Fork of Smoothie to port to mbed non-LPC targets.
Fork of Smoothie by
RrdGlcd.h
00001 #ifndef __RRDGLCD_H 00002 #define __RRDGLCD_H 00003 00004 /* 00005 This file is part of Smoothie (http://smoothieware.org/). The motion control part is heavily based on Grbl (https://github.com/simen/grbl). 00006 Smoothie is free software: you can redistribute it and/or modify it under the terms of the GNU General Public License as published by the Free Software Foundation, either version 3 of the License, or (at your option) any later version. 00007 Smoothie is distributed in the hope that it will be useful, but WITHOUT ANY WARRANTY; without even the implied warranty of MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the GNU General Public License for more details. 00008 You should have received a copy of the GNU General Public License along with Smoothie. If not, see <http://www.gnu.org/licenses/>. 00009 */ 00010 00011 /** 00012 * Based loosely on st7920.h from http://mbed.org/users/Bas/code/ST7920 and parts of the Arduino U8glib library. 00013 * Written by Jim Morris 00014 */ 00015 00016 #include <mbed.h> 00017 #include "libs/Kernel.h" 00018 #include "libs/utils.h" 00019 #include <libs/Pin.h> 00020 00021 00022 class RrdGlcd { 00023 public: 00024 /** 00025 *@brief Constructor, initializes the lcd on the respective pins. 00026 *@param mosi mbed pinname for mosi 00027 *@param sclk mbed name for sclk 00028 *@param cd Smoothie Pin for cs 00029 *@return none 00030 */ 00031 RrdGlcd (PinName mosi, PinName sclk, Pin cs); 00032 00033 virtual ~RrdGlcd(); 00034 00035 void setFrequency(int f); 00036 00037 void initDisplay(void); 00038 void clearScreen(void); 00039 void displayString(int row, int column, const char *ptr, int length); 00040 void refresh(); 00041 00042 /** 00043 *@brief Fills the screen with the graphics described in a 1024-byte array 00044 *@ 00045 *@param bitmap 128x64, bytes horizontal 00046 *@return none 00047 * 00048 */ 00049 void fillGDRAM(const uint8_t *bitmap); 00050 00051 // copy the bits in g, of X line size pixels, to x, y in frame buffer 00052 void renderGlyph(int x, int y, const uint8_t *g, int pixelWidth, int pixelHeight); 00053 00054 private: 00055 Pin cs; 00056 mbed::SPI* spi; 00057 void renderChar(uint8_t *fb, char c, int ox, int oy); 00058 void displayChar(int row, int column,char inpChr); 00059 00060 uint8_t *fb; 00061 bool inited; 00062 bool dirty; 00063 }; 00064 #endif 00065
Generated on Tue Jul 12 2022 20:09:02 by
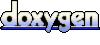