Fork of Smoothie to port to mbed non-LPC targets.
Fork of Smoothie by
StreamOutput.h
00001 /* 00002 This file is part of Smoothie (http://smoothieware.org/). The motion control part is heavily based on Grbl (https://github.com/simen/grbl). 00003 Smoothie is free software: you can redistribute it and/or modify it under the terms of the GNU General Public License as published by the Free Software Foundation, either version 3 of the License, or (at your option) any later version. 00004 Smoothie is distributed in the hope that it will be useful, but WITHOUT ANY WARRANTY; without even the implied warranty of MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the GNU General Public License for more details. 00005 You should have received a copy of the GNU General Public License along with Smoothie. If not, see <http://www.gnu.org/licenses/>. 00006 */ 00007 00008 #ifndef STREAMOUTPUT_H 00009 #define STREAMOUTPUT_H 00010 00011 #include <stdarg.h> 00012 #include <string.h> 00013 #include <stdio.h> 00014 00015 // This is a base class for all StreamOutput objects. 00016 // StreamOutputs are basically "things you can sent strings to". They are passed along with gcodes for example so modules can answer to those gcodes. 00017 // They are usually associated with a command source, but can also be a NullStreamOutput if we just want to ignore whatever is sent 00018 00019 class NullStreamOutput; 00020 00021 class StreamOutput { 00022 public: 00023 StreamOutput(){} 00024 virtual ~StreamOutput(){} 00025 00026 virtual int printf(const char* format, ...) __attribute__ ((format(printf, 2, 3))) { 00027 char b[64]; 00028 char *buffer; 00029 // Make the message 00030 va_list args; 00031 va_start(args, format); 00032 00033 int size = vsnprintf(b, 64, format, args) 00034 + 1; // we add one to take into account space for the terminating \0 00035 00036 if (size < 64) 00037 buffer = b; 00038 else 00039 { 00040 buffer = new char[size]; 00041 vsnprintf(buffer, size, format, args); 00042 } 00043 va_end(args); 00044 00045 puts(buffer); 00046 00047 if (buffer != b) 00048 delete[] buffer; 00049 00050 return size - 1; 00051 } 00052 virtual int _putc(int c) { return 1; } 00053 virtual int _getc(void) { return 0; } 00054 virtual int puts(const char* str) = 0; 00055 00056 static NullStreamOutput NullStream; 00057 }; 00058 00059 class NullStreamOutput : public StreamOutput { 00060 public: 00061 int puts(const char* str) { return strlen(str); } 00062 }; 00063 00064 #endif
Generated on Tue Jul 12 2022 20:09:02 by
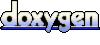