Fork of Smoothie to port to mbed non-LPC targets.
Fork of Smoothie by
descriptor.h
00001 #ifndef _DESCRIPTOR_H 00002 #define _DESCRIPTOR_H 00003 00004 #include <stdint.h> 00005 00006 #define DL_DEVICE 0x12 00007 #define DL_CONFIGURATION 0x09 00008 #define DL_INTERFACE 0x09 00009 #define DL_ENDPOINT 0x07 00010 #define DL_LANGUAGE 0x04 00011 #define DL_INTERFACE_ASSOCIATION 0x08 00012 00013 #define mA /2 00014 00015 #define DT_DEVICE 0x01 00016 #define DT_CONFIGURATION 0x02 00017 #define DT_STRING 0x03 00018 #define DT_LANGUAGE 0x03 00019 #define DT_INTERFACE 0x04 00020 #define DT_ENDPOINT 0x05 00021 #define DT_DEVICE_QUALIFIER 0x06 00022 #define DT_OTHER_SPEED 0x07 00023 #define DT_INTERFACE_POWER 0x08 00024 #define DT_OTG 0x09 00025 #define DT_DEBUG 0x0A 00026 #define DT_INTERFACE_ASSOCIATION 0x0B 00027 00028 #define USB_VERSION_1_0 0x0100 00029 #define USB_VERSION_1_1 0x0110 00030 #define USB_VERSION_2_0 0x0200 00031 #define USB_VERSION_3_0 0x0300 00032 00033 #define UC_PER_INTERFACE 0x00 00034 #define UC_AUDIO 0x01 00035 #define UC_COMM 0x02 00036 #define UC_HID 0x03 00037 #define UC_PHYSICAL 0x05 00038 #define UC_STILL_IMAGE 0x06 00039 #define UC_PRINTER 0x07 00040 #define UC_MASS_STORAGE 0x08 00041 #define UC_HUB 0x09 00042 #define UC_CDC_DATA 0x0A 00043 #define UC_CSCID 0x0B 00044 #define UC_CONTENT_SEC 0x0D 00045 #define UC_VIDEO 0x0E 00046 #define UC_WIRELESS_CONTROLLER 0xE0 00047 #define UC_MISC 0xEF 00048 #define UC_APP_SPEC 0xFE 00049 #define UC_VENDOR_SPEC 0xFF 00050 00051 #define SUBCLASS_IAD 0x02 00052 #define PROTOCOL_IAD 0x01 00053 00054 #define CA_BUSPOWERED 0x80 00055 #define CA_SELFPOWERED 0x40 00056 #define CA_REMOTEWAKEUP 0x20 00057 00058 #define EP_DIR_MASK 0x80 00059 #define EP_DIR_OUT 0x00 00060 #define EP_DIR_IN 0x80 00061 00062 #define EA_CONTROL 0x00 00063 #define EA_ISOCHRONOUS 0x01 00064 #define EA_BULK 0x02 00065 #define EA_INTERRUPT 0x03 00066 00067 #define EA_ISO_NONE 0x00 00068 #define EA_ISO_ASYNC 0x04 00069 #define EA_ISO_ADAPTIVE 0x08 00070 #define EA_ISO_SYNC 0x0C 00071 00072 #define EA_ISO_TYPE_DATA 0x00 00073 #define EA_ISO_TYPE_FEEDBACK 0x10 00074 #define EA_ISO_TYPE_EXPLICIT 0x20 00075 00076 #define SL_USENGLISH 0x0409 00077 #define SL_AUENGLISH 0x0C09 00078 #define SL_GERMAN 0x0407 00079 00080 #include "USBEndpoints.h" 00081 00082 typedef struct __attribute__ ((packed)) 00083 { 00084 uint8_t bLength; // descriptor length 00085 uint8_t bDescType; // descriptor type: see DT_* defines 00086 } 00087 usbdesc_base; 00088 00089 typedef struct __attribute__ ((packed)) 00090 { 00091 uint8_t bLength; // Device descriptor length (0x12) 00092 uint8_t bDescType; // DT_DEVICE (0x01) 00093 uint16_t bcdUSB; // USB Specification Number which device complies to - see USB_VERSION_* defines 00094 uint8_t bDeviceClass; // USB Device Class - see UC_* defines 00095 uint8_t bDeviceSubClass; // Subclass Code 00096 uint8_t bDeviceProtocol; // Protocol Code 00097 uint8_t bMaxPacketSize; // Maximum Packet Size for Zero Endpoint. Valid Sizes are 8, 16, 32, 64 00098 uint16_t idVendor; // Vendor ID 00099 uint16_t idProduct; // Product ID 00100 uint16_t bcdDevice; // Device Release Number 00101 uint8_t iManufacturer; // Index of Manufacturer String Descriptor 00102 uint8_t iProduct; // Index of Product String Descriptor 00103 uint8_t iSerialNumber; // Index of Serial Number String Descriptor 00104 uint8_t bNumConfigurations; // Number of Possible Configurations 00105 } 00106 usbdesc_device; 00107 00108 typedef struct __attribute__ ((packed)) 00109 { 00110 uint8_t bLength; // Configuration Descriptor Length (0x09) 00111 uint8_t bDescType; // DT_CONFIGURATION (0x02) 00112 uint16_t wTotalLength; // Total length in bytes of this descriptor plus all this configuration's interfaces plus their endpoints, see http://www.beyondlogic.org/usbnutshell/confsize.gif 00113 uint8_t bNumInterfaces; // Number of Interfaces 00114 uint8_t bConfigurationValue; // Value that host uses to select this configuration 00115 uint8_t iConfiguration; // Index of String Descriptor describing this configuration 00116 uint8_t bmAttributes; // bitmap. see CA_* defines 00117 uint8_t bMaxPower; // Max. Current = bMaxPower * 2mA 00118 } 00119 usbdesc_configuration; 00120 00121 typedef struct __attribute__ ((packed)) 00122 { 00123 uint8_t bLength; // Interface Descriptor Length (0x09) 00124 uint8_t bDescType; // DT_INTERFACE (0x04) 00125 uint8_t bInterfaceNumber; // Number of Interface 00126 uint8_t bAlternateSetting; // Value used to select alternative setting 00127 00128 uint8_t bNumEndPoints; // Number of Endpoints used for this interface 00129 uint8_t bInterfaceClass; // Class Code - see Device_Class_Enum 00130 uint8_t bInterfaceSubClass; // Subclass Code 00131 uint8_t bInterfaceProtocol; // Protocol Code 00132 00133 uint8_t iInterface; // Index of String Descriptor Describing this interface 00134 uint8_t selectedAlternate; // the currently selected alternate for this group of interfaces. Set on alternate 0 for easy lookups 00135 uint8_t dummy1; // pad to 32 bit boundary 00136 uint8_t dummy2; // pad to 32 bit boundary 00137 00138 USB_Class_Receiver *classReceiver; // who do we call when we receive a setup packet for this interface? 00139 } 00140 usbdesc_interface; 00141 00142 typedef struct __attribute__ ((packed)) 00143 { 00144 uint8_t bLength; // Endpoint Descriptor Length (0x07) 00145 uint8_t bDescType; // DT_ENDPOINT (0x05) 00146 uint8_t bEndpointAddress; // 0x00-0x0F = OUT endpoints, 0x80-0x8F = IN endpoints 00147 uint8_t bmAttributes; // bitmap, see Endpoint_Attributes_Enum 00148 00149 uint16_t wMaxPacketSize; // Maximum Packet Size this endpoint is capable of sending or receiving 00150 uint8_t bInterval; // Interval for polling endpoint data transfers. Value in frame counts. Ignored for Bulk & Control Endpoints. Isochronous must equal 1 and field may range from 1 to 255 for interrupt endpoints. 00151 uint8_t dummy; // pad to 32 bit boundary 00152 00153 USB_Endpoint_Receiver *epReceiver; // Who do we call when something happens on this endpoint? 00154 } 00155 usbdesc_endpoint; 00156 00157 typedef struct __attribute__ ((packed)) 00158 { 00159 uint8_t bLength; // String Descriptor Length (2 + 2*nLang) 00160 uint8_t bDescType; // DT_STRING (0x03) 00161 uint16_t wLangID[1]; // language code(s) 00162 } 00163 usbdesc_language; 00164 00165 typedef struct __attribute__ ((packed)) 00166 { 00167 uint8_t bLength; // 2 + strlen 00168 uint8_t bDescType; // DT_STRING (0x03) 00169 uint16_t str[]; // UNICODE string 00170 } 00171 usbdesc_string; 00172 00173 #define usbdesc_string_l(l) struct __attribute__ ((packed)) { uint8_t bLength; uint8_t bDescType; char16_t str[l]; } 00174 00175 #define usbstring(string) { sizeof(u ## string), DT_STRING, { u ## string } } 00176 00177 #define usbstring_init(name, string) struct __attribute__ ((packed)) { const uint8_t bLength; const uint8_t bDescType; char16_t str[sizeof(string)]; } name = { sizeof(u ## string), DT_STRING, { u ## string } } 00178 00179 #define usbstring_const_init(name, string) const struct __attribute__ ((packed)) { const uint8_t bLength; const uint8_t bDescType; const char16_t str[sizeof(string)]; } name = { sizeof(u ## string), DT_STRING, { u ## string } } 00180 00181 typedef struct __attribute__ ((packed)) 00182 { 00183 uint8_t bLength; 00184 uint8_t bDescType; 00185 uint8_t bFirstInterface; // interface index of the first interface for this function 00186 uint8_t bInterfaceCount; // number of contiguous interfaces used with this function 00187 uint8_t bFunctionClass; // Class code - see Device descriptor 00188 uint8_t bFunctionSubClass; // Subclass code 00189 uint8_t bFunctionProtocol; // Protocol code 00190 uint8_t iFunction; // function name string 00191 } 00192 usbdesc_iad; 00193 00194 #endif /* _DESCRIPTOR_H */
Generated on Tue Jul 12 2022 20:09:00 by
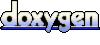