Fork of Smoothie to port to mbed non-LPC targets.
Fork of Smoothie by
USBEndpoints.h
00001 /* Copyright (c) 2010-2011 mbed.org, MIT License 00002 * 00003 * Permission is hereby granted, free of charge, to any person obtaining a copy of this software 00004 * and associated documentation files (the "Software"), to deal in the Software without 00005 * restriction, including without limitation the rights to use, copy, modify, merge, publish, 00006 * distribute, sublicense, and/or sell copies of the Software, and to permit persons to whom the 00007 * Software is furnished to do so, subject to the following conditions: 00008 * 00009 * The above copyright notice and this permission notice shall be included in all copies or 00010 * substantial portions of the Software. 00011 * 00012 * THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR IMPLIED, INCLUDING 00013 * BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY, FITNESS FOR A PARTICULAR PURPOSE AND 00014 * NONINFRINGEMENT. IN NO EVENT SHALL THE AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, 00015 * DAMAGES OR OTHER LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM, 00016 * OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE SOFTWARE. 00017 */ 00018 00019 #ifndef USBENDPOINTS_H 00020 #define USBENDPOINTS_H 00021 00022 #include "USBDevice_Types.h" 00023 00024 /* SETUP packet size */ 00025 #define SETUP_PACKET_SIZE (8) 00026 00027 /* Options flags for configuring endpoints */ 00028 #define DEFAULT_OPTIONS (0) 00029 #define SINGLE_BUFFERED (1U << 0) 00030 #define ISOCHRONOUS (1U << 1) 00031 #define RATE_FEEDBACK_MODE (1U << 2) /* Interrupt endpoints only */ 00032 00033 /* Endpoint transfer status, for endpoints > 0 */ 00034 typedef enum { 00035 EP_COMPLETED, /* Transfer completed */ 00036 EP_PENDING, /* Transfer in progress */ 00037 EP_INVALID, /* Invalid parameter */ 00038 EP_STALLED, /* Endpoint stalled */ 00039 } EP_STATUS; 00040 00041 class USB_State_Receiver { 00042 public: 00043 virtual bool USBEvent_busReset(void) = 0; 00044 virtual bool USBEvent_connectStateChanged(bool connected) = 0; 00045 virtual bool USBEvent_suspendStateChanged(bool suspended) = 0; 00046 }; 00047 00048 class USB_Frame_Receiver { 00049 public: 00050 virtual bool USBEvent_Frame(uint16_t) = 0; 00051 }; 00052 00053 class USB_Class_Receiver { 00054 public: 00055 virtual bool USBEvent_Request(CONTROL_TRANSFER&) = 0; 00056 virtual bool USBEvent_RequestComplete(CONTROL_TRANSFER&, uint8_t *buf, uint32_t length) = 0; 00057 }; 00058 00059 class USB_Endpoint_Receiver : public USB_Class_Receiver { 00060 public: 00061 virtual bool USBEvent_EPIn(uint8_t, uint8_t) = 0; 00062 virtual bool USBEvent_EPOut(uint8_t, uint8_t) = 0; 00063 }; 00064 00065 /* Include configuration for specific target */ 00066 #if defined(TARGET_LPC1768) || defined(TARGET_LPC2368) || defined(__LPC17XX__) 00067 #include "USBEndpoints_LPC17_LPC23.h" 00068 #elif defined(TARGET_LPC11U24) 00069 #include "USBEndpoints_LPC11U.h" 00070 #else 00071 #error "Unknown target type" 00072 #endif 00073 00074 #endif
Generated on Tue Jul 12 2022 20:09:03 by
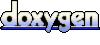