Fork of Smoothie to port to mbed non-LPC targets.
Fork of Smoothie by
Robot.h
00001 /* 00002 This file is part of Smoothie (http://smoothieware.org/). The motion control part is heavily based on Grbl (https://github.com/simen/grbl). 00003 Smoothie is free software: you can redistribute it and/or modify it under the terms of the GNU General Public License as published by the Free Software Foundation, either version 3 of the License, or (at your option) any later version. 00004 Smoothie is distributed in the hope that it will be useful, but WITHOUT ANY WARRANTY; without even the implied warranty of MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the GNU General Public License for more details. 00005 You should have received a copy of the GNU General Public License along with Smoothie. If not, see <http://www.gnu.org/licenses/>. 00006 */ 00007 00008 #ifndef ROBOT_H 00009 #define ROBOT_H 00010 00011 #include <string> 00012 using std::string; 00013 #include "libs/Module.h" 00014 #include "RobotPublicAccess.h" 00015 00016 #define NEXT_ACTION_DEFAULT 0 00017 #define NEXT_ACTION_DWELL 1 00018 #define NEXT_ACTION_GO_HOME 2 00019 00020 #define MOTION_MODE_SEEK 0 // G0 00021 #define MOTION_MODE_LINEAR 1 // G1 00022 #define MOTION_MODE_CW_ARC 2 // G2 00023 #define MOTION_MODE_CCW_ARC 3 // G3 00024 #define MOTION_MODE_CANCEL 4 // G80 00025 00026 #define PATH_CONTROL_MODE_EXACT_PATH 0 00027 #define PATH_CONTROL_MODE_EXACT_STOP 1 00028 #define PATH_CONTROL_MODE_CONTINOUS 2 00029 00030 #define PROGRAM_FLOW_RUNNING 0 00031 #define PROGRAM_FLOW_PAUSED 1 00032 #define PROGRAM_FLOW_COMPLETED 2 00033 00034 #define SPINDLE_DIRECTION_CW 0 00035 #define SPINDLE_DIRECTION_CCW 1 00036 00037 class Gcode; 00038 class BaseSolution; 00039 class StepperMotor; 00040 00041 class Robot : public Module { 00042 public: 00043 Robot(); 00044 void on_module_loaded(); 00045 void on_config_reload(void* argument); 00046 void on_gcode_received(void* argument); 00047 void on_get_public_data(void* argument); 00048 void on_set_public_data(void* argument); 00049 00050 void reset_axis_position(float position, int axis); 00051 void get_axis_position(float position[]); 00052 float to_millimeters(float value); 00053 float from_millimeters(float value); 00054 00055 BaseSolution* arm_solution; // Selected Arm solution ( millimeters to step calculation ) 00056 bool absolute_mode; // true for absolute mode ( default ), false for relative mode 00057 00058 private: 00059 void distance_in_gcode_is_known(Gcode* gcode); 00060 void append_milestone( float target[], float rate_mm_s); 00061 void append_line( Gcode* gcode, float target[], float rate_mm_s); 00062 //void append_arc(float theta_start, float angular_travel, float radius, float depth, float rate); 00063 void append_arc( Gcode* gcode, float target[], float offset[], float radius, bool is_clockwise ); 00064 00065 00066 void compute_arc(Gcode* gcode, float offset[], float target[]); 00067 00068 float theta(float x, float y); 00069 void select_plane(uint8_t axis_0, uint8_t axis_1, uint8_t axis_2); 00070 00071 float last_milestone[3]; // Last position, in millimeters 00072 bool inch_mode; // true for inch mode, false for millimeter mode ( default ) 00073 int8_t motion_mode; // Motion mode for the current received Gcode 00074 float seek_rate; // Current rate for seeking moves ( mm/s ) 00075 float feed_rate; // Current rate for feeding moves ( mm/s ) 00076 uint8_t plane_axis_0, plane_axis_1, plane_axis_2; // Current plane ( XY, XZ, YZ ) 00077 float mm_per_line_segment; // Setting : Used to split lines into segments 00078 float mm_per_arc_segment; // Setting : Used to split arcs into segmentrs 00079 float delta_segments_per_second; // Setting : Used to split lines into segments for delta based on speed 00080 00081 // Number of arc generation iterations by small angle approximation before exact arc trajectory 00082 // correction. This parameter maybe decreased if there are issues with the accuracy of the arc 00083 // generations. In general, the default value is more than enough for the intended CNC applications 00084 // of grbl, and should be on the order or greater than the size of the buffer to help with the 00085 // computational efficiency of generating arcs. 00086 int arc_correction; // Setting : how often to rectify arc computation 00087 float max_speeds[3]; // Setting : max allowable speed in mm/m for each axis 00088 00089 // Used by Stepper 00090 public: 00091 StepperMotor* alpha_stepper_motor; 00092 StepperMotor* beta_stepper_motor; 00093 StepperMotor* gamma_stepper_motor; 00094 00095 std::vector<StepperMotor*> actuators; 00096 00097 float seconds_per_minute; // for realtime speed change 00098 }; 00099 00100 // Convert from inches to millimeters ( our internal storage unit ) if needed 00101 inline float Robot::to_millimeters( float value ){ 00102 return this->inch_mode ? value * 25.4 : value; 00103 } 00104 inline float Robot::from_millimeters( float value){ 00105 return this->inch_mode ? value/25.4 : value; 00106 } 00107 inline void Robot::get_axis_position(float position[]){ 00108 memcpy(position, this->last_milestone, sizeof(float)*3 ); 00109 } 00110 00111 #endif
Generated on Tue Jul 12 2022 20:09:02 by
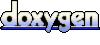