0x22, 0x01}; 00201 char KX022_Accel_CNTL2[2] = {0x18, 0xC1}; 00202 char KX022_Content_ReadData[6]; 00203 char KX022_Addr_Accel_ReadData = 0x06; 00204 float KX022_Accel_X; 00205 float KX022_Accel_Y; 00206 float KX022_Accel_Z; 00207 short int KX022_Accel_X_RawOUT = 0; 00208 short int KX022_Accel_Y_RawOUT = 0; 00209 short int KX022_Accel_Z_RawOUT = 0; 00210 int KX022_Accel_X_LB = 0; 00211 int KX022_Accel_X_HB = 0; 00212 int KX022_Accel_Y_LB = 0; 00213 int KX022_Accel_Y_HB = 0; 00214 int KX022_Accel_Z_LB = 0; 00215 int KX022_Accel_Z_HB = 0; 00216 float KX022_Accel[3]; 00217 #endif 00218 00219 #ifdef Pressure 00220 int Press_addr_w = 0xBA; //write 00221 int Press_addr_r = 0xBB; //read 00222 char PWR_DOWN[2] = {0x12, 0x01}; 00223 char SLEEP[2] = {0x13, 0x01}; 00224 char Mode_Control[2] = {0x14, 0xC4}; 00225 char Press_Content_ReadData[6]; 00226 char Press_Addr_ReadData =0x1A; 00227 int BM1383_Temp_highByte; 00228 int BM1383_Temp_lowByte; 00229 int BM1383_Pres_highByte; 00230 int BM1383_Pres_lowByte; 00231 int BM1383_Pres_leastByte; 00232 short int BM1383_Temp_Out; 00233 float BM1383_Temp_Conv_Out; 00234 float BM1383_Pres_Conv_Out; 00235 float_t BM1383[2]; // Temp is 0 and Pressure is 1 00236 float BM1383_Var; 00237 float BM1383_Deci; 00238 #endif 00239 00240 /**************************************************************************************************** 00241 // function prototypes 00242 ****************************************************************************************************/ 00243 bool init_mtsas(); 00244 void ReadAnalogTemp(); 00245 // void ReadAnalogUV (); // analog pin A4 on Arduino connector is not connected to the mDot on the UDK. 00246 void ReadHallSensor (); 00247 void ReadCOLOR (); 00248 void ReadRPR0521_ALS (); 00249 void ReadKMX62_Accel (); 00250 void ReadKMX62_Mag (); 00251 void ReadPressure (); 00252 void ReadKX022(); 00253 00254 // these options must match the settings on your Conduit 00255 // uncomment the following lines and edit their values to match your configuration 00256 static std::string config_network_name = "Arrow123"; 00257 static std::string config_network_pass = "Arrow123"; 00258 static uint8_t config_frequency_sub_band = 1; 00259 00260 /**************************************************************************************************** 00261 // main 00262 ****************************************************************************************************/ 00263 int main() 00264 { 00265 mts::MTSLog::setLogLevel(mts::MTSLog::TRACE_LEVEL); //NONE_, FATAL_, ERROR_, WARNING_, INFO_, DEBUG_, TRACE_ 00266 debug.baud(debug_baud); 00267 logInfo("starting..."); 00268 00269 00270 /**************************************************************************************************** 00271 Initialize I2C Devices ************ 00272 ****************************************************************************************************/ 00273 00274 #ifdef RPR0521 00275 i2c.write(RPR0521_addr_w, &RPR0521_ModeControl[0], 2, false); 00276 i2c.write(RPR0521_addr_w, &RPR0521_ALSPSControl[0], 2, false); 00277 i2c.write(RPR0521_addr_w, &RPR0521_Persist[0], 2, false); 00278 #endif 00279 00280 #ifdef KMX62 00281 i2c.write(KMX62_addr_w, &KMX62_CNTL2[0], 2, false); 00282 #endif 00283 00284 #ifdef COLOR 00285 i2c.write(BH1745_addr_w, &BH1745_persistence[0], 2, false); 00286 i2c.write(BH1745_addr_w, &BH1745_mode1[0], 2, false); 00287 i2c.write(BH1745_addr_w, &BH1745_mode2[0], 2, false); 00288 i2c.write(BH1745_addr_w, &BH1745_mode3[0], 2, false); 00289 #endif 00290 00291 #ifdef KX022 00292 i2c.write(KX022_addr_w, &KX022_Accel_CNTL1[0], 2, false); 00293 i2c.write(KX022_addr_w, &KX022_Accel_ODCNTL[0], 2, false); 00294 i2c.write(KX022_addr_w, &KX022_Accel_CNTL3[0], 2, false); 00295 i2c.write(KX022_addr_w, &KX022_Accel_TILT_TIMER[0], 2, false); 00296 i2c.write(KX022_addr_w, &KX022_Accel_CNTL2[0], 2, false); 00297 #endif 00298 00299 #ifdef Pressure 00300 i2c.write(Press_addr_w, &PWR_DOWN[0], 2, false); 00301 i2c.write(Press_addr_w, &SLEEP[0], 2, false); 00302 i2c.write(Press_addr_w, &Mode_Control[0], 2, false); 00303 #endif 00304 //End I2C Initialization Section ********************************************************** 00305 00306 //Initialize the mDot ******************************************************************** 00307 int32_t ret; 00308 mDot* dot; 00309 std::vector<uint8_t> data; 00310 std::string data_str = "hello! Jeff"; 00311 00312 // get a mDot handle 00313 dot = mDot::getInstance(); 00314 00315 // print library version information 00316 logInfo("version: %s", dot->getId().c_str()); 00317 00318 //******************************************* 00319 // configuration 00320 //******************************************* 00321 // reset to default config so we know what state we're in 00322 dot->resetConfig(); 00323 00324 dot->setLogLevel(mts::MTSLog::TRACE_LEVEL); //INFO_LEVEL 00325 00326 // set up the mDot with our network information: frequency sub band, network name, and network password 00327 // these can all be saved in NVM so they don't need to be set every time - see mDot::saveConfig() 00328 00329 // frequency sub band is only applicable in the 915 (US) frequency band 00330 // if using a MultiTech Conduit gateway, use the same sub band as your Conduit (1-8) - the mDot will use the 8 channels in that sub band 00331 // if using a gateway that supports all 64 channels, use sub band 0 - the mDot will use all 64 channels 00332 logInfo("setting frequency sub band"); 00333 if ((ret = dot->setFrequencySubBand(config_frequency_sub_band)) != mDot::MDOT_OK) { 00334 logError("failed to set frequency sub band %d:%s", ret, mDot::getReturnCodeString(ret).c_str()); 00335 } 00336 00337 logInfo("setting network name"); 00338 if ((ret = dot->setNetworkName(config_network_name)) != mDot::MDOT_OK) { 00339 logError("failed to set network name %d:%s", ret, mDot::getReturnCodeString(ret).c_str()); 00340 } 00341 00342 logInfo("setting network password"); 00343 if ((ret = dot->setNetworkPassphrase(config_network_pass)) != mDot::MDOT_OK) { 00344 logError("failed to set network password %d:%s", ret, mDot::getReturnCodeString(ret).c_str()); 00345 } 00346 00347 // a higher spreading factor allows for longer range but lower throughput 00348 // in the 915 (US) frequency band, spreading factors 7 - 10 are available 00349 // in the 868 (EU) frequency band, spreading factors 7 - 12 are available 00350 logInfo("setting TX spreading factor"); 00351 if ((ret = dot->setTxDataRate(mDot::SF_7)) != mDot::MDOT_OK) { 00352 logError("failed to set TX datarate %d:%s", ret, mDot::getReturnCodeString(ret).c_str()); 00353 } 00354 00355 // request receive confirmation of packets from the gateway 00356 logInfo("enabling ACKs"); 00357 if ((ret = dot->setAck(1)) != mDot::MDOT_OK) { 00358 logError("failed to enable ACKs %d:%s", ret, mDot::getReturnCodeString(ret).c_str()); 00359 } 00360 00361 // save this configuration to the mDot's NVM 00362 logInfo("saving config"); 00363 if (! dot->saveConfig()) { 00364 logError("failed to save configuration"); 00365 } 00366 //******************************************* 00367 // end of configuration 00368 //******************************************* 00369 00370 // attempt to join the network 00371 logInfo("joining network"); 00372 while ((ret = dot->joinNetwork()) != mDot::MDOT_OK) { 00373 logError("failed to join network %d:%s", ret, mDot::getReturnCodeString(ret).c_str()); 00374 // in the 868 (EU) frequency band, we need to wait until another channel is available before transmitting again 00375 osDelay(std::max((uint32_t)1000, (uint32_t)dot->getNextTxMs())); 00376 } 00377 00378 // format data for sending to the gateway 00379 for (std::string::iterator it = data_str.begin(); it != data_str.end(); it++) 00380 data.push_back((uint8_t) *it); 00381 00382 // END Initialization of mDot ****************************************************************** 00383 00384 Timer thpm_timer; 00385 thpm_timer.start(); // Timer data is set in the Variable seciton see misc variables Timer motion_timer; 00386 Timer print_timer; 00387 print_timer.start(); 00388 Timer motion_timer; 00389 motion_timer.start(); 00390 00391 #ifdef SMS 00392 Timer sms_timer; 00393 sms_timer.start(); 00394 #endif 00395 00396 while (true) { 00397 if (thpm_timer.read_ms() > thpm_interval_ms) { 00398 #ifdef AnalogTemp 00399 ReadAnalogTemp (); 00400 #endif 00401 00402 //#ifdef AnalogUV // analog pin A4 on Arduino connector is not connected to the mDot on the UDK. 00403 // ReadAnalogUV (); 00404 //#endif 00405 00406 #ifdef HallSensor 00407 ReadHallSensor (); 00408 #endif 00409 00410 #ifdef COLOR 00411 ReadCOLOR (); 00412 #endif 00413 00414 #ifdef RPR0521 //als digital 00415 ReadRPR0521_ALS (); 00416 #endif 00417 00418 #ifdef Pressure 00419 ReadPressure(); 00420 #endif 00421 thpm_timer.reset(); 00422 } 00423 00424 if (motion_timer.read_ms() > motion_interval_ms) { 00425 #ifdef KMX62 00426 ReadKMX62_Accel (); 00427 ReadKMX62_Mag (); 00428 #endif 00429 00430 #ifdef KX022 00431 ReadKX022 (); 00432 #endif 00433 motion_timer.reset(); 00434 } 00435 00436 if (print_timer.read_ms() > print_interval_ms) { 00437 logDebug("%s", wall_of_dash); 00438 logDebug("SENSOR DATA"); 00439 logDebug("temperature: %0.2f C", BM1383[0]); 00440 // logDebug("analog uv: %.1f mW/cm2", ML8511_output); // analog pin A4 on Arduino connector is not connected to the mDot on the UDK. 00441 logDebug("ambient Light %0.3f", RPR0521_ALS[0]); 00442 logDebug("proximity count %0.3f", RPR0521_ALS[1]); 00443 logDebug("hall effect: South %d\t North %d", Hall_Return[0],Hall_Return[1]); 00444 logDebug("pressure: %0.2f hPa", BM1383[1]); 00445 logDebug("magnetometer:\r\n\tx: %0.3f\ty: %0.3f\tz: %0.3f\tuT", MEMS_Mag[0], MEMS_Mag[1], MEMS_Mag[2]); 00446 logDebug("accelerometer:\r\n\tx: %0.3f\ty: %0.3f\tz: %0.3f\tg", MEMS_Accel[0], MEMS_Accel[1], MEMS_Accel[2]); 00447 logDebug("color:\r\n\tred: %ld\tgrn: %ld\tblu: %ld\t", BH1745[0], BH1745[1], BH1745[2]); 00448 logDebug("%s", wall_of_dash); 00449 print_timer.reset(); 00450 } 00451 00452 00453 00454 #ifdef SMS 00455 if (sms_timer.read_ms() > sms_interval_ms) { 00456 sms_timer.reset(); 00457 logInfo("SMS Send Routine"); 00458 printf(" In sms routine \r\n"); 00459 00460 } 00461 #endif 00462 00463 00464 wait_ms(10); 00465 } 00466 } 00467 00468 00469 /************************************************************************************************/ 00470 // Sensor data acquisition functions 00471 /************************************************************************************************/ 00472 #ifdef AnalogTemp 00473 void ReadAnalogTemp () 00474 { 00475 BDE0600_Temp_value = BDE0600_Temp.read_u16(); 00476 00477 BDE0600_output = (float)BDE0600_Temp_value * (float)0.000050354; //(value * (3.3V/65535)) 00478 BDE0600_output = (BDE0600_output-(float)1.753)/((float)-0.01068) + (float)30; 00479 00480 // printf("BDE0600 Analog Temp Sensor Data:\r\n"); 00481 // printf(" Temp = %.2f C\r\n", BDE0600_output); 00482 } 00483 #endif 00484 00485 //#ifdef AnalogUV // analog pin A4 on Arduino connector is not connected to the mDot on the UDK. 00486 //void ReadAnalogUV () 00487 //{ 00488 // ML8511_UV_value = ML8511_UV.read_u16(); 00489 // ML8511_output = (float)ML8511_UV_value * (float)0.000050354; //(value * (3.3V/65535)) //Note to self: when playing with this, a negative value is seen... Honestly, I think this has to do with my ADC converstion... 00490 // ML8511_output = (ML8511_output-(float)2.2)/((float)0.129) + 10; // Added +5 to the offset so when inside (aka, no UV, readings show 0)... this is the wrong approach... and the readings don't make sense... Fix this. 00491 00492 // printf("ML8511 Analog UV Sensor Data:\r\n"); 00493 // printf(" UV = %.1f mW/cm2\r\n", ML8511_output); 00494 00495 //} 00496 //#endif 00497 00498 00499 #ifdef HallSensor 00500 void ReadHallSensor () 00501 { 00502 00503 Hall_Return[0] = Hall_GPIO0; 00504 Hall_Return[1] = Hall_GPIO1; 00505 00506 // printf("BU52011 Hall Switch Sensor Data:\r\n"); 00507 // printf(" South Detect = %d\r\n", Hall_Return[0]); 00508 // printf(" North Detect = %d\r\n", Hall_Return[1]); 00509 00510 00511 } 00512 #endif 00513 00514 #ifdef COLOR 00515 void ReadCOLOR () 00516 { 00517 00518 //Read color data from the IC 00519 i2c.write(BH1745_addr_w, &BH1745_Addr_color_ReadData, 1, RepStart); 00520 i2c.read(BH1745_addr_r, &BH1745_Content_ReadData[0], 6, NoRepStart); 00521 00522 //separate all data read into colors 00523 BH1745[0] = (BH1745_Content_ReadData[1]<<8) | (BH1745_Content_ReadData[0]); 00524 BH1745[1] = (BH1745_Content_ReadData[3]<<8) | (BH1745_Content_ReadData[2]); 00525 BH1745[2] = (BH1745_Content_ReadData[5]<<8) | (BH1745_Content_ReadData[4]); 00526 00527 //Output Data into UART 00528 // printf("BH1745 COLOR Sensor Data:\r\n"); 00529 // printf(" Red = %d ADC Counts\r\n",BH1745[0]); 00530 // printf(" Green = %d ADC Counts\r\n",BH1745[1]); 00531 // printf(" Blue = %d ADC Counts\r\n",BH1745[2]); 00532 00533 } 00534 #endif 00535 00536 #ifdef RPR0521 //als digital 00537 void ReadRPR0521_ALS () 00538 { 00539 i2c.write(RPR0521_addr_w, &RPR0521_Addr_ReadData, 1, RepStart); 00540 i2c.read(RPR0521_addr_r, &RPR0521_Content_ReadData[0], 6, NoRepStart); 00541 00542 RPR0521_ALS[1] = (RPR0521_Content_ReadData[1]<<8) | (RPR0521_Content_ReadData[0]); 00543 RPR0521_ALS_D0_RAWOUT = (RPR0521_Content_ReadData[3]<<8) | (RPR0521_Content_ReadData[2]); 00544 RPR0521_ALS_D1_RAWOUT = (RPR0521_Content_ReadData[5]<<8) | (RPR0521_Content_ReadData[4]); 00545 RPR0521_ALS_DataRatio = (float)RPR0521_ALS_D1_RAWOUT / (float)RPR0521_ALS_D0_RAWOUT; 00546 00547 if(RPR0521_ALS_DataRatio < (float)0.595) { 00548 RPR0521_ALS[0] = ((float)1.682*(float)RPR0521_ALS_D0_RAWOUT - (float)1.877*(float)RPR0521_ALS_D1_RAWOUT); 00549 } else if(RPR0521_ALS_DataRatio < (float)1.015) { 00550 RPR0521_ALS[0] = ((float)0.644*(float)RPR0521_ALS_D0_RAWOUT - (float)0.132*(float)RPR0521_ALS_D1_RAWOUT); 00551 } else if(RPR0521_ALS_DataRatio < (float)1.352) { 00552 RPR0521_ALS[0] = ((float)0.756*(float)RPR0521_ALS_D0_RAWOUT - (float)0.243*(float)RPR0521_ALS_D1_RAWOUT); 00553 } else if(RPR0521_ALS_DataRatio < (float)3.053) { 00554 RPR0521_ALS[0] = ((float)0.766*(float)RPR0521_ALS_D0_RAWOUT - (float)0.25*(float)RPR0521_ALS_D1_RAWOUT); 00555 } else { 00556 RPR0521_ALS[0] = 0; 00557 } 00558 // printf("RPR-0521 ALS/PROX Sensor Data:\r\n"); 00559 // printf(" ALS = %0.2f lx\r\n", RPR0521_ALS[0]); 00560 // printf(" PROX= %0.2f ADC Counts\r\n", RPR0521_ALS[1]); //defined as a float but is an unsigned. 00561 00562 } 00563 #endif 00564 00565 #ifdef KMX62 00566 void ReadKMX62_Accel () 00567 { 00568 //Read Accel Portion from the IC 00569 i2c.write(KMX62_addr_w, &KMX62_Addr_Accel_ReadData, 1, RepStart); 00570 i2c.read(KMX62_addr_r, &KMX62_Content_Accel_ReadData[0], 6, NoRepStart); 00571 00572 //Note: The highbyte and low byte return a 14bit value, dropping the two LSB in the Low byte. 00573 // However, because we need the signed value, we will adjust the value when converting to "g" 00574 MEMS_Accel_Xout = (KMX62_Content_Accel_ReadData[1]<<8) | (KMX62_Content_Accel_ReadData[0]); 00575 MEMS_Accel_Yout = (KMX62_Content_Accel_ReadData[3]<<8) | (KMX62_Content_Accel_ReadData[2]); 00576 MEMS_Accel_Zout = (KMX62_Content_Accel_ReadData[5]<<8) | (KMX62_Content_Accel_ReadData[4]); 00577 00578 //Note: Conversion to G is as follows: 00579 // Axis_ValueInG = MEMS_Accel_axis / 1024 00580 // However, since we did not remove the LSB previously, we need to divide by 4 again 00581 // Thus, we will divide the output by 4096 (1024*4) to convert and cancel out the LSB 00582 MEMS_Accel[0] = ((float)MEMS_Accel_Xout/4096/2); 00583 MEMS_Accel[1] = ((float)MEMS_Accel_Yout/4096/2); 00584 MEMS_Accel[2] = ((float)MEMS_Accel_Zout/4096/2); 00585 00586 // Return Data to UART 00587 // printf("KMX62 Accel+Mag Sensor Data:\r\n"); 00588 // printf(" AccX= %0.2f g\r\n", MEMS_Accel[0]); 00589 // printf(" AccY= %0.2f g\r\n", MEMS_Accel[1]); 00590 // printf(" AccZ= %0.2f g\r\n", MEMS_Accel[2]); 00591 00592 } 00593 00594 void ReadKMX62_Mag () 00595 { 00596 00597 //Read Mag portion from the IC 00598 i2c.write(KMX62_addr_w, &KMX62_Addr_Mag_ReadData, 1, RepStart); 00599 i2c.read(KMX62_addr_r, &KMX62_Content_Mag_ReadData[0], 6, NoRepStart); 00600 00601 //Note: The highbyte and low byte return a 14bit value, dropping the two LSB in the Low byte. 00602 // However, because we need the signed value, we will adjust the value when converting to "g" 00603 MEMS_Mag_Xout = (KMX62_Content_Mag_ReadData[1]<<8) | (KMX62_Content_Mag_ReadData[0]); 00604 MEMS_Mag_Yout = (KMX62_Content_Mag_ReadData[3]<<8) | (KMX62_Content_Mag_ReadData[2]); 00605 MEMS_Mag_Zout = (KMX62_Content_Mag_ReadData[5]<<8) | (KMX62_Content_Mag_ReadData[4]); 00606 00607 //Note: Conversion to G is as follows: 00608 // Axis_ValueInG = MEMS_Accel_axis / 1024 00609 // However, since we did not remove the LSB previously, we need to divide by 4 again 00610 // Thus, we will divide the output by 4095 (1024*4) to convert and cancel out the LSB 00611 MEMS_Mag[0] = (float)MEMS_Mag_Xout/4096*(float)0.146; 00612 MEMS_Mag[1] = (float)MEMS_Mag_Yout/4096*(float)0.146; 00613 MEMS_Mag[2] = (float)MEMS_Mag_Zout/4096*(float)0.146; 00614 00615 // Return Data to UART 00616 // printf(" MagX= %0.2f uT\r\n", MEMS_Mag[0]); 00617 // printf(" MagY= %0.2f uT\r\n", MEMS_Mag[1]); 00618 // printf(" MagZ= %0.2f uT\r\n", MEMS_Mag[2]); 00619 00620 } 00621 #endif 00622 00623 #ifdef KX022 00624 void ReadKX022 () 00625 { 00626 00627 //Read KX022 Portion from the IC 00628 i2c.write(KX022_addr_w, &KX022_Addr_Accel_ReadData, 1, RepStart); 00629 i2c.read(KX022_addr_r, &KX022_Content_ReadData[0], 6, NoRepStart); 00630 00631 //Format Data 00632 KX022_Accel_X_RawOUT = (KX022_Content_ReadData[1]<<8) | (KX022_Content_ReadData[0]); 00633 KX022_Accel_Y_RawOUT = (KX022_Content_ReadData[3]<<8) | (KX022_Content_ReadData[2]); 00634 KX022_Accel_Z_RawOUT = (KX022_Content_ReadData[5]<<8) | (KX022_Content_ReadData[4]); 00635 00636 //Scale Data 00637 KX022_Accel[0] = (float)KX022_Accel_X_RawOUT / 16384; 00638 KX022_Accel[1] = (float)KX022_Accel_Y_RawOUT / 16384; 00639 KX022_Accel[2] = (float)KX022_Accel_Z_RawOUT / 16384; 00640 00641 //Return Data through UART 00642 // printf("KX022 Accelerometer Sensor Data: \r\n"); 00643 // printf(" AccX= %0.2f g\r\n", KX022_Accel[0]); 00644 // printf(" AccY= %0.2f g\r\n", KX022_Accel[1]); 00645 // printf(" AccZ= %0.2f g\r\n", KX022_Accel[2]); 00646 00647 } 00648 #endif 00649 00650 00651 #ifdef Pressure 00652 void ReadPressure () 00653 { 00654 00655 i2c.write(Press_addr_w, &Press_Addr_ReadData, 1, RepStart); 00656 i2c.read(Press_addr_r, &Press_Content_ReadData[0], 6, NoRepStart); 00657 00658 BM1383_Temp_Out = (Press_Content_ReadData[0]<<8) | (Press_Content_ReadData[1]); 00659 BM1383[0] = (float)BM1383_Temp_Out/32; 00660 00661 BM1383_Var = (Press_Content_ReadData[2]<<3) | (Press_Content_ReadData[3] >> 5); 00662 BM1383_Deci = ((Press_Content_ReadData[3] & 0x1f) << 6 | ((Press_Content_ReadData[4] >> 2))); 00663 BM1383_Deci = (float)BM1383_Deci* (float)0.00048828125; //0.00048828125 = 2^-11 00664 BM1383[1] = (BM1383_Var + BM1383_Deci); //question pending here... 00665 00666 // printf("BM1383 Pressure Sensor Data:\r\n"); 00667 // printf(" Temperature= %0.2f C\r\n", BM1383[0]); 00668 // printf(" Pressure = %0.2f hPa\r\n", BM1383[1]); 00669 00670 } 00671 #endif
Generated on Sun Jul 17 2022 07:44:43 by
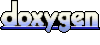