Fork of Smoothie to port to mbed non-LPC targets.
Fork of Smoothie by
descriptor_cdc.h
00001 #ifndef _DESCRIPTOR_CDC_H 00002 #define _DESCRIPTOR_CDC_H 00003 00004 #define DT_CDC_DESCRIPTOR 0x24 00005 #define DT_CDC_ENDPOINT 0x25 00006 00007 #define USB_CDC_SUBCLASS_DLC 0x01 00008 #define USB_CDC_SUBCLASS_ACM 0x02 00009 #define USB_CDC_SUBCLASS_TCM 0x03 00010 #define USB_CDC_SUBCLASS_MCCM 0x04 00011 #define USB_CDC_SUBCLASS_CAPI 0x05 00012 #define USB_CDC_SUBCLASS_ETHERNET 0x06 00013 #define USB_CDC_SUBCLASS_ATM 0x07 00014 #define USB_CDC_SUBCLASS_WHCM 0x08 00015 #define USB_CDC_SUBCLASS_DMM 0x09 00016 #define USB_CDC_SUBCLASS_MDLM 0x0a 00017 #define USB_CDC_SUBCLASS_OBEX 0x0b 00018 #define USB_CDC_SUBCLASS_EEM 0x0c 00019 #define USB_CDC_SUBCLASS_NCM 0x0d 00020 00021 #define USB_CDC_SUBTYPE_HEADER 0x00 00022 #define USB_CDC_SUBTYPE_CALL_MANAGEMENT 0x01 00023 #define USB_CDC_SUBTYPE_ACM 0x02 00024 #define USB_CDC_SUBTYPE_DLM 0x03 /* direct line management */ 00025 #define USB_CDC_SUBTYPE_TRF 0x04 /* telephone ringer */ 00026 #define USB_CDC_SUBTYPE_TCLSRCF 0x05 /* telephone call and line state reporting capabilities */ 00027 #define USB_CDC_SUBTYPE_UNION 0x06 00028 #define USB_CDC_SUBTYPE_COUNTRY 0x07 00029 #define USB_CDC_SUBTYPE_TOMF 0x08 /* telephone operational modes */ 00030 #define USB_CDC_SUBTYPE_USBTF 0x09 /* USB terminal */ 00031 #define USB_CDC_SUBTYPE_NETWORK_TERMINAL 0x0A 00032 #define USB_CDC_SUBTYPE_PUF 0x0B /* protocol unit */ 00033 #define USB_CDC_SUBTYPE_EUF 0x0C /* extension unit */ 00034 #define USB_CDC_SUBTYPE_MCMF 0x0D /* multi-channel management */ 00035 #define USB_CDC_SUBTYPE_CAPI_CM 0x0E /* CAPI control management */ 00036 #define USB_CDC_SUBTYPE_ETHERNET 0x0F 00037 #define USB_CDC_SUBTYPE_ATM_NETWORK 0x10 00038 #define USB_CDC_SUBTYPE_WHCM 0x11 /* wireless handset control model */ 00039 #define USB_CDC_SUBTYPE_MDLM 0x12 /* mobile direct line model */ 00040 #define USB_CDC_SUBTYPE_MDLM_DETAIL 0x13 /* MDLM detail */ 00041 #define USB_CDC_SUBTYPE_DMM 0x14 /* device management model */ 00042 #define USB_CDC_SUBTYPE_OBEX 0x15 00043 #define USB_CDC_SUBTYPE_CSF 0x16 /* command set */ 00044 #define USB_CDC_SUBTYPE_CSDF 0x17 /* command set detail */ 00045 #define USB_CDC_SUBTYPE_TCMF 0x18 /* telephone control model */ 00046 #define USB_CDC_SUBTYPE_OBEX_SI 0x19 /* OBEX service identifier */ 00047 #define USB_CDC_SUBTYPE_NCM 0x1A 00048 00049 #define USB_CDC_PROTOCOL_NONE 0x00 00050 #define USB_CDC_PROTOCOL_ITU_V250 0x01 00051 #define USB_CDC_PROTOCOL_PCCA_101 0x02 00052 #define USB_CDC_PROTOCOL_PCCA_101_O 0x03 00053 #define USB_CDC_PROTOCOL_GSM_7_07 0x04 00054 #define USB_CDC_PROTOCOL_3GPP_27_07 0x05 00055 #define USB_CDC_PROTOCOL_C_S0017_0 0x06 00056 #define USB_CDC_PROTOCOL_EEM 0x07 00057 #define USB_CDC_PROTOCOL_SEE_DESCRIPTOR 0xFE 00058 #define USB_CDC_PROTOCOL_VENDOR 0xFF 00059 00060 #define CDC_SET_LINE_CODING 0x20 00061 #define CDC_GET_LINE_CODING 0x21 00062 #define CDC_SET_CONTROL_LINE_STATE 0x22 00063 #define CDC_SEND_BREAK 0x23 00064 00065 // control line states 00066 #define CDC_CLS_DTR 0x01 00067 #define CDC_CLS_RTS 0x02 00068 00069 typedef struct __attribute__ ((packed)) { 00070 uint8_t bLength; // 5 00071 uint8_t bDescType; // DT_CDC_DESCRIPTOR (0x24) 00072 uint8_t bDescSubType; // USB_CDC_SUBTYPE_HEADER (0x00) 00073 uint16_t bcdCDC; 00074 } usbcdc_header; 00075 #define USB_CDC_LENGTH_HEADER sizeof(usbcdc_header) 00076 00077 typedef struct __attribute__ ((packed)) { 00078 uint8_t bLength; // 5 00079 uint8_t bDescType; // DT_CDC_DESCRIPTOR (0x24) 00080 uint8_t bDescSubType; // USB_CDC_SUBTYPE_CALL_MANAGEMENT (0x01) 00081 00082 uint8_t bmCapabilities; 00083 #define USB_CDC_CALLMGMT_CAP_CALLMGMT 0x01 00084 #define USB_CDC_CALLMGMT_CAP_DATAINTF 0x02 00085 00086 uint8_t bDataInterface; 00087 } usbcdc_callmgmt; 00088 #define USB_CDC_LENGTH_CALLMGMT sizeof(usbcdc_callmgmt) 00089 00090 typedef struct __attribute__ ((packed)) { 00091 uint8_t bLength; // 4 00092 uint8_t bDescType; // DT_CDC_DESCRIPTOR (0x24) 00093 uint8_t bDescSubType; // USB_CDC_SUBTYPE_ACM (0x02) 00094 00095 uint8_t bmCapabilities; 00096 #define USB_CDC_ACM_CAP_COMM 0x01 00097 #define USB_CDC_ACM_CAP_LINE 0x02 00098 #define USB_CDC_ACM_CAP_BRK 0x04 00099 #define USB_CDC_ACM_CAP_NOTIFY 0x08 00100 } usbcdc_acm; 00101 #define USB_CDC_LENGTH_ACM sizeof(usbcdc_acm) 00102 00103 typedef struct __attribute__ ((packed)) { 00104 uint8_t bLength; // 5+ 00105 uint8_t bDescType; // DT_CDC_DESCRIPTOR (0x24) 00106 uint8_t bDescSubType; // USB_CDC_SUBTYPE_UNION (0x06) 00107 00108 uint8_t bMasterInterface; 00109 uint8_t bSlaveInterface0; 00110 } usbcdc_union; 00111 #define USB_CDC_LENGTH_UNION sizeof(usbcdc_union) 00112 00113 typedef struct __attribute__ ((packed)) { 00114 uint8_t bLength; // 13 00115 uint8_t bDescType; // DT_CDC_DESCRIPTOR (0x24) 00116 uint8_t bDescSubType; // USB_CDC_SUBTYPE_ETHERNET (0x0F) 00117 00118 uint8_t iMacAddress; // index of MAC address string 00119 uint32_t bmEthernetStatistics; 00120 uint16_t wMaxSegmentSize; // 1514? 00121 uint16_t wNumberMCFilters; // 0 00122 uint8_t bNumberPowerFilters; // 0 00123 } usbcdc_ether; 00124 #define USB_CDC_LENGTH_ETHER sizeof(usbcdc_ether) 00125 00126 typedef struct __attribute__ ((packed)) { 00127 uint32_t dwDTERate; // data terminal rate, bits per second 00128 uint8_t bCharFormat; // 0: 1 stop bit, 1: 1.5 stop bits, 2: 2 stop bits 00129 uint8_t bParityType; // 0: none, 1: odd, 2: even, 3: mark, 4: space 00130 uint8_t bDataBits; // number of data bits (5, 6, 7, 8, 16) 00131 } usbcdc_line_coding; 00132 00133 #endif /* _DESCRIPTOR_CDC_H */
Generated on Tue Jul 12 2022 20:09:00 by
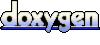