Fork of Smoothie to port to mbed non-LPC targets.
Fork of Smoothie by
Player.cpp
00001 /* 00002 This file is part of Smoothie (http://smoothieware.org/). The motion control part is heavily based on Grbl (https://github.com/simen/grbl) with additions from Sungeun K. Jeon (https://github.com/chamnit/grbl) 00003 Smoothie is free software: you can redistribute it and/or modify it under the terms of the GNU General Public License as published by the Free Software Foundation, either version 3 of the License, or (at your option) any later version. 00004 Smoothie is distributed in the hope that it will be useful, but WITHOUT ANY WARRANTY; without even the implied warranty of MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the GNU General Public License for more details. 00005 You should have received a copy of the GNU General Public License along with Smoothie. If not, see <http://www.gnu.org/licenses/>. 00006 */ 00007 00008 using namespace std; 00009 #include <vector> 00010 #include "libs/nuts_bolts.h" 00011 #include "libs/RingBuffer.h" 00012 #include "../communication/utils/Gcode.h" 00013 #include "libs/Module.h" 00014 #include "libs/Kernel.h" 00015 #include "Timer.h" // mbed.h lib 00016 #include "wait_api.h" // mbed.h lib 00017 #include "Block.h" 00018 #include "Player.h" 00019 #include "Planner.h" 00020 00021 Player::Player(){ 00022 this->current_block = NULL; 00023 this->looking_for_new_block = false; 00024 } 00025 00026 // Append a block to the list 00027 Block* Player::new_block(){ 00028 00029 // Clean up the vector of commands in the block we are about to replace 00030 // It is quite strange to do this here, we really should do it inside Block->pop_and_execute_gcode 00031 // but that function is called inside an interrupt and thus can break everything if the interrupt was trigerred during a memory access 00032 //Block* block = this->queue.get_ref( this->queue.size()-1 ); 00033 Block* block = this->queue.get_ref( this->queue.size() ); 00034 if( block->player == this ){ 00035 for(short index=0; index<block->gcodes.size(); index++){ 00036 block->gcodes.pop_back(); 00037 } 00038 } 00039 00040 // Create a new virgin Block in the queue 00041 this->queue.push_back(Block()); 00042 block = this->queue.get_ref( this->queue.size()-1 ); 00043 block->is_ready = false; 00044 block->initial_rate = -2; 00045 block->final_rate = -2; 00046 block->player = this; 00047 00048 return block; 00049 } 00050 00051 // Used by blocks to signal when they are ready to be used by the system 00052 void Player::new_block_added(){ 00053 if( this->current_block == NULL ){ 00054 this->pop_and_process_new_block(33); 00055 } 00056 } 00057 00058 // Process a new block in the queue 00059 void Player::pop_and_process_new_block(int debug){ 00060 if( this->looking_for_new_block ){ return; } 00061 this->looking_for_new_block = true; 00062 00063 if( this->current_block != NULL ){ this->looking_for_new_block = false; return; } 00064 00065 // Return if queue is empty 00066 if( this->queue.size() == 0 ){ 00067 this->current_block = NULL; 00068 // TODO : ON_QUEUE_EMPTY event 00069 this->looking_for_new_block = false; 00070 return; 00071 } 00072 00073 // Get a new block 00074 this->current_block = this->queue.get_ref(0); 00075 00076 // Tell all modules about it 00077 this->kernel->call_event(ON_BLOCK_BEGIN, this->current_block); 00078 00079 // In case the module was not taken 00080 if( this->current_block->times_taken < 1 ){ 00081 this->looking_for_new_block = false; 00082 this->current_block->release(); 00083 } 00084 00085 this->looking_for_new_block = false; 00086 00087 } 00088 00089 void Player::wait_for_queue(int free_blocks){ 00090 mbed::Timer t; 00091 while( this->queue.size() >= this->queue.capacity()-free_blocks ){ 00092 t.reset(); 00093 t.start(); 00094 this->kernel->call_event(ON_IDLE); 00095 t.stop(); 00096 if(t.read_us() < 500) 00097 wait_us(500 - t.read_us()); 00098 } 00099 }
Generated on Tue Jul 12 2022 20:09:02 by
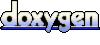