Fork of Smoothie to port to mbed non-LPC targets.
Fork of Smoothie by
utils.cpp
00001 /* 00002 This file is part of Smoothie (http://smoothieware.org/). The motion control part is heavily based on Grbl (https://github.com/simen/grbl). 00003 Smoothie is free software: you can redistribute it and/or modify it under the terms of the GNU General Public License as published by the Free Software Foundation, either version 3 of the License, or (at your option) any later version. 00004 Smoothie is distributed in the hope that it will be useful, but WITHOUT ANY WARRANTY; without even the implied warranty of MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the GNU General Public License for more details. 00005 You should have received a copy of the GNU General Public License along with Smoothie. If not, see <http://www.gnu.org/licenses/>. 00006 */ 00007 00008 #include "libs/Kernel.h" 00009 #include "libs/utils.h" 00010 #include "system_LPC17xx.h" 00011 using namespace std; 00012 #include <string> 00013 using std::string; 00014 #include <cstring> 00015 00016 volatile bool _isr_context = false; 00017 00018 uint16_t get_checksum(const string& to_check){ 00019 return get_checksum(to_check.c_str()); 00020 } 00021 00022 uint16_t get_checksum(const char* to_check){ 00023 // From: http://en.wikipedia.org/wiki/Fletcher%27s_checksum 00024 uint16_t sum1 = 0; 00025 uint16_t sum2 = 0; 00026 const char* p= to_check; 00027 char c; 00028 while((c= *p++) != 0) { 00029 sum1 = (sum1 + c) % 255; 00030 sum2 = (sum2 + sum1) % 255; 00031 } 00032 return (sum2 << 8) | sum1; 00033 } 00034 00035 void get_checksums(uint16_t check_sums[], const string key){ 00036 const string k = key+" "; 00037 check_sums[0] = 0x0000; 00038 check_sums[1] = 0x0000; 00039 check_sums[2] = 0x0000; 00040 size_t begin_key = 0; 00041 unsigned int counter = 0; 00042 while( begin_key < key.size()-1 ){ 00043 const size_t end_key = k.find_first_of(" .", begin_key); 00044 const string key_node = k.substr(begin_key, end_key - begin_key); 00045 check_sums[counter] = get_checksum(key_node); 00046 begin_key = end_key + 1; 00047 counter++; 00048 } 00049 } 00050 00051 bool is_alpha(int c) 00052 { 00053 if ((c >= 'a') && (c <= 'z')) return true; 00054 if ((c >= 'A') && (c <= 'Z')) return true; 00055 if ((c == '_')) return true; 00056 return false; 00057 } 00058 00059 bool is_digit(int c) 00060 { 00061 if ((c >= '0') && (c <= '9')) return true; 00062 return false; 00063 } 00064 00065 bool is_numeric(int c) 00066 { 00067 if (is_digit(c)) return true; 00068 if ((c == '.') || (c == '-')) return true; 00069 if ((c == 'e')) return true; 00070 return false; 00071 } 00072 00073 bool is_alphanum(int c) 00074 { 00075 return is_alpha(c) || is_numeric(c); 00076 } 00077 00078 bool is_whitespace(int c) 00079 { 00080 if ((c == ' ') || (c == '\t')) return true; 00081 return false; 00082 } 00083 00084 // Convert to lowercase 00085 string lc(string str){ 00086 for (unsigned int i=0; i<strlen(str.c_str()); i++) 00087 if (str[i] >= 0x41 && str[i] <= 0x5A) 00088 str[i] = str[i] + 0x20; 00089 return str; 00090 } 00091 00092 // Remove non-number characters 00093 string remove_non_number( string str ){ 00094 string number_mask = "0123456789-.abcdefpxABCDEFPX"; 00095 size_t found=str.find_first_not_of(number_mask); 00096 while (found!=string::npos){ 00097 //str[found]='*'; 00098 str.replace(found,1,""); 00099 found=str.find_first_not_of(number_mask); 00100 } 00101 return str; 00102 } 00103 00104 // Get the first parameter, and remove it from the original string 00105 string shift_parameter( string ¶meters ){ 00106 size_t beginning = parameters.find_first_of(" "); 00107 if( beginning == string::npos ){ string temp = parameters; parameters = ""; return temp; } 00108 string temp = parameters.substr( 0, beginning ); 00109 parameters = parameters.substr(beginning+1, parameters.size()); 00110 return temp; 00111 } 00112 00113 // Separate command from arguments 00114 string get_arguments( string possible_command ){ 00115 size_t beginning = possible_command.find_first_of(" "); 00116 if( beginning == string::npos ){ return ""; } 00117 return possible_command.substr( beginning + 1, possible_command.size() - beginning + 1); 00118 } 00119 00120 // Returns true if the file exists 00121 bool file_exists( const string file_name ){ 00122 bool exists = false; 00123 FILE *lp = fopen(file_name.c_str(), "r"); 00124 if(lp){ exists = true; } 00125 fclose(lp); 00126 return exists; 00127 } 00128 00129 // Prepares and executes a watchdog reset for dfu or reboot 00130 void system_reset( bool dfu ){ 00131 if(dfu) { 00132 LPC_WDT->WDCLKSEL = 0x1; // Set CLK src to PCLK 00133 uint32_t clk = SystemCoreClock / 16; // WD has a fixed /4 prescaler, PCLK default is /4 00134 LPC_WDT->WDTC = 1 * (float)clk; // Reset in 1 second 00135 LPC_WDT->WDMOD = 0x3; // Enabled and Reset 00136 LPC_WDT->WDFEED = 0xAA; // Kick the dog! 00137 LPC_WDT->WDFEED = 0x55; 00138 }else{ 00139 NVIC_SystemReset(); 00140 } 00141 } 00142 00143 // Convert a path indication ( absolute or relative ) into a path ( absolute ) 00144 string absolute_from_relative( string path ) 00145 { 00146 string cwd = THEKERNEL->current_path; 00147 00148 if ( path.empty() ) { 00149 return THEKERNEL->current_path; 00150 } 00151 00152 if ( path[0] == '/' ) { 00153 return path; 00154 } 00155 00156 string match = "../" ; 00157 while ( path.substr(0,3) == match ) { 00158 path = path.substr(3); 00159 unsigned found = cwd.find_last_of("/"); 00160 cwd = cwd.substr(0,found); 00161 } 00162 00163 match = ".." ; 00164 if ( path.substr(0,2) == match ) { 00165 path = path.substr(2); 00166 unsigned found = cwd.find_last_of("/"); 00167 cwd = cwd.substr(0,found); 00168 } 00169 00170 if ( cwd[cwd.length() - 1] == '/' ) { 00171 return cwd + path; 00172 } 00173 00174 return cwd + '/' + path; 00175 }
Generated on Tue Jul 12 2022 20:09:03 by
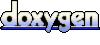