Fork of Smoothie to port to mbed non-LPC targets.
Fork of Smoothie by
Spindle.cpp
00001 /* 00002 This file is part of Smoothie (http://smoothieware.org/). The motion control part is heavily based on Grbl (https://github.com/simen/grbl). 00003 Smoothie is free software: you can redistribute it and/or modify it under the terms of the GNU General Public License as published by the Free Software Foundation, either version 3 of the License, or (at your option) any later version. 00004 Smoothie is distributed in the hope that it will be useful, but WITHOUT ANY WARRANTY; without even the implied warranty of MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the GNU General Public License for more details. 00005 You should have received a copy of the GNU General Public License along with Smoothie. If not, see <http://www.gnu.org/licenses/>. 00006 */ 00007 00008 #include "libs/Module.h" 00009 #include "libs/Kernel.h" 00010 #include "modules/communication/utils/Gcode.h" 00011 #include "modules/robot/Stepper.h" 00012 #include "Spindle.h" 00013 #include "libs/nuts_bolts.h" 00014 00015 Spindle::Spindle(){ 00016 00017 } 00018 00019 void Spindle::on_module_loaded() { 00020 if( !this->kernel->config->value( spindle_module_enable_checksum )->by_default(false)->as_bool() ){ return; } 00021 00022 // Settings 00023 this->on_config_reload(this); 00024 00025 this->register_for_event(ON_GCODE_EXECUTE); 00026 this->register_for_event(ON_PLAY); 00027 this->register_for_event(ON_PAUSE); 00028 this->register_for_event(ON_BLOCK_BEGIN); 00029 this->register_for_event(ON_BLOCK_END); 00030 } 00031 00032 00033 // Get config 00034 void Spindle::on_config_reload(void* argument){ 00035 this->spindle_pin= this->kernel->config->value(spindle_pin_checksum)->by_default("0.4" )->as_pin()->as_output(); 00036 } 00037 00038 // Turn spindle off spindle at the end of a move 00039 void Spindle::on_block_end(void* argument){ 00040 //this->spindle_pin->set(0); 00041 } 00042 00043 // Set spindle power at the beginning of a block 00044 void Spindle::on_block_begin(void* argument){ 00045 //this->set_spindle(); 00046 } 00047 00048 // When the play/pause button is set to pause, or a module calls the ON_PAUSE event 00049 void Spindle::on_pause(void* argument){ 00050 this->spindle_pin->set(0); 00051 } 00052 00053 // When the play/pause button is set to play, or a module calls the ON_PLAY event 00054 void Spindle::on_play(void* argument){ 00055 this->set_spindle(); 00056 } 00057 00058 // Turn spindle on/off depending on received GCodes 00059 void Spindle::on_gcode_execute(void* argument){ 00060 Gcode* gcode = static_cast<Gcode*>(argument); 00061 this->spindle_on = false; 00062 if( gcode->has_letter('G' )){ 00063 int code = gcode->get_value('G'); 00064 if( code == 0 ){ // G0 00065 this->kernel->serial->printf("off\r\n"); 00066 this->spindle_pin->set(0); 00067 this->spindle_on = false; 00068 }else if( code >= 1 && code <= 3 ){ // G1, G2, G3 00069 this->kernel->serial->printf("on\r\n"); 00070 this->spindle_pin->set(1); 00071 this->spindle_on = true; 00072 } 00073 } 00074 } 00075 00076 00077 void Spindle::set_spindle(){ 00078 if( this->spindle_on && this->kernel->stepper->current_block ){ 00079 this->spindle_pin->set(1); 00080 } 00081 }
Generated on Tue Jul 12 2022 20:09:02 by
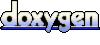